目录
1.空域高斯滤波器
function [OutImage,ConvAarray] = FilterGauss(InputImage,Size)
%================================================================
% 功能:单通道图像高斯滤波器
% 参数:InputImage为输入单通道图像,Size为模板尺寸
% 返回值:OutImage为InputImage同维数组
% 主要思路:利用卷积核,模板对称,利用相关等于卷积
% 备注:如果图像为多通道则需要重复调用
% 调用方法:OutImage = FilterGauss(InputImage)
% 日期:2019.12.02
% 作者:Leetion
[iLimit,jLimit] = size(InputImage);
OutImage = InputImage;
switch (Size)
case 3
ConvAarray =double((1/16)*[1 2 1;2 4 2;1 2 1]);
for yIndex = 2:iLimit-1
for xIndex = 2:jLimit-1
NeighbourArray = [InputImage(yIndex-1,xIndex-1) InputImage(yIndex-1,xIndex) InputImage(yIndex-1,xIndex+1);...
InputImage(yIndex,xIndex-1) InputImage(yIndex,xIndex) InputImage(yIndex,xIndex+1);...
InputImage(yIndex+1,xIndex-1) InputImage(yIndex+1,xIndex) InputImage(yIndex+1,xIndex+1)];
OutImage(yIndex,xIndex) = uint8(sum(sum(ConvAarray.*double(NeighbourArray))));
end
end
case 5
ConvAarray =double((1/90)*[1 2 4 2 1;2 4 8 4 2;4 8 16 8 4;2 4 8 4 2; 1 2 4 2 1]);
for yIndex = 3:iLimit-2
for xIndex = 3:jLimit-2
NeighbourArray = [InputImage(yIndex-2,xIndex-2) InputImage(yIndex-2,xIndex-1) InputImage(yIndex-2,xIndex) InputImage(yIndex-2,xIndex+1) InputImage(yIndex-2,xIndex+2);...
InputImage(yIndex-1,xIndex-2) InputImage(yIndex-1,xIndex-1) InputImage(yIndex-1,xIndex) InputImage(yIndex-1,xIndex+1) InputImage(yIndex-1,xIndex+2);...
InputImage(yIndex,xIndex-2) InputImage(yIndex,xIndex-1) InputImage(yIndex,xIndex) InputImage(yIndex,xIndex+1) InputImage(yIndex,xIndex+2);...
InputImage(yIndex+1,xIndex-2) InputImage(yIndex+1,xIndex-1) InputImage(yIndex+1,xIndex) InputImage(yIndex+1,xIndex+1) InputImage(yIndex+1,xIndex+2);...
InputImage(yIndex+2,xIndex-2) InputImage(yIndex+2,xIndex-1) InputImage(yIndex+2,xIndex) InputImage(yIndex+2,xIndex+1) InputImage(yIndex+2,xIndex+2)];
OutImage(yIndex,xIndex) = uint8(sum(sum(ConvAarray.*double(NeighbourArray))));
end
end
otherwise
warning("只支持三阶和五阶");
end
end
2.中值滤波
function OutImage = FilterMiddle(InputImage,Size)
%================================================================
% 功能:单通道图像中值滤波器
% 参数:InputImage为输入单通道图像,Size为邻域尺寸
% 返回值:OutImage为InputImage同维数组
% 主要思路:求解邻域中值
% 备注:如果图像为多通道则需要重复调用
% 调用方法:OutImage = FilterMiddle(InputImage)
% 日期:2019.12.02
% 作者:Leetion
[iLimit,jLimit] = size(InputImage);
OutImage = InputImage;
if Size(1)==1 && Size(2)==3
flag = 1;
elseif Size(1)==1 && Size(2)==5
flag = 2;
elseif Size(1)==3 && Size(2)==1
flag = 3;
elseif Size(1)==5 && Size(2)==1
flag = 4;
elseif Size(1)==3 && Size(2)==3
flag = 5;
elseif Size(1)==5 && Size(2)==5
flag = 6;
else
warning("不支持的尺寸")
end
switch(flag)
case 1
for yIndex = 1:iLimit
for xIndex = 2:jLimit-1
NeighbourArray = [InputImage(yIndex,xIndex-1) InputImage(yIndex,xIndex) InputImage(yIndex,xIndex+1)];
OutImage(yIndex,xIndex) = median(NeighbourArray);
end
end
case 2
for yIndex = 1:iLimit
for xIndex = 3:jLimit-2
NeighbourArray = [InputImage(yIndex,xIndex-2) InputImage(yIndex,xIndex-1) InputImage(yIndex,xIndex) InputImage(yIndex,xIndex+1) InputImage(yIndex,xIndex+2)];
OutImage(yIndex,xIndex) = median(NeighbourArray);
end
end
case 3
for yIndex = 2:iLimit-1
for xIndex = 1:jLimit
NeighbourArray = [InputImage(yIndex-1,xIndex),InputImage(yIndex,xIndex),InputImage(yIndex+1,xIndex)];
OutImage(yIndex,xIndex) = median(NeighbourArray);
end
end
case 4
for yIndex = 3:iLimit-2
for xIndex = 1:jLimit
NeighbourArray = [InputImage(yIndex-2,xIndex) InputImage(yIndex-1,xIndex),InputImage(yIndex,xIndex),InputImage(yIndex+1,xIndex) InputImage(yIndex+2,xIndex)];
OutImage(yIndex,xIndex) = median(NeighbourArray);
end
end
case 5
for yIndex = 2:iLimit-1
for xIndex = 2:jLimit-1
NeighbourArray = [InputImage(yIndex-1,xIndex-1) InputImage(yIndex-1,xIndex) InputImage(yIndex-1,xIndex+1)...
InputImage(yIndex,xIndex-1) InputImage(yIndex,xIndex) InputImage(yIndex,xIndex+1)...
InputImage(yIndex+1,xIndex-1) InputImage(yIndex+1,xIndex) InputImage(yIndex+1,xIndex+1)];
OutImage(yIndex,xIndex) = median(NeighbourArray);
end
end
case 6
for yIndex = 3:iLimit-2
for xIndex = 3:jLimit-2
NeighbourArray = [InputImage(yIndex-2,xIndex-2) InputImage(yIndex-2,xIndex-1) InputImage(yIndex-2,xIndex) InputImage(yIndex-2,xIndex+1) InputImage(yIndex-2,xIndex+2)...
InputImage(yIndex-1,xIndex-2) InputImage(yIndex-1,xIndex-1) InputImage(yIndex-1,xIndex) InputImage(yIndex-1,xIndex+1) InputImage(yIndex-1,xIndex+2)...
InputImage(yIndex,xIndex-2) InputImage(yIndex,xIndex-1) InputImage(yIndex,xIndex) InputImage(yIndex,xIndex+1) InputImage(yIndex,xIndex+2)...
InputImage(yIndex+1,xIndex-2) InputImage(yIndex+1,xIndex-1) InputImage(yIndex+1,xIndex) InputImage(yIndex+1,xIndex+1) InputImage(yIndex+1,xIndex+2)...
InputImage(yIndex+2,xIndex-2) InputImage(yIndex+2,xIndex-1) InputImage(yIndex+2,xIndex) InputImage(yIndex+2,xIndex+1) InputImage(yIndex+2,xIndex+2)];
OutImage(yIndex,xIndex) = median(NeighbourArray);
end
end
end
end
3.频率域高斯滤波
function OutImage = FreFilterGauss(InputImage,D0)
%================================================================
% 功能:频域高斯滤波
% 参数:InputImage为输入单通道图像,D0为截止频率
% 返回值:OutImage为输出图像
% 主要思路:FFT转换到频率域,频率域乘积高斯传函,再IFFT2到时域
% 备注:使用库函数计算FFT和IFFT
% 调用方法:OutImage = FreFilterGauss(InputImage,D0)
% 日期:2019.12.02
% 作者:Leetion
[iLimit,jLimit] = size(InputImage);
FreInputImage = fft2(InputImage);
for vIndex = 1:iLimit
for uIndex = 1:jLimit
Distance = sqrt((vIndex-(1+iLimit)/2)^2+(uIndex-(1+jLimit)/2)^2);
H = exp(-(Distance^2/(2*D0^2)));
FreOutImage(vIndex,uIndex) = H*FreInputImage(vIndex,uIndex);
end
end
OutImage = uint8(ifft2(FreOutImage));
end
4.滤波器测试脚本
%================================================================
% 功能:滤波器测试
% 主要思路:
% 备注:
% 日期:2019.11.29
% 作者:leetion
clc,clear
close all
InputImage = imread('eight.tif');
figure(1)
subplot(2,3,1);
imshow(InputImage);
title("原图");
InputImage = imnoise(InputImage,'gaussian',0.001,0.001);
% InputImage = imnoise(InputImage,'salt & pepper');
subplot(2,3,2);
imshow(InputImage);
title("Gaussian Noise");
OutImage = FilterGauss(InputImage,3);
subplot(2,3,3);
imshow(OutImage);
title("Gaussian Filter");
subplot(2,3,4);
OutImage = FilterMiddle(InputImage,[5,5]);
imshow(OutImage);
title("Middle Filter");
subplot(2,3,5);
OutImage = FreFilterGauss(InputImage,1500);
imshow(OutImage);
title("FreGauss Filter");
figure(2)
subplot(2,3,1);
imshow(InputImage);
title("原图");
InputImage = imnoise(InputImage,'salt & pepper');
subplot(2,3,2);
imshow(InputImage);
title("Gaussian Noise");
OutImage = FilterGauss(InputImage,3);
subplot(2,3,3);
imshow(OutImage);
title("Gaussian Filter");
subplot(2,3,4);
OutImage = FilterMiddle(InputImage,[5,5]);
imshow(OutImage);
title("Middle Filter");
subplot(2,3,5);
OutImage = FreFilterGauss(InputImage,1500);
imshow(OutImage);
title("FreGauss Filter");
5.测试结果
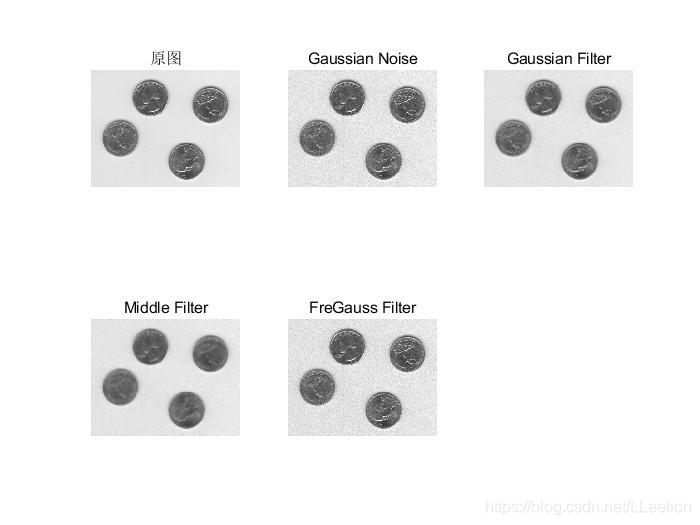
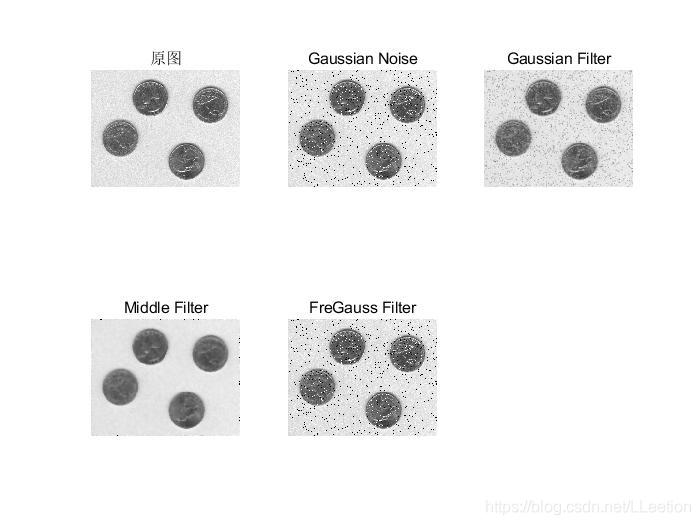