}
/**
-
解析指定路径下的二维码图片
-
@param filePath
-
@return
*/
private static String parseQRCode(String filePath) {
String content = “”;
try {
File file = new File(filePath);
BufferedImage image = ImageIO.read(file);
LuminanceSource source = new BufferedImageLuminanceSource(image);
Binarizer binarizer = new HybridBinarizer(source);
BinaryBitmap binaryBitmap = new BinaryBitmap(binarizer);
Map<DecodeHintType, Object> hints = new HashMap<>();
hints.put(DecodeHintType.CHARACTER_SET, “UTF-8”);
MultiFormatReader formatReader = new MultiFormatReader();
Result result = formatReader.decode(binaryBitmap, hints);
//设置返回值
content = result.getText();
} catch (Exception e) {
e.printStackTrace();
}
return content;
}
/**
-
根据内容,生成指定宽高、指定格式的二维码图片
-
@param text 内容
-
@param width 宽
-
@param height 高
-
@param format 图片格式
-
@return 生成的二维码图片路径
-
@throws Exception
*/
public static String generateQRCode(String text, int width, int height, String format, String pathName) throws Exception {
Hashtable<EncodeHintType, Object> hints = new Hashtable<>();
hints.put(EncodeHintType.CHARACTER_SET, “utf-8”);
BitMatrix bitMatrix = new MultiFormatWriter().encode(text, BarcodeFormat.QR_CODE, width, height, hints);
File outputFile = new File(pathName);
if (!outputFile.exists()) {
outputFile.mkdirs();
}
writeToFile(bitMatrix, format, outputFile);
return pathName;
}
//输出为文件
public static void writeToFile(BitMatrix matrix, String format, File file)
throws IOException {
BufferedImage image = toBufferedImage(matrix);
if (!ImageIO.write(image, format, file)) {
throw new IOException(“文件写入异常”);
}
}
//输出为流
public static void writeToStream(BitMatrix matrix, String format, OutputStream stream)
throws IOException {
BufferedImage image = toBufferedImage(matrix);
if (!ImageIO.write(image, format, stream)) {
throw new IOException(“文件写入异常”);
}
}
//缓冲图片
public static BufferedImage toBufferedImage(BitMatrix matrix) {
int width = matrix.getWidth();
int height = matrix.getHeight();
BufferedImage image = new BufferedImage(width, height, BufferedImage.TYPE_INT_RGB);
for (int x = 0; x < width; x++) {
for (int y = 0; y < height; y++) {
image.setRGB(x, y, matrix.get(x, y) ? BLACK : WHITE);
}
}
return image;
}
}
控制台输出
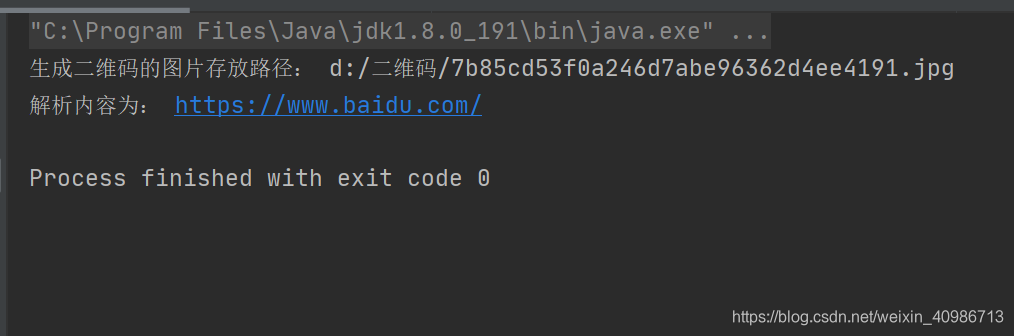
生成二维码图片效果
最后
由于细节内容实在太多了,为了不影响文章的观赏性,只截出了一部分知识点大致的介绍一下,每个小节点里面都有更细化的内容!
小编准备了一份Java进阶学习路线图(Xmind)以及来年金三银四必备的一份《Java面试必备指南》
,为了不影响文章的观赏性,只截出了一部分知识点大致的介绍一下,每个小节点里面都有更细化的内容!
[外链图片转存中…(img-yfKGpqZF-1714135394015)]
小编准备了一份Java进阶学习路线图(Xmind)以及来年金三银四必备的一份《Java面试必备指南》
[外链图片转存中…(img-PIFyicIV-1714135394015)]