100. Same Tree
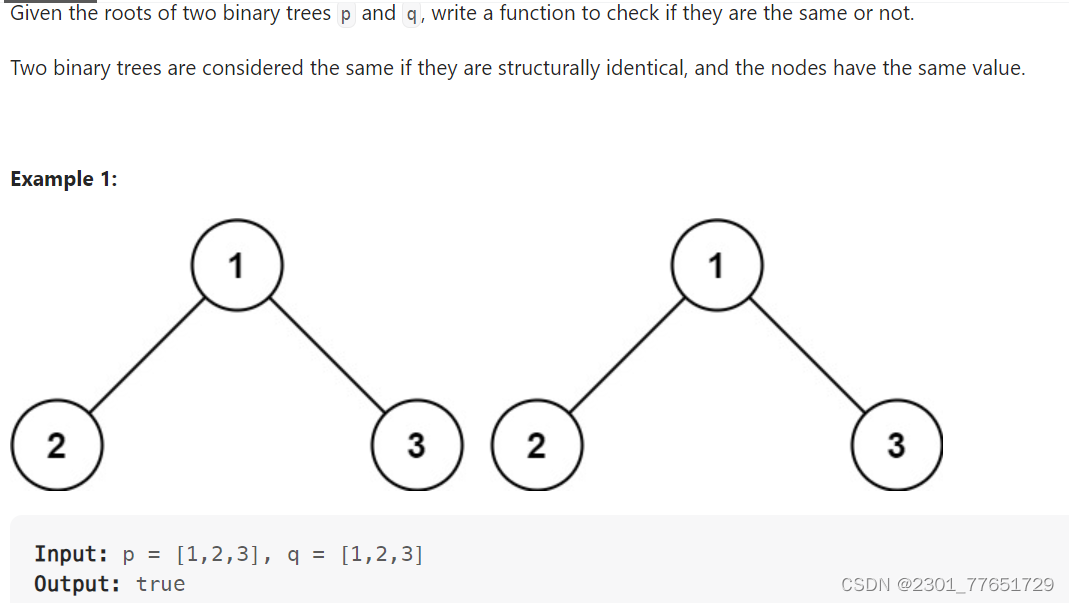
class Solution {
public boolean isSameTree(TreeNode p, TreeNode q) {
if(p == null && q == null) {
return true;
}
if(p == null || q == null) {
return false;
}
return (p.val == q.val) && isSameTree(p.left, q.left)
&& isSameTree(p.right, q.right);
}
}
101. Symmetric Tree
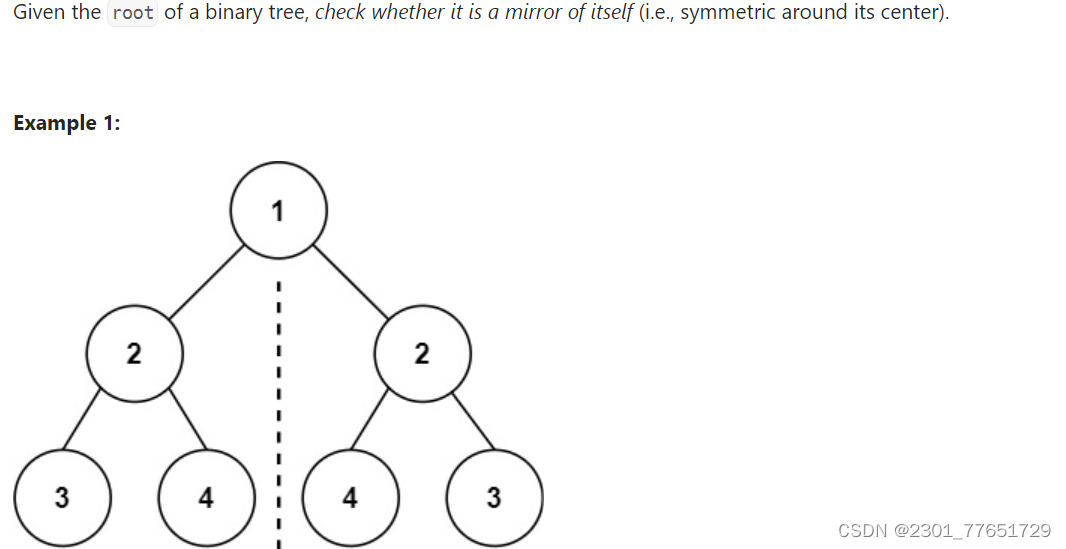
class Solution {
public boolean isSymmetric(TreeNode root) {
if(root == null) {
return true;
}
return valid(root.left, root.right);
}
public boolean valid(TreeNode leftT, TreeNode rightT) {
if(leftT == null && rightT == null) {
return true;
}
if(leftT == null || rightT == null || leftT.val != rightT.val) {
return false;
}
return valid(leftT.left, rightT.right) && valid(leftT.right, rightT.left);
}
}
226. Invert Binary Tree
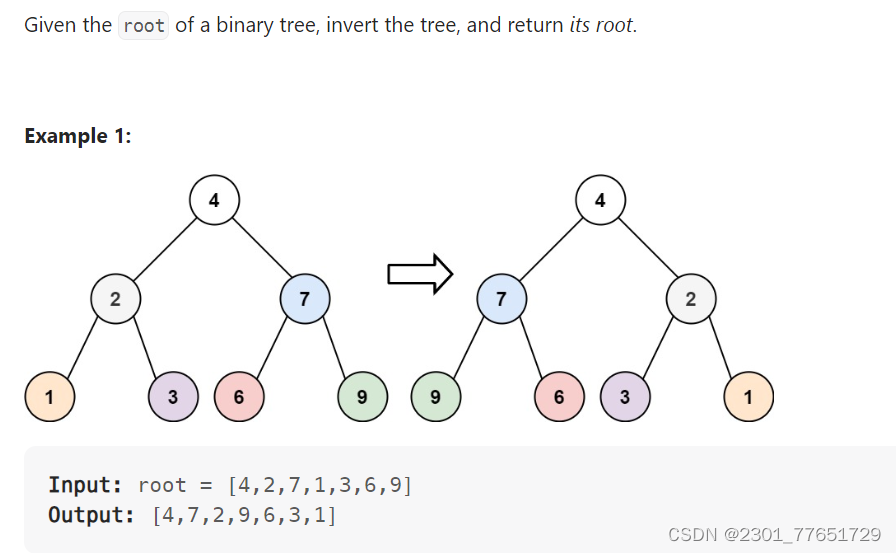
class Solution {
public TreeNode invertTree(TreeNode root) {
if(root != null) {
TreeNode temp = root.left;
root.left = root.right;
root.right = temp;
invertTree(root.right);
invertTree(root.left);
}
return root;
}
}
617. Merge Two Binary Trees
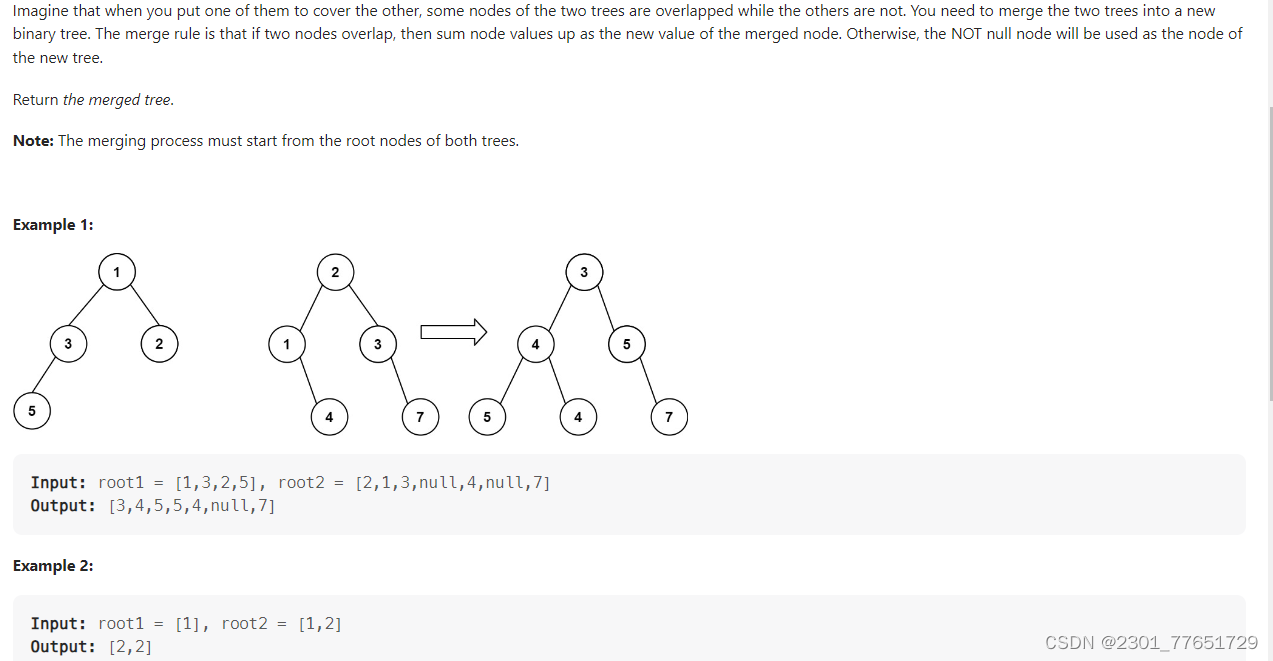
class Solution {
public TreeNode mergeTrees(TreeNode t1, TreeNode t2) {
if(t1 == null) {
return t2;
}
if(t2 == null) {
return t1;
}
TreeNode newHead = new TreeNode(t1.val + t2.val);
newHead.left = mergeTrees(t1.left, t2.left);
newHead.right = mergeTrees(t1.right, t2.right);
return newHead;
}
}
572. Subtree of Another Tree
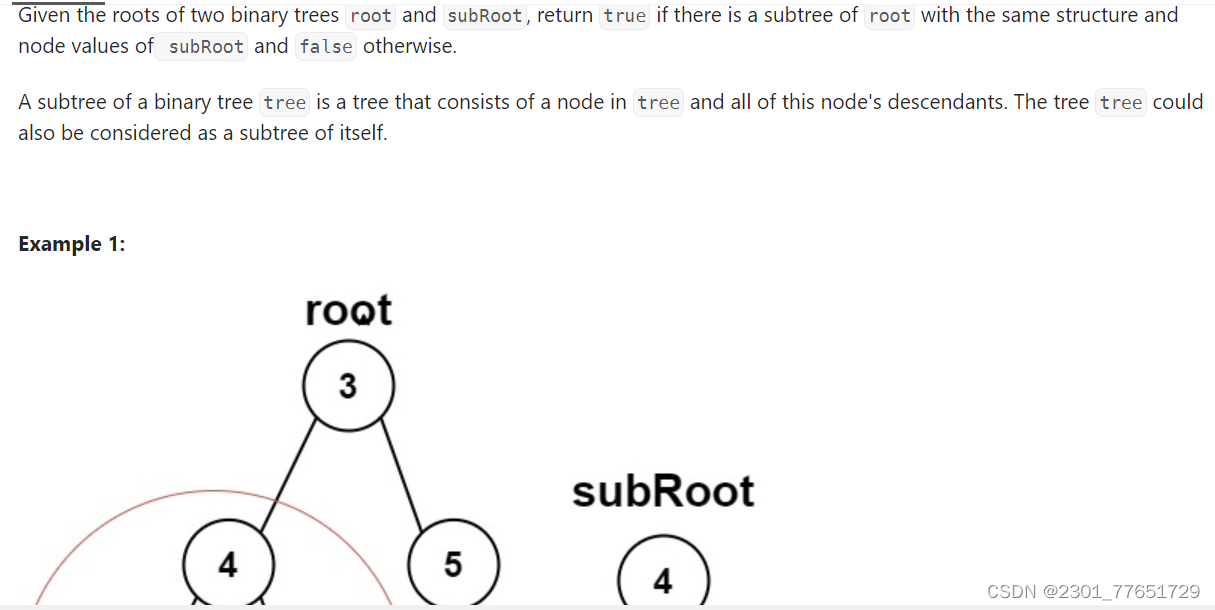
class Solution {
public boolean isSubtree(TreeNode root, TreeNode subRoot) {
if(root == null && subRoot == null) {
return true;
}
if(root == null && subRoot != null) {
return false;
}
if(root != null && subRoot == null) {
return true;
}
return isSameTree(root, subRoot) || isSubtree(root.left, subRoot) || isSubtree(root.right, subRoot);
}
public boolean isSameTree(TreeNode root, TreeNode subRoot) {
if(root == null && subRoot == null) {
return true;
}
if(root == null && subRoot != null) {
return false;
}
if(root != null && subRoot == null) {
return false;
}
return root.val == subRoot.val
&& isSameTree(root.left, subRoot.left)
&& isSameTree(root.right, subRoot.right);
}
}
543. Diameter of Binary Tree
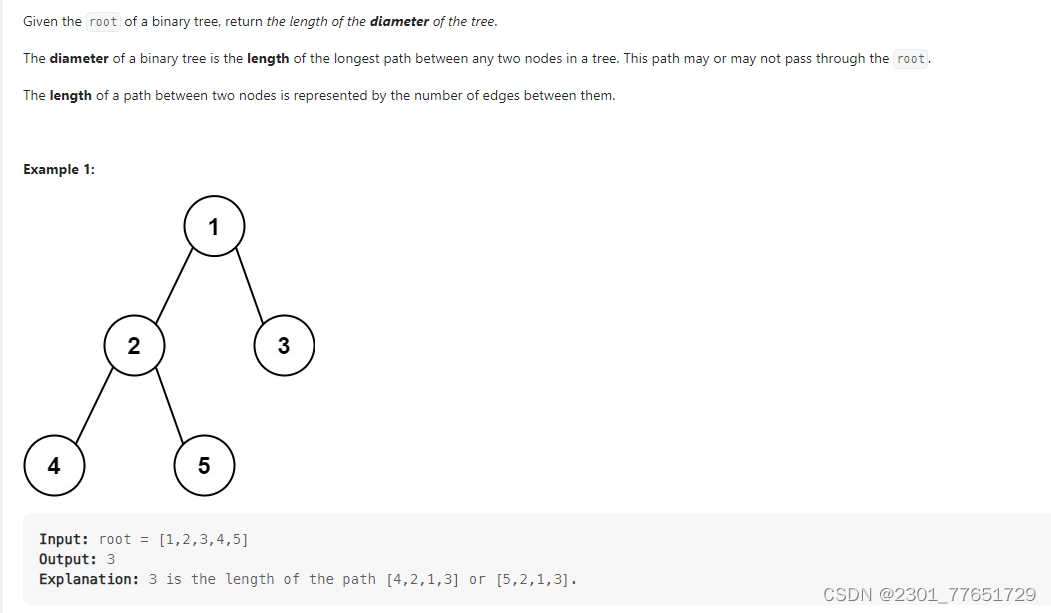
class Solution {
int ans = 0;
public int diameterOfBinaryTree(TreeNode root) {
depth(root);
return ans;
}
public int depth(TreeNode root) {
if(root == null) {
return 0;
}
int left = depth(root.left), right = depth(root.right);
ans = Math.max(left + right, ans);
return Math.max(left,right) + 1;
}
}
559. Maximum Depth of N-ary Tree
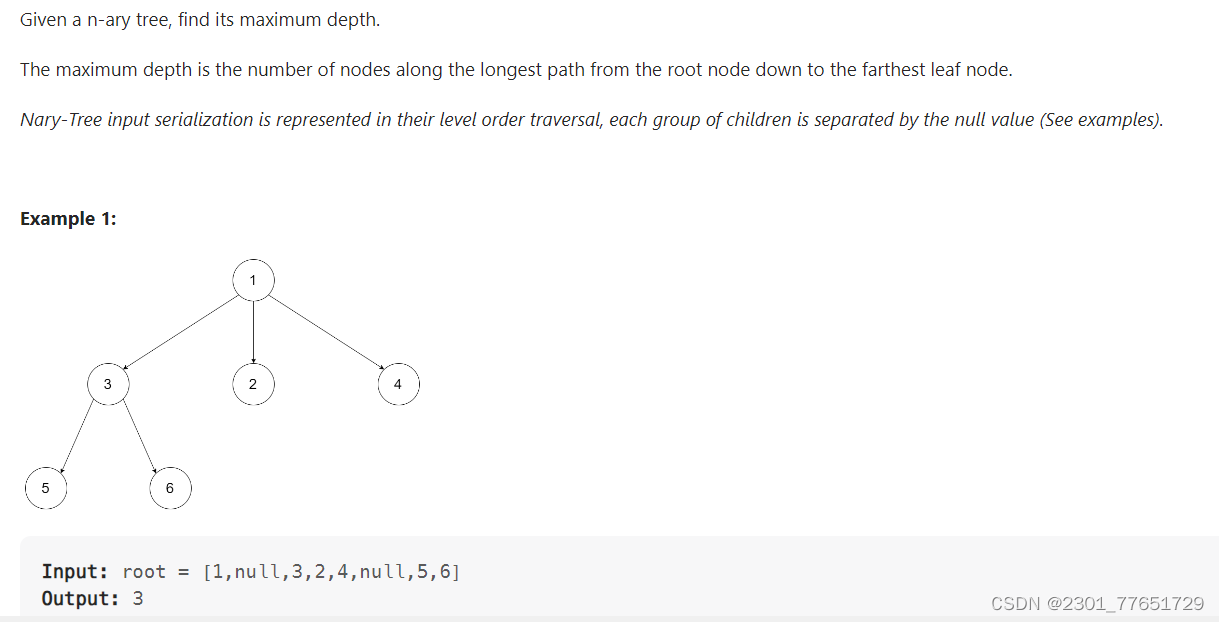
class Solution {
public int maxDepth(Node root) {
if(root == null) {
return 0;
}
int max = 0;
for(Node child : root.children) {
max = Math.max(max, maxDepth(child));
}
return max + 1;
}
}
671. Second Minimum Node In a Binary Tree
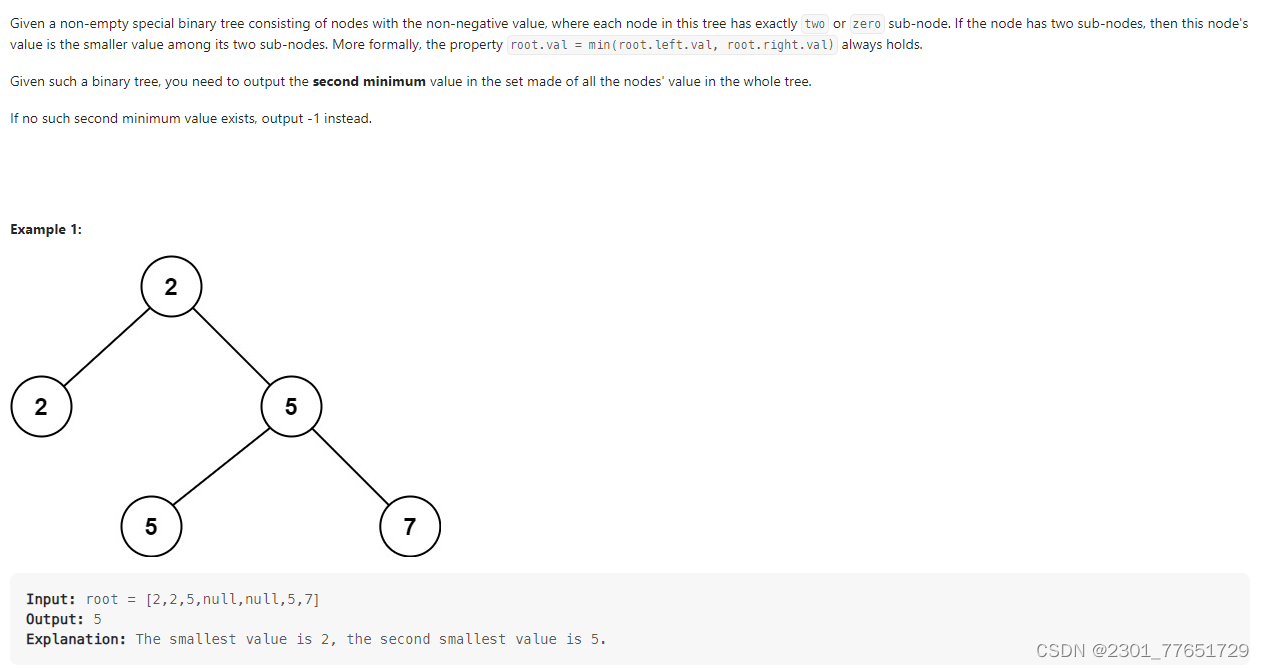
class Solution {
public ArrayList<Integer> nums = new ArrayList<>();
public int findSecondMinimumValue(TreeNode root) {
dfs(root);
Collections.sort(nums);
int num = nums.get(0);
for(int i=0;i<nums.size();i++){
if(nums.get(i)!=num){
return nums.get(i);
}
}
return -1;
}
void dfs(TreeNode node){
if(node==null){
return;
}
nums.add(node.val);
dfs(node.left);
dfs(node.right);
}
}
637. Average of Levels in Binary Tree
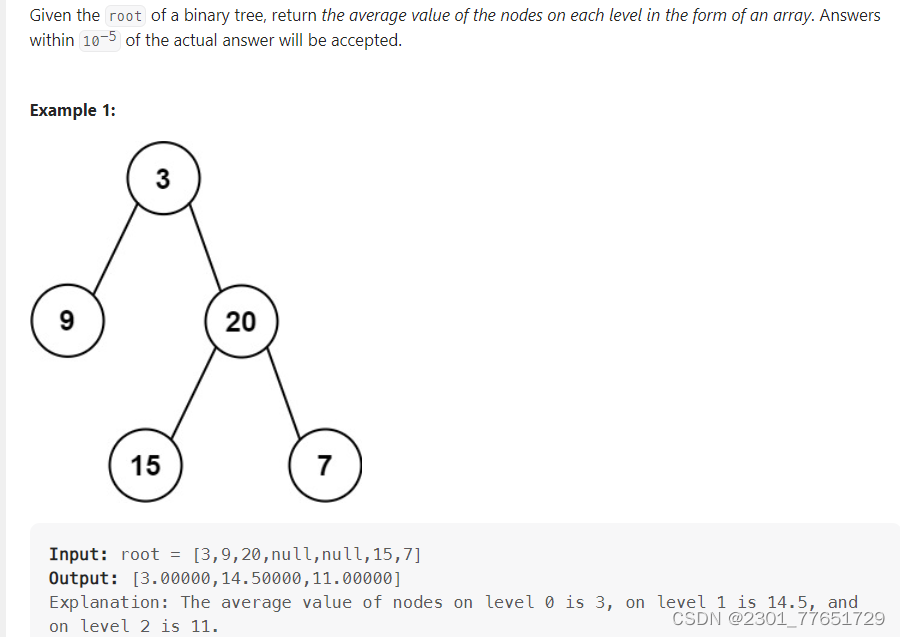
class Solution {
public List<Double> averageOfLevels(TreeNode root) {
List<Double> ans = new ArrayList();
if(root == null) {
return ans;
}
Queue<TreeNode> queue = new LinkedList();
queue.add(root);
while(!queue.isEmpty()) {
int n = queue.size();
double avg = 0l;
for(int i = 0; i < n; i++) {
TreeNode cur = queue.poll();
avg = avg + (double)cur.val;
if(cur.left != null) {
queue.add(cur.left);
}
if(cur.right != null) {
queue.add(cur.right);
}
}
avg = avg / n;
ans.add(avg);
}
return ans;
}
}
653. Two Sum IV - Input is a BST
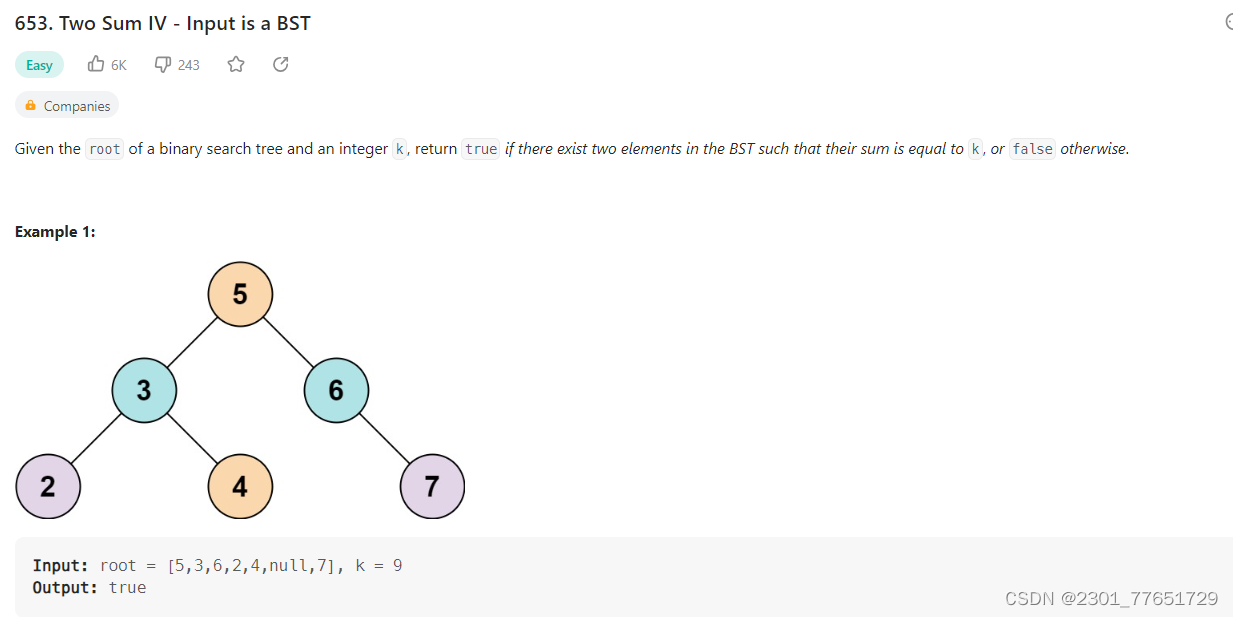
class Solution {
Set<Integer> set = new HashSet();
public boolean findTarget(TreeNode root, int k) {
if(root == null) {
return false;
}
if(set.contains(k - root.val)) {
return true;
}
set.add(root.val);
return findTarget(root.left, k) || findTarget(root.right, k);
}
}
104. Maximum Depth of Binary Tree
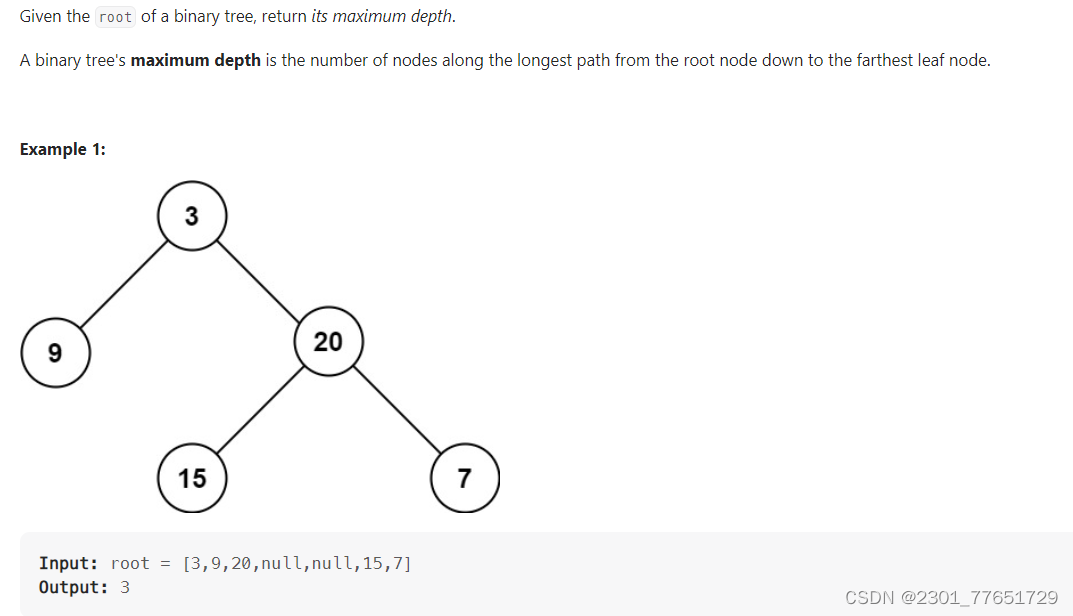
class Solution {
public int maxDepth(TreeNode root) {
if(root == null) {
return 0;
}
int left = maxDepth(root.left);
int right = maxDepth(root.right);
return Math.max(left, right) + 1;
}
}
111. Minimum Depth of Binary Tree
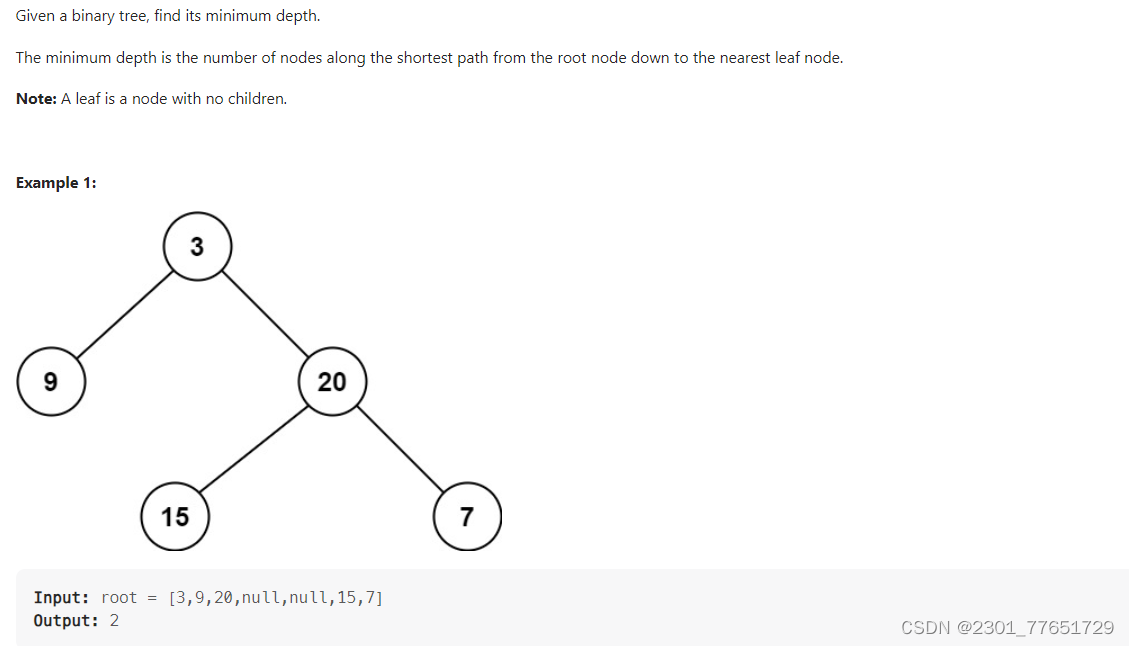
class Solution {
public int minDepth(TreeNode root) {
if(root == null) {
return 0;
}
if(root.left == null && root.right == null) {
return 1;
}
if(root.left == null) {
return minDepth(root.right) + 1;
}
if(root.right == null) {
return minDepth(root.left) + 1;
}
return Math.min(minDepth(root.left), minDepth(root.right)) + 1;
}
}
class Solution {
public int minDepth(TreeNode root) {
if(root == null) {
return 0;
}
Queue<TreeNode> q = new LinkedList();
int depth = 0;
q.offer(root);
while(!q.isEmpty()) {
int size = q.size();
depth++;
for(int i = 0; i < size; i++) {
TreeNode node = q.poll();
if(node.left == null && node.right == null) {
return depth;
}
if(node.left != null) {
q.offer(node.left);
}
if(node.right != null) {
q.offer(node.right);
}
}
}
return depth;
}
}
112. Path Sum
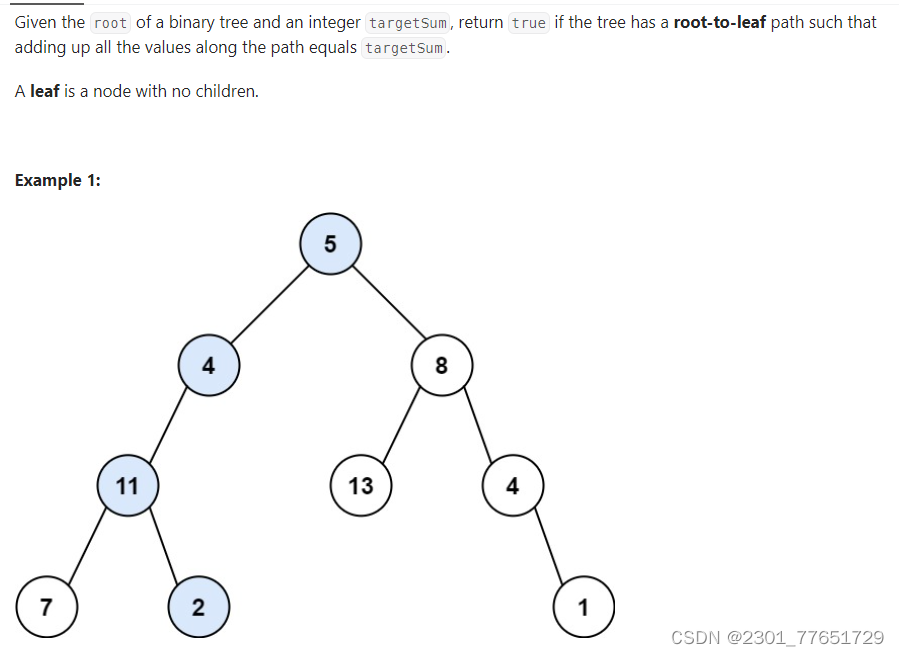
class Solution {
public boolean hasPathSum(TreeNode root, int targetSum) {
if(root == null) {
return false;
}
if(root.left == null && root.right == null) {
return targetSum == root.val;
}
return hasPathSum(root.left, targetSum - root.val) || hasPathSum(root.right, targetSum - root.val);
}
}
113. Path Sum II
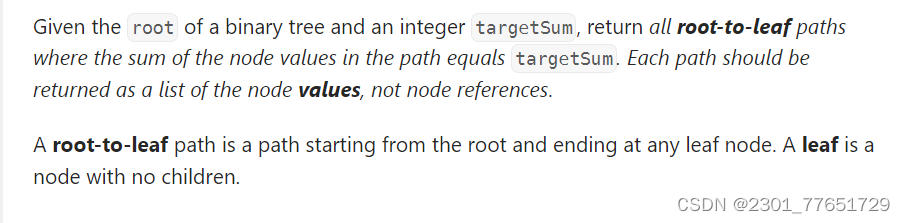
class Solution {
public List<List<Integer>> pathSum(TreeNode root, int targetSum) {
List<List<Integer>> ans=new ArrayList();
List<Integer> li=new ArrayList();
path(root,targetSum,ans,li);
return ans;
}
static void path(TreeNode root,int sum,List<List<Integer>> ans,List<Integer> li){
if(root==null){
return;
}
li.add(root.val);
if(root.left==null && root.right==null){
sum-=root.val;
if(sum==0){
ans.add(new ArrayList<>(li));
}
}
path(root.left,sum-root.val,ans,li);
path(root.right,sum-root.val,ans,li);
li.remove(li.size()-1);// remove the last element
}
}
404. Sum of Left Leaves
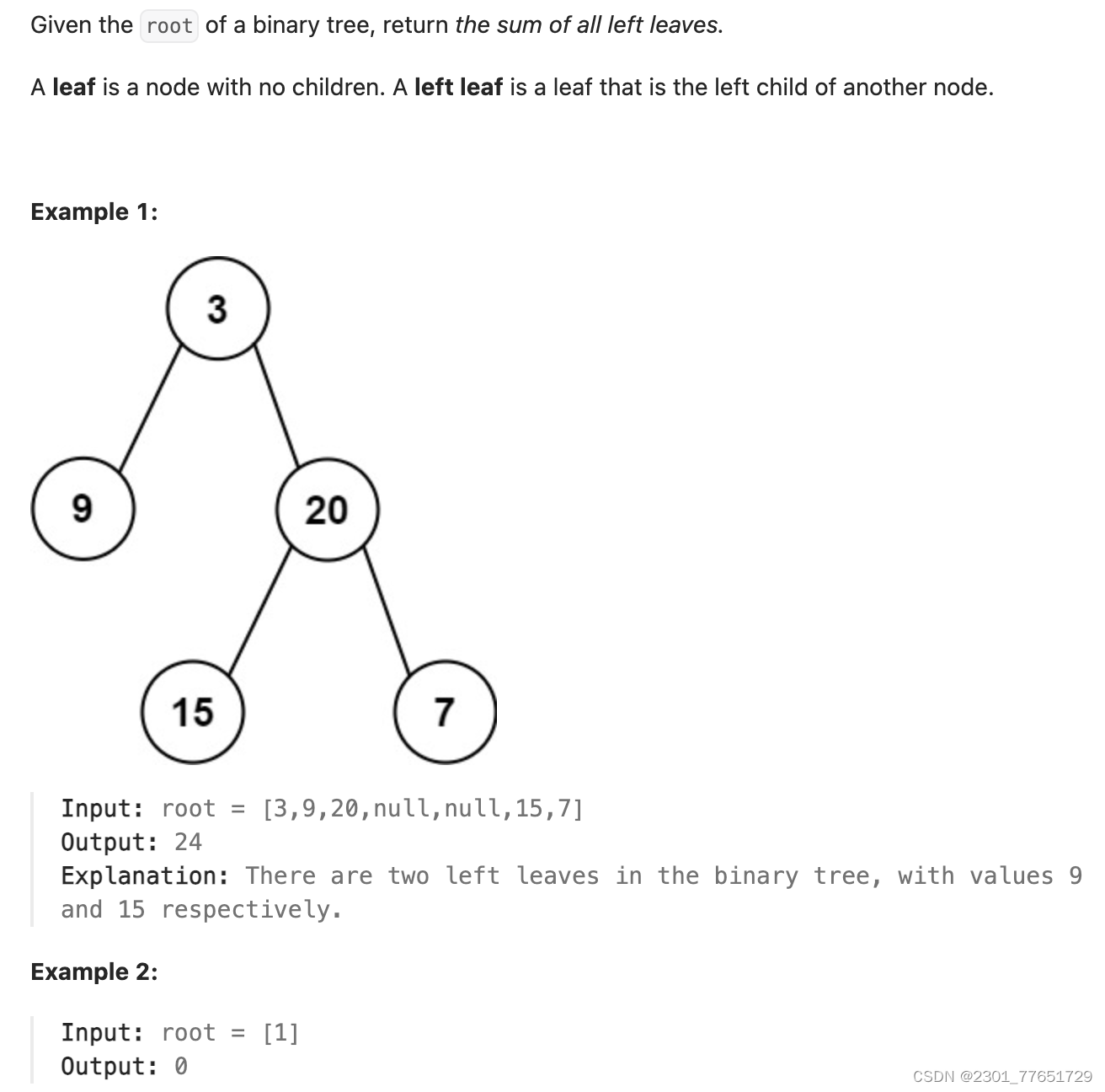
class Solution {
int sum = 0;
public int sumOfLeftLeaves(TreeNode root) {
cal(root);
return sum;
}
public void cal(TreeNode root) {
if(root == null) {
return ;
}
if(root.left != null && root.left.left == null && root.left.right == null) {
sum += root.left.val;
}
cal(root.left);
cal(root.right);
}
}
144. Binary Tree Preorder Traversal
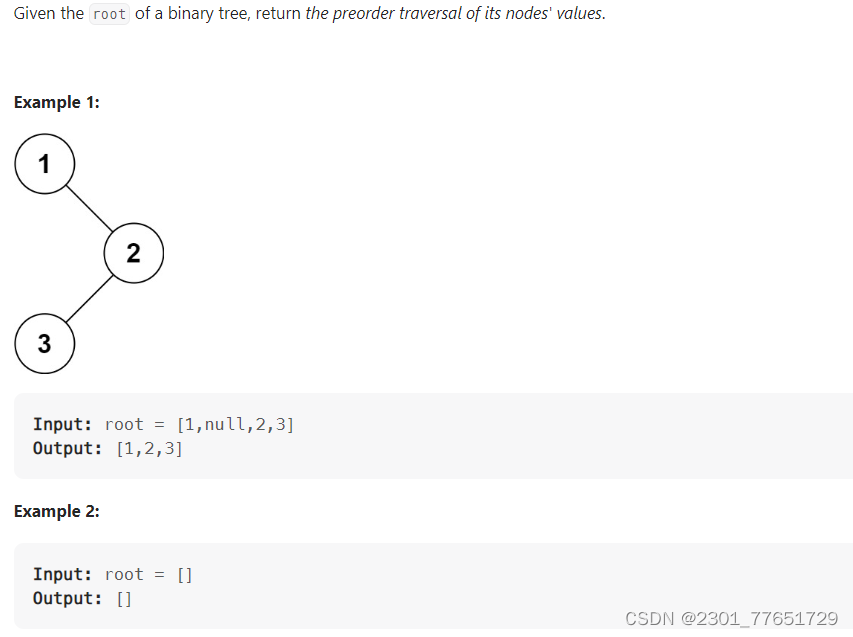
class Solution {
public void traversal(TreeNode root, List<Integer> res) {
if(root == null) {
return;
}
res.add(root.val);
traversal(root.left, res);
traversal(root.right, res);
}
public List<Integer> preorderTraversal(TreeNode root) {
List<Integer> res = new ArrayList();
traversal(root, res);
return res;
}
}
class Solution {
List<Integer> res = new ArrayList();
public List<Integer> preorderTraversal(TreeNode root) {
if(root == null) {
return res;
}
res.add(root.val);
preorderTraversal(root.left);
preorderTraversal(root.right);
return res;
}
}
589. N-ary Tree Preorder Traversal
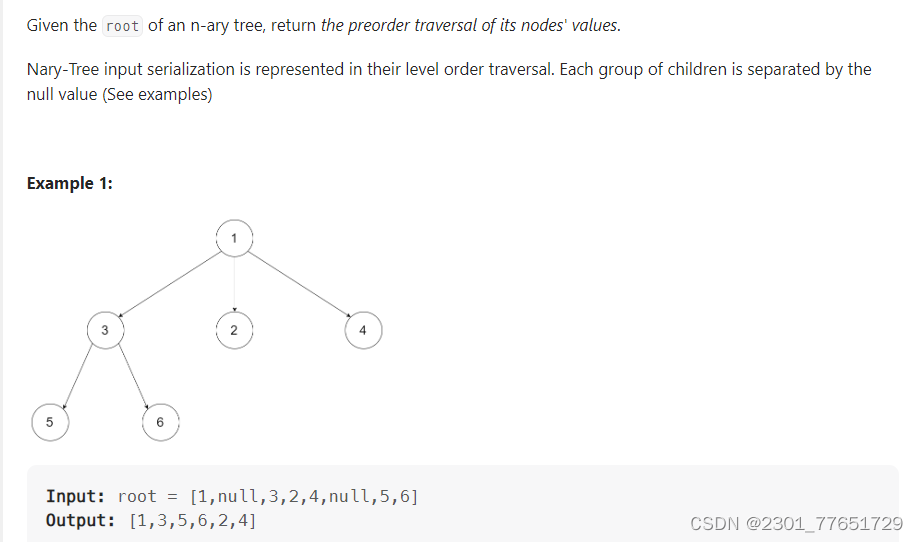
class Solution {
List<Integer> arr = new ArrayList();
public List<Integer> preorder(Node root) {
if(root == null) {
return arr;
}
arr.add(root.val);
for(Node i : root.children) {
preorder(i);
}
return arr;
}
}
606. Construct String from Binary Tree
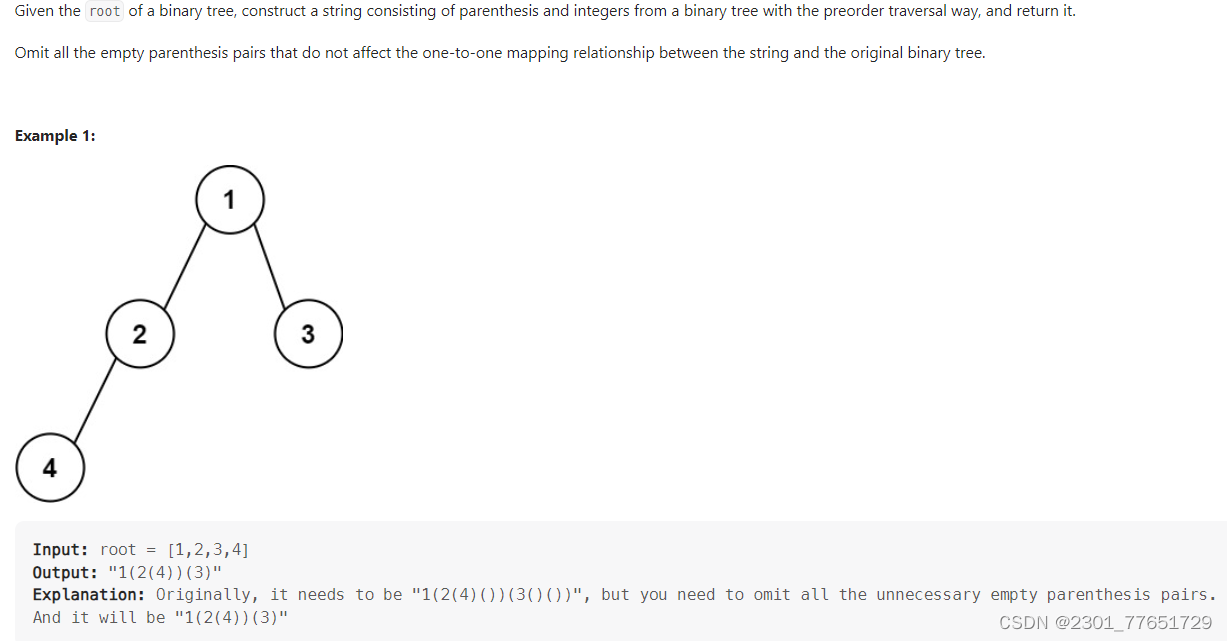
class Solution {
public String tree2str(TreeNode root) {
if(root == null)
return "";
if(root.left == null && root.right == null)
return root.val + "";
if(root.right == null)
return root.val + "(" + tree2str(root.left) + ")";
return root.val + "(" + tree2str(root.left) + ")(" + tree2str(root.right) + ")";
}
}
145. Binary Tree Postorder Traversal
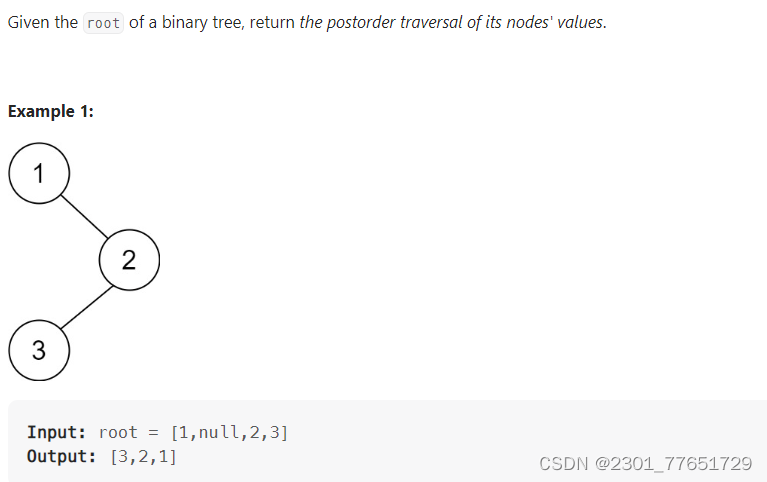
class Solution {
List<Integer> res = new ArrayList();
public List<Integer> postorderTraversal(TreeNode root) {
traversePostOrder(root);
return res;
}
private void traversePostOrder(TreeNode node) {
if (node == null) return;
if (node.left != null) {
traversePostOrder(node.left);
}
if (node.right != null) {
traversePostOrder(node.right);
}
res.add(node.val);
}
}
590. N-ary Tree Postorder Traversal
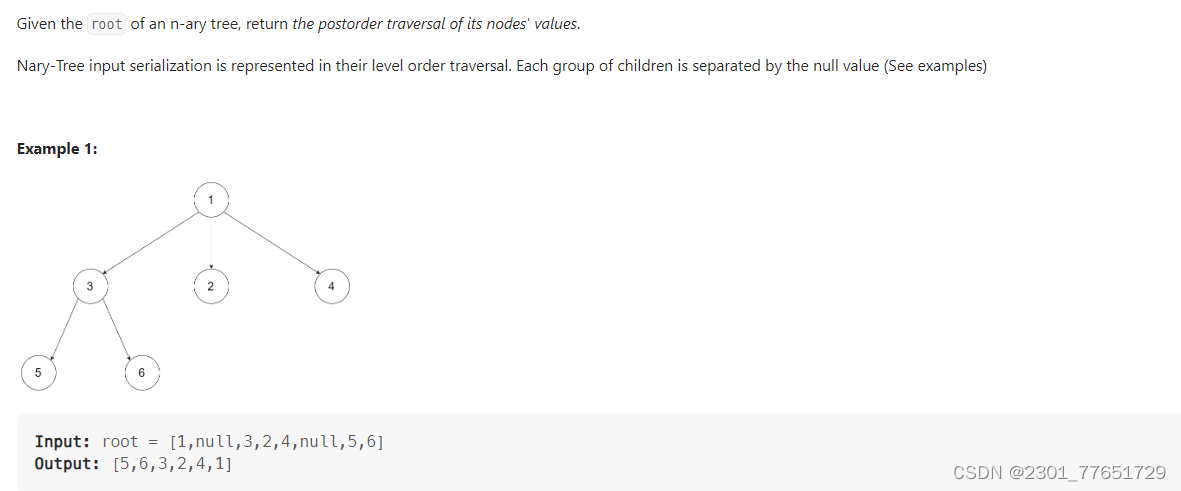
class Solution {
public List<Integer> postorder(Node root) {
List<Integer> result = new ArrayList<>();
if (root == null) {
return new ArrayList();
}
traverseTree(root, result);
return result;
}
private void traverseTree(Node root, List<Integer> result) {
if (root.children == null) {
result.add(root.val);
return;
}
for (Node n : root.children) {
traverseTree(n, result);
}
result.add(root.val);
}
}
94. Binary Tree Inorder Traversal
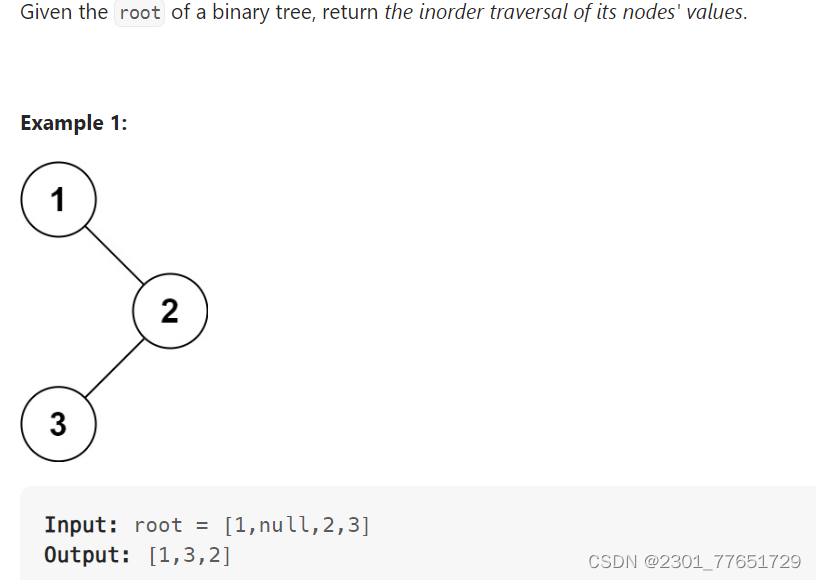
class Solution {
List<Integer> list = new ArrayList();
public List<Integer> inorderTraversal(TreeNode root) {
if(root == null) {
return list;
}
inorderTraversal(root.left);
list.add(root.val);
inorderTraversal(root.right);
return list;
}
}
Console
700. Search in a Binary Search Tree
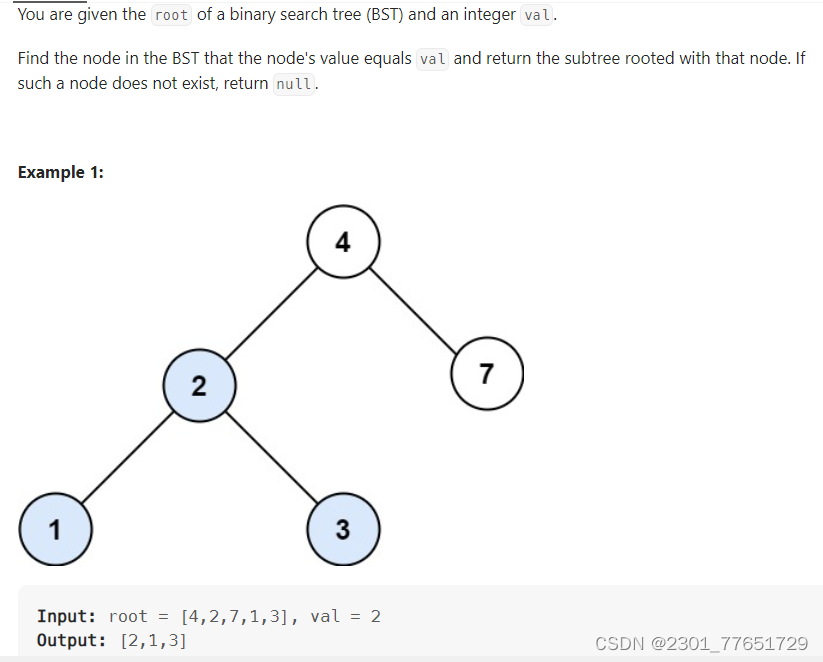
class Solution {
public TreeNode searchBST(TreeNode root, int val) {
if(root == null|| root.val == val ) {
return root;
}
if(root.val < val) {
return searchBST(root.right, val);
} else {
return searchBST(root.left, val);
}
}
}
530. Minimum Absolute Difference in BST
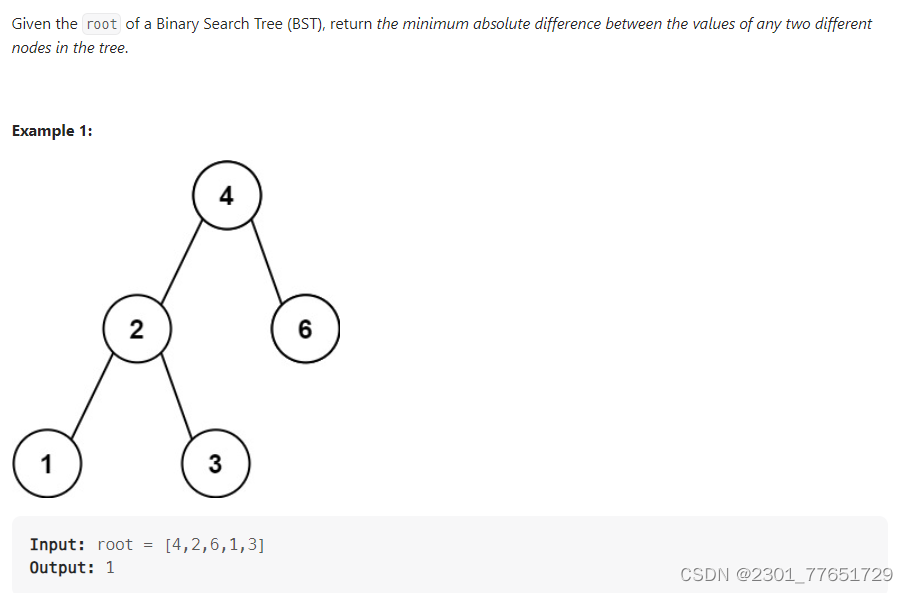
class Solution {
TreeNode pre = null;
int min = Integer.MAX_VALUE;
public int getMinimumDifference(TreeNode root) {
traverse(root);
return min;
}
public void traverse(TreeNode root) {
if(root == null) {
return;
}
traverse(root.left);
if(pre != null) {
int diff = Math.abs(pre.val - root.val);
min = Math.min(diff, min);
}
pre = root;
traverse(root.right);
}
}
110. Balanced Binary Tree
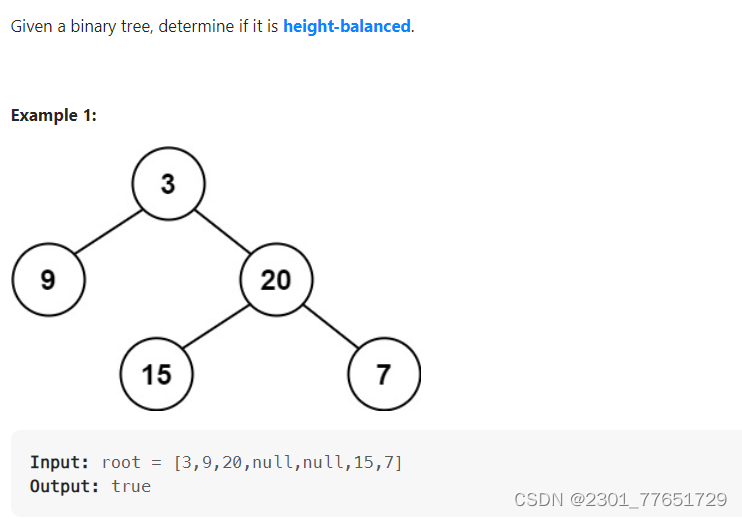
class Solution {
public boolean isBalanced(TreeNode root) {
if(root == null) {
return true;
}
if(Math.abs(help(root.left) - help(root.right)) <= 1) {
return isBalanced(root.left) && isBalanced(root.right);
} else {
return false;
}
}
public int help(TreeNode root) {
if(root == null) {
return 0;
}
return 1 + Math.max(help(root.left), help(root.right));
}
}
108. Convert Sorted Array to Binary Search Tree
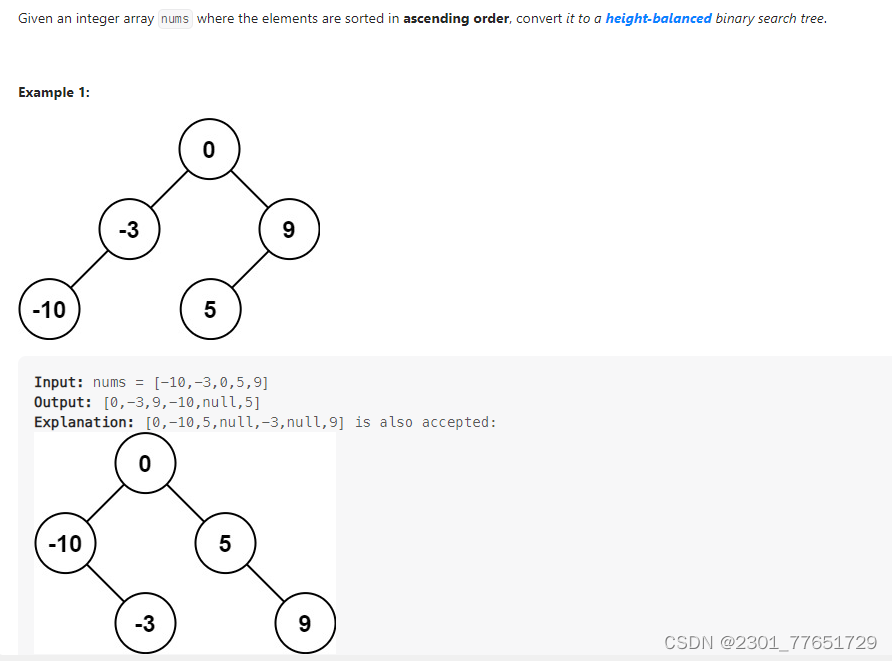
class Solution {
public TreeNode sortedArrayToBST(int[] nums) {
return build(nums, 0, nums.length - 1);
}
public TreeNode build(int[] nums, int left, int right) {
if(left > right) {
return null;
}
int mid = left + (right - left) / 2;
TreeNode root = new TreeNode(nums[mid]);
root.left = build(nums, left, mid - 1);
root.right = build(nums, mid + 1, right);
return root;
}
}
109. Convert Sorted List to Binary Search Tree
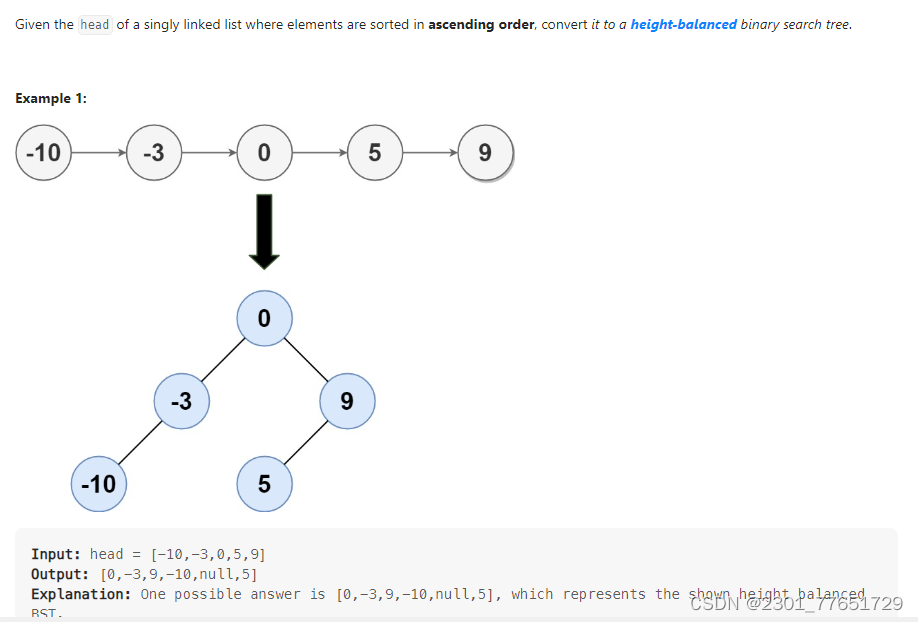
class Solution {
public TreeNode sortedListToBST(ListNode head) {
if(head == null) {
return null;
}
if(head.next == null) {
return new TreeNode(head.val);
}
ListNode fast = head, slow = head, pre = null;
while(fast != null && fast.next != null) {
fast = fast.next.next;
pre = slow;
slow = slow.next;
}
pre.next = null;
ListNode rightList = slow.next;
TreeNode root = new TreeNode(slow.val);
root.left = sortedListToBST(head);
root.right = sortedListToBST(rightList);
return root;
}
}
105. Construct Binary Tree from Preorder and Inorder Traversal
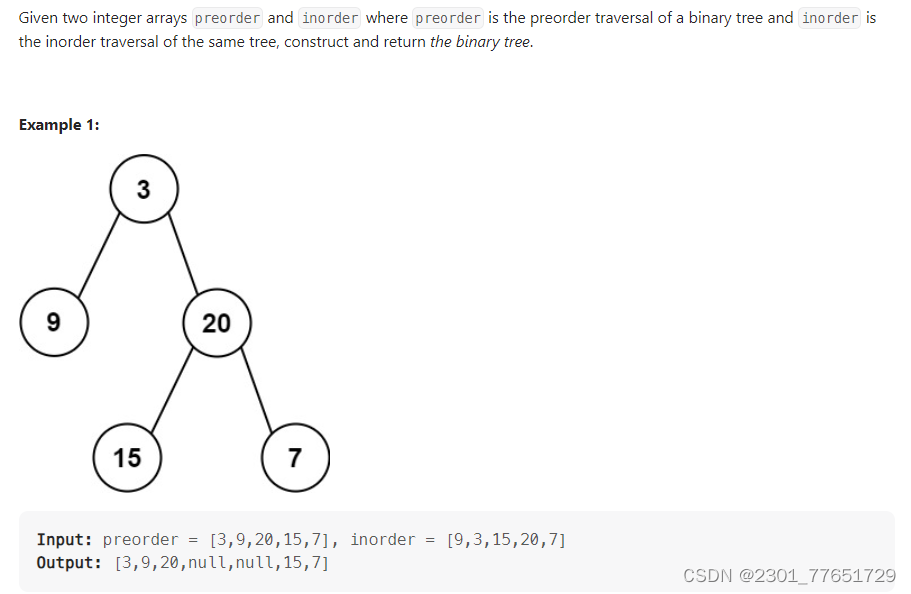
class Solution {
public TreeNode buildTree(int[] preorder, int[] inorder) {
int preLen = preorder.length, inLen = inorder.length;
Map<Integer, Integer> map = new HashMap();
for(int i = 0; i < inLen; i++) {
map.put(inorder[i], i);
}
return builderTree(preorder, 0, preLen - 1, map, 0, inLen - 1);
}
public TreeNode builderTree(int[] preorder, int preLeft, int preRight, Map<Integer, Integer> map, int inLeft, int inRight) {
if(preLeft > preRight || inLeft > inRight) {
return null;
}
int rootval = preorder[preLeft];
TreeNode root = new TreeNode(rootval);
int curIndex = map.get(rootval);
root.left = builderTree(preorder, preLeft + 1, curIndex - inLeft + preLeft, map, inLeft, curIndex - 1);
root.right = builderTree(preorder, curIndex - inLeft + preLeft + 1, preRight, map, curIndex + 1, inRight);
return root;
}
}
106. Construct Binary Tree from Inorder and Postorder Traversal
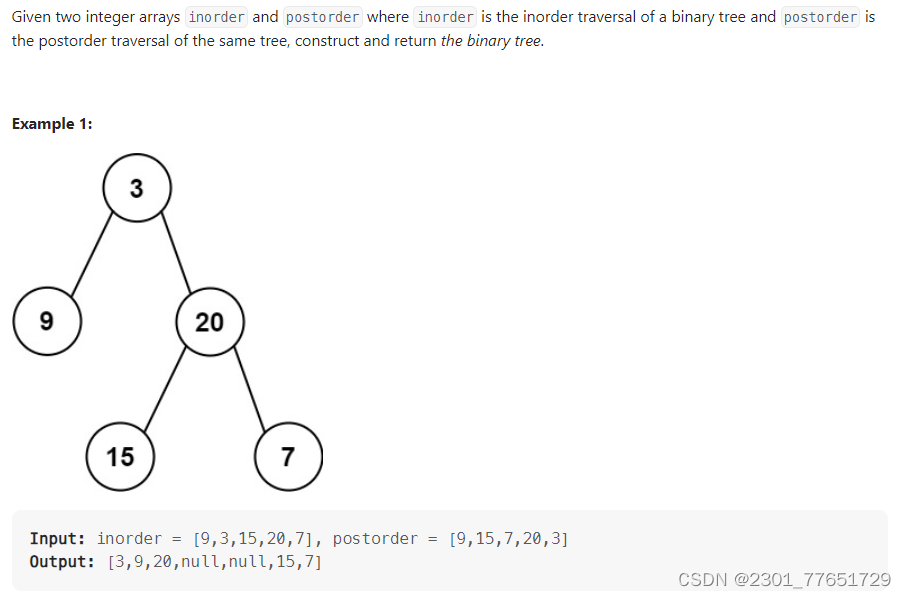
class Solution {
public TreeNode buildTree(int[] inorder, int[] postorder) {
Map<Integer, Integer> map = new HashMap<>();
for (int i = 0; i < inorder.length; i++) {
map.put(inorder[i], i);
}
return helper(map, postorder, 0, inorder.length - 1, 0, postorder.length - 1);
}
private TreeNode helper(Map<Integer, Integer> map, int[] postorder, int inLeft, int inRight, int poLeft, int poRight) {
if (inLeft > inRight) {
return null;
}
TreeNode root = new TreeNode(postorder[poRight]);
int inMid = map.get(root.val);
root.left = helper(map, postorder, inLeft, inMid - 1, poLeft, poLeft + inMid - inLeft - 1);
root.right = helper(map, postorder, inMid + 1, inRight, poRight - inRight + inMid, poRight - 1);
return root;
}
}
114. Flatten Binary Tree to Linked List
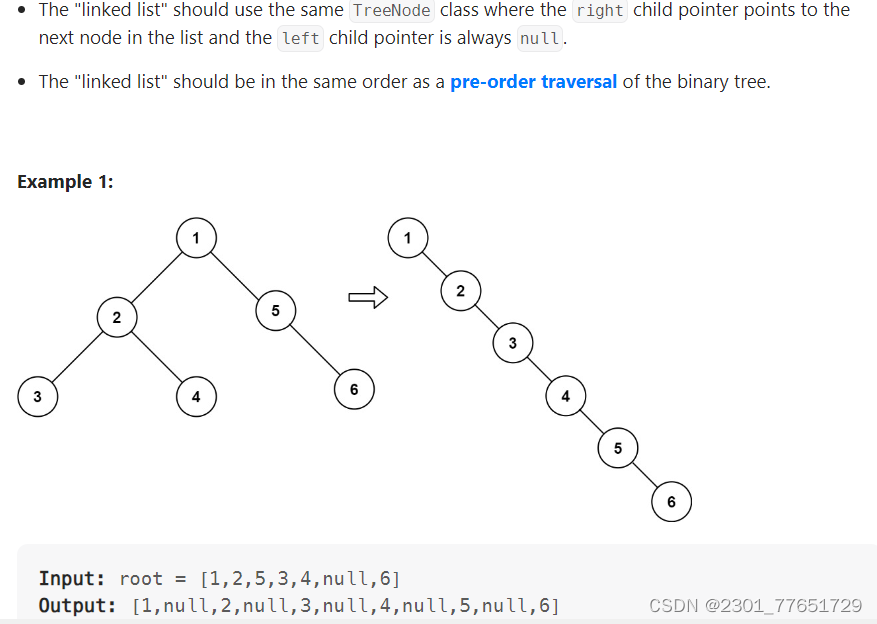
class Solution {
public void flatten(TreeNode root) {
if(root == null) return;
root = help(root);
}
public TreeNode help(TreeNode root) {
if(root == null) return null;
TreeNode l = help(root.left);
TreeNode r = help(root.right);
if(l == null) {
root.right = r;
} else {
root.right = l;
TreeNode cur = l;
while(cur.right != null) {
cur = cur.right;
}
cur.right = r;
root.left = null;
}
return root;
}
}
class Solution {
public void flatten(TreeNode root) {
if(root == null) return;
flatten(root.left);
flatten(root.right);
TreeNode temp = root.right;
root.right = root.left;
root.left = null;
while(root.right != null ) {
root = root.right;
}
root.right = temp;
}
}
235. Lowest Common Ancestor of a Binary Search Tree
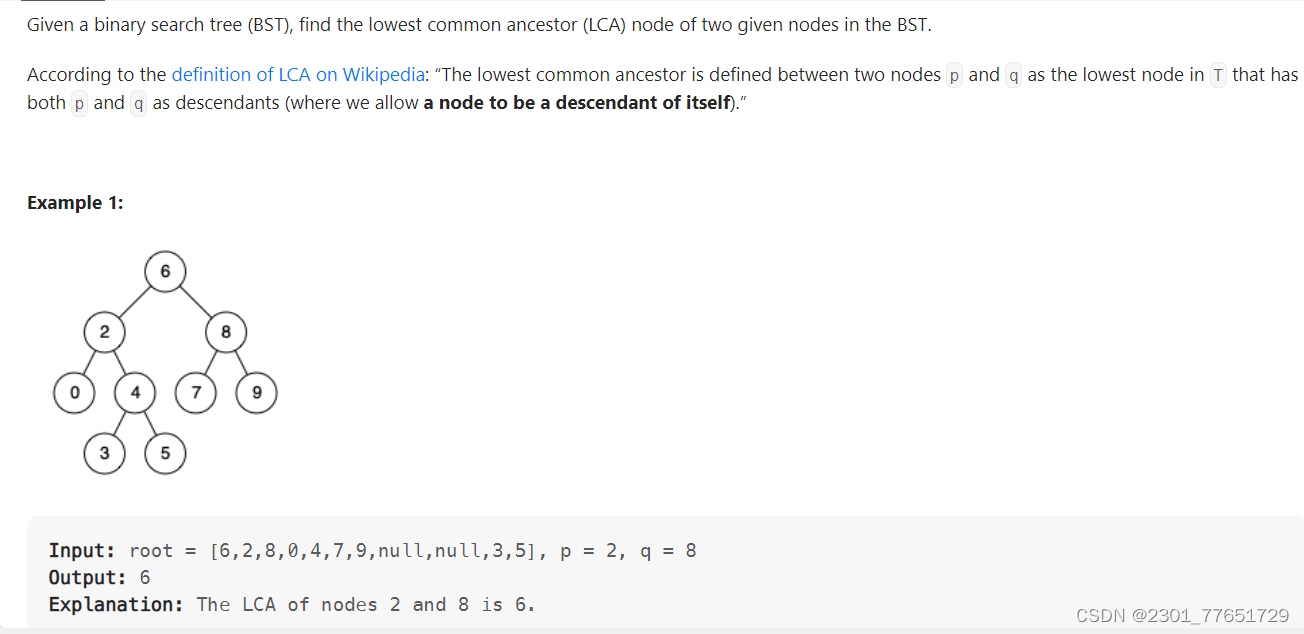
class Solution {
public TreeNode lowestCommonAncestor(TreeNode root, TreeNode p, TreeNode q) {
if(p.val == q.val) return p;
if(p.val == root.val || q.val == root.val) return root;
if(p.val < root.val && q.val < root.val) {
return lowestCommonAncestor(root.left, p, q);
} else if(p.val > root.val && q.val > root.val) {
return lowestCommonAncestor(root.right, p, q);
}
return root;
}
}
236. Lowest Common Ancestor of a Binary Tree
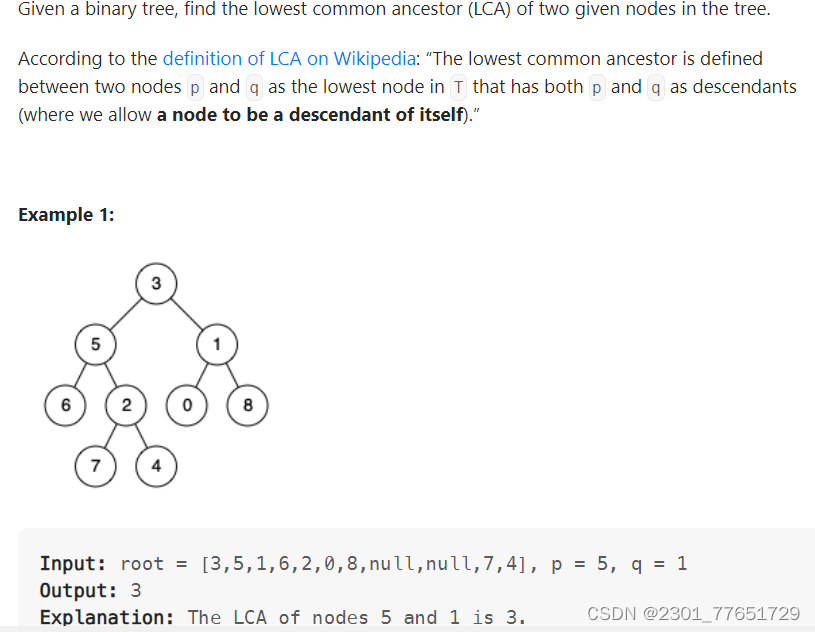
class Solution {
public TreeNode lowestCommonAncestor(TreeNode root, TreeNode p, TreeNode q) {
if(root == null) return null;
if(root.val == p.val || root.val == q.val) {
return root;
}
TreeNode left = lowestCommonAncestor(root.left, p, q);
TreeNode right = lowestCommonAncestor(root.right, p, q);
if(left != null && right != null) {
return root;
} else if(left != null) {
return left;
} else {
return right;
}
}
}
501. Find Mode in Binary Search Tree
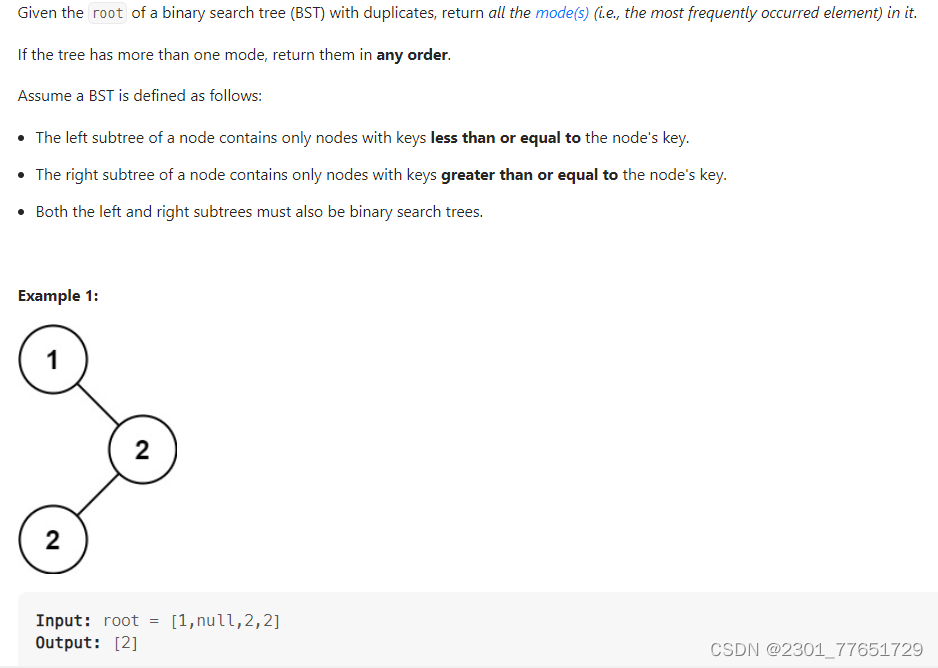
class Solution {
List<Integer> list = new ArrayList();
int count = 0;
int maxCount = Integer.MIN_VALUE;
TreeNode pre = null;
public int[] findMode(TreeNode root) {
dfs(root);
int size = list.size();
int[] res = new int[size];
for(int i = 0; i < size; i++) {
res[i] = list.get(i);
}
return res;
}
public void dfs(TreeNode root) {
if(root == null) return;
dfs(root.left);
if(pre != null && pre.val == root.val) {
count++;
} else {
count = 1;
}
if(count == maxCount) {
list.add(root.val);
} else if(count > maxCount) {
maxCount = count;
list.clear();
list.add(root.val);
}
pre = root;
dfs(root.right);
}
}
99. Recover Binary Search Tree
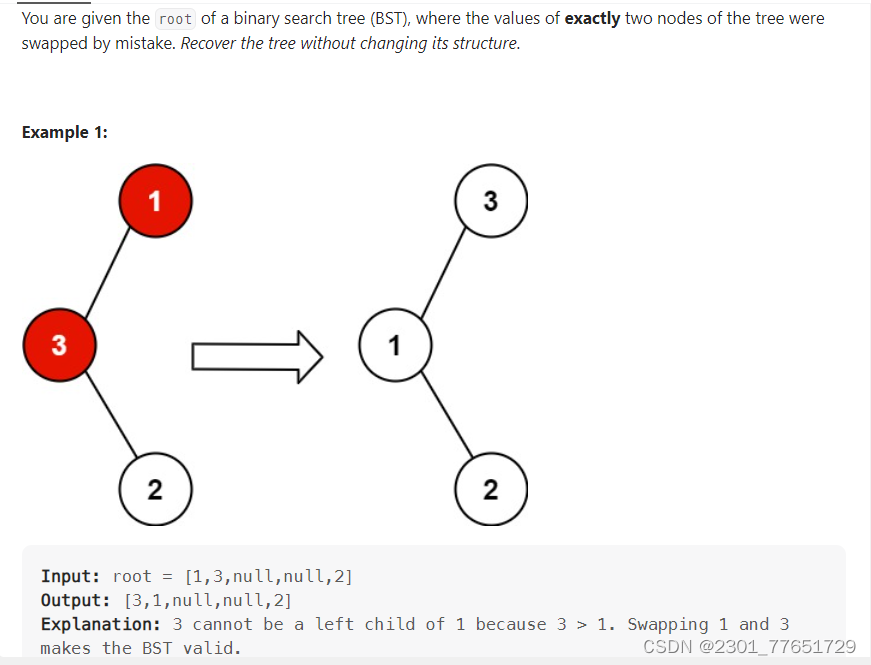
class Solution {
TreeNode one, two, pre;
public void recoverTree(TreeNode root) {
inorder(root);
int temp = one.val;
one.val = two.val;
two.val = temp;
}
public void inorder(TreeNode root) {
if(root == null) return;
inorder(root.left);
if(pre != null && pre.val > root.val) {
if(one == null) {
one = pre;
}
two = root;
}
pre = root;
inorder(root.right);
}
}