其中Runnable应该是我们最熟悉的接口,它只有一个run()函数,用于将耗时操作写在其中,该函数没有返回值。然后使用某个线程去执行该runnable即可实现多线程,Thread类在调用start()函数后就是执行的是Runnable的run()函数。Runnable的声明如下 :
[java] view plain copy
-
public interface Runnable {
-
/**
-
* When an object implementing interface
Runnable
is used -
* to create a thread, starting the thread causes the object’s
-
*
run
method to be called in that separately executing -
* thread.
-
*
-
*
-
* @see java.lang.Thread#run()
-
*/
-
public abstract void run();
-
}
Callable与Runnable的功能大致相似,Callable中有一个call()函数,但是call()函数有返回值,而Runnable的run()函数不能将结果返回给客户程序。Callable的声明如下 :
[java] view plain copy
-
public interface Callable {
-
/**
-
* Computes a result, or throws an exception if unable to do so.
-
*
-
* @return computed result
-
* @throws Exception if unable to compute a result
-
*/
-
V call() throws Exception;
-
}
可以看到,这是一个泛型接口,call()函数返回的类型就是客户程序传递进来的V类型。
Executor就是Runnable和Callable的调度容器,Future就是对于具体的Runnable或者Callable任务的执行结果进行
**取消、**查询是否完成、获取结果、设置结果操作。get方法会阻塞,直到任务返回结果(Future简介)。Future声明如下 :
[java] view plain copy
-
/**
-
* @see FutureTask
-
* @see Executor
-
* @since 1.5
-
* @author Doug Lea
-
* @param The result type returned by this Future’s get method
-
*/
-
public interface Future {
-
/**
-
* Attempts to cancel execution of this task. This attempt will
-
* fail if the task has already completed, has already been cancelled,
-
* or could not be cancelled for some other reason. If successful,
-
* and this task has not started when cancel is called,
-
* this task should never run. If the task has already started,
-
* then the mayInterruptIfRunning parameter determines
-
* whether the thread executing this task should be interrupted in
-
* an attempt to stop the task. *
-
*/
-
boolean cancel(boolean mayInterruptIfRunning);
-
/**
-
* Returns true if this task was cancelled before it completed
-
* normally.
-
*/
-
boolean isCancelled();
-
/**
-
* Returns true if this task completed.
-
*
-
*/
-
boolean isDone();
-
/**
-
* Waits if necessary for the computation to complete, and then
-
* retrieves its result.
-
*
-
* @return the computed result
-
*/
-
V get() throws InterruptedException, ExecutionException;
-
/**
-
* Waits if necessary for at most the given time for the computation
-
* to complete, and then retrieves its result, if available.
-
*
-
* @param timeout the maximum time to wait
-
* @param unit the time unit of the timeout argument
-
* @return the computed result
-
*/
-
V get(long timeout, TimeUnit unit)
-
throws InterruptedException, ExecutionException, TimeoutException;
-
}
FutureTask则是一个RunnableFuture,而RunnableFuture实现了Runnbale又实现了Futrue这两个接口,
[java] view plain copy
- public class FutureTask implements RunnableFuture
RunnableFuture
[java] view plain copy
-
public interface RunnableFuture extends Runnable, Future {
-
/**
-
* Sets this Future to the result of its computation
-
* unless it has been cancelled.
-
*/
-
void run();
-
}
另外它还可以包装Runnable和Callable, 由构造函数注入依赖。
[java] view plain copy
-
public FutureTask(Callable callable) {
-
if (callable == null)
-
throw new NullPointerException();
-
this.callable = callable;
-
this.state = NEW; // ensure visibility of callable
-
}
-
public FutureTask(Runnable runnable, V result) {
-
this.callable = Executors.callable(runnable, result);
-
this.state = NEW; // ensure visibility of callable
-
}
可以看到,Runnable注入会被Executors.callable()函数转换为Callable类型,即FutureTask最终都是执行Callable类型的任务。该适配函数的实现如下 :
[java] view plain copy
-
public static Callable callable(Runnable task, T result) {
-
if (task == null)
-
throw new NullPointerException();
-
return new RunnableAdapter(task, result);
-
}
RunnableAdapter适配器
[java] view plain copy
-
/**
-
* A callable that runs given task and returns given result
-
*/
-
static final class RunnableAdapter implements Callable {
-
final Runnable task;
-
final T result;
-
RunnableAdapter(Runnable task, T result) {
-
this.task = task;
-
this.result = result;
-
}
-
public T call() {
-
task.run();
-
return result;
-
}
-
}
由于FutureTask实现了Runnable,因此它既可以通过Thread包装来直接执行,也可以提交给ExecuteService来执行。
并且还可以直接通过get()函数获取执行结果,该函数会阻塞,直到结果返回。因此FutureTask既是Future、
**Runnable,**又是包装了Callable( 如果是Runnable最终也会被转换为Callable ), 它是这两者的合体。
[java] view plain copy
-
package com.effective.java.concurrent.task;
-
import java.util.concurrent.Callable;
-
import java.util.concurrent.ExecutionException;
-
import java.util.concurrent.ExecutorService;
-
import java.util.concurrent.Executors;
-
import java.util.concurrent.Future;
-
import java.util.concurrent.FutureTask;
-
/**
-
*
-
* @author mrsimple
-
*
-
*/
-
public class RunnableFutureTask {
-
/**
-
* ExecutorService
-
*/
-
static ExecutorService mExecutor = Executors.newSingleThreadExecutor();
-
/**
-
*
-
* @param args
-
*/
-
public static void main(String[] args) {
-
runnableDemo();
自我介绍一下,小编13年上海交大毕业,曾经在小公司待过,也去过华为、OPPO等大厂,18年进入阿里一直到现在。
深知大多数Java工程师,想要提升技能,往往是自己摸索成长或者是报班学习,但对于培训机构动则几千的学费,着实压力不小。自己不成体系的自学效果低效又漫长,而且极易碰到天花板技术停滞不前!
因此收集整理了一份《2024年Java开发全套学习资料》,初衷也很简单,就是希望能够帮助到想自学提升又不知道该从何学起的朋友,同时减轻大家的负担。
既有适合小白学习的零基础资料,也有适合3年以上经验的小伙伴深入学习提升的进阶课程,基本涵盖了95%以上Java开发知识点,真正体系化!
由于文件比较大,这里只是将部分目录截图出来,每个节点里面都包含大厂面经、学习笔记、源码讲义、实战项目、讲解视频,并且会持续更新!
如果你觉得这些内容对你有帮助,可以扫码获取!!(备注Java获取)
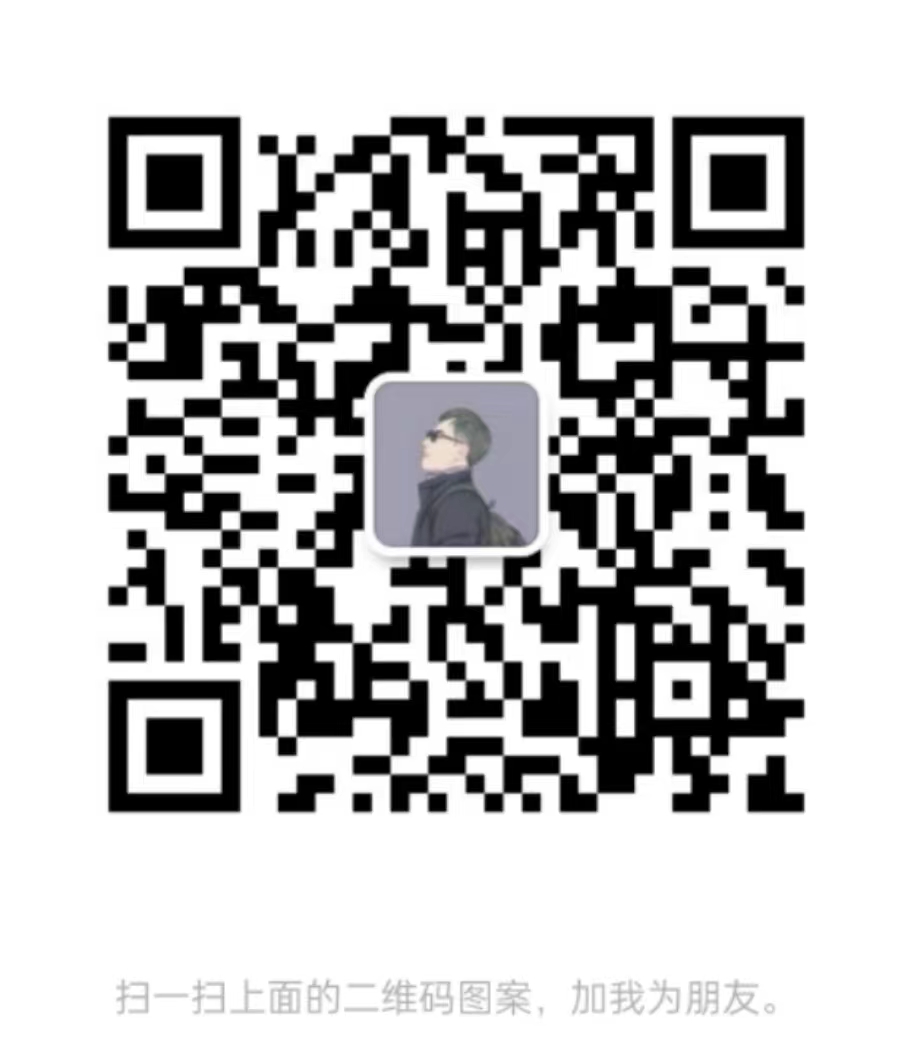
一线互联网大厂Java核心面试题库
正逢面试跳槽季,给大家整理了大厂问到的一些面试真题,由于文章长度限制,只给大家展示了部分题目,更多Java基础、异常、集合、并发编程、JVM、Spring全家桶、MyBatis、Redis、数据库、中间件MQ、Dubbo、Linux、Tomcat、ZooKeeper、Netty等等已整理上传,感兴趣的朋友可以看看支持一波!
《互联网大厂面试真题解析、进阶开发核心学习笔记、全套讲解视频、实战项目源码讲义》点击传送门即可获取!
mg.cn/images/e5c14a7895254671a72faed303032d36.jpg" alt=“img” style=“zoom: 33%;” />
一线互联网大厂Java核心面试题库
[外链图片转存中…(img-4650457o-1713168455679)]
正逢面试跳槽季,给大家整理了大厂问到的一些面试真题,由于文章长度限制,只给大家展示了部分题目,更多Java基础、异常、集合、并发编程、JVM、Spring全家桶、MyBatis、Redis、数据库、中间件MQ、Dubbo、Linux、Tomcat、ZooKeeper、Netty等等已整理上传,感兴趣的朋友可以看看支持一波!
《互联网大厂面试真题解析、进阶开发核心学习笔记、全套讲解视频、实战项目源码讲义》点击传送门即可获取!