既有适合小白学习的零基础资料,也有适合3年以上经验的小伙伴深入学习提升的进阶课程,涵盖了95%以上C C++开发知识点,真正体系化!
由于文件比较多,这里只是将部分目录截图出来,全套包含大厂面经、学习笔记、源码讲义、实战项目、大纲路线、讲解视频,并且后续会持续更新
}
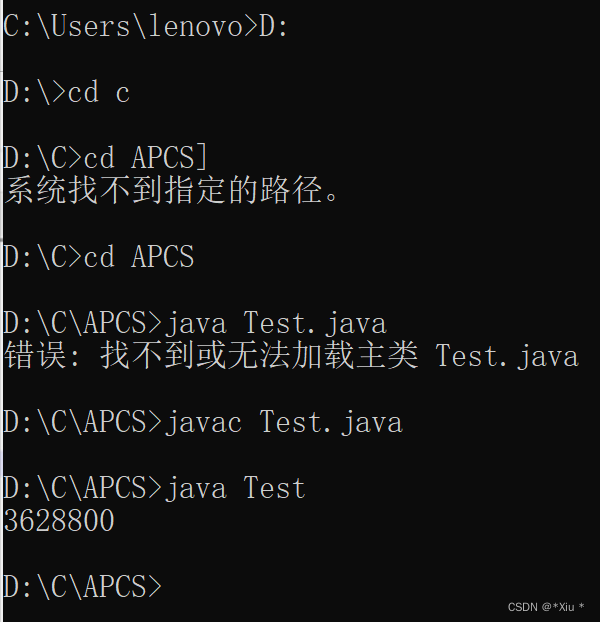
#### 2.Determine whether a number is even or odd,print out the result.
#### 3.Try using "+" operation between Strings,Strings and numbers.
class Test{
//程序入口
public static void main(String args[]){
int result=123456789*10;
//System.out.println(result);
int a=(int)(10*Math.random());
System.out.println(a);
System.out.println(a%2);
String introduce="My name is Java!";
String address="I live in computer";
System.out.println(introduce+address);
System.out.println(introduce+result);
System.out.println(a+result);
}
}
注意:如果出现了中文字符,则编译会产生编译失败,乱码,意思是出现了中文字符,不能编译成功。
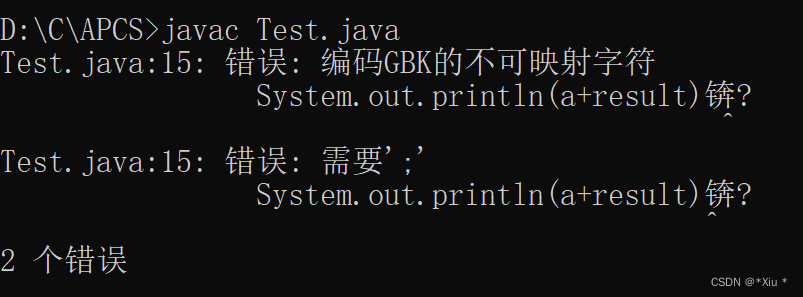
mac在terminal运行方式,相同的运行方式,使用cd 命令进入某个目录,用javac命令进行编译,再用java命令对生成的类文件运行出结果
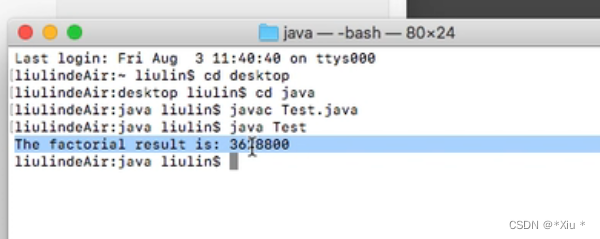
## Judge statement判断语句
计算机指令的执行顺序,就是我们执行的顺序,这个顺序不会变的,而且所有的指令都会被执行一遍。但是对于解决问题而言,这从从上到下的顺序是不合理的,一旦遇到需要选择的情况,则需要条件判断语句。
The statement form:
>
> if(boolean)
>
>
> {
>
>
> statements
>
>
> }
>
>
> //statements will be executed if Boolean expression is true.
>
>
>
例如,判断数字是奇数还是偶数,则需要使用if 判断语句,判断其实现的效果。
class Test{
//程序入口
public static void main(String args[]){
int a=(int)(10*Math.random());
System.out.println(a);
if(a%2==1){
System.out.println("The number"+a+"is an odd number!");
}
if(a%2==0){
System.out.println("The number "+a+"is an even number");
}
}
}
### 在条件判断语句中,经常会放一些运算符Relational operators,
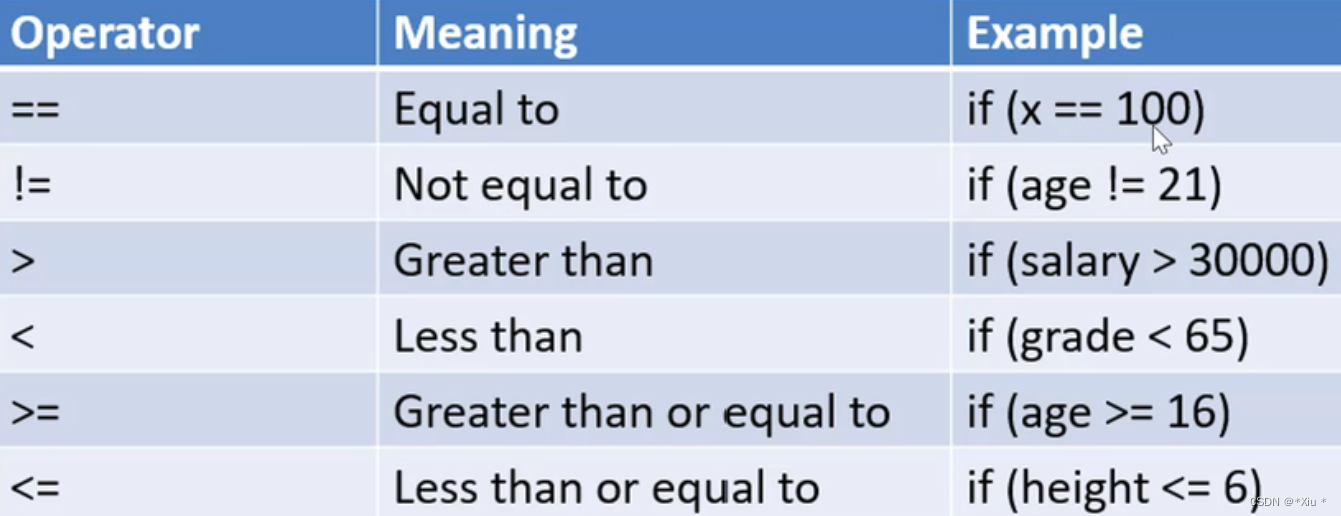
Notes:
The result is a Boolean (either true or false)
Except"==" ;all the relational operators only apply to type int,float and double.综上表格中这些关系操作符都是用来比较数和数之间的。
For"==";apply to type int ,float ,double and String.判断是否相等,既可以判断数,也可以判断字符类型,都可以。举个例子:
>
> String name1="David";
> String name2="david";
> System.out.println(name1==name2);
>
>
>
结果: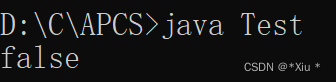
### Logical operators逻辑操作符(Only apply to boolean)

#### De Morgan's Law 德摩根定律 This law can be expressed as**( A ∪ B) ‘ = A ‘ ∩ B ‘**
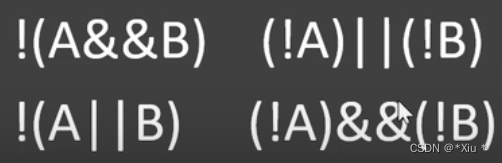
#### Precedence 优先级
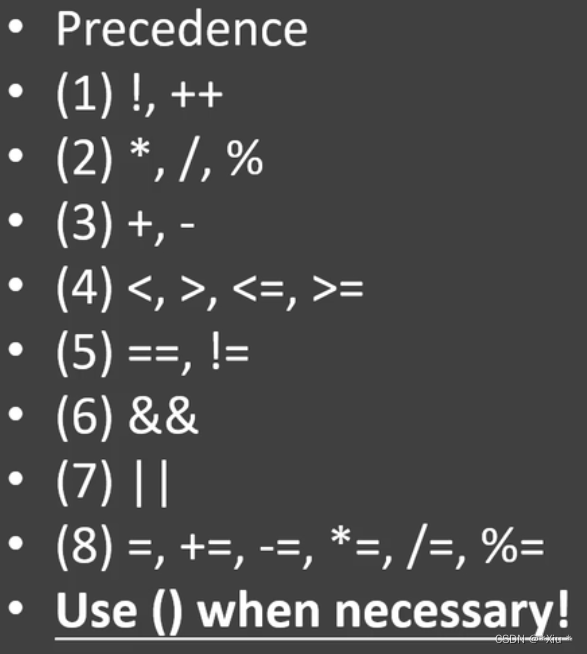 不需要记忆,先要运算的,就用括号给他括在一起,后运算的放在括号外面。
## Branch Statement
class Test{
//程序入口
public static void main(String args[]){
int a=(int)(10*Math.random());
//System.out.println(a);
if(a%2==1){
System.out.println("The number"+a+"is an odd number!");
}
else{
System.out.println("The number "+a+"is an even number");
}
}
----More about judge statement --If else statement分支语句
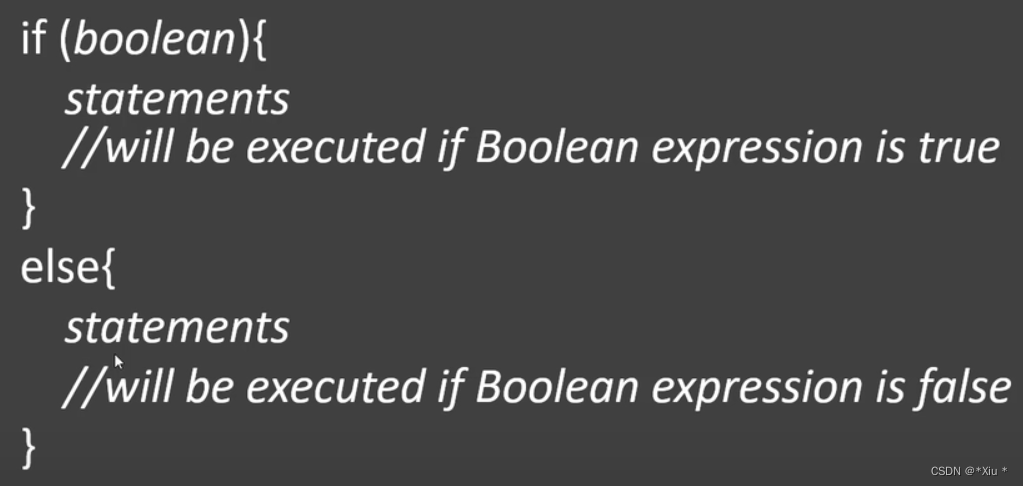
**QUE:** Determine the grade according to scores
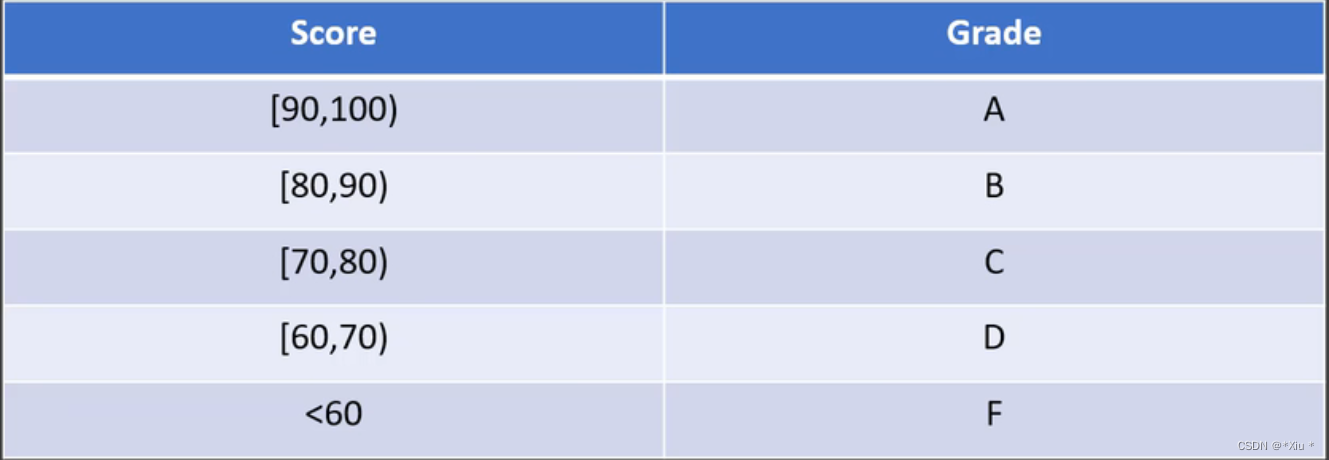
>
>
> ```
> class Test{
> //程序入口
> public static void main(String args[]){
> int score =67;
> if(score>=90 && score <100){
> System.out.println("A");
> }
> if(score>=80 && score <90){
> System.out.println("B");
> }
> if(score>=70 && score <80){
> System.out.println("C");
> }
> if(score>=60 && score <70){
> System.out.println("D");
> }
> if(score<60){
> System.out.println("Fail");
> }
> }
>
> }
> ```
>
> 这种写法,不免非常的繁琐,所以修改成if ---else if,等语句
>
>
>
> ```
> class Test{
> //程序入口
> public static void main(String args[]){
> int score =100;
> if(score>=90 && score <=100){
> System.out.println("A");
> }else if(score>=80){
> System.out.println("B");
> }else if(score>=70){
> System.out.println("C");
> }else if(score>=60){
> System.out.println("D");
> }else {
> System.out.println("Failed");
> }
>
> }
>
> }
> ```
>
> 每一个else if就是一个分支,一旦满足,则执行语句,一旦前面的语句没有被满足,则流换到下一个分支(这里可以强调一下,100这个数字,如果不在条件中添加,运行的结果,会自动流入下一个分支语句,从而输出B)。
>
>
>
**而两者的区别是什么?**
**一旦abc三个条件都满足。**
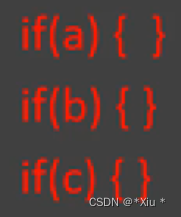前者,是执行的每一条if语句,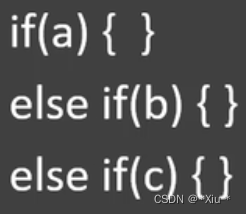后者,并没有执行每一条语句,而是只会执行第一条语句。并且相当于、等价于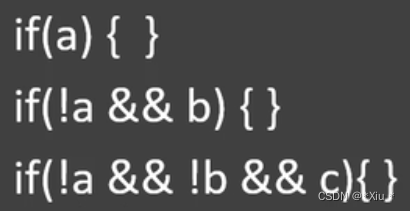。
## Loop Statement: the powerful tool of computer
>
> for (int i = 0; i <100; i++){
>
>
> }
>
>
> int i = 0,表示给i赋一个初始值;the start of the loop
>
>
> i<100 ,判断条件,当i满足这个条件时,循环继续 the loop condition; the loop ends when condition is not satisfied
>
>
> i++ , 循环进行一次后,会发生什么样的变化 the loop body--what to loop
>
>
> {} 中的内容为循环体。 the loop gap
>
>
>
> ```
> //循环输出1-10
> class Test{
> public static void main(String args[]){
> for(int i=1;i<=10;i+=2){
> System.out.println(i);
> }
> }
> }
> ```
>
>
在循环语句的语句体中,还是一个循环,这种语句叫做嵌套 nested,
循环体的里面,为内层循环,循环体的外面的循环,为外层循环。
### Scope of the variable in programs 变量的域
首先,变量不可以重名,否则程序会报错。
在Java中一个大括号,就是一个域。编译器通过大括号来判断从属关系,通过缩进格式,来增加代码的可读性。
### 第二次 CodingTime
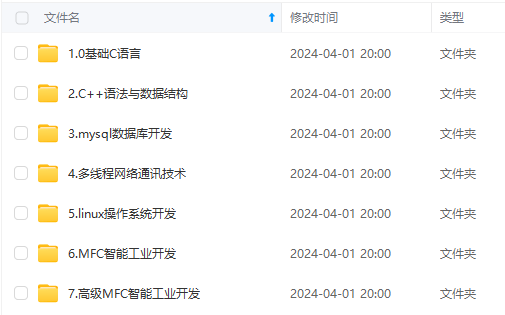
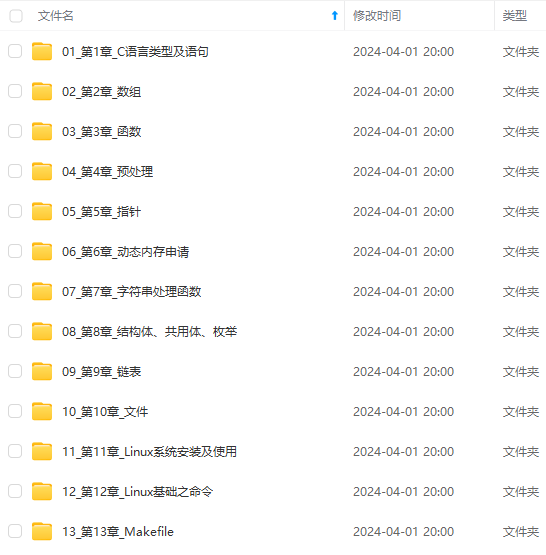
**既有适合小白学习的零基础资料,也有适合3年以上经验的小伙伴深入学习提升的进阶课程,涵盖了95%以上C C++开发知识点,真正体系化!**
**由于文件比较多,这里只是将部分目录截图出来,全套包含大厂面经、学习笔记、源码讲义、实战项目、大纲路线、讲解视频,并且后续会持续更新**
**[如果你需要这些资料,可以戳这里获取](https://bbs.csdn.net/topics/618668825)**
### 第二次 CodingTime
[外链图片转存中...(img-g033J1IM-1715880234143)]
[外链图片转存中...(img-PYq7jCOT-1715880234144)]
**既有适合小白学习的零基础资料,也有适合3年以上经验的小伙伴深入学习提升的进阶课程,涵盖了95%以上C C++开发知识点,真正体系化!**
**由于文件比较多,这里只是将部分目录截图出来,全套包含大厂面经、学习笔记、源码讲义、实战项目、大纲路线、讲解视频,并且后续会持续更新**
**[如果你需要这些资料,可以戳这里获取](https://bbs.csdn.net/topics/618668825)**