一、实验目的及要求
(1) 掌握Android的SharedPreferences的使用
(2) 掌握在Android中使用SQLite的方法
二、实验内容及步骤
任务:根据下述要求实现对应程序
1、 根据所给界面1完成登入功能,用户名为admin,密码为123456,如果用户名和密码不是admin和123456,则使用Toast提示“用户名或密码错误”,如果是则在checkbox选中的情况下,将用户名和密码保存,当此Activity再次启动时,将已保存的数据显示到界面中,实现记住密码功能。
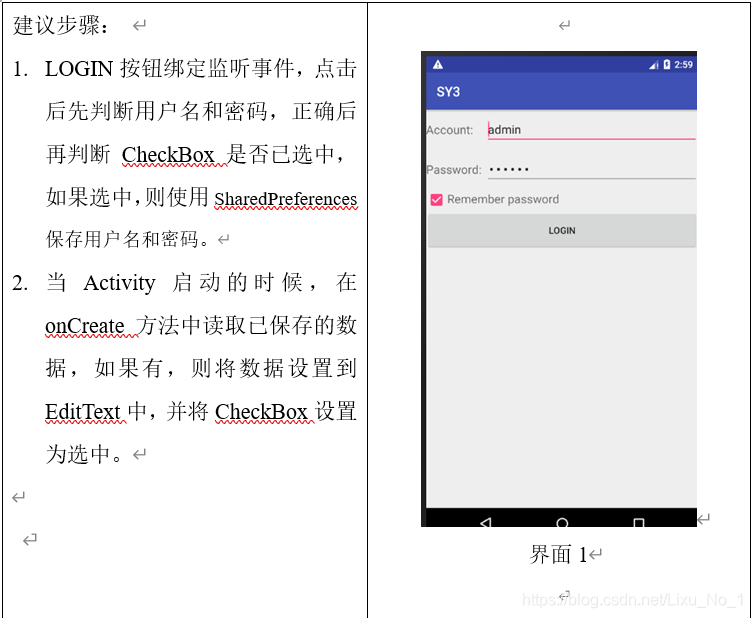
Java代码:
package com.example.test3