最后
现在其实从大厂招聘需求可见,在招聘要求上有高并发经验优先,包括很多朋友之前都是做传统行业或者外包项目,一直在小公司,技术搞的比较简单,没有怎么搞过分布式系统,但是现在互联网公司一般都是做分布式系统。
所以说,如果你想进大厂,想脱离传统行业,这些技术知识都是你必备的,下面自己手打了一份Java并发体系思维导图,希望对你有所帮助。
}
public static void main(String[] args) throws Exception
{
// Specify the size of the ring buffer, must be power of 2.
int bufferSize = 1024;
// Construct the Disruptor
Disruptor<TradeEvent> disruptor = new Disruptor<>(TradeEvent::new, bufferSize, DaemonThreadFactory.INSTANCE);
// Connect the handler
disruptor.handleEventsWith(LongEventMain::handleEvent);
// Start the Disruptor, starts all threads running
disruptor.start();
// Get the ring buffer from the Disruptor to be used for publishing.
RingBuffer<TradeEvent> ringBuffer = disruptor.getRingBuffer();
ByteBuffer bb = ByteBuffer.allocate(4);
for (int l = 0; true; l++)
{
bb.putInt(0, l);
ringBuffer.publishEvent(LongEventMain::translate, bb);
Thread.sleep(1000);
}
}
}
### 多个消费者
* **串行**
消费者之间必须等待上一个执行完成才能继续

需求:C1、添加订单号;C2、记录订单(入库);C3、打印订单详情
代码实现:
public class LongEventMain1 {
public static void handleEvent1(TradeEvent event, long sequence, boolean endOfBatch) {
event.setId(UUID.randomUUID().toString());
}
public static void handleEvent2(TradeEvent event, long sequence, boolean endOfBatch) {
System.out.println(String.format("订单号:%s,入库成功!!", event.getId()));
}
public static void handleEvent(TradeEvent event, long sequence, boolean endOfBatch) {
System.out.println(event);
}
public static void translate(TradeEvent event, long sequence, ByteBuffer buffer) {
event.setUserId(buffer.getInt(0));
}
public static void main(String[] args) throws Exception {
// Specify the size of the ring buffer, must be power of 2.
int bufferSize = 1024;
// Construct the Disruptor
Disruptor<TradeEvent> disruptor = new Disruptor<>(TradeEvent::new, bufferSize, DaemonThreadFactory.INSTANCE);
// Connect the handler
disruptor.handleEventsWith(LongEventMain1::handleEvent1)
.handleEventsWith(LongEventMain1::handleEvent2)
.handleEventsWith(LongEventMain1::handleEvent);
// Start the Disruptor, starts all threads running
disruptor.start();
// Get the ring buffer from the Disruptor to be used for publishing.
RingBuffer<TradeEvent> ringBuffer = disruptor.getRingBuffer();
ByteBuffer bb = ByteBuffer.allocate(8);
for (int l = 0; true; l++) {
bb.putInt(0, l);
ringBuffer.publishEvent(LongEventMain1::translate, bb);
Thread.sleep(1000);
}
}
}
* **并行**
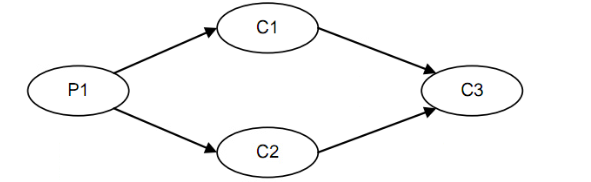
需求:
C1、添加订单状态
C2、添加订单名称
C3、打印订单详情
代码实现:
import com.lmax.disruptor.RingBuffer;
import com.lmax.disruptor.dsl.Disruptor;
import com.lmax.disruptor.util.DaemonThreadFactory;
import java.nio.ByteBuffer;
import java.util.Random;
import java.util.UUID;
public class LongEventMain2 {
private static Random random = new Random();
public static void handleEvent1(TradeEvent event, long sequence, boolean endOfBatch) {
event.setId(UUID.randomUUID().toString());
try {
int i = random.nextInt(10);
Thread.sleep(i);
} catch (InterruptedException e) {
e.printStackTrace();
}
System.out.println("====ID====");
}
public static void handleEvent2(TradeEvent event, long sequence, boolean endOfBatch) {
event.setName("order:"+event.getUserId());
try {
int i = random.nextInt(10);
Thread.sleep(i);
} catch (InterruptedException e) {
e.printStackTrace();
}
System.out.println("====Name====");
}
public static void handleEvent(TradeEvent event, long sequence, boolean endOfBatch) {
System.out.println(event);
}
public static void translate(TradeEvent event, long sequence, ByteBuffer buffer) {
event.setUserId(buffer.getInt(0));
}
public static void main(String[] args) throws Exception {
// Specify the size of the ring buffer, must be power of 2.
int bufferSize = 1024;
// Construct the Disruptor
Disruptor<TradeEvent> disruptor = new Disruptor<>(TradeEvent::new, bufferSize, DaemonThreadFactory.INSTANCE);
// Connect the handler
disruptor.handleEventsWith(LongEventMain2::handleEvent1,LongEventMain2::handleEvent2)
.then(LongEventMain2::handleEvent);
// Start the Disruptor, starts all threads running
disruptor.start();
// Get the ring buffer from the Disruptor to be used for publishing.
RingBuffer<TradeEvent> ringBuffer = disruptor.getRingBuffer();
ByteBuffer bb = ByteBuffer.allocate(8);
for (int l = 0; true; l++) {
bb.putInt(0, l);
ringBuffer.publishEvent(LongEventMain2::translate, bb);
Thread.sleep(1000);
}
}
}
* **并行加串行**
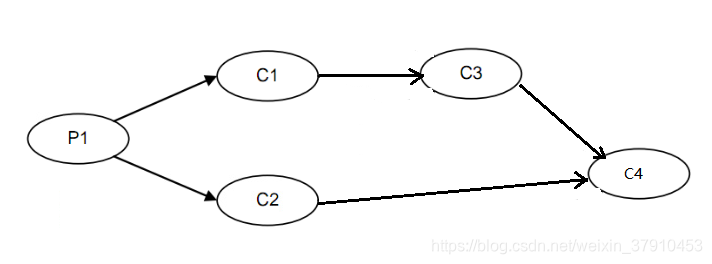
需求:
C1、生成订单号
C2、生成订单名称
C3、记录订单号的状态
C4、打印订单详情
代码实现:
import com.lmax.disruptor.EventHandler;
import com.lmax.disruptor.RingBuffer;
import com.lmax.disruptor.dsl.Disruptor;
import com.lmax.disruptor.util.DaemonThreadFactory;
import java.nio.ByteBuffer;
import java.util.Random;
import java.util.UUID;
public class LongEventMain3 {
private static Random random = new Random();
public static void handleEvent1(TradeEvent event, long sequence, boolean endOfBatch) {
event.setId(UUID.randomUUID().toString());
try {
int i = random.nextInt(10);
Thread.sleep(i);
} catch (InterruptedException e) {
e.printStackTrace();
}
System.out.println("====ID====");
}
public static void handleEvent3(TradeEvent event, long sequence, boolean endOfBatch) {
event.setStatus(event.getId().hashCode()%2);
System.out.println("====Status====");
}
public static void handleEvent2(TradeEvent event, long sequence, boolean endOfBatch) {
event.setName("order:"+event.getUserId());
try {
int i = random.nextInt(10);
Thread.sleep(i);
} catch (InterruptedException e) {
e.printStackTrace();
}
总结:心得体会
既然选择这个行业,选择了做一个程序员,也就明白只有不断学习,积累实战经验才有资格往上走,拿高薪,为自己,为父母,为以后的家能有一定的经济保障。
学习时间都是自己挤出来的,短时间或许很难看到效果,一旦坚持下来了,必然会有所改变。不如好好想想自己为什么想进入这个行业,给自己内心一个答案。
面试大厂,最重要的就是夯实的基础,不然面试官随便一问你就凉了;其次会问一些技术原理,还会看你对知识掌握的广度,最重要的还是你的思路,这是面试官比较看重的。
最后,上面这些大厂面试真题都是非常好的学习资料,通过这些面试真题能够看看自己对技术知识掌握的大概情况,从而能够给自己定一个学习方向。包括上面分享到的学习指南,你都可以从学习指南里理顺学习路线,避免低效学习。
大厂Java架构核心笔记(适合中高级程序员阅读):
iZ09EPaX-1715297897524)]