Swagger是一款可以用于设计、构建、文档并且执行API的框架。使用框架,可以轻松的创建一个API文档。使用Swagger有利于前后端分离开发,并且在测试的时候不需要在使用浏览器输入URL的方式访问Controller,可以直接在页面输入参数,然后单击按钮来访问。而且传统的输入URL测试方式对于post请求的传参比较麻烦。
- 如何使用Swagger
- 搭建项目框架
pom.xml文件,该文件完整内容如下
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/maven-v4_0_0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.microservice</groupId>
<artifactId>firstboot</artifactId>
<packaging>war</packaging>
<version>0.0.1-SNAPSHOT</version>
<name>firstboot Maven Webapp</name>
<url>http://maven.apache.org</url>
<!-- 引入spring boot的依赖 -->
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>1.4.3.RELEASE</version>
</parent>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<java.version>1.8</java.version>
<springfox.version>2.5.0</springfox.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>io.springfox</groupId>
<artifactId>springfox-swagger2</artifactId>
<version>${springfox.version}</version>
</dependency>
<dependency>
<groupId>io.springfox</groupId>
<artifactId>springfox-swagger-ui</artifactId>
<version>${springfox.version}</version>
</dependency>
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<version>1.16.8</version>
</dependency>
<dependency>
<groupId>org.mybatis.generator</groupId>
<artifactId>mybatis-generator-core</artifactId>
<version>1.3.2</version>
</dependency>
</dependencies>
<!-- 添加spring-boot的maven插件 -->
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-compiler-plugin</artifactId>
<version>3.1</version>
<configuration>
<source>1.8</source>
<target>1.8</target>
<encoding>UTF-8</encoding>
</configuration>
</plugin>
</plugins>
</build>
</project>
要使用Swagger,首先要引入两个依赖:springfox-swagger2和springfox-swagger-ui。除了必须的两个依赖外,还引入了lombok的依赖。lombok是一个主要用来消除pojo模板试代码(例如getter、setter等)的框架,当然也可以做一些其他事情。需要注意的是,无论是Eclipse还是IDEA想使用lombok,都要安装lombok插件。
在创建好pom.xml文件之后,开始创建主类,主类Application代码如下:
package com.microservice.firstboot;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import springfox.documentation.swagger2.annotations.EnableSwagger2;
@SpringBootApplication
@EnableSwagger2 //启动 Swagger
public class Application {
public static void main(String[] args){
SpringApplication.run(Application.class, args);
}
}
在该类中除了@SpringBootApplication注解外,还添加了@EnableSwagger2注解,启动Swagger
为了全面的看到Swagger的各种使用方式,这里创建一个模型类com.microservice.firstboot.model.User
代码如下:
package com.microservice.firstboot.model;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import lombok.AllArgsConstructor;
import lombok.Getter;
@ApiModel("用户模型")
@AllArgsConstructor
@Getter
public class User {
@ApiModelProperty("用户ID")
private int id;
@ApiModelProperty("用户姓名")
private String name;
@ApiModelProperty("用户密码")
private String password;
}
在该模型中使用了4个注解,分别如下:
- @Getter:是一个Lombok注解,用来为pojo类生成getter方法。如果不添加该注解,我们需要为pojo类生成getter方法,如果一个模型中属性较多,代码就不够简洁。
- @AllArgsConstructor:是一个Lombok注解,用来为pojo生成全参构造器
- @ApiModel:是一个Swagger注解,用来为pojo类做注释
- @ApiModelProperty:是一个Swagger注解,用来为pojo类中属性做注释
核心代码:
package com.microservice.firstboot.controller;
import com.microservice.firstboot.model.User;
import com.microservice.firstboot.model.Address;
import io.swagger.annotations.*;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RestController;
@Api("User 相关api")
@RestController
@RequestMapping("/user")
public class FirstSwaggerController {
@ApiOperation("根据ID获得用户信息")
@ApiImplicitParams({
@ApiImplicitParam(paramType="query",name="id",dataType="int",required=true,value="用户的id",defaultValue="1")
})
@ApiResponses({
@ApiResponse(code=400,message="请求参数没填好"),
@ApiResponse(code=404,message="请求路径没有或页面跳转路径不对")
})
@RequestMapping(value="/getUserInfo",method=RequestMethod.GET)
public User getUserInfo(@RequestParam("id")int id){
if(id==1){
return new User(1,"小红","123456");
}
return new User(2,"小刚","123456");
}
@ApiOperation("根据ID获得用户住址")
@ApiImplicitParams({
@ApiImplicitParam(paramType="query",name="id",dataType="int",required=true,value="用户的id",defaultValue="1")
})
@ApiResponses({
@ApiResponse(code=400,message="请求参数没填好"),
@ApiResponse(code=404,message="请求路径没有或页面跳转路径不对")
})
@RequestMapping(value="/getUserAddress",method=RequestMethod.GET)
public Address getUserAddress(@RequestParam("id")int id){
if(id==1){
return Address.builder().province("福建省").city("福州市").country("鼓楼区").build();//new User(1,"小红","123456");
}
return Address.builder().province("浙江省").city("杭州市").country("余杭区").build();
}
}
在该controller中,提供了一个简单的接口:根据id获取User。
- @Api:通常用来为一个controller类做注释
- @ApiOperation:通常用来为接口做注释,说明该接口的职能
- @ApiImplicitParams:用来包含接口的一组参数注解,可以将其简单的理解为参数注解的集合
- @ApiImplicitParam:用在@ApiImplicitParams注解中,说明一个请求参数的各个方面。该注解常用选项如下:
paramType,参数放置的位置,包含query、header、path、body、及form,最常用的是前4个。需要注意的是,query域中的值需要使用@RequestParam获取,path域中的值需要使用@pathVariable获取,body域中的值需要使用@RequestBody获取,否则可能出错。
name,参数名
dataType,参数类型
required,参数是否必须传
value ,参数的值
defaultValue ,参数的默认值
@ApiResponses:通常用来包含接口的一组响应注解,可以将其简单的理解为响应注解的集合
-
@ApiResponse:用在@ApiResponses中,一般用来表达一个错误的响应信息
- code http中code数字,例如400
- message 信息,例如请求参数没填好
这些注解就是开发中常用的注解:到此Spring Boot与Swagger的集成就完成了。
测试分析Swagger生成的API文档:
启动项目后,浏览器输入 http://localhost:8080/swagger-ui.html#/
使用Swagger进行接口调用如图
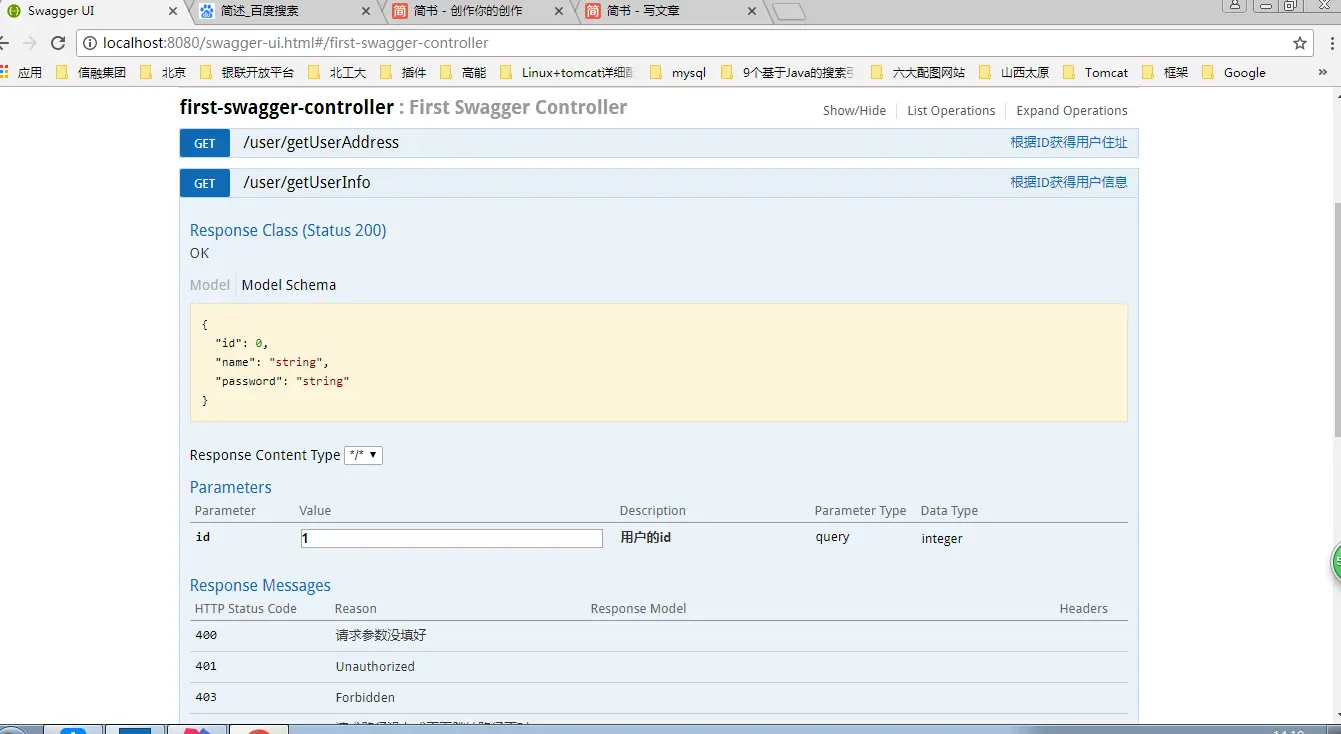
点个关注呗,我负责写,你负责看。
- 邮箱:ithelei@sina.cn
- 技术讨论群:687856230
- GoodLuck