-
trimToSize(maxSize);
-
return createdValue;
-
}
-
}
-
/**
-
* Caches {@code value} for {@code key}. The value is moved to the head of
-
* the queue.
-
*
-
* @return the previous value mapped by {@code key}.
-
*/
-
public final V put(K key, V value) {
-
if (key == null || value == null) {
-
throw new NullPointerException(“key == null || value == null”);
-
}
-
V previous;
-
synchronized (this) {
-
putCount++;
-
size += safeSizeOf(key, value);
-
previous = map.put(key, value);
-
if (previous != null) { //返回的先前的value值
-
size -= safeSizeOf(key, previous);
-
}
-
}
-
if (previous != null) {
-
entryRemoved(false, key, previous, value);
-
}
-
trimToSize(maxSize);
-
return previous;
-
}
-
/**
-
* @param maxSize the maximum size of the cache before returning. May be -1
-
* to evict even 0-sized elements.
-
* 清空cache空间
-
*/
-
private void trimToSize(int maxSize) {
-
while (true) {
-
K key;
-
V value;
-
synchronized (this) {
-
if (size < 0 || (map.isEmpty() && size != 0)) {
-
throw new IllegalStateException(getClass().getName()
-
+ “.sizeOf() is reporting inconsistent results!”);
-
}
-
if (size <= maxSize) {
-
break;
-
}
-
Map.Entry<K, V> toEvict = map.eldest();
-
if (toEvict == null) {
-
break;
-
}
-
key = toEvict.getKey();
-
value = toEvict.getValue();
-
map.remove(key);
-
size -= safeSizeOf(key, value);
-
evictionCount++;
-
}
-
entryRemoved(true, key, value, null);
-
}
-
}
-
/**
-
* Removes the entry for {@code key} if it exists.
-
* 删除key相应的cache项,返回相应的value
-
* @return the previous value mapped by {@code key}.
-
*/
-
public final V remove(K key) {
-
if (key == null) {
-
throw new NullPointerException(“key == null”);
-
}
-
V previous;
-
synchronized (this) {
-
previous = map.remove(key);
-
if (previous != null) {
-
size -= safeSizeOf(key, previous);
-
}
-
}
-
if (previous != null) {
-
entryRemoved(false, key, previous, null);
-
}
-
return previous;
-
}
-
/**
-
* Called for entries that have been evicted or removed. This method is
-
* invoked when a value is evicted to make space, removed by a call to
-
* {@link #remove}, or replaced by a call to {@link #put}. The default
-
* implementation does nothing.
-
* 当item被回收或者删掉时调用。改方法当value被回收释放存储空间时被remove调用,
-
* 或者替换item值时put调用,默认实现什么都没做。
-
*
The method is called without synchronization: other threads may
-
* access the cache while this method is executing.
-
*
-
* @param evicted true if the entry is being removed to make space, false
-
* if the removal was caused by a {@link #put} or {@link #remove}.
-
* true—为释放空间被删除;false—put或remove导致
-
* @param newValue the new value for {@code key}, if it exists. If non-null,
-
* this removal was caused by a {@link #put}. Otherwise it was caused by
-
* an eviction or a {@link #remove}.
-
*/
-
protected void entryRemoved(boolean evicted, K key, V oldValue, V newValue) {}
-
/**
-
* Called after a cache miss to compute a value for the corresponding key.
-
* Returns the computed value or null if no value can be computed. The
-
* default implementation returns null.
-
* 当某Item丢失时会调用到,返回计算的相应的value或者null
-
*
The method is called without synchronization: other threads may
-
* access the cache while this method is executing.
-
*
-
*
If a value for {@code key} exists in the cache when this method
-
* returns, the created value will be released with {@link #entryRemoved}
-
* and discarded. This can occur when multiple threads request the same key
-
* at the same time (causing multiple values to be created), or when one
-
* thread calls {@link #put} while another is creating a value for the same
-
* key.
-
*/
-
protected V create(K key) {
-
return null;
-
}
-
private int safeSizeOf(K key, V value) {
-
int result = sizeOf(key, value);
-
if (result < 0) {
-
throw new IllegalStateException("Negative size: " + key + “=” + value);
-
}
-
return result;
-
}
-
/**
-
* Returns the size of the entry for {@code key} and {@code value} in
-
* user-defined units. The default implementation returns 1 so that size
-
* is the number of entries and max size is the maximum number of entries.
-
* 返回用户定义的item的大小,默认返回1代表item的数量,最大size就是最大item值
-
*
An entry’s size must not change while it is in the cache.
-
*/
-
protected int sizeOf(K key, V value) {
-
return 1;
-
}
-
/**
-
* Clear the cache, calling {@link #entryRemoved} on each removed entry.
-
* 清空cacke
-
*/
-
public final void evictAll() {
-
trimToSize(-1); // -1 will evict 0-sized elements
-
}
-
/**
-
* For caches that do not override {@link #sizeOf}, this returns the number
-
* of entries in the cache. For all other caches, this returns the sum of
-
* the sizes of the entries in this cache.
-
*/
-
public synchronized final int size() {
-
return size;
-
}
-
/**
-
* For caches that do not override {@link #sizeOf}, this returns the maximum
-
* number of entries in the cache. For all other caches, this returns the
-
* maximum sum of the sizes of the entries in this cache.
-
*/
-
public synchronized final int maxSize() {
-
return maxSize;
-
}
-
/**
-
* Returns the number of times {@link #get} returned a value that was
-
* already present in the cache.
-
*/
-
public synchronized final int hitCount() {
-
return hitCount;
-
}
-
/**
-
* Returns the number of times {@link #get} returned null or required a new
-
* value to be created.
-
*/
-
public synchronized final int missCount() {
-
return missCount;
-
}
-
/**
-
* Returns the number of times {@link #create(Object)} returned a value.
-
*/
-
public synchronized final int createCount() {
-
return createCount;
-
}
-
/**
-
* Returns the number of times {@link #put} was called.
-
*/
-
public synchronized final int putCount() {
-
return putCount;
-
}
-
/**
-
* Returns the number of values that have been evicted.
-
* 返回被回收的数量
-
*/
-
public synchronized final int evictionCount() {
-
return evictionCount;
-
}
-
/**
-
* Returns a copy of the current contents of the cache, ordered from least
-
* recently accessed to most recently accessed. 返回当前cache的副本,从最近最少访问到最多访问
-
*/
-
public synchronized final Map<K, V> snapshot() {
-
return new LinkedHashMap<K, V>(map);
-
}
-
@Override public synchronized final String toString() {
-
int accesses = hitCount + missCount;
-
int hitPercent = accesses != 0 ? (100 * hitCount / accesses) : 0;
-
return String.format(“LruCache[maxSize=%d,hits=%d,misses=%d,hitRate=%d%%]”,
-
maxSize, hitCount, missCount, hitPercent);
-
}
-
}
自我介绍一下,小编13年上海交大毕业,曾经在小公司待过,也去过华为、OPPO等大厂,18年进入阿里一直到现在。
深知大多数初中级Android工程师,想要提升技能,往往是自己摸索成长或者是报班学习,但对于培训机构动则近万的学费,着实压力不小。自己不成体系的自学效果低效又漫长,而且极易碰到天花板技术停滞不前!
因此收集整理了一份《2024年Android移动开发全套学习资料》,初衷也很简单,就是希望能够帮助到想自学提升又不知道该从何学起的朋友,同时减轻大家的负担。
既有适合小白学习的零基础资料,也有适合3年以上经验的小伙伴深入学习提升的进阶课程,基本涵盖了95%以上Android开发知识点,真正体系化!
由于文件比较大,这里只是将部分目录截图出来,每个节点里面都包含大厂面经、学习笔记、源码讲义、实战项目、讲解视频,并且会持续更新!
如果你觉得这些内容对你有帮助,可以扫码获取!!(备注:Android)
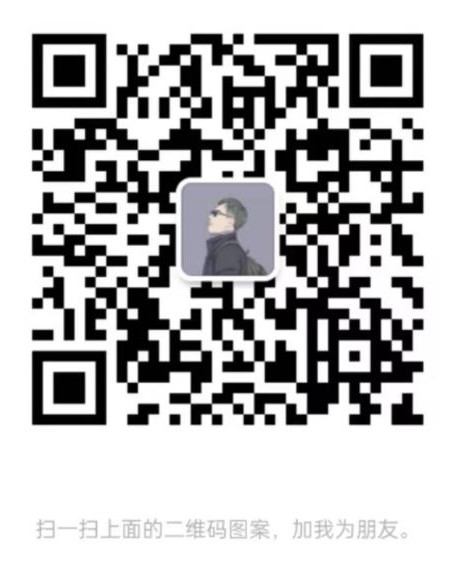
推荐学习资料
-
Android进阶学习全套手册
-
Android对标阿里P7学习视频
-
BAT TMD大厂Android高频面试题
《互联网大厂面试真题解析、进阶开发核心学习笔记、全套讲解视频、实战项目源码讲义》点击传送门即可获取!
里面都包含大厂面经、学习笔记、源码讲义、实战项目、讲解视频,并且会持续更新!**
如果你觉得这些内容对你有帮助,可以扫码获取!!(备注:Android)
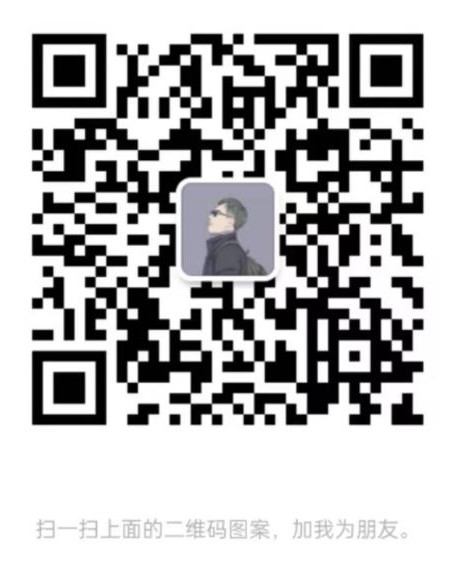
推荐学习资料
-
Android进阶学习全套手册
[外链图片转存中…(img-AOGVfTxA-1712431372233)]
-
Android对标阿里P7学习视频
[外链图片转存中…(img-LwG5brQL-1712431372233)]
-
BAT TMD大厂Android高频面试题
[外链图片转存中…(img-JXfynh2N-1712431372233)]
《互联网大厂面试真题解析、进阶开发核心学习笔记、全套讲解视频、实战项目源码讲义》点击传送门即可获取!