首先要在pycharm中安装pymysql的包
查询版本号
import pymysql
# 连接MySQL数据库, 本机 用户名 密码 数据库名
db=pymysql.connect('localhost','root','123456','zuoye')
# 获取光标
cursor=db.cursor()
# 执行MySQL语句
cursor.execute('select version()')
# 获取下一个查询结果集
data=cursor.fetchone()
print('database version :%s'%data)
db.close()
创建表
import pymysql
# 连接MySQL数据库, 本机 用户名 密码 数据库名
db=pymysql.connect('localhost','root','123456','zuoye')
# 获取光标
cursor=db.cursor()
sql='''CREATE TABLE tan(
id INT ,
tan_name VARCHAR (10)
)
'''
cursor.execute(sql)
db.close()
插入数据
import pymysql
# 连接MySQL数据库, 本机 用户名 密码 数据库名
db=pymysql.connect('localhost','root','123456','zuoye')
# 获取光标
cursor=db.cursor()
sql='''
insert into tan VALUES (1,'xiaowang'),(2,'xiaozhang'),(3,'xiaotan')
'''
try:
cursor.execute(sql)
# 提交到数据库中去执行
db.commit()
except:
# 回滚(返回原来的状况)
db.rollback()
db.close()
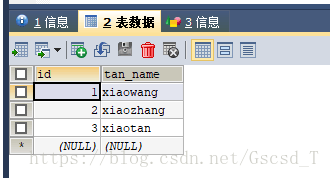
修改数据
import pymysql
# 连接MySQL数据库, 本机 用户名 密码 数据库名
db=pymysql.connect('localhost','root','123456','zuoye')
# 获取光标
cursor=db.cursor()
sql="update tan set tan_name='xiaoming' WHERE id=3"
try:
cursor.execute(sql)
db.commit()
except:
db.rollback()
db.close()
fetcharll: 返回得到的所有行
import pymysql
# 连接MySQL数据库, 本机 用户名 密码 数据库名
db=pymysql.connect('localhost','root','123456','zuoye')
# 获取光标
cursor=db.cursor()
sql="select * from tan WHERE id>1"
try:
cursor.execute(sql)
# fetchall:接收全部的返回结果行
results=cursor.fetchall()
print('results= ',results)
for row in results:
print('row= ',row)
id=row[0]
name=row[1]
print('id={0},name={1}'.format(id,name))
except:
print('ERROR:unble to fecth data')
db.close()
fetchone: 返回得到的第一行
import pymysql
# 连接MySQL数据库, 本机 用户名 密码 数据库名
db=pymysql.connect('localhost','root','123456','zuoye')
# 获取光标
cursor=db.cursor()
sql="select * from tan WHERE id>1"
try:
cursor.execute(sql)
# fetchall:返回第一个结果
results=cursor.fetchone()
print('results= ',results)
id=results[0]
name=results[1]
print('id={0},name={1}'.format(id,name))
except:
print('ERROR:unble to fecth data')
db.close()
rowcount :返回执行execute()方法后影响的行数
import pymysql
# 连接MySQL数据库, 本机 用户名 密码 数据库名
db=pymysql.connect('localhost','root','123456','zuoye')
# 获取光标
cursor=db.cursor()
sql="select * from tan WHERE id>1"
try:
cursor.execute(sql)
# rowcount:返回执行execute()方法后影响的行数
results=cursor.rowcount
print('影响了%d行'%results)
except:
print('ERROR:unble to fecth data')
db.close()
删除
import pymysql
# 连接MySQL数据库, 本机 用户名 密码 数据库名
db=pymysql.connect('localhost','root','123456','zuoye')
# 获取光标
cursor=db.cursor()
sql="delete from tan where id<2"
try:
cursor.execute(sql)
db.commit()
except:
db.rollback()
db.close()