网上学习资料一大堆,但如果学到的知识不成体系,遇到问题时只是浅尝辄止,不再深入研究,那么很难做到真正的技术提升。
一个人可以走的很快,但一群人才能走的更远!不论你是正从事IT行业的老鸟或是对IT行业感兴趣的新人,都欢迎加入我们的的圈子(技术交流、学习资源、职场吐槽、大厂内推、面试辅导),让我们一起学习成长!
#NONE 值是禁用发布确认模式,是默认值
#CORRELATED 值是发布消息成功到交换器后会触发回调方法
#SIMPLE 值经测试有两种效果,其一效果和 CORRELATED 值一样会触发回调方法,
# 其二在发布消息成功后使用 rabbitTemplate 调用 waitForConfirms 或 waitForConfirmsOrDie 方法等待 broker 节点返回发送结果,
# 根据返回结果来判定下一步的逻辑,要注意的点是 waitForConfirmsOrDie 方法如果返回 false 则会关闭 channel,
# 则接下来无法发送消息到 broker;
spring.rabbitmq.publisher-confirm-type=correlated
- NONE 值是禁用发布确认模式,是默认值
- CORRELATED 值是发布消息成功到交换器后会触发回调方法
- SIMPLE 值经测试有两种效果,其一效果和 CORRELATED 值一样会触发回调方法,其二在发布消息成功后使用rabbitTemplate 调用waitForConfirms 或 waitForConfirmsOrDie 方法等待 broker节点返回发送结果,根据返回结果来判定下一步的逻辑,要注意的点是waitForConfirmsOrDie 方法如果返回 false则会关闭 channel,则接下来无法发送消息到 broker;
3. 配置类
package com.hong.springboot.rabbitmq.config;
import org.springframework.amqp.core.\*;
import org.springframework.beans.factory.annotation.Qualifier;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
/\*\*
\* @Description: 发布确认高级版配置类
\* @Author: hong
\* @Date: 2024-03-05 20:52
\* @Version: 1.0
\*\*/
@Configuration
public class ConfirmConfig {
public static final String CONFIRM\_EXCHANGE\_NAME = "confirm.exchange";
public static final String CONFIRM\_QUEUE\_NAME = "confirm.queue";
public static final String CONFIRM\_ROUTING\_KEY = "key1";
//声明业务 Exchange
@Bean("confirmExchange")
public DirectExchange confirmExchange() {
return new DirectExchange(CONFIRM\_EXCHANGE\_NAME);
}
// 声明确认队列
@Bean("confirmQueue")
public Queue confirmQueue() {
return QueueBuilder.durable(CONFIRM\_QUEUE\_NAME).build();
}
// 声明确认队列绑定关系
@Bean
public Binding queueBinding(@Qualifier("confirmQueue") Queue queue,
@Qualifier("confirmExchange") DirectExchange exchange) {
return BindingBuilder.bind(queue).to(exchange).with(CONFIRM\_ROUTING\_KEY);
}
}
4.生产者
package com.hong.springboot.rabbitmq.controller;
import com.hong.springboot.rabbitmq.config.ConfirmConfig;
import lombok.extern.slf4j.Slf4j;
import org.springframework.amqp.rabbit.core.RabbitTemplate;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import java.text.SimpleDateFormat;
import java.util.Date;
/\*\*
\* @Description: 发布确认高级版生产者
\* @Author: hong
\* @Date: 2024-03-05 20:58
\* @Version: 1.0
\*\*/
@Slf4j
@RequestMapping("/confirm/")
@RestController
public class ConfirmProducerController {
@Autowired
private RabbitTemplate rabbitTemplate;
//http://localhost:8080/confirm/sendMsg/Hi,JAVA小生不才
@GetMapping("sendMsg/{message}")
public void sendMsg(@PathVariable String message) {
log.info("当前时间:{},发送信息给队列:{}",new SimpleDateFormat("yyyy-MM-dd HH:mm:ss").format(new Date()) , message);
rabbitTemplate.convertAndSend(ConfirmConfig.CONFIRM\_EXCHANGE\_NAME, ConfirmConfig.CONFIRM\_ROUTING\_KEY, message);
}
}
5.消费者
package com.hong.springboot.rabbitmq.consumer;
import com.hong.springboot.rabbitmq.config.ConfirmConfig;
import com.hong.springboot.rabbitmq.config.DelayedQueueConfig;
import lombok.extern.slf4j.Slf4j;
import org.springframework.amqp.core.Message;
import org.springframework.amqp.rabbit.annotation.RabbitListener;
import org.springframework.stereotype.Component;
import java.text.SimpleDateFormat;
import java.util.Date;
/\*\*
\* @Description: 发布确认高级版消费者
\* @Author: hong
\* @Date: 2024-03-05 21:05
\* @Version: 1.0
\*\*/
@Slf4j
@Component
public class ConfirmConsumer {
@RabbitListener(queues = ConfirmConfig.CONFIRM\_QUEUE\_NAME)
public void receiveConfirmMessage(Message message){
String msg = new String(message.getBody());
log.info("当前时间:{},收到信息{}",new SimpleDateFormat("yyyy-MM-dd HH:mm:ss").format(new Date()) , msg);
}
}
正常情况下,发送http://localhost:8080/confirm/sendMsg/Hi,JAVA小生不才
6.回调接口
package com.hong.springboot.rabbitmq.config;
import lombok.extern.slf4j.Slf4j;
import org.springframework.amqp.rabbit.connection.CorrelationData;
import org.springframework.amqp.rabbit.core.RabbitTemplate;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Component;
import javax.annotation.PostConstruct;
/\*\*
\* @Description: 发布确认高级版消息生产者的回调接口
\* @Author: hong
\* @Date: 2024-03-09 21:58
\* @Version: 1.0
\*\*/
@Slf4j
@Component
public class MyCallBack implements RabbitTemplate.ConfirmCallback{
@Autowired
private RabbitTemplate rabbitTemplate;
@PostConstruct
public void init(){
rabbitTemplate.setConfirmCallback(this);
}
/\*\*
\* 交换机不管是否收到消息的一个回调方法
\* 1.收到消息
\* correlationData 保存回调消息的id及相关信息
\* b true 交换机收到消息
\* s null
\* 2.未收到消息
\* correlationData 保存回调消息的id及相关信息
\* b false 交换机未收到消息
\* s 失败的原因
\* @param correlationData 消息相关数据
\* @param b 交换机是否收到消息
\* @param s 没收到消息的原因
\*/
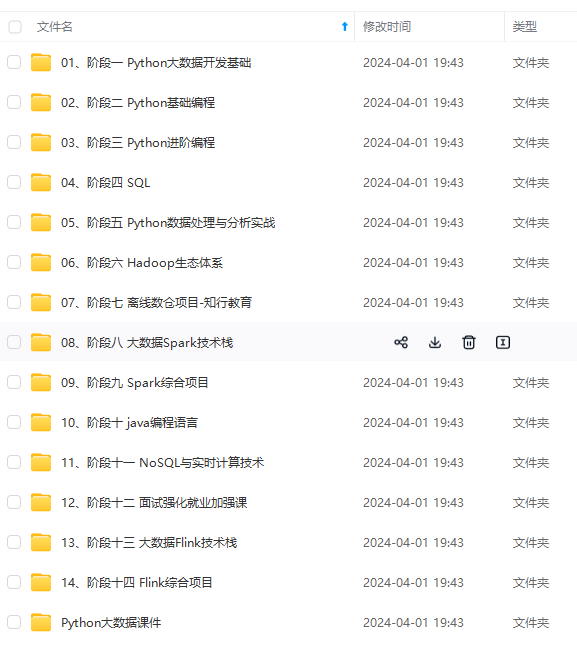
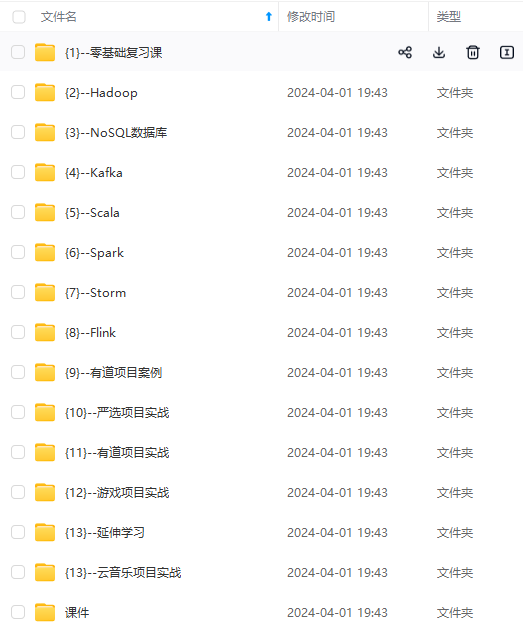
**网上学习资料一大堆,但如果学到的知识不成体系,遇到问题时只是浅尝辄止,不再深入研究,那么很难做到真正的技术提升。**
**[需要这份系统化资料的朋友,可以戳这里获取](https://bbs.csdn.net/topics/618545628)**
**一个人可以走的很快,但一群人才能走的更远!不论你是正从事IT行业的老鸟或是对IT行业感兴趣的新人,都欢迎加入我们的的圈子(技术交流、学习资源、职场吐槽、大厂内推、面试辅导),让我们一起学习成长!**
到问题时只是浅尝辄止,不再深入研究,那么很难做到真正的技术提升。**
**[需要这份系统化资料的朋友,可以戳这里获取](https://bbs.csdn.net/topics/618545628)**
**一个人可以走的很快,但一群人才能走的更远!不论你是正从事IT行业的老鸟或是对IT行业感兴趣的新人,都欢迎加入我们的的圈子(技术交流、学习资源、职场吐槽、大厂内推、面试辅导),让我们一起学习成长!**