object name:物件名称
qwidget:enable 使能本部件
geometry:窗口位置和大小设置
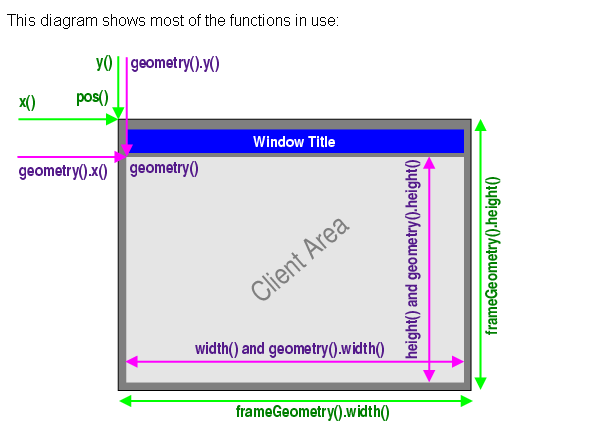
sizepolicy:
1. Fixed: 大小不能改变
2. Minimum: 已经是最小, 不能再被缩小, 但能放大.
3. Maximum: 已经是最大, 不能再被放大, 但能缩小.
4. Preferred: 控件的sizeHint()是他的sizeHint, 能被缩小, 放大.
5. Expanding: 控件可以自行增大或者缩小.
Preferred与Expanding的区别: 当有Preferred与Expanding的控件时, 只有Expanding的会被缩小与放大, Preferred的控件使用其sizeHint()作为大小, 大小不发生变化.
sizepolicy:
minisize:最小尺寸
maxsize:最大尺寸
sizeincrement:0*0
basesize:基本大小默认为0 与sizeincrement设置有关
palette:
font:字体
cursor:鼠标形状
mousetracking:
focuspolicy:获取焦点方式(tab键,单击,前两者,。。)
contextmenupolicy:
acceptdrops:支持drop事件
windowtitle:窗体标题
icon:窗体图标左上角的那个
windowopacity:
autofillbackground:自动填充背景色
stylesheet:样式
local:归属地
其他常用属性:
visible fullscreen focus clearfocus enabled
show() hide() raise() lower() close()
MOVEEVENT() RESIZEEVENT()
注意點:
1,编写程序时,初始化窗体时最好不要使用setGeometry()函数,而用resize()和move()代替,因为使用setGeometry()会导致窗体show()之后再错误的位置上停留很短暂的时间,带来闪烁现象!
Warning: Calling setGeometry() inside resizeEvent() or moveEvent() can lead to infinite recursion.
See the Window Geometry documentation for an overview of geometry issues with windows.
By default, this property contains a value that depends on the user's platform and screen geometry.
Access functions:
const QRect & | geometry () const |
void | setGeometry ( int x, int y, int w, int h ) |
void | setGeometry ( const QRect & ) |
See also frameGeometry(), rect(), move(), resize(), moveEvent(), resizeEvent(), minimumSize(), and maximumSize().
2,窗体位置初始化问题:
MainWindow::MainWindow(QWidget *parent) :
QMainWindow(parent), ui(new Ui::MainWindow)
{
ui->setupUi(this);
// this->geometry() is the same here...
setWindowState(Qt::WindowMaximized);
ui->webView->setGeometry(0, 60, geometry().width(), geometry().height()-60);
// ...as it is here.
}
As you may be able to tell, I'm trying to start the application with the window maximized and the QWebView also maximized. Basically, whenever the main window is resized, I also want to call ui->webView->setGeometry
with the update height and width. But MainWindow::geometry
doesn't seem to be updating. What am I doing wrong?
|
I would have to double check, but your geometry might not get updated properly until your main window gets a show event.
However, I would suggest you put your QWebView inside of a layout instead of trying to size it manually every time your main window changes size.
|
|
|
3,设置实例:
MyWidget::MyWidget(QWidget *parent): QWidget(parent)
{
setFixedSize(200, 120);
}
The QWidget::setGeometry() call sets both the widget's screen position and the size. It is equivalent to calling QWidget::move() followed by QWidget::resize().
4,层次问题
Qt中各个widget前后位置的设置
在Qt中,所有问题都要一分为二,讨论两种不同的情况:一个是最常用的QWidget体系,而另外一个则是QGraphicsWidget体系。
①:在常用的QWidget体系中,设置前后位置是主要是用raise(),underStack(),hide()函数。
理论为:在Qt中每个widget窗口都维持着自己的一个创建栈(widget's stack.),栈里边存放的是该窗口所有子widget窗口的标识符(代表其各个子widget)。当创建一个子widget后,系统便会将将代表其的标识符压入其父widget的创建栈中。而这各个子widget显示的前后顺序择是:栈顶的在最上边,栈底的最下边。所以:后压入栈的显示在上边,先入栈的在下边。 由此可知:后创建的子widget会显示出来!
那么:当我们创建了多个有重叠的子widget之后,最后创建的那个肯定是我们看到的那个。
那如何改变这种先后顺序呢?对于一个子widget,我们可以直接调用raise()函数来让其升到栈顶,从而显示在最上边用户面前,而不至于被遮挡; 当然我们也可以用underStack()来让其处于某个子widget下边,从而来指定前后顺序。
按照经验来看,其实只要使用raise()函数就足够了,需要显示谁,就直接让其调用raise()即可!!
②:对于QGraphicsWidget体系而言:
其内部放的是各个item,对于他们而言:我们是直接可以用setZValue()来设置各个item之间的前后位置。
当然:还有一种混合情况,亦即:一个QGraphicsScene,其中既可以 用addItem()来加入item;也可以addWidget()来加入QWidget对象。 此时对于这种既有item,又有Qwidget的情况,该如何定义彼此之间的前后位置呢?
其实当我们用addWidget()来想scene中加入QWidget时:该函数是有一个返回值的,为QGraphicsProxyWidget*类型指针。亦即:当我们调用addWidget()这个函数时,其一共做了两步操作:
一:系统首先把你原先的QWidget对象给转换成“item对象”。
二:而后再将这个转换后生成的item放到scene场景中。
但是其第一步操作中转换生成的“item对象”和真正的item对象还是不一样的,转换生成的仅仅是真正item的一种代理形式!即:生成了一个QGraphicsProxyWidget类型的代理item !! 但是对于这个代理item,我们也可以用setZValue()来设置其前后位置啦!
总结起来就是说:混合情况下,我们用addWidget()向一个scene场景中加入普通QWidget时,会返回一个QGraphicsProxyWidget*类型指针(其代表真正放入这个scene中的代理item),我们完全可以用它来调用setZValue()来设置前后位置,这样其便和普通Item的前后位置设置方式相统一一致了!!
(本条知识点来自
http://hi.baidu.com/zorru/blog/item/e24fbf0e6a8c4adb7bcbe19b.html)
5`窗体居中显示
原文地址:Qt 设置窗口居中显示作者:springbird
设置窗口居中显示
方法一:在窗口(QWidget类及派生类)的构造函数中添加如下代码:
#include <QDesktopWidget>
//.......
QDesktopWidget* desktop = QApplication::desktop(); // =qApp->desktop();也可以
move((desktop->width() - this->width())/2, (desktop->height() - this->height())/2);
//.......
重新编译后,该窗口启动时在屏幕居中的位置。
方法二:在调用show()函数后调用move()函数,j将窗口移动到屏幕中央。
#include <QDesktopWidget>
int main(int argc, char *argv[])
{
QApplication a(argc, argv);
MainWindow w;
w.move ((QApplication::desktop()->width() - w.width())/2,(QApplication::desktop()->height() - w.height())/2);
w.show();
return a.exec();
}