一、将健康报告存入MQ延时队列
二、任务调度PowerJob
三、健康报告生成及推送
四、构建过慢
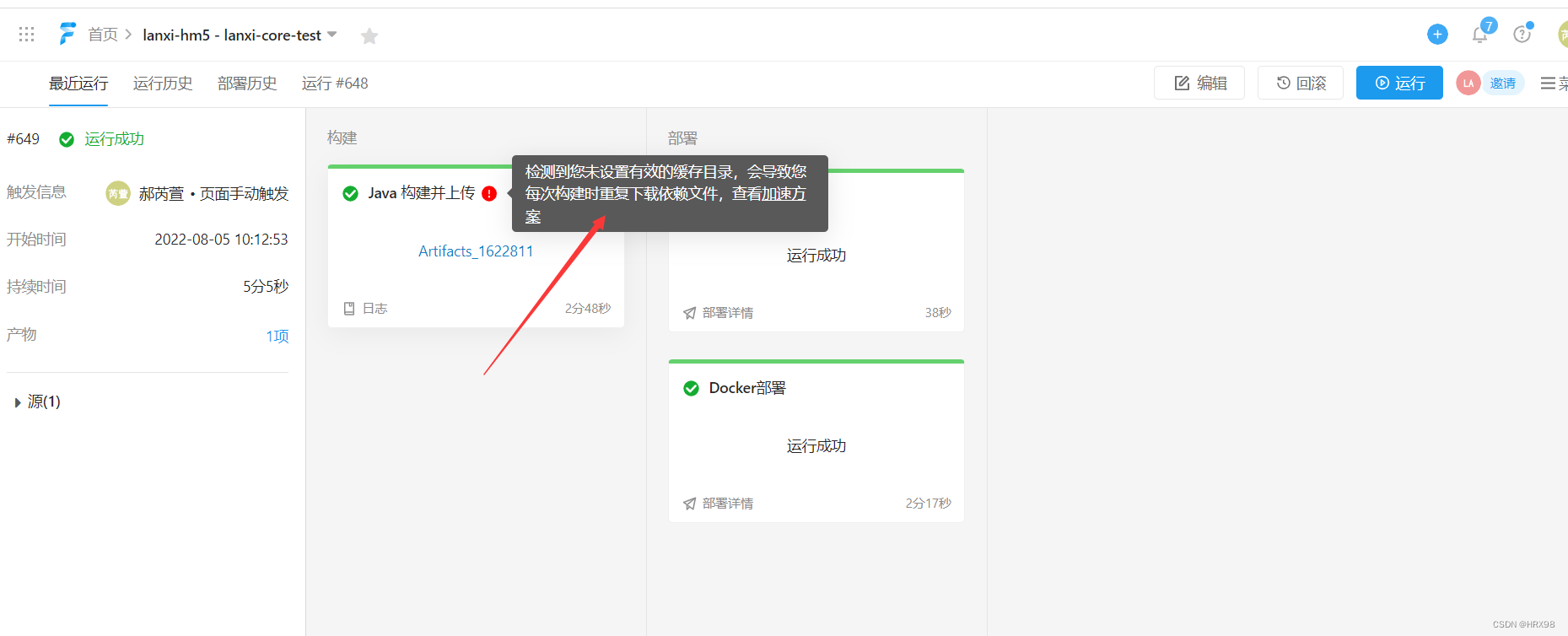
五、idea的core项目maven想deploy到本地失败
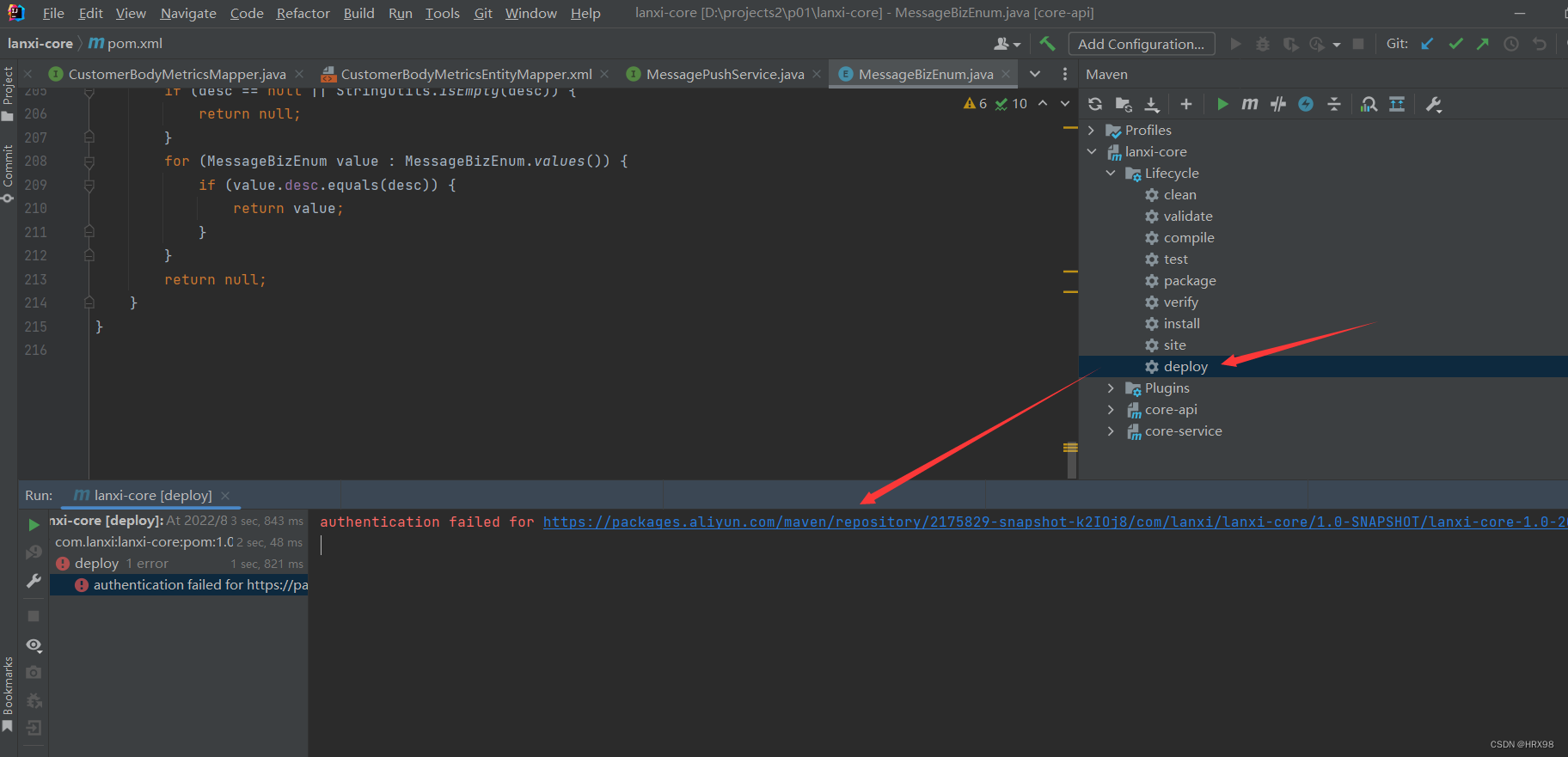
六、本地分支的代码报错,其他分支不报错?
七、代码
package com.lanxi.hm.util;
import com.alibaba.fastjson.JSON;
import com.alibaba.fastjson.JSONObject;
import com.baomidou.mybatisplus.core.conditions.query.LambdaQueryWrapper;
import com.lanxi.core.model.CustomerBodyMetrics;
import com.lanxi.core.model.MessageInfo;
import com.lanxi.core.model.MessageInfoWrapper;
import com.lanxi.core.model.enums.MessageBizEnum;
import com.lanxi.core.service.CustomerBodyMetricService;
import com.lanxi.core.service.MessagePushService;
import com.lanxi.hm.dao.hm.*;
import com.lanxi.hm.model.entity.hm.*;
import com.lanxi.hm.scheduler.powerjob.processor.HealthRecordProcessor;
import lombok.extern.slf4j.Slf4j;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Component;
import tech.powerjob.common.enums.ExecuteType;
import tech.powerjob.common.enums.ProcessorType;
import tech.powerjob.common.enums.TimeExpressionType;
import tech.powerjob.common.request.http.SaveJobInfoRequest;
import java.time.DayOfWeek;
import java.time.LocalDate;
import java.time.LocalDateTime;
import java.time.LocalTime;
import java.time.temporal.TemporalAdjusters;
import java.util.*;
import java.util.stream.Collectors;
@Slf4j
@Component
public class HealthRecordPushUtil {
private final String MSG_TITLE = "HEALTH_RECORD_PUSH_MSG_CENTER_TITLE";
@Autowired
private CustomerBodyMetricService customerBodyMetricService;
@Autowired
private CustomerPackageDao customerPackageDao;
@Autowired
private CustomerPackageDetailDao customerPackageDetailDao;
@Autowired
private HealthRecordDao healthRecordDao;
@Autowired
private CustomerDao customerDao;
@Autowired
private ServiceItemDao serviceItemDao;
@Autowired
private MessagePushService messagePushService;
public void createHealthRecords(){
List<CustomerEntity> customersAll = customerDao.list();
List<CustomerPackageEntity> customerPackageEntities = customerPackageDao.list().stream().collect(
Collectors.collectingAndThen(Collectors.toCollection(() -> new TreeSet<>(Comparator.comparing(o -> o.getCustomerId()))), ArrayList::new));
List<CustomerEntity> customers = new ArrayList<>();
for (CustomerPackageEntity cus : customerPackageEntities){
customers.add(customerDao.getById(cus.getCustomerId()));
}
customersAll.removeAll(customers);
List<CustomerEntity> customersB = new ArrayList<>();
List<CustomerBodyMetrics> customerBodyMatterList = customerBodyMetricService.listCount15();
for (CustomerBodyMetrics cbm : customerBodyMatterList){
CustomerEntity customer = customerDao.getById(cbm.getCustomerId());
customersB.add(customer);
}
customersB.retainAll(customersAll);
List<HealthRecordEntity> createDefaultList = new ArrayList<>();
for(CustomerEntity ce : customersB){
HealthRecordEntity healthRecordEntity = new HealthRecordEntity();
healthRecordEntity.setCustomerId(ce.getId());
healthRecordEntity.setTeamId(ce.getServiceTeamId());
healthRecordEntity.setPushCycle(1);
LocalDate now = LocalDate.now();
LocalDate lastMonth = now.minusMonths(1);
LocalDate firstDay = lastMonth.with(TemporalAdjusters.firstDayOfMonth());
LocalDate lastDay = lastMonth.with(TemporalAdjusters.lastDayOfMonth());
healthRecordEntity.setRecordTime(firstDay+" - "+lastDay);
healthRecordEntity.setHrType(0);
healthRecordEntity.setPushStatus(0);
healthRecordEntity.setTenantId(String.valueOf(ce.getTenantId()));
healthRecordDao.save(healthRecordEntity);
String type = "default";
this.sendToMsgCenter(healthRecordEntity.getTenantId(), MessageBizEnum.HEALTH_RECORD_PUSH, healthRecordEntity.getId(), healthRecordEntity.getCustomerId(), type);
}
for(CustomerEntity c : customers){
List<CustomerPackageDetailEntity> customerPackageDetailEntityList = new ArrayList<>();
LambdaQueryWrapper<CustomerPackageEntity> wrapper = new LambdaQueryWrapper<>();
wrapper.eq(CustomerPackageEntity::getCustomerId, c.getId());
List<CustomerPackageEntity> list = customerPackageDao.list(wrapper);
for (CustomerPackageEntity cp : list){
LambdaQueryWrapper<CustomerPackageDetailEntity> wrapper1 = new LambdaQueryWrapper<>();
wrapper1.eq(CustomerPackageDetailEntity::getCustomerPackageId, cp.getId());
customerPackageDetailEntityList = customerPackageDetailDao.list(wrapper1).stream().collect(
Collectors.collectingAndThen(Collectors.toCollection(() -> new TreeSet<>(Comparator.comparing(CustomerPackageDetailEntity::getServiceItemId))), ArrayList::new));
}
for (CustomerPackageDetailEntity cpd : customerPackageDetailEntityList){
HealthRecordEntity healthRecordEntity = new HealthRecordEntity();
healthRecordEntity.setCustomerId(c.getId());
healthRecordEntity.setTeamId(c.getServiceTeamId());
String params = serviceItemDao.getById(cpd.getServiceItemId()).getParams();
Map paramsMap = JSON.parseObject(params, Map.class);
String cycle = (String) paramsMap.get("cycle");
if ("week".equals(cycle)){
healthRecordEntity.setPushCycle(0);
LocalDate now = LocalDate.now();
LocalDate todayOfLastWeek = now.minusDays(7);
LocalDate monday = todayOfLastWeek.with(TemporalAdjusters.previous(DayOfWeek.SUNDAY)).plusDays(1);
LocalDate sunday = todayOfLastWeek.with(TemporalAdjusters.next(DayOfWeek.MONDAY)).minusDays(1);
healthRecordEntity.setRecordTime(monday+" - "+sunday);
} else if ("month".equals(cycle)) {
healthRecordEntity.setPushCycle(1);
LocalDate now = LocalDate.now();
LocalDate lastMonth = now.minusMonths(1);
LocalDate firstDay = lastMonth.with(TemporalAdjusters.firstDayOfMonth());
LocalDate lastDay = lastMonth.with(TemporalAdjusters.lastDayOfMonth());
healthRecordEntity.setRecordTime(firstDay+" - "+lastDay);
} else if ("quarter".equals(cycle)) {
healthRecordEntity.setPushCycle(2);
LocalDate now = LocalDate.now();
int monthValue = now.getMonthValue();
if (monthValue <= 3){
LocalDate lastYear = now.minusYears(1);
LocalDate firstDay = LocalDate.of(lastYear.getYear(), 10,1);
LocalDate lastDay = LocalDate.of(lastYear.getYear(), 12,31);
healthRecordEntity.setRecordTime(firstDay+" - "+lastDay);
} else if(monthValue <= 6){
LocalDate firstDay = LocalDate.of(now.getYear(), 1,1);
LocalDate lastDay = LocalDate.of(now.getYear(), 3,31);
healthRecordEntity.setRecordTime(firstDay+" - "+lastDay);
} else if(monthValue <= 9){
LocalDate firstDay = LocalDate.of(now.getYear(), 4,1);
LocalDate lastDay = LocalDate.of(now.getYear(), 6,30);
healthRecordEntity.setRecordTime(firstDay+" - "+lastDay);
} else {
LocalDate firstDay = LocalDate.of(now.getYear(), 7,1);
LocalDate lastDay = LocalDate.of(now.getYear(), 9,30);
healthRecordEntity.setRecordTime(firstDay+" - "+lastDay);
}
} else if ("year".equals(cycle)) {
healthRecordEntity.setPushCycle(3);
LocalDate now = LocalDate.now();
LocalDate lastYear = now.minusYears(1);
LocalDate firstDay = lastYear.with(TemporalAdjusters.firstDayOfYear());
LocalDate lastDay = lastYear.with(TemporalAdjusters.lastDayOfYear());
healthRecordEntity.setRecordTime(firstDay+" - "+lastDay);
}
healthRecordEntity.setHrType(0);
healthRecordEntity.setPushStatus(0);
healthRecordDao.save(healthRecordEntity);
}
}
}
public SaveJobInfoRequest getRequest(String jobName, String cron) {
SaveJobInfoRequest request = new SaveJobInfoRequest();
request.setJobName(jobName);
request.setProcessorType(ProcessorType.BUILT_IN);
request.setExecuteType(ExecuteType.STANDALONE);
request.setProcessorInfo(HealthRecordProcessor.class.getName());
request.setTimeExpressionType(TimeExpressionType.CRON);
request.setTimeExpression(cron);
request.setEnable(true);
return request;
}
public String getCron(String params) {
Map paramsMap = JSON.parseObject(params, Map.class);
String cycle = (String) paramsMap.get("cycle");
String type = (String) paramsMap.get("type");
if ("default".equals(type)){
return "0 0 0 1 * ?";
} else if ("package".equals(type)) {
if ("week".equals(cycle)){
return "0 0 0 ? * MON";
} else if ("month".equals(cycle)) {
return "0 0 0 1 * ?";
} else if ("quarter".equals(cycle)) {
return "0 0 0 1 4,7,10,1 ?";
} else if ("year".equals(cycle)) {
return "0 0 0 1 1 ? *";
}
}
return "0 0 8 * * ?";
}
public void sendToMsgCenter(String tenantId, MessageBizEnum messageBizEnum, Integer healthRecordId, String customerId, String type) {
String title = "";
String content = "";
JSONObject relateData = new JSONObject();
relateData.put("healthRecordId", healthRecordId);
MessageInfoWrapper messageInfoWrapper = new MessageInfoWrapper();
MessageInfo messageInfo = new MessageInfo();
title = "健康报告";
messageInfo.setTitle(title);
messageInfo.setContent(content);
messageInfo.setTargetPerson(customerId);
messageInfo.setRelateData(relateData);
messageInfo.setCreater("system");
messageInfoWrapper.setMessageInfo(messageInfo);
LocalDateTime dateTime = LocalDateTime.now();
if ("default".equals(type)){
dateTime = LocalDateTime.of(LocalDate.now(), LocalTime.of(8,0,0));
messagePushService.sendToMessageCenter(tenantId,messageBizEnum,messageInfoWrapper, dateTime);
} else if ("package".equals(type)){
messagePushService.sendMessageCenterToCustomer(tenantId,messageBizEnum,messageInfoWrapper, dateTime);
}
}
}