文件结构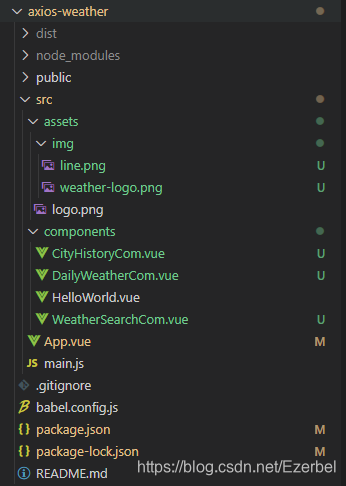
CityHistoryCom.vue
<template>
<div>
<a class="cityLink" @click="chooseCity($event)" >{{city}}</a>
</div>
</template>
<script>
export default {
props:["city"],
methods: {
chooseCity:function(e){
let city = e.target.innerText;
this.$emit('get-city-weather',city);
}
},
}
</script>
<style scoped>
a:hover{
color:brown;
text-decoration: blue;
}
</style>
DailyWeatherCom.vue
<template>
<div class="dailyItem">
<h1 class="typeInfo">{{info.type}}</h1>
<div class="tmpInfo">
<span class="tmpSpan">{{info.high}}</span>~<span class="tmpSpan">{{info.low}}</span>
</div>
<div class="dateInfo">{{info.date}}</div>
</div>
</template>
<script>
export default {
props:["info"],
data:function() {
return {
}
}
}
</script>
<style scoped>
.typeInfo{
color:orange;
}
.dailyItem{
width: 180px;
height: 300px;
background-image: none;
background-size: 1px 130px;
background-repeat: no-repeat;
overflow: hidden;
padding-left: 10px;
padding-right: 10px;
display: flex;
flex-direction: column;
align-items: center;
justify-content: center;
}
.tmpSpan{
font-size: 15px;
}
.tmpInfo{
height: 30px;
color: darkgoldenrod;
}
.dateInfo{
height: 100px;
}
</style>
WeatherSearchCom.vue
<template>
<div class="main">
<div class="logo">
<img src="../assets/img/weather-logo.png">
</div>
<div class="searchBox" >
<input type="text" v-model="cityName" @keyup.enter="getWeather" class="cityInput">
<button type="button" @click="getWeather" class="searchBtn">搜索</button>
</div>
<div class="cityItems">
<ul class="cityList">
<CityHistoryCom v-for="(city,index) in cityList" @get-city-weather='getCityWeather' v-bind:city="city" v-bind:key="index" class="cityItem"></CityHistoryCom>
</ul>
</div>
<hr/>
<div class="dailyItems">
<ul class="weatherList">
<DailyWeatherCom v-for="(info,index) in weatherList" v-bind:info="info" v-bind:key="index"></DailyWeatherCom>
</ul>
</div>
</div>
</template>
<script>
import axios from 'axios';
import DailyWeatherCom from "./DailyWeatherCom";
import CityHistoryCom from "./CityHistoryCom";
export default {
components:{
DailyWeatherCom,
CityHistoryCom
},
data(){
return {
wtype:"多云",
cityName:"北京",
cityList:[],
weatherList:[]
}
},
watch:{
},
methods: {
mounted:function(){
this.getCityList();
},
getCityList:function(){
console.log("getCityList");
let listStr =localStorage.weatherSearchList;
this.cityList = JSON.parse(listStr);
},
updateHistory:function(){
var cityName = this.cityName;
let listStr =localStorage.weatherSearchList;
let cityArr = null;
if(listStr==null){
cityArr = new Array();
cityArr.push(cityName);
}else{
cityArr = JSON.parse(listStr);
var index = cityArr.indexOf(cityName);
if(index>=0){
cityArr.splice(index,1);
}
cityArr.unshift(cityName);
}
this.cityList = cityArr.splice(0,8);
cityArr = this.cityList;
listStr = JSON.stringify(cityArr);
localStorage.setItem("weatherSearchList",listStr);
console.log(listStr);
},
getCityWeather:function(city){
this.cityName = city;
console.log("parent getCityWeather");
this.getWeather();
},
getWeather:function(){
console.log("get weather···");
var cityName = this.cityName;
var that = this;
axios.get(`http://wthrcdn.etouch.cn/weather_mini?city=${cityName}`).then(
function(response){
console.log(response);
that.weatherList = response.data.data.forecast;
that.updateHistory();
},function(err){
console.log("err···");
}
)
.catch(function(err){
console.log(err);
})
}
}
}
</script>
<style scoped>
*{
margin: 0;
padding: 0;
box-sizing: border-box;
}
.cityList{
width: 800px;
height: 30px;
display:flex;
flex-direction: row;
justify-content: center;
flex-wrap: wrap;
align-items: center;
}
.cityItem{
margin-left: 10px;
margin-right: 10px;
}
.logo{
height: 80px;
}
.main{
display: flex;
flex-direction: column;
justify-content: center;
align-items: center;
}
.searchBox{
display:flex;
flex-direction: row;
justify-content: center;
align-content: center;
}
.searchBtn{
width: 100px;
height: 35px;
background-color: #41a1cb;
color: white;
font-size: 18px;
outline: none;
border: 1px solid #41a1cb;
}
.cityInput{
width: 500px;
height: 35px;
outline: none;
font-size: 18px;
border-color: #41a1cb;
border-width: 1px;
padding-left: 10px;
padding-right: 10px;
}
.weatherList{
display: flex;
flex-direction: row;
justify-content: center;
align-items: center;
}
</style>
测试案例
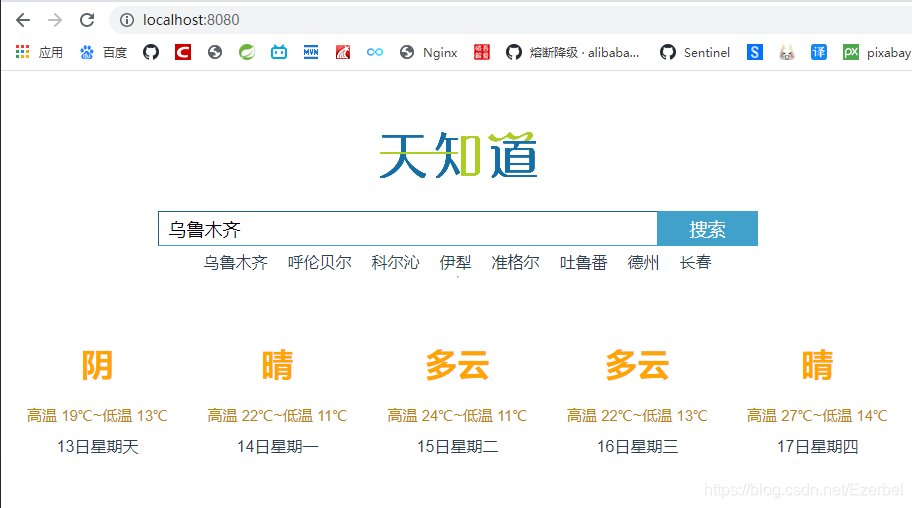