ActiveAndroid算是一个轻量级的ORM框架,简单地通过如save()和delete()等方法来做到增删改查等操作。配置起来也还算简单。
开始
在AndroidManifest.xml中我们需要添加这两个
AA_DB_NAME
(这个name不能改,但是是可选的,如果不写的话 是默认的"Application.db"这个值)-
AA_DB_VERSION
(optional – defaults to 1)...
这个<application>
是必须指定的,但你也可以使用自己的Application,继承自com.activeandroid.app.Application
1
|
public
class
MyApplication
extends
com.activeandroid.app.Application { ...
|
如果你不想或者不能继承com.activeandroid.app.Application
的话,那么就这样
1
2
3
4
5
6
7
8
9
10
11
12
|
public
class
MyApplication
extends
SomeLibraryApplication {
@Override
public
void
onCreate() {
super
.onCreate();
ActiveAndroid.initialize(
this
);
}
@Override
public
void
onTerminate() {
super
.onTerminate();
ActiveAndroid.dispose();
}
}
|
ActiveAndroid.initialize(this);
做初始化工作,ActiveAndroid.dispose();
做清理工作
创建数据库模型
我们使用@Table(name = "Items")
来表示表,使用@Column(name = "Name")
来表示列,ActiveAndroid会使用自增长的ID作为主键,然后按照注解描述,将类对应映射为数据库表。
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
|
@Table
(name =
"Items"
)
public
class
Item
extends
Model {
@Column
(name =
"Name"
)
public
String name;
@Column
(name =
"Category"
)
public
Category category;
public
Item(){
super
();
}
public
Item(String name, Category category){
super
();
this
.name = name;
this
.category = category;
}
}
|
依赖关系的数据库表
假如Item和Category是多对一的关系,那么我们可以这样子创建他们的类
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
|
@Table
(name =
"Items"
)
public
class
Item
extends
Model {
@Column
(name =
"Name"
)
public
String name;
@Column
(name =
"Category"
)
public
Category category;
}
<!-- lang: java -->
@Table
(name =
"Categories"
)
public
class
Category
extends
Model {
@Column
(name =
"Name"
)
public
String name;
public
List<Item> items() {
return
getMany(Item.
class
,
"Category"
);
}
}
|
如何保存和更新数据到数据库
单挑插入
保存Category对象
1
2
3
|
Category restaurants =
new
Category();
restaurants.name =
"Restaurants"
;
restaurants.save();
|
分配了一个category并且保存到数据库
1
2
3
4
|
Item item =
new
Item();
item.category = restaurants;
item.name =
"Outback Steakhouse"
;
item.save();
|
批量插入
如果你要批量插入数据,最好使用事务(transaction)。
1
2
3
4
5
6
7
8
9
10
11
12
|
ActiveAndroid.beginTransaction();
try
{
for
(
int
i =
0
; i <
100
; i++) {
Item item =
new
Item();
item.name =
"Example "
+ i;
item.save();
}
ActiveAndroid.setTransactionSuccessful();
}
finally
{
ActiveAndroid.endTransaction();
}
|
使用事务的话只用了 40ms,不然的话需要4秒。
删除记录
我们有三种方式删除一条记录
1
2
3
4
5
6
7
8
9
|
Item item = Item.load(Item.
class
,
1
);
item.delete();
<!-- lang: java -->
Item.delete(Item.
class
,
1
);
<!-- lang: java -->
new
Delete().from(Item.
class
).where(
"Id = ?"
,
1
).execute();
|
很简单吧
查询数据库
作者将查询做的非常像SQLite的原生查询语句,几乎涵盖了所有的指令 com.activeandroid.query包下有以下类
- Delete
- From
- Join
- Select
- Set
- Update
我们举例说明吧
1
2
3
4
5
6
7
|
public
static
Item getRandom(Category category) {
return
new
Select()
.from(Item.
class
)
.where(
"Category = ?"
, category.getId())
.orderBy(
"RANDOM()"
)
.executeSingle();
}
|
对应的sqlite查询语句就是 select * from Item where Category = ? order by RANDOM()
当然还支持其他非常多的指令
- limit
- offset
- as
- desc/asc
- inner/outer/cross join
- group by
- having 等等
大家可以在ActiveAndroid项目下的tests工程找到测试用例,有非常多详细的描述。
来自:http://linkyan.com/2013/05/about-activeandroid/
5 个最好的 Android ORM 框架
如果你正在开发一个Android应用程序,您可能需要在某个地方存储数据。您可以选择云服务(在这种情况下,使用 SyncAdapter 将是一个不错的主意),或存储您的数据在嵌入式SQLite数据库中。如果你选择第二个选项,你可能需要使用ORM。 在这篇文章中,将介绍一些你可以考虑在你的Android应用程序中使用的ORM框架。
OrmLite
OrmLite is the first Android ORM that comes to my mind. However OrmLite is not an Android ORM, it’s a Java ORM with SQL databases support. It can be used anywhere Java is used, such as JDBC connections, Spring, and also Android.
It makes heavy usage of annotations, such as @DatabaseTable
for each class that defines a table, or @DatabaseField
for each field in the class.
A simple example of using OrmLite to define a table would be something like this:
01 | @DatabaseTable (tableName = "users" ) |
02 | public class User { |
03 | @DatabaseField (id = true ) |
04 | private String username; |
05 | @DatabaseField |
06 | private String password; |
07 |
08 | public User() { |
09 | // ORMLite needs a no-arg constructor |
10 | } |
11 | public User(String username, String password) { |
12 | this .username = username; |
13 | this .password = password; |
14 | } |
15 |
16 | // Implementing getter and setter methods |
17 | public String getUserame() { |
18 | return this .username; |
19 | } |
20 | public void setName(String username) { |
21 | this .username = username; |
22 | } |
23 | public String getPassword() { |
24 | return this .password; |
25 | } |
26 | public void setPassword(String password) { |
27 | this .password = password; |
28 | } |
29 | } |
OrmLite for Android is open source and you can find it on GitHub. For more information readits official documentation here.
SugarORM
SugarORM is an ORM built only for Android. It comes with an API which is both simple to learn and simple to remember. It creates necessary tables itself, gives you a simple methods of creating one-to-one and one-to-many relationships, and also simplifies CRUD by using only 3 functions, save()
, delete()
and find()
(or findById()
).
Configure your application to use SugarORM by adding these four meta-data
tags to your apps AndroidManifest.xml
:
1 | <meta-data android:name= "DATABASE" android:value= "my_database.db" /> |
2 | <meta-data android:name= "VERSION" android:value= "1" /> |
3 | <meta-data android:name= "QUERY_LOG" android:value= "true" /> |
4 | <meta-data android:name= "DOMAIN_PACKAGE_NAME" android:value= "com.my-domain" /> |
Now you may use this ORM by extending it in the classes you need to make into tables, like this:
01 | public class User extends SugarRecord<User> { |
02 | String username; |
03 | String password; |
04 | int age; |
05 | @Ignore |
06 | String bio; //this will be ignored by SugarORM |
07 |
08 | public User() { } |
09 |
10 | public User(String username, String password, int age){ |
11 | this .username = username; |
12 | this .password = password; |
13 | this .age = age; |
14 | } |
15 | } |
So adding a new user would be:
1 | User johndoe = new User(getContext(), "john.doe" , "secret" , 19 ); |
2 | johndoe.save(); //stores the new user into the database |
Deleting all the users of age 19 would be:
1 | List<User> nineteens = User.find(User. class , "age = ?" , new int []{ 19 }); |
2 | foreach(user in nineteens) { |
3 | user.delete(); |
4 | } |
For more, read SugarORM’s online documentation.
GreenDAO
When it comes to performance, ‘fast’ and GreenDAO are synonymous. As stated on its website, “most entities can be inserted, updated and loaded at rates of several thousand entities per second”. If it wasn’t that good, these apps wouldn’t be using it. Compared to OrmLite, it is almost 4.5 times faster.
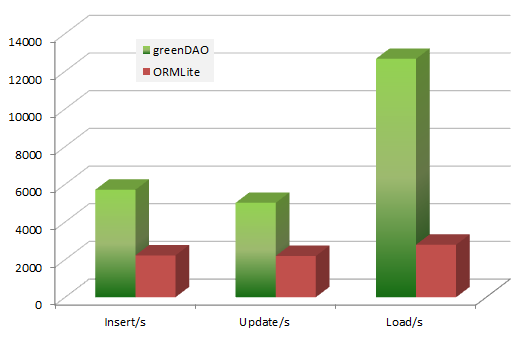
greenDAO vs OrmLite
Speaking of size, it is smaller than 100kb, so doesn’t affect APK size very much.
Follow this tutorial, which uses Android Studio to show the usage of GreenDAO in an Android application. You can view the GreenDAO source code on GitHub, and read the GreenDAO official documentation.
Active Android
Much like other ORMs, ActiveAndroid helps you store and retrieve records from SQLite without writing SQL queries.
Including ActiveAndroid in your project involves adding a jar
file into the /libs
folder of your Android project. As stated in the Getting started guide, you can clone the source code from GitHub and compile it using Maven. After including it, you should add these meta-data
tags into your app’s AndroidManifest.xml
:
1 | <meta-data android:name= "AA_DB_NAME" android:value= "my_database.db" /> |
2 | <meta-data android:name= "AA_DB_VERSION" android:value= "1" /> |
After adding these tags, you can call ActiveAndroid.initialize()
in your activity like this:
1 | public class MyActivity extends Activity { |
2 | @Override |
3 | public void onCreate(Bundle savedInstanceState) { |
4 | super .onCreate(savedInstanceState); |
5 | ActiveAndroid.initialize( this ); |
6 |
7 | //rest of the app |
8 | } |
9 | } |
Now that the application is configured to use ActiveAndroid, you may create Models as Java classes by using Annotations
:
01 | @Table (name = "User" ) |
02 | public class User extends Model { |
03 | @Column (name = "username" ) |
04 | public String username; |
05 |
06 | @Column (name = "password" ) |
07 | public String password; |
08 |
09 | public User() { |
10 | super (); |
11 | } |
12 |
13 | public User(String username,String password) { |
14 | super (); |
15 | this .username = username; |
16 | this .password = password; |
17 | } |
18 | } |
This is a simple example of ActiveAndroid usage. The documentation will help you understand the usage of ActiveAndroid ORM further.
Realm
Finally Realm is a ‘yet-to-come’ ORM for Android which currently only exists. It is built on C++, and runs directly on your hardware (not interpreted) which makes it really fast. The code for iOS is open source, and you can find it on GitHub.
On the website you will find some use cases of Realm in both Objective-C and Swift, and also a Registration form to get the latest news for the Android version.
Final words
These are not the only Android ORMs on the market. Other examples are Androrm and ORMDroid.
SQL knowledge is a skill that every developer should have, but writing SQL queries is boring, especially when there are so many ORMs out there. When they make your job simpler, why not use them in the first place?
How about you? What Android ORM do you use? Comment your choice below