203
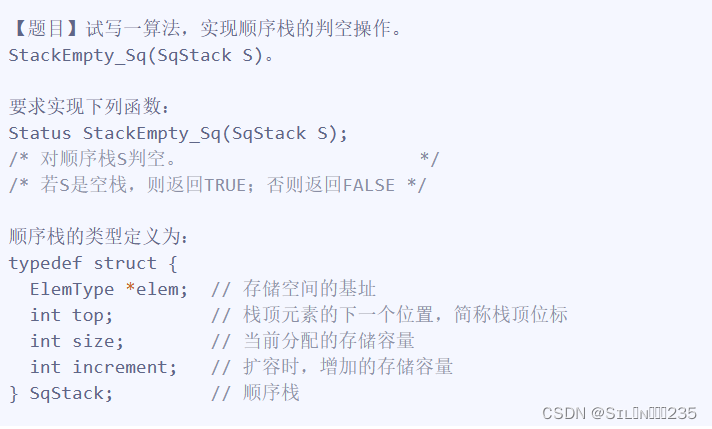
#include "allinclude.h" //DO NOT edit this line
Status StackEmpty_Sq(SqStack S)
{
// Add your code here
if(S.top==NULL)
{
return TRUE;
}
else {
return FALSE;
}
}
205
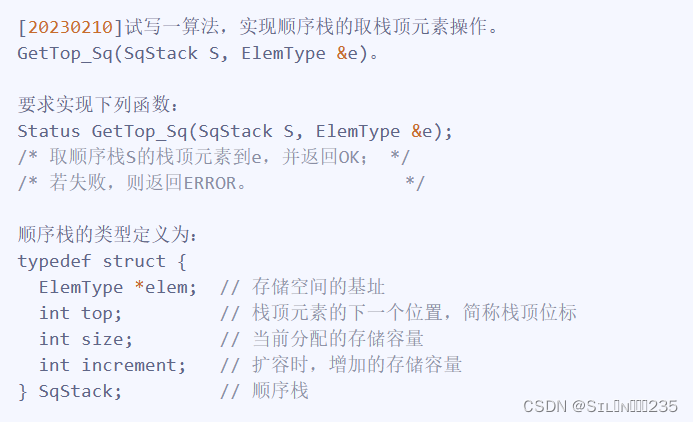
#include "allinclude.h" //DO NOT edit this line
Status GetTop_Sq(SqStack S, ElemType &e)
{// Add your code here
if(S.top==NULL)
{
return ERROR;
}
else{
e=S.elem[S.top-1];
return OK;
}
}
207
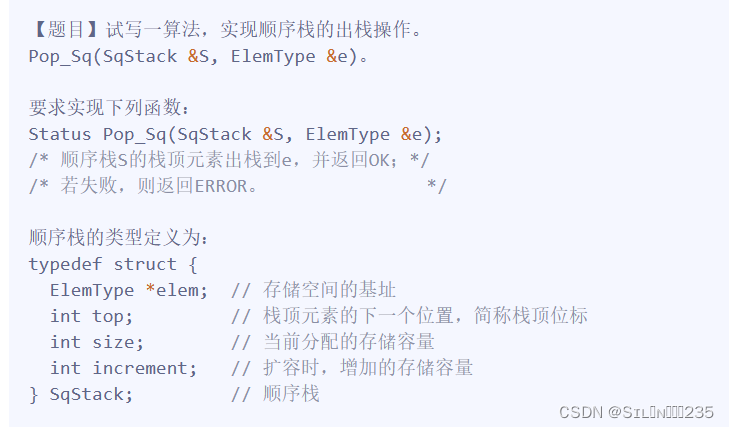
#include "allinclude.h" //DO NOT edit this line
Status Pop_Sq(SqStack &S, ElemType &e) {
// Add your code here
if(S.top==NULL)
{
return ERROR;
}
else
{
e=S.elem[S.top-1];
--S.top;
return OK;
}
}
211
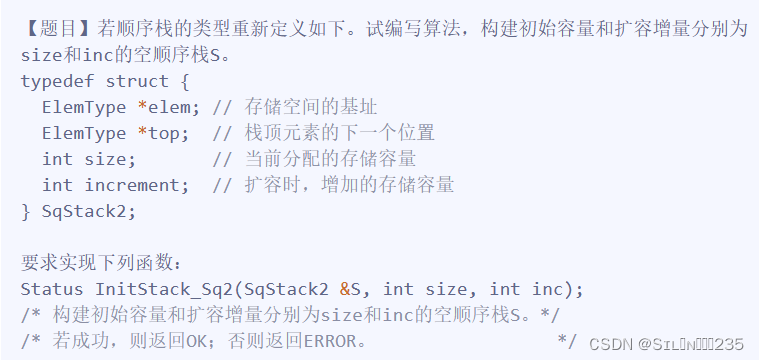
#include "allinclude.h" //DO NOT edit this line
Status InitStack_Sq2(SqStack2 &S, int size, int inc) {
// Add your code here
S.elem=(ElemType*)malloc(size*sizeof(ElemType));//分配存储空间
if(S.elem==NULL||inc<=0||size<=0)
{
return ERROR;//不成功的情况
}
S.top=S.elem;//置S为空栈
S.size=size;//初始容量值
S.increment=inc;//初始增量值
return OK;
}
213
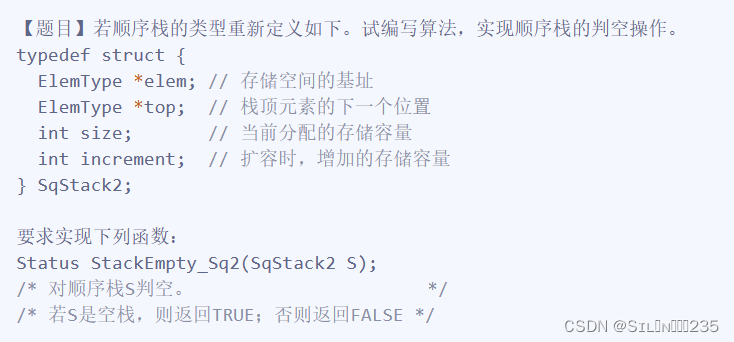
#include "allinclude.h" //DO NOT edit this line
Status StackEmpty_Sq2(SqStack2 S)
{
// Add your code here
if(S.top==S.elem)
{
return TRUE;
}
else
{
return FALSE;
}
}
215
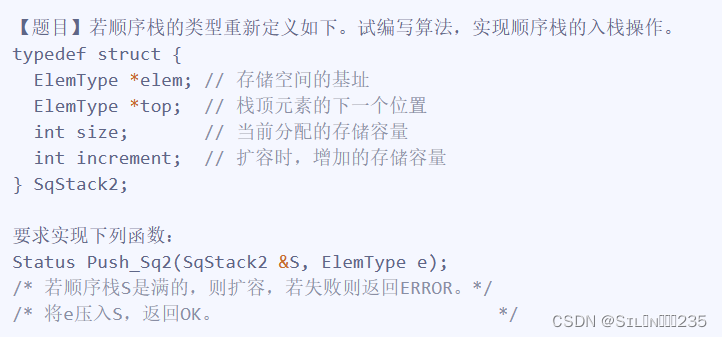
#include "allinclude.h" //DO NOT edit this line
Status Push_Sq2(SqStack2 &S, ElemType e) {
// Add your code here
/*if(S.top>=S.elem+S.size)
{
S.elem=(ElemType*)realloc(S.elem,(S.size+1)*sizeof(ElemType));
if(NULL==S.elem)
{
return ERROR;
}
S.size+=1;
}
*(S.top++)=e;
return OK;*/
if(S.top>=S.elem+S.size)
{
S.elem=(ElemType*)realloc(S.elem,(S.size+S.increment)*sizeof(ElemType));
if(NULL==S.elem)
{
return ERROR;
}
S.size+=S.increment;
}
*(S.top++)=e;
return OK;
}
217
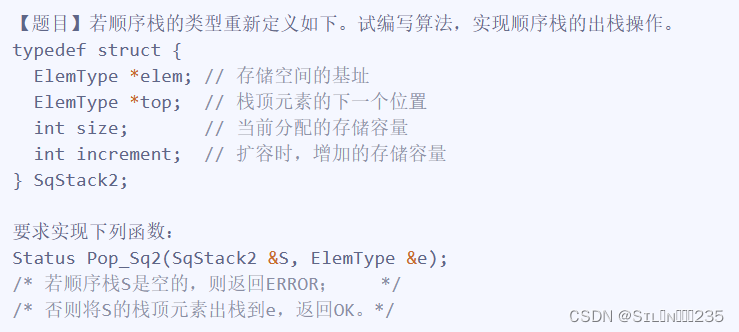
#include "allinclude.h" //DO NOT edit this line
Status Pop_Sq2(SqStack2 &S, ElemType &e) {
// Add your code here
//S.elem=(ElemType*)malloc(S.size*sizeof(ElemType));
if(S.elem==S.top)
{
return ERROR;
}
e=*(S.top-1);
S.top--;
return OK;
}
219
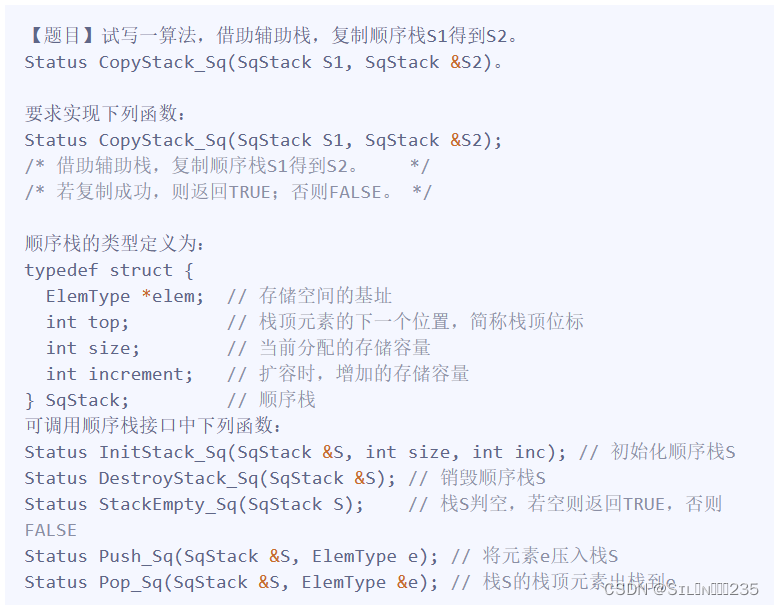
#include "allinclude.h" //DO NOT edit this line
Status CopyStack_Sq(SqStack S1, SqStack &S2) {
// Add your code here
S2.elem=(ElemType*)malloc(S1.size*sizeof(ElemType));
if(StackEmpty_Sq(S1))
{
S2.top=0;
return TRUE;
}
else {
S2.elem=S1.elem;
S2.top=S1.top;
}
return TRUE;
}
220
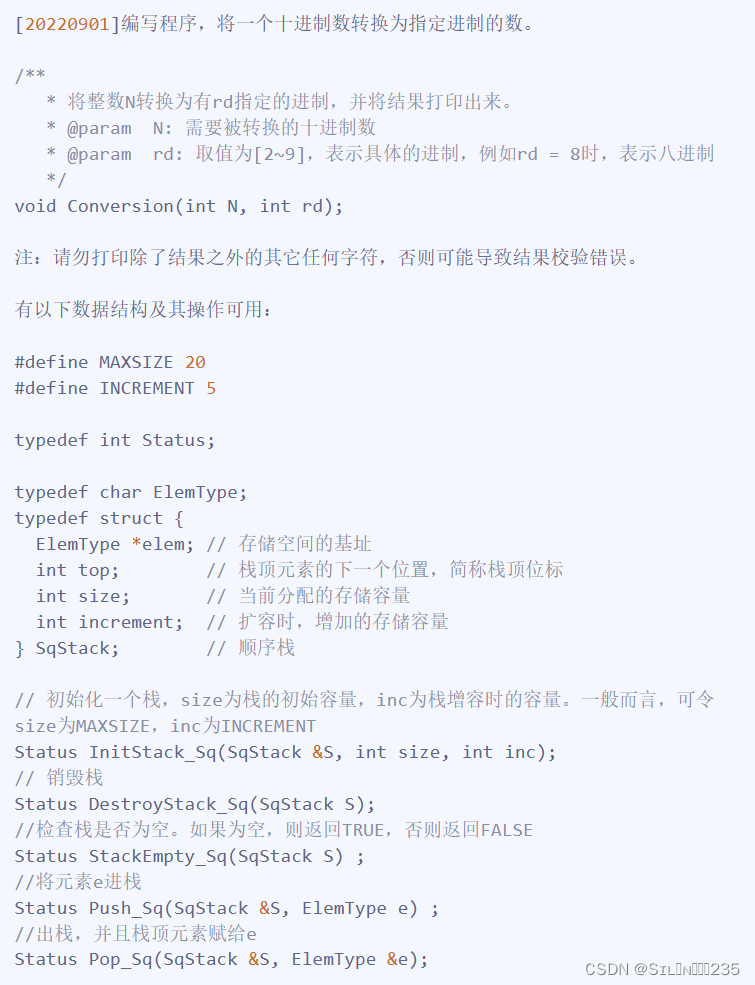
#include "allinclude.h"
void Conversion(int N, int rd)
{ // Add your code here
SqStack S;
ElemType e;
InitStack_Sq(S,10,5);//栈S的初始容量为10
while(N!=0){
Push_Sq(S,N%rd);//将N除以8的余数入栈
N/=rd;//N取值为其除以8的商
}
while(FALSE==StackEmpty_Sq(S)){//依次输出栈的余数
Pop_Sq(S,e);
printf("%d",e);
}
}
221
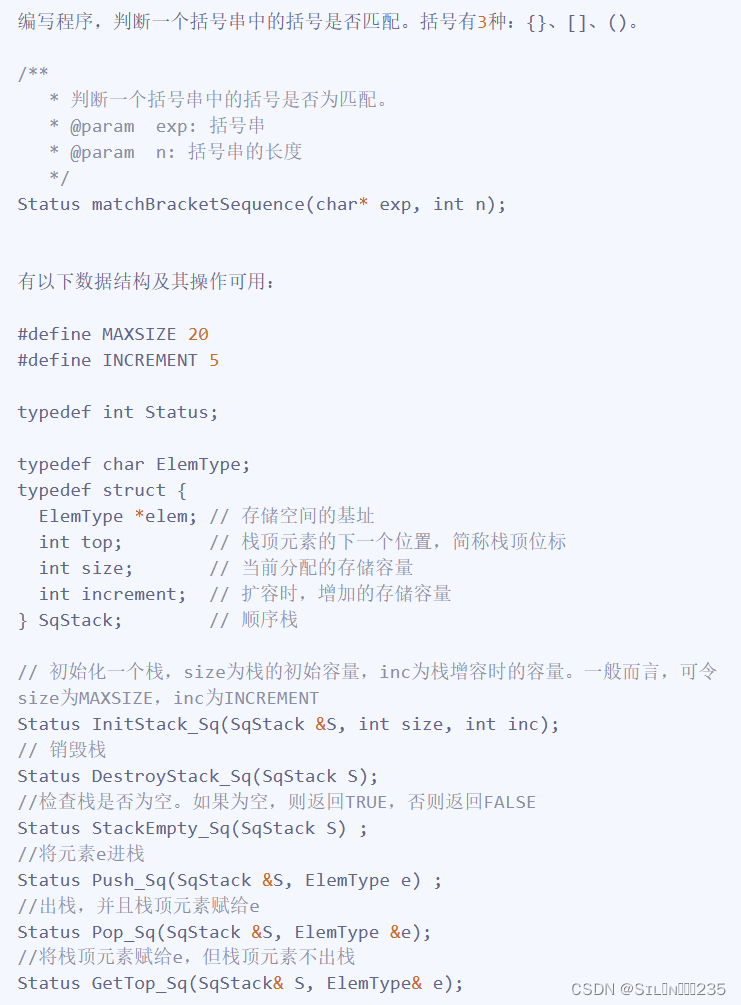
#include "allinclude.h"
Status matchBracketSequence(char* exp, int n)
{ // Add your code here
int i=0;
ElemType e;//基址
SqStack S;//设置栈S
InitStack_Sq(S,n,INCREMENT);//设置S为空栈
while(i<n)//循环
{
switch(exp[i])//分支语句判断括号类型
{
case '(':
case '[':
case '{':
Push_Sq(S,exp[i]);//将前括号放入栈
i++;
break;
case ')':
case ']':
case '}':
if(TRUE==StackEmpty_Sq(S))//判断是否有前括号
{
return ERROR;
}
else
{
GetTop_Sq(S,e);//取出最后放入的前括号
if((exp[i]==')'&&e=='(')||(exp[i]==']'&&e=='[')||(exp[i]=='}'&&e=='{'))//判断是否匹配
{
Pop_Sq(S,e);//将匹配上的前括号出栈
i++;
}
else
{
return ERROR;
}
}
break;
}
}
if(TRUE==StackEmpty_Sq(S))//判断栈是否为空栈,是则全部括号对应上
{
return OK;
}
else//否则存在前括号没有对应的后括号,使得前括号无法出栈
{
return ERROR;
}
}
223
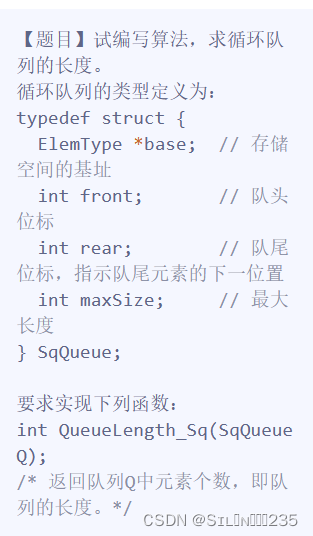
#include "allinclude.h" //DO NOT edit this line
int QueueLength_Sq(SqQueue Q) {
// Add your code here
int i=0;
//①
/*while(Q.rear!=Q.front)
{
if(Q.front==Q.maxSize-1)
{
Q.front=0;
}
else
{
Q.front++;
}
i++;
}*/
//②
/*while(Q.rear!=Q.front)
{
Q.front=(Q.front+1)%Q.maxSize;
i++;
}*/
//③
/*while(Q.front!=Q.rear)
{
Q.front++;
if(Q.front==Q.maxSize)
{
Q.front=0;
}
i++;
}*/
return i;
}
225
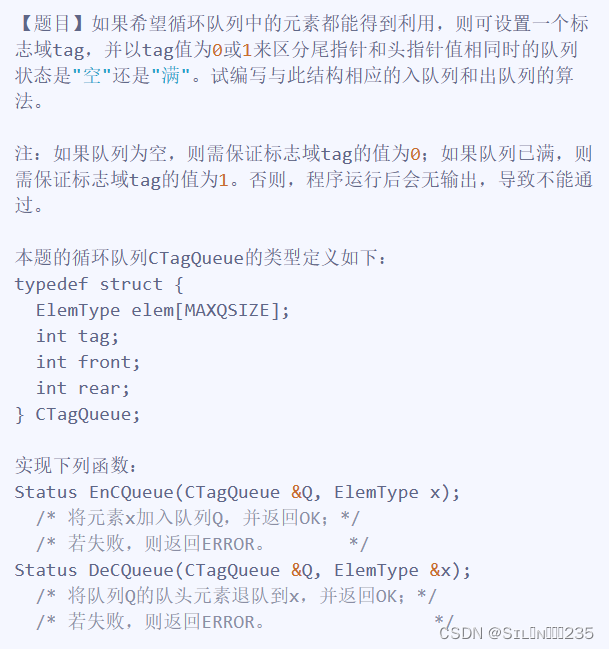
#include "allinclude.h" //DO NOT edit this line
Status EnCQueue(CTagQueue &Q, ElemType x) {
// Add your code here
if(Q.front==Q.rear&&Q.tag==1)
{
return ERROR;//队列为空报错
}
else
{
Q.rear[Q.elem]=x;
Q.rear=(Q.rear+1)%MAXSIZE;
}
if(Q.rear==Q.front)//判断加完后是满
{
Q.tag=1;
}
return OK;
}
Status DeCQueue(CTagQueue &Q, ElemType &x){
// Add your code here
if(Q.front==Q.rear&&Q.tag==0)
{
return ERROR; //队列为空报错
}
else
{
x=Q.front[Q.elem];
Q.front=(Q.front+1)%MAXSIZE;
}
if(Q.rear==Q.front)//判断出队后是否为空
{
Q.tag=0;
}
return OK;
}
227
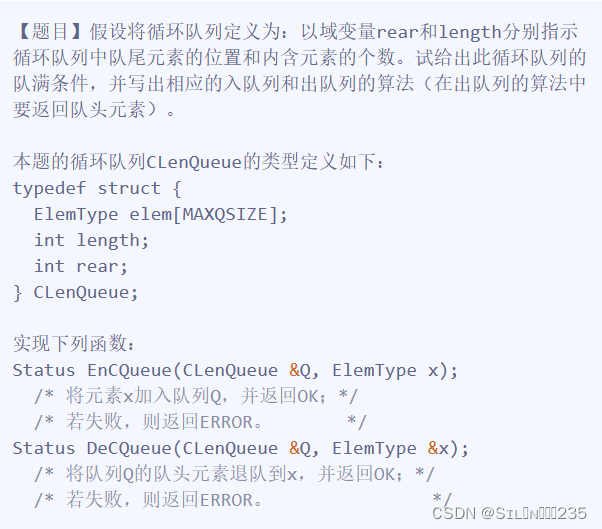
#include "allinclude.h" //DO NOT edit this line
Status EnCQueue(CLenQueue &Q, ElemType x) { //加入队列
// Add your code here
if(Q.length==MAXQSIZE)
{
return ERROR;//队满报错
}
else
{
Q.rear=(Q.rear+1)%MAXQSIZE;
Q.elem[Q.rear]=x;
Q.length++;
return OK;
}
}
Status DeCQueue(CLenQueue &Q, ElemType &x){//取队头元素
// Add your code here
if(Q.length==0)
{
return ERROR;//队空报错
}
else
{
x=Q.elem[(Q.rear+MAXQSIZE-Q.length+1)%MAXQSIZE];
Q.length--;
}
return OK;
}
232
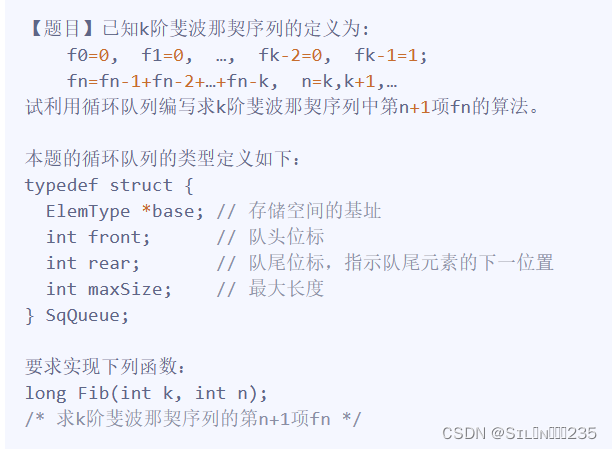
#include "allinclude.h" //DO NOT edit this line
long Fib(int k, int n) {
// Add your code here
SqQueue S;
if(k<2||n<0)//不存在的情况
{
return ERROR;
}
if(n<k-1)//k-1前面的项都是0
{
return 0;
}
else if(n==k-1)//第k-1项时为1
{
return 1;
}
else {
S.base=(ElemType*)malloc((n+1)*sizeof(ElemType));//开辟空间
S.base[k-1]=1;
int i,j,sum;
for(i=0;i<k-1;i++)
{
S.base[i]=0;
}
for(i=k;i<n+1;i++)
{
sum=0;
for(j=i-k;j<i;j++)
{
sum+=S.base[j];
}
S.base[i]=sum;
}
}
return S.base[n];
}
233
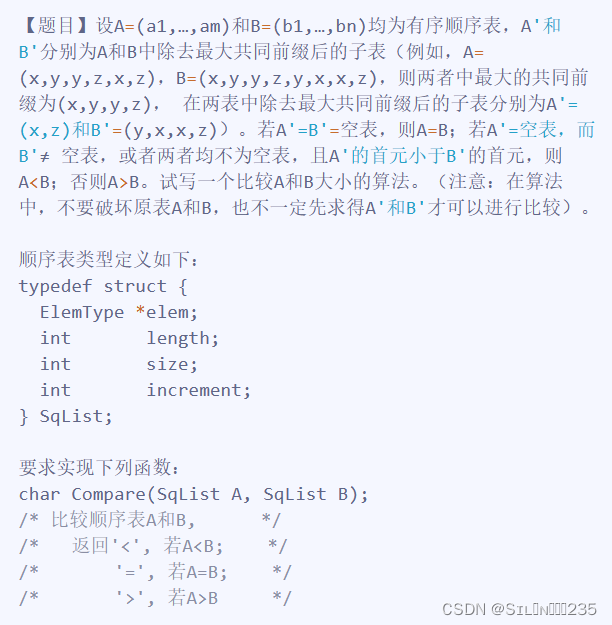
#include "allinclude.h" //DO NOT edit this line
char Compare(SqList A, SqList B)
{ // Add your code here
int i=0,j=0;
if(A.elem==NULL&&B.elem==NULL)//两个均为空表
{
return '=';
}
else if(A.elem==NULL&&B.elem!=NULL)//A为空表,B不为空表
{
return '<';
}
else if(A.elem!=NULL&&B.elem==NULL)//A不为空表,B为空表
{
return '>';
}
else //均不为空表
{
while(i<A.length&&j<A.length)
{
if(A.elem[i]==B.elem[j])//相等比较两个的下一项
{
i++;
j++;
}
else if(A.elem[i]>B.elem[j])//A的元素大于B的元素
{
return '>';
}
else if(B.elem[j]>A.elem[i])//B的元素大于A的元素
{
return '<';
}
}
if(i<A.length)//B已经没有元素
{
return '>';
}
else if(j<B.length)//A已经没有元素
{
return '<';
}
else
{
return '=';//两个均没有元素
}
}
}
235
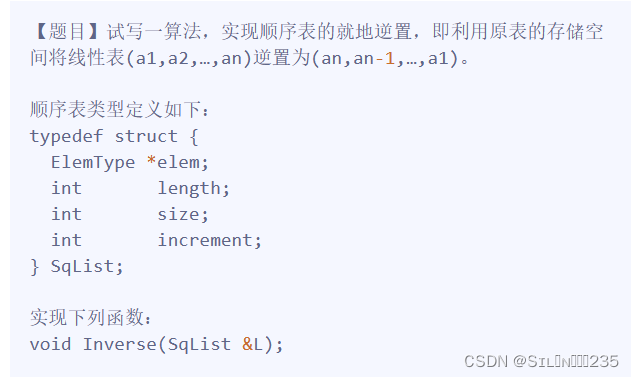
#include "allinclude.h" //DO NOT edit this line
void Inverse(SqList &L)
{ // Add your code here
int i=0,t;
for(i=0;i<L.length/2;i++)
{
t=L.elem[i];
L.elem[i]=L.elem[L.length-i-1];
L.elem[L.length-i-1]=t;
}
}
245
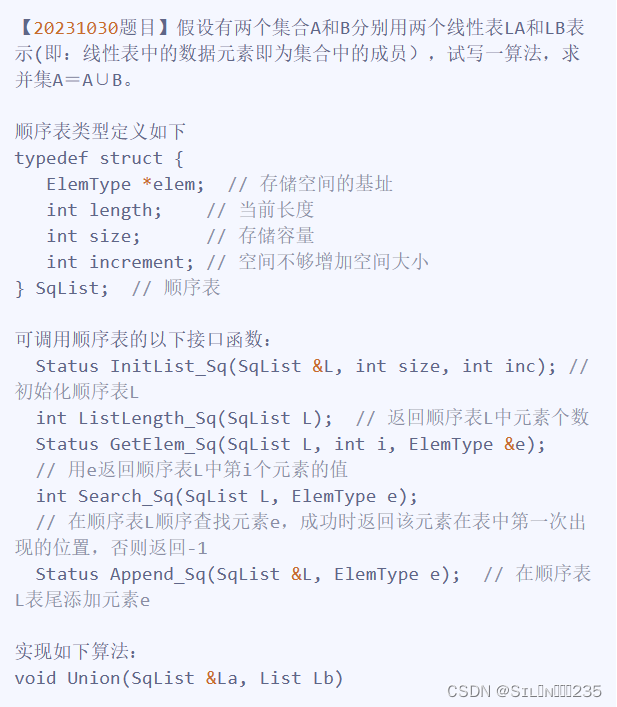
#include "allinclude.h" //DO NOT edit this line
void Union(SqList &La, List Lb)
{ // Add your code here
int count = 0;
for(int i=0;i<Lb.length;i++)
{
if(Search_Sq(La,Lb.elem[i])==-1)//判断B中的元素是否在A中,在A中查找
{
count++;//找到多的个数
}
La.elem=(ElemType*)realloc(La.elem,(La.size+count)*sizeof(ElemType));//重新分配空间
if(NULL==La.elem)//重新分配失败的情况
{
return ;
}
for(int i=0;i<Lb.length;i++)
{
if(Search_Sq(La,Lb.elem[i])==-1)//判断B中的元素是否在A中,在A中查找
{
Append_Sq(La,Lb.elem[i]);//添加元素
}
}
}
}
253
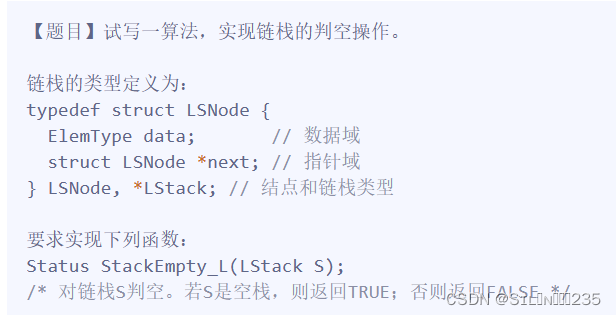
#include "allinclude.h" //DO NOT edit this line
Status StackEmpty_L(LStack S)
{ // Add your code here
if(S==NULL)
{
return TRUE;
}
else {
return FALSE;
}
}
255
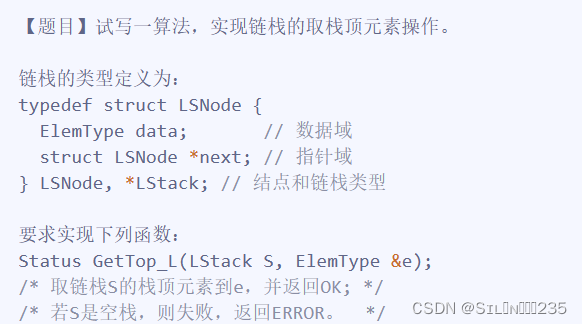
#include "allinclude.h" //DO NOT edit this line
Status GetTop_L(LStack S, ElemType &e)
{ // Add your code here
if(S==NULL)
{
return ERROR;
}
else
{
e=S->data;
return OK;
}
}
261
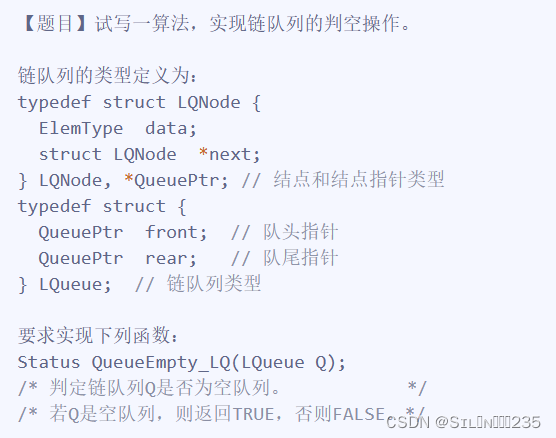
#include "allinclude.h" //DO NOT edit this line
Status QueueEmpty_LQ(LQueue Q)
{ // Add your code here
if(Q.front==NULL)
{
return TRUE;
}
else{
return FALSE;
}
}
263
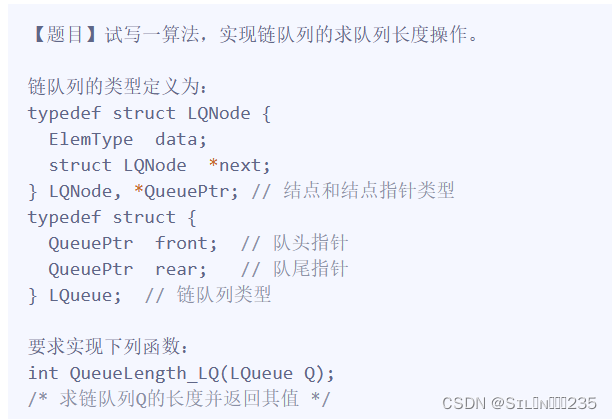
#include "allinclude.h" //DO NOT edit this line
int QueueLength_LQ(LQueue Q)
{ // Add your code here
LQNode* p;
p=Q.front;
int length=1;
if(Q.front==NULL)//判空
{
return 0;
}
while(p!=Q.rear)
{
p=p->next;
length++;
}
return length;
}
268
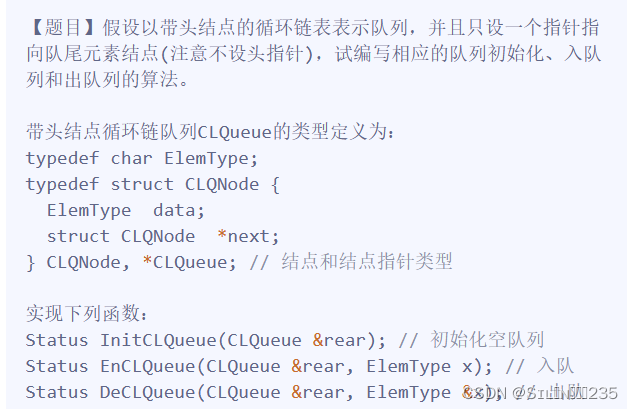
#include "allinclude.h" //DO NOT edit this line
Status InitCLQueue(CLQueue &rear)
{ // Add your code here
rear=(CLQueue)malloc(sizeof(CLQNode));
if(NULL==rear)
{
return ERROR;
}
else{
rear->next=rear;
return OK;
}
}
Status EnCLQueue(CLQueue &rear, ElemType x)
{ // Add your code here
CLQueue p;
p=(CLQueue)malloc(sizeof(CLQNode));
if(p==NULL)
{
return ERROR;
}
p->data=x;
p->next=rear->next;
rear->next=p;
rear=p;
}
Status DeCLQueue(CLQueue &rear, ElemType &x)
{ // Add your code here
CLQueue p,q;
q=rear->next;
if(rear->next==rear)//空队列
{
return ERROR;
}
p=q->next;
x=p->data;
q->next=p->next;
free(p);
return OK;
}
271
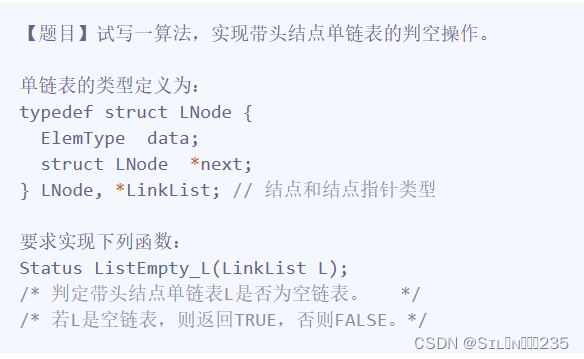
#include "allinclude.h" //DO NOT edit this line
Status ListEmpty_L(LinkList L)
{ // Add your code here
if(L->next==NULL)
{
return TRUE;
}
else
{
return FALSE;
}
}
273
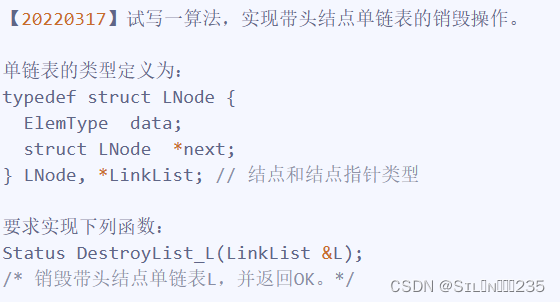
#include "allinclude.h" //DO NOT edit this line
Status DestroyList_L(LinkList &L)
{ // Add your code here
LNode *p;
while(L!=NULL)
{
p=L->next;
free(L);
L=p;
}
return OK;
}
275
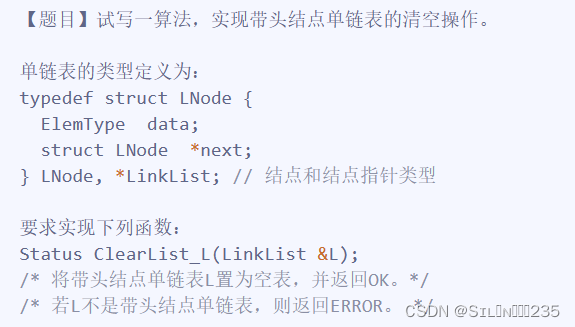
#include "allinclude.h" //DO NOT edit this line
Status ClearList_L(LinkList &L)
{ // Add your code here
LNode *p,*q;
if(L==NULL)
{
return ERROR;
}
else
{
p=L->next;
while(p!=NULL)
{
q=p->next;
free(p);
p=q;
}
}
L->next=NULL;
return OK;
}
277
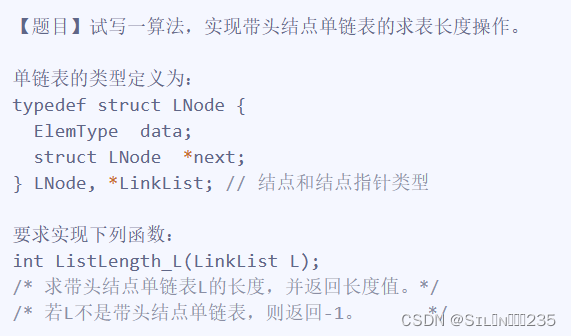
#include "allinclude.h" //DO NOT edit this line
int ListLength_L(LinkList L)
{ // Add your code here
LNode *p;
if(L==NULL)
{
return -1;
}
p=L->next;
int length=0;
while(p)
{
length++;
p=p->next;
}
return length;
}
283
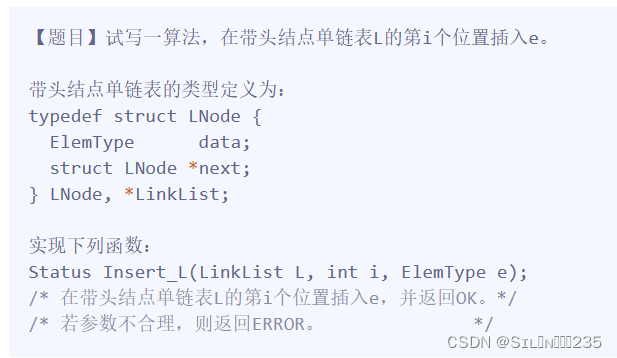
#include "allinclude.h" //DO NOT edit this line
Status Insert_L(LinkList L, int i, ElemType e)
{ // Add your code here
LNode *q,*p;
q=(LNode*)malloc(sizeof(LNode));
q->data=e;
p=L;
int j=1;
while(i>j&&p)
{
p=p->next;
j++;
}
if(NULL==p||q==NULL||i<1)
{
return ERROR;
}
q->next=p->next;
p->next=q;
return OK;
}
284
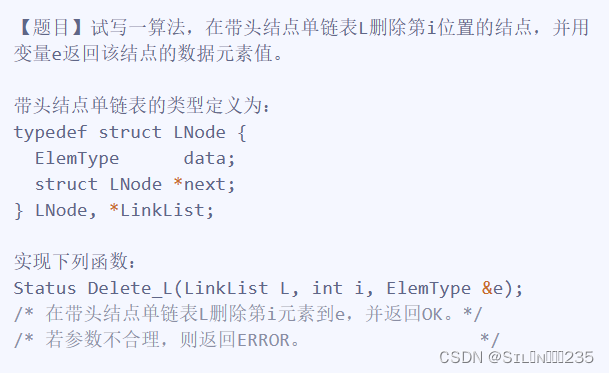
#include "allinclude.h" //DO NOT edit this line
Status Delete_L(LinkList L, int i, ElemType &e)
{ // Add your code here
LNode*p,*q;
p=L;
int j=1;
if(i<1)
{
return ERROR;
}
while(i>j&&p)
{
p=p->next;
j++;
}
if(NULL==p||p->next==NULL)
{
return ERROR;
}
q=p->next;
p->next=q->next;
e=q->data;
free(q);
return OK;
}
286
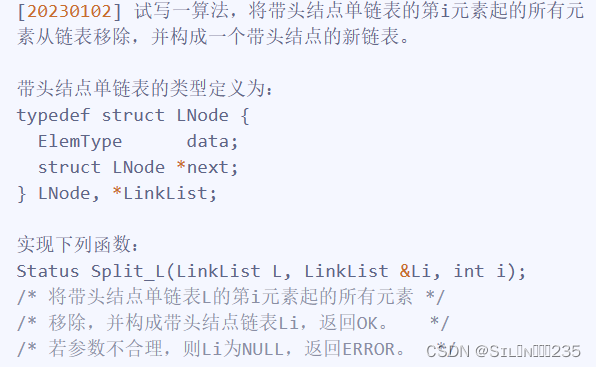
#include "allinclude.h" //DO NOT edit this line
Status Split_L(LinkList L, LinkList &Li, int i)
{ // Add your code here
LNode*p,*q;
q=(LNode*)malloc(sizeof(LNode));
q->data=NULL;
p=L;
int j=1;
while(j<i&&p)
{
p=p->next;
j++;
}
if(p==NULL||p->next==NULL||i<1)
{
Li=NULL;
return ERROR;
}
q->next=p->next;
Li=q->next;
Li=q;
p->next=NULL;
return OK;
}
288
#include "allinclude.h" //DO NOT edit this line
Status Cut_L(LinkList L, int i)
{ // Add your code here
LNode*p,*q;
if(NULL==L||L->next==NULL||i<1)
{
return ERROR;
}
p=L;
int j=1;
while(j<i&&p)
{
p=p->next;
j++;
if(p->next==NULL)
{
return ERROR;
}
}
p->next=NULL;
while(p)
{
q=p->next;
p=q;
}
return OK;
}
290
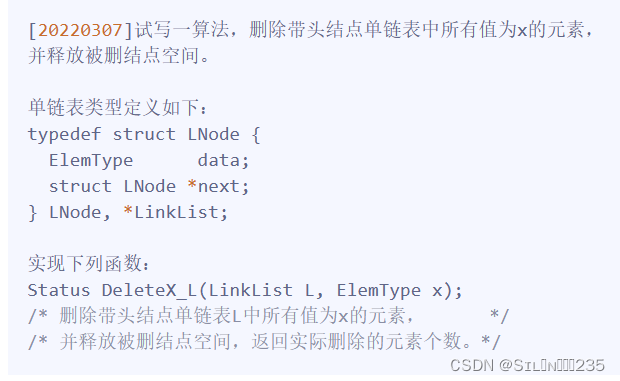
#include "allinclude.h" //DO NOT edit this line
Status DeleteX_L(LinkList L, ElemType x)
{ // Add your code here
LNode *p,*q;
int i=0;
p=L;
while(p)
{
if(p==NULL||p->next==NULL)
{
return i;
}
if(p->next->data==x)
{
q=p->next->next;
p->next=q;
i++;
}
p=p->next;
}
return i;
}
291
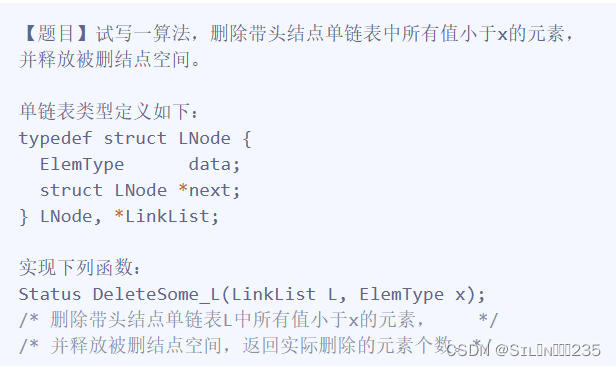
#include "allinclude.h" //DO NOT edit this line
Status DeleteSome_L(LinkList L, ElemType x)
{ // Add your code here
LNode *p,*q,*t;
int i=0;
p=L->next;
q=L;
while(p)
{
if(p->data<x)
{
t=p;
p=p->next;
free(t);
q->next=p;
i++;
}
else
{
q=p;
p=p->next;
}
}
return i;
}
293
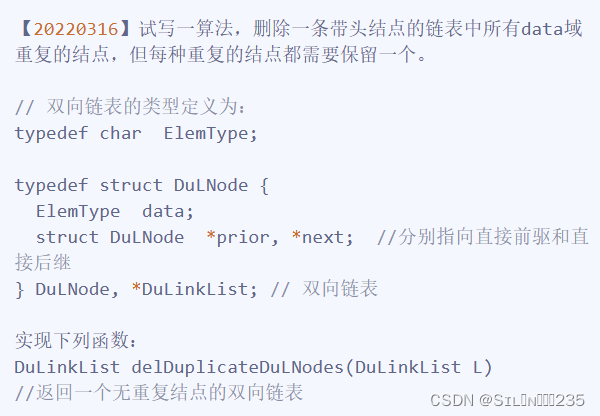
#include "allinclude.h" //DO NOT edit this line
DuLinkList delDuplicateDuLNodes(DuLinkList L)
{ // Add your code here
if (L == NULL)
{
return ERROR;
}
DuLinkList p, q;
for (p=L->next;p!=NULL;p=p->next)
{
for (q=p->next;q!=NULL;q=q->next)
{
if (p->data==q->data)
{
q->prior->next=q->next;
if (q->next!=NULL)
{
q->next->prior=q->prior;
}
free(q);
}
}
}
return L;
}
294
#include "allinclude.h" //DO NOT edit this line
void reverseDuLinkList(DuLinkList L)
{ // Add your code here
if(L == NULL || L->next == NULL)
{
return;
}
DuLinkList p = L->next; // p指向第一个结点
L->next = NULL;
while(p!= NULL)
{
DuLinkList q = p->next; // q指向p的后继结点
p->next = L->next;
if(L->next != NULL)
{
L->next->prior = p;
}
L->next = p;
p->prior = L;
p = q;
}
}
295
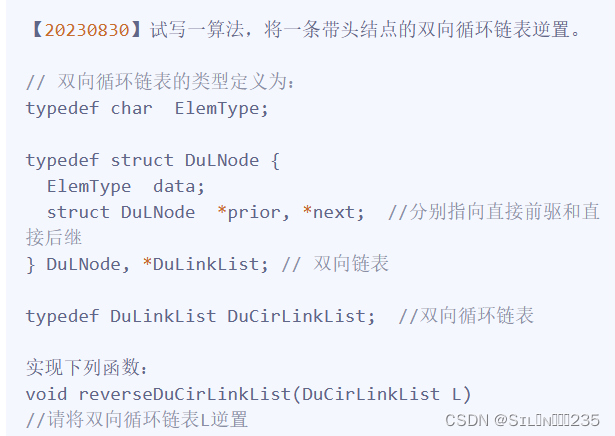
#include "allinclude.h" //DO NOT edit this line
void reverseDuCirLinkList(DuCirLinkList L)
{ // Add your code here
if(L==NULL||L->next==L) // 空链表或只有头结点
{
return;
}
DuLinkList p=L->next; // p指向第一个结节点
L->next=L; // 头结点的next指针指向自己
while(p!=L)
{
DuLinkList q=p->next; // q指向p的后继结点
p->next=L->next;
p->prior=L;
L->next->prior=p;
L->next=p;
p=q;
}
}
297
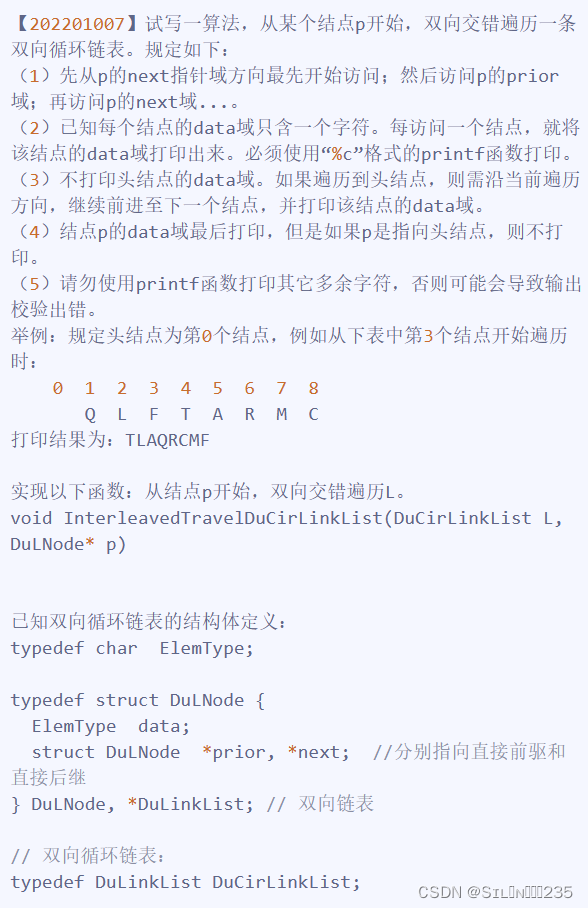
#include "allinclude.h" //DO NOT edit this line
void InterleavedTravelDuCirLinkList(DuCirLinkList L, DuLNode* p)
{ // Add your code here
DuLNode *q,*t;
if(L==NULL)
{
return ;
}
if(p==L&&p->next==L)
{
return ;
}
q=p->next;
t=p->prior;
while(1)
{
if(t==L&&q==L)
{
break;
}
if(t==q)
{
printf("%c",q->data);
break;
}
if(L==q)
{
q=q->next;
if(t==q)
{
printf("%c",q->data);
break;
}
}
if(L==t)
{
t=t->prior;
if(t==q)
{
printf("%c",q->data);
break;
}
}
printf("%c",q->data);
printf("%c",t->data);
if(t->prior==q)
{
break;
}
q=q->next;
t=t->prior;
}
printf("%c",p->data);
}
298
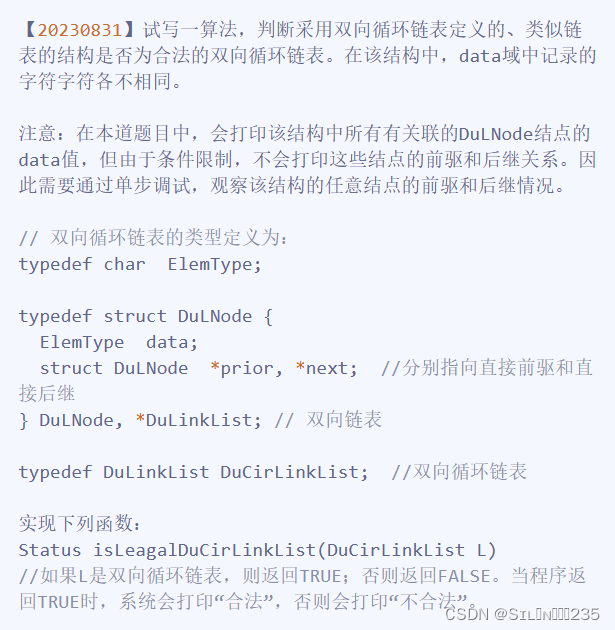
#include "allinclude.h" //DO NOT edit this line
Status isLeagalDuCirLinkList(DuCirLinkList L)
{ // Add your code here
// 判断是否为空链表
if (L == NULL)
{
return TRUE;
}
DuLinkList p = L; // 当前节点
DuLinkList q;
do {
while (q != L) {
q = p->next;
if(p==NULL||p->next==NULL)
{
return FALSE;
}
if (p!=q->prior) {
return FALSE;
}
p=p->next;
}
p = p->next;
} while (p != L);
return TRUE;
}