import React, {Component, createRef} from "react";
export default class TodoInput extends Component{
state={
inputValue:'输入代办事件', // 定义input输入框内容
}
inputRef=createRef() // 定义ref绑定DOM元素,作用是为下面自动获取焦点做准备
// 输入框中输入的内容设置给state作用1:输入框内容改变2:后面提交添加事件拿到input内容
handleChang=Event=>{
this.setState({
inputValue:Event.target.value
})
}
// 添加代办事件
handleDown=Event=>{
// 验证下是否为空
if(this.state.inputValue==='' || this.state.inputValue===null) return
if(Event.keyCode ===13){
this.add()
}
}
// 添加处理函数
add=()=>{
// add方法通过props从App传入
this.props.add(this.state.inputValue)
this.state.inputValue=''
// ref绑定后通过inputRef.current拿到DOM元素
this.inputRef.current.focus()
}
render() {
return(
<div className="panel-block">
<p className="control has-icons-left">
<input
className="input is-success"
type="text"
placeholder="输入代办事项"
value={this.state.inputValue}
onChange={this.handleChang}
onKeyDown={this.handleDown.bind(this)} //该变this指向
ref={this.inputRef}
/>
</p>
</div>
)
}
}
- 官方解析图
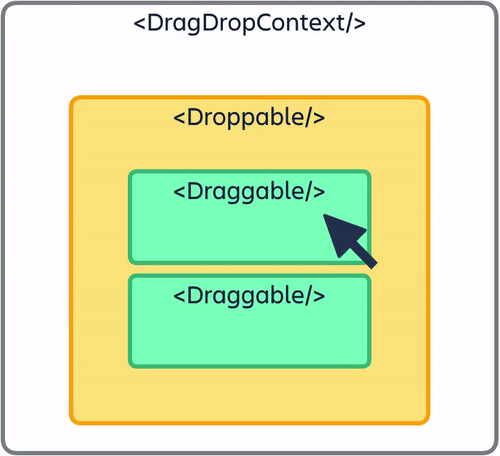
- 格式DragDropContext最外面盒子Droppable第二层盒子
<DragDropContext onDragEnd={this.onDragEnd}>
<Droppable droppableId='columns'>
{provided=>(
<\*
ref={provided.innerRef}
{...provided.droppableProps}
// 官方固定格式
>
自己的代码
<\*/>
{provided.placeholder}
)}
</Droppable>
</DragDropContext>
<Draggable draggableId={String(id)} index={this.props.index}>
{provided=>(
<\*
{...provided.draggableProps}
{...provided.dragHandleProps}
ref={provided.innerRef}
>
自己的代码
<\*/>
{provided.placeholder}
)}
</Draggable>
- 一但移动(从第5个事件移动到第4个事件)onDragEnd回调函数打印结果console.log(result)
{draggableId: '5', type: 'DEFAULT', source: {…}, reason: 'DROP', mode: 'FLUID', …}
combine: null
destination: {droppableId: 'columns', index: 4} // 移动到第4个事件
draggableId: "5"
mode: "FLUID"
reason: "DROP"
source: {index: 5, droppableId: 'columns'} // 移动的第5个事件
type: "DEFAULT"
[[Prototype]]: Object
components/TodoList.jsx
import React,{Component} from "react";
import TodoItem from "./TodoItem";
import PropTypes from 'prop-types'
import {DragDropContext} from 'react-beautiful-dnd'
import {Droppable} from 'react-beautiful-dnd'
export default class TodoList extends Component{
// 类型检查
static propTypes={
todos:PropTypes.array.isRequired,
}
// 默认值
static defaultProps = {
todos: [],
}
// 根据choice数值决定渲染事项
state={
choice:1
}
// react-beautiful-dnd核心处理函数,负责交换后的处理(可以自定义)
onDragEnd=result=>{
console.log(result)
const {destination,source,draggableId}=result
if(!destination){ // 移动到了视图之外
return
}
if( // 移动到原来位置,也就是位置不变
destination.droppableId===source.droppableId &&
destination.index===source.index
){ return;}
const newTaskIds=Array.from(this.props.todos) // 转化为真正的数组
newTaskIds.splice(source.index,1) // 删除移动的数组
newTaskIds.splice(destination.index,0,this.props.todos[source.index]) // 在移动到的位置初放置被删除的数组
// 调用App文件中的drag执行交换后的更改
this.props.drag(newTaskIds)
}
// 点击时渲染不同DOM
choice=(num)=>{
this.setState({
choice:num
})
}
render() {
let uls=null
if(this.state.choice===1){
uls=(<DragDropContext onDragEnd={this.onDragEnd}>
<Droppable droppableId='columns'>
{provided=>(
<ul
ref={provided.innerRef}
{...provided.droppableProps}
>
{this.props.todos.length>0
? this.props.todos.map((todo,index)=>{
return (
<TodoItem key={todo.id} todo={todo} index={index}/>
)
})
:<div>添加代办事项</div>
}
{provided.placeholder}
</ul>
)}
</Droppable>
</DragDropContext>)
}else if(this.state.choice===2){
// 过滤下事件
let newtodos=this.props.todos.filter(todo=> todo.completed)
uls=(<DragDropContext onDragEnd={this.onDragEnd}>
<Droppable droppableId='columns'>
{provided=>(
<ul
ref={provided.innerRef}
{...provided.droppableProps}
>
{newtodos.length>0
? newtodos.map((todo,index)=>{
return (
<TodoItem key={todo.id} todo={todo} index={index}/>
)
})
:<div>暂无已完成事件</div>
}
{provided.placeholder}
</ul>
)}
</Droppable>
</DragDropContext>)
}else if(this.state.choice===3){
// 过滤下事件
let newtodos=this.props.todos.filter(todo=> !todo.completed)
uls=(<DragDropContext onDragEnd={this.onDragEnd}>
<Droppable droppableId='columns'>
{provided=>(
<ul
ref={provided.innerRef}
{...provided.droppableProps}
>
{newtodos.length>0
? newtodos.map((todo,index)=>{
return (
<TodoItem key={todo.id} todo={todo} index={index}/>
)
})
:<div>暂无未完成事件</div>
}
{provided.placeholder}
</ul>
)}
</Droppable>
</DragDropContext>)
}
return(
<>
<p className="panel-tabs">
<a className="is-active" onClick={()=>this.choice(1)}>所有</a>
<a onClick={()=>this.choice(2)}>已完成</a>
<a onClick={()=>this.choice(3)}>未完成</a>
</p>
{uls}
</>
)
}
}
components/TodoFoot.jsx
return(
<Consumer>
{value=>{
const {结构要用的属性或方法的名字} = value
return(
自己的代码,value中含有Provider中传入的所有值
)
}}
</Consumer>
)
import React,{Component} from "react";
import {Consumer} from '../untils/with-context'
export default class TodoFoot extends Component{
render() {
return(
<Consumer>
{
value => {
const {allCheckbox,todos,delCompelted} = value
const completedNum =todos.filter(todo=>todo.completed).length
const AllChecked =todos.length?todos.every(todo=>todo.completed):false
return(
<div>
<label className="panel-block">
<input type="checkbox" checked={AllChecked} onChange={(event)=>allCheckbox(event.target.checked)}/>全选
<span>已完成{completedNum}</span>
<span>一共{todos.length}</span>
</label>
<div className="panel-block">
<button className="button is-success is-outlined is-fullwidth" onClick={delCompelted}>
删除已完成
</button>
</div>
</div>
)
}
}
</Consumer>
)
}
}
package.json中的三方包资源
{
"name": "react-demo",
"version": "0.1.0",
"private": true,
"dependencies": {
"@testing-library/jest-dom": "^5.11.4",
"@testing-library/react": "^11.1.0",
"@testing-library/user-event": "^12.1.10",
"bulma-start": "^0.0.5",
"react": "^17.0.2",
"react-beautiful-dnd": "^13.1.0",
"react-dom": "^17.0.2",
"react-native": "^0.68.2",
"react-scripts": "4.0.3",
"redux-persist": "^6.0.0",
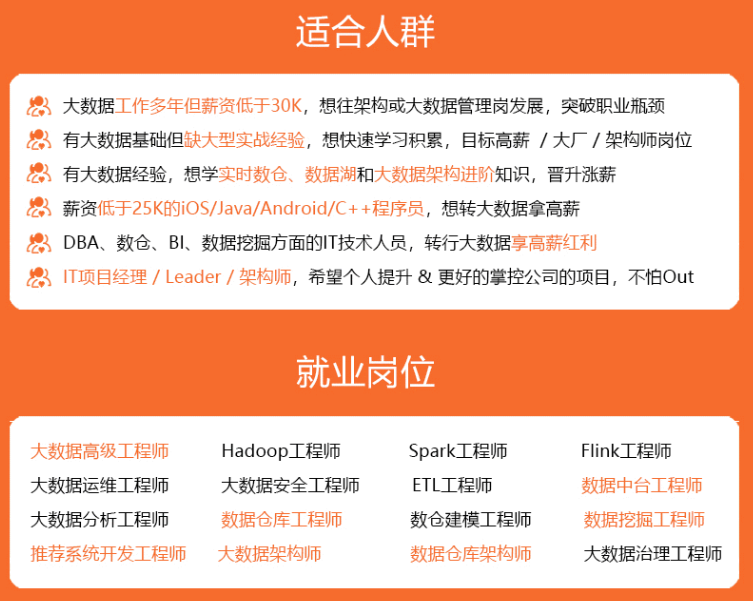
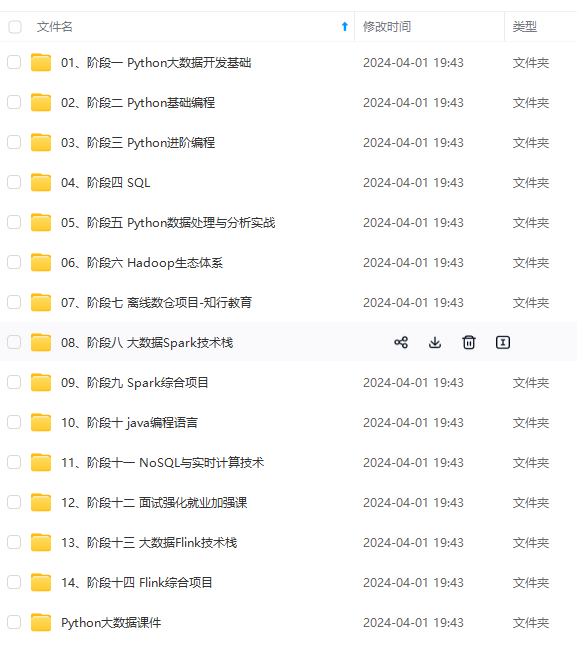
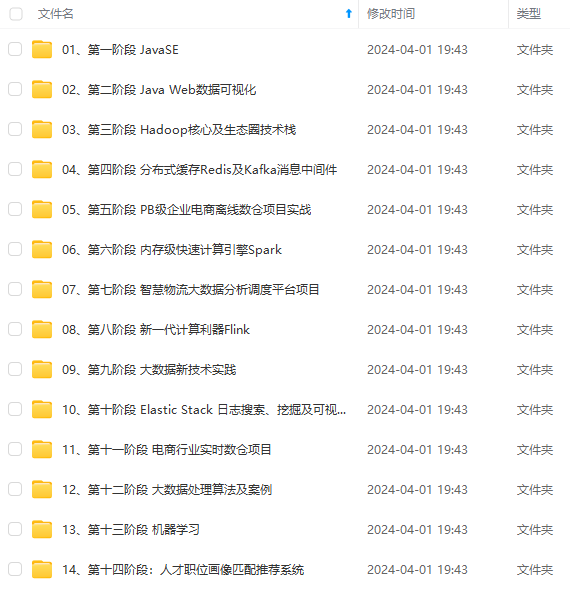
**既有适合小白学习的零基础资料,也有适合3年以上经验的小伙伴深入学习提升的进阶课程,涵盖了95%以上大数据知识点,真正体系化!**
**由于文件比较多,这里只是将部分目录截图出来,全套包含大厂面经、学习笔记、源码讲义、实战项目、大纲路线、讲解视频,并且后续会持续更新**
**[需要这份系统化资料的朋友,可以戳这里获取](https://bbs.csdn.net/topics/618545628)**
6.0.0",
[外链图片转存中...(img-sJzGkvP0-1714161615351)]
[外链图片转存中...(img-F42qwRvH-1714161615352)]
[外链图片转存中...(img-NUzPMp6l-1714161615352)]
**既有适合小白学习的零基础资料,也有适合3年以上经验的小伙伴深入学习提升的进阶课程,涵盖了95%以上大数据知识点,真正体系化!**
**由于文件比较多,这里只是将部分目录截图出来,全套包含大厂面经、学习笔记、源码讲义、实战项目、大纲路线、讲解视频,并且后续会持续更新**
**[需要这份系统化资料的朋友,可以戳这里获取](https://bbs.csdn.net/topics/618545628)**