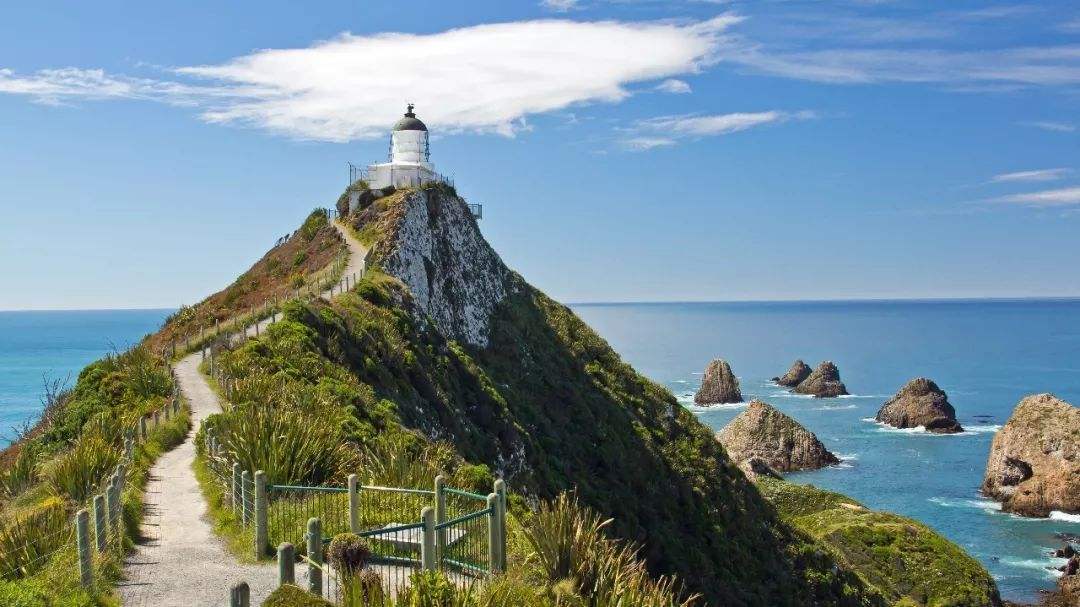
在最近的需求开发中,涉及到利用Python划分季度,值得注意的是Demand和Supply划分季度有所区别
季度划分逻辑
将上述表格进行整理大致可划分如下
注意:上述逻辑提出的日期大致在6月中旬,现在日期是2022年8月20日, 也就是Demand以及Supply已经过了Q2季度,由于客户要求只需要保留三个季度参与计算,因此编写的代码逻辑需要考虑根据最新本地日期自动更新划分季度,以(今天)2022年8月20日划分季度呈现结果如下所示
思路:
1.编写出Demand的Q1、Q2、Q3以及Q4的Cut off函数
import datetime
def deal_new_time(new_time):
"""
时间格式字符串转换函数
举例:2022/09/05 => 2022/9/5
"""
deal_new_time = new_time.split('/')
year = deal_new_time[0]
month = deal_new_time[1]
month = str(int(month))
day = deal_new_time[2]
day = str(int(day))
str_list = [year, month, day]
res = '/'.join(str_list)
return res
def Demand_Time(mon):
"""
可理解为获取4月、7月、10月、1月的第二个星期一的具体日期
举例:2023/4/10
Demand:
Q1 Cut off: 2nd week of Apri
Q2 Cut off: 2nd week of July
Q3 Cut off: 2nd week of Oct
Q4 Cut off: 2nd week of Jan
"""
import calendar
c = calendar.Calendar(firstweekday=calendar.SUNDAY)
today = datetime.datetime.today()
# 获取当前年份
current_year = today.year
# 获取当前月份
current_month = today.month
month = mon
if current_month >= mon + 3:
year = current_year + 1
else:
year = current_year
if current_month in(1,2,3) and month == 1:
year = year + 1
monthcal = c.monthdatescalendar(year,month)
second_monday = [day for week in monthcal for day in week if \
day.weekday() == calendar.MONDAY and \
day.month == month][1]
new_time = datetime.datetime.strftime(second_monday, "%Y/%m/%d")
second_monday_res = deal_new_time(new_time)
return second_monday_res
# 2023/4/10
q1_demand_time = Demand_Time(4)
# 2022/7/11
q2_demand_time = Demand_Time(7)
# 2022/10/10
q3_demand_time = Demand_Time(10)
# 2023/1/9
q4_demand_time = Demand_Time(1)
2.编写出Supply的Q1、Q2、Q3以及Q4的Cut off函数
import datetime
def deal_new_time(new_time):
"""
时间格式字符串转换函数
举例:2022/09/05 => 2022/9/5
"""
deal_new_time = new_time.split('/')
year = deal_new_time[0]
month = deal_new_time[1]
month = str(int(month))
day = deal_new_time[2]
day = str(int(day))
str_list = [year, month, day]
res = '/'.join(str_list)
return res
def Supply_Time(mon):
"""
可理解为获取3月、6月、9月、12月的第三个星期一的具体日期
举例:2023/3/21
Demand:
Q1 Cut off: 3rd week of March
Q2 Cut off: 3rd week of June
Q3 Cut off: 3rd week of Sep
Q4 Cut off: 3rd week of Dec
"""
import calendar
c = calendar.Calendar(firstweekday=calendar.SUNDAY)
today = datetime.datetime.today()
# 获取当前年份
current_year = today.year
# 获取当前月份
current_month = today.month
month = mon
if current_month >= mon + 4:
year = current_year + 1
else:
year = current_year
if current_month in(1,2) and month == 1:
year = year + 1
monthcal = c.monthdatescalendar(year,month)
third_monday = [day for week in monthcal for day in week if \
day.weekday() == calendar.MONDAY and \
day.month == month][2]
new_time = datetime.datetime.strftime(third_monday, "%Y/%m/%d")
third_monday_res = deal_new_time(new_time)
return third_monday_res
# 2023/3/20
q1_supply_time = Supply_Time(3)
# 2022/6/20
q2_supply_time = Supply_Time(6)
# 2022/9/19
q3_supply_time = Supply_Time(9)
# 2022/12/19
q4_supply_time = Supply_Time(12)
3.划分季度区间(左闭右开)
Demand划分季度逻辑
将所得到的q1_demand_time、q2_demand_time、q3_demand_time、q4_demand_time放入到列表list中,并进行排序
q1_demand_time = '2023/4/10'
q2_demand_time = '2022/7/11'
q3_demand_time = '2022/10/10'
q4_demand_time = '2023/1/9'
list_time = [q1_demand_time,q2_demand_time,q3_demand_time,q4_demand_time]
import datetime
# 将日期转化为便于比较大小时间戳
def get_list(date):
return datetime.datetime.strptime(date,"%Y/%m/%d").timestamp()
list_time_sort = sorted(list_time,key=lambda date:get_list(date))
# 左闭右开
quarter1_demand = list_time_sort[0:1]
quarter2_demand = list_time_sort[1:2]
quarter3_demand = list_time_sort[2:3]
同理Supply划分季度逻辑如下:
q1_supply_time = '2023/3/20'
q2_supply_time = '2022/6/20'
q3_supply_time = '2022/9/19'
q4_supply_time = '2022/12/19'
list_time = [q1_supply_time,q2_supply_time,q3_supply_time,q4_supply_time]
import datetime
# 将日期转化为便于比较大小时间戳
def get_list(date):
return datetime.datetime.strptime(date,"%Y/%m/%d").timestamp()
list_time_sort = sorted(list_time,key=lambda date:get_list(date))
# 左闭右开
quarter1_supply = list_time_sort[0:1]
quarter2_supply = list_time_sort[1:2]
quarter3_supply = list_time_sort[2:3]
补充阅读资料
Python获取每月特定日期https://blog.csdn.net/Hudas/article/details/125322063Python对列表日期元素进行排序
https://blog.csdn.net/Hudas/article/details/126489119?spm=1001.2014.3001.5502