异步函数的使用规则
正常情况下我们的函数时串行的运行的,这里称之为主函数.
异步函数:与主函数并行运行.
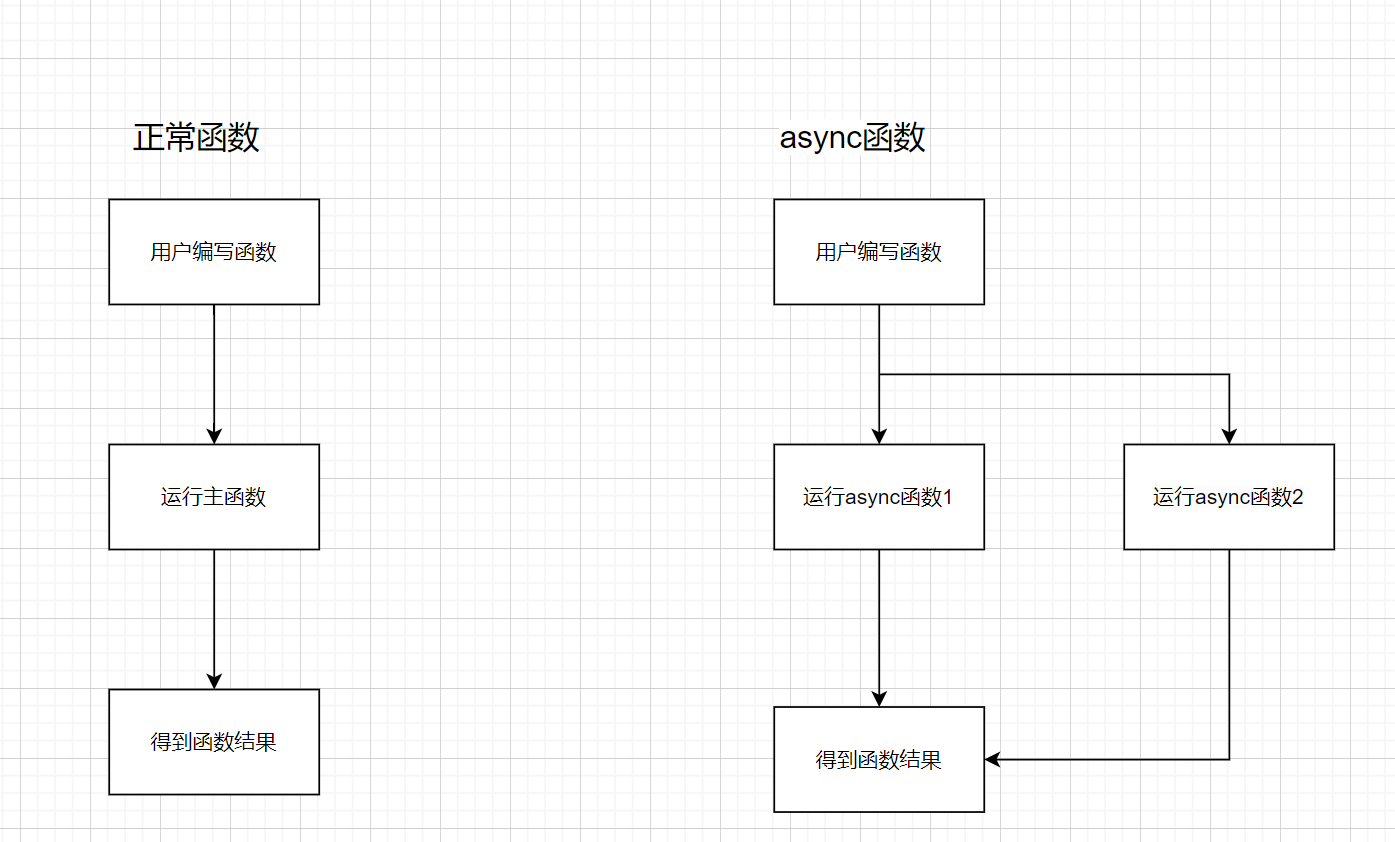
Python异步函数即async必须在普通函数的命名前加上async
示例:
async def case_b():
print('start', get_time(), 'case_b')
await asyncio.sleep(1)
print('end', get_time(), 'case_b')
执行async函数
if __name__ == '__main__':
asyncio.run(case_b())
async函数内等待:只阻塞当前async函数
await asyncio.sleep(2)

async函数的代码示例
import asyncio
import time
from x_mock.m_mock import m_mock
def get_time():
return m_mock.mock('@time("%H:%M:%S.%f")')
async def case_a():
print('start', get_time(), 'case_a')
await asyncio.sleep(2) # 只阻塞当前函数,所以比case_b 多等 1s,下面这句最后打印
print('end', get_time(), 'case_a')
async def case_b():
print('start', get_time(), 'case_b')
await asyncio.sleep(1)
print('end', get_time(), 'case_b')
async def main():
await asyncio.gather(
case_a(),
case_b()
)
if __name__ == '__main__':
start = time.time()
# asyncio.run(main()) # 运行方式1
# 运行方式2
loop = asyncio.get_event_loop()
loop.run_until_complete(main())
print(time.time() - start)
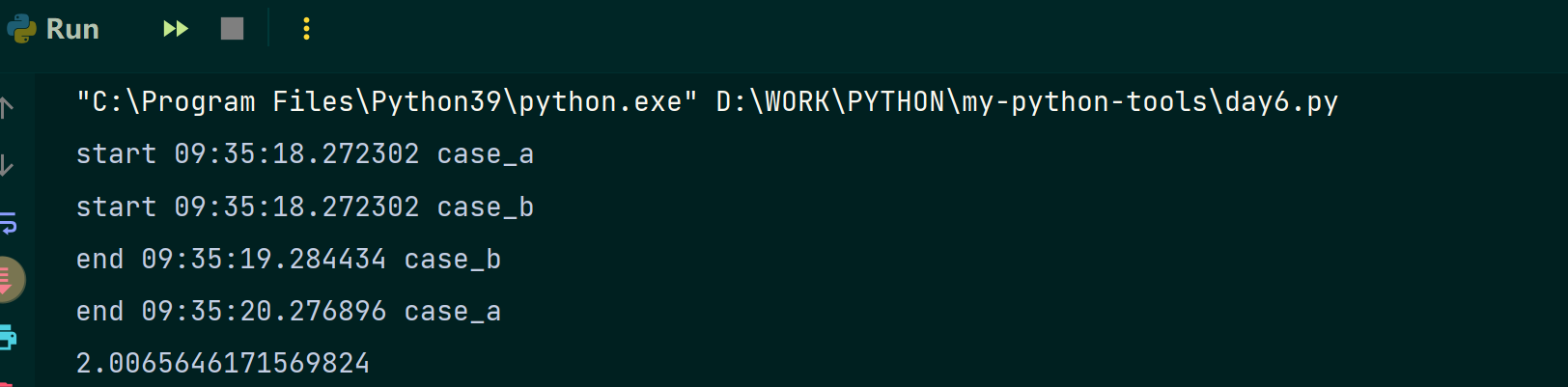
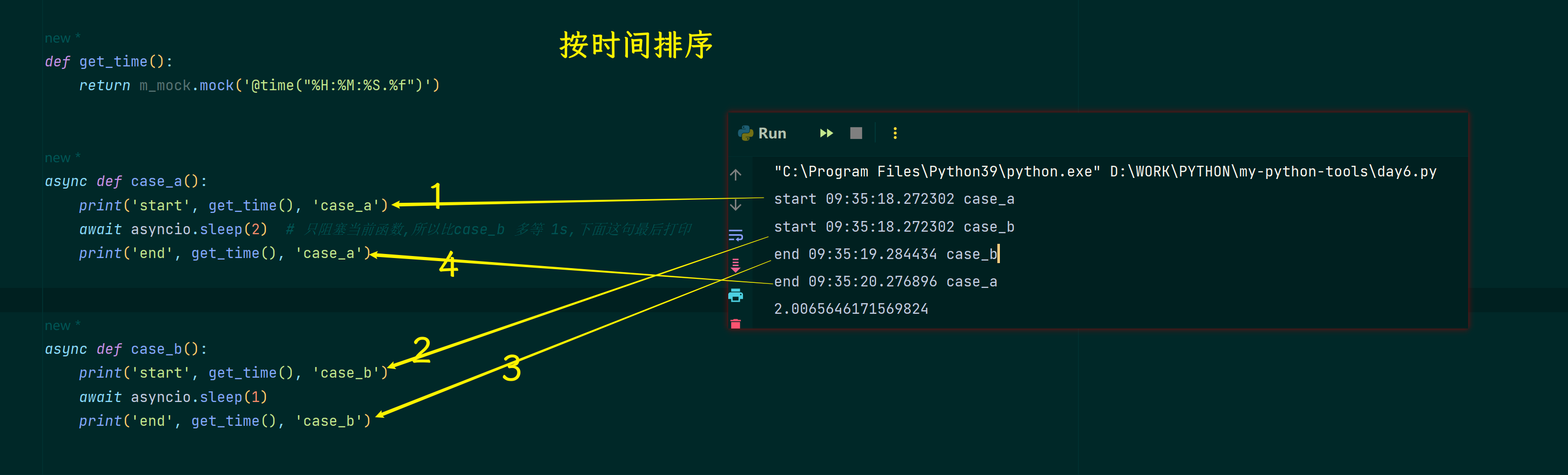
通过上述代码示例可以发现case_a和case_b通过async并行运行了,但是case_a由于使用await等待2s比case_b的结果晚一秒出现.
打印异步函数的返回值示例
import asyncio
import time
from x_mock.m_mock import m_mock
def get_time():
return m_mock.mock('@time("%H:%M:%S.%f")')
async def case_a():
print('start', get_time(), 'case_a')
await asyncio.sleep(2) # 只阻塞当前函数,所以比case_b 多等 1s,下面这句最后打印
print('end', get_time(), 'case_a')
return 'case_a'
async def case_b():
print('start', get_time(), 'case_b')
await asyncio.sleep(1)
print('end', get_time(), 'case_b')
return 'case_b'
async def main():
return await asyncio.gather(
case_a(),
case_b()
)
if __name__ == '__main__':
start = time.time()
# asyncio.run(main()) # 运行方式1,不建议,会有一些报错
# 运行方式2
loop = asyncio.get_event_loop()
print(loop.run_until_complete(main()))
print(time.time() - start)