网上学习资料一大堆,但如果学到的知识不成体系,遇到问题时只是浅尝辄止,不再深入研究,那么很难做到真正的技术提升。
一个人可以走的很快,但一群人才能走的更远!不论你是正从事IT行业的老鸟或是对IT行业感兴趣的新人,都欢迎加入我们的的圈子(技术交流、学习资源、职场吐槽、大厂内推、面试辅导),让我们一起学习成长!
let mut queue = std::collections::VecDeque::new();
queue.push\_back(tree);
while !queue.is\_empty() {
let mut head = Some(Box::new(ListNode::new(0)));
let mut tail = head.as\_mut();
let size = queue.len();
for _ in 0..size {
let node = queue.pop\_front().unwrap().unwrap();
let mut node = node.borrow\_mut();
if node.left.is\_some() {
queue.push\_back(node.left.take());
}
if node.right.is\_some() {
queue.push\_back(node.right.take());
}
tail.as\_mut().unwrap().next = Some(Box::new(ListNode::new(node.val)));
tail = tail.unwrap().next.as\_mut();
}
ans.push(head.as\_mut().unwrap().next.take());
}
ans
}
}
---
### go
/**
* Definition for a binary tree node.
* type TreeNode struct {
* Val int
* Left *TreeNode
* Right *TreeNode
* }
*/
/**
* Definition for singly-linked list.
* type ListNode struct {
* Val int
* Next *ListNode
* }
*/
func listOfDepth(tree *TreeNode) []*ListNode {
var ans []*ListNode
queue := []*TreeNode{tree}
for len(queue) > 0 {
head := &ListNode{}
tail := head
size := len(queue)
for i := 0; i < size; i++ {
node := queue[i]
if node.Left != nil {
queue = append(queue, node.Left)
}
if node.Right != nil {
queue = append(queue, node.Right)
}
tail.Next = &ListNode{Val: node.Val}
tail = tail.Next
}
ans = append(ans, head.Next)
queue = queue[size:]
}
return ans
}
---
### typescript
/**
* Definition for a binary tree node.
* class TreeNode {
* val: number
* left: TreeNode | null
* right: TreeNode | null
* constructor(val?: number, left?: TreeNode | null, right?: TreeNode | null) {
* this.val = (val=undefined ? 0 : val)
* this.left = (left=undefined ? null : left)
* this.right = (right===undefined ? null : right)
* }
* }
*/
/**
* Definition for singly-linked list.
* class ListNode {
* val: number
* next: ListNode | null
* constructor(val?: number, next?: ListNode | null) {
* this.val = (val=undefined ? 0 : val)
* this.next = (next=undefined ? null : next)
* }
* }
*/
function listOfDepth(tree: TreeNode | null): Array<ListNode | null> {
const ans = [];
const queue = [tree];
while (queue.length > 0) {
const head = new ListNode();
let tail = head;
const size = queue.length;
for (let i = 0; i < size; ++i) {
const { val, left, right } = queue.shift();
left && queue.push(left);
right && queue.push(right);
tail.next = new ListNode(val);
tail = tail.next;
}
ans.push(head.next);
}
return ans;
};
---
### python
Definition for a binary tree node.
class TreeNode:
def __init__(self, x):
self.val = x
self.left = None
self.right = None
Definition for singly-linked list.
class ListNode:
def __init__(self, x):
self.val = x
self.next = None
class Solution:
def listOfDepth(self, tree: TreeNode) -> List[ListNode]:
ans = []
q = collections.deque()
q.append(tree)
while len(q) > 0:
head = ListNode()
tail = head
size = len(q)
for _ in range(size):
node = q.popleft()
node.left and q.append(node.left)
node.right and q.append(node.right)
tail.next = ListNode(node.val)
tail = tail.next
ans.append(head.next)
return ans
---
### c
/**
* Definition for a binary tree node.
* struct TreeNode {
* int val;
* struct TreeNode *left;
* struct TreeNode *right;
* };
*/
/**
* Definition for singly-linked list.
* struct ListNode {
* int val;
* struct ListNode *next;
* };
*/
int getDepth(struct TreeNode* tree) {
if (!tree) {
return 0;
}
int leftDepth = getDepth(tree->left);
int rightDepth = getDepth(tree->right);
return fmax(leftDepth, rightDepth) + 1;
}
/**
* Note: The returned array must be malloced, assume caller calls free().
*/
struct ListNode** listOfDepth(struct TreeNode* tree, int* returnSize){
int depth = getDepth(tree);
struct ListNode **ans = malloc(depth * sizeof(struct ListNode *));
*returnSize = 0;
struct TreeNode *queue[(int) pow(2, depth) - 1];
queue[0] = tree;
int start = 0;
int end = 1;
while (start < end) {
struct ListNode head = {};
struct ListNode *tail = &head;
int curEnd = end;
while (start < curEnd) {
struct TreeNode *node = queue[start++];
if (node->left) {
queue[end++] = node->left;
}
if (node->right) {
queue[end++] = node->right;
}
tail->next = malloc(sizeof(struct ListNode));
tail->next->val = node->val;
tail->next->next = NULL;
tail = tail->next;
}
ans[(*returnSize)++] = head.next;
}
return ans;
}
---
### c++
/**
* Definition for a binary tree node.
* struct TreeNode {
* int val;
* TreeNode *left;
* TreeNode *right;
* TreeNode(int x) : val(x), left(NULL), right(NULL) {}
* };
*/
/**
* Definition for singly-linked list.
* struct ListNode {
* int val;
* ListNode *next;
* ListNode(int x) : val(x), next(NULL) {}
* };
*/
class Solution {
public:
vector<ListNode*> listOfDepth(TreeNode* tree) {
vector<ListNode *> ans;
queue<TreeNode \*> q;
q.push(tree);
while (q.size() > 0) {
ListNode head = ListNode(0);
ListNode \*tail = &head;
int size = q.size();
for (int i = 0; i < size; ++i) {
TreeNode \*node = q.front();
q.pop();
if (node->left != NULL) {
q.push(node->left);
}
if (node->right != NULL) {
q.push(node->right);
}
tail->next = new ListNode(node->val);
tail = tail->next;
}
ans.emplace\_back(head.next);
}
return ans;
}
};
---
### java
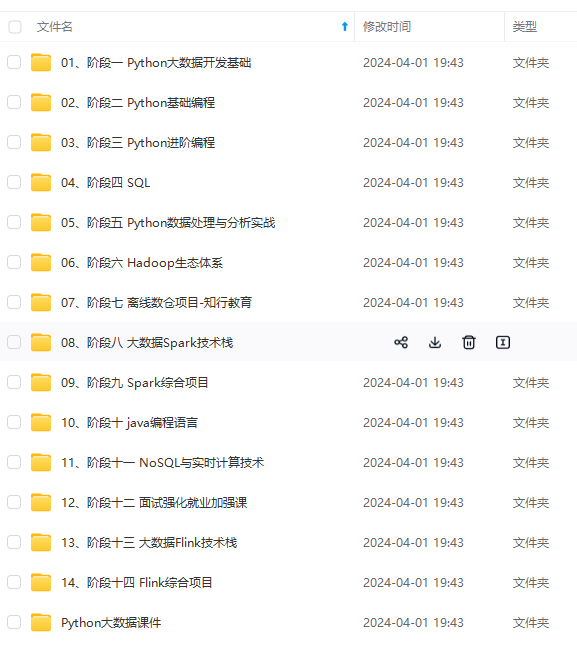
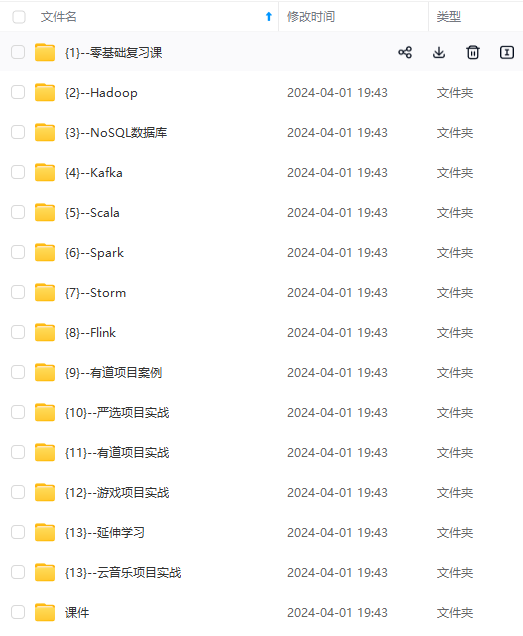
**网上学习资料一大堆,但如果学到的知识不成体系,遇到问题时只是浅尝辄止,不再深入研究,那么很难做到真正的技术提升。**
**[需要这份系统化资料的朋友,可以戳这里获取](https://bbs.csdn.net/topics/618545628)**
**一个人可以走的很快,但一群人才能走的更远!不论你是正从事IT行业的老鸟或是对IT行业感兴趣的新人,都欢迎加入我们的的圈子(技术交流、学习资源、职场吐槽、大厂内推、面试辅导),让我们一起学习成长!**
-
### java
[外链图片转存中...(img-cLaQT1t9-1715598848360)]
[外链图片转存中...(img-po3wHLg4-1715598848360)]
**网上学习资料一大堆,但如果学到的知识不成体系,遇到问题时只是浅尝辄止,不再深入研究,那么很难做到真正的技术提升。**
**[需要这份系统化资料的朋友,可以戳这里获取](https://bbs.csdn.net/topics/618545628)**
**一个人可以走的很快,但一群人才能走的更远!不论你是正从事IT行业的老鸟或是对IT行业感兴趣的新人,都欢迎加入我们的的圈子(技术交流、学习资源、职场吐槽、大厂内推、面试辅导),让我们一起学习成长!**