- 使用window.onhashchange实现简单的路由
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<script src='js/vue.min.js'></script>
</head>
<body>
<div id="app">
<a href="#/zhuye">主页</a>
<a href="#/keji">科技</a>
<a href="#/caijing">财经</a>
<a href="#/yule">娱乐</a>
<component :is="comNm"></component>
</div>
</body>
<script>
const zhuye = {
template: '<h1>主页信息</h1>'
}
const keji = {
template: '<h1>科技信息</h1>'
}
const caijing = {
template: '<h1>财经信息</h1>'
}
const yule = {
template: '<h1>娱乐信息</h1>'
}
const vm = new Vue({
el: '#app',
data: {
comNm: 'zhuye'
},
components: {
zhuye,
keji,
caijing,
yule
}
})
window.onhashchange = function() {
console.log(location.hash)
switch (location.hash.slice(1)) {
case '/zhuye':
vm.comNm = 'zhuye';
break;
case '/keji':
vm.comNm = 'keji';
break;
case '/caijing':
vm.comNm = 'caijing';
break;
case '/yule':
vm.comNm = 'yule';
break;
}
}
</script>
</html>
- Vue Router 路由
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<script src='js/vue.min.js'></script>
<script src="js/vue-router_3.0.2.js"></script>
</head>
<body>
<div id="app">
<router-link to="/zhuye">主页</router-link>
<router-link to="/keji">科技</router-link>
<router-link to="/caijing">财经</router-link>
<router-link to="/yule">娱乐</router-link>
<router-view></router-view>
</div>
</body>
<script>
const zhuye = {
template: '<h1>主页信息</h1>'
}
const keji = {
template: '<h1>科技信息</h1>'
}
const caijing = {
template: '<h1>财经信息</h1>'
}
const yule = {
template: '<h1>娱乐信息</h1>'
}
var router = new VueRouter({
routes: [{
path: "/",
redirect: '/keji'
}, {
path: '/zhuye',
component: zhuye
}, {
path: '/keji',
component: keji
}, {
path: '/caijing',
component: caijing
}, {
path: '/yule',
component: yule
}]
})
const vm = new Vue({
el: '#app',
router
})
</script>
</html>
- Vue Router 动态匹配
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<script src='js/vue.min.js'></script>
<script src="js/vue-router_3.0.2.js"></script>
</head>
<body>
<div id="app">
<router-link to="/zhuye/1">主页</router-link>
<router-link to="/zhuye/2">主页</router-link>
<router-link to="/zhuye/3">主页</router-link>
<router-link to="/zhuye/4">主页</router-link>
<router-link to="/keji">科技</router-link>
<router-link to="/caijing">财经</router-link>
<router-link to="/yule">娱乐</router-link>
<router-view></router-view>
</div>
</body>
<script>
const zhuye = {
template: '<h1>主页信息{{$route.params.id}}</h1>'
}
const keji = {
template: '<h1>科技信息</h1>'
}
const caijing = {
template: '<h1>财经信息</h1>'
}
const yule = {
template: '<h1>娱乐信息</h1>'
}
var router = new VueRouter({
routes: [{
path: "/",
redirect: '/keji'
}, {
path: '/zhuye/:id',
component: zhuye
}, {
path: '/keji',
component: keji
}, {
path: '/caijing',
component: caijing
}, {
path: '/yule',
component: yule
}]
})
const vm = new Vue({
el: '#app',
router
})
</script>
</html>
- VueRouter 嵌套
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<script src='js/vue.min.js'></script>
<script src="js/vue-router_3.0.2.js"></script>
</head>
<body>
<div id="app">
<router-link to="/zhuye">主页</router-link>
<router-link to="/keji">科技</router-link>
<router-link to="/caijing">财经</router-link>
<router-link to="/yule">娱乐</router-link>
<router-view></router-view>
</div>
</body>
<script>
const zhuye = {
template: '<h1>主页信息</h1>'
}
const keji = {
template: '<h1>科技信息</h1>'
}
const caijing = {
template: '<h1>财经信息</h1>'
}
const yule = {
template: `<div>
<h1>娱乐信息</h1>
<hr>
<router-link to="/yule/ldh">刘德华</router-link>
<router-link to="/yule/zxy">张学友</router-link>
<router-view/>
</div>
`
}
const ldh = {
template: '<h1>刘德华信息</h1>'
}
const zxy = {
template: '<h1>张学友信息</h1>'
}
var router = new VueRouter({
routes: [{
path: "/",
redirect: '/keji'
}, {
path: '/zhuye',
component: zhuye
}, {
path: '/keji',
component: keji
}, {
path: '/caijing',
component: caijing
}, {
path: '/yule',
component: yule,
children: [{
path: '/yule/ldh',
component: ldh
}, {
path: '/yule/zxy',
component: zxy
}]
}]
})
const vm = new Vue({
el: '#app',
router
})
</script>
</html>
- Vue Router 动态传参props
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<script src='js/vue.min.js'></script>
<script src="js/vue-router_3.0.2.js"></script>
</head>
<body>
<div id="app">
<router-link to="/zhuye/1">主页</router-link>
<router-link to="/zhuye/2">主页</router-link>
<router-link to="/zhuye/3">主页</router-link>
<router-link to="/zhuye/4">主页</router-link>
<router-link to="/keji">科技</router-link>
<router-link to="/caijing">财经</router-link>
<router-link to="/yule">娱乐</router-link>
<router-view></router-view>
</div>
</body>
<script>
const zhuye = {
props: ["id"],
template: '<h1>主页信息---{{id}}</h1>'
}
const keji = {
template: '<h1>科技信息</h1>'
}
const caijing = {
template: '<h1>财经信息</h1>'
}
const yule = {
template: '<h1>娱乐信息</h1>'
}
var router = new VueRouter({
routes: [{
path: "/",
redirect: '/keji'
}, {
path: '/zhuye/:id',
component: zhuye,
props: true
}, {
path: '/keji',
component: keji
}, {
path: '/caijing',
component: caijing
}, {
path: '/yule',
component: yule
}]
})
const vm = new Vue({
el: '#app',
router
})
</script>
</html>
- Vue Router 对象式传参
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<script src='js/vue.min.js'></script>
<script src="js/vue-router_3.0.2.js"></script>
</head>
<body>
<div id="app">
<router-link to="/zhuye/1">主页</router-link>
<router-link to="/zhuye/2">主页</router-link>
<router-link to="/zhuye/3">主页</router-link>
<router-link :to="{name:'zhuye',params:{id:3}}">主页</router-link>
<router-link to="/keji">科技</router-link>
<router-link to="/caijing">财经</router-link>
<router-link to="/yule">娱乐</router-link>
<router-view></router-view>
</div>
</body>
<script>
const zhuye = {
props: ["uname", 'id'],
template: '<h1>主页信息---{{uname}}---{{id}}</h1>'
}
const keji = {
template: '<h1>科技信息</h1>'
}
const caijing = {
template: '<h1>财经信息</h1>'
}
const yule = {
template: '<h1>娱乐信息</h1>'
}
var router = new VueRouter({
routes: [{
path: "/",
redirect: '/keji'
}, {
name: 'zhuye',
path: '/zhuye/:id',
component: zhuye,
props: route => ({
uname: 'kathy',
age: 30,
id: route.params.id
})
}, {
path: '/keji',
component: keji
}, {
path: '/caijing',
component: caijing
}, {
path: '/yule',
component: yule
}]
})
const vm = new Vue({
el: '#app',
router
})
</script>
</html>
- 编程式导航
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<script src='js/vue.min.js'></script>
<script src="js/vue-router_3.0.2.js"></script>
</head>
<body>
<div id="app">
<router-link to="/zhuye/1">主页</router-link>
<router-link to="/zhuye/2">主页</router-link>
<router-link to="/zhuye/3">主页</router-link>
<router-link :to="{name:'zhuye',params:{id:3}}">主页</router-link>
<router-link to="/keji">科技</router-link>
<router-link to="/caijing">财经</router-link>
<router-link to="/yule">娱乐</router-link>
<router-view></router-view>
</div>
</body>
<script>
const zhuye = {
props: ["uname", 'id'],
template: `<div>
<h1>主页信息---{{uname}}---{{id}}</h1>
<button @click="goRegister">跳转到娱乐页面</button>
</div>`,
methods: {
goRegister: function() {
this.$router.push('/yule');
}
}
}
const keji = {
template: '<h1>科技信息</h1>'
}
const caijing = {
template: '<h1>财经信息</h1>'
}
const yule = {
template: `<div>
<h1>娱乐信息</h1>
<button @click="goBack">后退</button>
</div>`,
methods: {
goBack: function() {
this.$router.go(-1);
}
}
}
var router = new VueRouter({
routes: [{
path: "/",
redirect: '/keji'
}, {
name: 'zhuye',
path: '/zhuye/:id',
component: zhuye,
props: route => ({
uname: 'kathy',
age: 30,
id: route.params.id
})
}, {
path: '/keji',
component: keji
}, {
path: '/caijing',
component: caijing
}, {
path: '/yule',
component: yule
}]
})
const vm = new Vue({
el: '#app',
router
})
</script>
</html>
- 综合案例
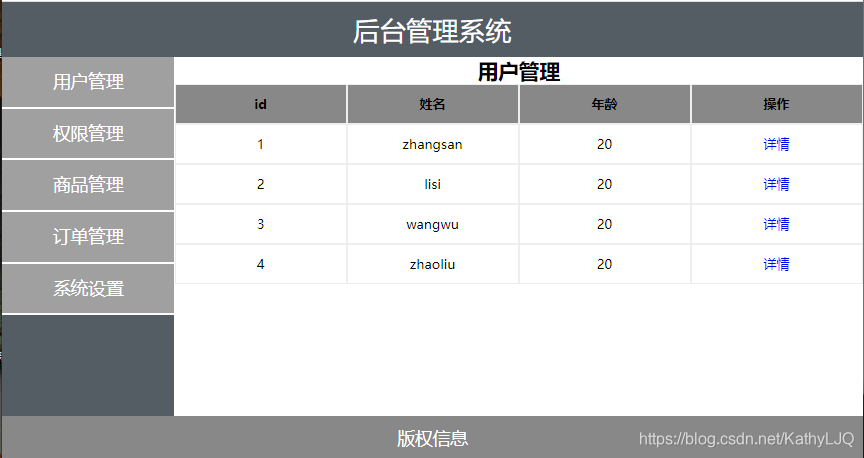
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<script src='js/vue.min.js'></script>
<script src="js/vue-router_3.0.2.js"></script>
<style>
* {
margin: 0;
padding: 0;
}
body {
width: 100%;
height: 100%;
}
header {
height: 50px;
background-color: #545c64;
color: #fff;
line-height: 50px;
text-align: center;
font-size: 24px;
}
.main {
position: absolute;
top: 50px;
bottom: 40px;
display: flex;
width: 100%;
overflow: hidden;
}
.nav {
flex: 2;
height: 100%;
font-size: 16px;
}
.nav ul {
width: 100%;
list-style: none;
height: 100%;
text-align: center;
background-color: #545c64;
}
.nav ul li {
height: 45px;
width: 100%;
border-bottom: 2px solid white;
background-color: #a0a0a0;
}
.nav ul li a {
display: block;
width: 100%;
height: 100%;
line-height: 45px;
color: white;
text-decoration: none;
}
.content {
flex: 8;
height: 100%;
}
.wrapper {
text-align: center;
width: 100%;
}
footer {
position: absolute;
bottom: 0;
width: 100%;
height: 40px;
background-color: #888;
line-height: 40px;
text-align: center;
font-size: 16px;
color: white;
}
table {
margin: 0 auto;
width: 100%;
border-collapse: collapse;
}
table thead {
width: 100%;
}
table thead tr {
background-color: #888;
}
tr {
display: flex;
width: 100%;
}
td,
th {
flex: 3;
border: 1px solid #eee;
line-height: 35px;
font-size: 12px;
}
td a {
cursor: pointer;
color: blue;
}
</style>
</head>
<body>
<div id="app">
<router-view></router-view>
</div>
</body>
<script>
const App = {
template: `<div>
<header>后台管理系统</header>
<div class="main">
<div class="nav">
<ul>
<li><a href="#"><router-link to="/users">用户管理</router-link></a></li>
<li><a href="#"><router-link to="/permission">权限管理</router-link></a></a></li>
<li><a href="#"><router-link to="/goods">商品管理</router-link></a></li>
<li><a href="#"><router-link to="/order">订单管理</router-link></a></li>
<li><a href="#"><router-link to="/system">系统设置</router-link></a></li>
</ul>
</div>
<div class="content">
<div class="wrapper">
<router-view/>
</div>
</div>
</div>
<footer>版权信息</footer>
</div>
`
}
const Users = {
data() {
return {
userlist: [{
id: 1,
name: "zhangsan",
age: 20
}, {
id: 2,
name: "lisi",
age: 20
}, {
id: 3,
name: "wangwu",
age: 20
}, {
id: 4,
name: "zhaoliu",
age: 20
}, ]
}
},
template: `<div>
<h3>用户管理</h3>
<table>
<thead><tr><th>id</th><th>姓名</th><th>年龄</th><th>操作</th></tr></thead>
<tbody :key="item.id" v-for="item in userlist">
<tr>
<td>{{item.id}}</td>
<td>{{item.name}}</td>
<td>{{item.age}}</td>
<td><a @click="detail(item.id)">详情</a></td>
</tr>
</tbody>
</table>
</div>`,
methods: {
detail: function(id) {
this.$router.push('/detail/' + id)
}
}
}
const Detail = {
props: ['id'],
template: `<div>
<h1>{{$route.params.id}}详情</h1>
<button @click="goBack()">后退</button>
</div>`,
methods: {
goBack() {
this.$router.go(-1)
}
}
}
const Permission = {
template: `<div>
<h3>权限管理</h3>
</div>`
}
const Goods = {
template: `<div>
<h3>商品管理</h3>
</div>`
}
const Order = {
template: `<div>
<h3>订单管理</h3>
</div>`
}
const System = {
template: `<div>
<h3>系统管理</h3>
</div>`
}
const router = new VueRouter({
routes: [{
path: '/',
component: App,
children: [{
path: '/users',
component: Users
}, {
path: '/permission',
component: Permission
}, {
path: '/goods',
component: Goods
}, {
path: '/system',
component: System
}, {
path: '/order',
component: Order
}, {
path: '/detail/:id',
component: Detail,
props: true
}]
}, ]
})
const vm = new Vue({
el: '#app',
router
})
</script>
</html>