div四效果实现
需求如下
- 鼠标点击进行移动
- 拖拽四条边实现div大小变化
- 拖拽四个角实现div大小变化
- 按住shift键只能拖拽鼠标变化量大的方向
效果图
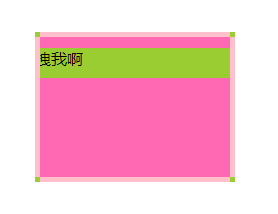
创建一个div 设置样式
<div><p>拽我啊</p></div>
<script>
var div = document.querySelector('div')
var p = document.querySelector('div p')
// 基本样式
div.style.width = '200px'
div.style.height = '150px'
div.style.background = 'hotpink'
div.style.position = 'absolute'
div.style.top = 0
div.style.left = 0
p.style.cursor = 'move'
p.style.height = '30px'
p.style.width = '100%'
p.style.background = 'yellowgreen'
p.style.userSelect = 'none'
// 移动效果 shift键按下效果
function move() {
p.onmousedown = function (evt) {
var e = evt || window.event
// 记录鼠标位置和盒子当前位置
var mouseX = e.clientX
var mouseY = e.clientY
var divT = div.offsetTop
var divL = div.offsetLeft
window.onmousemove = function (evt) {
var e = evt || window.event
// 计算差值
var X = e.clientX
var Y = e.clientY
var chaX = X - mouseX
var chaY = Y - mouseY
div.style.top = divT + chaY + 'px'
div.style.left = divL + chaX + 'px'
// shift按键效果
if (evt.shiftKey && chaX > chaY) {
div.style.top = divT + 'px'
div.style.left = divL + chaX + 'px'
}
if (evt.shiftKey && chaX < chaY) {
div.style.left = divL + 'px'
div.style.top = divT + chaY + 'px'
}
}
window.onmouseup = function (evt) {
var e = evt || window.event
window.onmousemove = null
window.onmouseup = null
}
}
}
move()
// 四条边基本样式
function border(evt) {
var div = document.querySelector('div')
var borT = document.createElement('div')
borT.style.position = 'absolute'
borT.style.top = 0
borT.style.left = 0
borT.style.background = 'pink'
borT.style.width = '100%'
borT.style.height = '5px'
borT.style.cursor = 'ns-resize'
var borB = document.createElement('div')
borB.style.position = 'absolute'
borB.style.bottom = 0
borB.style.left = 0
borB.style.background = 'pink'
borB.style.width = '100%'
borB.style.height = '5px'
borB.style.cursor = 'ns-resize'
var borL = document.createElement('div')
borL.style.position = 'absolute'
borL.style.top = 0
borL.style.left = 0
borL.style.background = 'pink'
borL.style.width = '5px'
borL.style.height = '100%'
borL.style.cursor = 'ew-resize'
var borR = document.createElement('div')
borR.style.position = 'absolute'
borR.style.top = 0
borR.style.right = 0
borR.style.background = 'pink'
borR.style.width = '5px'
borR.style.height = '100%'
borR.style.cursor = 'ew-resize'
borT.className = 'top'
borB.className = 'bottom'
borL.className = 'left'
borR.className = 'right'
div.appendChild(borT)
div.appendChild(borB)
div.appendChild(borL)
div.appendChild(borR)
}
border()
// 四条边拖拽效果
function zhuaiB() {
var div = document.querySelector('div')
var left = document.querySelector('.left')
var right = document.querySelector('.right')
var top = document.querySelector('.top')
var bottom = document.querySelector('.bottom')
// left边
left.onmousedown = function (evt) {
var leftL = div.offsetLeft
var leftW = div.offsetWidth
var mouseX = evt.clientX
var mouseY = evt.clientY
window.onmousemove = function (evt) {
var x = evt.clientX
var y = evt.clientY
var chaX = x - mouseX
div.style.left = leftL + chaX + 'px'
div.style.width = leftW - chaX + 'px'
window.onmouseup = function (evt) {
window.onmousemove = null
window.onmouseup = null
}
}
}
// right边
right.onmousedown = function (evt) {
var rightL = div.offsetLeft
var rightW = div.offsetWidth
var mouseX = evt.clientX
var mouseY = evt.clientY
window.onmousemove = function (evt) {
var x = evt.clientX
var y = evt.clientY
var chaX = x - mouseX
div.style.width = rightW + chaX + 'px'
window.onmouseup = function (evt) {
window.onmousemove = null
window.onmouseup = null
}
}
}
// top 边
top.onmousedown = function (evt) {
var topT = div.offsetTop
var topH = div.offsetHeight
var mouseX = evt.clientX
var mouseY = evt.clientY
window.onmousemove = function (evt) {
var x = evt.clientX
var y = evt.clientY
var chaY = y - mouseY
div.style.top = topT + chaY + 'px'
div.style.height = topH - chaY + 'px'
window.onmouseup = function (evt) {
window.onmousemove = null
window.onmouseup = null
}
}
}
// bottom边
bottom.onmousedown = function (evt) {
var bottomT = div.offsetTop
var bottomH = div.offsetHeight
var mouseX = evt.clientX
var mouseY = evt.clientY
window.onmousemove = function (evt) {
var x = evt.clientX
var y = evt.clientY
var chaY = y - mouseY
div.style.height = bottomH + chaY + 'px'
window.onmouseup = function (evt) {
window.onmousemove = null
window.onmouseup = null
}
}
}
}
zhuaiB()
// 四个角基本样式
function corner() {
var div = document.querySelector('div')
var left_top = document.createElement('div')
left_top.style.position = 'absolute'
left_top.style.top = '0'
left_top.style.left = '0'
left_top.style.width = '5px'
left_top.style.height = '5px'
left_top.style.background = 'yellowgreen'
left_top.style.cursor = 'nwse-resize'
var right_top = document.createElement('div')
right_top.style.position = 'absolute'
right_top.style.top = '0'
right_top.style.right = '0'
right_top.style.width = '5px'
right_top.style.height = '5px'
right_top.style.background = 'yellowgreen'
right_top.style.cursor = 'nesw-resize'
var left_bottom = document.createElement('div')
left_bottom.style.position = 'absolute'
left_bottom.style.bottom = '0'
left_bottom.style.left = '0'
left_bottom.style.width = '5px'
left_bottom.style.height = '5px'
left_bottom.style.background = 'yellowgreen'
left_bottom.style.cursor = 'nesw-resize'
var right_bottom = document.createElement('div')
right_bottom.style.position = 'absolute'
right_bottom.style.bottom = '0'
right_bottom.style.right = '0'
right_bottom.style.width = '5px'
right_bottom.style.height = '5px'
right_bottom.style.background = 'yellowgreen'
right_bottom.style.cursor = 'nwse-resize'
div.appendChild(left_top)
div.appendChild(right_top)
div.appendChild(left_bottom)
div.appendChild(right_bottom)
}
corner()
// 四个角拖拽效果
function zhuaiC() {
// 和四条边一样,不再赘述
}
zhuaiC()
</script>