// 截取后半部分的地址 ? 后面的部分 也是要查询的地址
req.query = querystring.parse(url.split('?')[1])
// 处理 post data
getPostData(req).then(postData => {
// 解析 POST 请求中的数据
req.body = postData
// 处理 blog 路由
const blogData = handleBlogRouter(req, res)
// 请求成功 格式化 blogData 并结束
if (blogData) {
res.end(
JSON.stringify(blogData)
)
return
}
// 处理 user 路由
const userData = handleUserRouter(req, res)
// 请求成功 格式化 userData 并结束
if (userData) {
res.end(
JSON.stringify(userData)
)
return
}
// 未命中路由,返回 404
res.writeHead(404, {
"Content-type": "text/plain"
})
// 在浏览器显示的内容
res.write("404 Not Found\n")
// 结束服务端和客户端的连接
res.end()
})
}
// 模块化 把 serverHandle 暴露出去
module.exports = serverHandle
…/src/model/resModel.js 文件
* 基础模型(对象类型和字符串类型)
* 成功的模型(继承)
* 失败的模型(继承)
// 基础模型
class BaseModel {
// data 是对象类型 message 是字符串类型
constructor(data, message) {
if (typeof data === ‘string’) {
this.message = data
data = null
message = null
}
if (data) {
this.data = data
}
if (message) {
this.message = message
}
}
}
// 成功模型 errno 赋值 0
class SuccessModel extends BaseModel {
constructor(data, message) {
// 继承父类
super(data, message)
// 成功的值 0
this.errno = 0
}
}
// 失败模型 errno 赋值 -1
class ErrorModel extends BaseModel {
constructor(data, message) {
// 继承父类
super(data, message)
// 失败的值 -1
this.errno = -1
}
}
// 导出共享
module.exports = {
SuccessModel,
ErrorModel
}
…/src/router/blog.js 文件
* 博客相关路由接口
* 调用相关函数
* 返回实例
// 导入博客和用户控制器相关内容
const { getList, getDetail, newBlog, updateBlog, delBlog } = require(‘…/controller/blog’)
// 导入成功和失败的模型
const { SuccessModel, ErrorModel } = require(‘…/model/resModel’)
// blog 路由
const handleBlogRouter = (req, res) => {
const method = req.method // GET/POST
const id = req.query.id // 获取 id
// 获取博客列表 GET 请求·
if (method === 'GET' && req.path === '/api/blog/list') {
// 博客的作者 req.query 用在 GET 请求中
const author = req.query.author || ''
// 博客的关键字
const keyword = req.query.keyword || ''
// 执行函数 获取博客列表数据
const listData = getList(author, keyword)
// 创建并返回成功模型的实例对象
return new SuccessModel(listData)
}
// 获取博客详情 GET 请求
if (method === 'GET' && req.path === '/api/blog/detail') {
// 获取博客详情数据
const data = getDetail(id)
// 创建并返回成功模型的实例对象
return new SuccessModel(data)
}
// 新建一篇博客 POST 请求
if (method === 'POST' && req.path === '/api/blog/new') {
// req.body 用于获取请求中的数据(用在 POST 请求中)
const data = newBlog(req.body)
// 创建并返回成功模型的实例对象
return new SuccessModel(data)
}
// 更新一篇博客
if (method === 'POST' && req.path === '/api/blog/update') {
// 传递两个参数 id 和 req.body
const result = updateBlog(id, req.body)
if (result) {
return new SuccessModel()
} else {
return new ErrorModel('更新博客失败')
}
}
// 删除一篇博客
if (method === 'POST' && req.path === '/api/blog/delete') {
const result = delBlog(id)
if (result) {
return new SuccessModel()
} else {
return new ErrorModel('删除博客失败')
}
}
}
// 导出
module.exports = handleBlogRouter
…/src/router/user.js 文件
* 博客相关路由接口
* 调用相关函数
* 返回实例
// 导入用户登录内容
const { loginCheck } = require(‘…/controller/user’)
// 导入成功和失败的模板
const { SuccessModel, ErrorModel } = require(‘…/model/resModel’)
// user 路由
const handleUserRouter = (req, res) => {
const method = req.method
// 登录
if (method === 'POST' && req.path === '/api/user/login') {
const { username, password } = req.body
// 传入两个参数 用户名 密码
const result = loginCheck(username, password)
if (result) {
return new SuccessModel()
}
return new ErrorModel('登录失败')
}
}
// 导出共享
module.exports = handleUserRouter
…/src/controller/blog.js 文件
* 博客的相关数据处理
* 返回的是假数据(后续会使用数据库)
// 获取博客列表(通过作者和关键字)
const getList = (author, keyword) => {
// 先返回假数据(格式是正确的)
return [{
id: 1,
title: ‘标题1’,
content: ‘内容1’,
createTime: 1665832332896,
author: ‘zahuopu’
},
{
id: 1,
title: ‘标题2’,
content: ‘内容2’,
createTime: 1665832418727,
author: ‘xiaoming’
},
]
}
// 获取博客详情(通过 id)
const getDetail = (id) => {
// 先返回假数据
return {
id: 1,
title: ‘标题1’,
content: ‘内容1’,
createTime: 1665832332896,
author: ‘zahuopu’
}
}
// 新建博客 newBlog 若没有,就给它一个空对象
const newBlog = (blogData = {}) => {
// blogData 是一个博客对象 包含 title content 属性
console.log(‘newBlog’, blogData)
return {
id: 3 // 表示新建博客,插入到数据表里的 id
}
}
// 更新博客(通过 id 更新)
const updateBlog = (id, blogData = {}) => {
// id 就是要更新博客的 id
// blogData 是一个博客对象 包含 title content 属性
console.log(‘update blog’, id, blogData)
return true
}
// 删除博客(通过 id 删除)
const delBlog = (id) => {
return true
}
// 导出共享
module.exports = {
getList,
getDetail,
newBlog,
updateBlog,
delBlog
}
…/src/controller/user.js 文件
* 用户登录的相关数据处理
* 返回的是假数据(后续会使用数据库)
// 登录(通过用户名和密码)
const loginCheck = (username, password) => {
// 先使用假数据
if (username === ‘zahuopu’ && password === ‘123’) {
return true
}
return false
}
// 导出共享
module.exports = {
loginCheck
}
### 三、各个接口的测试
现在我们 **已经完成** 博客项目的开发了,接下来进入到 **测试环节~~**
通过关键字和作者 **查询博客(GET 请求)**

通过 id 获取 **博客详情(GET 请求)**

通过 ApiPost/Postman 工具测试 **新增博客(POST 请求)**
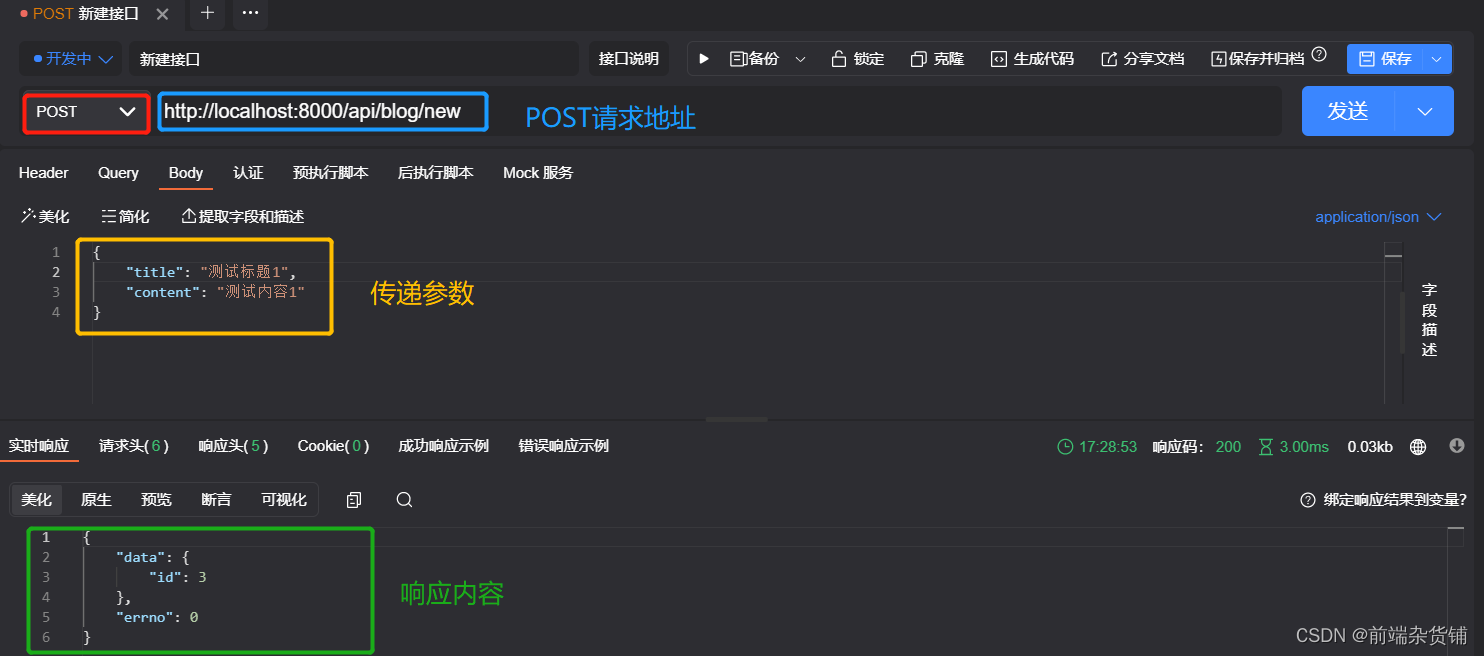
通过 ApiPost/Postman 工具测试 **更新博客(POST 请求)**
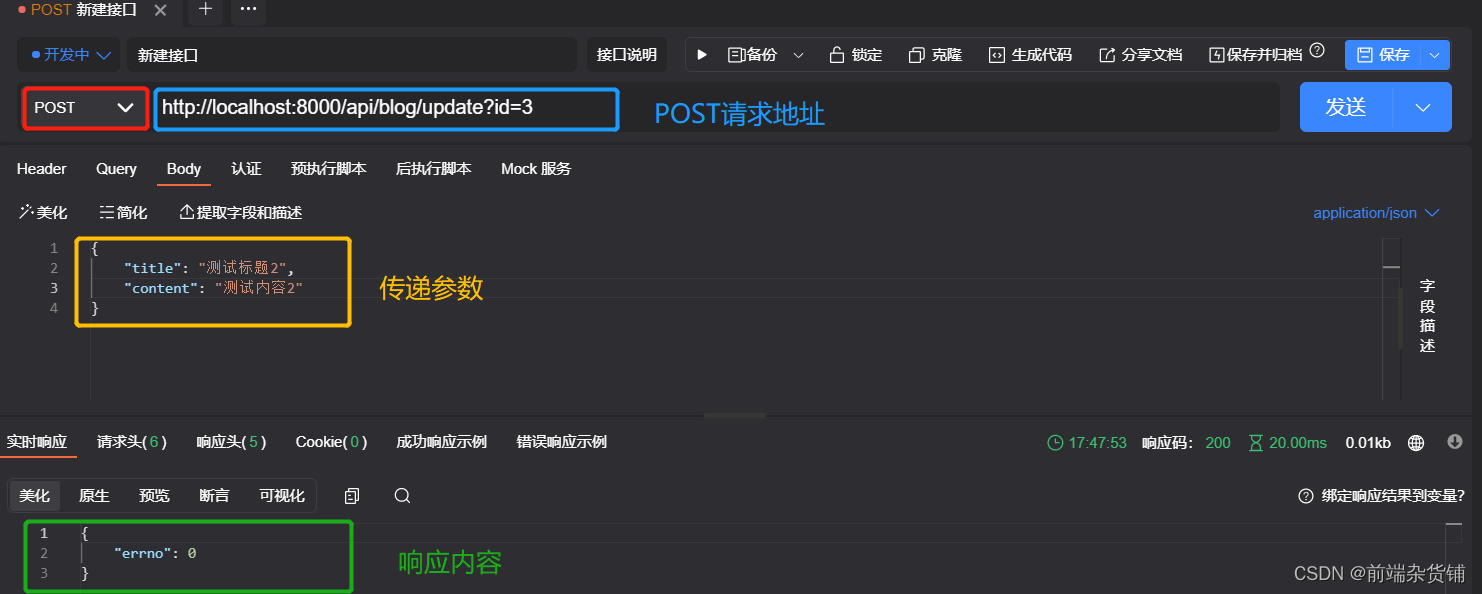

通过 ApiPost/Postman 工具测试 **删除博客(POST 请求)**
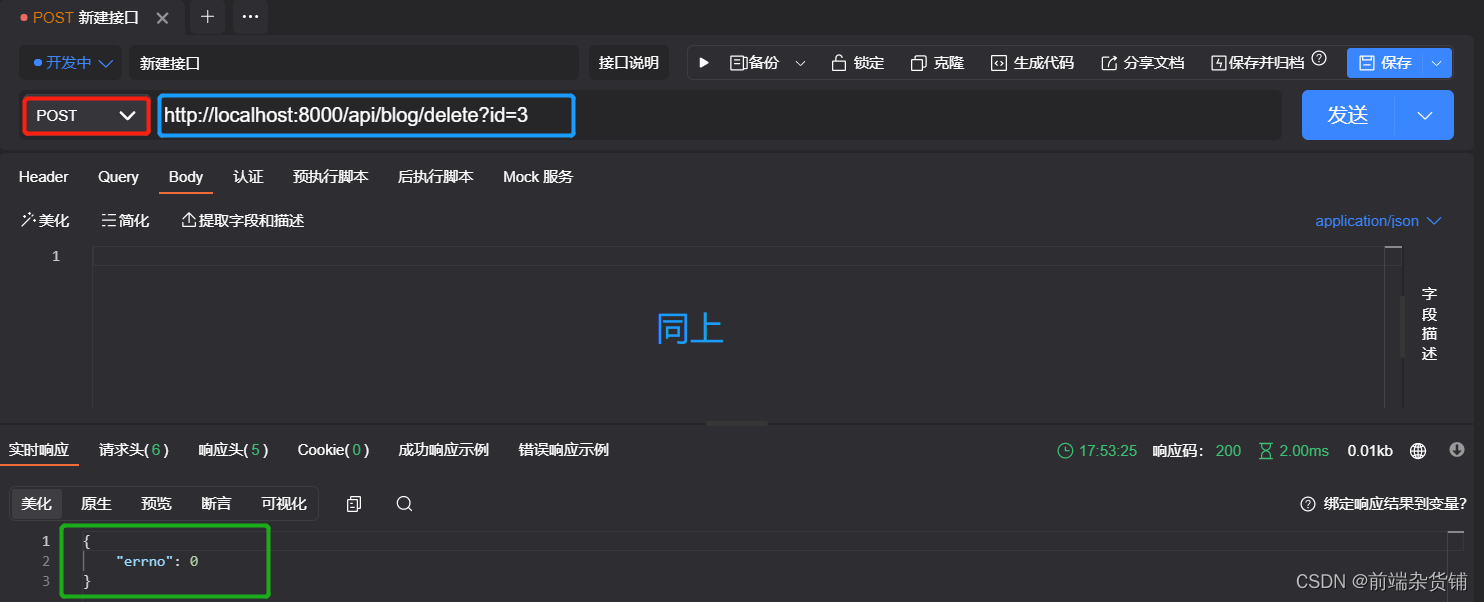
通过 ApiPost/Postman 工具测试 **登录请求(POST 请求)**
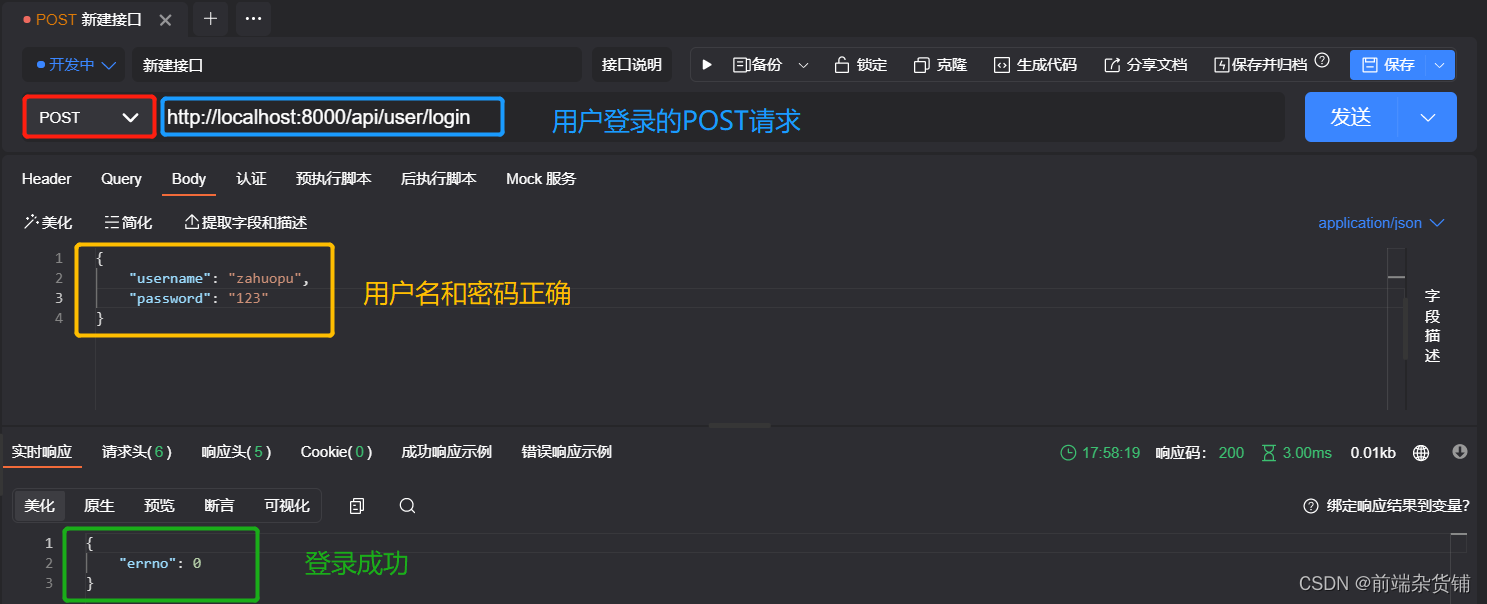
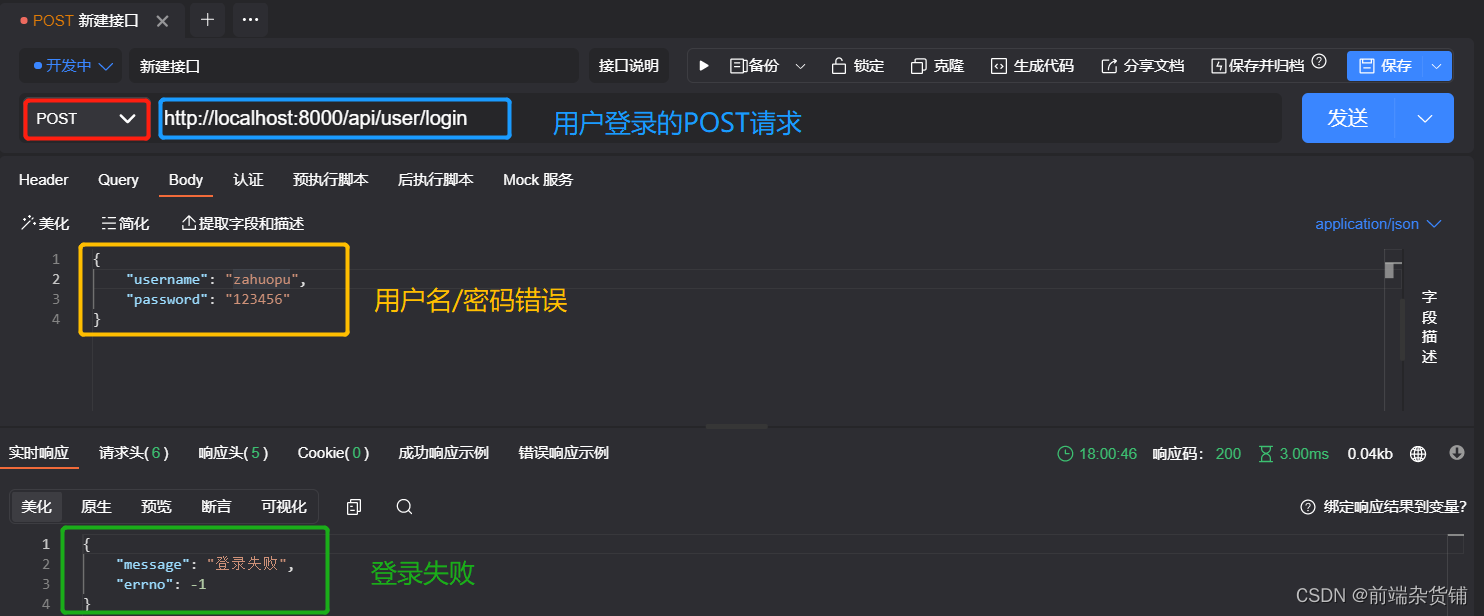
### 四、写在最后(附源码)
至此,开发博客的项目(假数据版,并没有使用数据库)就完成了。
**后续会对该项目进行多次重构**【多种框架(express,koa)和数据库(mysql,sequelize,mongodb)】
**如果你需要该项目的 源码,请通过本篇文章最下面的方式 加入 进来~~**
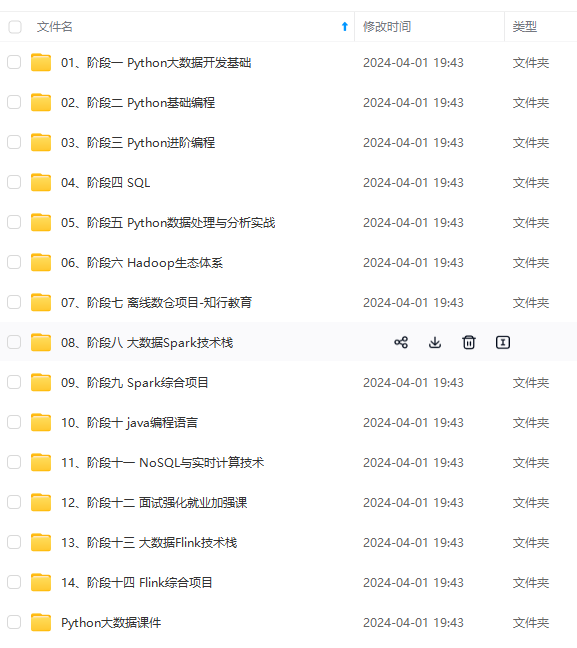
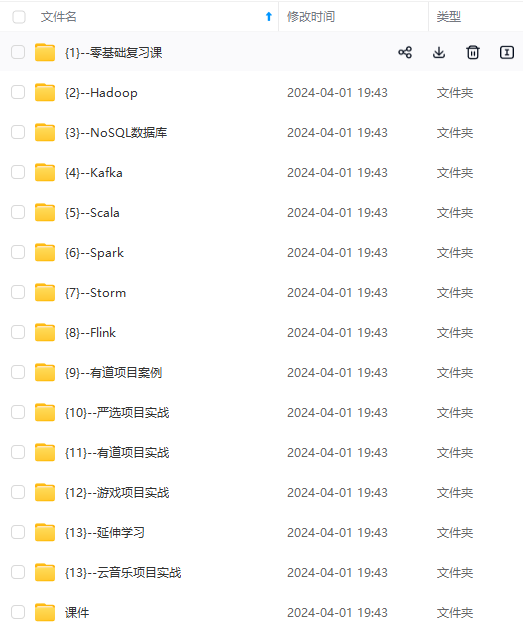
**网上学习资料一大堆,但如果学到的知识不成体系,遇到问题时只是浅尝辄止,不再深入研究,那么很难做到真正的技术提升。**
**[需要这份系统化资料的朋友,可以戳这里获取](https://bbs.csdn.net/topics/618545628)**
**一个人可以走的很快,但一群人才能走的更远!不论你是正从事IT行业的老鸟或是对IT行业感兴趣的新人,都欢迎加入我们的的圈子(技术交流、学习资源、职场吐槽、大厂内推、面试辅导),让我们一起学习成长!**
】
**如果你需要该项目的 源码,请通过本篇文章最下面的方式 加入 进来~~**
[外链图片转存中...(img-i7wT4CYC-1714645347755)]
[外链图片转存中...(img-FxARLwjD-1714645347756)]
**网上学习资料一大堆,但如果学到的知识不成体系,遇到问题时只是浅尝辄止,不再深入研究,那么很难做到真正的技术提升。**
**[需要这份系统化资料的朋友,可以戳这里获取](https://bbs.csdn.net/topics/618545628)**
**一个人可以走的很快,但一群人才能走的更远!不论你是正从事IT行业的老鸟或是对IT行业感兴趣的新人,都欢迎加入我们的的圈子(技术交流、学习资源、职场吐槽、大厂内推、面试辅导),让我们一起学习成长!**