大致效果如下
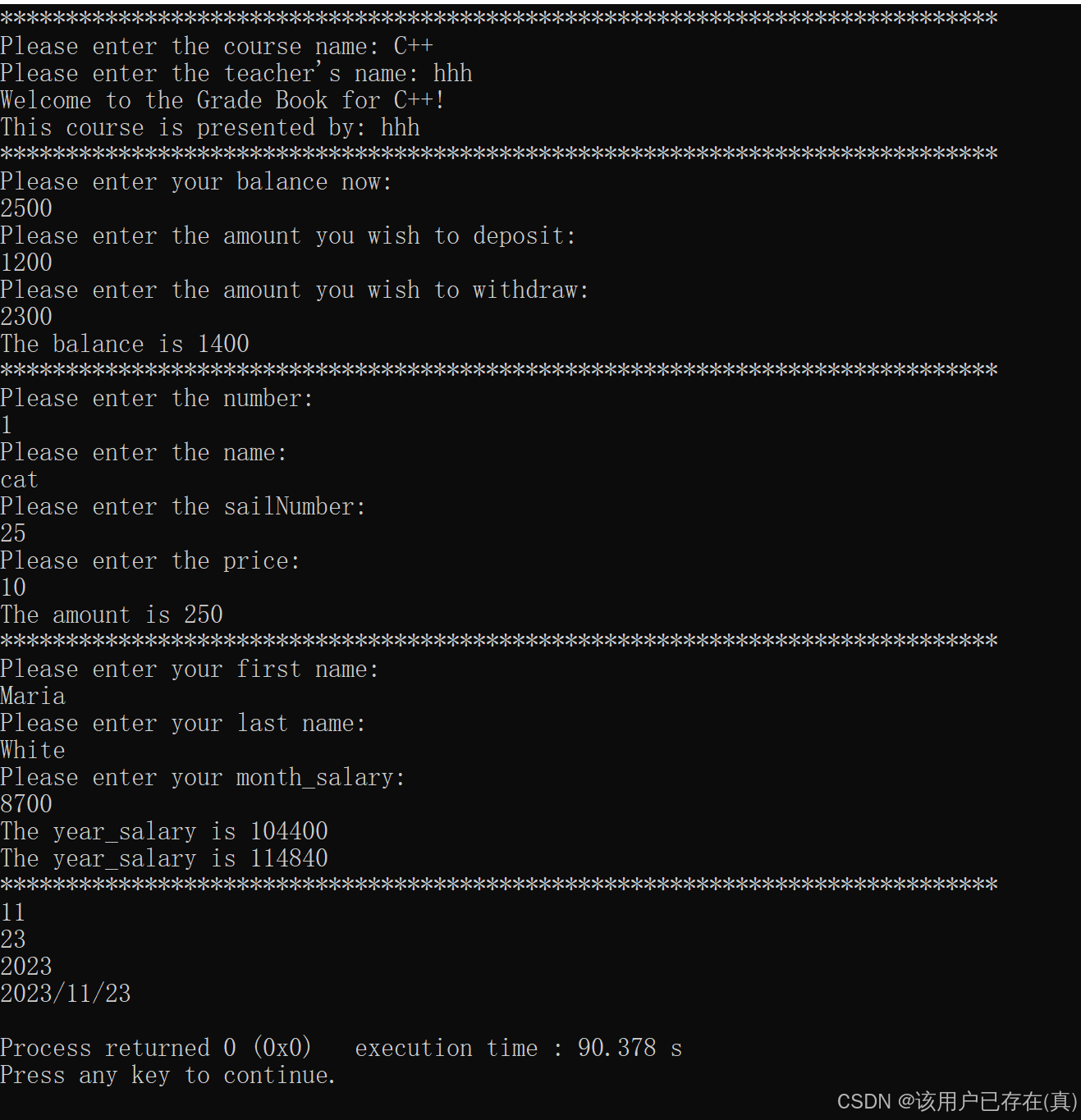
GradeBook.h
/**
* 打印课程及任课教师
*/
#ifndef GRADEBOOK
#define GRADEBOOK
#include <string>
using namespace std;
class GradeBook
{
public:
GradeBook();
GradeBook(string n1,string n2);
~GradeBook();
void setCourseName(string n);
void setTeacherName(string n);
string getCourseName();
string getTeacherName();
void displayMessage();
private:
string courseName;
string teacherName;
};
#endif // GRADEBOOK
GradeBook.cpp
#include "GradeBook.h"
#include <iostream>
#include <string>
using namespace std;
GradeBook::GradeBook()
{
}
GradeBook::GradeBook(string n1,string n2)
{
courseName = n1;
teacherName = n2;
}
GradeBook::~GradeBook()
{
}
void GradeBook::setCourseName(string n)
{
this->courseName = n;
}
void GradeBook::setTeacherName(string n)
{
this->teacherName = n;
}
string GradeBook::getCourseName()
{
return courseName;
}
string GradeBook::getTeacherName()
{
return teacherName;
}
void GradeBook::displayMessage()
{
cout << "Welcome to the Grade Book" << " for " << courseName << "!" << endl;
cout << "This course is presented by: " << teacherName << endl;
}
Account.h
/**
* 返回余额
* void credit(int a);//存钱后的余额
* void debit(int b);//取钱后的余额
*/
#ifndef ACCOUNT
#define ACCOUNT
class Account
{
public:
Account();
Account(int b);
~Account();
void setBalance(int b);
int getBalance();
void credit(int a);
void debit(int b);
private:
int balance;
};
#endif // ACCOUNT
Account.cpp
#include "Account.h"
#include <iostream>
using namespace std;
Account::Account()
{
}
Account::Account(int b)
{
if(b < 0)
{
cout << "The balance is invalid." << endl;
balance = 0;
}
else
balance = b;
}
Account::~Account()
{
}
// void Account::setBalance(int b)
// {
// balance = b;
// }
//
int Account::getBalance()
{
return balance;
}
void Account::credit(int a)
{
balance += a;
}
void Account::debit(int b)
{
balance -= b;
}
Invoice.h
/**
* 返回总售价
*
*/
#ifndef INVOICE
#define INVOICE
#include <iostream>
#include <string>
using namespace std;
class Invoice
{
public:
Invoice();
Invoice(string a,string b,int c,int d);
~Invoice();
void setNumber(string a);
void setName(string b);
void setSailNumber(int c);
void setPrice(int d);
string getNumber();
string getName();
int getSailNumber();
int getPrice();
int getInvoiceAmount();
private:
string number;//商品号
string name;//商品描述
int sailNumber;//出售数量
int price;//单价
};
#endif // INVOICE
Invoice.cpp
#include "Invoice.h"
#include <iostream>
#include <string>
using namespace std;
Invoice::Invoice()
{
}
Invoice::Invoice(string a,string b,int c,int d)
{
number = a;
name = b;
sailNumber = c;
price = d;
}
Invoice::~Invoice()
{
}
void Invoice::setNumber(string a)
{
number = a;
}
void Invoice::setName(string b)
{
name = b;
}
void Invoice::setSailNumber(int c)
{
sailNumber = c;
}
void Invoice::setPrice(int d)
{
price = d;
}
string Invoice::getNumber()
{
return number;
}
string Invoice::getName()
{
return name;
}
int Invoice::getSailNumber()
{
return sailNumber;
}
int Invoice::getPrice()
{
return price;
}
int Invoice::getInvoiceAmount()
{
if(sailNumber < 0)
{
cout << "The sailNumber is 0" << endl;
return 0;
}
if(price < 0)
{
cout << "The price is 0" << endl;
return 0;
}
else
return sailNumber * price;
}
Employee.h
/**
*
* 返回年薪
*/
#ifndef EMPLOYEE
#define EMPLOYEE
#include <iostream>
using namespace std;
class Employee
{
public:
Employee();
Employee(string fn,string ln,int s);
~Employee();
void setFirstName(string fn);
void setLastName(string ln);
void setMonthSalary(int s);
string getFirstName();
string getLastName();
int getMonthSalary();
void compute_year_salary();
void compute_newYear_salary();
private:
string first_name;
string last_name;
int month_salary;
};
#endif // EMPLOYEE
Employee.cpp
#include "Employee.h"
#include <iostream>
using namespace std;
Employee::Employee()
{
}
Employee::Employee(string fn,string ln,int s):first_name(fn),last_name(ln)
{
setMonthSalary(s);
}
Employee::~Employee()
{
}
void Employee::setFirstName(string fn)
{
first_name = fn;
}
void Employee::setLastName(string ln)
{
last_name = ln;
}
void Employee::setMonthSalary(int s)
{
if(s < 0)
{
month_salary = 0;
}
else
month_salary = s;
}
string Employee::getFirstName()
{
return first_name;
}
string Employee::getLastName()
{
return last_name;
}
int Employee::getMonthSalary()
{
return month_salary;
}
void Employee::compute_year_salary()
{
cout << "The year_salary is " << month_salary * 12 << endl;
}
void Employee::compute_newYear_salary()
{
month_salary *= (1 + 0.1) ;
compute_year_salary();
}
Date.h
/**
* 打印年/月/日
*
*/
#ifndef DATE
#define DATE
class Date
{
public:
Date();
Date(int m,int d,int y);
~Date();
void setMonth(int m);
void setDay(int d);
void setYear(int y);
int getMonth();
int getDay();
int getYear();
void print();
private:
int month;
int day;
int year;
};
#endif // DATE
Date.cpp
#include "Date.h"
#include <iostream>
using namespace std;
Date::Date()
{
}
Date::Date(int m,int d,int y):day(d),year(y)
{
setMonth(m);
}
Date::~Date()
{
}
void Date::setMonth(int m)
{
if(m >= 1 && m <= 12)
{
month = m;
}
else
month = 1;
}
void Date::setDay(int d)
{
day = d;
}
void Date::setYear(int y)
{
year = y;
}
int Date::getMonth()
{
return month;
}
int Date::getDay()
{
return day;
}
int Date::getYear()
{
return year;
}
void Date::print()
{
cout << getYear() << "/" << getMonth() << "/" << getDay() << endl;
}
main.cpp
#include <iostream>
#include "GradeBook.h"
#include "Account.h"
#include "Invoice.h"
#include "Employee.h"
#include "Date.h"
#include <string>
using namespace std;
int main()
{
cout << "****************************************************************************" << endl;
GradeBook gradeBook;
string n1;
//cout << "Initial course name is: " << gradeBook.getCourseName() << endl;
cout << "Please enter the course name: ";
getline(cin,n1);
gradeBook.setCourseName(n1);
string n2;
cout << "Please enter the teacher's name: ";
getline(cin,n2);
gradeBook.setTeacherName(n2);
gradeBook.displayMessage();
cout << "****************************************************************************" << endl;
int w;
cout << "Please enter your balance now: " << endl;
cin >> w;
Account a(w);
int m;
cout << "Please enter the amount you wish to deposit: " << endl;
cin >> m;
a.credit(m);
int t;
cout << "Please enter the amount you wish to withdraw: " << endl;
cin >> t;
a.debit(t);
cout << "The balance is " << a.getBalance() << endl;//25 + 10 - 30
cout << "****************************************************************************" << endl;
string n;
cout << "Please enter the number: " << endl;
cin >> n;
string b;
cout << "Please enter the name: " << endl;
cin >> b;
int c;
cout << "Please enter the sailNumber: " << endl;
cin >> c;
int d;
cout << "Please enter the price: " << endl;
cin >> d;
Invoice invoice(n,b,c,d);
cout << "The amount is " << invoice.getInvoiceAmount() << endl;
cout << "****************************************************************************" << endl;
string f;
cout << "Please enter your first name: " << endl;
cin >> f;
string l;
cout << "Please enter your last name: " << endl;
cin >> l;
int s;
cout << "Please enter your month_salary: " << endl;
cin >> s;
Employee em(f,l,s);
em.compute_year_salary();
em.compute_newYear_salary();
cout << "****************************************************************************" << endl;
int month;
int day;
int year;
cin >> month >> day >> year;
Date date(month,day,year);
date.print();
return 0;
}