最后
不知道你们用的什么环境,我一般都是用的Python3.6环境和pycharm解释器,没有软件,或者没有资料,没人解答问题,都可以免费领取(包括今天的代码),过几天我还会做个视频教程出来,有需要也可以领取~
给大家准备的学习资料包括但不限于:
Python 环境、pycharm编辑器/永久激活/翻译插件
python 零基础视频教程
Python 界面开发实战教程
Python 爬虫实战教程
Python 数据分析实战教程
python 游戏开发实战教程
Python 电子书100本
Python 学习路线规划
网上学习资料一大堆,但如果学到的知识不成体系,遇到问题时只是浅尝辄止,不再深入研究,那么很难做到真正的技术提升。
一个人可以走的很快,但一群人才能走的更远!不论你是正从事IT行业的老鸟或是对IT行业感兴趣的新人,都欢迎加入我们的的圈子(技术交流、学习资源、职场吐槽、大厂内推、面试辅导),让我们一起学习成长!
模板字符串是一种更简单的机制,用于处理用户生成的格式字符串
from string import Template
language = Python
t = Template( $name is great! )
t.substitute(name=language) ## Substitutes name with language variable
Python is great!
#####Pyperclip
Pyperclip是一个Python库,可以帮助我们轻松复制和粘贴字符串
首先使用pip安装
pip install pyperclip
>>> import pyperclip
>>> pyperclip.copy( Hello World ) ## Copy Hello World
>>> pyperclip.paste()
Hello World
Number
Python中有三种数字类型
* int (e.g. 2, 4, 20)
bool (e.g. False and True, acting like 0 and 1)
* float (e.g. 5.0, 1.6)
* complex (e.g. 5+6j, 4-3j)
Basic Functions
a = pow(2, 3) ## Or: 2 \*\* 3
b = abs(5) ## <real> = abs(<num>)
Constants
from math import e, pi
Trigonometry
from math import cos, acos, sin, asin, tan, atan, degrees, radians
Logarithm
from math import log, log10, log2
<float> = log(<real> [, base]) # Base e, if not specified.
Infinity, nan
from math import inf, nan, isinf, isnan
Or:
float( inf ), float( nan )
Statistics
from statistics import mean, median, variance, pvariance, pstdev
Random
from random import random, randint, choice, shuffle
<float> = random()
<int> = randint(from\_inclusive, to\_inclusive)
<el> = choice(<list>)
shuffle(<list>)
Combinatorics
每个函数都返回一个迭代器。
如果要打印迭代器,需要将其传递给list()函数!
from itertools import product, combinations, combinations\_with\_replacement, permutations
>>> product([0, 1], repeat=3)
[(0, 0, 0), (0, 0, 1), (0, 1, 0), (0, 1, 1),
(1, 0, 0), (1, 0, 1), (1, 1, 0), (1, 1, 1)]
>>> product( ab , 12 )
[( a , 1 ), ( a , 2 ),
( b , 1 ), ( b , 2 )]
>>> combinations( abc , 2)
[( a , b ), ( a , c ), ( b , c )]
>>> combinations\_with\_replacement( abc , 2)
[( a , a ), ( a , b ), ( a , c ),
( b , b ), ( b , c ),
( c , c )]
>>> permutations( abc , 2)
[( a , b ), ( a , c ),
( b , a ), ( b , c ),
( c , a ), ( c , b )]
"""
def test\_integer\_numbers():
"""Integer type
Int, or integer, is a whole number, positive or negative,
without decimals, of unlimited length.
"""
positive\_integer = 1
negative\_integer = -3255522
big\_integer = 35656222554887711
assert isinstance(positive\_integer, int)
assert isinstance(negative\_integer, int)
assert isinstance(big\_integer, int)
def test\_booleans():
"""Boolean
Booleans represent the truth values False and True. The two objects representing the values
False and True are the only Boolean objects. The Boolean type is a subtype of the integer type,
and Boolean values behave like the values 0 and 1, respectively, in almost all contexts, the
exception being that when converted to a string, the strings "False" or "True" are returned,
respectively.
"""
true\_boolean = True
false\_boolean = False
assert true\_boolean
assert not false\_boolean
assert isinstance(true\_boolean, bool)
assert isinstance(false\_boolean, bool)
# Let s try to cast boolean to string.
assert str(true\_boolean) == "True"
assert str(false\_boolean) == "False"
def test\_float\_numbers():
"""Float type
Float, or "floating point number" is a number, positive or negative,
containing one or more decimals.
"""
float\_number = 7.0
# Another way of declaring float is using float() function.
float\_number\_via\_function = float(7)
float\_negative = -35.59
assert float\_number == float\_number\_via\_function
assert isinstance(float\_number, float)
assert isinstance(float\_number\_via\_function, float)
assert isinstance(float\_negative, float)
# Float can also be scientific numbers with an "e" to indicate
# the power of 10.
float\_with\_small\_e = 35e3
float\_with\_big\_e = 12E4
assert float\_with\_small\_e == 35000
assert float\_with\_big\_e == 120000
assert isinstance(12E4, float)
assert isinstance(-87.7e100, float)
def test\_complex\_numbers():
"""Complex Type"""
complex\_number\_1 = 5 + 6j
complex\_number\_2 = 3 - 2j
assert isinstance(complex\_number\_1, complex)
assert isinstance(complex\_number\_2, complex)
assert complex\_number\_1 \* complex\_number\_2 == 27 + 8j
def test\_number\_operators():
"""Basic operations"""
# Addition.
assert 2 + 4 == 6
# Multiplication.
assert 2 \* 4 == 8
# Division always returns a floating point number.
assert 12 / 3 == 4.0
assert 12 / 5 == 2.4
assert 17 / 3 == 5.666666666666667
# Modulo operator returns the remainder of the division.
assert 12 % 3 == 0
assert 13 % 3 == 1
# Floor division discards the fractional part.
assert 17 // 3 == 5
# Raising the number to specific power.
assert 5 \*\* 2 == 25 # 5 squared
assert 2 \*\* 7 == 128 # 2 to the power of 7
# There is full support for floating point; operators with
# mixed type operands convert the integer operand to floating point.
assert 4 \* 3.75 - 1 == 14.0
**File**
* Read File
* Write a File
* Copy File
* Move File
* Readline
Read a file
在Python 3中,如果文件未以二进制模式打开,则编码将由locale.getpreferredencoding(False)或用户输入确定。
>>> with open("/etc/hosts", encoding="utf-8") as f:
... content = f.read()
...
>>> print(type(content))
<class str >
In python3 binary mode
>>> with open("/etc/hosts", "rb") as f:
... content = f.read()
...
>>> print(type(content))
<class bytes >
In python2
## The content of the file is a byte string, not a Unicode string.
>>> with open("/etc/passwd") as f:
... content = f.read()
>>> print(type(content))
<type str >
>>> print(type(content.decode("utf-8")))
<type unicode >
Write a File
>>> file\_content = "Python is great!"
>>> with open("check.txt", "w") as file:
... file.write(file\_content)
Copy a file
>>> from distutils.file\_util import copy\_file
>>> copy\_file("a", "b")
( b , 1)
Move a File
>>> from distutils.file\_util import move\_file
>>> move\_file("./a", "./b")
./b
Readline
## If you are not using linux machine try to change the file path to read
>>> with open("/etc/hosts") as f:
... for line in f:
... print(line, end= )
...
127.0.0.1 localhost
255.255.255.255 broadcasthost
::1 localhost
**Python Functions**
* Lambda
* Declare Function
* Document Function
* Get Function Name
* Arguments
* Decorator
* Generator
Lambda
>>> fn = lambda x: x\*\*3
>>> fn(2)
8
>>> (lambda x: x\*\*3)(2)
8
>>> (lambda x: [x \* \_ for \_ in range(10)])(3)
[0, 3, 6, 9, 12, 15, 18, 21, 24, 27]
Declare Function
def fn\_name(): ## Where fn\_name represents the function name
........
........
Get Function Name
## First Declare a function
def fn\_name():
.......
.......
>>> fn\_name.\_\_name\_\_
fn\_name
Document a Function
## Document a function with three single quotes
def check():
This function is documented
return
>>> check.\_\_doc\_\_
This function is documented
Arguments
>>> def multiply(a, b=0): ## b is 0 by default
... return a \* b
...
>>> multiply(1, 3) ## 3 \* 1
3
>>> multiply(5) ## 5 \* 0
0
>>> multiply(5, b=3) ## 5 \* 3
15
Generator
def count(start, step):
while True:
yield start
start += step
>>> counter = count(10, 5)
>>> next(counter)
(15)
>>> next(counter) ## Increments by 5 from the previous result
(20)
>>> next(counter)
(25)
>>> next(counter), next(counter), next(counter)
(30, 35, 40)
Decorator
>>> from functools import wraps
>>> def decorator\_func(func):
... @wraps(func)
... def wrapper(\*args, \*\*kwargs):
... print("Before calling {}.".format(func.\_\_name\_\_))
... ret = func(\*args, \*\*kwargs)
... print("After calling {}.".format(func.\_\_name\_\_))
... return ret
... return wrapper
...
>>> @decorator\_func
... def check():
... print("Inside check function.")
...
>>> check()
Before calling check.
Inside check function.
After calling check.
**Classes**
* Definition
* Class Objects
* Instance Objects
* Method Objects
* Inheritance
* Multiple Inheritance
Class Definition
Python是一种面向对象的编程语言。 Python中的几乎所有东西都是一个对象,具有其属性和方法。 Class类似于对象构造函数,或者是用于创建对象的“蓝图”。
def test_class():
“”“Class definition.”“”
# Class definitions, like function definitions (def statements) must be executed before they
# have any effect. (You could conceivably place a class definition in a branch of an if
# statement, or inside a function.)
class GreetingClass:
“”“Example of the class definition
This class contains two public methods and doesn t contain constructor.
“””
name = Bisrat
def say_hello(self):
“”“Class method.”“”
# The self parameter is a reference to the class itself, and is used to access variables
# that belongs to the class. It does not have to be named self , you can call it
# whatever you like, but it has to be the first parameter of any function in the class.
return Hello + self.name
def say_goodbye(self):
“”“Class method.”“”
return Goodbye + self.name
# When a class definition is entered, a new namespace is created, and used as the local scope —
# thus, all assignments to local variables go into this new namespace. In particular, function
# definitions bind the name of the new function here.
# Class instantiation uses function notation. Just pretend that the class object is a
# parameterless function that returns a new instance of the class. For example the following
# code will creates a new instance of the class and assigns this object to the local variable.
greeter = GreetingClass()
assert greeter.say_hello() == Hello Bisrat
assert greeter.say_goodbye() == Goodbye Bisrat
print(type(content))
**Class Objects**
def test\_class\_objects():
"""Class Objects.
Class objects support two kinds of operations:
- attribute references
- instantiation.
"""
# ATTRIBUTE REFERENCES use the standard syntax used for all attribute references in
# Python: obj.name. Valid attribute names are all the names that were in the class’s namespace
# when the class object was created. For class MyCounter the following references are valid
# attribute references:
class ComplexNumber:
"""Example of the complex numbers class"""
real = 0
imaginary = 0
def get\_real(self):
"""Return real part of complex number."""
return self.real
def get\_imaginary(self):
"""Return imaginary part of complex number."""
return self.imaginary
assert ComplexNumber.real == 0
# \_\_doc\_\_ is also a valid attribute, returning the docstring belonging to the class
assert ComplexNumber.\_\_doc\_\_ == Example of the complex numbers class
# Class attributes can also be assigned to, so you can change the value of
# ComplexNumber.counter by assignment.
ComplexNumber.real = 10
assert ComplexNumber.real == 10
# CLASS INSTANTIATION uses function notation. Just pretend that the class object is a
# parameterless function that returns a new instance of the class. For example
# (assuming the above class):
complex\_number = ComplexNumber()
assert complex\_number.real == 10
assert complex\_number.get\_real() == 10
# Let s change counter default value back.
ComplexNumber.real = 10
assert ComplexNumber.real == 10
# The instantiation operation (“calling” a class object) creates an empty object. Many classes
# like to create objects with instances customized to a specific initial state. Therefore a
# class may define a special method named \_\_init\_\_(), like this:
class ComplexNumberWithConstructor:
"""Example of the class with constructor"""
def \_\_init\_\_(self, real\_part, imaginary\_part):
self.real = real\_part
self.imaginary = imaginary\_part
def get\_real(self):
"""Return real part of complex number."""
return self.real
def get\_imaginary(self):
"""Return imaginary part of complex number."""
return self.imaginary
complex\_number = ComplexNumberWithConstructor(3.0, -4.5)
assert complex\_number.real, complex\_number.imaginary == (3.0, -4.5)
#### **Instance Object**
def test\_instance\_objects():
# DATA ATTRIBUTES need not be declared; like local variables, they spring into existence when
# they are first assigned to. For example, if x is the instance of MyCounter created above,
# the following piece of code will print the value 16, without leaving a trace.
# pylint: disable=too-few-public-methods
class DummyClass:
pass
dummy\_instance = DummyClass()
# pylint: disable=attribute-defined-outside-init
dummy\_instance.temporary\_attribute = 1
assert dummy\_instance.temporary\_attribute == 1
del dummy\_instance.temporary\_attribute
**Method Objects**
class MyCounter:
"""A simple example of the counter class"""
counter = 10
def get\_counter(self):
"""Return the counter"""
return self.counter
def increment\_counter(self):
"""Increment the counter"""
self.counter += 1
return self.counter
def test\_method\_objects():
"""Method Objects."""
# object types can have methods as well. For example, list objects have methods called append,
counter = MyCounter()
assert counter.get\_counter() == 10
get\_counter = counter.get\_counter
assert get\_counter() == 10
assert counter.get\_counter() == 10
assert MyCounter.get\_counter(counter) == 10
**Inheritance**
# pylint: disable=too-few-public-methods
class Person:
"""Example of the base class"""
def \_\_init\_\_(self, name):
self.name = name
def get\_name(self):
"""Get person name"""
return self.name
# The syntax for a derived class definition looks like this.
class Employee(Person):
def \_\_init\_\_(self, name, staff\_id):
Person.\_\_init\_\_(self, name)
# You may also use super() here in order to avoid explicit using of parent class name:
# >>> super().\_\_init\_\_(name)
self.staff\_id = staff\_id
def get\_full\_id(self):
"""Get full employee id"""
return self.get\_name() + , + self.staff\_id
def test\_inheritance():
"""Inheritance."""
# There’s nothing special about instantiation of derived classes: DerivedClassName() creates a
# new instance of the class. Method references are resolved as follows: the corresponding class
# attribute is searched, descending down the chain of base classes if necessary, and the method
# reference is valid if this yields a function object.
person = Person( Bisrat )
employee = Employee( John , A23 )
assert person.get\_name() == Bisrat
assert employee.get\_name() == John
assert employee.get\_full\_id() == John, A23
# Python has two built-in functions that work with inheritance:
#
# - Use isinstance() to check an instance’s type: isinstance(obj, int) will be True only if
# obj.\_\_class\_\_ is int or some class derived from int.
#
# - Use issubclass() to check class inheritance: issubclass(bool, int) is True since bool is
# a subclass of int. However, issubclass(float, int) is False since float is not a subclass
# of int.
assert isinstance(employee, Employee)
assert not isinstance(person, Employee)
assert isinstance(person, Person)
assert isinstance(employee, Person)
assert issubclass(Employee, Person)
assert not issubclass(Person, Employee)
**Multiple Inheritance**
def test_multiple_inheritance():
“”“Multiple Inheritance”“”
class Clock:
“”“Clock class”“”
time = 10:17 PM
def get_time(self):
“”“Get current time
Method is hardcoded just for multiple inheritance illustration.
“””
return self.time
class Calendar:
“”“Calendar class”“”
date = 12/08/2018
def get_date(self):
“”“Get current date
Method is hardcoded just for multiple inheritance illustration.
“””
return self.date
# Python supports a form of multiple inheritance as well. A class definition with multiple
# base classes looks like this.
class CalendarClock(Clock, Calendar):
calendar_clock = CalendarClock()
assert calendar_clock.get_date() == 12/08/2018
assert calendar_clock.get_time() == 11:23 PM
**Date and Time**
* DateTime
* Time Delta
* Calendar
datetime
from datetime import date
from datetime import time
from datetime import datetime
>>> today = date.today()
>>> print(today)
2019-03-04
>>> print(today.day, today.month, today.year)
(4, 3, 2019)
>>> print(today.weekday()) ## returns an integers in the range 0 to 6, where 0 represents Monday and 6 represents Sunday.
0
>>> today = datetime.now()
>>> print(today)
2019-03-04 21:55:56.228000
>>> time = datetime.time(datetime.now())
>>> print(time)
21:56:16.040000
Time Delta
如果你也是看准了Python,想自学Python,在这里为大家准备了丰厚的免费**学习**大礼包,带大家一起学习,给大家剖析Python兼职、就业行情前景的这些事儿。
### 一、Python所有方向的学习路线
Python所有方向路线就是把Python常用的技术点做整理,形成各个领域的知识点汇总,它的用处就在于,你可以按照上面的知识点去找对应的学习资源,保证自己学得较为全面。
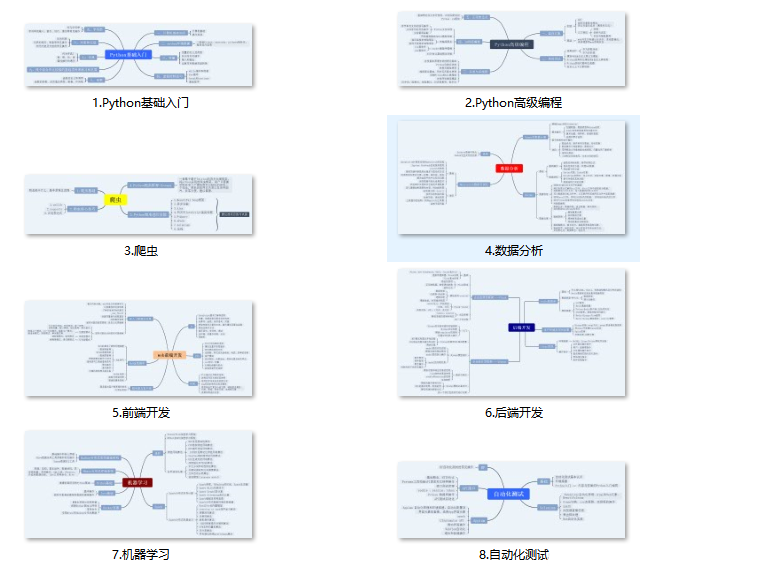
### 二、学习软件
工欲善其必先利其器。学习Python常用的开发软件都在这里了,给大家节省了很多时间。
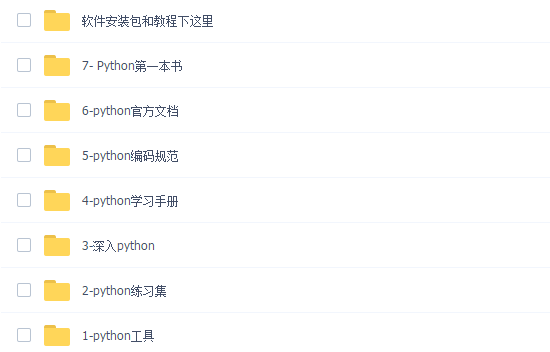
### 三、全套PDF电子书
书籍的好处就在于权威和体系健全,刚开始学习的时候你可以只看视频或者听某个人讲课,但等你学完之后,你觉得你掌握了,这时候建议还是得去看一下书籍,看权威技术书籍也是每个程序员必经之路。
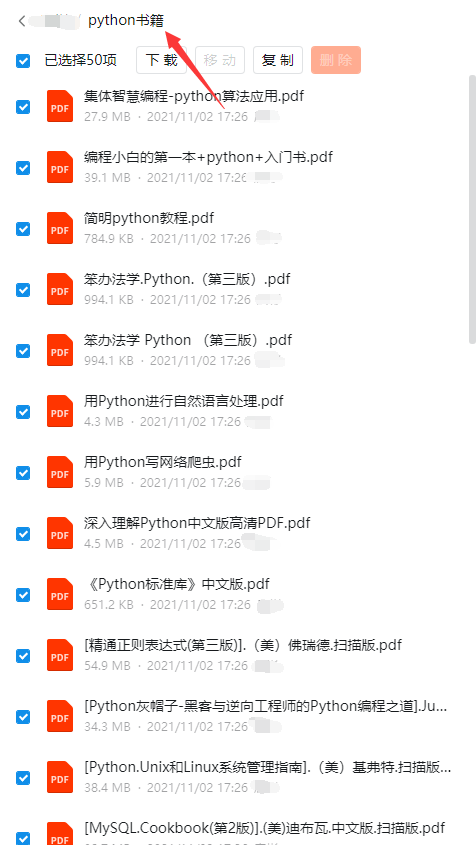
### 四、入门学习视频
我们在看视频学习的时候,不能光动眼动脑不动手,比较科学的学习方法是在理解之后运用它们,这时候练手项目就很适合了。
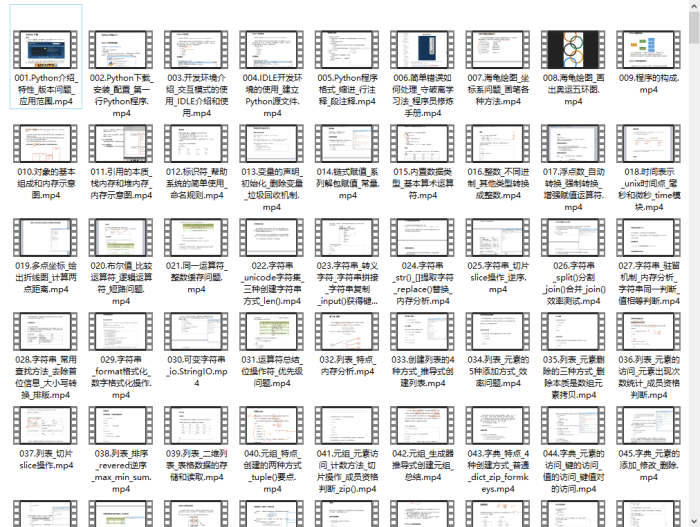
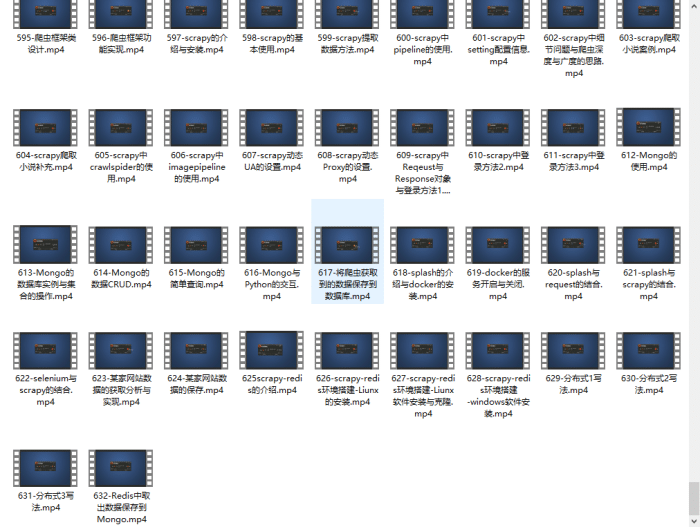
### 四、实战案例
光学理论是没用的,要学会跟着一起敲,要动手实操,才能将自己的所学运用到实际当中去,这时候可以搞点实战案例来学习。
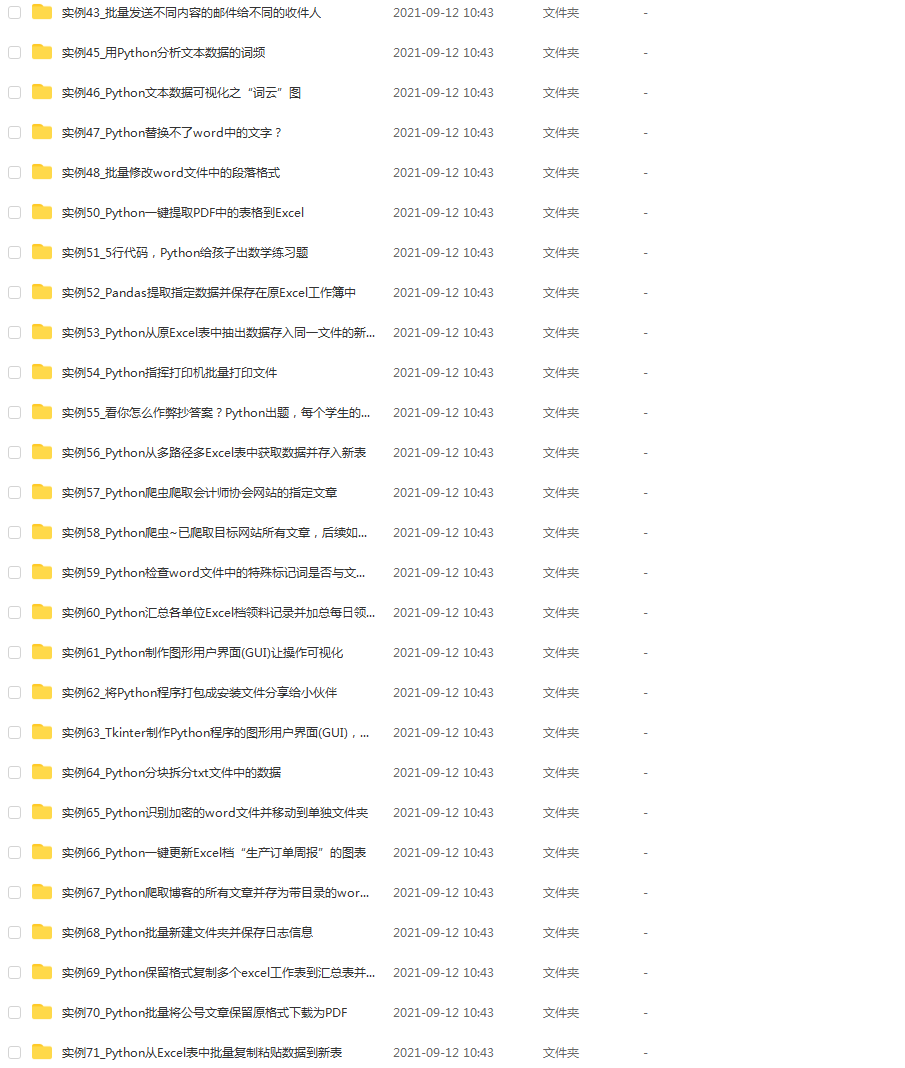
### 五、面试资料
我们学习Python必然是为了找到高薪的工作,下面这些面试题是来自阿里、腾讯、字节等一线互联网大厂最新的面试资料,并且有阿里大佬给出了权威的解答,刷完这一套面试资料相信大家都能找到满意的工作。
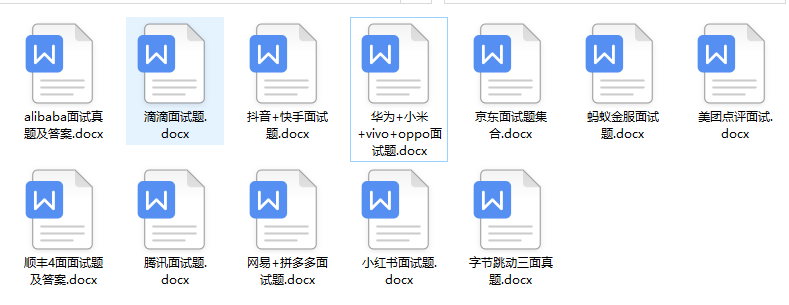
成为一个Python程序员专家或许需要花费数年时间,但是打下坚实的基础只要几周就可以,如果你按照我提供的学习路线以及资料有意识地去实践,你就有很大可能成功!
最后祝你好运!!!
**网上学习资料一大堆,但如果学到的知识不成体系,遇到问题时只是浅尝辄止,不再深入研究,那么很难做到真正的技术提升。**
**[需要这份系统化学习资料的朋友,可以戳这里获取](https://bbs.csdn.net/forums/4304bb5a486d4c3ab8389e65ecb71ac0)**
**一个人可以走的很快,但一群人才能走的更远!不论你是正从事IT行业的老鸟或是对IT行业感兴趣的新人,都欢迎加入我们的的圈子(技术交流、学习资源、职场吐槽、大厂内推、面试辅导),让我们一起学习成长!**
也是每个程序员必经之路。
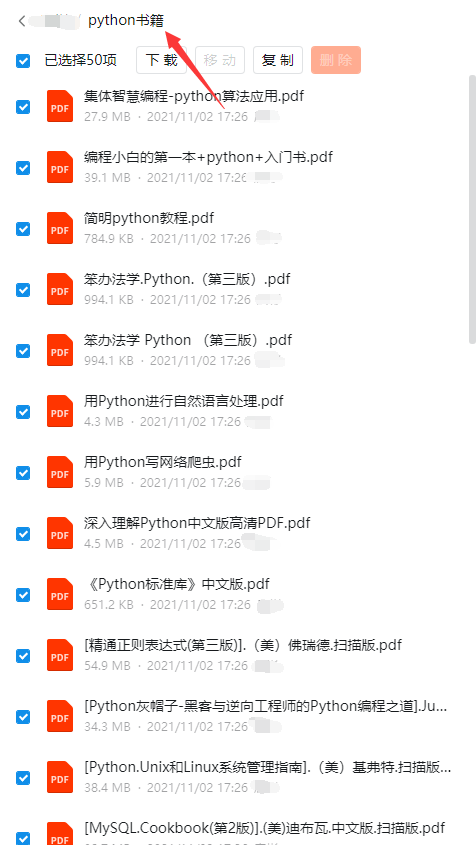
### 四、入门学习视频
我们在看视频学习的时候,不能光动眼动脑不动手,比较科学的学习方法是在理解之后运用它们,这时候练手项目就很适合了。
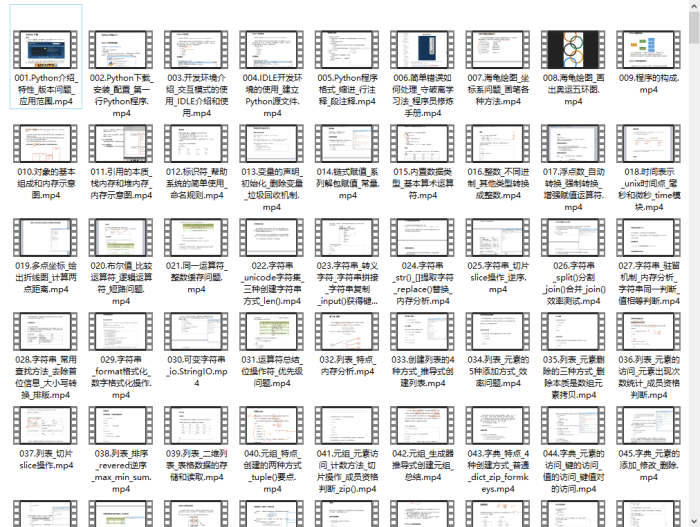
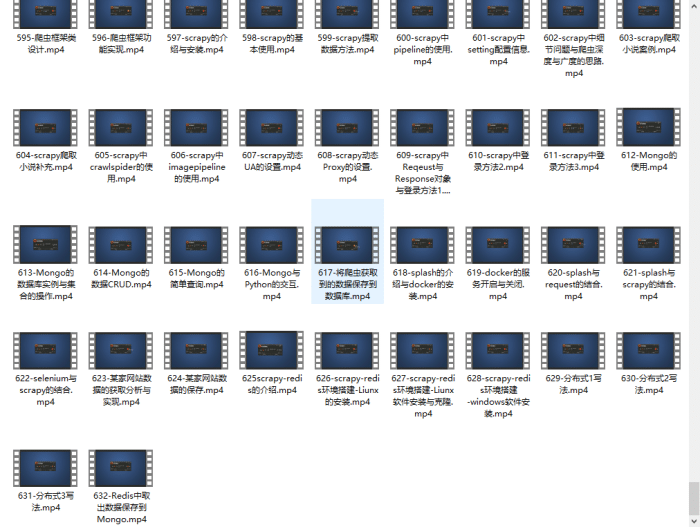
### 四、实战案例
光学理论是没用的,要学会跟着一起敲,要动手实操,才能将自己的所学运用到实际当中去,这时候可以搞点实战案例来学习。
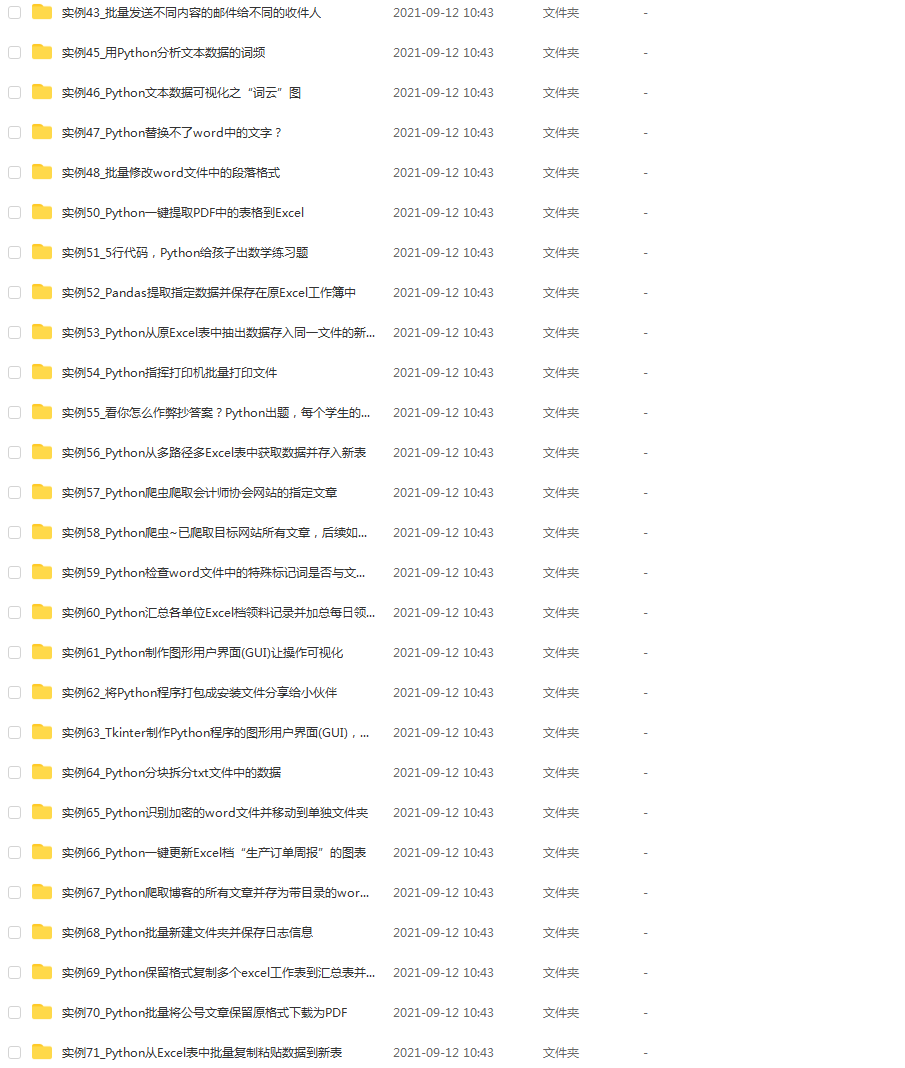
### 五、面试资料
我们学习Python必然是为了找到高薪的工作,下面这些面试题是来自阿里、腾讯、字节等一线互联网大厂最新的面试资料,并且有阿里大佬给出了权威的解答,刷完这一套面试资料相信大家都能找到满意的工作。
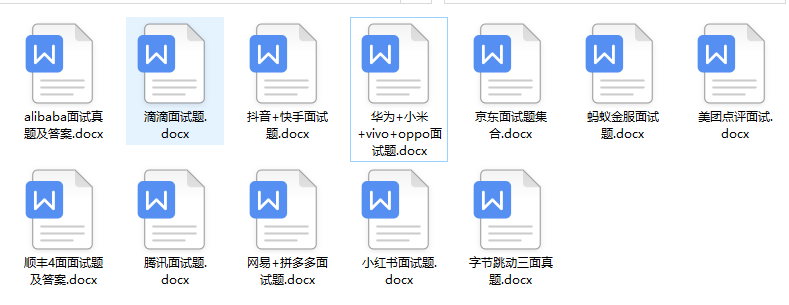
成为一个Python程序员专家或许需要花费数年时间,但是打下坚实的基础只要几周就可以,如果你按照我提供的学习路线以及资料有意识地去实践,你就有很大可能成功!
最后祝你好运!!!
**网上学习资料一大堆,但如果学到的知识不成体系,遇到问题时只是浅尝辄止,不再深入研究,那么很难做到真正的技术提升。**
**[需要这份系统化学习资料的朋友,可以戳这里获取](https://bbs.csdn.net/forums/4304bb5a486d4c3ab8389e65ecb71ac0)**
**一个人可以走的很快,但一群人才能走的更远!不论你是正从事IT行业的老鸟或是对IT行业感兴趣的新人,都欢迎加入我们的的圈子(技术交流、学习资源、职场吐槽、大厂内推、面试辅导),让我们一起学习成长!**