网上学习资料一大堆,但如果学到的知识不成体系,遇到问题时只是浅尝辄止,不再深入研究,那么很难做到真正的技术提升。
一个人可以走的很快,但一群人才能走的更远!不论你是正从事IT行业的老鸟或是对IT行业感兴趣的新人,都欢迎加入我们的的圈子(技术交流、学习资源、职场吐槽、大厂内推、面试辅导),让我们一起学习成长!
printf("%d ",a[i]);
}
printf("\n");
}
2.快速排序(随机快排)
#include
#include
#include
#include
while(i<j&&a[i]<x) //从左往右找第一个大于等于x的值
i++;
if(i<j)
{
a[j--]=a[i];
}
}
a[i]=x; //循环完成后,i之前的元素都小于x,i之后的元素都大于x
quickSort(a,l,i-1);
quickSort(a,i+1,r);
}
}
int main()
{
srand((unsigned)time(NULL));
int a[20];
for(int i=0; i<20; i++)
{
a[i]=rand()%101;
}
for(int i=0; i<20; i++)
{
printf(“%d “,a[i]);
}
printf(”\n”);
quickSort(a,0,20-1);
for(int i=0; i<20; i++)
{
printf(“%d “,a[i]);
}
printf(”\n”);
}
## 哈夫曼树的构造
[构造哈夫曼树的样例分析](https://bbs.csdn.net/topics/618668825)
## 二叉搜索树的创建
#include
#include
#include
#include
#include
#include
#include
using namespace std;
struct Binary_Node{
int data;
Binary_Node *left;
Binary_Node *right;
};
Binary_Node* createBinaryTree(int data,Binary_Node root){
if(root==NULL)
{
root=(Binary_Node)malloc(sizeof(Binary_Node));
root->left=NULL;
root->right=NULL;
root->data=data;
}
else if(data<root->data){
root->left=createBinaryTree(data ,root->left);
}
else
root->right=createBinaryTree(data,root->right);
return root;
}
void print(Binary_Node *root){
if(root!=NULL)
{
print(root->left);
printf(“%d\n”,root->data);
print(root->right);
}
}
int main ()
{
Binary_Node *root=NULL;
int a[]={10,14 ,16,12,6,4,8};
for(int i=0;i<7;i++)
root=createBinaryTree(a[i],root);
print(root);
return 0;
}
## 二叉搜索树与双向链表
#include
#include
#include
#include
#include
#include
#include
using namespace std;
struct Binary_Node{
int data;
Binary_Node *left;
Binary_Node *right;
};
Binary_Node* createBinaryTree(int data,Binary_Node root){
if(root==NULL)
{
root=(Binary_Node)malloc(sizeof(Binary_Node));
root->left=NULL;
root->right=NULL;
root->data=data;
}
else if(data<root->data){
root->left=createBinaryTree(data ,root->left);
}
else
root->right=createBinaryTree(data,root->right);
return root;
}
//convertNode ,将一个二叉树转换成双向链表.
void convertNode(Binary_Node *node ,Binary_Node **lastNode){
//第二个参数是二重指针,因为后续代码需要更改指针的值所以,需要二重指针,若使用一重指针,则只改变了形参指针的值并未真正的修改实参指针的值,即真正修改指针的值用二重指针
if(node==NULL)
return ;
Binary_Node *current=node;
if(current->left!=NULL)
convertNode(current->left,lastNode);
current->left=*lastNode;
if((*lastNode)!=NULL)
(*lastNode)->right=current;
*lastNode=current;
if(current->right!=NULL)
convertNode(current->right,lastNode);
}
//将表头指针反过来(上面的函数最终指向链表的尾节点)
Binary_Node * convert(Binary_Node *root){
Binary_Node *lastNode=NULL;
convertNode(root,&lastNode);
Binary_Node *head=lastNode;
while(head!=NULL&&head->left!=NULL)
head=head->left;
return head;
}
//打印双向链表
void printList(Binary_Node *root){
while(root!=NULL){
printf(“%d\n”,root->data);
root=root->right;
}
}
void print(Binary_Node *root){
if(root!=NULL)
{
print(root->left);
printf(“%d\n”,root->data);
print(root->right);
}
}
int main ()
{
Binary_Node *root=NULL;
int a[]={10,14 ,16,12,6,4,8};
for(int i=0;i<7;i++)
root=createBinaryTree(a[i],root);
print(root);
root=convert(root);
printList(root);
return 0;
}
## 面试遇到的一些题
### 写一个对链表的快排:
[参照大佬的博客](https://bbs.csdn.net/topics/618668825)
### 在一个单向链表中找倒数第k个数:
[很好的图解](https://bbs.csdn.net/topics/618668825)
### N个乱序的数字中寻找最大的k个数:
思路:维护一个大小为k的小根堆,堆顶元素是最大K 个数中最小的一个,即第K个元素
处理过程对于数组中的每一个元素X,判断与堆顶的大小
如果X 比堆顶小,则不需要改变原来的堆, 因为这个元素比最大的K 个 数小。
如果X比堆顶大,要用X 替换堆顶的元素Y 。调整堆的时间复杂度为O(log2K)。
时间复杂度: O (N \* log2 K ),算法只需要扫描所有的数据一次
空间复杂度:大小为K的数组,只需要存储一个容量为K 的堆。
注意、大多数情况下,堆可以全部载入内存。如果K 仍然很大,我们可以尝试先找最大的K ’个元素,然后找第K ’+1个到第2 \* K ’
元素,如此类推(其中容量K ’的堆可以完全载入内存)。这时,每求出K’个数,就遍历一遍数据了
#错题:
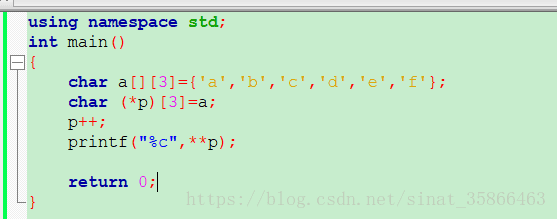
输出 d
堆排序的最坏也是nlogn
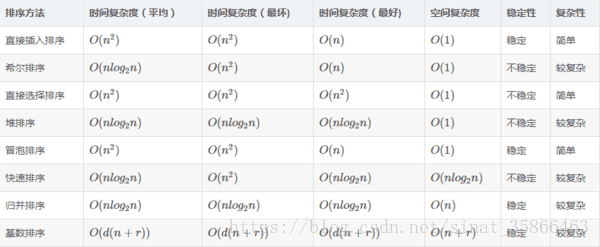
记得学习哈希冲突的解决方法,以及平均查找长度,
空串也是子串,即abc的子串共有 1 + 2+3 +1 =6 +1 =7,注意要减去相同的子串
## 有趣的题目:
[数组越界,for循环](https://bbs.csdn.net/topics/618668825)
## socket编程
[linux下socket编程学习](https://bbs.csdn.net/topics/618668825)
[windows下socket编程学习](https://bbs.csdn.net/topics/618668825)
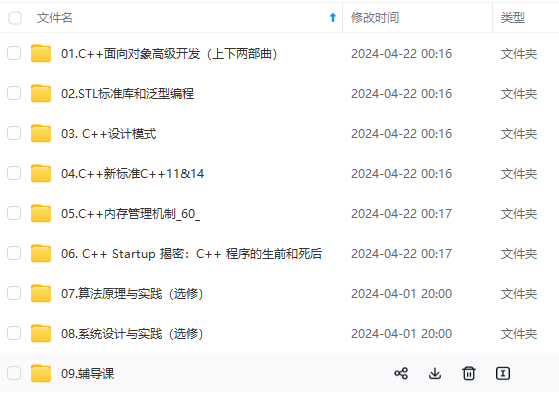
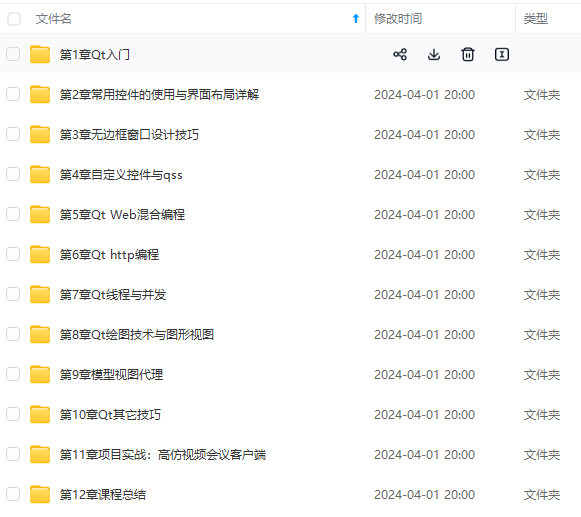
**网上学习资料一大堆,但如果学到的知识不成体系,遇到问题时只是浅尝辄止,不再深入研究,那么很难做到真正的技术提升。**
**[需要这份系统化的资料的朋友,可以添加戳这里获取](https://bbs.csdn.net/topics/618668825)**
**一个人可以走的很快,但一群人才能走的更远!不论你是正从事IT行业的老鸟或是对IT行业感兴趣的新人,都欢迎加入我们的的圈子(技术交流、学习资源、职场吐槽、大厂内推、面试辅导),让我们一起学习成长!**
中...(img-LDp5Ueds-1715812605278)]
[外链图片转存中...(img-Vu52Mnp9-1715812605278)]
**网上学习资料一大堆,但如果学到的知识不成体系,遇到问题时只是浅尝辄止,不再深入研究,那么很难做到真正的技术提升。**
**[需要这份系统化的资料的朋友,可以添加戳这里获取](https://bbs.csdn.net/topics/618668825)**
**一个人可以走的很快,但一群人才能走的更远!不论你是正从事IT行业的老鸟或是对IT行业感兴趣的新人,都欢迎加入我们的的圈子(技术交流、学习资源、职场吐槽、大厂内推、面试辅导),让我们一起学习成长!**