//点
Mypoint begPos = { 1,1 };
Mypoint endPos = { 8,8 };
//辅助地图,标记起点走过
pathMap PathMap[ROWS][COLS] = { 0 };
PathMap[begPos.row][begPos.row].isFind = true;
//栈 起点入栈
Stack stack;
init(&stack);
push(&stack, &begPos);
//标记没有找到终点
bool isFindEnd = false;
//当前点
Mypoint currentPos;
currentPos.row = begPos.row;
currentPos.col = begPos.col;
//预测点
Mypoint searchPos;
🤡4、死胡同问题
回退机制
栈结构:先入后出,后入先出
1 走过就入栈
2 遇到死胡同(每个方向都试过还不能走 最后一个方向右都不能走)回退
2.1 pop 出栈一个
2.2 跳到当前栈顶元素处
例如下图,当走到8,1的时候遇到死胡同,这里只需要把栈顶元素删掉,然后跳到当前栈顶元素
😼5、Stack代码
.h文件
#ifndef \_MY\_STACK\_H\_
#define \_MY\_STACK\_H\_
#include"Mytypes.h"
#include<string.h>
#include<stdlib.h>
typedef struct Stack
{
Mypoint\* pArr; //记录内存首地址
int size; //记录当前元素个数
int capacity; //记录当前容量
}Stack;
//初始化
void init(Stack\* S);
//添加元素
void push(Stack\* S, Mypoint\* pos);
//删除元素
void pop(Stack\* S);
//获取栈顶元素
Mypoint\* getTop(Stack\* S);
//判断栈是否为空
bool isEmpty(Stack\* S);
#endif // !\_MY\_STACK\_H\_
.c文件
#include "MyStack.h"
#include<assert.h>
void init(Stack\* S)
{
S->size = S->capacity = 0;
S->pArr = NULL;
}
void push(Stack\* S, Mypoint\* pos)
{
if ( S->size >= S->capacity )
{
S->capacity += (((S->capacity >> 1) > 1) ? (S->capacity >> 1) : 1);
Mypoint\* king = malloc(sizeof(Mypoint) \* (S->capacity));
assert(king);
if ( S->pArr )
{
memcpy(king, S->pArr, sizeof(Mypoint) \* (S->size));
free(S->pArr);
}
S->pArr = king;
}
S->pArr[S->size].row = pos->row;
S->pArr[S->size].col = pos->col;
S->size++;
}
void pop(Stack\* S)
{
S->size--;
}
Mypoint\* getTop(Stack\* S)
{
return (S->pArr + (S->size - 1));
}
bool isEmpty(Stack\* S)
{
return (S->size == 0);
}
💝6、算法代码
.h文件
#ifndef \_MY\_TYPES\_H\_
#define \_MY\_TYPES\_H\_
#include<stdio.h>
#include<stdbool.h>
#define ROWS 10
#define COLS 10
//地图区分
enum type { road, wall };
//方向类型,上左下右
enum direct { p_up, p_left, p_down, p_right };
//定义点类型
typedef struct Mypoint
{
int row, col;
}Mypoint;
//辅助地图类
typedef struct pathMap
{
int dir; //记录当前方向
bool isFind; //是否走过 false没走过 true走过
}pathMap;
#endif
.c文件
#include"Mytypes.h"
#include"MyStack.h"
#include<windows.h>
void drawMap(int map[ROWS][COLS],Mypoint\* p)
{
Sleep(300);
system("cls");
for (int i = 0; i < ROWS; i++)
{
for (int j = 0; j < COLS; j++)
{
if (p->row == i && p->col == j)
{
printf("人");
}
else if (wall == map[i][j])
{
printf("墙");
}
else
{
printf(" ");
}
}
printf("\n");
}
}
int main()
{
//地图 1表示障碍 0表示路
int map[ROWS][COLS] = {
{ 1, 1, 1, 1, 1, 1, 1, 1, 1, 1 },
{ 1, 0, 1, 1, 0, 0, 0, 1, 1, 1 },
{ 1, 0, 1, 1, 0, 1, 0, 0, 0, 1 },
{ 1, 0, 1, 1, 0, 1, 0, 1, 0, 1 },
{ 1, 0, 1, 1, 0, 1, 0, 1, 0, 1 },
{ 1, 0, 0, 0, 0, 1, 0, 1, 0, 1 },
{ 1, 0, 1, 1, 1, 1, 0, 1, 0, 1 },
{ 1, 0, 1, 1, 1, 1, 0, 1, 0, 1 },
{ 1, 0, 1, 1, 1, 1, 0, 1, 0, 1 },
{ 1, 1, 1, 1, 1, 1, 1, 1, 1, 1 },
};
//点
Mypoint begPos = { 1,1 };
Mypoint endPos = { 8,8 };
//辅助地图,标记起点走过
pathMap PathMap[ROWS][COLS] = { 0 };
PathMap[begPos.row][begPos.row].isFind = true;
//栈 起点入栈
Stack stack;
init(&stack);
push(&stack, &begPos);
//标记没有找到终点
bool isFindEnd = false;
//当前点
Mypoint currentPos;
currentPos.row = begPos.row;
currentPos.col = begPos.col;
//预测点
Mypoint searchPos;
//寻路
while (1)
{
//找到试探点
searchPos.row = currentPos.row;
searchPos.col = currentPos.col;
//根据当前点的试探方向,确定试探点
switch (PathMap[currentPos.row][currentPos.col].dir)
{
case p_up:
searchPos.row--;
//试探方向改变
PathMap[currentPos.row][currentPos.col].dir = p_left;
//判断能不能走
if ( road == map[searchPos.row][searchPos.col] &&
PathMap[searchPos.row][searchPos.col].isFind == false )
{
//走
currentPos.row = searchPos.row;
currentPos.col = searchPos.col;
//记录走过
PathMap[currentPos.row][currentPos.col].isFind = true;
//入栈
push(&stack, ¤tPos);
}
break;
case p_left:
searchPos.col--;
//试探方向改变
PathMap[currentPos.row][currentPos.col].dir = p_down;
//判断能不能走
if (road == map[searchPos.row][searchPos.col] &&
PathMap[searchPos.row][searchPos.col].isFind == false)
{
//走
currentPos.row = searchPos.row;
currentPos.col = searchPos.col;
//记录走过
PathMap[currentPos.row][currentPos.col].isFind = true;
//入栈
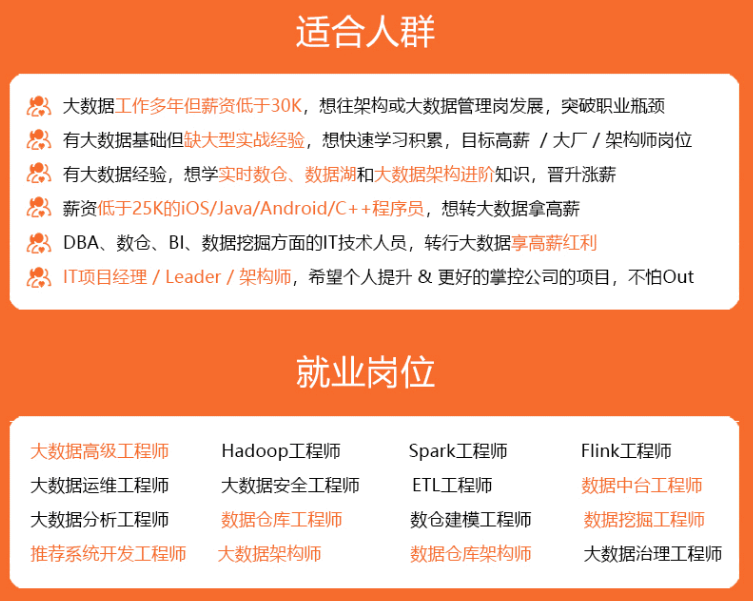
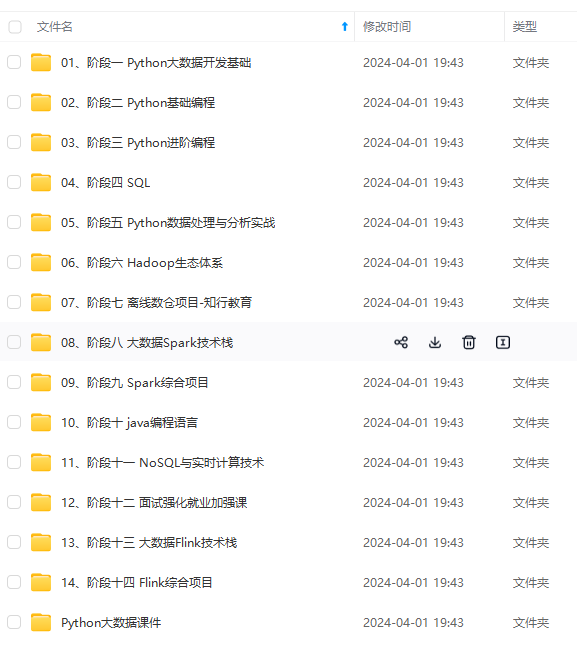
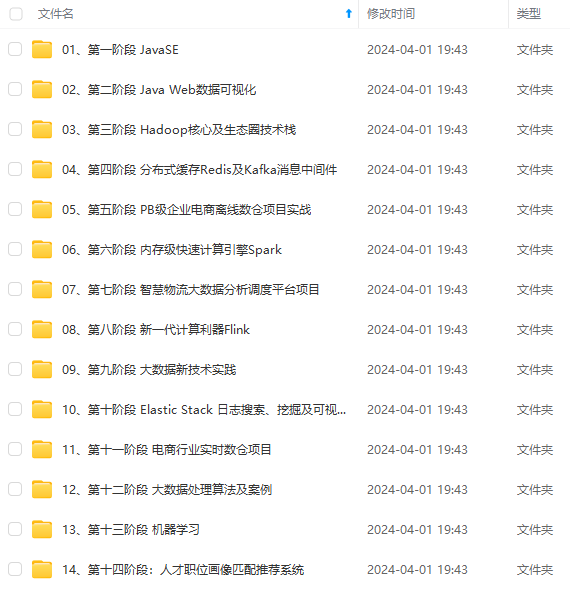
**既有适合小白学习的零基础资料,也有适合3年以上经验的小伙伴深入学习提升的进阶课程,涵盖了95%以上大数据知识点,真正体系化!**
**由于文件比较多,这里只是将部分目录截图出来,全套包含大厂面经、学习笔记、源码讲义、实战项目、大纲路线、讲解视频,并且后续会持续更新**
**[需要这份系统化资料的朋友,可以戳这里获取](https://bbs.csdn.net/topics/618545628)**
.(img-SrrhCG22-1714691305515)]
[外链图片转存中...(img-XmOFQxwS-1714691305515)]
**既有适合小白学习的零基础资料,也有适合3年以上经验的小伙伴深入学习提升的进阶课程,涵盖了95%以上大数据知识点,真正体系化!**
**由于文件比较多,这里只是将部分目录截图出来,全套包含大厂面经、学习笔记、源码讲义、实战项目、大纲路线、讲解视频,并且后续会持续更新**
**[需要这份系统化资料的朋友,可以戳这里获取](https://bbs.csdn.net/topics/618545628)**