网上学习资料一大堆,但如果学到的知识不成体系,遇到问题时只是浅尝辄止,不再深入研究,那么很难做到真正的技术提升。
一个人可以走的很快,但一群人才能走的更远!不论你是正从事IT行业的老鸟或是对IT行业感兴趣的新人,都欢迎加入我们的的圈子(技术交流、学习资源、职场吐槽、大厂内推、面试辅导),让我们一起学习成长!
}
### 4.编写dao接口的实现类
java dao接口的实现类代码
package com.syj.dao.user;
import com.syj.dao.BaseDao;
import com.syj.entity.User;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
public class UserDaoImpl implements UserDao{
//得到要登录的用户
public User getLoginUser(Connection connection, String userCode) throws SQLException {
PreparedStatement pstm = null;
ResultSet rs = null;
User user = null;
if(connection != null){
String sql = "select \* from smbms\_user where userCode=?";
Object[] params = {userCode};
rs = BaseDao.execute(connection,pstm,rs,sql,params);
if(rs.next()){
user = new User();
user.setId(rs.getInt("id"));
user.setUserCode(rs.getString("userCode"));
user.setUserName(rs.getString("userName"));
user.setUserPassword(rs.getString("userPassword"));
user.setGender(rs.getInt("gender"));
user.setBirthday(rs.getDate("birthday"));
user.setPhone(rs.getString("phone"));
user.setAddress(rs.getString("address"));
user.setUserRole(rs.getInt("userRole"));
user.setCreatedBy(rs.getInt("createdBy"));
user.setCreationDate(rs.getTimestamp("creationDate"));
user.setModifyBy(rs.getInt("modifyBy"));
user.setModifyDate(rs.getTimestamp("modifyDate"));
}
BaseDao.closeResource(null,pstm,rs);
}
return user;
}
}
### 5.业务层接口
java service接口代码
package com.syj.service.user;
import com.syj.entity.User;
public interface UserService {
//用户登录
public User login(String userCode,String password);
}
### 6.业务层实现类
java serviceImpl实现类代码
package com.syj.service.user;
import com.syj.dao.BaseDao;
import com.syj.dao.user.UserDao;
import com.syj.dao.user.UserDaoImpl;
import com.syj.entity.User;
import java.sql.Connection;
import java.sql.SQLException;
public class UserServiceImpl implements UserService{
private UserDao userDao;
public UserServiceImpl(){
userDao = new UserDaoImpl();
}
public User login(String userCode, String password) {
Connection connection = null;
User user = null;
connection = BaseDao.getConnection();
try {
user = userDao.getLoginUser(connection,userCode);
} catch (SQLException e) {
e.printStackTrace();
}finally {
BaseDao.closeResource(connection,null,null);
}
return user;
}
}
### 7.编写Servlet
java 代码
package com.syj.servlet;
import com.syj.entity.User;
import com.syj.service.user.UserService;
import com.syj.service.user.UserServiceImpl;
import com.syj.util.Constants;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
public class LoginServlet extends HttpServlet {
@Override
protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
String userCode = req.getParameter("userCode");
String userPassword = req.getParameter("userPassword");
UserService userService = new UserServiceImpl();
User user = userService.login(userCode, userPassword);
if(user != null){
req.getSession().setAttribute(Constants.USER_SESSION,user);
resp.sendRedirect("jsp/frame.jsp");
}else{
req.setAttribute("error","用户名或者密码不正确");
req.getRequestDispatcher("login.jsp").forward(req,resp);
}
}
@Override
protected void doPost(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
doGet(req, resp);
}
}
### 8.注册servlet
xml代码
LoginServlet com.syj.servlet.LoginServlet LoginServlet /login.do
### 9.测试访问确保以上功能成功
## 三、登陆功能优化
### 注销功能:
思路:移除Session,返回登录界面
java servlet代码
package com.syj.servlet;
import com.syj.util.Constants;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
public class LogoutServlet extends HttpServlet {
@Override
protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
req.getSession().removeAttribute(Constants.USER_SESSION);
resp.sendRedirect(“/login.jsp”);
}
@Override
protected void doPost(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
doGet(req, resp);
}
}
xml 代码
<servlet>
<servlet-name>LogoutServlet</servlet-name>
<servlet-class>com.syj.servlet.LogoutServlet</servlet-class>
</servlet>
<servlet-mapping>
<servlet-name>LogoutServlet</servlet-name>
<url-pattern>/jsp/logout.do</url-pattern>
</servlet-mapping>
## 四、登录拦截优化
### 编写一个过滤器并注册
java 代码
package com.syj.filter;
import com.syj.entity.User;
import com.syj.util.Constants;
import javax.servlet.*;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
public class SysFilter implements Filter {
public void init(FilterConfig filterConfig) throws ServletException {
}
public void doFilter(ServletRequest req, ServletResponse resp, FilterChain chain) throws IOException, ServletException {
HttpServletRequest request = (HttpServletRequest) req;
HttpServletResponse response = (HttpServletResponse) resp;
User user = (User) request.getSession().getAttribute(Constants.USER_SESSION);
if(user==null){
response.sendRedirect("/smbms/error.jsp");
}else{
chain.doFilter(req,resp);
}
}
public void destroy() {
}
}
xml 代码
<filter>
<filter-name>SysFilter</filter-name>
<filter-class>com.syj.filter.SysFilter</filter-class>
</filter>
<filter-mapping>
<filter-name>SysFilter</filter-name>
<url-pattern>/jsp/*</url-pattern>
</filter-mapping>
测试,登录,注销,权限,以上功能都要保证执行成功
## 五、密码修改
### 1.导入前端素材
pwdmodify.jsp
>
> <li.><a.href=“${pageContext.request.contextPath }/jsp/pwdmodify.jsp”>密码修改</li.>
>
>
>
### 2.写项目,建议从底层向上写
### 3.UserDao接口
//修改用户密码
public int updatePwd(Connection connection,int id,int password) throws SQLException;
### 4.UserDao接口实现类
//修改用户密码
public int updatePwd(Connection connection, int id, int password) throws SQLException {
PreparedStatement pstm = null;
int execute = 0;
if(connection != null){
String sql = “update smbms_user set userPassword = ? where id = ?”;
Object[] params = {password,id};
execute = BaseDao.execute(connection,pstm,sql,params);
BaseDao.closeResource(null,pstm,null);
}
return execute;
}
### 5.UserService层
//根据用户Id修改密码
public boolean updatePwd(int id,int pwd);
### 6.UserService实现类
public boolean updatePwd(int id, int pwd) {
Connection connection = null;
boolean flag = false;
//修改密码
try {
connection = BaseDao.getConnection();
if(userDao.updatePwd(connection,id,pwd) > 0){
flag = true;
}
} catch (SQLException e) {
e.printStackTrace();
}finally {
BaseDao.closeResource(connection,null,null);
}
return false;
}
### 7. servlet控制器层
记得实现复用,需要提取方法
@Override
package com.mario.servlet;
import com.mario.entity.User;
import com.mario.service.user.UserService;
import com.mario.service.user.UserServiceImpl;
import com.mario.util.Constants;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
public class UserServlet extends HttpServlet {
@Override
protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
String method = req.getParameter("method");
if(method.equals("savepwd") && method != null){
this.updatePwd(req,resp);
}
}
@Override
protected void doPost(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
doGet(req, resp);
}
public void updatePwd(HttpServletRequest req, HttpServletResponse resp){
Object obj = req.getSession().getAttribute(Constants.USER_SESSION);
String newpassword = req.getParameter("newpassword");
System.out.println(newpassword);
boolean flag = false;
if(obj != null && newpassword != null){
UserService userService = new UserServiceImpl();
flag = userService.updatePwd(((User) obj).getId(), newpassword);
if(flag){
req.setAttribute("message","修改密码成功");
req.getSession().removeAttribute(Constants.USER_SESSION);
}else{
req.setAttribute("message","密码修改失败");
}
}else{
req.setAttribute("message","新密码有问题");
}
try {
req.getRequestDispatcher("pwdmodify.jsp").forward(req,resp);
} catch (ServletException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
}
}
### 8. 测试
### 优化密码修改使用Ajax
### 1.阿里巴巴的fastjson
pom.xml
com.alibaba fastjson 1.2.61
### 2.后台修改的代码
package com.mario.servlet;
import com.alibaba.fastjson.JSONArray;
import com.mario.entity.User;
import com.mario.service.user.UserService;
import com.mario.service.user.UserServiceImpl;
import com.mario.util.Constants;
import com.mysql.jdbc.StringUtils;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
import java.io.PrintWriter;
import java.util.HashMap;
import java.util.Map;
public class UserServlet extends HttpServlet {
@Override
protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
String method = req.getParameter("method");
if(method.equals("savepwd") && method != null){
this.updatePwd(req,resp);
}else if(method.equals("pwdmodify") && method != null){
this.pwdModify(req,resp);
}
}
@Override
protected void doPost(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
doGet(req, resp);
}
//修改密码
public void updatePwd(HttpServletRequest req, HttpServletResponse resp){
Object obj = req.getSession().getAttribute(Constants.USER_SESSION);
String newpassword = req.getParameter("newpassword");
System.out.println(newpassword);
boolean flag = false;
if(obj != null && newpassword != null){
UserService userService = new UserServiceImpl();
flag = userService.updatePwd(((User) obj).getId(), newpassword);
if(flag){
req.setAttribute("message","修改密码成功");
req.getSession().removeAttribute(Constants.USER_SESSION);
}else{
req.setAttribute("message","密码修改失败");
}
}else{
req.setAttribute("message","新密码有问题");
}
try {
req.getRequestDispatcher("pwdmodify.jsp").forward(req,resp);
} catch (ServletException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
}
//验证旧密码,session中有用户的密码
public void pwdModify(HttpServletRequest req, HttpServletResponse resp){
Object obj = req.getSession().getAttribute(Constants.USER_SESSION);
String oldpassword = req.getParameter("oldpassword");
Map<String, String> resultMap = new HashMap<String, String>();
if(obj==null){
resultMap.put("result","sessionerror");
}else if(StringUtils.isNullOrEmpty(oldpassword)){
resultMap.put("result","error");
}else{
String userPassword = ((User) obj).getUserPassword();
if(oldpassword.equals(userPassword)){
resultMap.put("result","true");
}else{
resultMap.put("result","false");
}
}
try {
resp.setContentType("application/json");
PrintWriter writer = resp.getWriter();
writer.write(JSONArray.toJSONString(resultMap));
writer.flush();
writer.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
### 3.测试
## 六、用户管理实现
### 1.获取用户数量
#### 1.UserDao
//查询用户总数
public int getUserCount(Connection connection,String username,int userRole) throws SQLException;
#### 2.UserDaoImpl
public int getUserCount(Connection connection, String username, int userRole) throws SQLException {
PreparedStatement pstm = null;
ResultSet rs = null;
int count = 0;
if(connection != null){
StringBuilder sql = new StringBuilder();
sql.append("select count(1) as count from smbms\_user u,smbms\_role r where u.userRole = r.id");
ArrayList<Object> list = new ArrayList<Object>();
if(!StringUtils.isNullOrEmpty(username)){
sql.append(" and u.userName like ? ");
list.add("%"+username+"%");
}
if(userRole>0){
sql.append(" and u.userRole = ? ");
list.add(userRole);
}
Object[] params = list.toArray();
rs = BaseDao.execute(connection,pstm,rs,sql.toString(),params);
if(rs.next()){
count = rs.getInt("count");
}
BaseDao.closeResource(null,pstm,rs);
}
return count;
}
#### 3.UserService
//查询记录数
public int getUserCount(String username,int userRole);
#### 4.UserServiceImpl
public int getUserCount(String username, int userRole) {
Connection connection = null;
int count = 0;
try {
connection = BaseDao.getConnection();
count = userDao.getUserCount(connection,username,userRole);
} catch (SQLException e) {
e.printStackTrace();
}finally {
BaseDao.closeResource(connection,null,null);
}
return count;
}
### 2.用户列表页导入
#### 1.UserDao
//通过用户输入的条件查询用户列表
public List getUserList(Connection connection, String userName, int userRole, int currentPageNo, int pageSize) throws Exception;
#### 2.UserDaoImpl
//通过用户输入的条件查询用户列表
public List getUserList(Connection connection, String userName, int userRole, int currentPageNo, int pageSize) throws Exception {
List userList = new ArrayList();
PreparedStatement pstm=null;
ResultSet rs=null;
if(connection!=null){
StringBuffer sql = new StringBuffer();
sql.append(“select u.*,r.roleName as userRoleName from smbms_user u,smbms_role r where u.userRole = r.id”);
List list = new ArrayList();
if(!StringUtils.isNullOrEmpty(userName)){
sql.append(" and u.userName like ?“);
list.add(”%“+userName+”%“);
}
if(userRole > 0){
sql.append(” and u.userRole = ?“);
list.add(userRole);
}
sql.append(” order by creationDate DESC limit ?,?");
currentPageNo = (currentPageNo-1)*pageSize;
list.add(currentPageNo);
list.add(pageSize);
Object[] params = list.toArray();
System.out.println("sql ----> " + sql.toString());
rs = BaseDao.execute(connection,pstm,rs,sql.toString(),params);
while(rs.next()){
User _user = new User();
_user.setId(rs.getInt("id"));
_user.setUserCode(rs.getString("userCode"));
_user.setUserName(rs.getString("userName"));
_user.setGender(rs.getInt("gender"));
_user.setBirthday(rs.getDate("birthday"));
_user.setPhone(rs.getString("phone"));
_user.setUserRole(rs.getInt("userRole"));
_user.setUserRoleName(rs.getString("userRoleName"));
userList.add(_user);
}
BaseDao.closeResource(null, pstm, rs);
}
return userList;
}
#### 3.UserService
//根据条件查询用户列表
public List getUserList(String queryUserName, int queryUserRole, int currentPageNo, int pageSize);
#### 4.UserServiceImpl
public List getUserList(String queryUserName, int queryUserRole, int currentPageNo, int pageSize) {
Connection connection = null;
List userList = null;
try {
connection = BaseDao.getConnection();
userList = userDao.getUserList(connection, queryUserName,queryUserRole,currentPageNo,pageSize);
} catch (Exception e) {
e.printStackTrace();
}finally{
BaseDao.closeResource(connection, null, null);
}
return userList;
}
### 3.获取角色操作
#### 1.RoleDao
//获取角色列表
public List getRoleList(Connection connection) throws SQLException;
#### 2.RoleDaoImpl
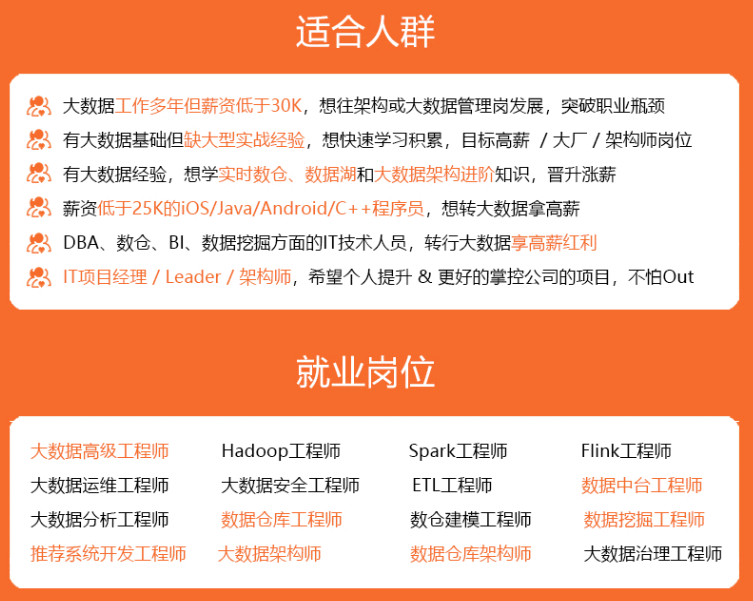
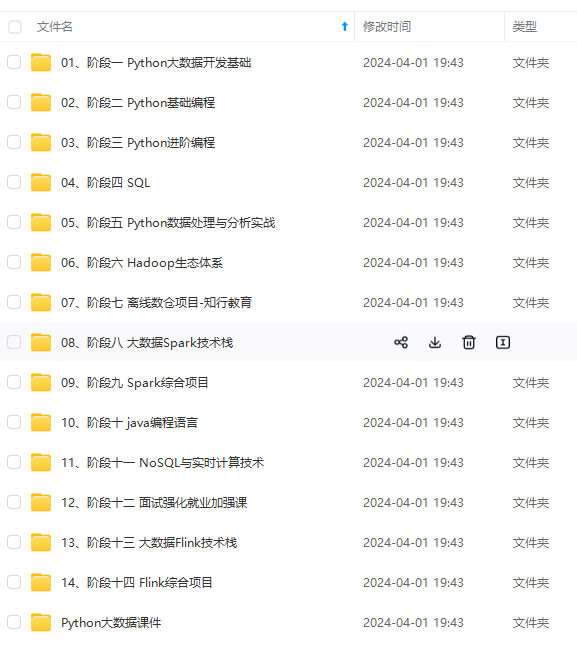
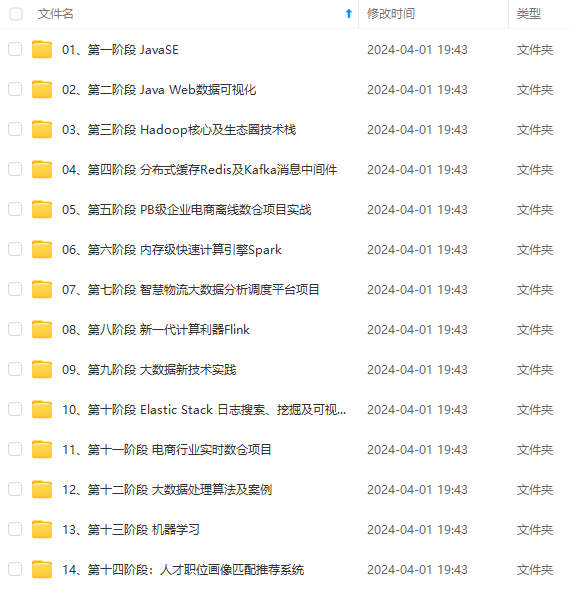
**既有适合小白学习的零基础资料,也有适合3年以上经验的小伙伴深入学习提升的进阶课程,涵盖了95%以上大数据知识点,真正体系化!**
**由于文件比较多,这里只是将部分目录截图出来,全套包含大厂面经、学习笔记、源码讲义、实战项目、大纲路线、讲解视频,并且后续会持续更新**
**[需要这份系统化资料的朋友,可以戳这里获取](https://bbs.csdn.net/topics/618545628)**
serList = userDao.getUserList(connection, queryUserName,queryUserRole,currentPageNo,pageSize);
} catch (Exception e) {
e.printStackTrace();
}finally{
BaseDao.closeResource(connection, null, null);
}
return userList;
}
3.获取角色操作
1.RoleDao
//获取角色列表
public List<Role> getRoleList(Connection connection) throws SQLException;
2.RoleDaoImpl
[外链图片转存中…(img-P1o9YhrV-1715834655555)]
[外链图片转存中…(img-xBSfqWYO-1715834655555)]
[外链图片转存中…(img-rTcFxuoI-1715834655556)]
既有适合小白学习的零基础资料,也有适合3年以上经验的小伙伴深入学习提升的进阶课程,涵盖了95%以上大数据知识点,真正体系化!
由于文件比较多,这里只是将部分目录截图出来,全套包含大厂面经、学习笔记、源码讲义、实战项目、大纲路线、讲解视频,并且后续会持续更新