既有适合小白学习的零基础资料,也有适合3年以上经验的小伙伴深入学习提升的进阶课程,涵盖了95%以上Go语言开发知识点,真正体系化!
由于文件比较多,这里只是将部分目录截图出来,全套包含大厂面经、学习笔记、源码讲义、实战项目、大纲路线、讲解视频,并且后续会持续更新
struct TreeNode\* node2 = que2[queleft2++];
if (node1->val != node2->val) {
return false;
}
struct TreeNode\* left1 = node1->left;
struct TreeNode\* right1 = node1->right;
struct TreeNode\* left2 = node2->left;
struct TreeNode\* right2 = node2->right;
if ((left1 == NULL) ^ (left2 == NULL)) {
return false;
}
if ((right1 == NULL) ^ (right2 == NULL)) {
return false;
}
if (left1 != NULL) {
queright1++;
que1 = realloc(que1, sizeof(struct TreeNode\*) \* queright1);
que1[queright1 - 1] = left1;
}
if (right1 != NULL) {
queright1++;
que1 = realloc(que1, sizeof(struct TreeNode\*) \* queright1);
que1[queright1 - 1] = right1;
}
if (left2 != NULL) {
queright2++;
que2 = realloc(que2, sizeof(struct TreeNode\*) \* queright2);
que2[queright2 - 1] = left2;
}
if (right2 != NULL) {
queright2++;
que2 = realloc(que2, sizeof(struct TreeNode\*) \* queright2);
que2[queright2 - 1] = right2;
}
}
return queleft1 == queright1 && queleft2 == queright2;
}
class Solution {
public:
bool isSameTree(TreeNode* p, TreeNode* q) {
if (p == nullptr && q == nullptr) {
return true;
} else if (p == nullptr || q == nullptr) {
return false;
}
queue <TreeNode*> queue1, queue2;
queue1.push§;
queue2.push(q);
while (!queue1.empty() && !queue2.empty()) {
auto node1 = queue1.front();
queue1.pop();
auto node2 = queue2.front();
queue2.pop();
if (node1->val != node2->val) {
return false;
}
auto left1 = node1->left, right1 = node1->right, left2 = node2->left, right2 = node2->right;
if ((left1 == nullptr) ^ (left2 == nullptr)) {
return false;
}
if ((right1 == nullptr) ^ (right2 == nullptr)) {
return false;
}
if (left1 != nullptr) {
queue1.push(left1);
}
if (right1 != nullptr) {
queue1.push(right1);
}
if (left2 != nullptr) {
queue2.push(left2);
}
if (right2 != nullptr) {
queue2.push(right2);
}
}
return queue1.empty() && queue2.empty();
}
};
### [226. 翻转二叉树]( )
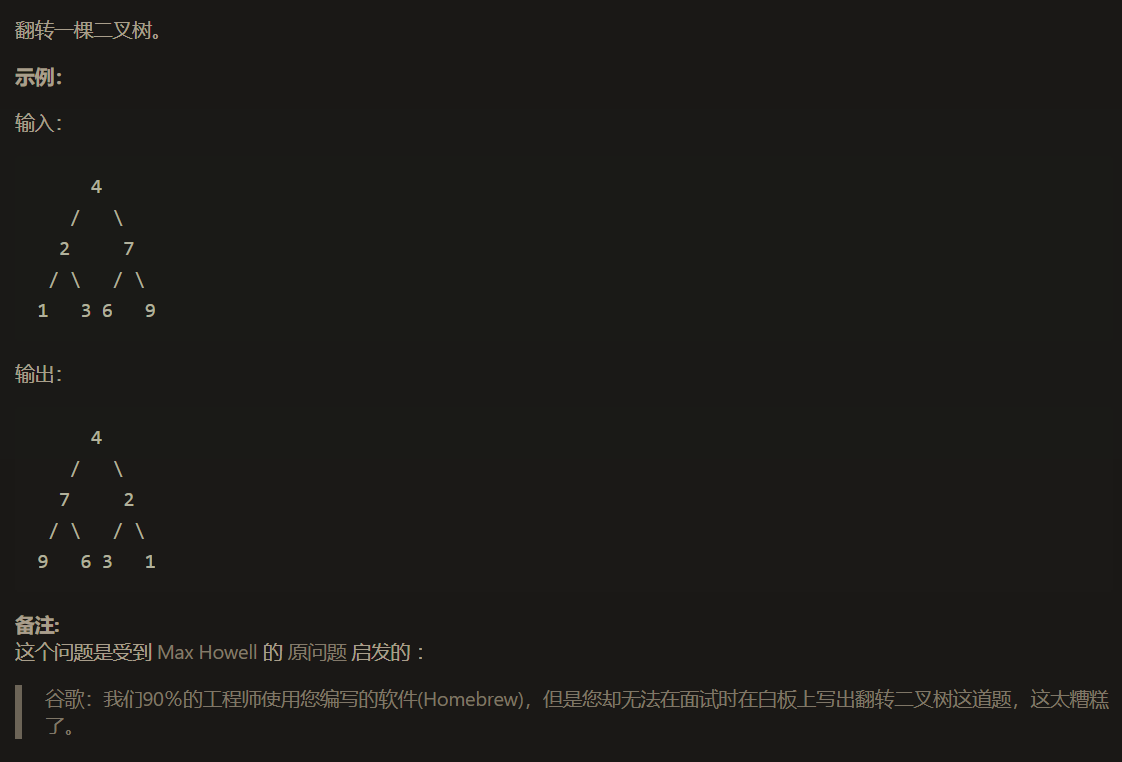
[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-eHkkuHEY-1631410165745)(https://raw.githubusercontent.com/xkyvvv/blogpic/main/pic1/image-20210906233211449.png)]
struct TreeNode* invertTree(struct TreeNode* root) {
if (root == NULL) {
return NULL;
}
struct TreeNode* left = invertTree(root->left);
struct TreeNode* right = invertTree(root->right);
root->left = right;
root->right = left;
return root;
}
class Solution {
public:
TreeNode* invertTree(TreeNode* root) {
if (root == nullptr) {
return nullptr;
}
TreeNode* left = invertTree(root->left);
TreeNode* right = invertTree(root->right);
root->left = right;
root->right = left;
return root;
}
};
### [144. 二叉树的前序遍历]( )
[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-IVY2Xsww-1631410165745)(https://raw.githubusercontent.com/xkyvvv/blogpic/main/pic1/image-20210906233405175.png)]
[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-VqSyQqMO-1631410165746)(https://raw.githubusercontent.com/xkyvvv/blogpic/main/pic1/image-20210906233442557.png)]
void preorder(struct TreeNode* root, int* res, int* resSize) {
if (root == NULL) {
return;
}
res[(*resSize)++] = root->val;
preorder(root->left, res, resSize);
preorder(root->right, res, resSize);
}
int* preorderTraversal(struct TreeNode* root, int* returnSize) {
int* res = malloc(sizeof(int) * 2000);
*returnSize = 0;
preorder(root, res, returnSize);
return res;
}
class Solution {
public:
void preorder(TreeNode *root, vector &res) {
if (root == nullptr) {
return;
}
res.push_back(root->val);
preorder(root->left, res);
preorder(root->right, res);
}
vector<int> preorderTraversal(TreeNode \*root) {
vector<int> res;
preorder(root, res);
return res;
}
};
[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-4Ui2W7OE-1631410165746)(https://raw.githubusercontent.com/xkyvvv/blogpic/main/pic1/image-20210906233539153.png)]
int* preorderTraversal(struct TreeNode* root, int* returnSize) {
int* res = malloc(sizeof(int) * 2000);
*returnSize = 0;
if (root == NULL) {
return res;
}
struct TreeNode\* stk[2000];
struct TreeNode\* node = root;
int stk_top = 0;
while (stk_top > 0 || node != NULL) {
while (node != NULL) {
res[(\*returnSize)++] = node->val;
stk[stk_top++] = node;
node = node->left;
}
node = stk[--stk_top];
node = node->right;
}
return res;
}
class Solution {
public:
vector preorderTraversal(TreeNode* root) {
vector res;
if (root == nullptr) {
return res;
}
stack<TreeNode\*> stk;
TreeNode\* node = root;
while (!stk.empty() || node != nullptr) {
while (node != nullptr) {
res.emplace\_back(node->val);
stk.emplace(node);
node = node->left;
}
node = stk.top();
stk.pop();
node = node->right;
}
return res;
}
};
### [111. 二叉树的最小深度]( )
[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-ccT0q5XO-1631410165747)(https://raw.githubusercontent.com/xkyvvv/blogpic/main/pic1/image-20210906233645598.png)]
[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-x0EskIlp-1631410165747)(https://raw.githubusercontent.com/xkyvvv/blogpic/main/pic1/image-20210906233711706.png)]
int minDepth(struct TreeNode *root) {
if (root == NULL) {
return 0;
}
if (root->left == NULL && root->right == NULL) {
return 1;
}
int min_depth = INT_MAX;
if (root->left != NULL) {
min_depth = fmin(minDepth(root->left), min_depth);
}
if (root->right != NULL) {
min_depth = fmin(minDepth(root->right), min_depth);
}
return min_depth + 1;
}
class Solution {
public:
int minDepth(TreeNode *root) {
if (root == nullptr) {
return 0;
}
if (root->left == nullptr && root->right == nullptr) {
return 1;
}
int min_depth = INT_MAX;
if (root->left != nullptr) {
min_depth = min(minDepth(root->left), min_depth);
}
if (root->right != nullptr) {
min_depth = min(minDepth(root->right), min_depth);
}
return min_depth + 1;
}
};
[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-ydP328lE-1631410165748)(https://raw.githubusercontent.com/xkyvvv/blogpic/main/pic1/image-20210906233802403.png)]
typedef struct {
int val;
struct TreeNode *node;
struct queNode *next;
} queNode;
void init(queNode **p, int val, struct TreeNode *node) {
(*p) = (queNode *)malloc(sizeof(queNode));
(*p)->val = val;
(*p)->node = node;
(*p)->next = NULL;
}
int minDepth(struct TreeNode *root) {
if (root == NULL) {
return 0;
}
queNode \*queLeft, \*queRight;
init(&queLeft, 1, root);
queRight = queLeft;
while (queLeft != NULL) {
struct TreeNode \*node = queLeft->node;
int depth = queLeft->val;
if (node->left == NULL && node->right == NULL) {
return depth;
}
if (node->left != NULL) {
init(&queRight->next, depth + 1, node->left);
queRight = queRight->next;
}
if (node->right != NULL) {
init(&queRight->next, depth + 1, node->right);
queRight = queRight->next;
}
queLeft = queLeft->next;
}
return false;
}
class Solution {
public:
int minDepth(TreeNode *root) {
if (root == nullptr) {
return 0;
}
queue<pair<TreeNode \*, int> > que;
que.emplace(root, 1);
while (!que.empty()) {
TreeNode \*node = que.front().first;
int depth = que.front().second;
que.pop();
if (node->left == nullptr && node->right == nullptr) {
return depth;
}
if (node->left != nullptr) {
que.emplace(node->left, depth + 1);
}
if (node->right != nullptr) {
que.emplace(node->right, depth + 1);
}
}
return 0;
}
};
### [112. 路径总和]( )
[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-00hgieFP-1631410165748)(https://raw.githubusercontent.com/xkyvvv/blogpic/main/pic1/image-20210906233938807.png)]
[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-H8hDaV3s-1631410165748)(https://raw.githubusercontent.com/xkyvvv/blogpic/main/pic1/image-20210906234012608.png)]
typedef struct queNode {
int val;
struct TreeNode *node;
struct queNode *next;
} queNode;
void init(struct queNode **p, int val, struct TreeNode *node) {
(*p) = (struct queNode *)malloc(sizeof(struct queNode));
(*p)->val = val;
(*p)->node = node;
(*p)->next = NULL;
}
bool hasPathSum(struct TreeNode *root, int sum) {
if (root == NULL) {
return false;
}
struct queNode *queLeft, *queRight;
init(&queLeft, root->val, root);
queRight = queLeft;
while (queLeft != NULL) {
struct TreeNode *now = queLeft->node;
int temp = queLeft->val;
if (now->left == NULL && now->right == NULL) {
if (temp == sum) return true;
}
if (now->left != NULL) {
init(&queRight->next, now->left->val + temp, now->left);
queRight = queRight->next;
}
if (now->right != NULL) {
init(&queRight->next, now->right->val + temp, now->right);
queRight = queRight->next;
}
queLeft = queLeft->next;
}
return false;
}
class Solution {
public:
bool hasPathSum(TreeNode *root, int sum) {
if (root == nullptr) {
return false;
}
queue<TreeNode *> que_node;
queue que_val;
que_node.push(root);
que_val.push(root->val);
while (!que_node.empty()) {
TreeNode *now = que_node.front();
int temp = que_val.front();
que_node.pop();
que_val.pop();
if (now->left == nullptr && now->right == nullptr) {
if (temp == sum) {
return true;
}
continue;
}
if (now->left != nullptr) {
que_node.push(now->left);
que_val.push(now->left->val + temp);
}
if (now->right != nullptr) {
que_node.push(now->right);
que_val.push(now->right->val + temp);
}
}
return false;
}
};
### [145. 二叉树的后序遍历]( )
[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-SEiyjvXK-1631410165749)(https://raw.githubusercontent.com/xkyvvv/blogpic/main/pic1/image-20210906234156320.png)]
[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-EAUHEPx9-1631410165749)(https://raw.githubusercontent.com/xkyvvv/blogpic/main/pic1/image-20210906234226463.png)]
void postorder(struct TreeNode *root, int *res, int *resSize) {
if (root == NULL) {
return;
}
postorder(root->left, res, resSize);
postorder(root->right, res, resSize);
res[(*resSize)++] = root->val;
}
int *postorderTraversal(struct TreeNode *root, int *returnSize) {
int *res = malloc(sizeof(int) * 2001);
*returnSize = 0;
postorder(root, res, returnSize);
return res;
}
class Solution {
public:
void postorder(TreeNode *root, vector &res) {
if (root == nullptr) {
return;
}
postorder(root->left, res);
postorder(root->right, res);
res.push_back(root->val);
}
vector<int> postorderTraversal(TreeNode \*root) {
vector<int> res;
postorder(root, res);
return res;
}
};
[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-tHyQn7hP-1631410165750)(https://raw.githubusercontent.com/xkyvvv/blogpic/main/pic1/image-20210906234322640.png)]
int *postorderTraversal(struct TreeNode *root, int *returnSize) {
int *res = malloc(sizeof(int) * 2001);
*returnSize = 0;
if (root == NULL) {
return res;
}
struct TreeNode **stk = malloc(sizeof(struct TreeNode *) * 2001);
int top = 0;
struct TreeNode *prev = NULL;
while (root != NULL || top > 0) {
while (root != NULL) {
stk[top++] = root;
root = root->left;
}
root = stk[–top];
if (root->right == NULL || root->right == prev) {
res[(*returnSize)++] = root->val;
prev = root;
root = NULL;
} else {
stk[top++] = root;
root = root->right;
}
}
return res;
}
class Solution {
public:
vector postorderTraversal(TreeNode *root) {
vector res;
if (root == nullptr) {
return res;
}
stack<TreeNode \*> stk;
TreeNode \*prev = nullptr;
while (root != nullptr || !stk.empty()) {
while (root != nullptr) {
stk.emplace(root);
root = root->left;
}
root = stk.top();
stk.pop();
if (root->right == nullptr || root->right == prev) {
res.emplace\_back(root->val);
prev = root;
root = nullptr;
} else {
stk.emplace(root);
root = root->right;
}
}
return res;
}
};
[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-XExe5TJK-1631410165750)(https://raw.githubusercontent.com/xkyvvv/blogpic/main/pic1/image-20210906234403833.png)]
void addPath(int *vec, int *vecSize, struct TreeNode *node) {
int count = 0;
while (node != NULL) {
++count;
vec[(*vecSize)++] = node->val;
node = node->right;
}
for (int i = (*vecSize) - count, j = (*vecSize) - 1; i < j; ++i, --j) {
int t = vec[i];
vec[i] = vec[j];
vec[j] = t;
}
}
int *postorderTraversal(struct TreeNode *root, int *returnSize) {
int *res = malloc(sizeof(int) * 2001);
*returnSize = 0;
if (root == NULL) {
return res;
}
struct TreeNode \*p1 = root, \*p2 = NULL;
while (p1 != NULL) {
p2 = p1->left;
if (p2 != NULL) {
while (p2->right != NULL && p2->right != p1) {
p2 = p2->right;
}
if (p2->right == NULL) {
p2->right = p1;
p1 = p1->left;
continue;
} else {
p2->right = NULL;
addPath(res, returnSize, p1->left);
}
}
p1 = p1->right;
}
addPath(res, returnSize, root);
return res;
}
class Solution {
public:
void addPath(vector &vec, TreeNode *node) {
int count = 0;
while (node != nullptr) {
++count;
vec.emplace_back(node->val);
node = node->right;
}
reverse(vec.end() - count, vec.end());
}
vector<int> postorderTraversal(TreeNode \*root) {
vector<int> res;
if (root == nullptr) {
return res;
}
TreeNode \*p1 = root, \*p2 = nullptr;
while (p1 != nullptr) {
p2 = p1->left;
if (p2 != nullptr) {
while (p2->right != nullptr && p2->right != p1) {
p2 = p2->right;
}
if (p2->right == nullptr) {
p2->right = p1;
p1 = p1->left;
continue;
} else {
p2->right = nullptr;
addPath(res, p1->left);
}
}
p1 = p1->right;
}
addPath(res, root);
return res;
}
};
### [110. 平衡二叉树]( )
[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-5Ck6xuXv-1631410165751)(https://raw.githubusercontent.com/xkyvvv/blogpic/main/pic1/image-20210906234611310.png)]
[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-efecf3g3-1631410165751)(https://raw.githubusercontent.com/xkyvvv/blogpic/main/pic1/image-20210906234654446.png)]
int height(struct TreeNode* root) {
if (root == NULL) {
return 0;
} else {
return fmax(height(root->left), height(root->right)) + 1;
}
}
bool isBalanced(struct TreeNode* root) {
if (root == NULL) {
return true;
} else {
return fabs(height(root->left) - height(root->right)) <= 1 && isBalanced(root->left) && isBalanced(root->right);
}
}
class Solution {
public:
int height(TreeNode* root) {
if (root == NULL) {
return 0;
} else {
return max(height(root->left), height(root->right)) + 1;
}
}
bool isBalanced(TreeNode\* root) {
if (root == NULL) {
return true;
} else {
return abs(height(root->left) - height(root->right)) <= 1 && isBalanced(root->left) && isBalanced(root->right);
}
}
};
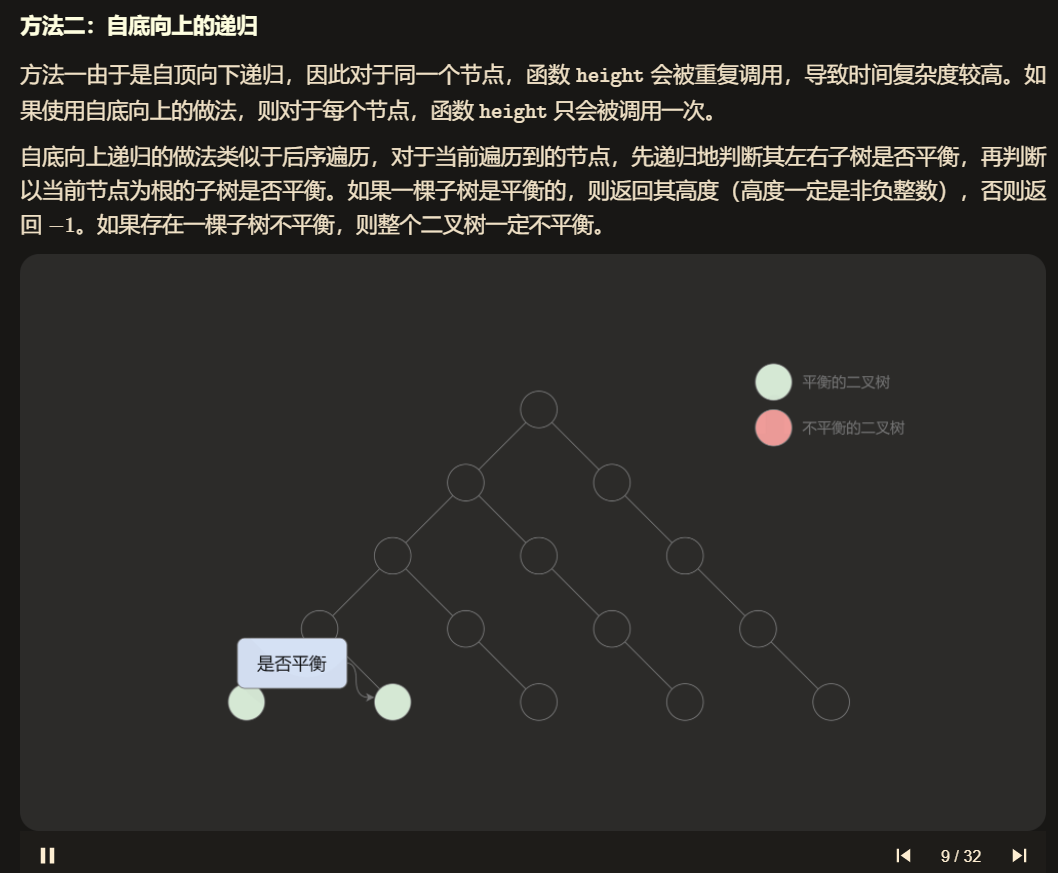
int height(struct TreeNode* root) {
if (root == NULL) {
return 0;
}
int leftHeight = height(root->left);
int rightHeight = height(root->right);
if (leftHeight == -1 || rightHeight == -1 || fabs(leftHeight - rightHeight) > 1) {
return -1;
} else {
return fmax(leftHeight, rightHeight) + 1;
}
}
bool isBalanced(struct TreeNode* root) {
return height(root) >= 0;
}
class Solution {
public:
int height(TreeNode* root) {
if (root == NULL) {
return 0;
}
int leftHeight = height(root->left);
int rightHeight = height(root->right);
if (leftHeight == -1 || rightHeight == -1 || abs(leftHeight - rightHeight) > 1) {
return -1;
} else {
return max(leftHeight, rightHeight) + 1;
}
}
bool isBalanced(TreeNode\* root) {
return height(root) >= 0;
}
};
### [114. 二叉树展开为链表]( )
[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-7hIa3TA8-1631410165752)(https://raw.githubusercontent.com/xkyvvv/blogpic/main/pic1/image-20210906235203849.png)]
[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-AQjmOXnn-1631410165752)(https://raw.githubusercontent.com/xkyvvv/blogpic/main/pic1/image-20210906235425413.png)]
int num;
void flatten(struct TreeNode* root) {
num = 0;
struct TreeNode** l = (struct TreeNode**)malloc(0);
preorderTraversal(root, &l);
for (int i = 1; i < num; i++) {
struct TreeNode *prev = l[i - 1], *curr = l[i];
prev->left = NULL;
prev->right = curr;
}
free(l);
}
void preorderTraversal(struct TreeNode* root, struct TreeNode*** l) {
if (root != NULL) {
num++;
(*l) = (struct TreeNode**)realloc((*l), sizeof(struct TreeNode*) * num);
(*l)[num - 1] = root;
preorderTraversal(root->left, l);
preorderTraversal(root->right, l);
}
}
class Solution {
public:
void flatten(TreeNode* root) {
vector<TreeNode*> l;
preorderTraversal(root, l);
int n = l.size();
for (int i = 1; i < n; i++) {
TreeNode *prev = l.at(i - 1), *curr = l.at(i);
prev->left = nullptr;
prev->right = curr;
}
}
void preorderTraversal(TreeNode\* root, vector<TreeNode\*> &l) {
if (root != NULL) {
l.push\_back(root);
preorderTraversal(root->left, l);
preorderTraversal(root->right, l);
}
}
};
void flatten(struct TreeNode* root) {
struct TreeNode** stk = (struct TreeNode**)malloc(0);
int stk_top = 0;
struct TreeNode** vec = (struct TreeNode**)malloc(0);
int vec_len = 0;
struct TreeNode* node = root;
while (node != NULL || stk_top != 0) {
while (node != NULL) {
vec_len++;
vec = (struct TreeNode**)realloc(vec, sizeof(struct TreeNode*) * vec_len);
vec[vec_len - 1] = node;
stk_top++;
stk = (struct TreeNode**)realloc(stk, sizeof(struct TreeNode*) * stk_top);
stk[stk_top - 1] = node;
node = node->left;
}
node = stk[–stk_top];
node = node->right;
}
for (int i = 1; i < vec_len; i++) {
struct TreeNode *prev = vec[i - 1], *curr = vec[i];
prev->left = NULL;
prev->right = curr;
}
free(stk);
free(vec);
}
class Solution {
public:
void flatten(TreeNode* root) {
auto v = vector<TreeNode*>();
auto stk = stack<TreeNode*>();
TreeNode *node = root;
while (node != nullptr || !stk.empty()) {
while (node != nullptr) {
v.push_back(node);
stk.push(node);
node = node->left;
}
node = stk.top(); stk.pop();
node = node->right;
}
int size = v.size();
for (int i = 1; i < size; i++) {
auto prev = v.at(i - 1), curr = v.at(i);
prev->left = nullptr;
prev->right = curr;
}
}
};
[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-s1hNkuMD-1631410165752)(https://raw.githubusercontent.com/xkyvvv/blogpic/main/pic1/image-20210906235425413.png)]
void flatten(struct TreeNode *root) {
if (root == NULL) {
return;
}
struct TreeNode **stk = (struct TreeNode **)malloc(sizeof(struct TreeNode *));
int stk_top = 1;
stk[0] = root;
struct TreeNode *prev = NULL;
while (stk_top != 0) {
struct TreeNode *curr = stk[–stk_top];
if (prev != NULL) {
prev->left = NULL;
prev->right = curr;
}
struct TreeNode *left = curr->left, *right = curr->right;
if (right != NULL) {
stk_top++;
stk = (struct TreeNode **)realloc(stk, sizeof(struct TreeNode *) * stk_top);
stk[stk_top - 1] = right;
}
if (left != NULL) {
stk_top++;
stk = (struct TreeNode **)realloc(stk, sizeof(struct TreeNode *) * stk_top);
stk[stk_top - 1] = left;
}
prev = curr;
}
free(stk);
}
class Solution {
public:
void flatten(TreeNode* root) {
if (root == nullptr) {
return;
}
auto stk = stack<TreeNode*>();
stk.push(root);
TreeNode *prev = nullptr;
while (!stk.empty()) {
TreeNode *curr = stk.top(); stk.pop();
if (prev != nullptr) {
prev->left = nullptr;
prev->right = curr;
}
TreeNode *left = curr->left, *right = curr->right;
if (right != nullptr) {
stk.push(right);
}
if (left != nullptr) {
stk.push(left);
}
prev = curr;
}
网上学习资料一大堆,但如果学到的知识不成体系,遇到问题时只是浅尝辄止,不再深入研究,那么很难做到真正的技术提升。
一个人可以走的很快,但一群人才能走的更远!不论你是正从事IT行业的老鸟或是对IT行业感兴趣的新人,都欢迎加入我们的的圈子(技术交流、学习资源、职场吐槽、大厂内推、面试辅导),让我们一起学习成长!
return;
}
auto stk = stack<TreeNode*>();
stk.push(root);
TreeNode *prev = nullptr;
while (!stk.empty()) {
TreeNode *curr = stk.top(); stk.pop();
if (prev != nullptr) {
prev->left = nullptr;
prev->right = curr;
}
TreeNode *left = curr->left, *right = curr->right;
if (right != nullptr) {
stk.push(right);
}
if (left != nullptr) {
stk.push(left);
}
prev = curr;
}
[外链图片转存中…(img-uGjlCZnl-1715709887470)]
[外链图片转存中…(img-BEzENwVi-1715709887470)]
网上学习资料一大堆,但如果学到的知识不成体系,遇到问题时只是浅尝辄止,不再深入研究,那么很难做到真正的技术提升。
一个人可以走的很快,但一群人才能走的更远!不论你是正从事IT行业的老鸟或是对IT行业感兴趣的新人,都欢迎加入我们的的圈子(技术交流、学习资源、职场吐槽、大厂内推、面试辅导),让我们一起学习成长!