Sex
varchar(10) CHARACTER SET utf8 COLLATE utf8_general_ci NOT NULL,
Phone
varchar(20) CHARACTER SET utf8 COLLATE utf8_general_ci NOT NULL,
Email
varchar(30) CHARACTER SET utf8 COLLATE utf8_general_ci NOT NULL,
PRIMARY KEY (Id
) USING BTREE
) ENGINE = InnoDB CHARACTER SET = utf8 COLLATE = utf8_general_ci ROW_FORMAT = Dynamic;
– Records of stuinfo
INSERT INTO stuinfo
VALUES (‘105001’, ‘黄晋江’, 35, ‘数计院’, ‘男’, ‘18050193364’, ‘48577342@qq.com’);
INSERT INTO stuinfo
VALUES (‘105002’, ‘叶小白’, 21, ‘数计院’, ‘男’, ‘18056789321’, ‘4793247@qq.com’);
INSERT INTO stuinfo
VALUES (‘105003’, ‘林幼玲’, 19, ‘医学院’, ‘女’, ‘15745492821’, ‘4488742@qq.com’);
INSERT INTO stuinfo
VALUES (‘105004’, ‘白凌琳’, 20, ‘文学院’, ‘女’, ‘180437289678’, ‘75834538@qq.com’);
INSERT INTO stuinfo
VALUES (‘105005’, ‘廖江土’, 22, ‘数计院’, ‘男’, ‘18050400657’, ‘73476432@qq.com’);
INSERT INTO stuinfo
VALUES (‘105009’, ‘黄晋江’, 77, ‘数计院’, ‘男’, ‘18050193364’, ‘48577342111@qq.com’);
SET FOREIGN_KEY_CHECKS = 1;
二、系统展示
======
1.登录页面
2.主页面
3.查询学生信息
4.添加学生信息
5.修改学生信息
三、部分代码
=======
StudentDao
package cn.fjnu.edu.dao;
import cn.fjnu.edu.model.Student;
import java.util.List;
public interface StudentDao {
public boolean Create(Student student) throws Exception;
public boolean Update(Student student) throws Exception;
public boolean Delete(Student student) throws Exception;
public boolean findLogin(Student student) throws Exception;
List findAll(String keyWord) throws Exception;
}
StuDaoImpl
package cn.fjnu.edu.daoimpl;
import cn.fjnu.edu.dao.StudentDao;
import cn.fjnu.edu.model.Student;
import cn.fjnu.edu.util.DBUtil;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.Statement;
import java.util.ArrayList;
import java.util.List;
public class StuDaoImpl implements StudentDao {
final String strCreate = “insert into stuinfo values(?,?,?,?,?,?,?)”;
final String strDelete = “delete from stuinfo where 1=1”;
final String strUpdate = “update stuinfo set”;
final String strFind = “select * from stuinfo where Name like ? or Dep like ? or Id like ? or Age like ? or Sex like ? or Phone like ? or Email like ?”;
final String strLogin = “select Name from stuinfo where id=? and Phone=?”;
@Override
public boolean Create(Student student) throws Exception {
DBUtil msh = new DBUtil();
Connection conn = msh.getConnection();
PreparedStatement pstmt = conn.prepareStatement(strCreate);
pstmt.setString(1, student.getId());
pstmt.setString(2, student.getName());
pstmt.setInt(3, student.getAge());
pstmt.setString(4, student.getDep());
pstmt.setString(5, student.getSex());
pstmt.setString(6, student.getPhone());
pstmt.setString(7, student.getEmail());
int i = pstmt.executeUpdate();
pstmt.close();
if (i > 0)
return true;
else
return false;
}
@Override
public boolean Update(Student student) throws Exception {
DBUtil msh = new DBUtil();
Connection conn = msh.getConnection();
Statement stmt = conn.createStatement();
String str = strUpdate;
if (!(student.getName().equals(null))) {
str += " Name=‘" + student.getName() + "’ ";
}
if (!(student.getDep().equals(null)))
str += “,Dep='” + student.getDep() + “'”;
if (!(student.getSex().equals(null)))
str += “,Sex='” + student.getSex() + “'”;
if (!(student.getPhone().equals(null)))
str += “,Phone='” + student.getPhone() + “'”;
if (!(student.getEmail().equals(null)))
str += “,Email='” + student.getEmail() + “'”;
if (student.getAge() != 0) {
str += “,Age=” + student.getAge() + “”;
}
str += " where Id=" + student.getId() + “;”;
System.out.println(str);
int i = stmt.executeUpdate(str);
stmt.close();
msh.closeConnection(conn);
if (i > 0)
return true;
else {
System.out.println(i + " errorD");
return false;
}
}
@Override
public boolean Delete(Student student) throws Exception {
DBUtil msh = new DBUtil();
Connection conn = msh.getConnection();
Statement stmt = conn.createStatement();
String str = strDelete;
if (!(“”.equals(student.getId())))
str += " and Id=" + student.getId();
int i = stmt.executeUpdate(str);
stmt.close();
msh.closeConnection(conn);
if (i > 0)
return true;
else
return false;
}
@Override
public boolean findLogin(Student student) throws Exception {
DBUtil msh = new DBUtil();
Connection conn = msh.getConnection();
PreparedStatement pstmt = conn.prepareStatement(strCreate);
boolean flag = false;
try {
pstmt = conn.prepareStatement(strLogin);
pstmt.setString(1, student.getId());
pstmt.setString(2, student.getPhone());
ResultSet rs = pstmt.executeQuery();
if (rs.next()) {
student.setName(rs.getString(1));
flag = true;
}
} catch (Exception e) {
throw e;
} finally {
if (pstmt != null) {
try {
pstmt.close();
} catch (Exception e) {
throw e;
}
}
}
return flag;
}
@Override
public List findAll(String keyWord) throws Exception {
List all = new ArrayList();
DBUtil msh = new DBUtil();
Connection conn = msh.getConnection();
PreparedStatement pstmt = conn.prepareStatement(strFind);
pstmt.setString(1, “%” + keyWord + “%”);
pstmt.setString(2, “%” + keyWord + “%”);
pstmt.setString(3, “%” + keyWord + “%”);
pstmt.setString(4, “%” + keyWord + “%”);
pstmt.setString(5, “%” + keyWord + “%”);
pstmt.setString(6, keyWord);
pstmt.setString(7, keyWord);
ResultSet rs = pstmt.executeQuery();
Student people = null;
while (rs.next()) {
people = new Student();
people.setId(rs.getString(1));
people.setName(rs.getString(2));
people.setAge(rs.getInt(3));
people.setDep(rs.getString(4));
people.setSex(rs.getString(5));
people.setPhone(rs.getString(6));
people.setEmail(rs.getString(7));
all.add(people);
}
pstmt.close();
msh.closeConnection(conn);
return all;
}
}
StudentService
package cn.fjnu.edu.service;
import cn.fjnu.edu.model.Student;
import java.util.List;
public interface StudentService {
public boolean Create(Student student) throws Exception;
public boolean Update(Student student) throws Exception;
public boolean Delete(Student student) throws Exception;
public boolean findLogin(Student student) throws Exception;
List findAll(String keyWord) throws Exception;
}
StudentServiceImpl
package cn.fjnu.edu.serviceimpl;
import cn.fjnu.edu.daoimpl.StuDaoImpl;
import cn.fjnu.edu.model.Student;
import cn.fjnu.edu.service.StudentService;
import java.util.List;
public class StudentServiceImpl implements StudentService {
@Override
public boolean Create(Student student) throws Exception {
自我介绍一下,小编13年上海交大毕业,曾经在小公司待过,也去过华为、OPPO等大厂,18年进入阿里一直到现在。
深知大多数Java工程师,想要提升技能,往往是自己摸索成长或者是报班学习,但对于培训机构动则几千的学费,着实压力不小。自己不成体系的自学效果低效又漫长,而且极易碰到天花板技术停滞不前!
因此收集整理了一份《2024年Java开发全套学习资料》,初衷也很简单,就是希望能够帮助到想自学提升又不知道该从何学起的朋友,同时减轻大家的负担。
既有适合小白学习的零基础资料,也有适合3年以上经验的小伙伴深入学习提升的进阶课程,基本涵盖了95%以上Java开发知识点,真正体系化!
由于文件比较大,这里只是将部分目录截图出来,每个节点里面都包含大厂面经、学习笔记、源码讲义、实战项目、讲解视频,并且会持续更新!
如果你觉得这些内容对你有帮助,可以扫码获取!!(备注Java获取)
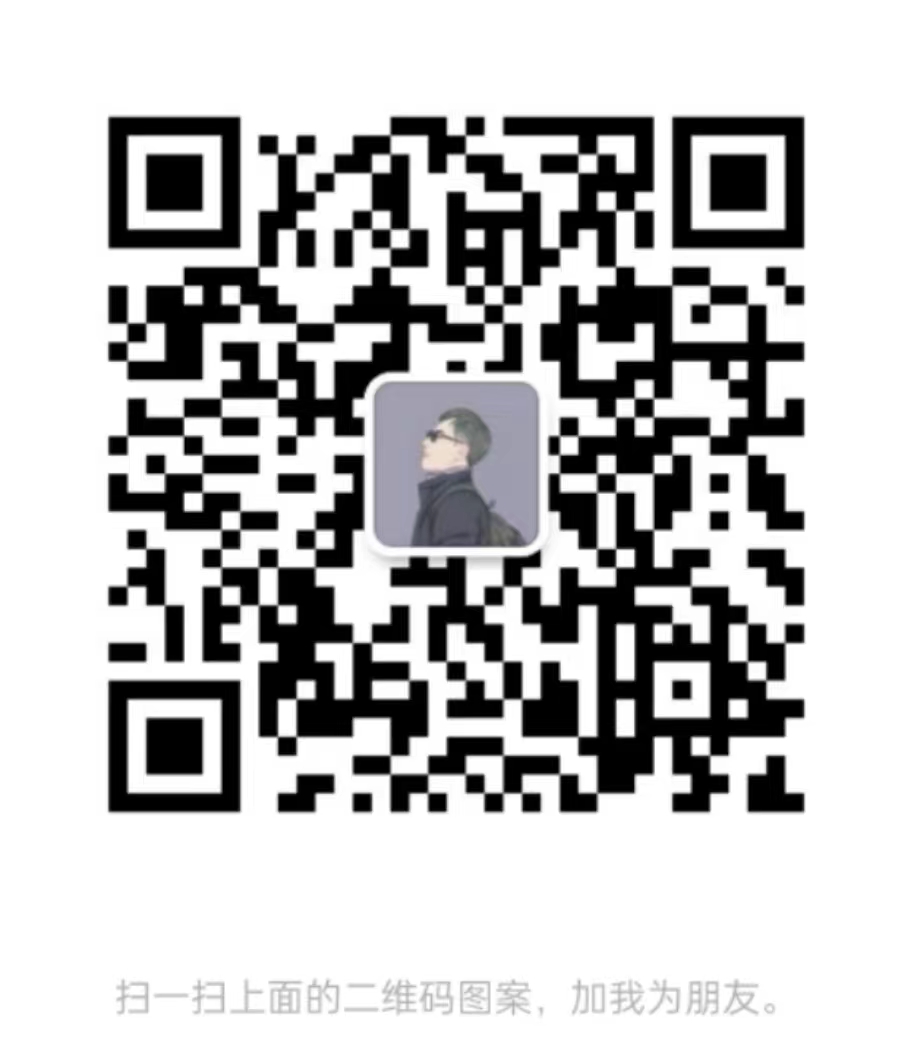
最后
码字不易,觉得有帮助的可以帮忙点个赞,让更多有需要的人看到
又是一年求职季,在这里,我为各位准备了一套Java程序员精选高频面试笔试真题,来帮助大家攻下BAT的offer,题目范围从初级的Java基础到高级的分布式架构等等一系列的面试题和答案,用于给大家作为参考
以下是部分内容截图
《一线大厂Java面试题解析+核心总结学习笔记+最新讲解视频+实战项目源码》,点击传送门即可获取!
升的进阶课程,基本涵盖了95%以上Java开发知识点,真正体系化!**
由于文件比较大,这里只是将部分目录截图出来,每个节点里面都包含大厂面经、学习笔记、源码讲义、实战项目、讲解视频,并且会持续更新!
如果你觉得这些内容对你有帮助,可以扫码获取!!(备注Java获取)
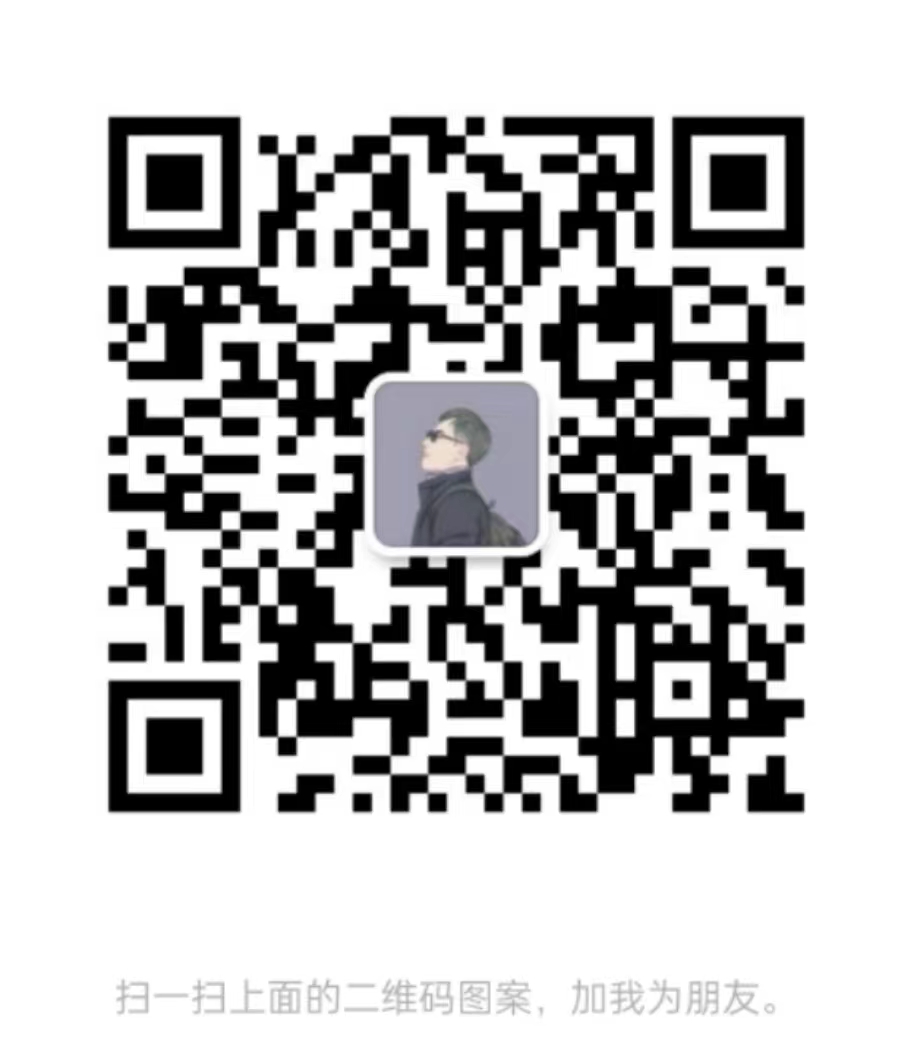
最后
码字不易,觉得有帮助的可以帮忙点个赞,让更多有需要的人看到
又是一年求职季,在这里,我为各位准备了一套Java程序员精选高频面试笔试真题,来帮助大家攻下BAT的offer,题目范围从初级的Java基础到高级的分布式架构等等一系列的面试题和答案,用于给大家作为参考
以下是部分内容截图
[外链图片转存中…(img-h9f9T8Da-1711972708047)]
《一线大厂Java面试题解析+核心总结学习笔记+最新讲解视频+实战项目源码》,点击传送门即可获取!