网上学习资料一大堆,但如果学到的知识不成体系,遇到问题时只是浅尝辄止,不再深入研究,那么很难做到真正的技术提升。
一个人可以走的很快,但一群人才能走的更远!不论你是正从事IT行业的老鸟或是对IT行业感兴趣的新人,都欢迎加入我们的的圈子(技术交流、学习资源、职场吐槽、大厂内推、面试辅导),让我们一起学习成长!
* --end;
* }
* psl->a[pos]=x;
* ++psl->size;
* 这种写法 pos会隐式提升为size_t(unsigned int)类型
* end 和 pos都为0之后,再--end,此时end变为一个非常大的数字
* 注意这里的隐式提升
* end和pos,俩个不能有一个是size_t类型,不然程序会出现错误
*/
#### 数据查找
int SLPfine(SL* psl, SLDatatype x)
{
assert(psl);
int i = 0;
for (i = 0; i < psl->size; i++)
{
if (psl->a[i] == x)
return i;
}
return -1;
}
#### 数据打印
void SLPprint(SL* psl)
{
assert(psl);
int i = 0;
for (i = 0; i < psl->size; i++)
{
printf("%d ", psl->a[i]);
}
}
#### 数据修改
void SLModify(SL* psl, size_t pos, SLDatatype x)
{
assert(psl);
assert(pos < psl->size);
psl->a[pos] = x;
}
### 顺序表缺点
>
> 1. 中间/头部的插入删除,时间复杂度为O(N)
>
>
> 2. 增容需要申请新空间,拷贝数据,释放旧空间。会有不小的消耗。
>
>
> 3. 增容一般是呈2倍的增长,势必会有一定的空间浪费。例如当前容量为100,满了以后增容到 200,我们再继续插入了5个数据,后面没有数据插入了,那么就浪费了95个数据空间。
>
>
>
## 链表
>
> 链表:链表是一种物理存储结构上非连续、非顺序的存储结构,数据元素的逻辑顺序是通过链表 中的指针链接次序实现的 。 单链表不需要初始化
>
>
> 
>
>
>
>
### 结构体的创建
>
> 正确写法
>
>
>
>
> ```
> typedef int SLTDataType;
> typedef struct SlistNode
> {
> SLTDataType data;
> struct SlistNode* next;
> }SLTNode;
> ```
>
> 错误写法
>
>
>
> ```
> typedef struct SlistNode
> {
> SLTDataType data;
> struct SlistNode* next;
> //SLTNode*next; 错误写法,先有蛋还是先有鸡?
> // SlistNode* next; 错误写法,
> }SLTNode;
> ```
>
>
> ```
> typedef struct SlistNode
> {
> SLTDataType data;
> struct SlistNode* next;
> }SLTNode,*PSLNode;
>
> ```
>
> SLTNode\*= PSLNode= struct SlistNode\*,这三个等价
>
>
>
### 打印链表
>
>
>
> ```
> void SListprint(SLTNode* phead)
> {
> //phead可能会为空这里不需要断言
> SLTNode* cur = phead;
> while (cur != NULL)
> {
> printf("%d->", cur->data);
> cur = cur->next;
> }
>
> }
> ```
>
> 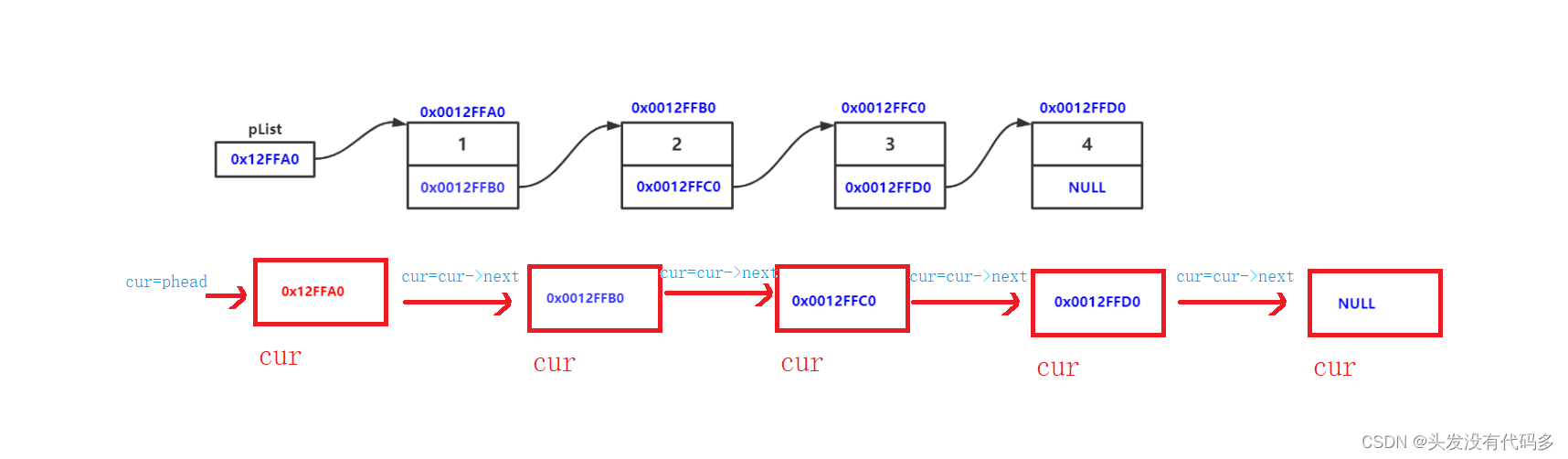
>
>
>
>
### 新节点的建立
>
>
> ```
> SLTNode* BuySLTnode(SLTDataType x)
> {
> SLTNode* newnode = (SLTNode*)malloc(sizeof(SLTNode));
> if (newnode == NULL)
> {
> perror("malloc fail");
> exit(-1);
> }
> newnode->data = x;
> newnode->next = NULL;
> return newnode;
> }
> ```
>
> 需要新节点的地方会很多,建立一个新的函数专门建立节点
>
>
>
### 头部插入
>
> 
>
>
> 创建新节点前,phead指向第一个节点
>
>
> 
>
>
>
> 创建新节点:先让newnode->next指向phead, phead指向第一个节点的地址,也就是说新节点通过phead拿到了原来第一个节点的地址
>
>
> 
>
>
> 然后让phead指向新节点地址
>
>
>
> ```
> void SListpushfront(SLTNode* phead, SLTDataType x)
> {
> SLTNode newnode;//错误写法
> SLTNode* newnode =(SLTNode*) malloc(sizeof(SLTNode));
> }
> ```
>
> 上面为错误代码不能用是因为:不能用局部节点,不然一出该函数就会被销毁,因此我们要用上面的函数来创建节点
>
>
> 方式1:
>
>
>
> ```
> void SListpushfront(SLTNode* phead, SLTDataType x)
> {
> SLTNode* newnode = BuySLTnode(x);
> newnode->next = phead;
> phead = newnode;
> }
> ```
>
> 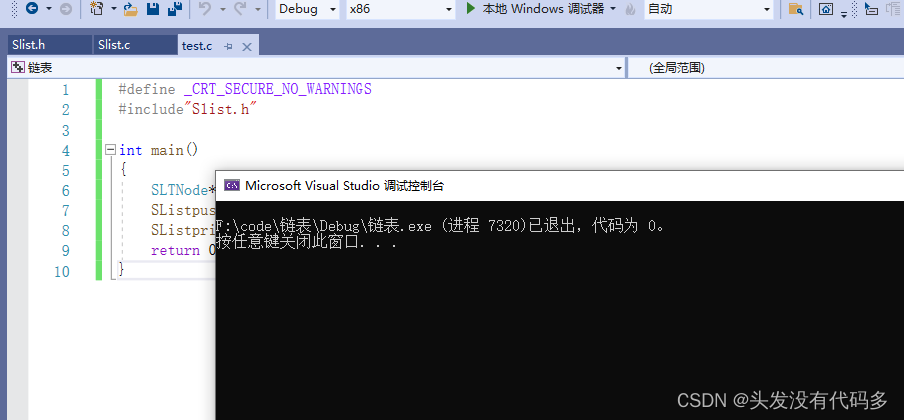
>
>
> 运行后无法打印,这是因为我们在传参的时候没有传地址 ,因此在出建立节点的函数的时候会对形参销毁
>
>
> 方式二,传地址
>
>
>
> ```
> SLTNode* plist=NULL;
> SListpushfront(&plist, 5);
> void SListpushfront(SLTNode**pphead, SLTDataType x)
> {
> SLTNode* newnode = BuySLTnode(x);
> newnode->next =*pphead;
> *pphead = newnode;
> }
> ```
>
> pphea存的是plist的地址,\*pphead的plist=等号右边的值,可参考下图,此时能正常打印。
>
>
> 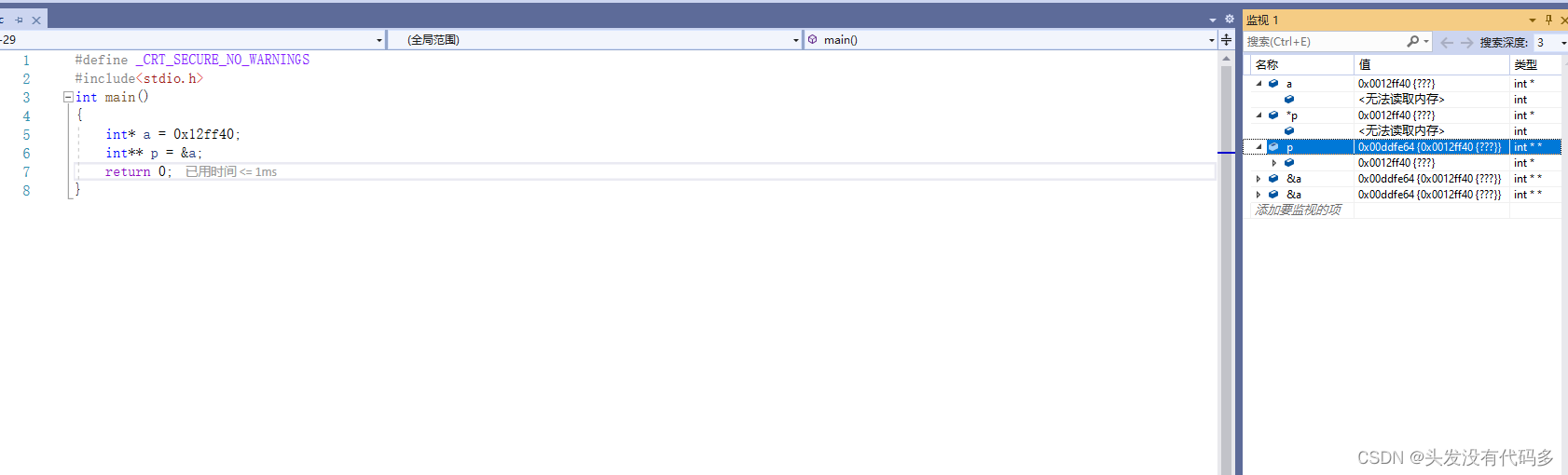
>
>
>
>
### 尾部插入
>
> 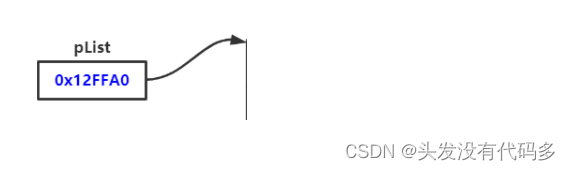
>
>
>
> 
>
>
> 先建立一个新的节点
>
>
> 分为俩种情况,第一种头节点指向空,第二种头节点指向不为空
>
>
> 第一种情况:头节点为空,建立新的节点后,直接让头节点指向新节点
>
>
> 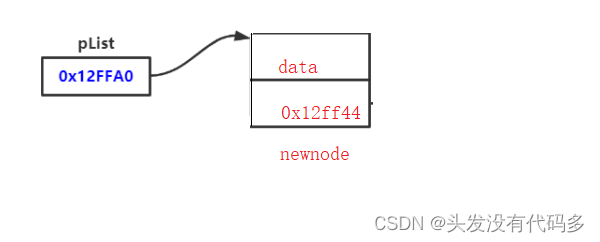
>
>
> 第二种情况,头节点不为空,创建一个跟节点类型相同的结构体变量,然后让这个变量指向头节点,之后检查后面的每个节点,若有一个节点为的next为空,则在此处插入新节点
>
>
> 
>
>
>
> tail发现这个节点的next指向为空,然后让这个节点的next指向下一个节点的地址,随着程序的结束tail也会随之消失
>
>
> 
>
>
>
> ```
> void SListpushback(SLTNode** pphead, SLTDataType x)
> {
> //pphead不可能为空,pphead是plist的地址,pphead永远不为空
> //1.链表为空
> SLTNode* newnode = BuySLTnode(x);
> if (*pphead == NULL)
> {
> *pphead = newnode;
> }
> //2.不为空
> else
> {
> SLTNode* tail = *pphead;
> while (tail->next != NULL)
> {
> tail =tail->next;
> }
> tail->next = newnode;
> }
> }
> ```
>
> 频繁的malloc会使效率降低
>
>
>
>
### 头部节点删除
>
> 
>
>
> 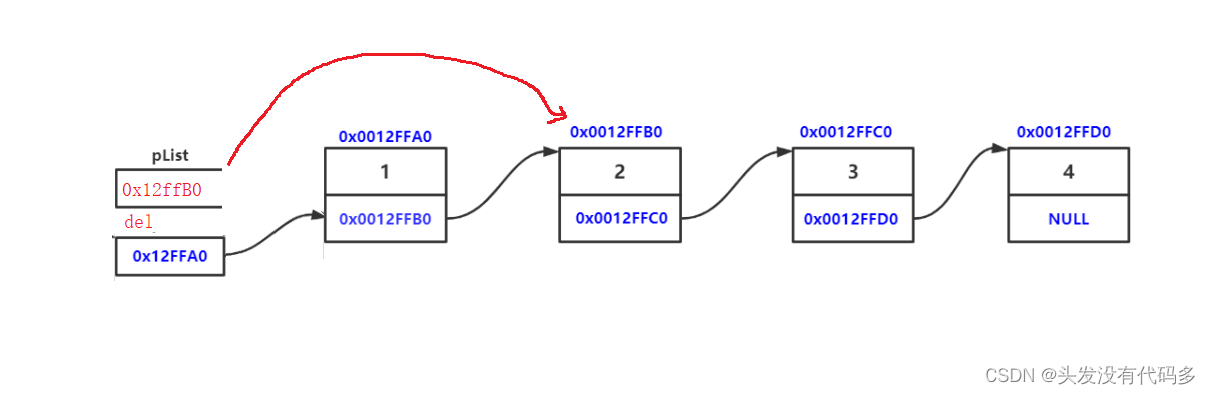 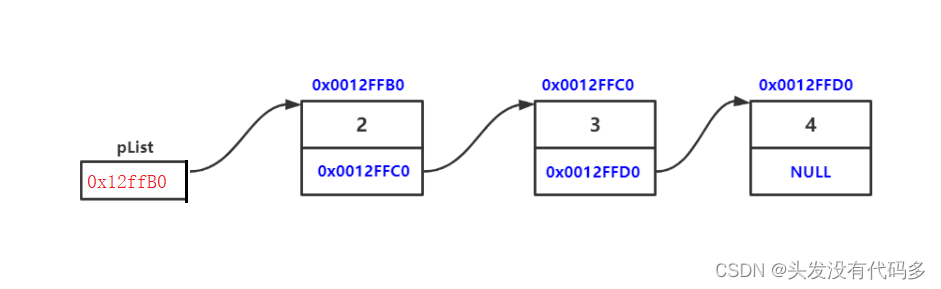
>
>
> 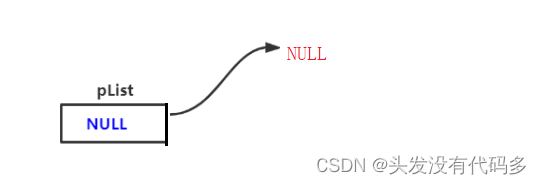
>
>
>
>
>
> ```
> void SListpopfront(SLTNode** pphead)//头删
> {
> SLTNode* del = *pphead;
> *pphead = (*pphead)->next;
> free(del);//删除第一个节点
> del = NULL;
> }
> ```
>
> 当全部删完后,plist指向NULL,此时就不能再删了。程序会崩溃,此时plsit为空,(\*pphead)->next也为空,del也为空,因此加一个检查条件
>
>
>
> ```
> void SListpopfront(SLTNode** pphead)//头删
> {
> if(*pphead==NULL)
> {
> return;
> }
> SLTNode* del = *pphead;
> *pphead = (*pphead)->next;
> free(del);//删除第一个节点
> del = NULL;
> }
> ```
>
> 或
>
>
>
> ```
> void SListpopfront(SLTNode** pphead)//头删
> {
> assert(*pphead!=NULL);
> SLTNode* del = *pphead;
> *pphead = (*pphead)->next;
> free(del);//删除第一个节点
> del = NULL;
> }
> ```
>
>
>
### 尾部节点删除
>
> 要判断尾部有一个节点还是多个节点甚至没有节点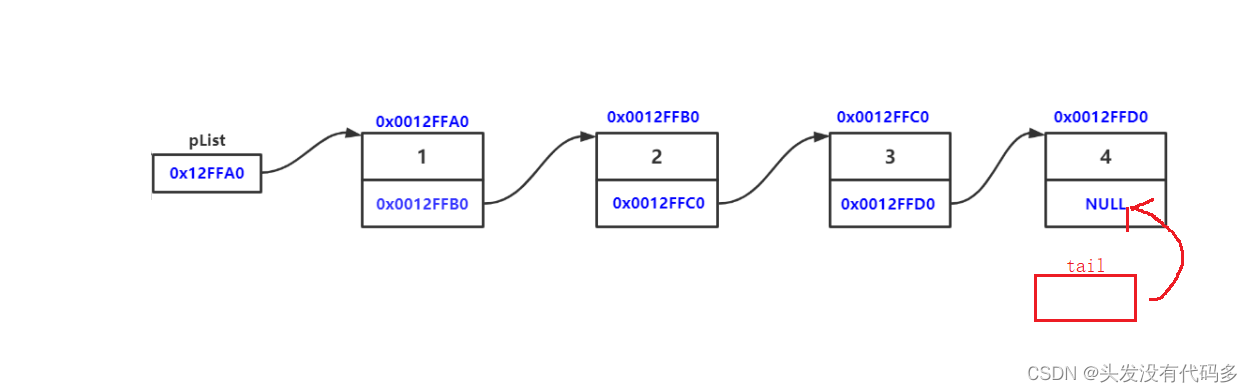
>
>
> 当tail->next为空,删除该节点,然后让前一个节点指向NULL
>
>
> 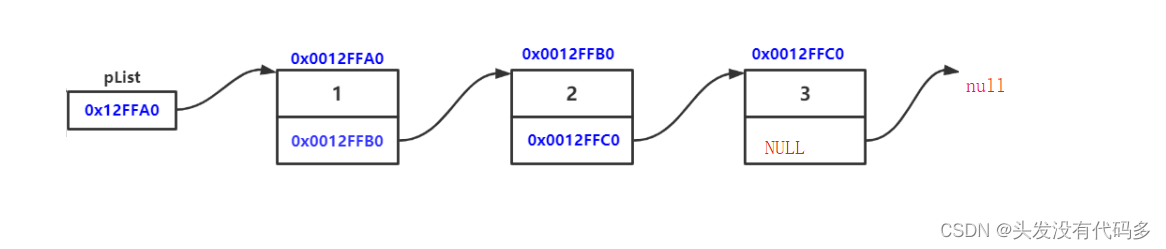
>
>
>
>
> ```
> void SListpopback(SLTNode** pphead)
> {
> if(*pphead==NULL)
> return;
> if((*pphead)->next==NULL)
> {
> free(*pphead);
> *pphead=NULL;
> }
> else
> {
> SLTNode* prev = NULL;
> SLTNode* tail = *pphead;
> while (tail->next!= NULL)
> {
> prev = tail;
> tail = tail->next;
> }
> prev->next = NULL;
> free(tail);
> tail = NULL;
> }
> }
> ```
>
> 或
>
>
> 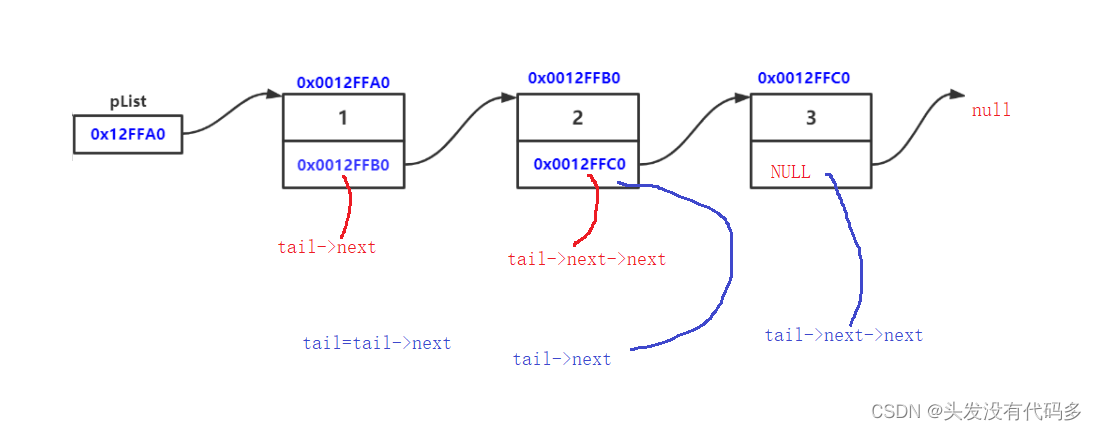
>
>
>
>
> ```
> void SListpopback(SLTNode** pphead)
> {
> if(*pphead==NULL)
> return;
> if((*pphead)->next==NULL)
> {
> free(*pphead);
> *pphead=NULL;
> }
> else
> {
>
> SLTNode* tail = *pphead;
> while (tail->next->next!= NULL)
> {
> tail = tail->next;
> }
> free(tail->next);
> tail->next = NULL;
> }
> }
> ```
>
>
>
### 节点的销毁
>
> 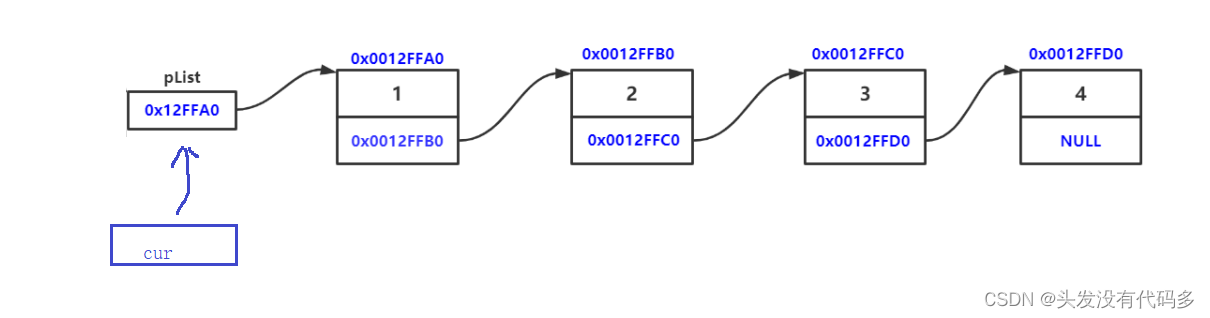 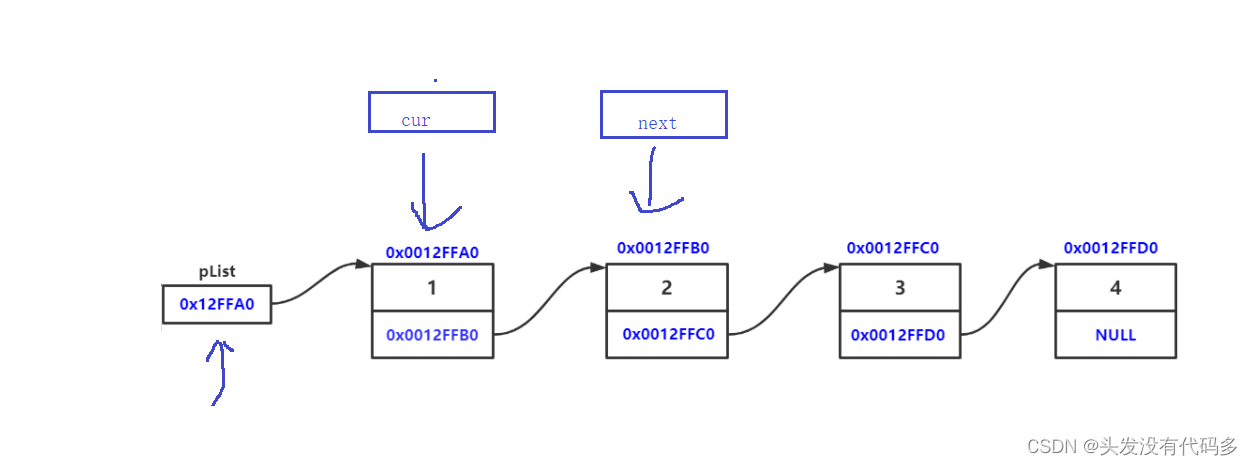
>
>
> 释放掉cur,cur=next ,不断往下走,最后把头节点置空
>
>
>
>
> ```
> void SListDestory(SLTNode** pphead)//用二级指针会更好
> {
>
> SLTNode* cur = *pphead;
> while (cur)
> {
> SLTNode* next = cur->next;
> free(cur);
> cur = next;
> }
> *pphead = NULL;
> }
> ```
>
>
>
### 链表查找
>
>
>
> ```
> SLTNode *SListFind(SLTNode** pphead, SLTDataType x)
> {
> SLTNode* cur = *pphead;
> while (cur != NULL)
> {
> if (cur->data == x)
> {
> return cur;
> }
> cur = cur->next;
> }
> return NULL;
> }
> ```
>
> 挨个查找。
>
>
>
### 随即插入
>
>
> ```
> void SListInsert(SLTNode** pphead, SLTNode* pos, SLTDataType x)
> {
> assert(pos);
> if (pos == *pphead)
> {
> SListpushfront(pphead, x);
> }
> else
> {
> SLTNode* prve = *pphead;
> while (prve->next != pos)
> {
> prve = prve->next;
> assert(prve);//找不到
> }
> SLTNode* newnode = BuySLTnode(x);
> prve->next = newnode;
> newnode->next = pos;
> }
> }
> ```
>
> 从一个数的前面插入,若果头插则用头插函数
>
>
>
### 后插
>
>
> ```
> void SListInsertAfter(SLTNode** pphead, SLTNode* pos, SLTDataType x)
> {
> SLTNode* newnode = BuySLTnode(x);
> newnode->next = pos->next;
> pos->next = newnode;
> }
> ```
>
>
>
### 删除某节点前面的节点
>
>
>
> ```
> void SlistErase(SLTNode** pphead, SLTNode* pos)
> {
> assert(pos);
> if (*pphead == pos)
> {
> SListpopfront(pphead);
> }//如果是头删,用头删函数
> else
> {
> SLTNode* prev = *pphead;
> if (prev->next != pos)
> {
> prev = prev->next;
> assert(prev);//判断pos是否属于本链表
> }
> prev->next = pos->next;
> free(pos);
> pos = NULL;
> }
> }
> ```
>
>
>
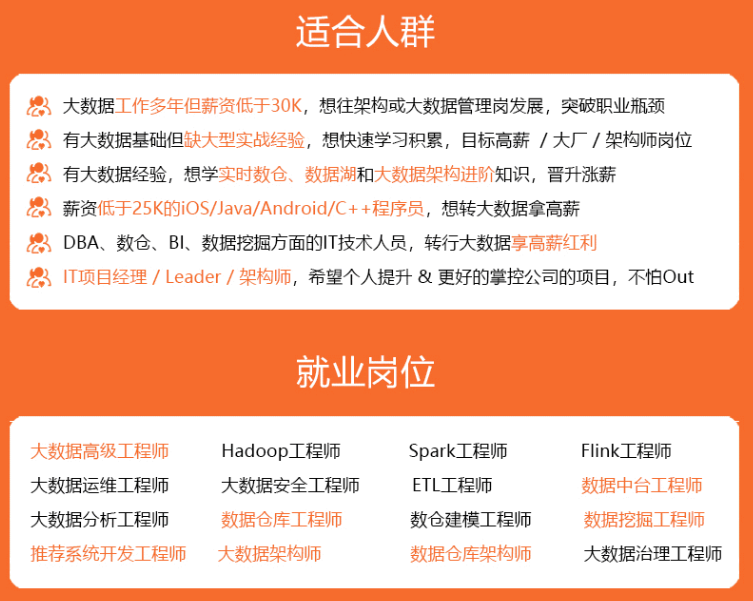
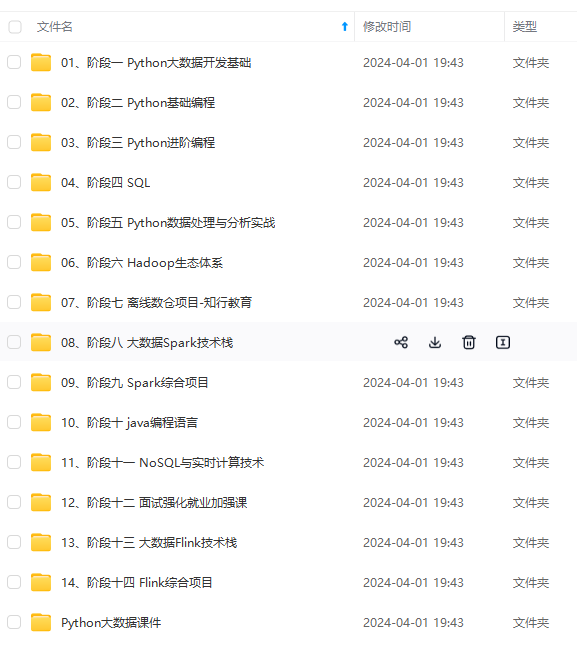
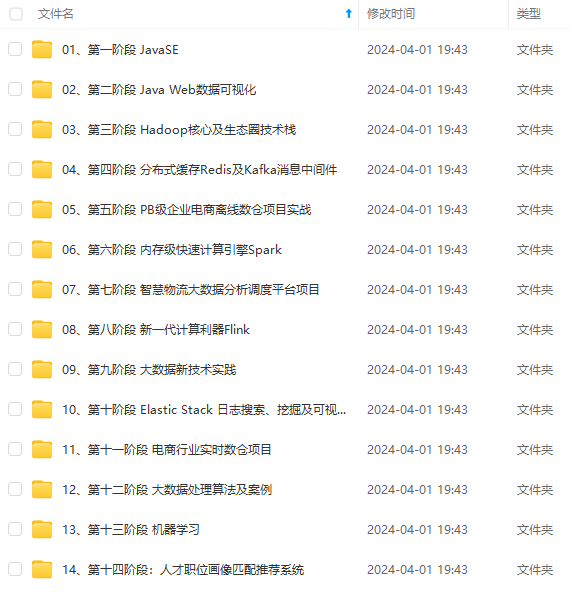
**既有适合小白学习的零基础资料,也有适合3年以上经验的小伙伴深入学习提升的进阶课程,涵盖了95%以上大数据知识点,真正体系化!**
**由于文件比较多,这里只是将部分目录截图出来,全套包含大厂面经、学习笔记、源码讲义、实战项目、大纲路线、讲解视频,并且后续会持续更新**
**[需要这份系统化资料的朋友,可以戳这里获取](https://bbs.csdn.net/forums/4f45ff00ff254613a03fab5e56a57acb)**
{
> SListpopfront(pphead);
> }//如果是头删,用头删函数
> else
> {
> SLTNode* prev = *pphead;
> if (prev->next != pos)
> {
> prev = prev->next;
> assert(prev);//判断pos是否属于本链表
> }
> prev->next = pos->next;
> free(pos);
> pos = NULL;
> }
> }
> ```
>
>
>
[外链图片转存中...(img-Vsm1bBDK-1715292624083)]
[外链图片转存中...(img-0fxjfnNV-1715292624083)]
[外链图片转存中...(img-hXC5qPiX-1715292624084)]
**既有适合小白学习的零基础资料,也有适合3年以上经验的小伙伴深入学习提升的进阶课程,涵盖了95%以上大数据知识点,真正体系化!**
**由于文件比较多,这里只是将部分目录截图出来,全套包含大厂面经、学习笔记、源码讲义、实战项目、大纲路线、讲解视频,并且后续会持续更新**
**[需要这份系统化资料的朋友,可以戳这里获取](https://bbs.csdn.net/forums/4f45ff00ff254613a03fab5e56a57acb)**