1、目录
2、controller
3、mapper
4、pojo
PageBean
Poet
Result
5、service
PoetService
PoetServiceA
6、xml文件
7、index6.html
8、运行页面
1、目录
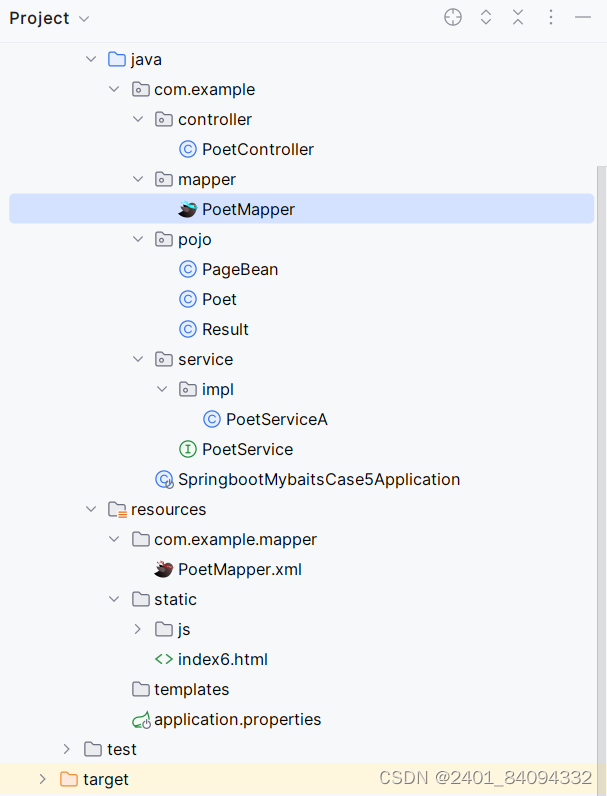
2、controller
package com.example.controller;
import com.example.pojo.PageBean;
import com.example.pojo.Poet;
import com.example.pojo.Result;
import com.example.service.PoetService;
import lombok.extern.slf4j.Slf4j;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.*;
@Slf4j
@RestController
public class PoetController {
@Autowired
private PoetService poetService;
//查询
@GetMapping("/index")
public Result select(){
return Result.success(poetService.selectData());
}
//分页查询
@GetMapping("/poets/{page}/{pageSize}")
public Result page(@PathVariable Integer page,
@PathVariable Integer pageSize,
String author,String style){
//记录日志
log.info("分页查询:{},{},{},{}",page,pageSize,author,style);
//调用service分页查询
PageBean pageBean = poetService.page(page,pageSize,author,style);
//响应
return Result.success(pageBean);
}
//新增
@RequestMapping("/poet")
public Result insert(@RequestBody Poet poet){
poetService.insert(poet);
return Result.success();
}
//删除
@DeleteMapping("/delete/{id}")
public Result delete(@PathVariable Integer id){
poetService.delete(id);
return Result.success();
}
//修改 先查询,再修改
@RequestMapping("/poets/{id}")
public Result getById(@PathVariable Integer id){
Poet poet = poetService.getById(id);
return Result.success(poet);
}
@PutMapping("/update")
public Result update(@RequestBody Poet poet){
poetService.update(poet);
return Result.success();
}
}
3、mapper
package com.example.mapper;
import com.example.pojo.Poet;
import org.apache.ibatis.annotations.*;
import java.util.List;
@Mapper
public interface PoetMapper {
//@Select("select p_id as id, name, gender, dynasty, title, style from poet")
List<Poet> selectPoet();
//@Insert("insert into poet (name, gender, dynasty, title, style) " +"values (#{name},#{gender},#{dynasty},#{title},#{style})")
void insert(Poet poet);
//@Delete("delete from poet where p_id=#{id}")
void delete(Integer id);
//@Select("select p_id as id, name, gender, dynasty, title, style from poet where p_id=#{id}")
Poet getById(Integer id);
//@Update("update poet set " +"name=#{name},gender=#{gender},dynasty=#{dynasty},title=#{title},style=#{style} where p_id=#{id}")
void update(Poet poet);
//诗人信息 - 条件查询
public List<Poet> list(@Param("author") String author, @Param("style") String style);
}
4、pojo
PageBean
package com.example.pojo;
import lombok.AllArgsConstructor;
import lombok.Data;
import lombok.NoArgsConstructor;
import java.util.List;
//分页查询结果封装
@Data
@AllArgsConstructor
@NoArgsConstructor
public class PageBean {
private Long total; // 总记录数
private List rows; // 数据列表
}
Poet
package com.example.pojo;
import lombok.AllArgsConstructor;
import lombok.Data;
import lombok.NoArgsConstructor;
import java.time.LocalDate;
import java.time.LocalDateTime;
@Data
@AllArgsConstructor
@NoArgsConstructor
public class Poet {
private Integer id; //id(主键)
private String author; //姓名
private String gender; //性别
private String dynasty; //朝代
private String title; //头衔
private String style; //风格
private LocalDateTime updateTime; //修改时间
}
Result
package com.example.pojo;
import lombok.AllArgsConstructor;
import lombok.Data;
import lombok.NoArgsConstructor;
@Data
@AllArgsConstructor
@NoArgsConstructor
public class Result {
Integer code;
String msg;
Object data;
public static Result success(){
return new Result(1,"success",null);
}
public static Result success(Object data){
return new Result(1,"success",data);
}
public static Result error(String msg){
return new Result(0,msg,null);
}
}
5、service
PoetService
package com.example.service;
import com.example.pojo.PageBean;
import com.example.pojo.Poet;
import java.util.List;
public interface PoetService {
PageBean page(Integer page, Integer pageSize, String author, String style);
List<Poet> selectData();
void insert(Poet poet);
void delete(Integer id);
Poet getById(Integer id);
void update(Poet poet);
}
PoetServiceA
package com.example.service.impl;
import com.example.mapper.PoetMapper;
import com.example.pojo.PageBean;
import com.example.pojo.Poet;
import com.example.service.PoetService;
import com.github.pagehelper.Page;
import com.github.pagehelper.PageHelper;
import com.github.pagehelper.PageInfo;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import java.util.List;
@Service
public class PoetServiceA implements PoetService {
@Autowired
private PoetMapper poetMapper;
@Override
public PageBean page(Integer page, Integer pageSize, String author, String style) {
// 设置分页参数
PageHelper.startPage(page, pageSize);
// 执行分页查询
List<Poet> poetList = poetMapper.list(author,style);
// System.out.println(peomList);
// 获取分页结果
PageInfo<Poet> p = new PageInfo<>(poetList);
//封装PageBean
PageBean pageBean = new PageBean(p.getTotal(), p.getList());
return pageBean;
}
public List<Poet> selectData(){
return poetMapper.selectPoet();
}
public void insert(Poet poet){
poetMapper.insert(poet);
}
public void delete(Integer id){
poetMapper.delete(id);
}
public Poet getById(Integer id){
return poetMapper.getById(id);
}
public void update(Poet poet){
poetMapper.update(poet);
}
}
6、xml文件
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapper
PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.example.mapper.PoetMapper">
<!-- 查询-->
<select id="selectPoet" resultType="com.example.pojo.Poet">
select * from poet
</select>
<!-- 条件分页查询-->
<select id="list" resultType="com.example.pojo.Poet">
select * from poet
<where>
<if test="author != null and author != '' ">
name like concat('%', #{author},'%')
</if>
<if test="style != null and style != '' ">
and style like concat('%',#{style},'%')
</if>
</where>
</select>
<!-- 新增-->
<insert id="insert">
insert into poet (author, gender, dynasty, title, style)
values (#{author},#{gender},#{dynasty},#{title},#{style})
</insert>
<!-- 删除-->
<delete id="delete">
delete from poet where p_id=#{id}
</delete>
<!-- 修改 先查询-->
<select id="getById" resultType="com.example.pojo.Poet">
select p_id as id, author, gender, dynasty, title, style
from poet
where p_id=#{id}
</select>
<!-- 再查询-->
<update id="update">
update poet
set author=#{author},gender=#{gender},dynasty=#{dynasty},title=#{title},style=#{style}
where p_id=#{id}
</update>
</mapper>
7、index6.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>分页条件查询</title>
<link rel="stylesheet" href="js/element.css">
</head>
<body style="align:center">
<div id="app" style="width: 80%;align:center">
<h1 align="center">诗人信息</h1>
<p align="center">
<el-form :inline="true" :model="formInline" class="demo-form-inline">
<el-form-item label="姓名">
<el-input v-model="formInline.author" placeholder="姓名"></el-input>
</el-form-item>
<el-form-item label="风格">
<el-input v-model="formInline.style" placeholder="风格"></el-input>
</el-form-item>
<el-form-item>
<el-button type="primary" @click="onSubmit">查询</el-button>
</el-form-item>
</el-form>
</p>
<el-table
:data="tableData.filter(data => !search || data.author.toLowerCase().includes(search.toLowerCase()))"
style="width: 100%;align:center;font-size: 20px">
<!-- <el-table-column-->
<!-- label="id"-->
<!-- prop="p_id">-->
<!-- </el-table-column>-->
<el-table-column
label="姓名"
prop="author">
</el-table-column>
<el-table-column
label="朝代"
prop="dynasty">
</el-table-column>
<el-table-column
label="头衔"
prop="title">
</el-table-column>
<el-table-column
label="风格"
prop="style">
</el-table-column>
<el-table-column
align="right">
<template slot="header" slot-scope="scope">
<el-input
v-model="search"
size="mini"
placeholder="输入关键字搜索"/>
</template>
<template slot-scope="scope">
<el-button
size="mini"
@click="handleEdit(scope.$index, scope.row)">编辑</el-button>
<el-button
size="mini"
type="danger"
@click="handleDelete(scope.$index, scope.row)">删除</el-button>
</template>
</el-table-column>
</el-table>
<p align="center">
<el-pagination
layout="total, sizes, prev, pager, next, jumper"
@size-change="handleSizeChange"
@current-change="handleCurrentChange"
:current-page="currentPage"
:page-sizes="[3, 5, 10, 20]"
:page-size="pageSize"
:total="total">
</el-pagination>
</p>
</div>
<!-- 引入组件库 -->
<script src="js/jquery.min.js"></script>
<script src="js/vue.js"></script>
<script src="js/element.js"></script>
<script src="js/axios-0.18.0.js"></script>
<script>
new Vue({
el:"#app",
data: {
search: '',
currentPage: 1,
pageSize: 4,
total: null,
tableData: [],
formInline: {
author: '',
style: ''
}
},
methods: {
handleEdit(index, row) {
console.log(index, row);
},
handleDelete(index, row) {
console.log(index, row);
},
handleSizeChange(val) {
this.pageSize = val;
this.findAll();
console.log(`每页 ${val} 条`);
},
handleCurrentChange(val) {
this.currentPage = val;
this.findAll();
console.log(`当前页: ${val}`);
},
onSubmit() {
var url = `/poets/${this.currentPage}/${this.pageSize}?author=${encodeURIComponent(this.formInline.author)}&style=${encodeURIComponent(this.formInline.style)}`
console.log(this.formInline.author);
console.log(this.formInline.style);
axios.get(url)
.then(res =>{
this.tableData = res.data.data.rows;
this.total=res.data.data.total;
console.log(this.tableData);
console.log(this.total);
})
.catch(error=>{
console.error(error);
})
},
findAll() {
var url = `/poets/${this.currentPage}/${this.pageSize}`
axios.get(url)
.then(res =>{
this.tableData = res.data.data.rows;
this.total=res.data.data.total;
console.log(this.tableData);
console.log(this.total);
})
.catch(error=>{
console.error(error);
})
}
},
created(){
this.findAll();
}
})
</script>
</body>
</html>
8、运行页面
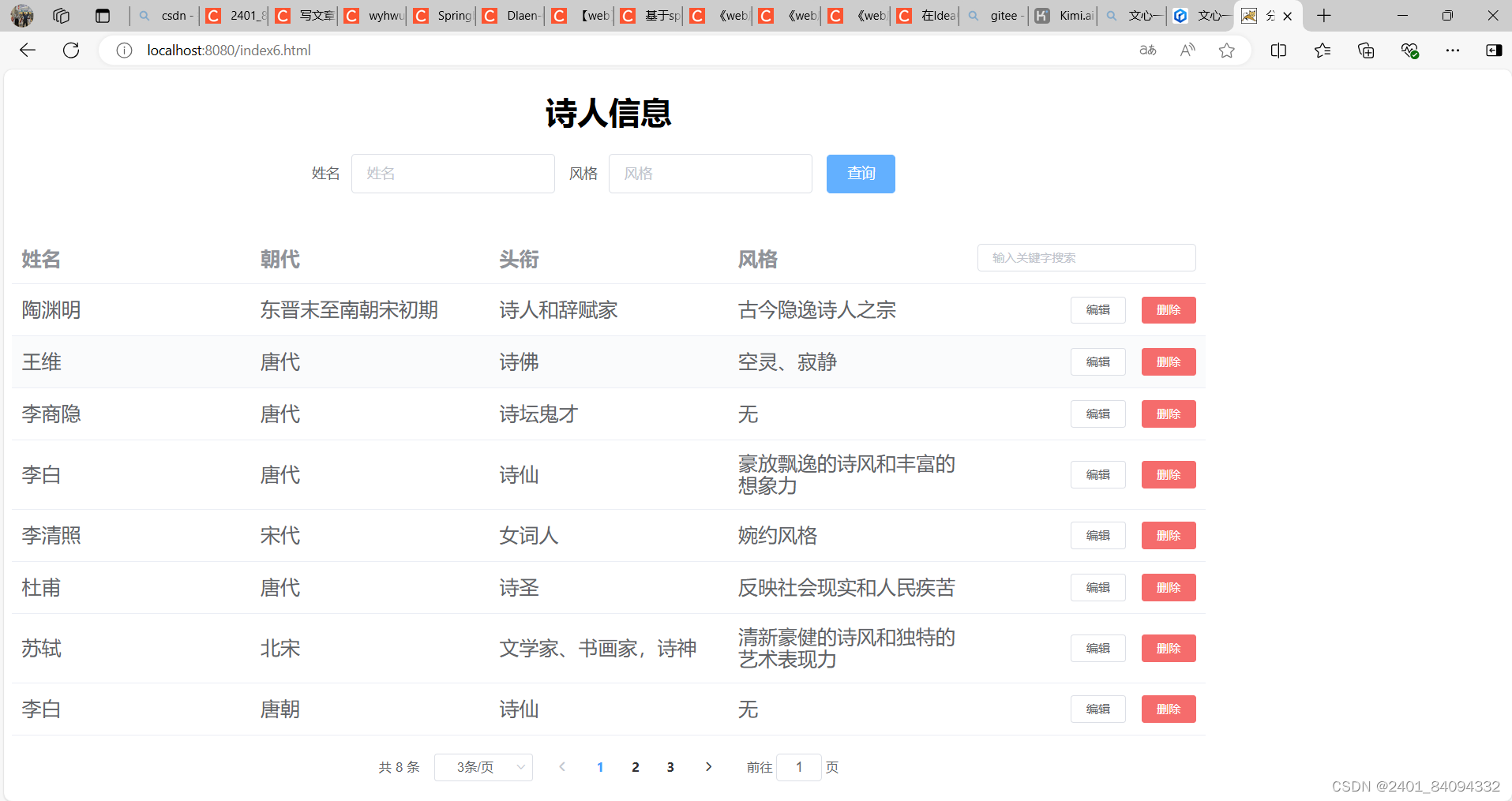
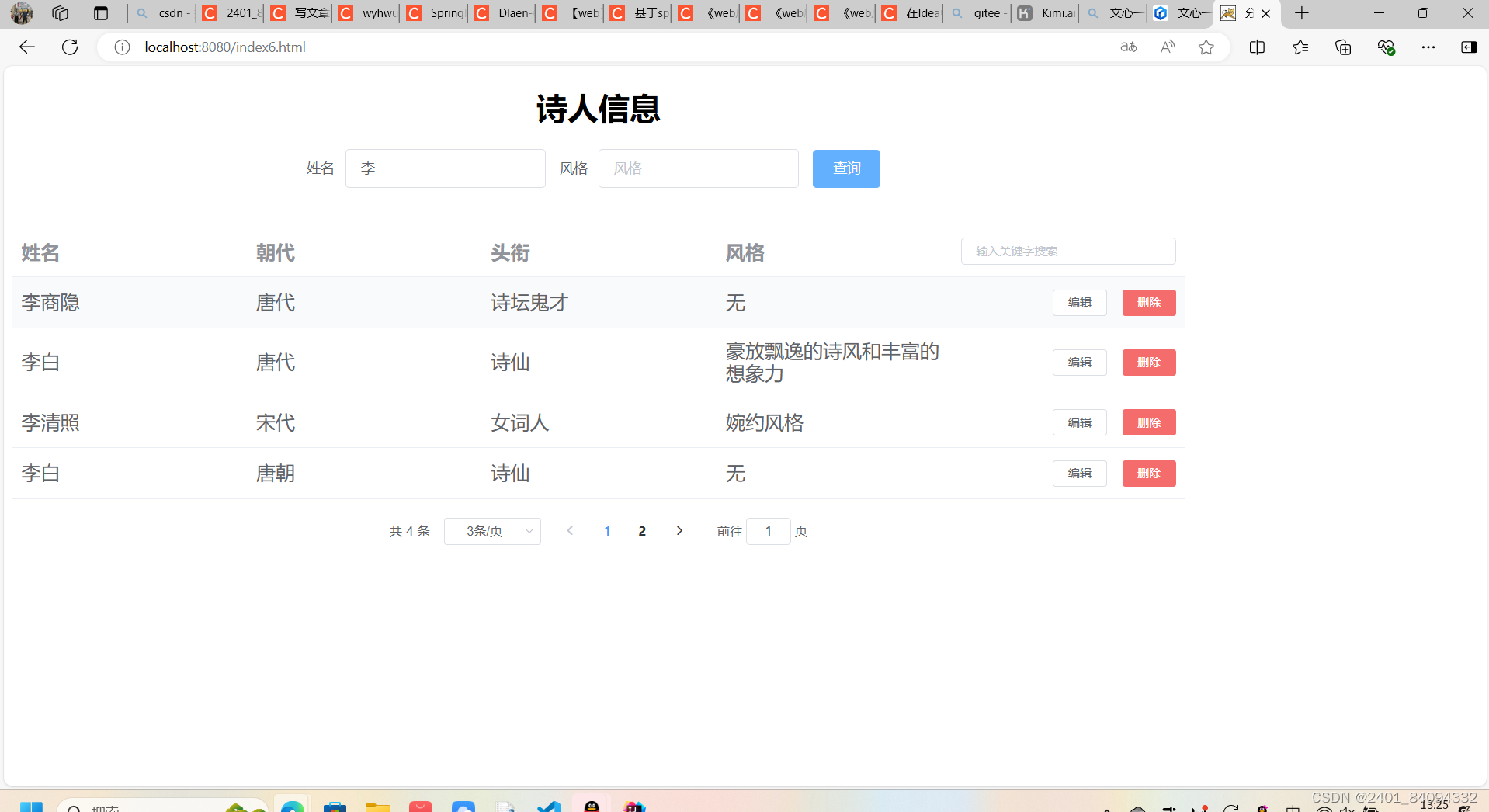