static {
int scale = unsafe.arrayIndexScale(int[].class);
if ((scale & (scale - 1)) != 0)
throw new Error(“data type scale not a power of two”);
shift = 31 - Integer.numberOfLeadingZeros(scale);
}
private long checkedByteOffset(int i) {
if (i < 0 || i >= array.length)
throw new IndexOutOfBoundsException("index " + i);
return byteOffset(i);
}
private static long byteOffset(int i) {
return ((long) i << shift) + base;
}
用于定位元素偏移量的控制值。
举例说明,int scale = 4;1个int类型,在java中占用4个字节。
Integer.numberOfLeadingZeros(scale); 返回 scale 高位连续0的个数,得出shift = 2, 而shift在如下方法使用
得出结论了吧,shift就是 用来定位数组中的内存位置,用来移位用的,每向左移动移位,在不越界的情况下,想当于乘以2。也就是int类型的长度为4,也就是第0个位置是0,第1(i)个位置是4,,第二个(i)位置是8,也就是偏移位置等于 i * 4,也就是 i << 2;总结出一个乘法转换成移位操作的案例: a * (一个2的幂(n)的数) = a << n; 给出一个指定2的幂的数,怎么算成n,参照shift的计算方法。
附上AtomicIntegerArray 源码
/*
-
ORACLE PROPRIETARY/CONFIDENTIAL. Use is subject to license terms.
*/
/*
-
Written by Doug Lea with assistance from members of JCP JSR-166
-
Expert Group and released to the public domain, as explained at
-
http://creativecommons.org/publicdomain/zero/1.0/
*/
package java.util.concurrent.atomic;
import sun.misc.Unsafe;
import java.util.*;
/**
-
An {@code int} array in which elements may be updated atomically.
-
See the {@link java.util.concurrent.atomic} package
-
specification for description of the properties of atomic
-
variables.
-
@since 1.5
-
@author Doug Lea
*/
public class AtomicIntegerArray implements java.io.Serializable {
private static final long serialVersionUID = 2862133569453604235L;
private static final Unsafe unsafe = Unsafe.getUnsafe();
private static final int base = unsafe.arrayBaseOffset(int[].class);
private static final int shift;
private final int[] array;
static {
int scale = unsafe.arrayIndexScale(int[].class);
if ((scale & (scale - 1)) != 0)
throw new Error(“data type scale not a power of two”);
shift = 31 - Integer.numberOfLeadingZeros(scale);
}
private long checkedByteOffset(int i) {
if (i < 0 || i >= array.length)
throw new IndexOutOfBoundsException("index " + i);
return byteOffset(i);
}
private static long byteOffset(int i) {
return ((long) i << shift) + base;
}
/**
-
Creates a new AtomicIntegerArray of the given length, with all
-
elements initially zero.
-
@param length the length of the array
*/
public AtomicIntegerArray(int length) {
array = new int[length];
}
/**
-
Creates a new AtomicIntegerArray with the same length as, and
-
all elements copied from, the given array.
-
@param array the array to copy elements from
-
@throws NullPointerException if array is null
*/
public AtomicIntegerArray(int[] array) {
// Visibility guaranteed by final field guarantees
this.array = array.clone();
}
/**
-
Returns the length of the array.
-
@return the length of the array
*/
public final int length() {
return array.length;
}
/**
-
Gets the current value at position {@code i}.
-
@param i the index
-
@return the current value
*/
public final int get(int i) {
return getRaw(checkedByteOffset(i));
}
private int getRaw(long offset) {
return unsafe.getIntVolatile(array, offset);
}
/**
-
Sets the element at position {@code i} to the given value.
-
@param i the index
-
@param newValue the new value
*/
public final void set(int i, int newValue) {
unsafe.putIntVolatile(array, checkedByteOffset(i), newValue);
}
/**
-
Eventually sets the element at position {@code i} to the given value.
-
@param i the index
-
@param newValue the new value
-
@since 1.6
*/
public final void lazySet(int i, int newValue) {
unsafe.putOrderedInt(array, checkedByteOffset(i), newValue);
}
/**
-
Atomically sets the element at position {@code i} to the given
-
value and returns the old value.
-
@param i the index
-
@param newValue the new value
-
@return the previous value
*/
public final int getAndSet(int i, int newValue) {
long offset = checkedByteOffset(i);
while (true) {
int current = getRaw(offset);
if (compareAndSetRaw(offset, current, newValue))
return current;
}
}
/**
-
Atomically sets the element at position {@code i} to the given
-
updated value if the current value {@code ==} the expected value.
-
@param i the index
-
@param expect the expected value
-
@param update the new value
-
@return true if successful. False return indicates that
-
the actual value was not equal to the expected value.
*/
public final boolean compareAndSet(int i, int expect, int update) {
return compareAndSetRaw(checkedByteOffset(i), expect, update);
}
private boolean compareAndSetRaw(long offset, int expect, int update) {
return unsafe.compareAndSwapInt(array, offset, expect, update);
}
/**
-
Atomically sets the element at position {@code i} to the given
-
updated value if the current value {@code ==} the expected value.
-
May fail spuriously
-
and does not provide ordering guarantees, so is only rarely an
-
appropriate alternative to {@code compareAndSet}.
-
@param i the index
-
@param expect the expected value
-
@param update the new value
-
@return true if successful.
*/
public final boolean weakCompareAndSet(int i, int expect, int update) {
return compareAndSet(i, expect, update);
}
/**
-
Atomically increments by one the element at index {@code i}.
-
@param i the index
-
@return the previous value
*/
public final int getAndIncrement(int i) {
return getAndAdd(i, 1);
}
/**
-
Atomically decrements by one the element at index {@code i}.
-
@param i the index
-
@return the previous value
*/
public final int getAndDecrement(int i) {
return getAndAdd(i, -1);
}
/**
-
Atomically adds the given value to the element at index {@code i}.
-
@param i the index
自我介绍一下,小编13年上海交大毕业,曾经在小公司待过,也去过华为、OPPO等大厂,18年进入阿里一直到现在。
深知大多数Java工程师,想要提升技能,往往是自己摸索成长或者是报班学习,但对于培训机构动则几千的学费,着实压力不小。自己不成体系的自学效果低效又漫长,而且极易碰到天花板技术停滞不前!
因此收集整理了一份《2024年Java开发全套学习资料》,初衷也很简单,就是希望能够帮助到想自学提升又不知道该从何学起的朋友,同时减轻大家的负担。
既有适合小白学习的零基础资料,也有适合3年以上经验的小伙伴深入学习提升的进阶课程,基本涵盖了95%以上Java开发知识点,真正体系化!
由于文件比较大,这里只是将部分目录截图出来,每个节点里面都包含大厂面经、学习笔记、源码讲义、实战项目、讲解视频,并且会持续更新!
如果你觉得这些内容对你有帮助,可以扫码获取!!(备注Java获取)
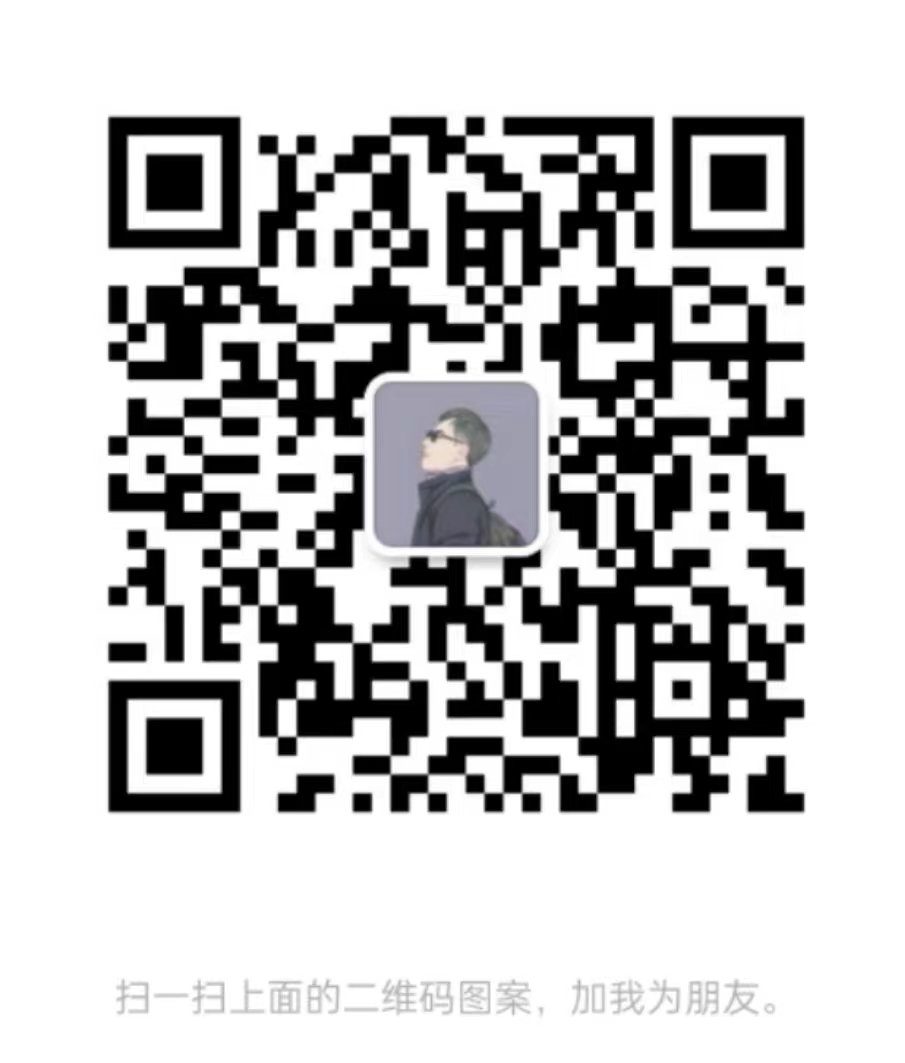
最后
现在正是金三银四的春招高潮,前阵子小编一直在搭建自己的网站,并整理了全套的**【一线互联网大厂Java核心面试题库+解析】:包括Java基础、异常、集合、并发编程、JVM、Spring全家桶、MyBatis、Redis、数据库、中间件MQ、Dubbo、Linux、Tomcat、ZooKeeper、Netty等等**
《互联网大厂面试真题解析、进阶开发核心学习笔记、全套讲解视频、实战项目源码讲义》点击传送门即可获取!
扫码获取!!(备注Java获取)**
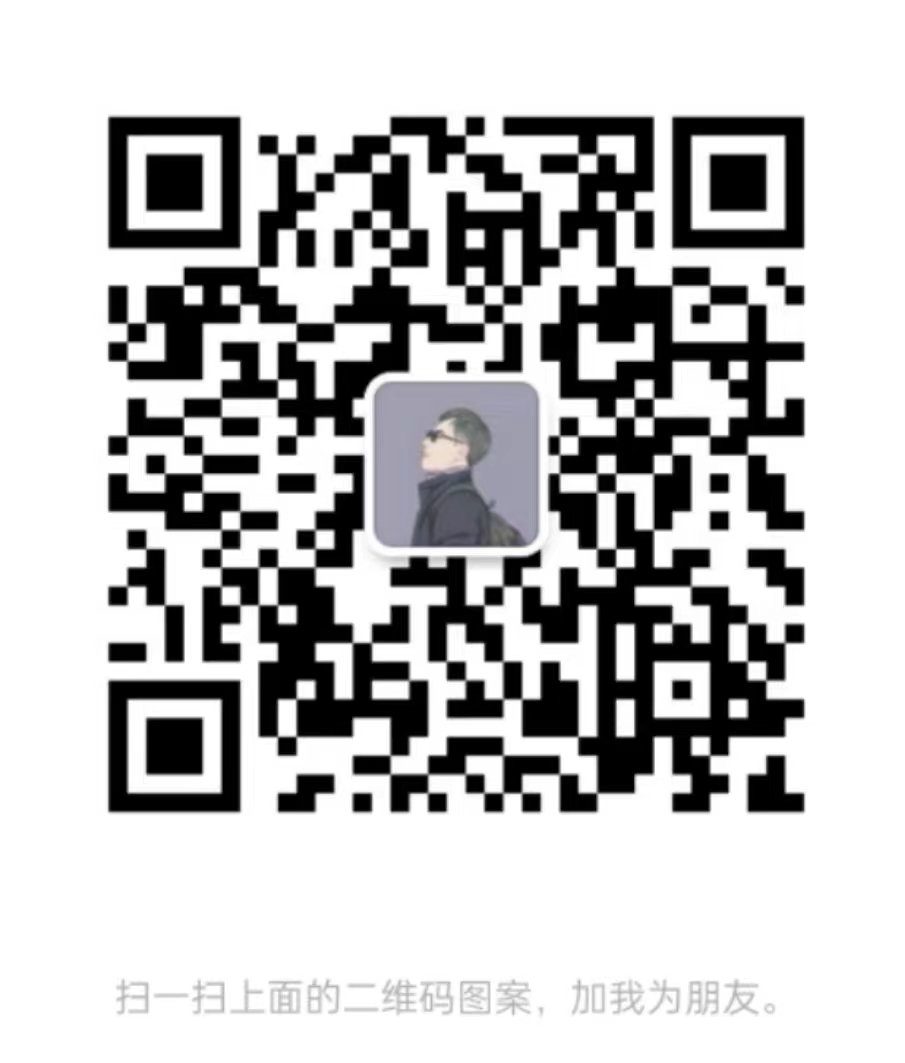
最后
现在正是金三银四的春招高潮,前阵子小编一直在搭建自己的网站,并整理了全套的**【一线互联网大厂Java核心面试题库+解析】:包括Java基础、异常、集合、并发编程、JVM、Spring全家桶、MyBatis、Redis、数据库、中间件MQ、Dubbo、Linux、Tomcat、ZooKeeper、Netty等等**
[外链图片转存中…(img-Z1ydQ9Ld-1713308076152)]
《互联网大厂面试真题解析、进阶开发核心学习笔记、全套讲解视频、实战项目源码讲义》点击传送门即可获取!