目录
一:关于广度优先遍历BFS
广度优先遍历(Breadth-First-Search,BFS)类似于二叉树的层序遍历算法。基本思想是:首先访问起始顶点,接着由
出发,依次访问
的各个未访问过的邻接顶点
,
,...,
,然后依次访问
,
,...,
的所有未被访问过的邻接顶点;再从这些访问过的顶点出发,访问它们所有未被访问过的邻接顶点,直至途中所有顶点都被访问过为止。若此时图中尚有顶点未被访问,则选择图中一个未曾被访问过的顶点作为起始点,重复上述过程,直至图中所有顶点都被访问到为止。
换句话说,广度优先搜索遍历图的过程是以为起点,由近至远依次访问和
有路径相通的顶点。广度优先搜索是一种分层的查找过程,每向前走一步可能访问一批顶点,不像深度优先搜索那样有往回倒退的情况,因此他不是一个递归算法。为了实现逐层的访问,算法必须借助一个辅助队列,用来记忆正在访问的顶点的下一层顶点。
如下图所示的图G,从2号点开始广度优先遍历:
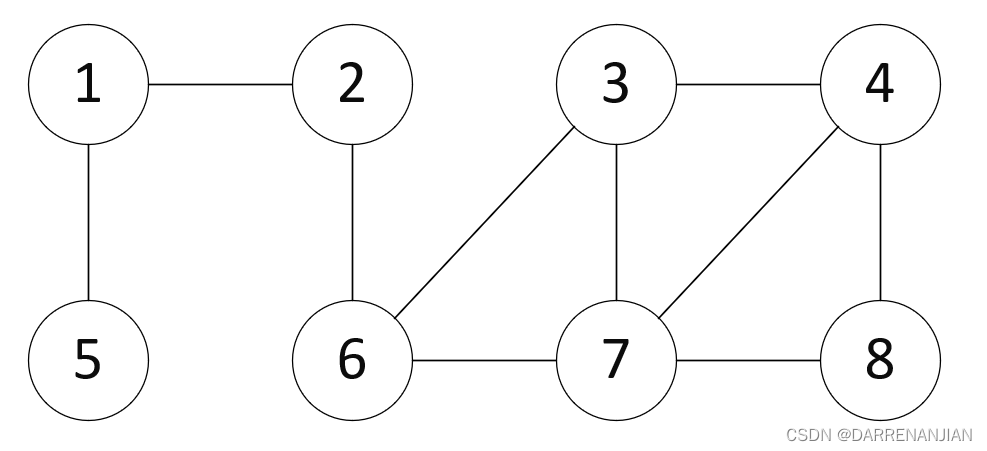
step 1:遍历自身节点2
step 2:遍历节点1,6
step 3:遍历节点5
step 4:遍历节点3,7
step 5:遍历节点4
step 6:遍历节点8
二:如何实现广度优先遍历
step1(初始化):对图像进行广度遍历搜索,在这里我们首先设置一个一维矩阵V,然后对一维矩阵V初始化(所有节点设置为false未访问),再引入一个队列Q,这个队列Q为空。接着我们从号顶点开始遍历,设置一维矩阵的
号节点为true,接着将
号节点入队列。result是记录结果的变量。
step2(第一层循环当队头元素为空结束):接着用temp记录队头元素的数值,当temp不等于空的时候
step3:(第二层循环找到行所有满足条件的元素):开始第二次循环:寻找没有被访问过且邻接矩阵第
行不等于0的列
,设置访问矩阵V的第
列为true,记录结果,然后将
号节点入队列。最后队列Q的队头元素出队列,删除队头元素并且用result记录删队头元素的值。
一直循环,直到temp记录的队列头值为空。
三:广度优先遍历的代码实现
在闵老师的代码实现中,我们构造的是Graph类的对象tempGraph,接着用tempGraph调用调用String breadthFirstTraversal(int num(决定从哪个点开始遍历))函数,所以在这里我只解释breadthFirstTraversal函数,而Graph的其他函数和成员变量,详见第32天博客。
初始化:设置了一个队列tempQueue,设置了resultString用来记录最终的结果(字符串),定义整型变量tempNumNodes得到tempGraph中邻接矩阵维数大小,创造访问矩阵tempVisitedArray用来记录每一个被遍历过的节点(大小等于tempNumNodes),设置初始点的访问位为true,resultString记录第一个节点num,并且将这个节点入队列。
CircleObjectQueue tempQueue = new CircleObjectQueue();
String resultString = "";
int tempNumNodes = connectivityMatrix.getRows();
boolean[] tempVisitedArray = new boolean[tempNumNodes];
//Initialize the queue.
//Visit before enqueue.
tempVisitedArray[paraStartIndex] = true;
resultString += paraStartIndex;
tempQueue.enqueue(new Integer(paraStartIndex));
核心代码:本来这一部分我想做成两个循环分开的,可是这样对于读者来说可能不好理解,所以在这里暂且写到一起。
用tempIndex记录队列出队的值,当tempIndex不为空的时候:开始第二轮循环:在邻接矩阵的tempIndex行里面寻找:1)没有被访问的节点,2)并且和第tempIndex行是直接连通的节点,将寻找到的第数的访问位设置为true,resultString记录这个
,将第
个节点入队,第二轮循环结束。然后又用tempIndex记录队列出队的值,和第一层循环一样,知道tempIndex为空,结束第一层循环。
最终我们得到结果resultString。
在这里补充一点,因为闵老师在构建队列这个类CircleObjectQueue的时候,传入的参数是一个抽象类object,所以我们会将传入的int型数字paraStartIndex通过new Integer(paraStartIndex)转化为一个类,再入队列。在这里提一句是为了避免将int型变量和inter类相混淆。
//Now visit the rest of the graph.
int tempIndex;
Integer tempInteger = (Integer)tempQueue.dequeue();
while (tempInteger != null) {
tempIndex = tempInteger.intValue();
//Enqueue all its unvisited neighbors.
for (int i = 0; i < tempNumNodes; i ++) {
if (tempVisitedArray[i]) {
continue; //Already visited.
}//Of if
if (connectivityMatrix.getData()[tempIndex][i] == 0) {
continue; //Not directly connected.
}//Of if
//Visit before enqueue.
tempVisitedArray[i] = true;
resultString += i;
tempQueue.enqueue(new Integer(i));
}//Of for i
//Take out one from the head.
tempInteger = (Integer)tempQueue.dequeue();
}//Of while
return resultString;
}//Of breadthFirstTraversal
四:代码展示
主类:
package Day_33;
import Day_32.Graph;
public class demo1 {
/**
*********************
* The entrance of the program.
*
* @param args
* Not used now.
*********************
*/
public static void main(String args[]) {
System.out.println("Hello!");
Graph tempGraph = new Graph(3);
// System.out.println(tempGraph);
// tempGraph.getConnectivityTest();
tempGraph.breadthFirstTraversalTest();
}// Of main
}
调用类:(为了方便理解我把Graph所有代码都写在这个地方了)
package Day_32;
import Day_22.CircleObjectQueue;
import Day_31.IntMatrix;
public class Graph {
/**
* The connectivity matrix.
*/
IntMatrix connectivityMatrix;
/**
*********************
* The first constructor.
*
* @param paraNumNodes
* The number of nodes in the graph.
*********************
*/
public Graph(int paraNumNodes) {
connectivityMatrix = new IntMatrix(paraNumNodes, paraNumNodes);
}// Of the first constructor
/**
*********************
* The second constructor.
*
* @param paraMatrix
* The data matrix.
*********************
*/
public Graph(int[][] paraMatrix) {
connectivityMatrix = new IntMatrix(paraMatrix);
}// Of the second constructor
/**
*********************
* Overrides the method claimed in Object, the superclass of any class.
*********************
*/
public String toString() {
String resultString = "This is the connectivity matrix of the graph.\r\n"
+ connectivityMatrix;
return resultString;
}// Of toString
/**
*********************
* Get the connectivity of the graph.
*
* @throws Exception
* for internal error.
*********************
*/
public boolean getConnectivity() throws Exception {
// Step 1. Initialize accumulated matrix: M_a = I.
IntMatrix tempConnectivityMatrix = IntMatrix.getIdentityMatrix(connectivityMatrix.getData().length);
// Step 2. Initialize M^1.
IntMatrix tempMultipliedMatrix = new IntMatrix(connectivityMatrix);
// Step 3. Determine the actual connectivity.
for (int i = 0; i < connectivityMatrix.getData().length - 1; i++) {
// M_a = M_a + M^k
tempConnectivityMatrix.add(tempMultipliedMatrix);
// M^k
tempMultipliedMatrix = IntMatrix.multiply(tempMultipliedMatrix, connectivityMatrix);
} // Of for i
// Step 4. Check the connectivity.
System.out.println("The connectivity matrix is: " + tempConnectivityMatrix);
int[][] tempData = tempConnectivityMatrix.getData();
for (int i = 0; i < tempData.length; i++) {
for (int j = 0; j < tempData.length; j++) {
if (tempData[i][j] == 0) {
System.out.println("Node " + i + " cannot reach " + j);
return false;
} // Of if
} // Of for j
} // Of for i
return true;
}// Of getConnectivity
/**
*********************
* Unit test for getConnectivity.
*********************
*/
public static void getConnectivityTest() {
// Test an undirected graph.
int[][] tempMatrix = { { 0, 1, 0 }, { 1, 0, 1 }, { 0, 1, 0 } };
Graph tempGraph2 = new Graph(tempMatrix);
System.out.println(tempGraph2);
boolean tempConnected = false;
try {
tempConnected = tempGraph2.getConnectivity();
} catch (Exception ee) {
System.out.println(ee);
} // Of try.
System.out.println("Is the graph connected? " + tempConnected);
// Test a directed graph.
// Remove one arc to form a directed graph.
tempGraph2.connectivityMatrix.setValue(1, 0, 0);
tempConnected = false;
try {
tempConnected = tempGraph2.getConnectivity();
} catch (Exception ee) {
System.out.println(ee);
} // Of try.
System.out.println("Is the graph connected? " + tempConnected);
}// Of getConnectivityTest
/**
*********************
* Breadth first traversal.
*
* @param paraStartIndex The start index.
* @return The sequence of the visit.
*********************
*/
public String breadthFirstTraversal(int paraStartIndex) {
CircleObjectQueue tempQueue = new CircleObjectQueue();
String resultString = "";
int tempNumNodes = connectivityMatrix.getRows();
boolean[] tempVisitedArray = new boolean[tempNumNodes];
//Initialize the queue.
//Visit before enqueue.
tempVisitedArray[paraStartIndex] = true;
resultString += paraStartIndex;
tempQueue.enqueue(new Integer(paraStartIndex));
//Now visit the rest of the graph.
int tempIndex;
Integer tempInteger = (Integer)tempQueue.dequeue();
while (tempInteger != null) {
tempIndex = tempInteger.intValue();
//Enqueue all its unvisited neighbors.
for (int i = 0; i < tempNumNodes; i ++) {
if (tempVisitedArray[i]) {
continue; //Already visited.
}//Of if
if (connectivityMatrix.getData()[tempIndex][i] == 0) {
continue; //Not directly connected.
}//Of if
//Visit before enqueue.
tempVisitedArray[i] = true;
resultString += i;
tempQueue.enqueue(new Integer(i));
}//Of for i
//Take out one from the head.
tempInteger = (Integer)tempQueue.dequeue();
}//Of while
return resultString;
}//Of breadthFirstTraversal
/**
*********************
* Unit test for breadthFirstTraversal.
*********************
*/
public static void breadthFirstTraversalTest() {
// Test an undirected graph.
int[][] tempMatrix = { { 0, 1, 1, 0 }, { 1, 0, 0, 1 }, { 1, 0, 0, 1}, { 0, 1, 1, 0} };
Graph tempGraph = new Graph(tempMatrix);
System.out.println(tempGraph);
String tempSequence = "";
try {
tempSequence = tempGraph.breadthFirstTraversal(2);
} catch (Exception ee) {
System.out.println(ee);
} // Of try.
System.out.println("The breadth first order of visit: " + tempSequence);
}//Of breadthFirstTraversalTest
}
五:数据测试
闵老师的图像G和对应邻接矩阵M
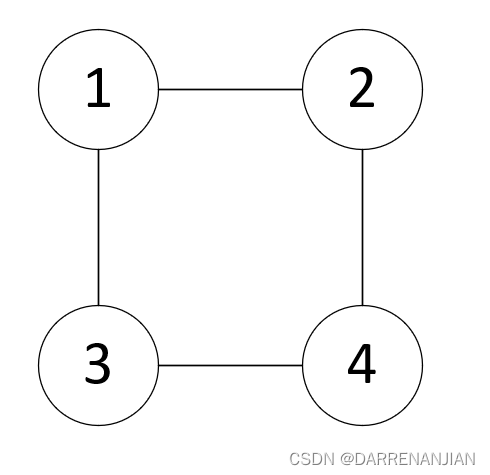
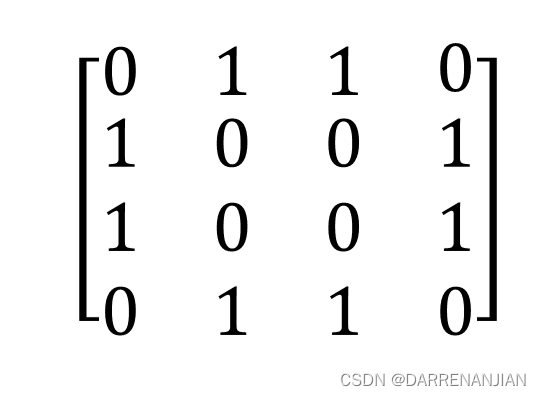
得到的结果
六:总结
图的广度遍历,非常常见的算法题,在数据结构中和离散数学的图论中我们都有接触,广度遍历不需要使用递归算法,仅仅需要设置一个队列存储进出的元素即可。相信二叉树的层序遍历读者都能够理解,其实图的广度遍历就相当于稍微复杂的二叉树的层序遍历,不同是二叉树我们知道这个节点的相连的节点最多有两个节点,而图的某一个节点我们是不知道这个节点所连接的节点的个数的,所以在这里我们构造了一个访问矩阵(一维)用来存储访问过的节点,并且第二层循环里面遍历寻找一个节点
的直接相连的节点
,再以和二叉树层序遍历相同的方式压入队列,周而复始得到最终的resultString字符串。