既有适合小白学习的零基础资料,也有适合3年以上经验的小伙伴深入学习提升的进阶课程,涵盖了95%以上物联网嵌入式知识点,真正体系化!
由于文件比较多,这里只是将部分目录截图出来,全套包含大厂面经、学习笔记、源码讲义、实战项目、大纲路线、电子书籍、讲解视频,并且后续会持续更新
std::thread::id main_thread_id = std::this_thread::get\_id();
std::cout<<main_thread_id<<std::endl;
while (true)
{
}
}
运行结果(ubuntu20):

### 3.2.3 等待线程结束
* 在Linux下等待线程结束
Linux 线程库提供了`pthread_join`函数,用来等待某线程的退出并接收他的返回值
这种操作被称为汇接(join)
Declared in: pthread.h
static int pthread_join(pthread_t __th, void * *__thread_return)
参数`__th`是需要等待的线程ID;参数\_\_thread\_\_return是输出参数,用于接受被等待线程的退出码,
可以再调用`pthread_exit()`时指定退出码
Declared in: pthread.h
static void pthread_exit(void *__retval)
其中`__retval`可以通过\_\_thread\_return参数获得
下面来展示一个实例,在程序启动时开启一个工作线程,工作线程将当前系统时间写入一个文件后,主线程等待工作线程退出后,从文件中读取时间,并将其输出
#include
#include
#include <pthread.h>
#include
#define TIME_FILENAME “time.txt”
void* fileThreadFunc(void* arg)
{
time_t now = time(nullptr);
tm* t = localtime(&now);
char timeStr[32]={0};
snprintf(timeStr,32,“%04d/%02d %02d:%02d:%02d”,
t->tm_year+1900,
t->tm_mon+1,
t->tm_mday,
t->tm_hour,
t->tm_min,
t->tm_sec);
FILE* fp = fopen(TIME_FILENAME,“w”);
if(fp == nullptr)
{
printf(“打开文件(time.txt)失败\n”);
return nullptr;
}
size_t sizeToWrite=strlen(timeStr) +1;
size_t ret = fwrite(timeStr,1,sizeToWrite,fp);
if(ret != sizeToWrite)
{
printf(“写入错误!\n”);
}
fclose(fp);
return nullptr;
}
int main()
{
pthread_t fileThreadID;
int ret = pthread_create(&fileThreadID, nullptr,fileThreadFunc, nullptr);
if(ret != 0)
{
printf(“创建线程失败\n”);
return -1;
}
int* retval;
pthread_join(fileThreadID,(void**)&retval);
FILE* fp = fopen(TIME_FILENAME,“r”);
if(fp == nullptr)
{
printf(“打开文件失败!\n”);
return -2;
}
char buf[32] = {0};
int sizeRead = fread(buf,1,32,fp);
if(sizeRead == 0)
{
printf("读取文件失败");
fclose(fp);
return -3;
}
printf("Current time is: %s.\n",buf);
fclose(fp);
return 0;
}
执行结果如下:

* Windows 等待线程结束
在Windows下我们可以使用`WaitForSingleObject function (synchapi.h)`和`WaitForMultipleObjects function (synchapi.h)`
* **C++11提供的等待线程结束的函数**
#include
#include
#include
#define TIME_FILENAME “time.txt”
void fileThreadFunc()
{
time_t now = time(nullptr);
tm* t = localtime(&now);
char timeStr[32]={0};
snprintf(timeStr,32,“%04d/%02d %02d:%02d:%02d”,
t->tm_year+1900,
t->tm_mon+1,
t->tm_mday,
t->tm_hour,
t->tm_min,
t->tm_sec);
FILE* fp = fopen(TIME_FILENAME,“w”);
if(fp == nullptr)
{
printf(“打开文件(time.txt)失败\n”);
return ;
}
size_t sizeToWrite=strlen(timeStr) +1;
size_t ret = fwrite(timeStr,1,sizeToWrite,fp);
if(ret != sizeToWrite)
{
printf(“写入错误!\n”);
}
fclose(fp);
return;
}
int main()
{
std::thread t(fileThreadFunc);
if(t.joinable()) t.join();
FILE\* fp = fopen(TIME_FILENAME,"r");
if(fp == nullptr)
{
printf("打开文件失败!\n");
return -2;
}
char buf[32] = {0};
int sizeRead = fread(buf,1,32,fp);
if(sizeRead == 0)
{
printf("读取文件失败");
fclose(fp);
return -3;
}
printf("Current time is: %s.\n",buf);
fclose(fp);
return 0;
}
### 3.4.3 C++11 对整型变量原子操作的支持
C++11 提供了对整形变量原子操作的支持
即`std::atomic`这是一个模板类型
Defined in header
template< class T >
struct atomic;
例子
#include
#include
int main()
{
std::atomic value{1};
value++;//自增,原子操作
std::cout<<value<<std::endl;
}
### C++ 线程同步对象
C++ 新标准中新增用于线程同步的`std::mutex`和`std::condition_variable`
### 3.7.1 `std::mutex`系列
这个系列类型的对象均提供了加锁`lock`,尝试加锁`trylock`和解锁`unlock`的方法
#include
#include
#include
#include
int g_num =0 ;
std::mutex g_num_mutex;
void slow_increment(int id)
{
for (int i = 0; i < 10; ++i)
{
g_num_mutex.lock();
++g_num;
std::cout<<id<<“->”<<g_num<<std::endl;
g_num_mutex.unlock();
}
std::this_thread::sleep_for(std::chrono::seconds(1));
}
int main()
{
std::thread t1(slow_increment,0);
std::thread t2(slow_increment,1);
t1.join();
t2.join();
return 0;
}
输出:
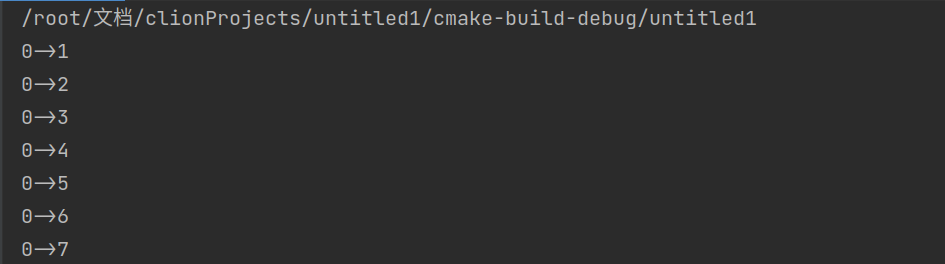
### 3.7.2 `std::shared_mutex`
`std::shared_mutex`的底层实现是操作系统提供的读写锁,也就是说,在有多个线程对共享资源读且少许线程对共享资源写的情况下,`std::shared_mutex`比`std::mutex`效率更高
`shared_mutex` 通常用于多个读线程能同时访问同一资源而不导致数据竞争,但只有一个写线程能访问的情形。
### 3.7.3 `std::condition_variable`
#### Member functions
| [(constructor)](https://bbs.csdn.net/topics/618679757) | constructs the object (public member function) |
| --- | --- |
| [(destructor)](https://bbs.csdn.net/topics/618679757) | destructs the object (public member function) |
| operator=[deleted] | not copy-assignable (public member function) |
| Notification | |
| [notify\_one](https://bbs.csdn.net/topics/618679757) | notifies one waiting thread (public member function) |
| [notify\_all](https://bbs.csdn.net/topics/618679757) | notifies all waiting threads (public member function) |
| Waiting | |
| [wait](https://bbs.csdn.net/topics/618679757) | blocks the current thread until the condition variable is woken up (public member function) |
| [wait\_for](https://bbs.csdn.net/topics/618679757) | blocks the current thread until the condition variable is woken up or after the specified timeout duration (public member function) |
| [wait\_until](https://bbs.csdn.net/topics/618679757) | blocks the current thread until the condition variable is woken up or until specified time point has been reached (public member function) |
| Native handle | |
| [native\_handle](https://bbs.csdn.net/topics/618679757) | returns the native handle (public member function) |
`
[TCP网络编程的基本流程](https://bbs.csdn.net/topics/618679757)
[Linux与C++11多线程编程(学习笔记)](https://bbs.csdn.net/topics/618679757)
[Linux select函数用法和原理](https://bbs.csdn.net/topics/618679757)
[socket的阻塞模式和非阻塞模式(send和recv函数在阻塞和非阻塞模式下的表现)](https://bbs.csdn.net/topics/618679757)
[connect函数在阻塞和非阻塞模式下的行为](https://bbs.csdn.net/topics/618679757)
[获取socket对应的接收缓冲区中的可读数据量](https://bbs.csdn.net/topics/618679757)
---
1. CRT原先是指Microsoft开发的C Runtime Library(C语言运行时库),用于操作系统的开发及运行。后来在此基础上开发了C++ Runtime Library,所以现在CRT是指Microsoft开发的C/C++ Runtime Library。在VC的CRT/SRC目录下,可以看到CRT的源码,不仅有C的,也有C++的。CRT(Microsoft’s C/C++ Runtime Library)的一个真子集(主要是C++ Runtime Library)是一个符合(或至少是企图符合)C++标准的C++库。而Windows API(以及Windows的其他许多部分)都是在CRT的基础上开发的。 [↩︎](#fnref1)
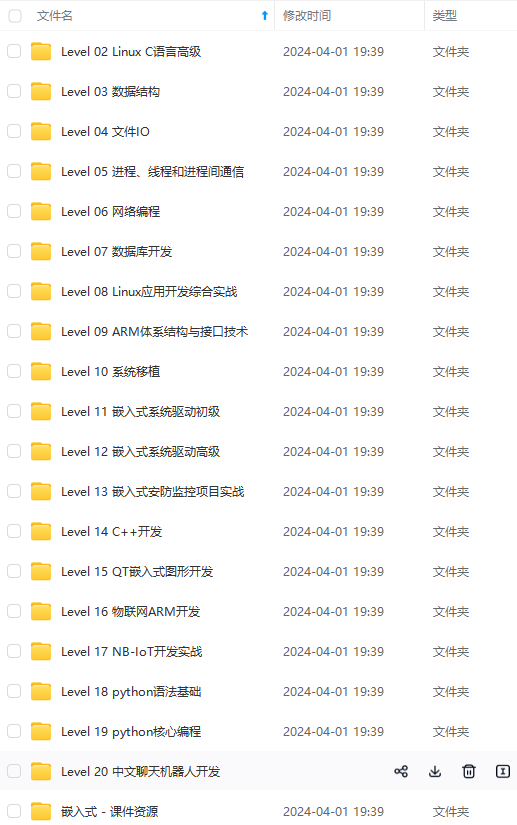
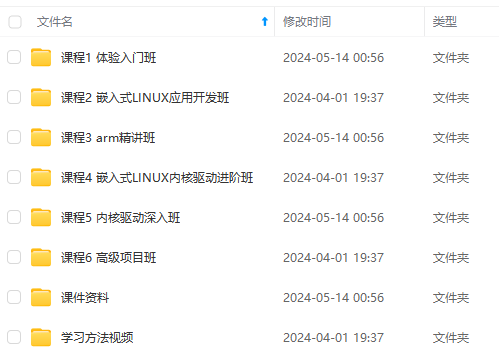
**既有适合小白学习的零基础资料,也有适合3年以上经验的小伙伴深入学习提升的进阶课程,涵盖了95%以上物联网嵌入式知识点,真正体系化!**
**由于文件比较多,这里只是将部分目录截图出来,全套包含大厂面经、学习笔记、源码讲义、实战项目、大纲路线、电子书籍、讲解视频,并且后续会持续更新**
**[如果你需要这些资料,可以戳这里获取](https://bbs.csdn.net/topics/618679757)**
ws的其他许多部分)都是在CRT的基础上开发的。 [↩︎](#fnref1)
[外链图片转存中...(img-zrDmcVkN-1715720326440)]
[外链图片转存中...(img-1iErpVox-1715720326441)]
**既有适合小白学习的零基础资料,也有适合3年以上经验的小伙伴深入学习提升的进阶课程,涵盖了95%以上物联网嵌入式知识点,真正体系化!**
**由于文件比较多,这里只是将部分目录截图出来,全套包含大厂面经、学习笔记、源码讲义、实战项目、大纲路线、电子书籍、讲解视频,并且后续会持续更新**
**[如果你需要这些资料,可以戳这里获取](https://bbs.csdn.net/topics/618679757)**