958. Check Completeness of a Binary Tree
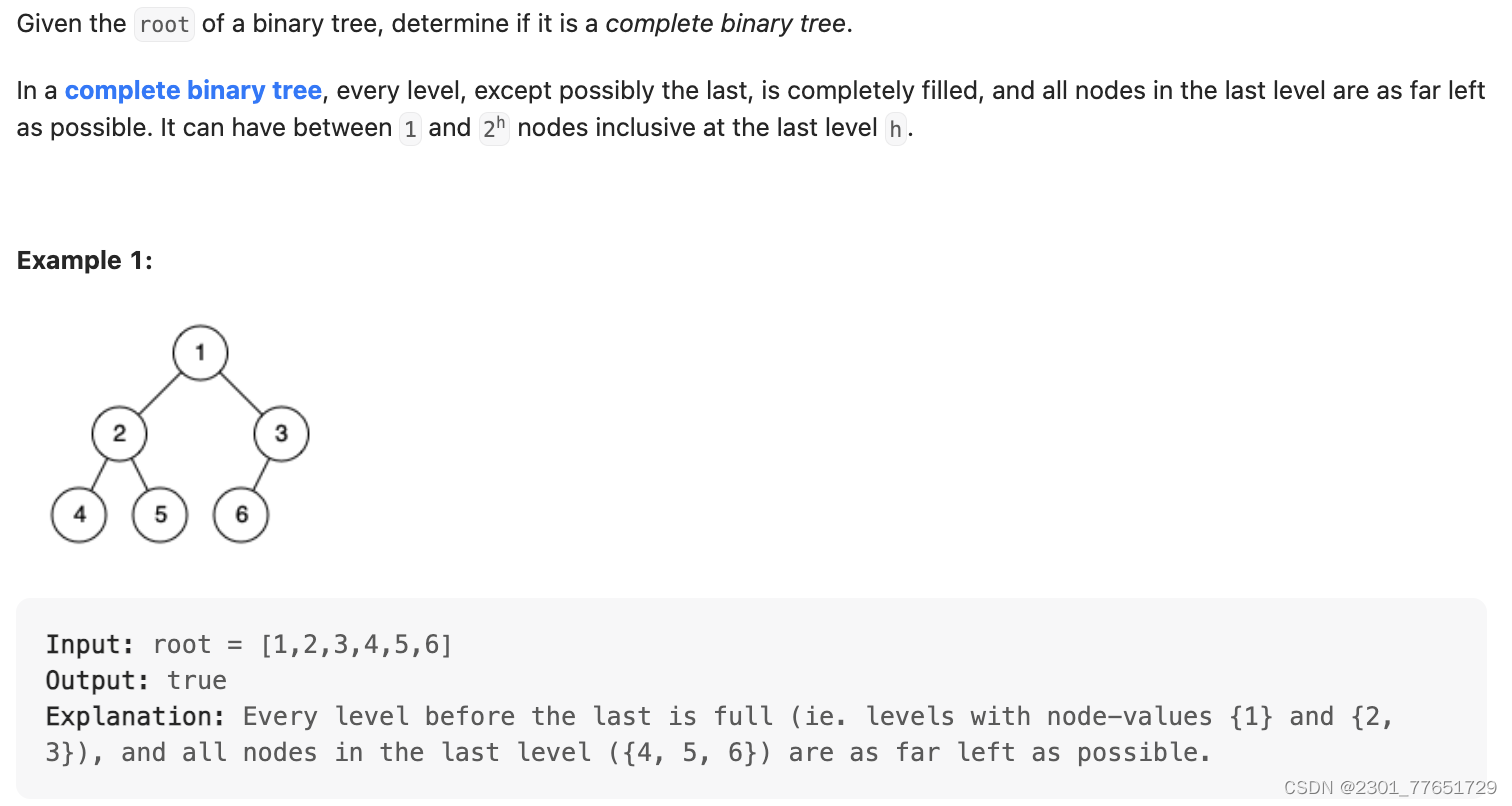
class Solution {
public boolean isCompleteTree(TreeNode root) {
if(root == null) return true;
Queue<TreeNode> q = new LinkedList();
q.offer(root);
while(q.peek() != null) {
TreeNode cur = q.poll();
q.offer(cur.left);
q.offer(cur.right);
}
while(q.peek() == null && !q.isEmpty())
q.poll();
return q.isEmpty();
}
}
965. Univalued Binary Tree
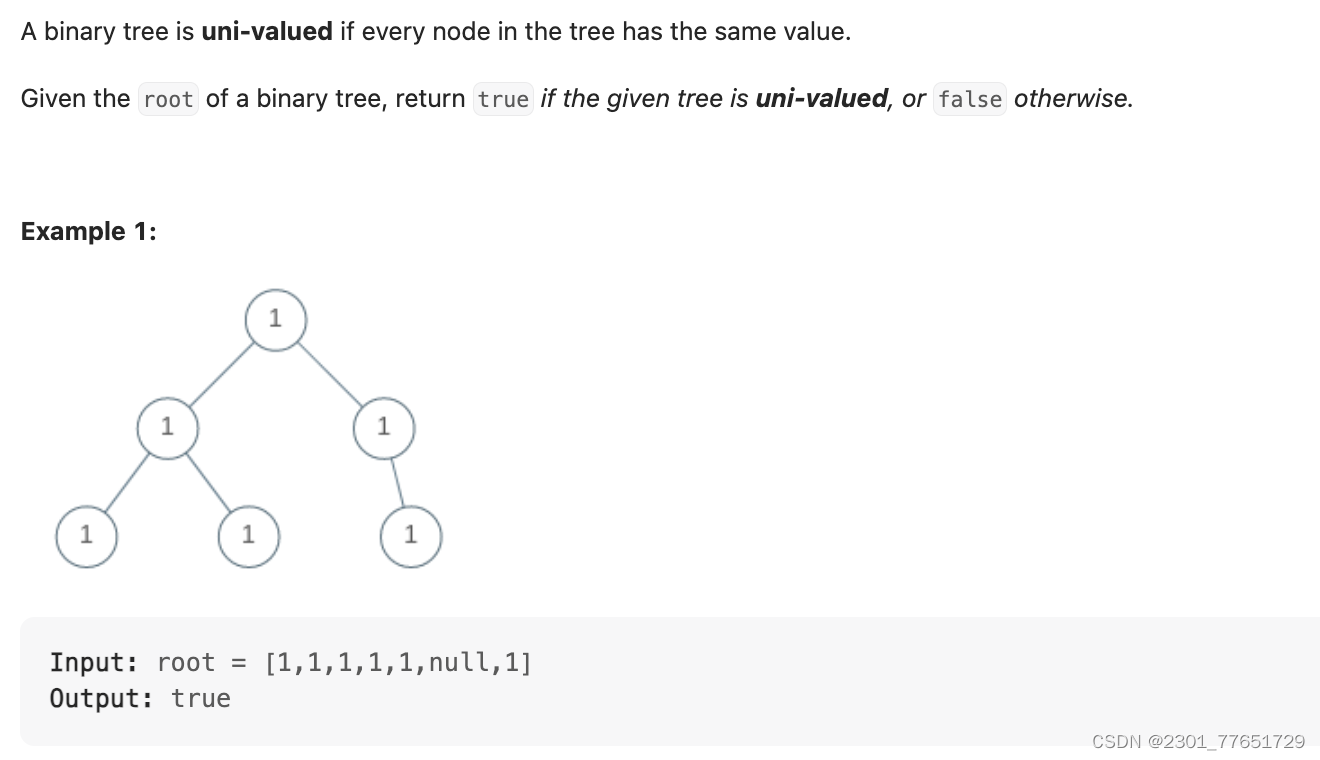
class Solution {
public boolean isUnivalTree(TreeNode root) {
if(root == null) return true;
if(root.left != null && root.left.val != root.val) return false;
if(root.right != null && root.right.val != root.val) return false;
return isUnivalTree(root.left) && isUnivalTree(root.right);
}
}
967. Numbers With Same Consecutive Differences✖️
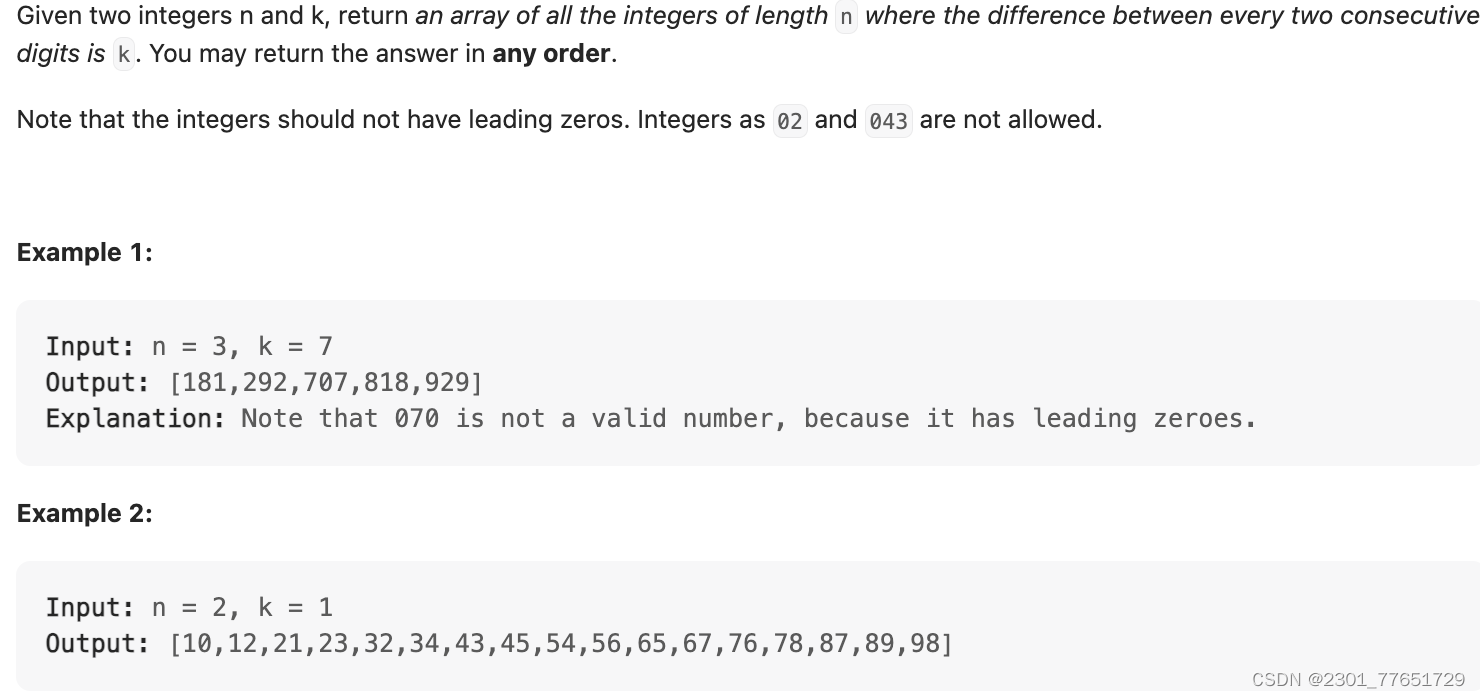
class Solution {
public int[] numsSameConsecDiff(int n, int k) {
List<Integer> cur = Arrays.asList(1, 2, 3, 4, 5, 6, 7, 8, 9);
for(int i = 2; i <= n; i++) {
List<Integer> cur2 = new ArrayList();
for(int x : cur) {
int y = x % 10;
if(y + k < 10) cur2.add(x * 10 + y + k);
if(k > 0 && y - k >= 0) cur2.add(x * 10 + y - k);
}
cur = cur2;
}
return cur.stream().mapToInt(j ->j).toArray();
}
}
993. Cousins in Binary Tree
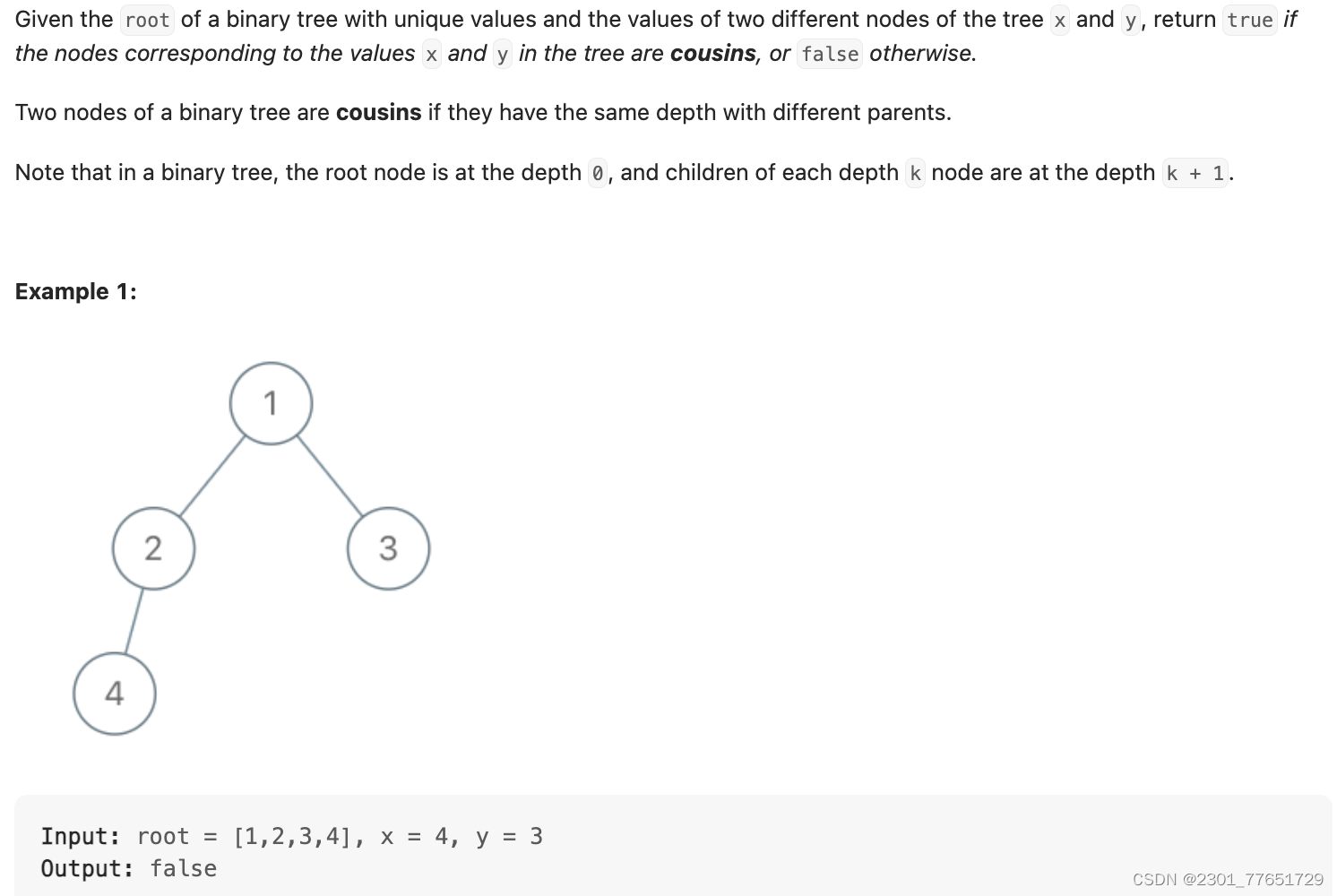
class Solution {
public boolean isCousins(TreeNode root, int x, int y) {
if(root == null) return false;
Queue<TreeNode> q = new LinkedList();
q.add(root);
while(!q.isEmpty()) {
List<Integer> level = new ArrayList();
int size = q.size();
for(int i = 0; i < size; i++) {
TreeNode temp = q.poll();
if(temp.left != null && temp.right != null) {
if(temp.left.val == x && temp.right.val == y) {
return false;
}
if(temp.right.val == x && temp.left.val == y) return false;
}
level.add(temp.val);
if(temp.left != null) q.offer(temp.left);
if(temp.right != null) q.offer(temp.right);
}
if(level.contains(x) && level.contains(y)) return true;
}
return false;
}
}
1161. Maximum Level Sum of a Binary Tree
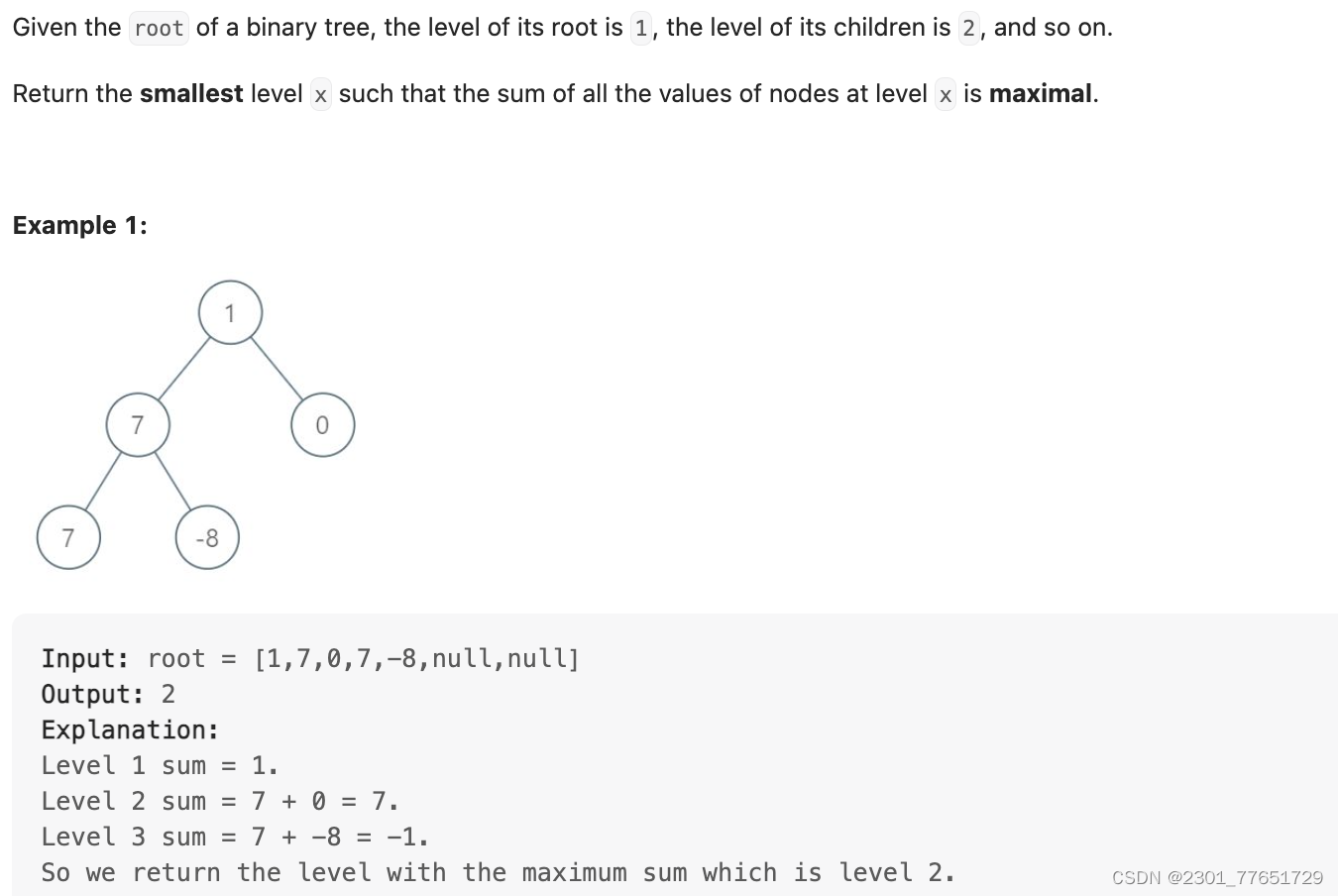
class Solution {
public int maxLevelSum(TreeNode root) {
Queue<TreeNode> q = new LinkedList();
if(root != null) {
q.offer(root);
}
int max = Integer.MIN_VALUE;
int maxlevel = 1;
for(int level = 1; !q.isEmpty(); ++level) {
int size = q.size();
int sum = 0;
for(int i = 0; i < size; i++) {
TreeNode cur = q.poll();
if(cur.left != null) q.offer(cur.left);
if(cur.right != null) q.offer(cur.right);
sum += cur.val;
}
if(max < sum) {
max = sum;
maxlevel = level;
}
}
return maxlevel;
}
}
863. All Nodes Distance K in Binary Tree✖️
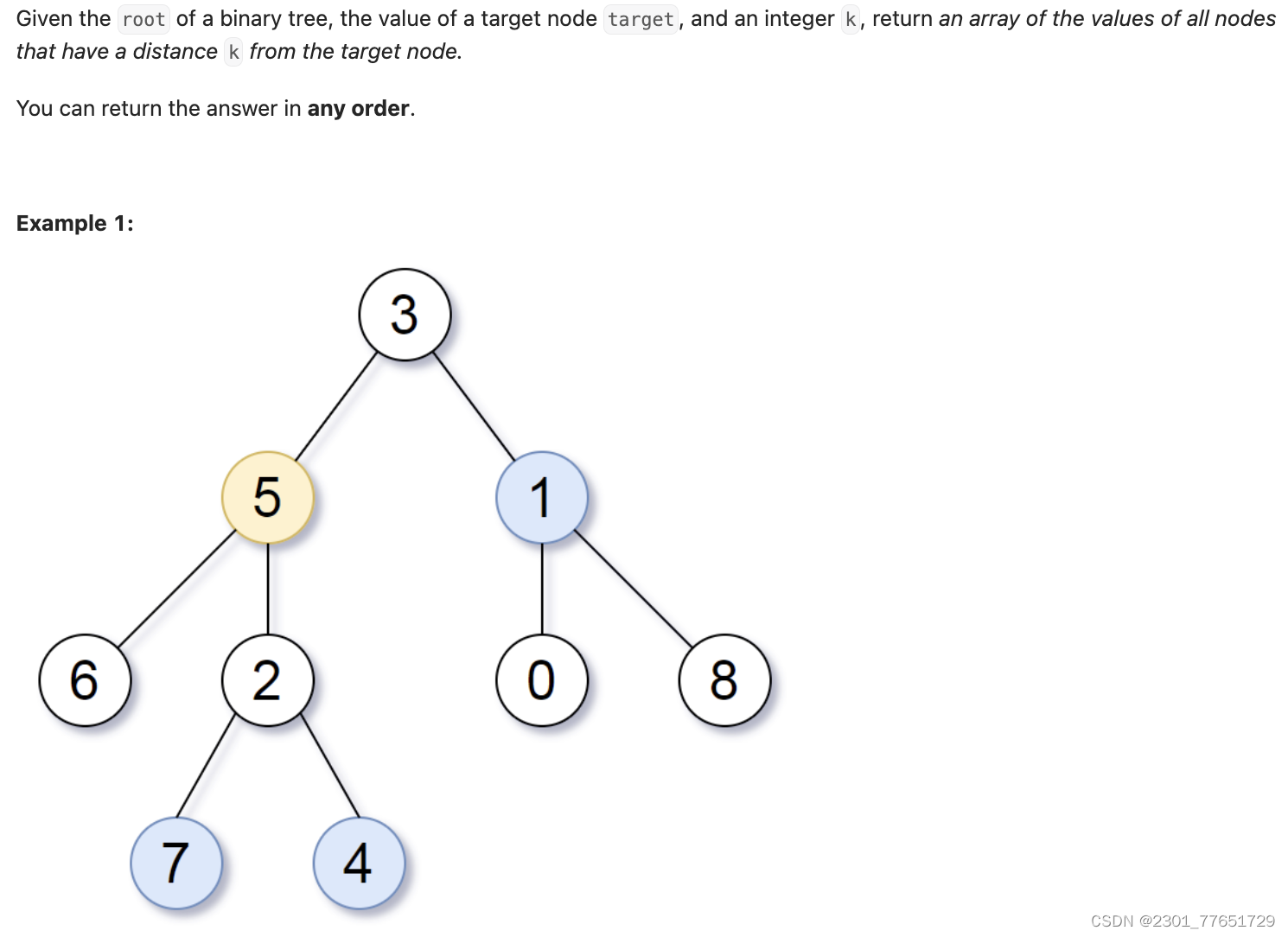
class Solution {
public List<Integer> distanceK(TreeNode root, TreeNode target, int k) {
List<Integer> ans = new ArrayList();
Map<Integer, TreeNode> parent = new HashMap();
Queue<TreeNode> queue = new LinkedList();
queue.offer(root);
while(!queue.isEmpty()) {
int size = queue.size();
for(int i = 0; i < size; i++) {
TreeNode top = queue.poll();
if(top.left != null) {
parent.put(top.left.val, top);
queue.offer(top.left);
}
if(top.right != null) {
parent.put(top.right.val, top);
queue.offer(top.right);
}
}
}
Map<Integer, Integer> vis = new HashMap();
queue.offer(target);
while(k > 0 && !queue.isEmpty()) {
int size = queue.size();
for(int i = 0; i < size; i++) {
TreeNode top = queue.poll();
vis.put(top.val, 1);
if(top.left != null && !vis.containsKey(top.left.val)) {
queue.offer(top.left);
}
if(top.right != null && !vis.containsKey(top.right.val)) {
queue.offer(top.right);
}
if(parent.containsKey(top.val) && !vis.containsKey(parent.get(top.val).val)) {
queue.offer(parent.get(top.val));
}
}
k--;
}
while(!queue.isEmpty()) {
ans.add(queue.poll().val);
}
return ans;
}
}
783. Minimum Distance Between BST Nodes
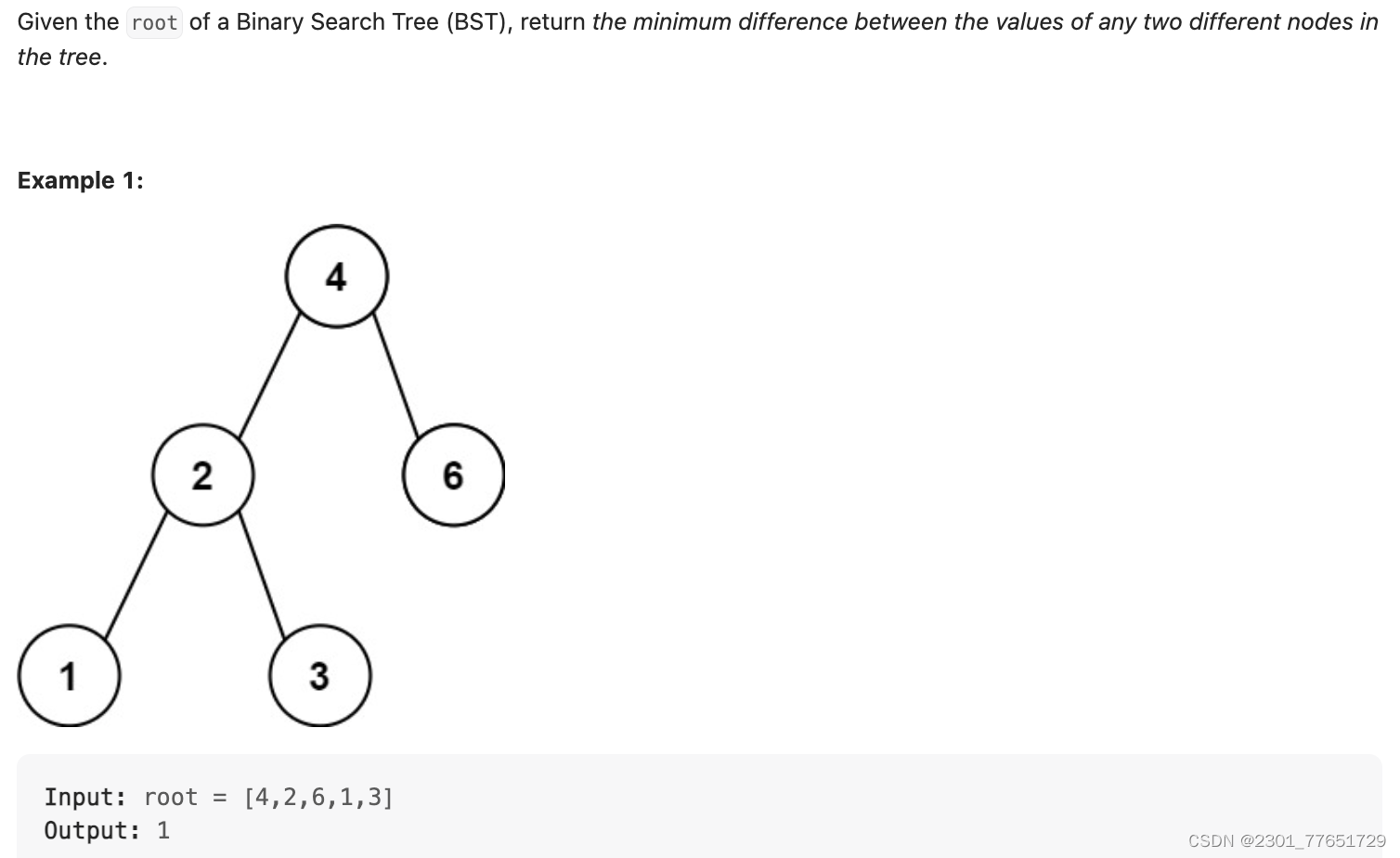
class Solution {
TreeNode pre = null;
int mindiff = Integer.MAX_VALUE;
public int minDiffInBST(TreeNode root) {
if(root == null) return mindiff;
minDiffInBST(root.left);
if(pre != null) mindiff = Math.min(mindiff, root.val - pre.val);
pre = root;
minDiffInBST(root.right);
return mindiff;
}
}
429. N-ary Tree Level Order Traversal
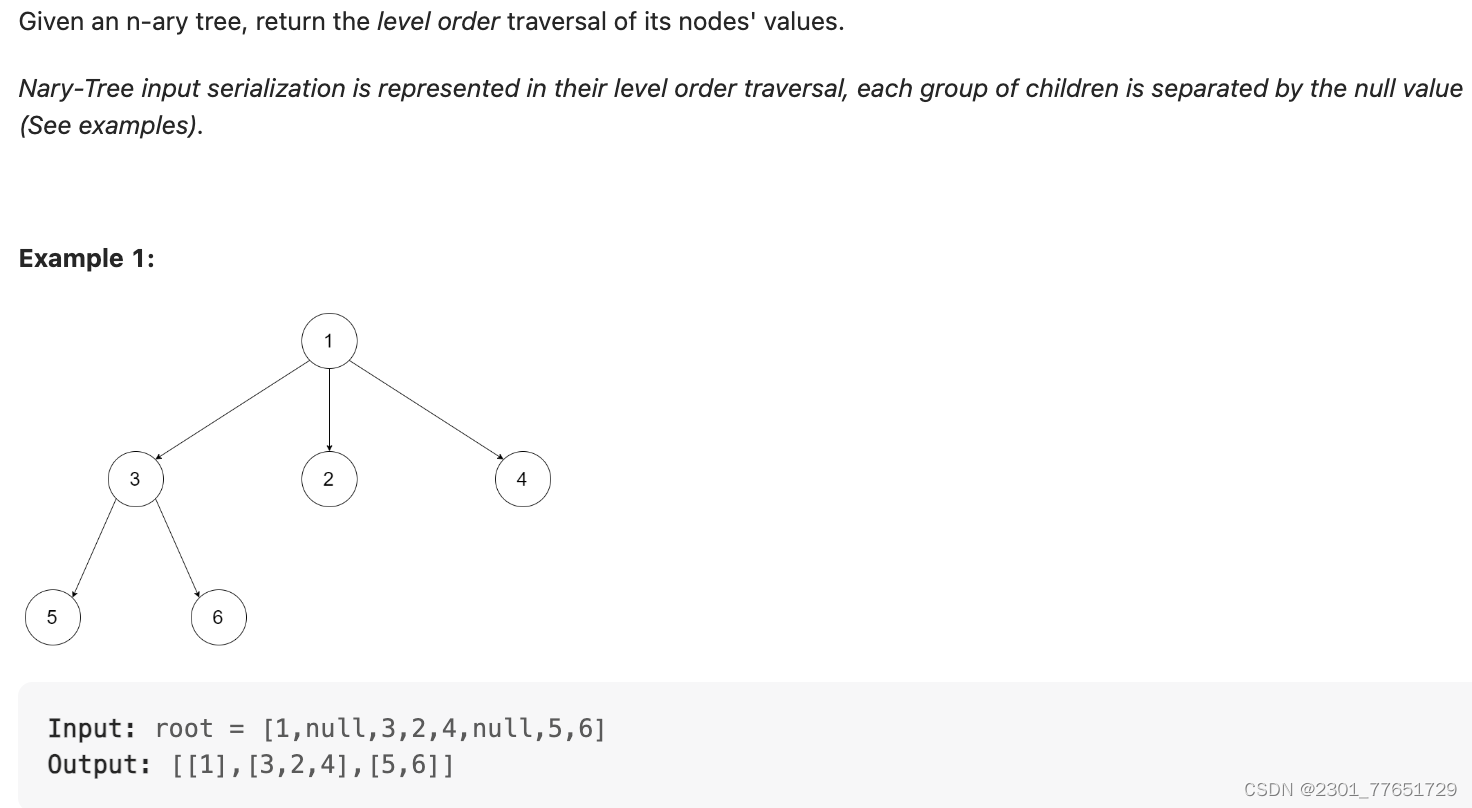
class Solution {
public List<List<Integer>> levelOrder(Node root) {
List<List<Integer>> res = new ArrayList();
if(root == null) return res;
Queue<Node> q = new LinkedList();
q.offer(root);
while(!q.isEmpty()) {
int size = q.size();
List<Integer> list = new ArrayList();
for(int i = 0; i < size; i++) {
list.add(q.peek().val);
for(Node node : q.peek().children) {
q.offer(node);
}
q.poll();
}
res.add(list);
}
return res;
}
}
515. Find Largest Value in Each Tree Row
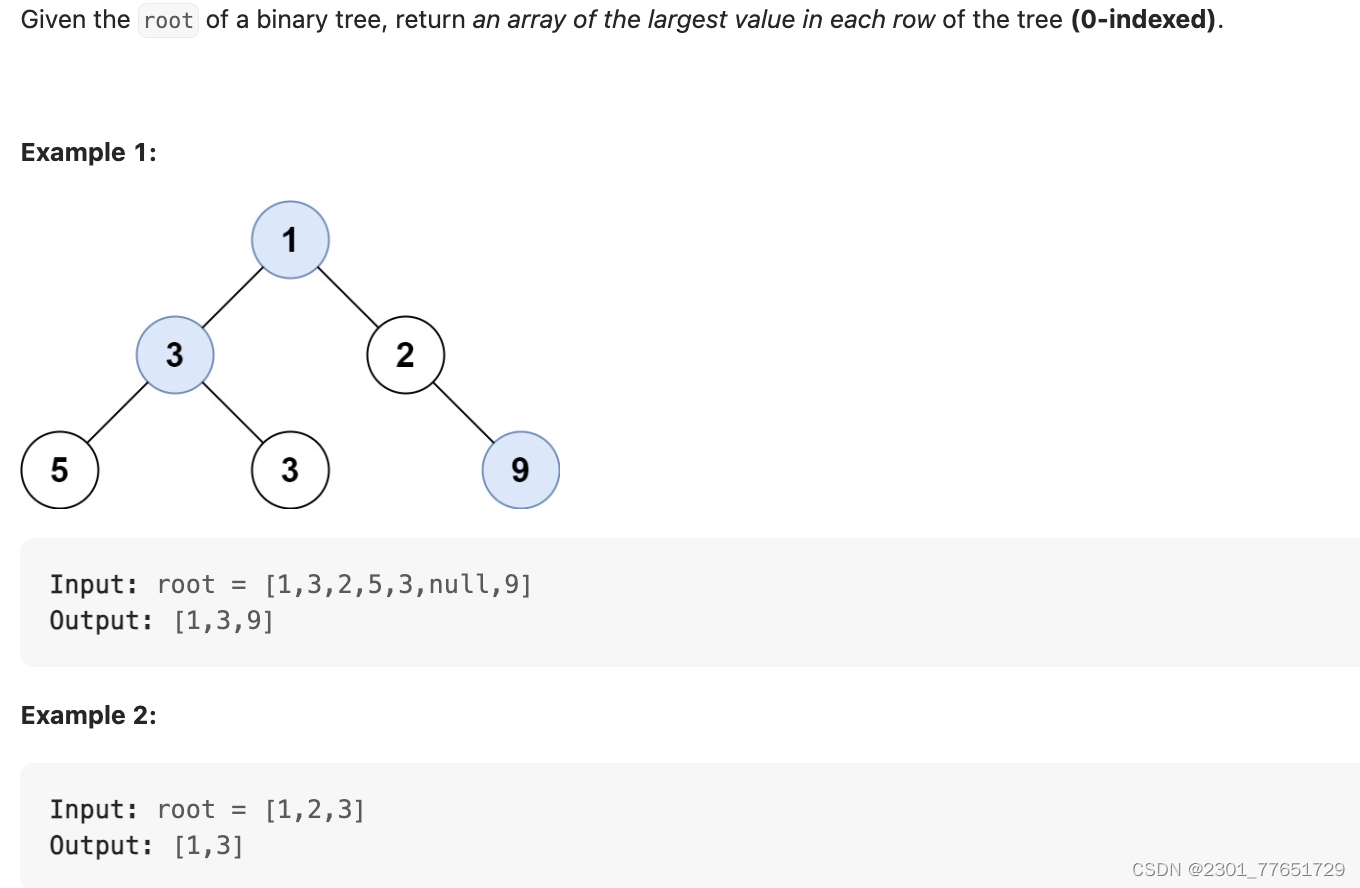
class Solution {
public List<Integer> largestValues(TreeNode root) {
List<Integer> ans = new LinkedList();
if(root == null) {
return ans;
}
Queue<TreeNode> q = new LinkedList();
q.add(root);
while(!q.isEmpty()) {
int size = q.size();
int max = Integer.MIN_VALUE;
for(int i = 0; i < size; i++) {
TreeNode cur = q.poll();
if(cur.left != null) q.offer(cur.left);
if(cur.right != null) q.offer(cur.right);
max = Math.max(max, cur.val);
}
ans.add(max);
}
return ans;
}
}
623. Add One Row to Tree
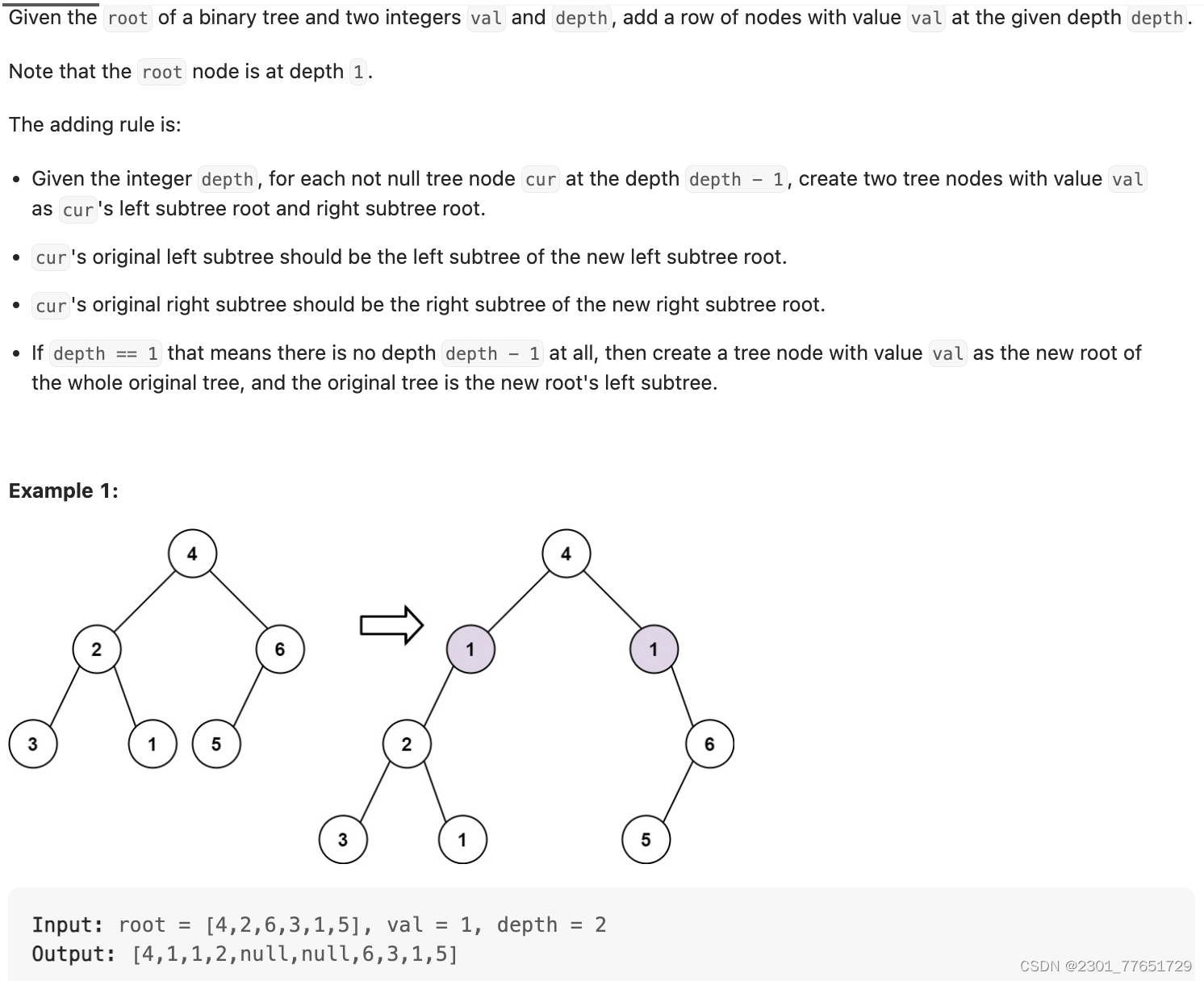
class Solution {
public TreeNode addOneRow(TreeNode root, int v, int d) {
if(d == 1) {
TreeNode new_root = new TreeNode(v);
new_root.left = root;
return new_root;
}
Queue<TreeNode> queue = new LinkedList();
queue.offer(root);
int curDepth = 1;
while(!queue.isEmpty()) {
int size = queue.size();
for(int i = 0; i < size; i++) {
TreeNode cur = queue.poll();
TreeNode left = cur.left;
TreeNode right = cur.right;
if(curDepth == d - 1) {
cur.left = new TreeNode(v);
cur.left.left = left;
cur.right = new TreeNode(v);
cur.right.right = right;
} else {
if(left != null) {
queue.offer(left);
}
if(right != null) {
queue.offer(right);
}
}
}
curDepth++;
}
return root;
}
}
662. Maximum Width of Binary Tree ✖️
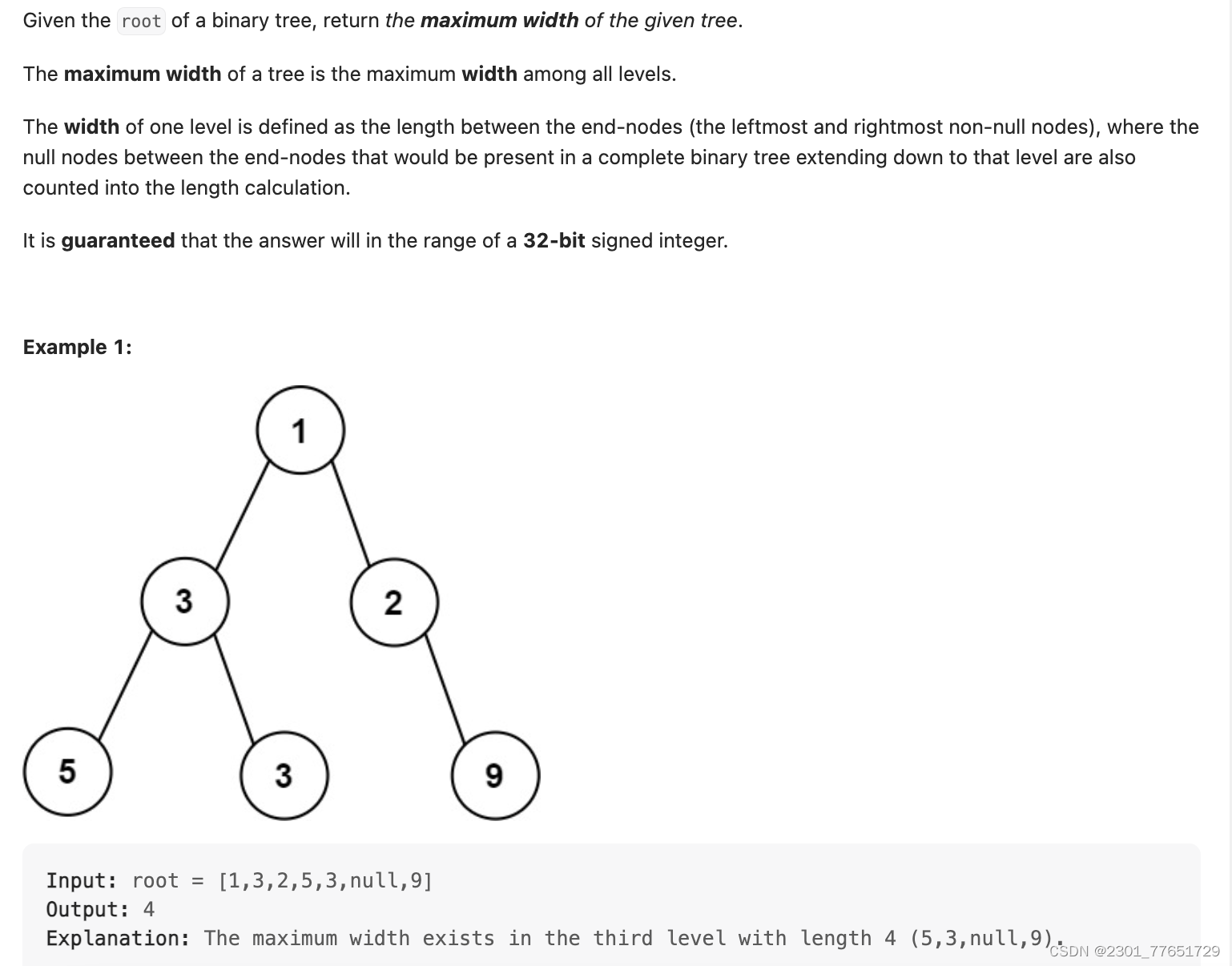
class Solution {
class Node {
TreeNode node;
int idx;
Node(TreeNode node, int idx){
this.node = node;
this.idx = idx;
}
}
public int widthOfBinaryTree(TreeNode root) {
Queue<Node> queue = new LinkedList<>();
queue.add(new Node(root,0));
int max = 0;
while(!queue.isEmpty())
{
int size = queue.size();
int start = 0, end = 0;
for(int i=0; i<size; i++)
{
Node eachNode = queue.remove();
int index = eachNode.idx;
if(i==0)
start = index; //start and end index for each level
if(i==size-1)
end = index;
if(eachNode.node.left!=null)
queue.add(new Node(eachNode.node.left, 2*eachNode.idx));
if(eachNode.node.right!=null)
queue.add(new Node(eachNode.node.right, 2*eachNode.idx+1));
}
max = Math.max(max, end - start + 1);
}
return max;
}
}
655. Print Binary Tree✖️
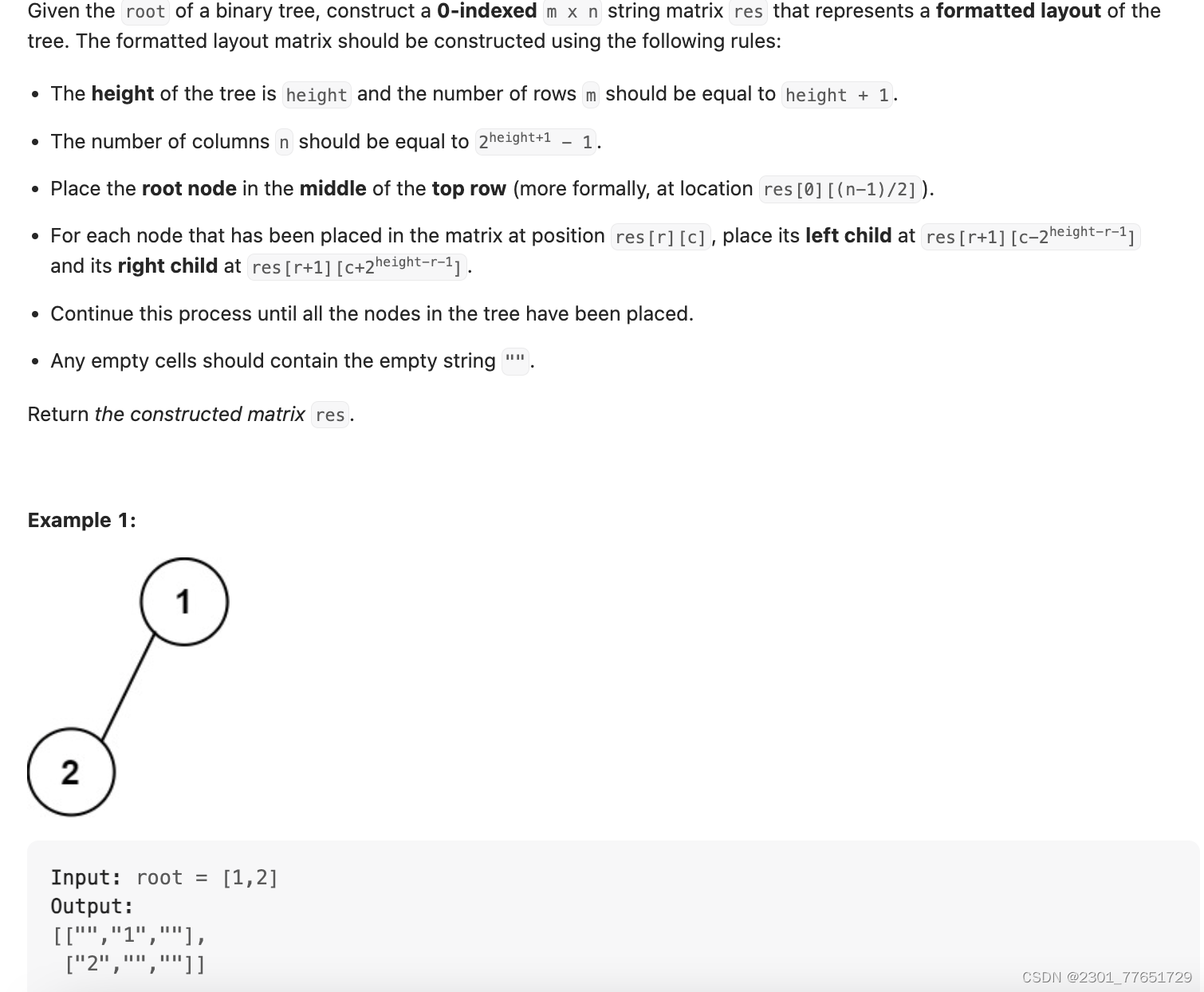
class Solution {
public List<List<String>> printTree(TreeNode root) {
List<List<String>> res = new ArrayList();
int height = getHeight(root);
int row = height + 1;
int column = (int) Math.pow(2, height+1) - 1;
for(int k=0; k<row; k++){
List<String> list = new ArrayList();
for(int i=0; i<column; i++){
list.add("");
}
res.add(list);
}
int left = 0;
int right = column-1;
int level=0;
print(res, left, right, level, root);
return res;
}
public void print(List<List<String>> res, int left, int right, int level, TreeNode root){
if(root == null) return;
int mid = left+(right-left)/2;
res.get(level).set(mid, String.valueOf(root.val));
print(res, left, mid-1, level+1, root.left);
print(res, mid+1, right, level+1, root.right);
}
public int getHeight(TreeNode root){
if (root==null) return -1;
int left = getHeight(root.left);
int right = getHeight(root.right);
return Math.max(left, right)+1;
}
}
322. Coin Change ✖️
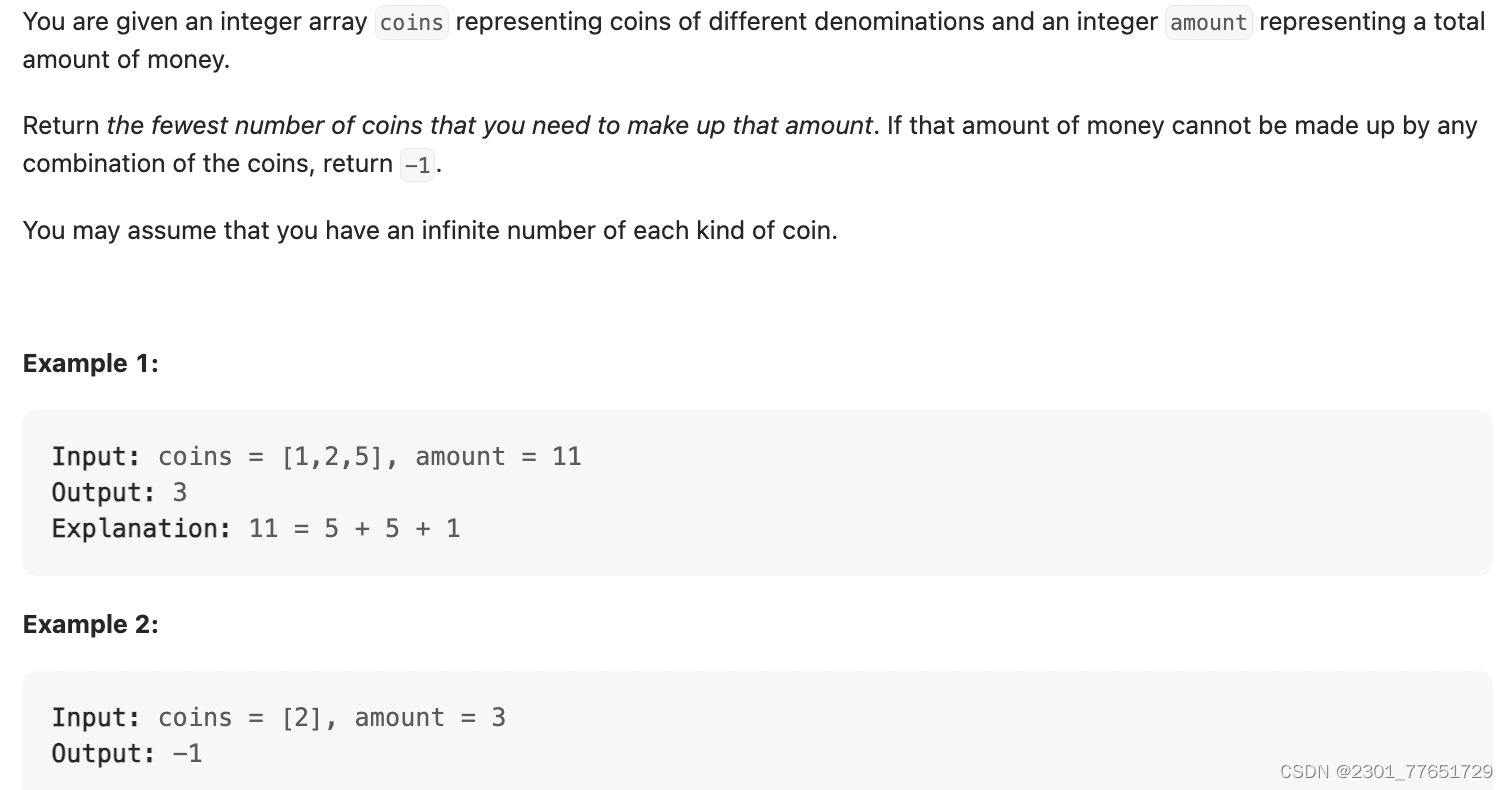
class Solution {
public int coinChange(int[] coins, int amount) {
if(coins == null || amount < 1) return 0;
Deque<Integer> queue = new ArrayDeque();
Set<Integer> visited = new HashSet();
queue.addFirst(amount);
visited.add(amount);
int level = 0;
while(!queue.isEmpty()) {
int size = queue.size();
while(size-- > 0) {
int cur = queue.removeLast();
if(cur == 0) return level;
if(cur < 0) continue;
for(int coin : coins) {
int next = cur - coin;
if(next >=0 && !visited.contains(next)) {
queue.addFirst(next);
visited.add(next);
}
}
}
level++;
}
return -1;
}
}
279. Perfect Squares ✖️
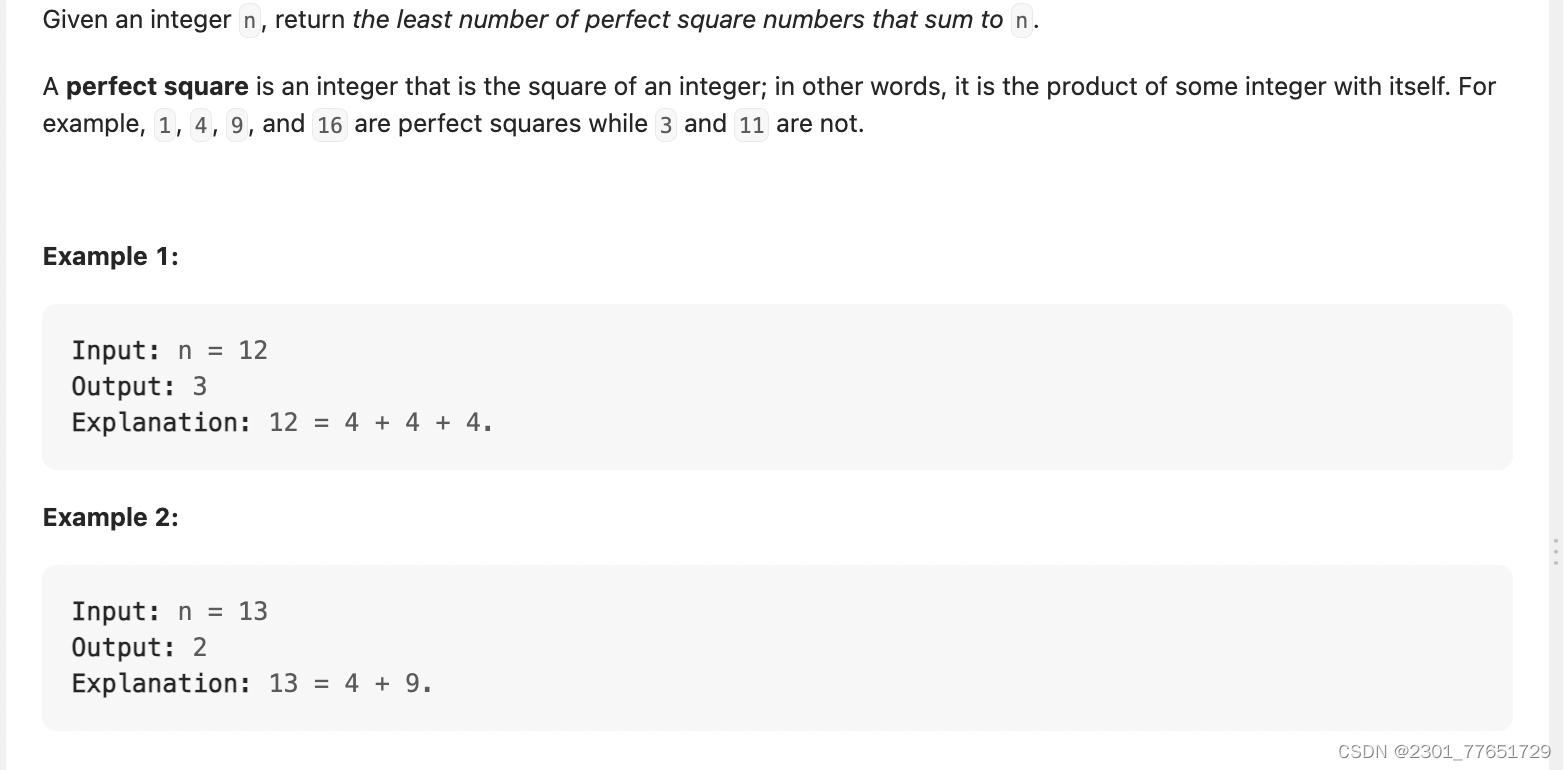
class Solution {
public int numSquares(int n) {
Deque<Integer> queue = new LinkedList();
Set<Integer> set = new HashSet();
if(n > 0) {
queue.offer(n);
}
int num = 0;
while(!queue.isEmpty()) {
num++;
int size = queue.size();
for(int i = 0; i < size; i++) {
int val = queue.poll();
if(!set.add(val)) continue;
for(int j = 1; j <= Math.sqrt(val); j++) {
if(val - (j * j) == 0) return num;
queue.offer(val - (j * j));
}
}
}
return num;
}
}
199. Binary Tree Right Side View
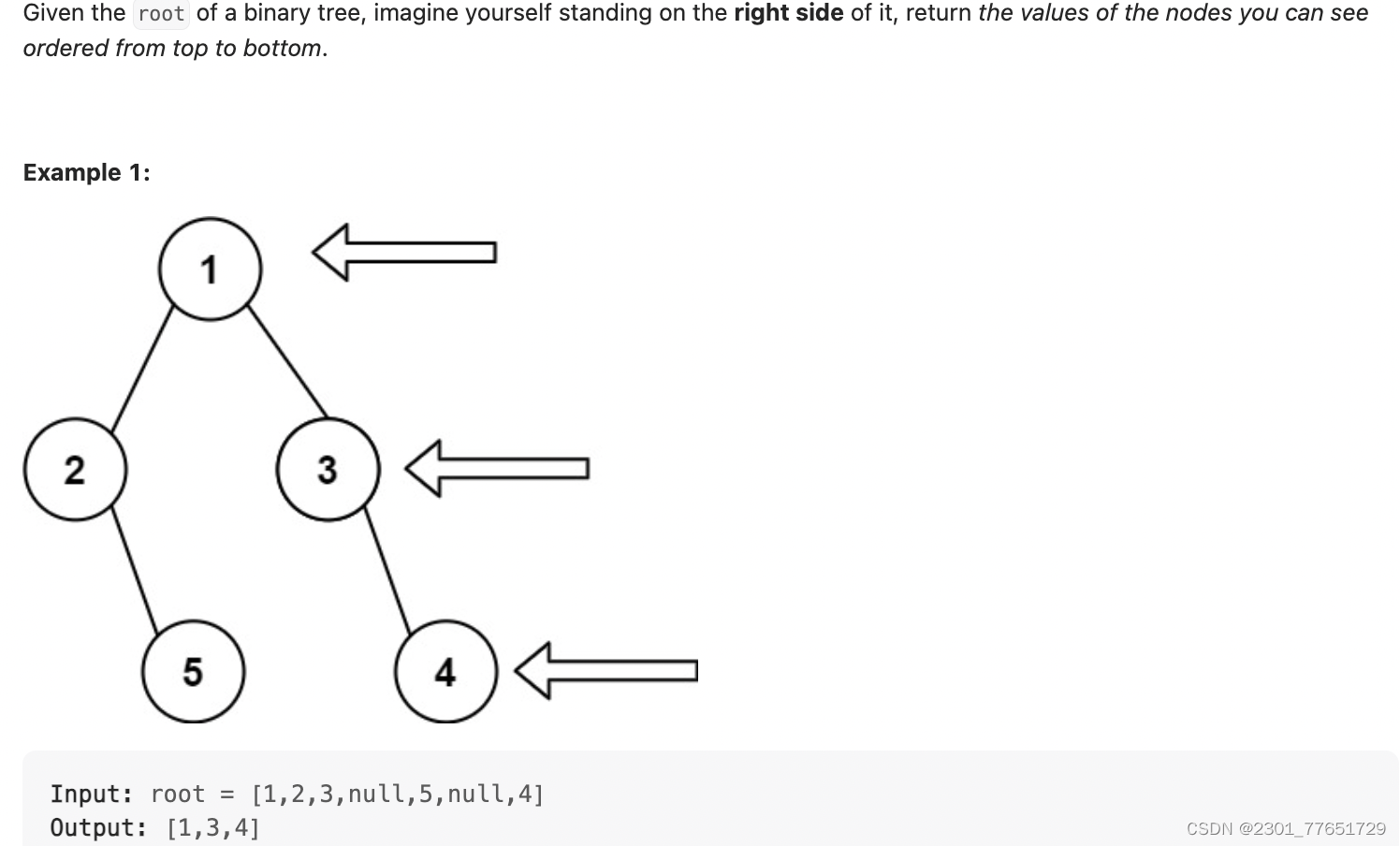
class Solution {
public List<Integer> rightSideView(TreeNode root) {
List<Integer> res = new LinkedList();
Deque<TreeNode> queue = new LinkedList();
if(root == null) return res;
queue.add(root);
while(! queue.isEmpty()) {
int size = queue.size();
TreeNode cur = null;
while(size > 0) {
cur = queue.poll();
if(cur.left != null) {
queue.offer(cur.left);
}
if(cur.right != null) {
queue.offer(cur.right);
}
size--;
}
res.add(cur.val);
}
return res;
}
}
130. Surrounded Regions ✖️
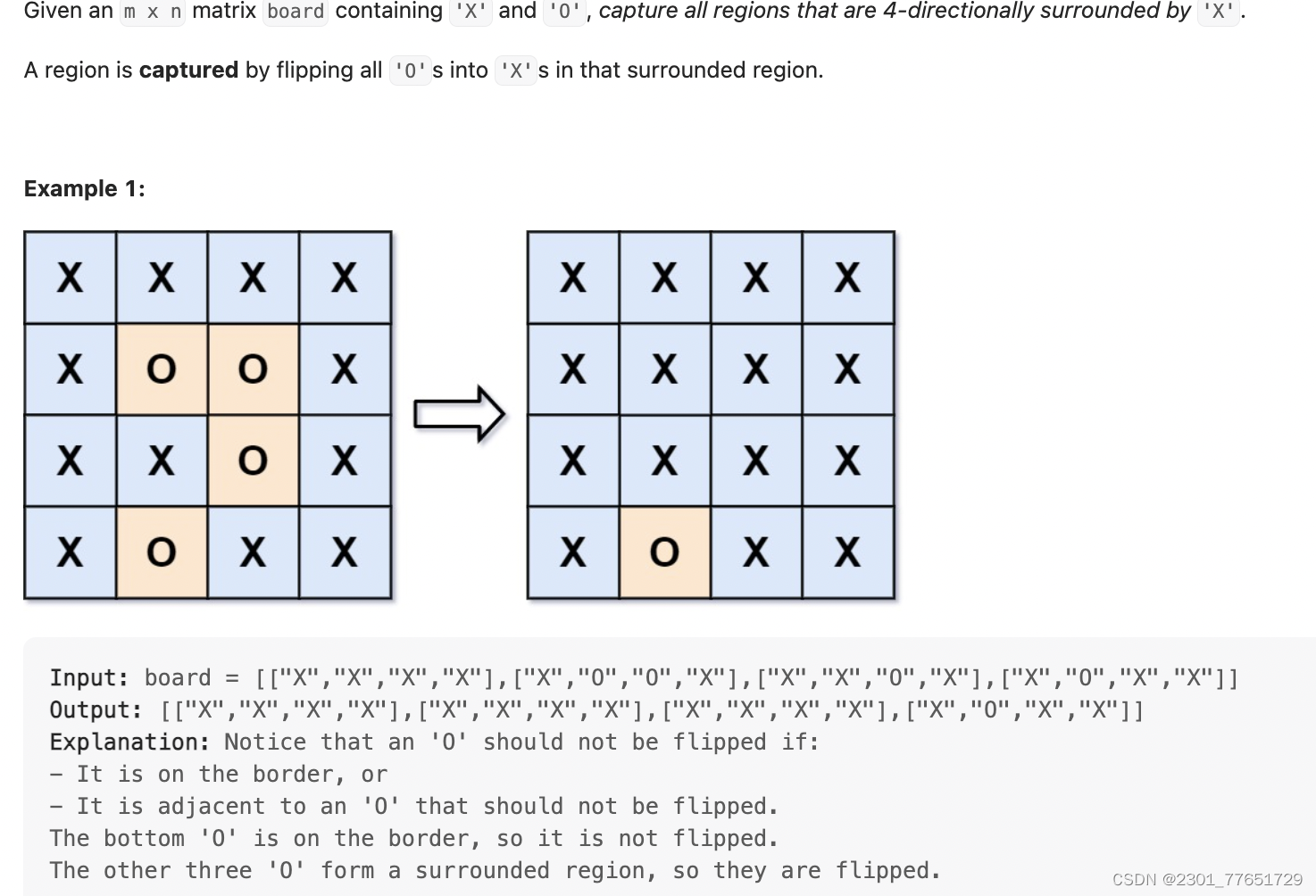
class Solution {
private void DFS(char[][] board,int r,int c,int rsize,int csize){
if(r<0||c<0||r==rsize||c==csize||board[r][c]!='O')return;
board[r][c] = 'P';
DFS(board,r+1,c,rsize,csize);
DFS(board,r,c+1,rsize,csize);
DFS(board,r-1,c,rsize,csize);
DFS(board,r,c-1,rsize,csize);
}
public void solve(char[][] board) {
if(board.length==0)return;
int row = board.length,col = board[0].length;
for(int i=0;i<col;i++){
DFS(board,0,i,row,col);//for FIRST ROW
DFS(board,row-1,i,row,col);//for LAST ROW
}
for(int i=0;i<row;i++){
DFS(board,i,0,row,col);//for FIRST COLUMN
DFS(board,i,col-1,row,col);//for LAST COLUMN
}
for(int i=0;i<row;i++)
for(int j=0;j<col;j++)
if(board[i][j]=='O')board[i][j]='X';
else if(board[i][j]=='P')board[i][j]='O';
}
}
129. Sum Root to Leaf Numbers
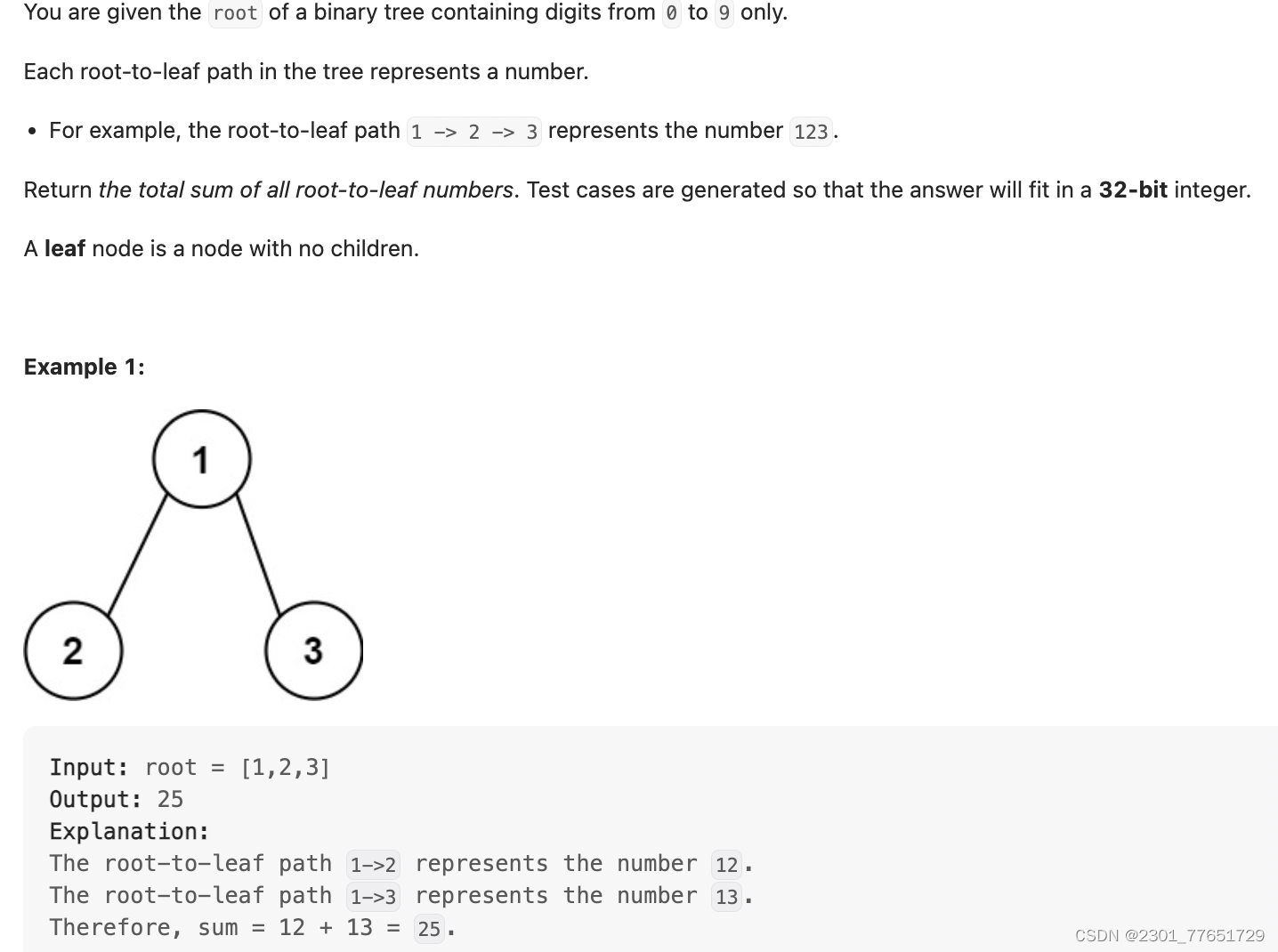
class Solution {
int sum = 0;
public int sumNumbers(TreeNode root) {
helper(root, "");
return sum;
}
void helper(TreeNode root, String str) {
if(root == null) return;
str += root.val;
if(root.left == null && root.right == null) {
sum += Integer.parseInt(str);
return;
}
helper(root.left, str);
helper(root.right, str);
}
}
class Solution {
public int sumNumbers(TreeNode root) {
if(root == null) return 0;
return helper(root, 0);
}
public int helper(TreeNode node, int val) {
if(node == null) return 0;
val = val * 10 + node.val;
if(node.left == null && node.right == null) {
return val;
}
return helper(node.left, val) + helper(node.right, val);
}
}
124. Binary Tree Maximum Path Sum
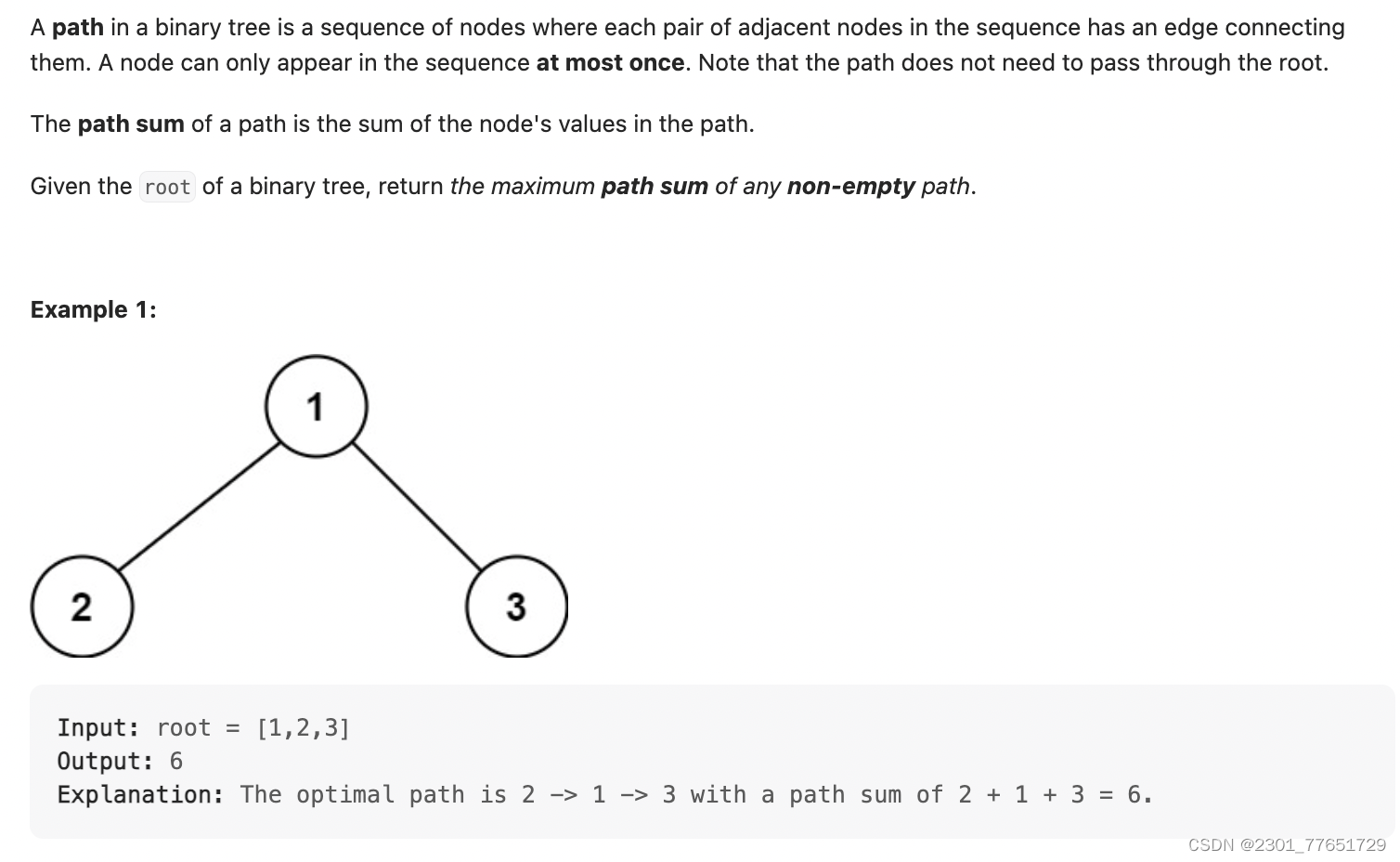
class Solution {
int res = Integer.MIN_VALUE;
public int maxPathSum(TreeNode root) {
helper(root);
return res;
}
public int helper (TreeNode root) {
if(root == null) return 0;
int left = Math.max(helper(root.left), 0);
int right = Math.max(helper(root.right), 0);
res = Math.max(res, root.val + left + right);
return root.val + Math.max(left, right);
}
}
117. Populating Next Right Pointers in Each Node II
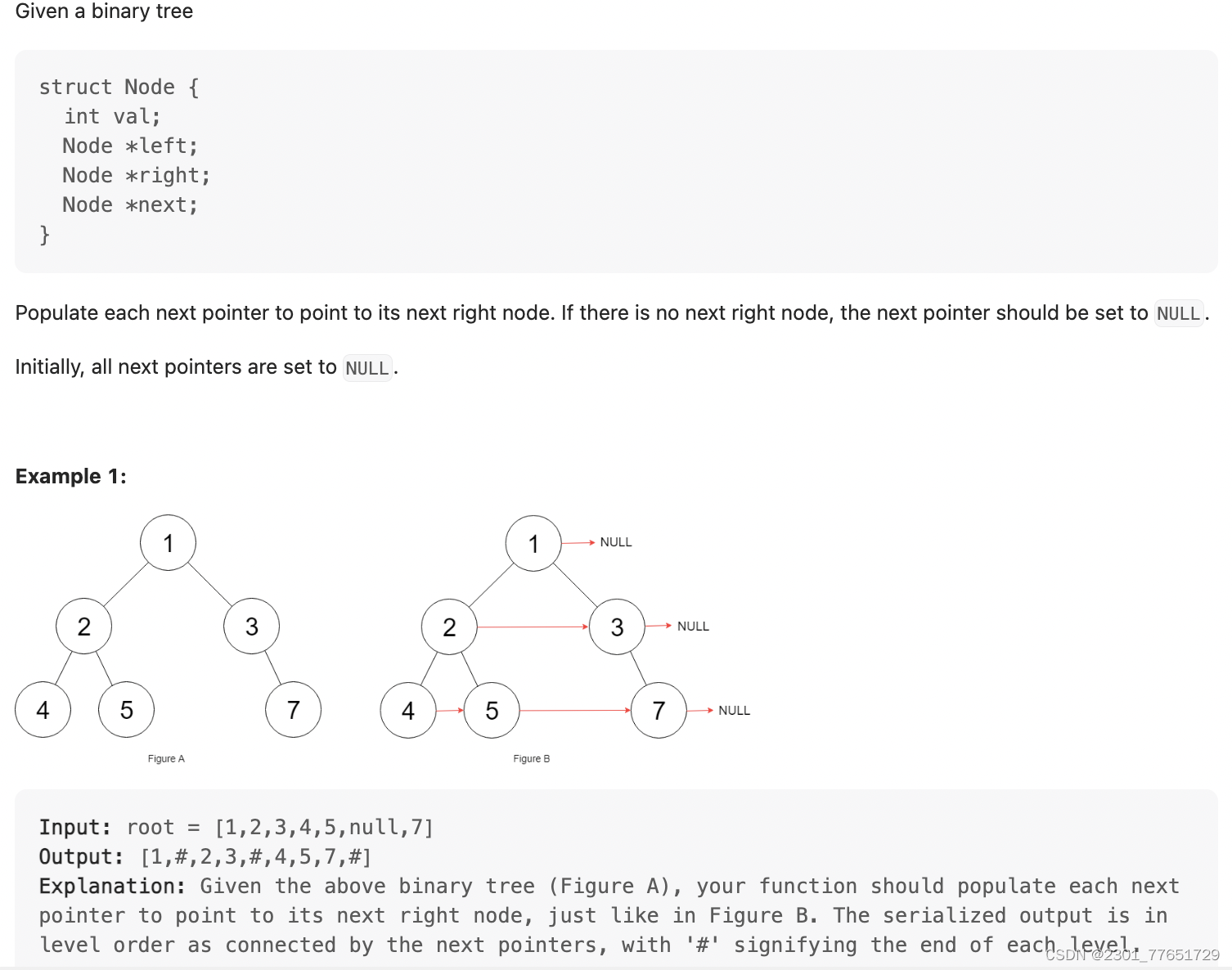
class Solution {
public Node connect(Node root) {
Node fake = new Node(0);
Node pre = fake;
Node root1 = root;
while(root != null) {
if(root.left != null) {
pre.next = root.left;
pre = pre.next;
}
if(root.right != null) {
pre.next = root.right;
pre = pre.next;
}
root = root.next;
if(root == null) {
pre = fake;
root = fake.next;
fake.next = null;
}
}
return root1;
}
}
public Node connect_bfs(Node root) {
if (root == null) {
return root;
}
Queue<Node> queue = new LinkedList<>();
queue.offer(root);
while (!queue.isEmpty()) {
int size = queue.size();
List<Node> levelNodes = new ArrayList<>();
for (int i = 0; i < size; i++) {
Node curNode = queue.poll();
levelNodes.add(curNode);
if (curNode.left != null) {
queue.add(curNode.left);
}
if (curNode.right != null) {
queue.add(curNode.right);
}
}
for (int i = 0; i < levelNodes.size() - 1; i++) {
Node node = levelNodes.get(i);
node.next = levelNodes.get(i + 1);
}
}
return root;
}
116. Populating Next Right Pointers in Each Node
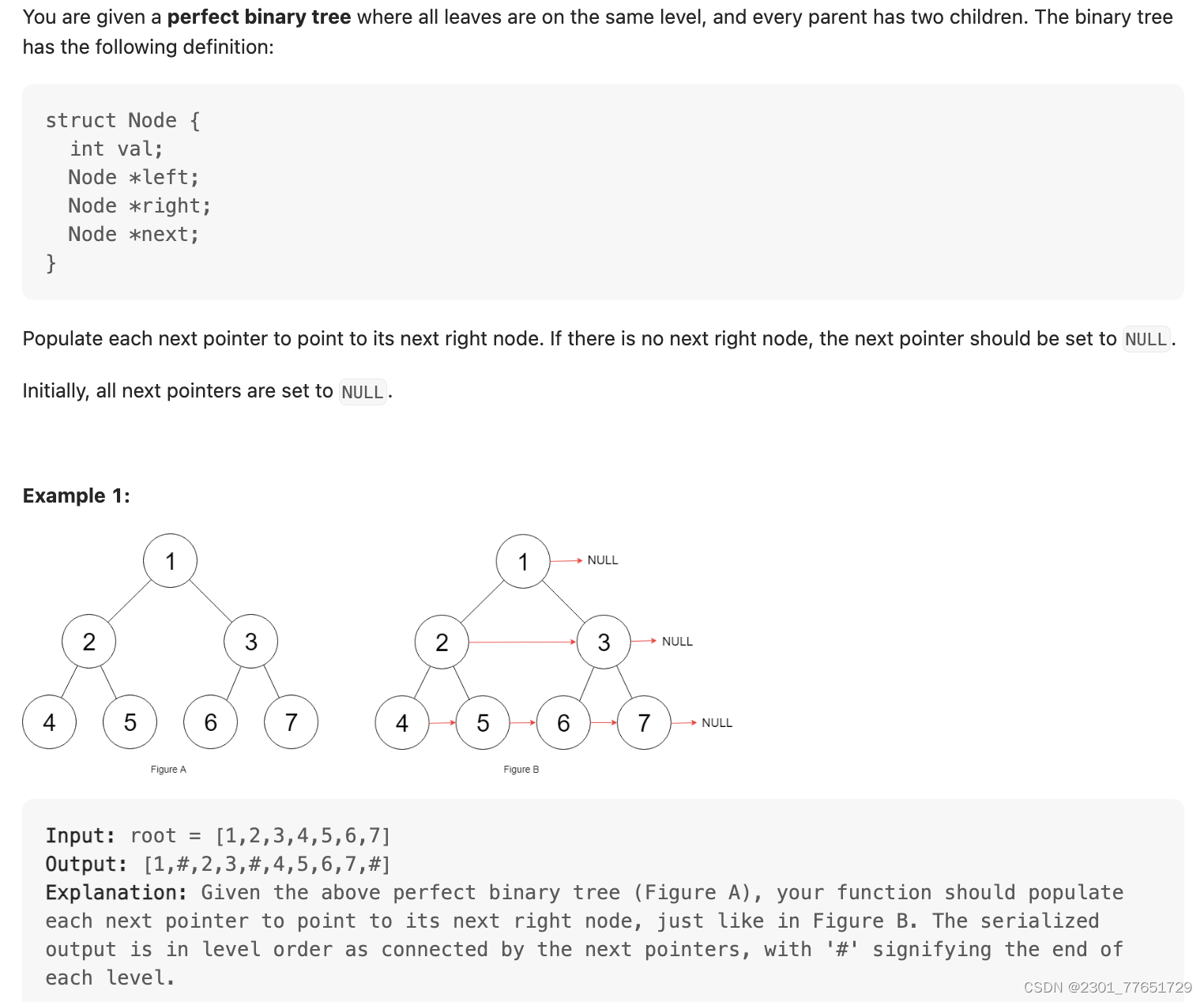
class Solution {
public Node connect(Node root) {
if(root == null) return null;
if(root.left != null) {
root.left.next = root.right;
}
if(root.right != null && root.next != null) {
root.right.next = root.next.left;
}
connect(root.left);
connect(root.right);
return root;
}
}
107. Binary Tree Level Order Traversal II
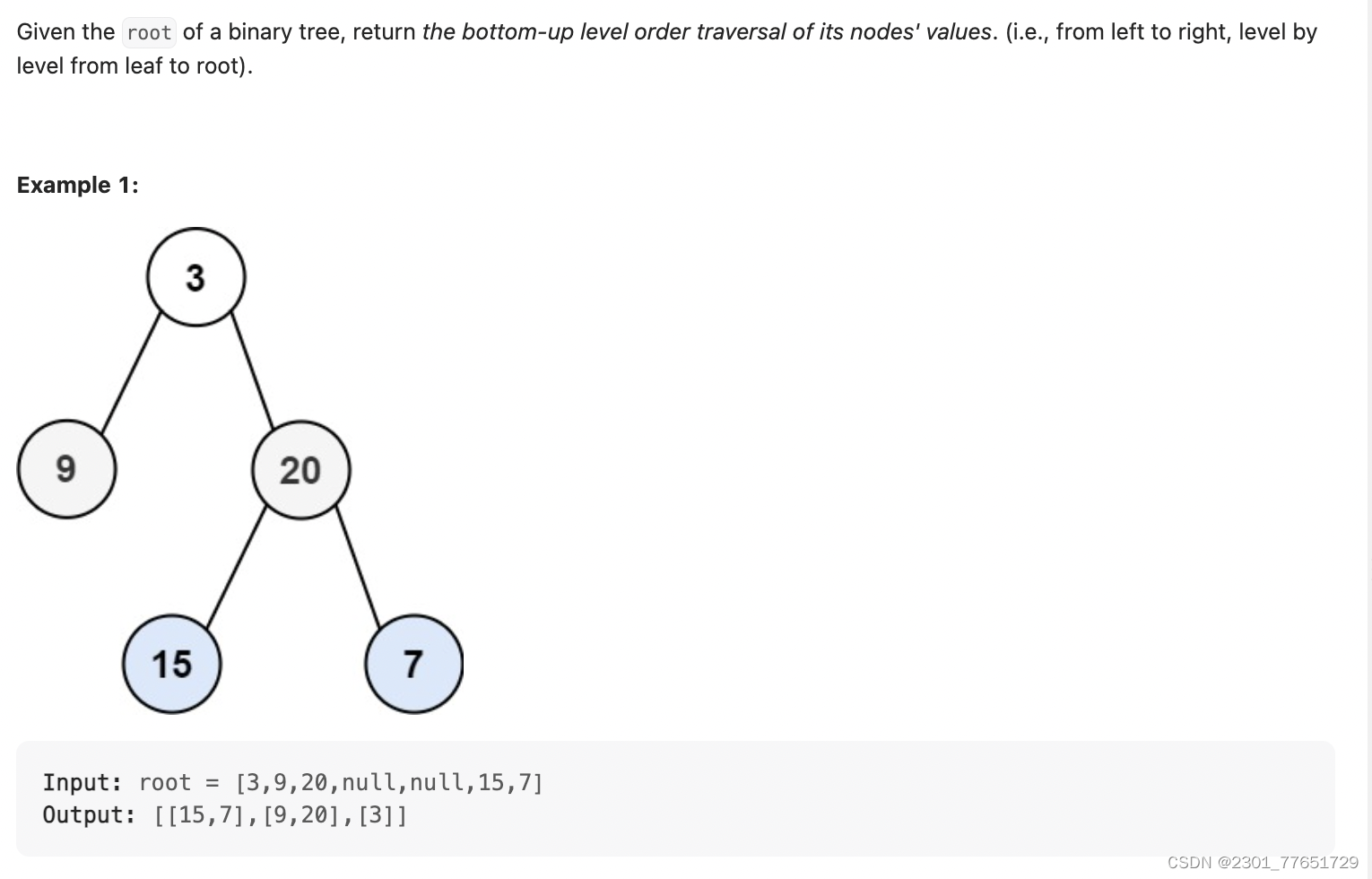
class Solution {
public List<List<Integer>> levelOrderBottom(TreeNode root) {
Stack<List<Integer>> res = new Stack();
Queue<TreeNode> queue = new LinkedList();
if(root == null) return res;
queue.offer(root);
while(!queue.isEmpty()) {
List<Integer> list = new ArrayList();
int len = queue.size();
for(int i = 0; i < len; i++) {
TreeNode cur = queue.poll();
list.add(cur.val);
if(cur.left != null) queue.offer(cur.left);
if(cur.right != null) queue.offer(cur.right);
}
res.push(list);
}
List<List<Integer>> finalRes = new ArrayList();
while(!res.isEmpty()) {
finalRes.add(res.pop());
}
return finalRes;
}
}
103. Binary Tree Zigzag Level Order Traversal
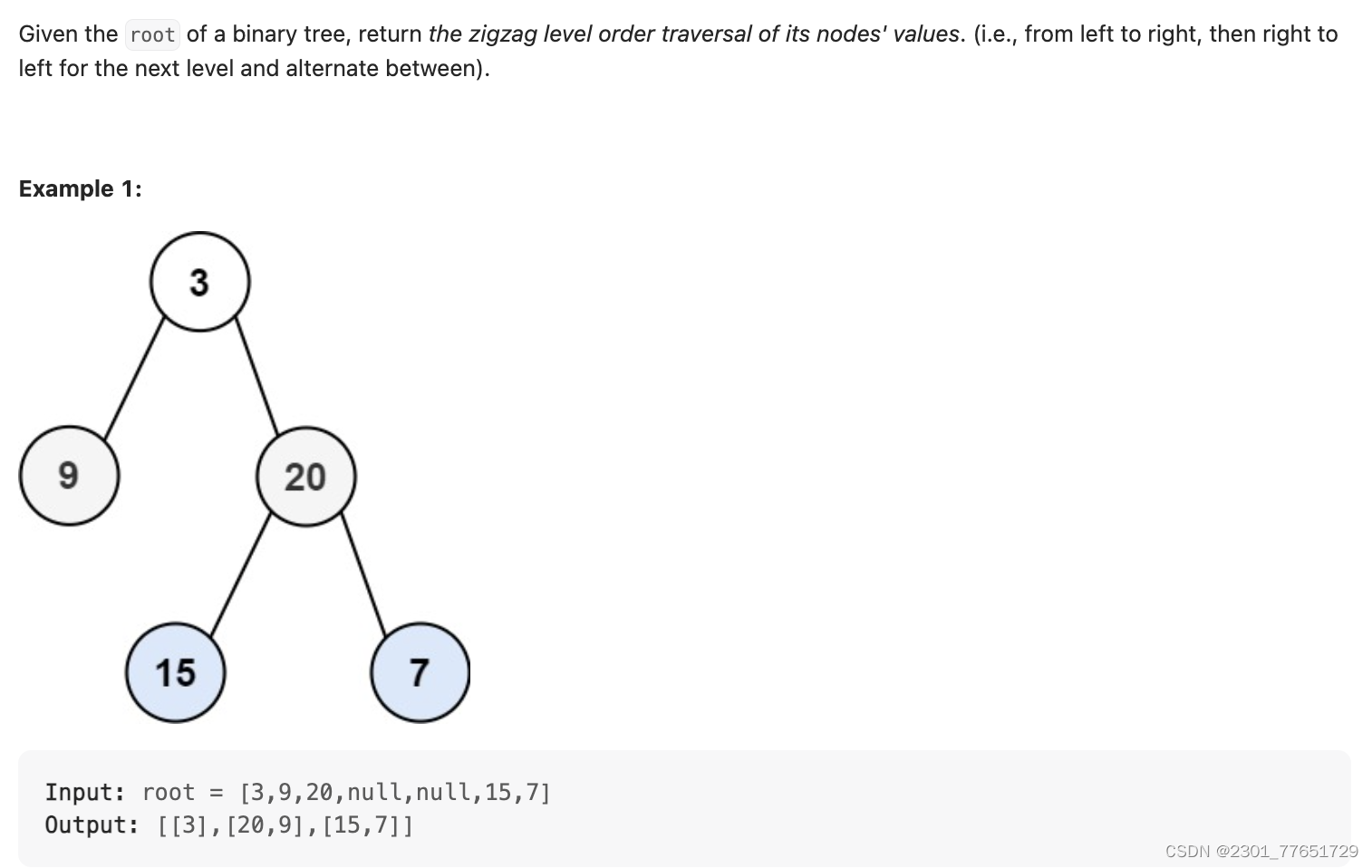
class Solution {
public List<List<Integer>> zigzagLevelOrder(TreeNode root) {
List<List<Integer>> res = new ArrayList();
if(root == null) return res;
Stack<TreeNode> s1 = new Stack();
Stack<TreeNode> s2 = new Stack();
s1.push(root);
while(!s1.isEmpty() || !s2.isEmpty()) {
List<Integer> tmp = new ArrayList();
while(!s1.isEmpty()) {
root = s1.pop();
tmp.add(root.val);
if(root.left != null) s2.push(root.left);
if(root.right != null) s2.push(root.right);
}
res.add(tmp);
tmp = new ArrayList<Integer>();
while(!s2.isEmpty()) {
root = s2.pop();
tmp.add(root.val);
if(root.right != null) s1.push(root.right);
if(root.left != null) s1.push(root.left);
}
if(!tmp.isEmpty()) res.add(tmp);
}
return res;
}
}
102. Binary Tree Level Order Traversal
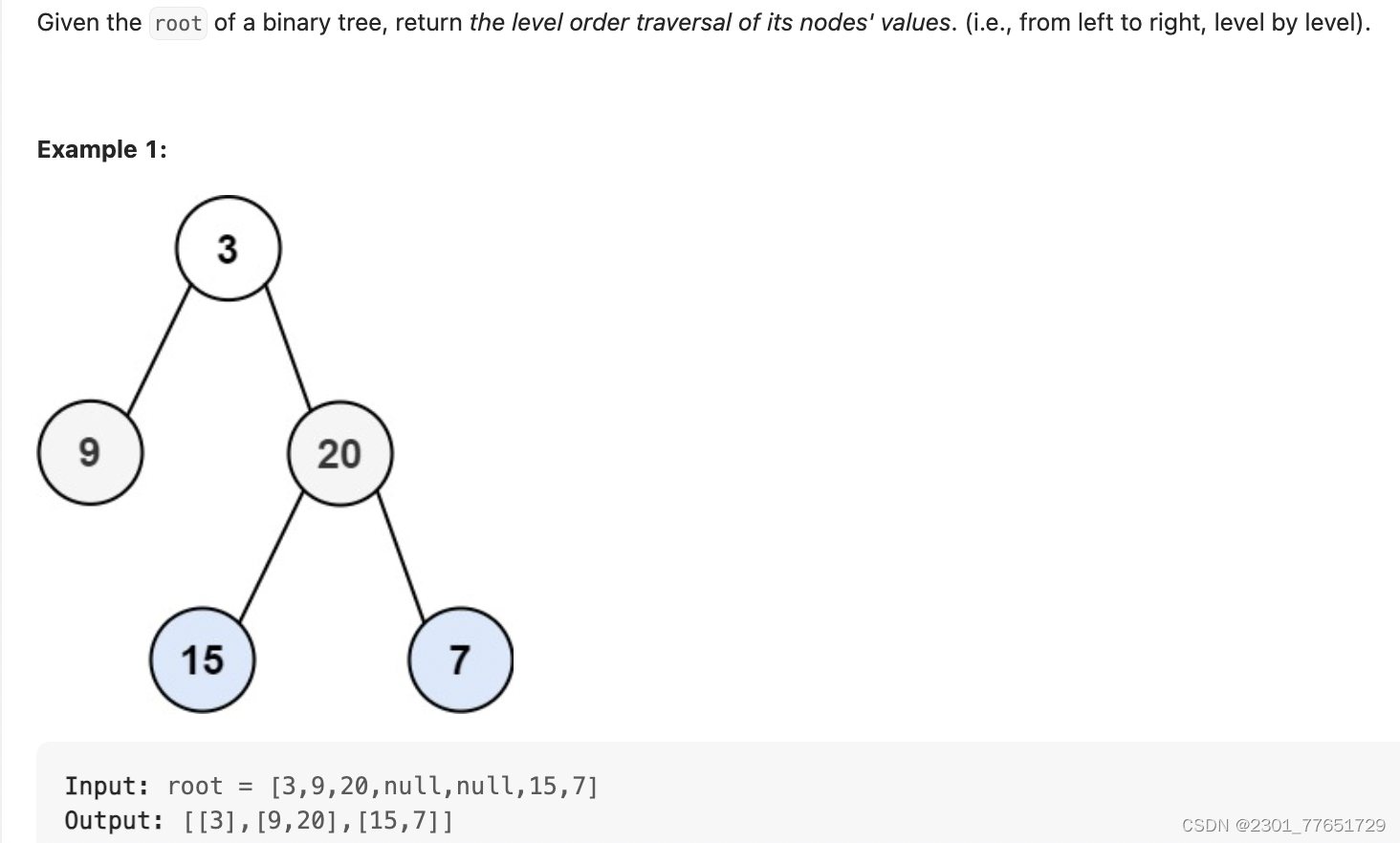
class Solution {
public List<List<Integer>> levelOrder(TreeNode root) {
List<List<Integer>> res = new ArrayList<List<Integer>>();
Deque<TreeNode> queue = new ArrayDeque();
if(root == null) {
return res;
}
queue.add(root);
while(!queue.isEmpty()) {
int len = queue.size();
List<Integer> row = new ArrayList();
for(int i = 0; i < len; i++) {
TreeNode cur = queue.poll();
row.add(cur.val);
if(cur.left != null) queue.add(cur.left);
if(cur.right != null) queue.add(cur.right);
}
res.add(row);
}
return res;
}
}