二级目录开发——商品分组及分组下的商品条目的增删改查,排序(上下移动排序)
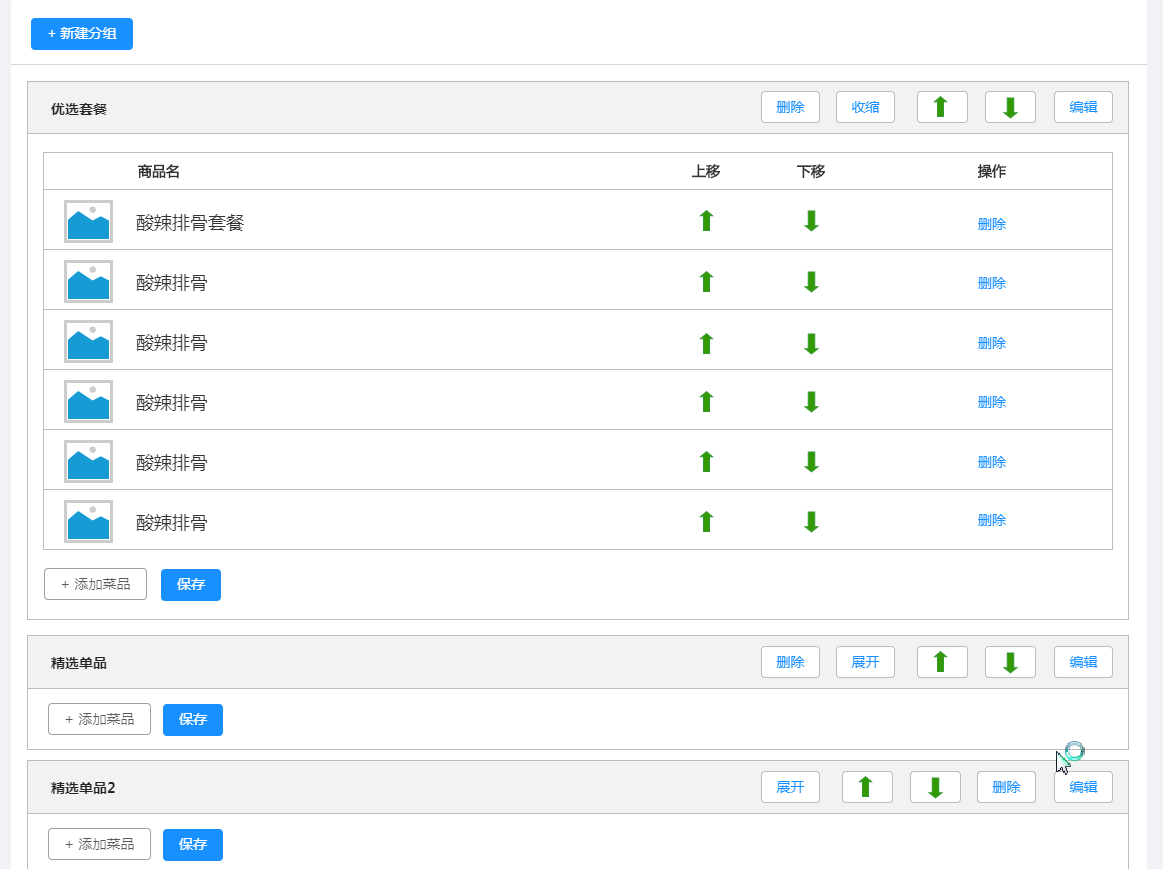
1、展示数据
2、展示数据(汇总+各分组)
3、商品数据详情处理
4、商品属性处理
5、查询所有商品
6、展示数据处理
7、添加分类
8、获取DB中的当前最大排序值
9、删除分组及分组下的所有类目
10、统计
11、编辑分组名
12、删除分组
13、批量删除
14、分组下添加商品条目
15、分组排序
16、分组内部商品移动排序
/**
* @Author: Be.insighted
* Description:商品分组及子层数据的CRUD
* @date Create on 2020/4/10 11:03
**/
@Service
@Slf4j
public class StoreGoodsCategoryService {
@Autowired
private IMenuCategoryDao iMenuCategoryDao;
@Autowired
private IMenuCategoryItemDao iMenuCategoryItemDao;
@Autowired
private GoodsServer goodsServer;
/**
* 展示
* @param storeId
* @return
*/
public List<MenuCategoryGoodsVO> findAllMenuCategory(String storeId) {
if (StringUtils.isEmpty(storeId)) return new ArrayList<>();
List<StoreGoodsCategory> categories = iMenuCategoryDao.findAllByStoreIdOrderBySortIndexAsc(storeId);
if (CollectionUtils.isEmpty(categories)) return new ArrayList<>();
// 获取店铺点餐单商品
List<GoodsDto> goodsDtos = getAllStoreGoodsFromGoodsServer();
log.info("店铺所有商品:{},大小{}", goodsDtos, goodsDtos.size());
if (CollectionUtils.isEmpty(goodsDtos)) {
List<MenuCategoryGoodsVO> ret0 = new ArrayList<>(categories.size());
for (int i = 0; i < categories.size(); i++) {
MenuCategoryGoodsVO vo = new MenuCategoryGoodsVO();
vo.setId(categories.get(i).getId()).setSortIndex(categories.get(i).getSortIndex()).setCategoryName(categories.get(i).getCategoryName()).setList(new ArrayList<>(0));
ret0.add(vo);
}
return ret0;
}
List<MenuCategoryGoodsVO> ret = new ArrayList<>();
Map<String, GoodsDto> goodsId2GoodsDtoMap = goodsDtos.stream().collect(Collectors.toMap(GoodsDto::getGoods_id, g -> g));
log.info("店铺所有商品map:{}", goodsId2GoodsDtoMap);
for (StoreGoodsCategory category : categories) {
List<StoreGoodsCategoryItem> menuGoods = iMenuCategoryItemDao.findAllByCategoryIdOrderBySortIndexAsc(category.getId());
List<StoreGoodsVO> temp = transferMenuGoods(menuGoods, goodsId2GoodsDtoMap);
StoreGoodsCategory menuCategory = iMenuCategoryDao.getOne(category.getId());
MenuCategoryGoodsVO vo = new MenuCategoryGoodsVO();
vo.setCategoryName(menuCategory.getCategoryName())
.setSortIndex(menuCategory.getSortIndex())
.setId(menuCategory.getId())
.setList(temp);
ret.add(vo);
}
return ret;
}
/**
* 展示
* @param req
* @return
*/
@Transactional(readOnly = true)
public List<StoreGoodsMenuGoodsVO> findStoreMenuGoods(QueryStoreGoodsMenuGoodsReq req) {
String storeId = req.getStore_id();
List<StoreGoodsCategory> allCategory = iMenuCategoryDao.findAllByStoreIdOrderBySortIndexAsc(req.getStore_id());
List<GoodsDto> goodsDtos = getStoreGoodsFromGoodsServer(storeId);
log.warn("店铺所有商品数据:{}", goodsDtos);
StoreGoodsMenuGoodsVO all = new StoreGoodsMenuGoodsVO();
all.setCategoryName("全部");
if (CollectionUtils.isEmpty(goodsDtos)){
List<StoreGoodsMenuGoodsVO> ret = new ArrayList<>(1 + allCategory.size());
all.setMenuGoods(new ArrayList<>(0));
ret.add(all);
for (StoreGoodsCategory e : allCategory){
StoreGoodsMenuGoodsVO s = new StoreGoodsMenuGoodsVO();
s.setMenuGoods(new ArrayList<>(0));
s.setCategoryName(e.getCategoryName());
ret.add(s);
}
return ret;
}
Map<String, GoodsDto> goodsId2GoodsDtoMap = goodsDtos.stream().collect(Collectors.toMap(GoodsDto::getGoods_id, g -> g));
// 获取分类下的商品并通过商业云补充商品其他属性
List<StoreGoodsMenuGoodsVO> ret = new ArrayList<>(allCategory.size());
all.setMenuGoods(goodsDtos);
ret.add(all);
for (StoreGoodsCategory category : allCategory) {
List<StoreGoodsCategoryItem> goodsInCategory = iMenuCategoryItemDao.findAllByCategoryIdOrderBySortIndexAsc(category.getId());
// 填充商品详情
StoreGoodsMenuGoodsVO one = new StoreGoodsMenuGoodsVO();
if (CollectionUtils.isEmpty(goodsInCategory)) {
one.setCategoryName(category.getCategoryName()).setMenuGoods(new ArrayList<>(0));
} else {
List<GoodsDto> temp = transferGoodsId2GoodsDetail(goodsInCategory, goodsId2GoodsDtoMap);
one.setCategoryName(category.getCategoryName()).setMenuGoods(temp);
}
ret.add(one);
}
return ret;
}
/**
* 商品详情处理
* @param goodsInCategory
* @param id2GoodsDtoMap
* @return
*/
private List<GoodsDto> transferGoodsId2GoodsDetail(List<StoreGoodsCategoryItem> goodsInCategory, Map<String, GoodsDto> id2GoodsDtoMap) {
List<GoodsDto> temp = new ArrayList<>(goodsInCategory.size());
for (StoreGoodsCategoryItem e : goodsInCategory) {
if(id2GoodsDtoMap.containsKey(e.getGoodsId())){
temp.add(id2GoodsDtoMap.get(e.getGoodsId()));
}
}
return temp;
}
/**
* 展示商品属性
*
* @param storeId
* @return
*/
public List<GoodsDto> getStoreGoodsFromGoodsServer(String storeId) {
QueryGoodsByStoreReqDto reqDto = new QueryGoodsByStoreReqDto();
reqDto.setStore_id(storeId).setGoods_type(0);
GoodsListResultDto ret = goodsServer.queryGoodsByStore(reqDto);
if (ret.getCode() != 0)
throw new BaseRetException(BaseRet.createFailureRet(ret.getMsg()));
log.warn("从商业云查询店铺的商品:{}", ret.getData());
return ret.getData();
}
/**
* 查询所有商品
* @return
*/
public List<GoodsDto> getAllStoreGoodsFromGoodsServer() {
QueryGoodsByStoreReqDto reqDto = new QueryGoodsByStoreReqDto();
reqDto.setGoods_type(0).setPageno(1).setPagesize(Integer.MAX_VALUE).setOrder("create_time").setSort("ASC");
GoodsListResultDto ret = goodsServer.queryGoodsByStore(reqDto);
if (ret.getCode() != 0)
throw new BaseRetException(BaseRet.createFailureRet(ret.getMsg()));
log.warn("从商业云查询店铺的商品:{}", ret.getData());
return ret.getData();
}
/**
* 展示数据处理
* @param menuGoods
* @param map
* @return
*/
public List<StoreGoodsVO> transferMenuGoods(List<StoreGoodsCategoryItem> menuGoods, Map<String, GoodsDto> map) {
List<StoreGoodsVO> temp = new ArrayList<>(menuGoods.size());
for (StoreGoodsCategoryItem e : menuGoods) {
StoreGoodsVO storeGoodsVO = new StoreGoodsVO();
BeanUtils.copyProperties(e, storeGoodsVO);
if (!CollectionUtils.isEmpty(map)) {
storeGoodsVO.setGoodsName(map.get(e.getGoodsId()).getGoods_name());
if (!CollectionUtils.isEmpty(map.get(e.getGoodsId()).getGoods_image())) {
storeGoodsVO.setGoodsImage(map.get(e.getGoodsId()).getGoods_image().get(0).getImage_path());
}
}
temp.add(storeGoodsVO);
}
return temp;
}
/**
* 添加分类
* @param req
* @return
*/
@Transactional(rollbackFor = Exception.class)
public BaseRet menuCategoryAdd(AddCategoryReq req) {
int count = iMenuCategoryDao.countByStoreIdAndCategoryName(req.getStoreId(), req.getName());
if (count > 0) {
throw new BaseRetException(BaseRet.createFailureRet("该店铺已存在分组此名"));
}
StoreGoodsCategory menuCategory = new StoreGoodsCategory();
Integer maxSortIndex = getMaxSortIndex(req.getStoreId());
menuCategory.setCategoryName(req.getName()).setStoreId(req.getStoreId()).setSortIndex(maxSortIndex + 1);
iMenuCategoryDao.save(menuCategory);
return BaseRet.createSuccessRet();
}
/**
* 获取DB中的当前最大排序值
* @param storeId
* @return
*/
private Integer getMaxSortIndex(String storeId) {
StoreGoodsCategory maxSortIndex = iMenuCategoryDao.findTopByStoreIdOrderBySortIndexDesc(storeId);
if (maxSortIndex == null) {
return -1;
} else {
return maxSortIndex.getSortIndex();
}
}
/**
* 删除分组及分组下的所有类目
* @param id
*/
@Transactional(rollbackFor = Exception.class)
public void deleteMenuCategoryAndStoreGoods(String id) {
iMenuCategoryItemDao.deleteAllByCategoryId(id);
iMenuCategoryDao.delete(id);
}
/**
* 计数
* @param id
* @return
*/
public int countNumOfCategory(String id) {
return iMenuCategoryItemDao.countByCategoryId(id);
}
/**
* 编辑分组名
* @param req
* @return
*/
@Transactional(rollbackFor = Exception.class)
public BaseRet updateMenuCategory(UpdateCategoryReq req) {
StoreGoodsCategory e = iMenuCategoryDao.findById(req.getId());
if (e == null) {
log.warn("updateMenuCategory() 无该分组");
return BaseRet.createFailureRet("该店铺不存在该分组");
}
int count = iMenuCategoryDao.countByCategoryName(req.getName());
if (count > 0) {
return BaseRet.createFailureRet("该店铺已存在该分组");
}
e.setCategoryName(req.getName());
iMenuCategoryDao.save(e);
return BaseRet.createSuccessRet();
}
/**
* 删除分类
* @param categoryId
* @param goodsId
*/
@Transactional(rollbackFor = Exception.class)
public void removeMenuCategoryInnerGoods(String categoryId, String goodsId) {
iMenuCategoryItemDao.deleteByCategoryIdAndGoodsId(categoryId, goodsId);
}
/**
* 批量删除
* @param req
*/
@Transactional(rollbackFor = Exception.class)
public void categoryInnerGoodsReliveBatch(ReliveBatchCategoryGoodsReq req) {
iMenuCategoryItemDao.deleteAllByCategoryIdAndGoodsIdIn(req.getCategoryId(), req.getGoodsIds());
}
/**
* 分组下添加商品
* @param req
* @return
*/
@Transactional(rollbackFor = Exception.class)
public BaseRet menuCategoryAddStoreGoods(AddBatchStoreGoodsReq req) {
String storeId = req.getStoreId();
String categoryId = req.getCategoryId();
List<StoreGoodsCategoryItem> menuGoodsList = new ArrayList<>(10);
req.getStoreGoodsDtos().forEach(e -> {
if (!categoryId.equals(e.getCategoryId())) {
throw new BaseRetException(BaseRet.createFailureRet("存在不同点餐单分类,操作失败"));
}
StoreGoodsCategoryItem menuGoods = new StoreGoodsCategoryItem();
menuGoods.setCategoryId(categoryId).setStoreId(storeId)
.setGoodsId(e.getGoodsId());
menuGoodsList.add(menuGoods);
});
List<StoreGoodsCategoryItem> all = iMenuCategoryItemDao.findAllByCategoryIdOrderBySortIndexDesc(categoryId);
Integer maxSortIndex = 0;
if (!CollectionUtils.isEmpty(all)) {
maxSortIndex = all.get(0).getSortIndex() + 1;
}
for (StoreGoodsCategoryItem e : menuGoodsList) {
e.setSortIndex(maxSortIndex++);
}
iMenuCategoryItemDao.save(menuGoodsList);
return BaseRet.createSuccessRet();
}
/**
* 分组排序
* @param id
* @param storeId
* @param move
* @return
*/
@Transactional(rollbackFor = Exception.class)
public BaseRet moveMenuCategory(String id, String storeId, Boolean move) {
Integer count = iMenuCategoryDao.countById(id);
Integer all = iMenuCategoryDao.countByStoreId(storeId);
if (all < 2 || count < 1) {
throw new BaseRetException(BaseRet.createFailureRet("该店铺分组数量不足"));
}
StoreGoodsCategory source = iMenuCategoryDao.getOne(id);
List<StoreGoodsCategory> list = iMenuCategoryDao.findAllByStoreIdOrderBySortIndexAsc(storeId);
Integer sourceIndex = source.getSortIndex();
Integer destinationIndex;
for (int i = 0; i < list.size(); i++) {
if (list.get(i) == source) {
StoreGoodsCategory destination;
if (move) {
if (i == 0) throw new BaseRetException(BaseRet.createFailureRet("已在顶部"));
destination = list.get(i - 1);
destinationIndex = destination.getSortIndex();
} else {
if (i == list.size() - 1) throw new BaseRetException(BaseRet.createFailureRet("已在底部"));
destination = list.get(i + 1);
destinationIndex = destination.getSortIndex();
}
source.setSortIndex(destinationIndex);
destination.setSortIndex(sourceIndex);
List<StoreGoodsCategory> list1 = new ArrayList<>(2);
list1.add(source);
list1.add(destination);
iMenuCategoryDao.save(list1);
}
}
return BaseRet.createSuccessRet();
}
/**
* 内部排序
* @param req
*/
@Transactional(rollbackFor = Exception.class)
public void moveMenuCategoryInnerGoods(MoveStoreGoodsReq req) {
String categoryId = req.getCategoryId();
String goodsId = req.getGoodsId();
StoreGoodsCategoryItem exist = iMenuCategoryItemDao.findByCategoryIdAndGoodsId(categoryId, goodsId);
if (null == exist) {
throw new BaseRetException(BaseRet.createFailureRet("该分组或商品不存在"));
}
int sourceIndex = exist.getSortIndex();
List<StoreGoodsCategoryItem> list = iMenuCategoryItemDao.findAllByCategoryIdOrderBySortIndexAsc(categoryId);
if (list.size() < 2) {
throw new BaseRetException(BaseRet.createFailureRet("该分组下商品数目不足"));
}
List<StoreGoodsCategoryItem> itemList = new ArrayList<>(2);
int affectSortIndex = 0;
StoreGoodsCategoryItem affectedObject;
for (int i = 0; i < list.size(); i++) {
if (list.get(i) == exist) {
if (req.getMove()){
if (i == 0){
return;
}
affectedObject = list.get(i - 1);
affectSortIndex = affectedObject.getSortIndex();
}else {
if ( i == list.size() - 1) {
return;
}
affectedObject = list.get(i + 1);
affectSortIndex = affectedObject.getSortIndex();
}
exist.setSortIndex(affectSortIndex);
affectedObject.setSortIndex(sourceIndex);
itemList.add(exist);
itemList.add(affectedObject);
iMenuCategoryItemDao.save(itemList);
}
}
}
}