371. Sum of Two Integers
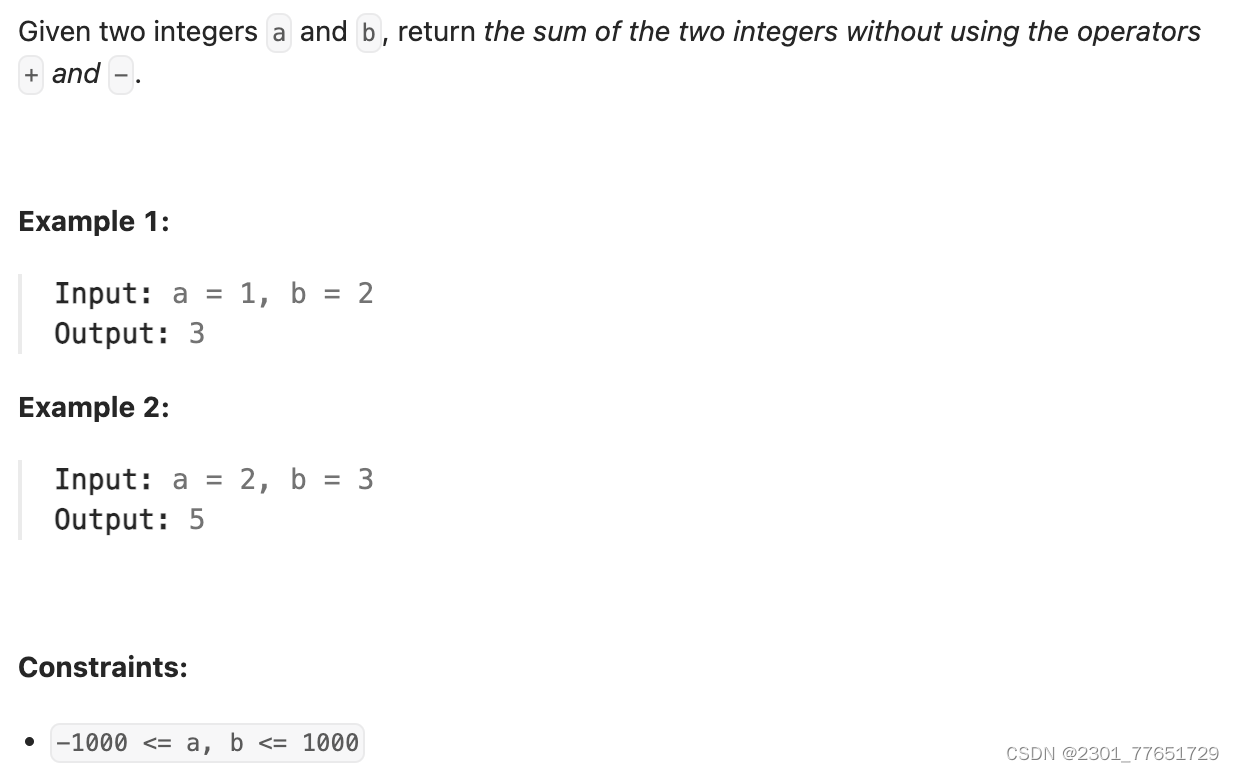
class Solution {
public int getSum(int a, int b) {
// 或运算 只要有1就为1 不能用与运算因为1&1还是1 可结果应该为0
while(b != 0) {
int ans = a ^ b;
int carry = (a & b) << 1;
a = ans;
b = carry;
}
return a;
}
}
201. Bitwise AND of Numbers Range
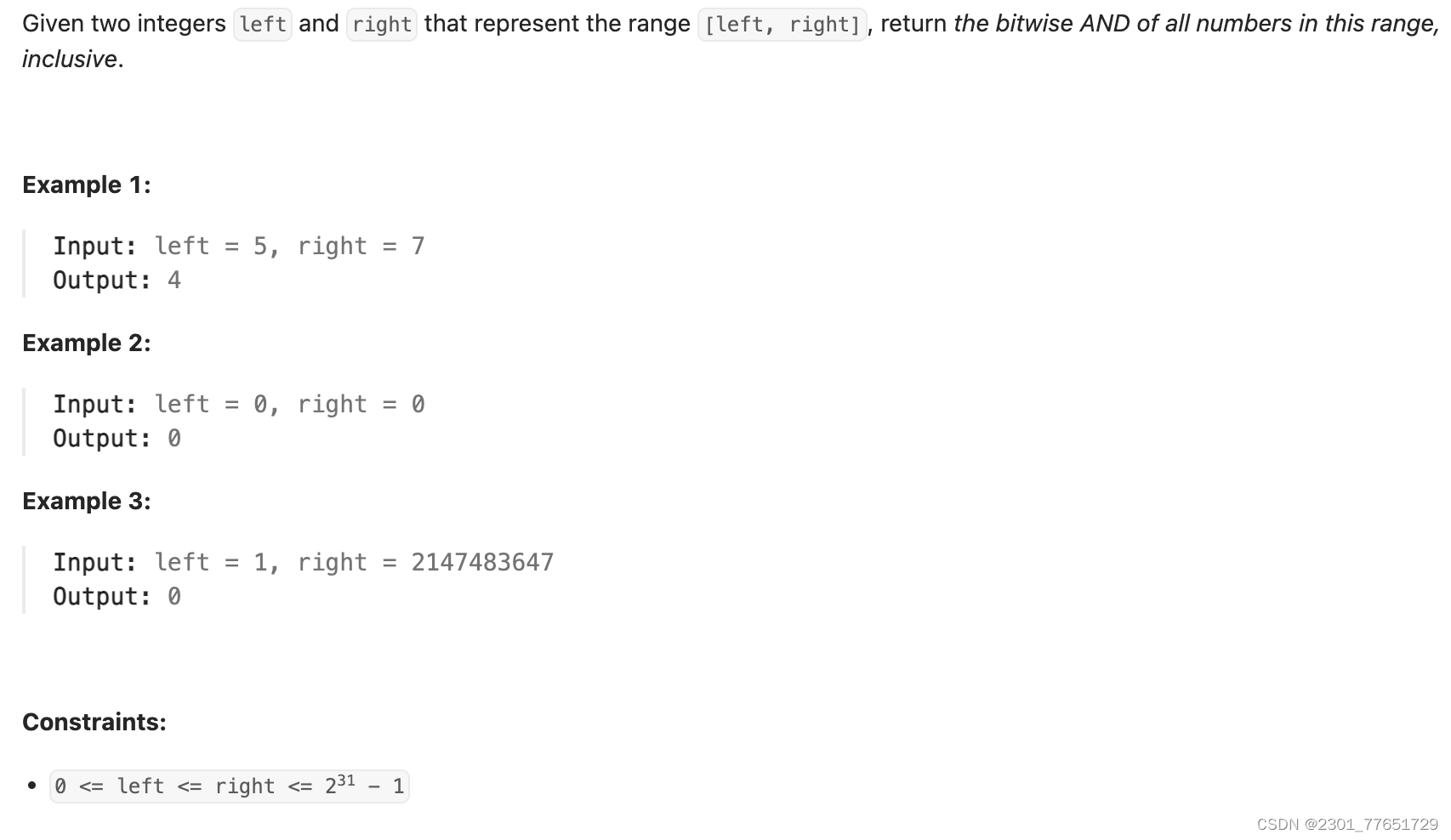
class Solution {
public int rangeBitwiseAnd(int left, int right) {
/*
Desired Range: [5,12]
Starting from 12, the loop will first do
12 & 11 = 8
next iteration, the loop will do
8 & 7 = 0
why did we skip anding of 10,9? Because even if we did so,
the result would eventually be anded with 8 whose value
would be lesser than equal to 8.
Hence, you start from the range end and
keep working your way down the range till you reach the start.
*/
while(left < right) {
right = right & (right - 1);
}
return right;
}
}
318. Maximum Product of Word Lengths
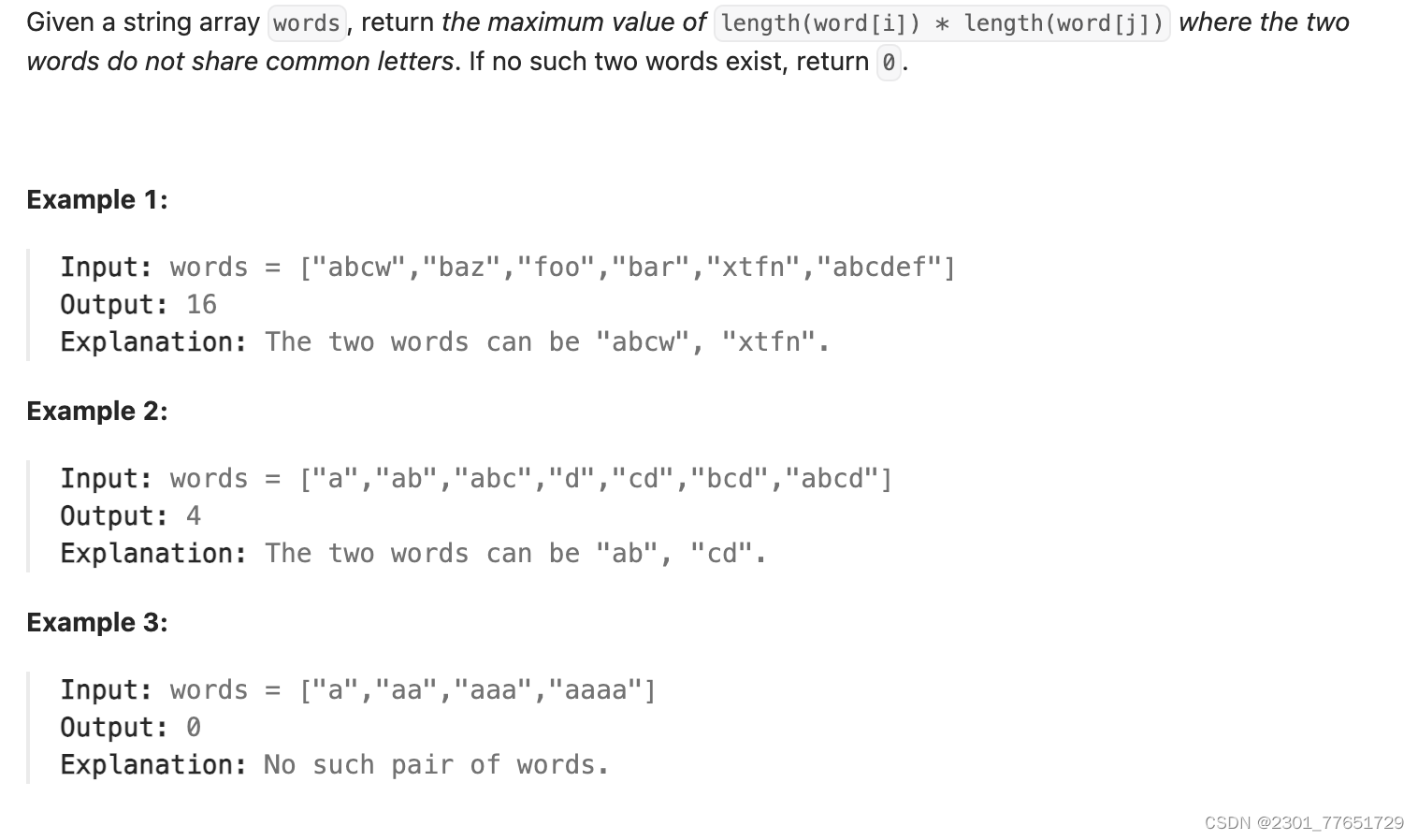
class Solution {
public int maxProduct(String[] words) {
int res = 0;
//26个字母26位,每一个字母出现一次那么所在位置即为1
int len = words.length;
int[] newBit = new int[len];//用来存储每个word的二进制值
for(int i = 0; i < len; i++) {
String word = words[i];
for(int j = 0; j < word.length(); j++) {
newBit[i] |= (1 << (word.charAt(j) - 'a'));
// Set the corresponding bit to 1 using bitwise OR. This operation keeps track of the presence of each letter in the word.
//或操作可以记录每个word的每个字母是否出现过,或运算只要有1就是1
}
}
for(int i = 0; i < len; i++) {
for(int j = i + 1; j < len; j++) {
//与运算,同时为1才是1,不然都是0,0与任何运算都是0,结果为0 代表可以重复字母出现
if ((newBit[i] & newBit[j]) == 0) {
res = Math.max(res, words[i].length() * words[j].length());
}
}
}
return res;
}
}
504. Base 7
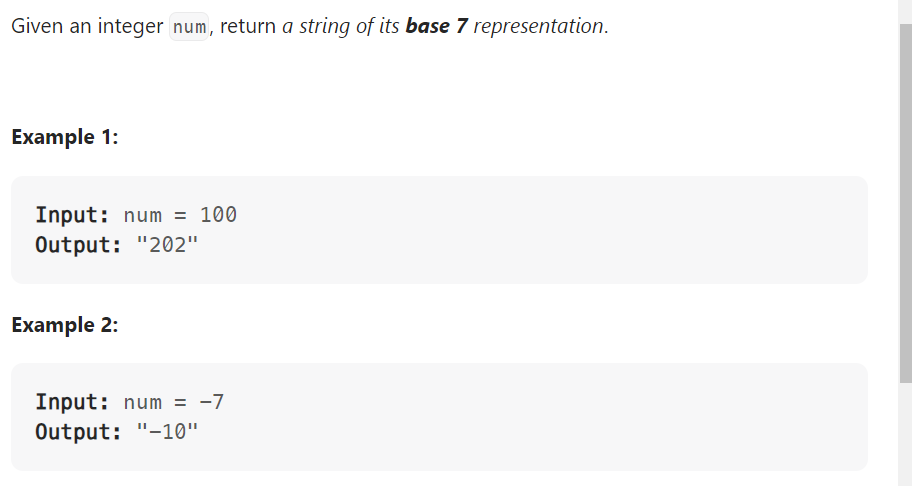
class Solution {
public String convertToBase7(int num) {
StringBuilder res = new StringBuilder();
boolean flag = false;
if(num == 0) {
return "0";
}
if(num < 0) {
flag = true;
}
while(num != 0) {
res.append(Math.abs(num % 7));
num /= 7;
}
if(flag) {
res.append("-");
}
return res.reverse().toString();
// return Integer.toString(num, 7); //Integer.toString(int,int)将整数转换成对应的进制
}
}
9. Palindrome Number
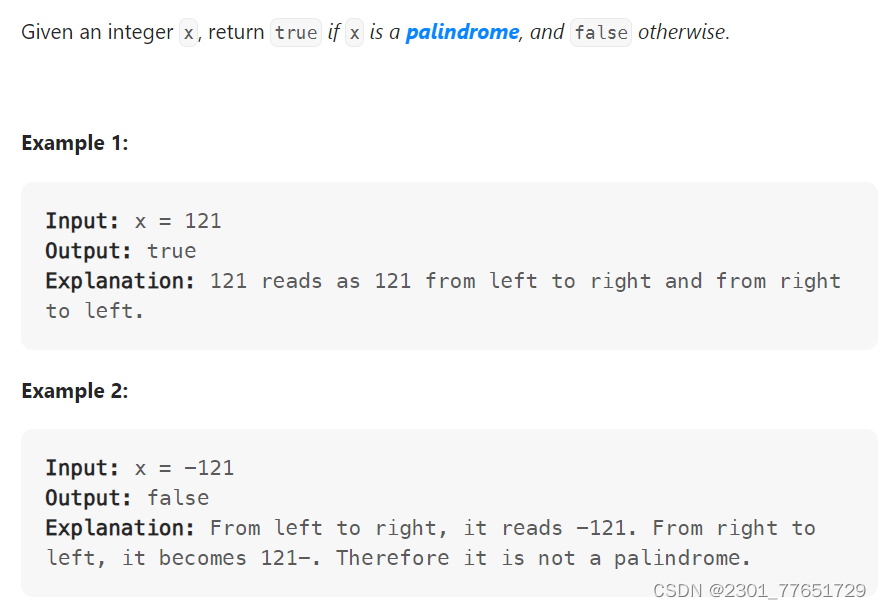
class Solution {
public boolean isPalindrome(int x) {
String xx = Integer.toString(x);//String.valueOf()convert to string
int len = xx.length();
if(len <= 1) {
return true;
}
for(int i = 0; i < len / 2; i++) {
if(xx.charAt(i) != xx.charAt(len-1-i)) {
return false;//judge false first
}
}
return true;
}
}
class Solution {
public boolean isPalindrome(int x) {
String xx = Integer.toString(x);//String.valueOf()convert to string
int len = xx.length();
if(len <= 1) {
return true;
}
int left = 0;
int right = len - 1;
while(left < right) {
if(xx.charAt(left) != xx.charAt(right)) {
return false;
}
left++;
right--;
}
return true;
}
}
231. Power of Two
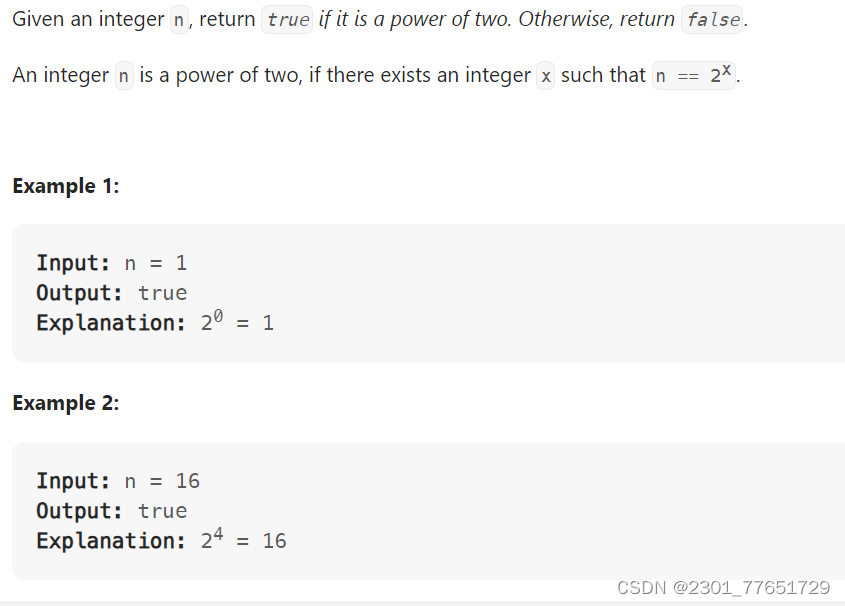
class Solution {
public boolean isPowerOfTwo(int n) {
if(n <= 0) {
return false;
}
if(n == 1) {
return true;
}
while(n > 1) {
if(n % 2 != 0) {
return false;
}
n /= 2;
}
return true;
}
}
class Solution {
public boolean isPowerOfTwo(int n) {
if(n <= 0) {
return false;
}
if(n == 1) {
return true;
}
if(n % 2 != 0) {
return false;
} else {
return isPowerOfTwo(n / 2);
}
}
}
263. Ugly Number
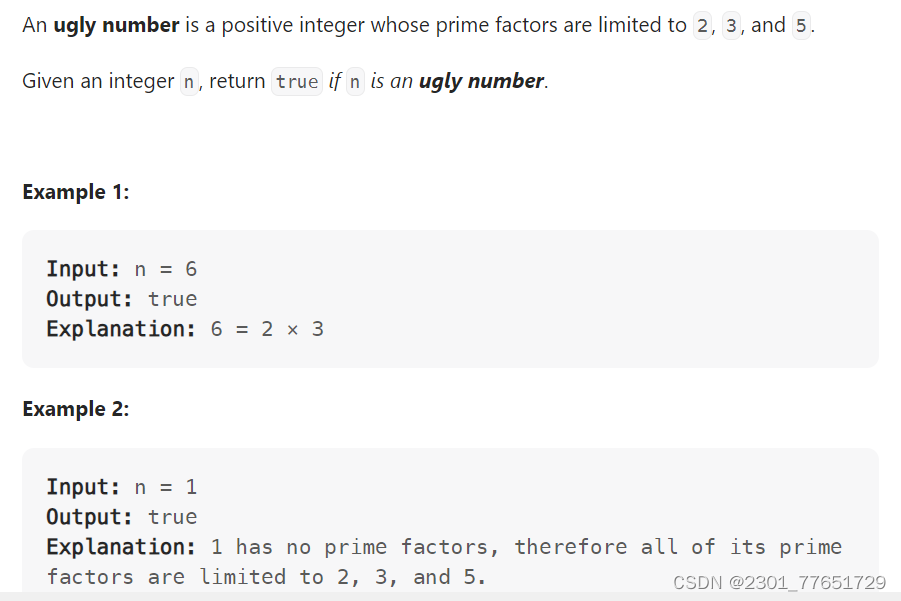
class Solution {
public boolean isUgly(int n) {
if (n <= 0) return false;
while(n > 1) {
if (n % 2 == 0) {
n = n / 2;
} else if (n % 3 == 0) {
n = n / 3;
} else if (n % 5 == 0) {
n = n / 5;
} else break;
}
return (n == 1);
}
}
190. Reverse Bits
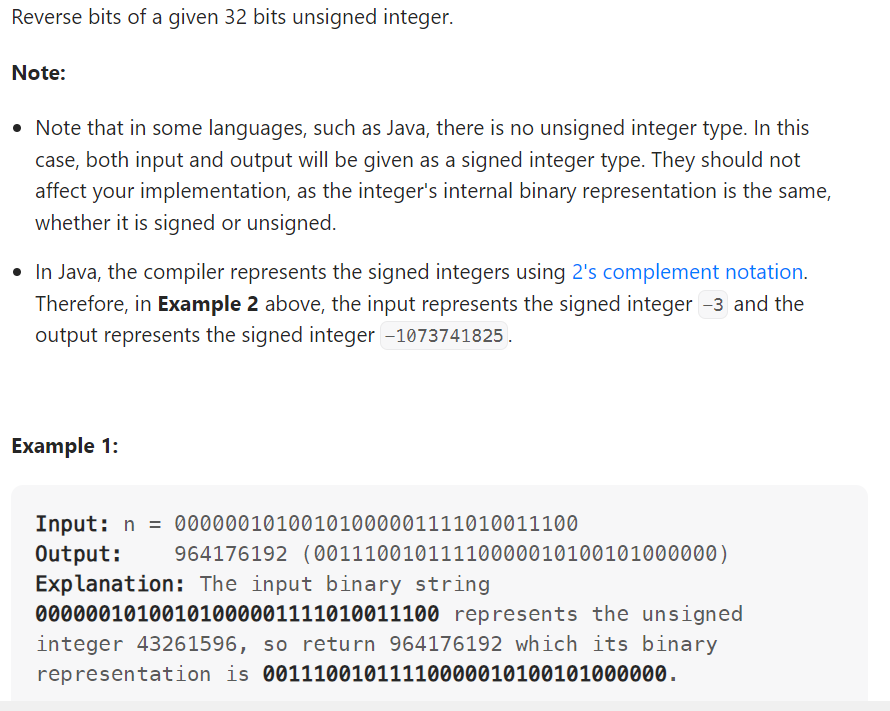
public class Solution {
// you need treat n as an unsigned value
public int reverseBits(int n) {
int res = 0;
for(int i = 0; i < 32; i++) {
res = (res << 1) ^ (1 & n);
n = n >> 1;
}
return res;
}
}
492. Construct the Rectangle
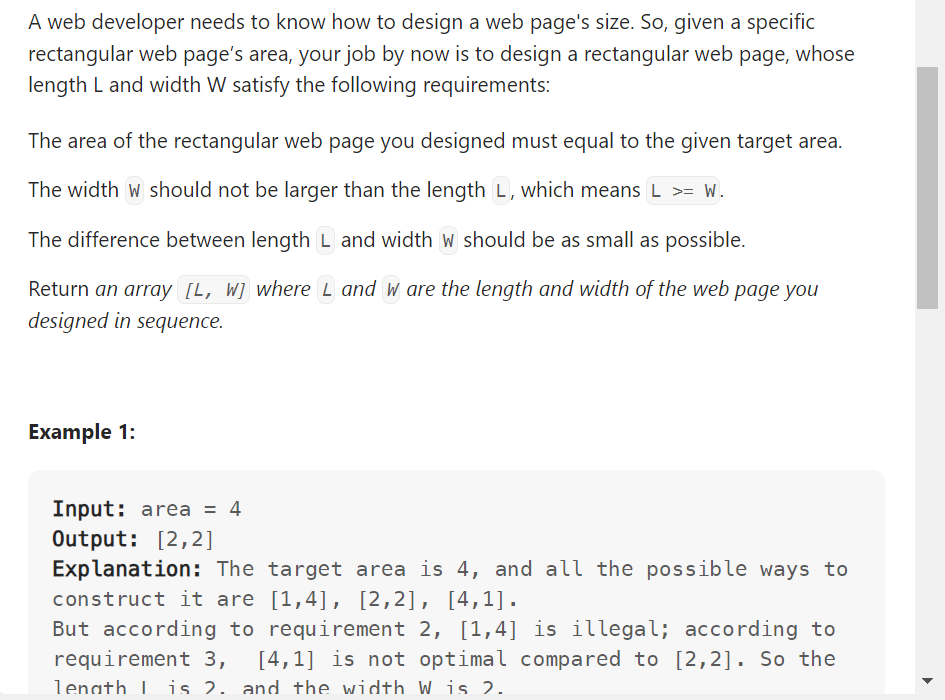
class Solution {
public int[] constructRectangle(int area) {
int wid = (int) Math.sqrt(area);
while(area % wid != 0) {
wid--;
}
return new int[] { area / wid, wid};
}
}
class Solution {
public int[] constructRectangle(int area) {
for(int i =(int) Math.sqrt(area); i > 0; i--) {
if(i * (area / i) == area) {
return new int[]{area / i, i};
}
}
return new int[]{area,1};
}
}
50. Pow(x, n)
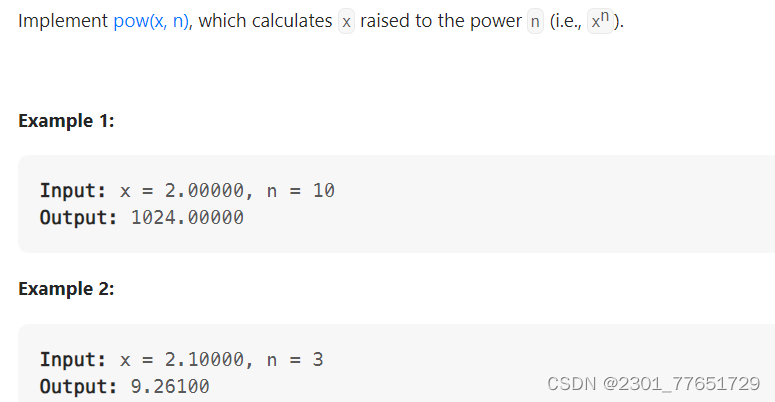
class Solution {
public double myPow(double x, int n) {
return Math.pow(x, n); //math.pow returns double data type
}
}
372. Super Pow ✖️
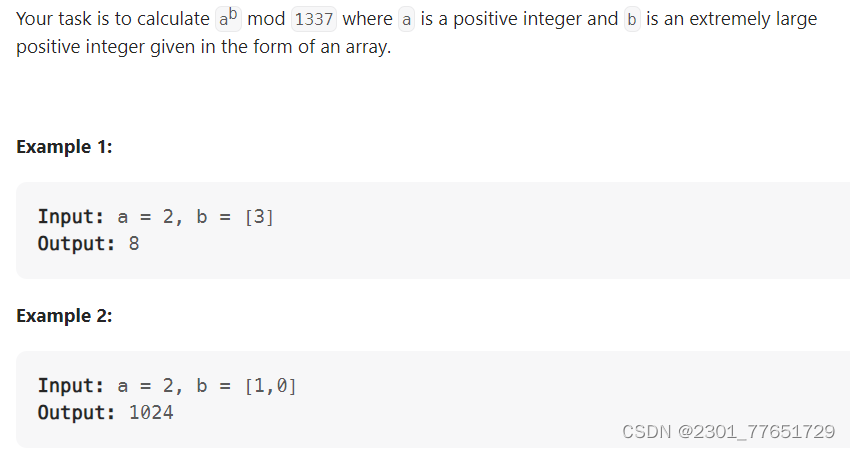
class Solution {
int mod = 1337;
public int superPow(int a, int[] b) {
return dfs(a, b, b.length - 1);
}
int dfs(int a, int[] b, int u) {
if (u == -1) return 1;
return qpow(dfs(a, b, u - 1), 10) * qpow(a, b[u]) % mod;
}
int qpow(int a, int b) {
int ans = 1;
a %= mod;
while(b != 0) {
if((b & 1) != 0) ans = ans * a % mod;
a = a * a % mod;
b>>=1;
}
return ans;
}
}