可以使用统一的接口对对象进行管理
主要使用的类型
//一个基础类型, 一般位于内核对象的最前方, 用于记录一个对象的信息 struct rt\_object { /\*内核对象名称\*/ char name[RT_NAME_MAX]; /\*内核对象类型\*/ rt\_uint8\_t type; /\*内核对象的参数\*/ rt\_uint8\_t flag; /\*内核对象管理链表\*/ rt\_list\_t list; };
/\*\*可以使用的对象类型 \* The object type can be one of the follows with specific \* macros enabled: \* - Thread \* - Semaphore \* - Mutex \* - Event \* - MailBox \* - MessageQueue \* - MemHeap \* - MemPool \* - Device \* - Timer \* - Unknown \* - Static \*/ enum rt\_object\_class\_type { RT_Object_Class_Null = 0x00, /\*\*< The object is not used. \*/ RT_Object_Class_Thread = 0x01, /\*\*< 对象为线程类型 \*/ RT_Object_Class_Semaphore = 0x02, /\*\*< 对象为信号量类型 \*/ RT_Object_Class_Mutex = 0x03, /\*\*< 对象为互斥量类型 \*/ RT_Object_Class_Event = 0x04, /\*\*< 对象为事件类型 \*/ RT_Object_Class_MailBox = 0x05, /\*\*< 对象为邮箱类型 \*/ RT_Object_Class_MessageQueue = 0x06, /\*\*< 对象为消息队列类型 \*/ RT_Object_Class_MemHeap = 0x07, /\*\*< memory heap. \*/ RT_Object_Class_MemPool = 0x08, /\*\*< memory pool. \*/ RT_Object_Class_Device = 0x09, /\*\*< 对象为设备类型 \*/ RT_Object_Class_Timer = 0x0a, /\*\*< 对象为定时器类型 \*/ RT_Object_Class_Unknown = 0x0c, /\*\*< 对象类型未知 \*/ RT_Object_Class_Static = 0x80 /\*\*< 对象为静态对象, 这是一个标志位 \*/ };
/\*\* \* The information of the kernel object, 这一个是用于记录初始化信息的临时变量 \*/ struct rt\_object\_information { enum rt\_object\_class\_type type; /\*\*< object class type \*/ rt\_list\_t object_list; /\*\*< object list \*/ rt\_size\_t object_size; /\*\*< object size \*/ };
RT-Thread使用一个结构体数组
static struct rt_object_information rt_object_container[RT_Object_Info_Unknown]
保存默认的信息
管理的API
初始化一个静态的对象
/\*\*实际上是对一个以及存在的类型进行赋值以及链表的插入
\* This function will initialize an object and add it to object system
\* management.
\*
\* @param object the specified object to be initialized.
\* @param type the object type.
\* @param name the object name. In system, the object's name must be unique.
\*/
void rt\_object\_init(struct rt\_object \*object,
enum rt\_object\_class\_type type,
const char \*name)
系统会把这个对象放置到对象容器中进行管理,即初始化对象的一些参数,然后把这个对象节点插入到对象容器的对象链表中
脱离一个对象
/\*\*
\* This function will detach a static object from object system,
\* and the memory of static object is not freed.
\*
\* @param object the specified object to be detached.
\*/
void rt\_object\_detach(rt\_object\_t object)
使得一个静态内核对象从内核对象容器中脱离出来,即从内核对象容器链表上删除相应的对象节点。
动态创建以及删除
/\*\*清除类型以及链表的脱离
\* This function will allocate an object from object system
\*
\* @param type the type of object
\* @param name the object name. In system, the object's name must be unique.
\*
\* @return object
\*/
rt\_object\_t rt\_object\_allocate(enum rt\_object\_class\_type type, const char \*name)
/\*\*多了一个释放内存
\* This function will delete an object and release object memory.
\*
\* @param object the specified object to be deleted.
\*/
void rt\_object\_delete(rt\_object\_t object)
判断一个对象是不是静态对象
/\*\*判断static标志位
\* This function will judge the object is system object or not.
\* Normally, the system object is a static object and the type
\* of object set to RT\_Object\_Class\_Static.
\*
\* @param object the specified object to be judged.
\*
\* @return RT\_TRUE if a system object, RT\_FALSE for others.
\*/
rt\_bool\_t rt\_object\_is\_systemobject(rt\_object\_t object)
实际的管理示例(动态)
- 以下用线程的初始化为例
/\*\*
\* This function will create a thread object and allocate thread object memory
\* and stack.
\*
\* @param name the name of thread, which shall be unique
\* @param entry the entry function of thread
\* @param parameter the parameter of thread enter function
\* @param stack\_size the size of thread stack
\* @param priority the priority of thread
\* @param tick the time slice if there are same priority thread
\*
\* @return the created thread object
\*/
rt\_thread\_t rt\_thread\_create(const char \*name,
void (\*entry)(void \*parameter),
void \*parameter,
rt\_uint32\_t stack_size,
rt\_uint8\_t priority,
rt\_uint32\_t tick)
{
struct rt\_thread \*thread;
void \*stack_start;
//获取一个线程对象
thread = (struct rt\_thread \*)rt\_object\_allocate(RT_Object_Class_Thread,
name);
if (thread == RT_NULL)
return RT_NULL;
//获取一块栈区
stack_start = (void \*)RT\_KERNEL\_MALLOC(stack_size);
if (stack_start == RT_NULL)
{
/\* allocate stack failure \*/
rt\_object\_delete((rt\_object\_t)thread);
return RT_NULL;
}
//这个函数主要是用来填充信息的, 以及栈的初始化, 不在这里讨论(详细看线程管理的笔记, 以后会更新)
\_rt\_thread\_init(thread,
name,
entry,
parameter,
stack_start,
stack_size,
priority,
tick);
return thread;
}
/\*\*
\* This function will allocate an object from object system
\*
\* @param type the type of object
\* @param name the object name. In system, the object's name must be unique.
\*
\* @return object
\*/
## 最后
**自我介绍一下,小编13年上海交大毕业,曾经在小公司待过,也去过华为、OPPO等大厂,18年进入阿里一直到现在。**
**深知大多数Java工程师,想要提升技能,往往是自己摸索成长,自己不成体系的自学效果低效漫长且无助。**
**因此收集整理了一份《2024年嵌入式&物联网开发全套学习资料》,初衷也很简单,就是希望能够帮助到想自学提升又不知道该从何学起的朋友,同时减轻大家的负担。**
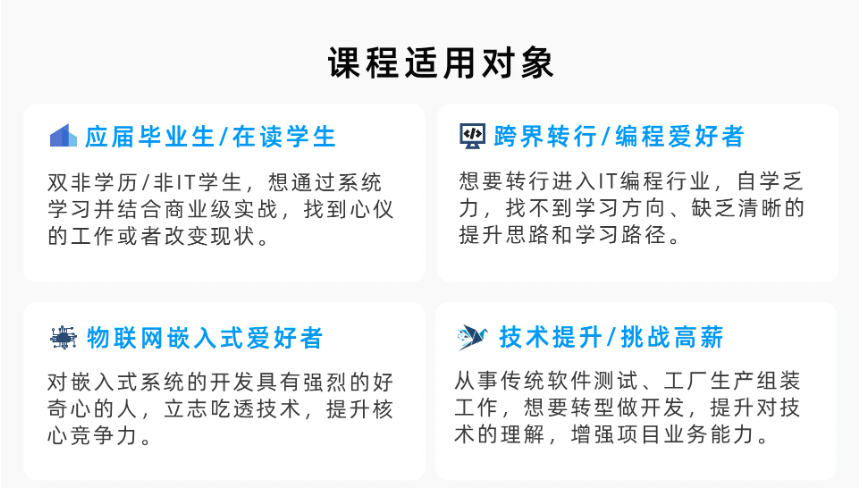
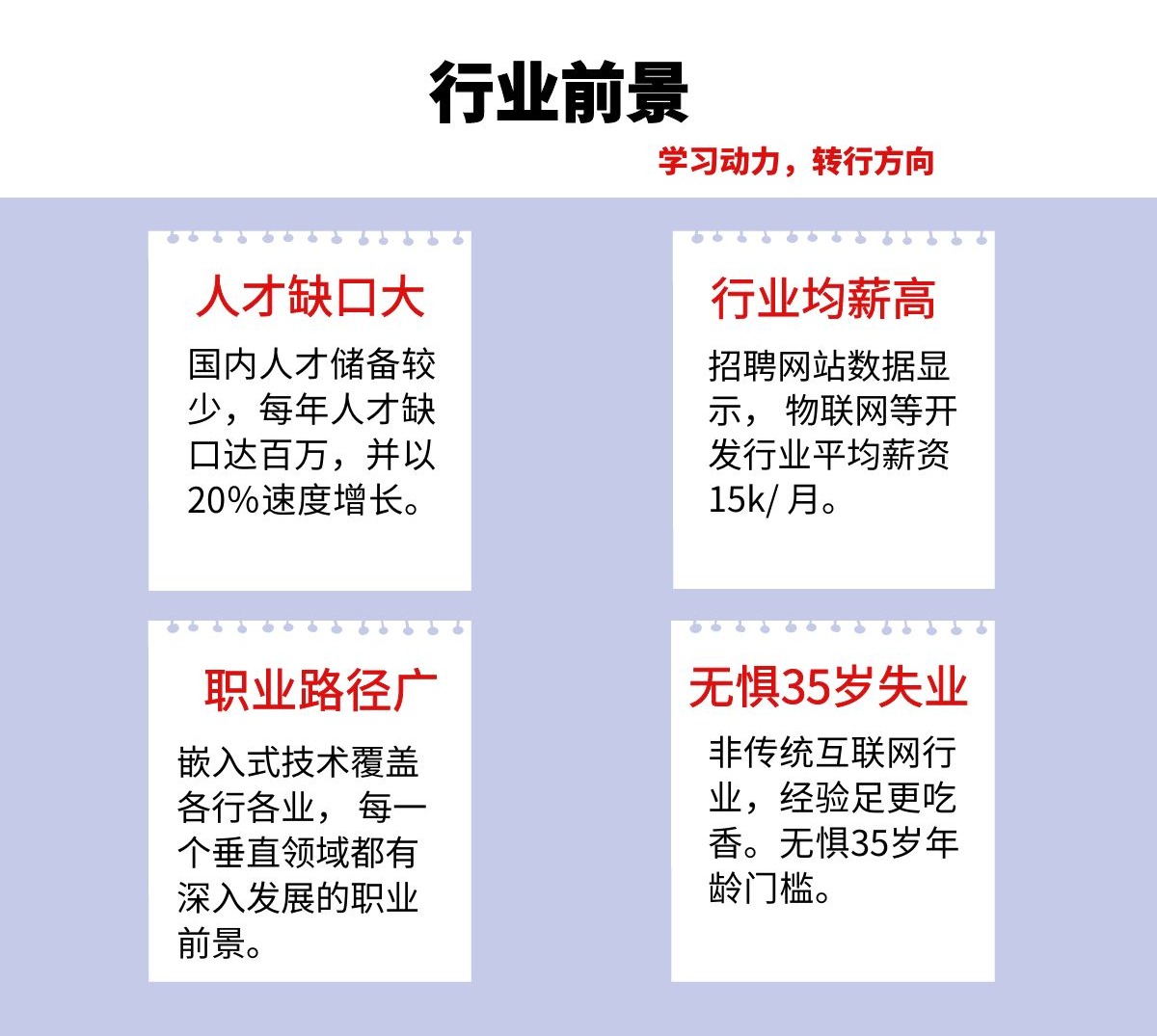
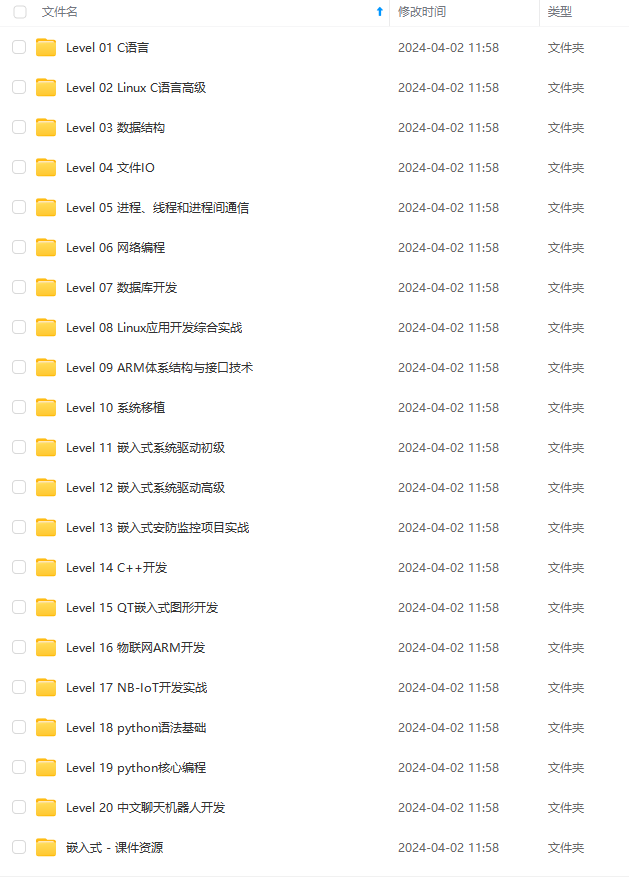
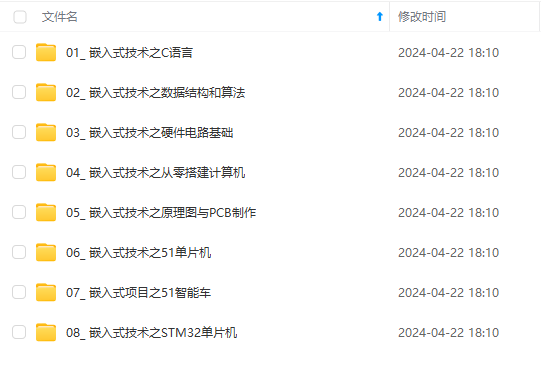
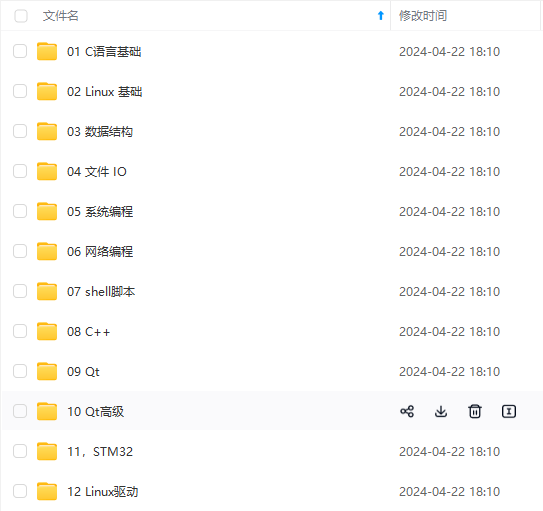
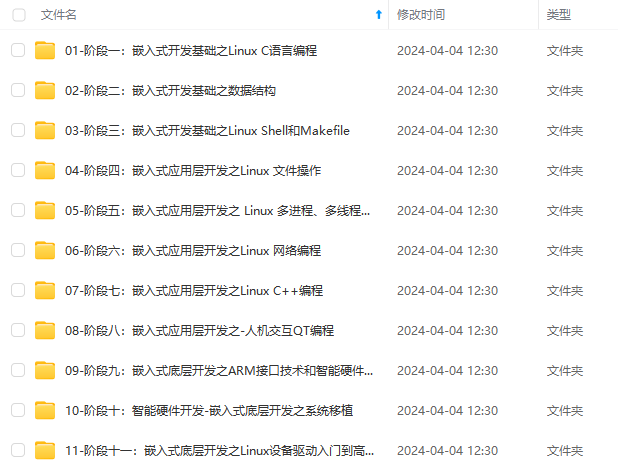
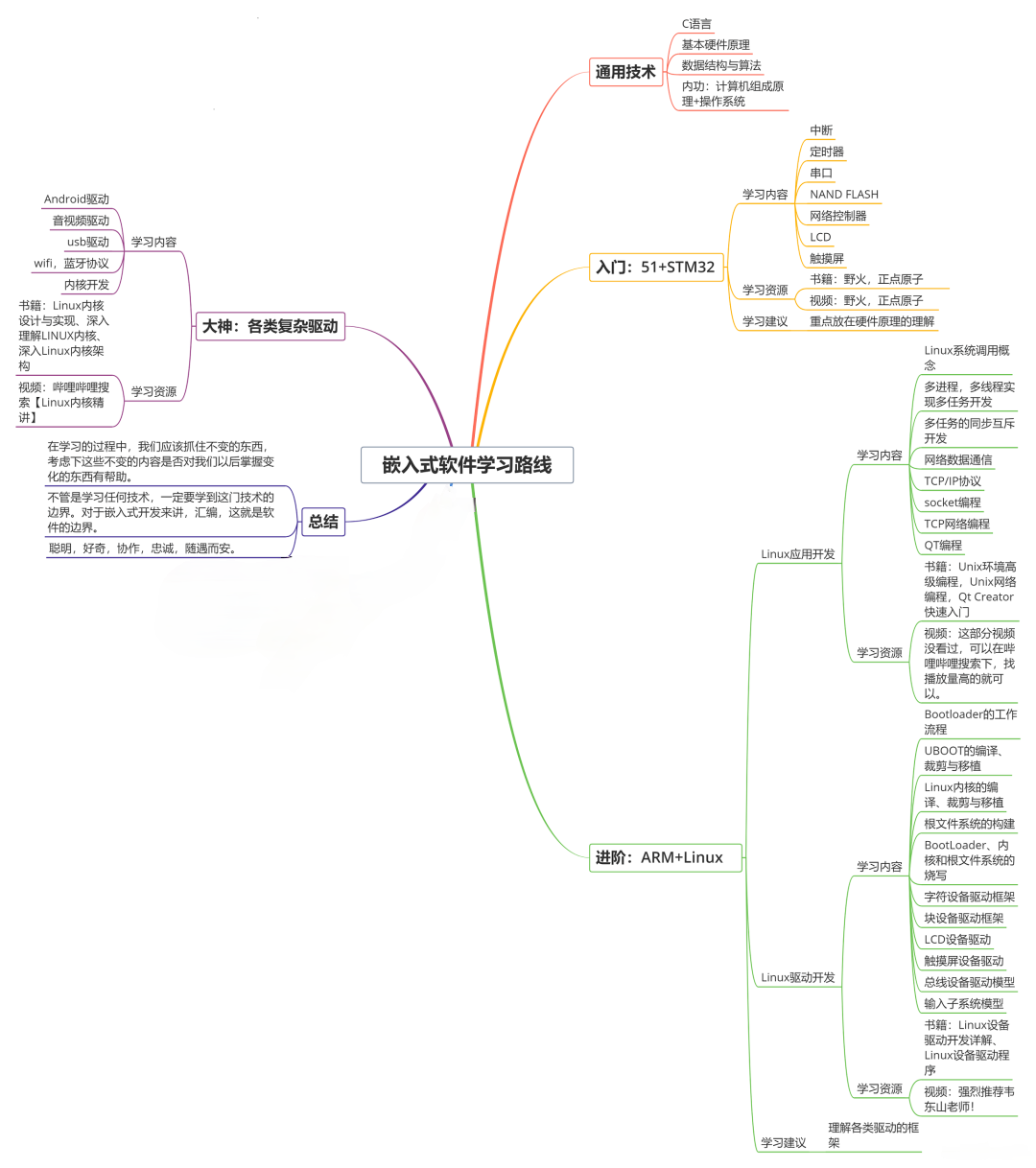
**既有适合小白学习的零基础资料,也有适合3年以上经验的小伙伴深入学习提升的进阶课程,基本涵盖了95%以上嵌入式&物联网开发知识点,真正体系化!**
[**如果你觉得这些内容对你有帮助,需要这份全套学习资料的朋友可以戳我获取!!**](https://bbs.csdn.net/topics/618654289)
**由于文件比较大,这里只是将部分目录大纲截图出来,每个节点里面都包含大厂面经、学习笔记、源码讲义、实战项目、讲解视频,并且后续会持续更新**!!
0fH35ptX-1715597515689)]
**既有适合小白学习的零基础资料,也有适合3年以上经验的小伙伴深入学习提升的进阶课程,基本涵盖了95%以上嵌入式&物联网开发知识点,真正体系化!**
[**如果你觉得这些内容对你有帮助,需要这份全套学习资料的朋友可以戳我获取!!**](https://bbs.csdn.net/topics/618654289)
**由于文件比较大,这里只是将部分目录大纲截图出来,每个节点里面都包含大厂面经、学习笔记、源码讲义、实战项目、讲解视频,并且后续会持续更新**!!