160. Intersection of Two Linked Lists*
https://leetcode.com/problems/intersection-of-two-linked-lists/
题目描述
Write a program to find the node at which the intersection of two singly linked lists begins.
For example, the following two linked lists:
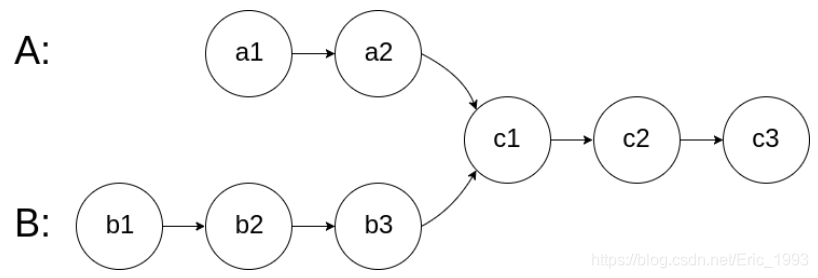
begin to intersect at node c1.
Example 1:
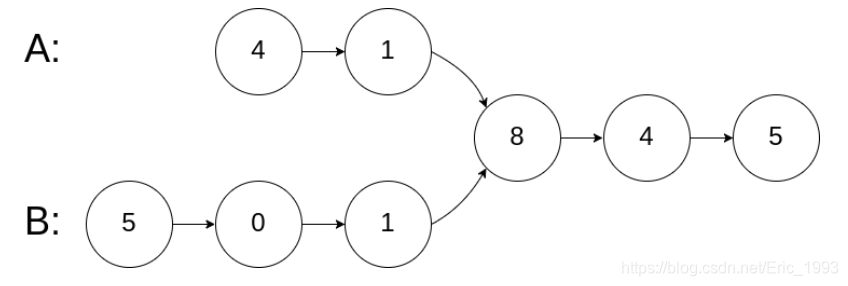
Input: intersectVal = 8, listA = [4,1,8,4,5], listB = [5,0,1,8,4,5], skipA = 2, skipB = 3
Output: Reference of the node with value = 8
Input Explanation: The intersected node's value is 8 (note that this must not be 0 if the two lists intersect). From the head of A, it reads as [4,1,8,4,5]. From the head of B, it reads as [5,0,1,8,4,5]. There are 2 nodes before the intersected node in A; There are 3 nodes before the intersected node in B.
Example 2:
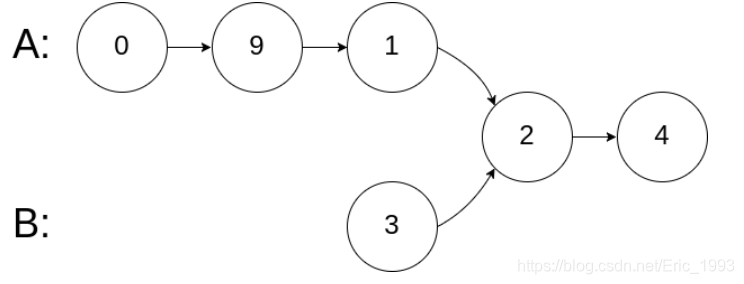
Input: intersectVal = 2, listA = [0,9,1,2,4], listB = [3,2,4], skipA = 3, skipB = 1
Output: Reference of the node with value = 2
Input Explanation: The intersected node's value is 2 (note that this must not be 0 if the two lists intersect). From the head of A, it reads as [0,9,1,2,4]. From the head of B, it reads as [3,2,4]. There are 3 nodes before the intersected node in A; There are 1 node before the intersected node in B.
Example 3:
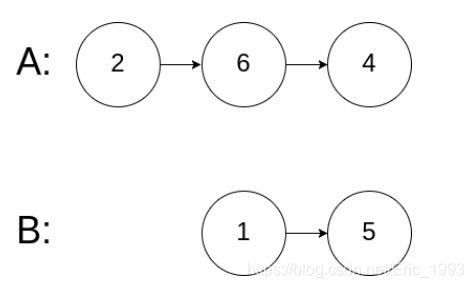
Input: intersectVal = 0, listA = [2,6,4], listB = [1,5], skipA = 3, skipB = 2
Output: null
Input Explanation: From the head of A, it reads as [2,6,4]. From the head of B, it reads as [1,5]. Since the two lists do not intersect, intersectVal must be 0, while skipA and skipB can be arbitrary values.
Explanation: The two lists do not intersect, so return null.
Notes:
- If the two linked lists have no intersection at all, return
null
. - The linked lists must retain their original structure after the function returns.
- You may assume there are no cycles anywhere in the entire linked structure.
- Your code should preferably run in O ( n ) O(n) O(n) time and use only O ( 1 ) O(1) O(1) memory.
C++ 实现 1
从 142. Linked List Cycle II** 这道题可以吸取一点处理思路.
这道题也要设置双指针, 但需要注意到下面的事实:
1 -> 2 -> 3 -> 4 -> 5 -> 6 -> NULL
/
7 -> 8
对于上面两个链表, 假设 1->4
的长度为 a, 7->4
的长度为 b, 4->NULL
的长度为 c,
那么, 如果设 p1 来遍历链表 1, p2 来遍历链表 2, 那么由于链表 2 比较短, 所以 p2 肯定比 p1 先到 NULL, 那么此时让 p2 指向链表 1(对, 没错是链表 1), 然后两个指针继续移动, 当 p1 移到 NULL 时, 再让 p1 指向链表 2, 然后两个指针继续移动, 最后一定会在 4 这个节点处相遇.
这是因为, 此时, p2 走过的路径长度为 b + c + a, 而 p1 走过的长度为 a + c + b, 两个长度相等!
如果两个链表不相交, 那么最终两个指针都会指向 NULL. 此时, 两个链表的移动距离是 m + n.
总之, 最后就是让 p
和 q
分别来遍历链表 1 和链表 2, 如果 p
到了链表 1 的末尾, 那么就让 p
转去遍历链表 2, 而如果 q
达到了链表 2 的末尾, q
同样也拿去遍历链表 1. 只需要判断最后 p
和 q
是否指向同一个节点.
class Solution {
public:
ListNode *getIntersectionNode(ListNode *headA, ListNode *headB) {
auto p = headA, q = headB;
while (p != q) {
p = p == nullptr ? headB : p->next;
q = q == nullptr ? headA : q->next;
}
return p;
}
};