摘要:
具有图书管理员管理员登录,用户登录,图书添加、图书删除、图书修改、借书、还书等功能
1、新建项目
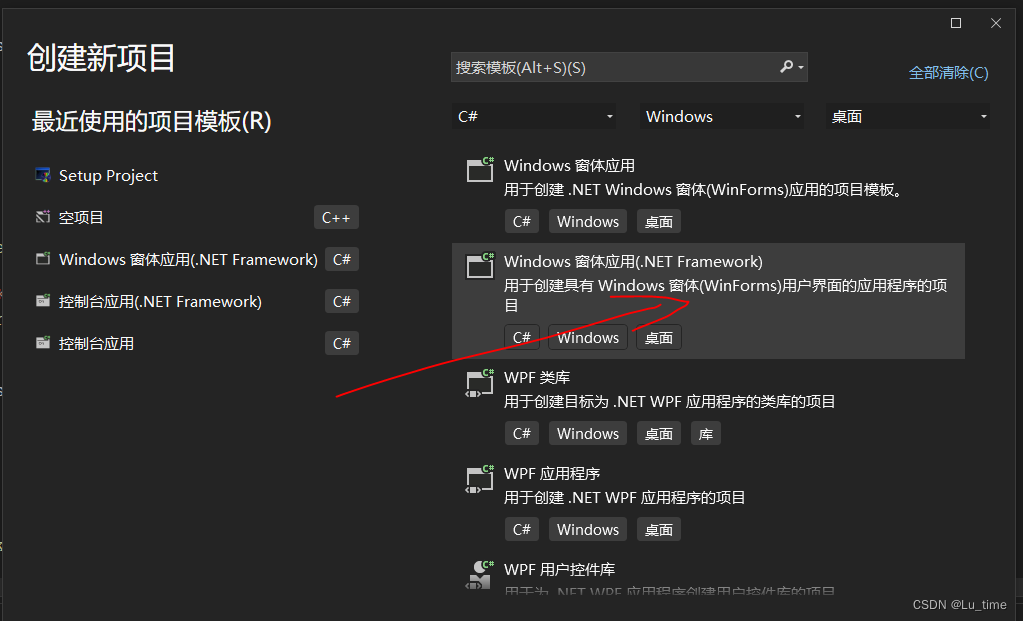
1-1、首先创建数据库连接类库,并命名,在类里写入以下语句
1-1-1、//新建这几个数据表
1-1-2、//下面是链接类库代码
SqlConnection sc;
public SqlConnection connect()
{
string str = @"Data Source =DESKTOP-MQFHV47;Initial Catalog=BookDB;Integrated Security=True";
sc = new SqlConnection(str);
sc.Open();
return sc;
}
public SqlCommand command(string sql)
{
SqlCommand cmd = new SqlCommand(sql, connect());
return cmd;
}
public int Execute(string sql)
{
return command(sql).ExecuteNonQuery();
}
public SqlDataReader read(string sql)
{
return command(sql).ExecuteReader();
}
public void Daoclose()
{
sc.Close();
}
1-2、接下来创建data数据类,这里存放用户的ID和姓名
namespace project
{
internal class Data
{
public static string UID = "", UName = "";
}
}
2、分别添加这些属性的窗口,添加类型如上图的窗口类型相同
2-1、登录界面
3、分别布局窗口
3-1、admin1窗口,有menustrip控件和label控件
3-2、admin2窗口
3-3、admin21窗口
3-4、admin22窗口
3-5、user1窗口
3-6、user2窗口
3-7、user3窗口
4、之后在每个窗口控件上添加各项代码
4-1、//admin窗口代码
namespace project
{
public partial class admin1 : Form
{
public admin1()
{
InitializeComponent();
}
private void 图书管理ToolStripMenuItem_Click(object sender, EventArgs e)
{
admin2 admin= new admin2();
admin.ShowDialog();
}
private void admin1_Load(object sender, EventArgs e)
{
}
}
}
4-2、//admin2代码
dao.Daoclose();
}
public void TableName()
{
dataGridView1.Rows.Clear();
Dao dao = new Dao();
string sql = $"select*from t_book where name like'%{textBox2.Text}%'";
IDataReader dc = dao.read(sql);
while (dc.Read())
{
dataGridView1.Rows.Add(dc[0].ToString(), dc[1].ToString(), dc[2].ToString(), dc[3].ToString(), dc[4].ToString());
}
dc.Close();
dao.Daoclose();
}
private void button3_Click(object sender, EventArgs e)
{
try
{
string id = dataGridView1.SelectedRows[0].Cells[0].Value.ToString();
label2.Text = id + dataGridView1.SelectedRows[0].Cells[1].Value.ToString();
DialogResult dr = MessageBox.Show("确认删除吗?","信息提示",MessageBoxButtons.OKCancel,MessageBoxIcon.Question);
if (dr == DialogResult.OK)
{
string sql = $"delete from t_book where id= '{id}' ";
Dao dao = new Dao();
if (dao.Execute(sql) > 0)
{
MessageBox.Show("删除成功");
Table();
}
else
{
MessageBox.Show("删除失败");
}
dao.Daoclose();
}
}
catch
{
MessageBox.Show("请先在表格中选择要删除的图书记录!","信息提示",MessageBoxButtons.OK,MessageBoxIcon.Error);
}
}
private void dataGridView1_Click(object sender, EventArgs e)
{
label2.Text = dataGridView1.SelectedRows[0].Cells[0].Value.ToString()+ " "
+dataGridView1.SelectedRows[0].Cells[1].Value.ToString();
}
private void button1_Click(object sender, EventArgs e)
{
admin21 a= new admin21();
a.ShowDialog();
}
private void button2_Click(object sender, EventArgs e)
{
try
{
string id = dataGridView1.SelectedRows[0].Cells[0].Value.ToString();
string name = dataGridView1.SelectedRows[0].Cells[1].Value.ToString();
string author = dataGridView1.SelectedRows[0].Cells[2].Value.ToString();
string press = dataGridView1.SelectedRows[0].Cells[3].Value.ToString();
string number = dataGridView1.SelectedRows[0].Cells[4].Value.ToString();
admin22 admin=new admin22(id,name,author,press,number);
admin.ShowDialog();
Table();//刷新数据
}
catch
{
MessageBox.Show("ERROR");
}
}
private void button5_Click(object sender, EventArgs e)
{
TableID();
}
private void button6_Click(object sender, EventArgs e)
{
TableName();
}
private void button4_Click(object sender, EventArgs e)
{
Table();
textBox1.Text = "";
textBox2.Text = "";
}
}
}
4-3、//admin21代码
namespace project
{
public partial class admin21 : Form
{
public admin21()
{
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e)
{
if (textBox1.Text != "" && textBox2.Text != "" && textBox3.Text != "" && textBox4.Text != "" && textBox5.Text != "" )
{
Dao dao = new Dao();
string sql =
$"insert into t_book values( '{textBox1.Text}','{textBox2.Text}','{textBox3.Text}','{textBox4.Text}',{textBox5.Text})";
dao.Execute(sql);
int n = dao.Execute(sql);
if (n > 0)
{
MessageBox.Show("添加成功");
}
else
{
MessageBox.Show("添加失败");
}
textBox1.Text = "";
textBox2.Text = "";
textBox3.Text = "";
textBox4.Text = "";
textBox5.Text = "";
}
else
{
MessageBox.Show("输入不能有空项");
}
}
private void button2_Click(object sender, EventArgs e)
{
textBox1.Text = "";
textBox2.Text = "";
textBox3.Text = "";
textBox4.Text = "";
textBox5.Text = "";
}
private void admin21_Load(object sender, EventArgs e)
{
}
}
}
4-4、//admin22代码
namespace project
{
public partial class admin22 : Form
{
string ID = "";
public admin22()
{
InitializeComponent();
}
public admin22(string id,string name,string author,string press,string number)
{
InitializeComponent();
ID=textBox1.Text = id;
textBox2.Text = name;
textBox3.Text = author;
textBox4.Text = press;
textBox5.Text = number;
}
private void button1_Click(object sender, EventArgs e)
{
string sql = $"update t_book set id='{textBox1.Text}',[name]='{textBox2.Text}'," +
$"author='{textBox3.Text}',press='{textBox4.Text}',number={textBox5.Text} where id='{ID}'";
Dao dao=new Dao();
if (dao.Execute(sql) > 0)
{
MessageBox.Show("修改成功");
this.Close();
}
}
private void admin22_Load(object sender, EventArgs e)
{
}
}
}
4-5、//Login的代码
namespace project
{
public partial class Login : Form
{
public Login()
{
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e)
{
if (textBox1.Text != "" && textBox2.Text != "")
{
login();
}
else
{
MessageBox.Show("输入有空项,请重新输入");
}
}
public void login()
{
if (radioButtonUser.Checked == true)
{
Dao dao = new Dao();
string sql = String.Format("select*from t_user where id= '{0}' and paw='{1}'", textBox1.Text, textBox2.Text);
IDataReader dc = dao.read(sql);
//MessageBox.Show(dc[0].ToString() + " "+dc["name"].ToString());
if (dc.Read())
{
Data.UID = dc["id"].ToString();
Data.UName = dc["name"].ToString();
MessageBox.Show("登陆成功");
user1 user = new user1();
this.Hide();
user.ShowDialog();
this.Show();
}
else
{
MessageBox.Show("登录失败");
}
dao.Daoclose();
}
if (radioButtonAdmin.Checked == true)
{
Dao dao = new Dao();
string sql = String.Format("select*from t_admin where id= '{0}' and paw='{1}'", textBox1.Text, textBox2.Text);
IDataReader dc = dao.read(sql);
//MessageBox.Show(dc[0].ToString() + " "+dc["name"].ToString());
if (dc.Read())
{
MessageBox.Show("登陆成功");
admin1 a = new admin1();
this.Hide();
a.ShowDialog();
this.Show();
}
else
{
MessageBox.Show("登录失败");
}
dao.Daoclose();
}
}
private void Login_Load(object sender, EventArgs e)
{
}
}
}
4-5、//user1代码
namespace project
{
public partial class user1 : Form
{
public user1()
{
InitializeComponent();
label1.Text = $"欢迎{Data.UName}登录";
}
private void 图书查看和借阅ToolStripMenuItem_Click(object sender, EventArgs e)
{
user2 user2 = new user2();
user2.ShowDialog();
}
private void user1_Load(object sender, EventArgs e)
{
//admin2 admin = new admin2();
}
private void 当前借出图书和归还ToolStripMenuItem_Click(object sender, EventArgs e)
{
user3 user3 = new user3();
user3.ShowDialog();
}
}
}
4-6、//user2代码
namespace project
{
public partial class user2 : Form
{
public user2()
{
InitializeComponent();
Table();
}
private void user2_Load(object sender, EventArgs e)
{
}
public void Table()
{
dataGridView1.Rows.Clear();
Dao dao = new Dao();
string sql = "select*from t_book";
IDataReader dc = dao.read(sql);
while (dc.Read())
{
dataGridView1.Rows.Add(dc[0].ToString(), dc[1].ToString(), dc[2].ToString(), dc[3].ToString(), dc[4].ToString());
}
dc.Close();
dao.Daoclose();
}
private void 借出图书_Click(object sender, EventArgs e)
{
string id = dataGridView1.SelectedRows[0].Cells[0].Value.ToString();
int number = int.Parse(dataGridView1.SelectedRows[0].Cells[4].Value.ToString());
if (number < 1)
{
MessageBox.Show("库存不足,请联系管理员购入");
}
else
{
string sql = $"insert into t_lend([uid],bid,[datetime]) values('{Data.UID}'," +
$"'{id}',getdate());update t_book set number=number-1 where id='{id}'";
Dao dao = new Dao();
if (dao.Execute(sql) > 1)
{
MessageBox.Show($"用户{Data.UName}借出了图书{ id}!");
Table();
}
}
}
}
}
4-8、//user3代码
namespace project
{
public partial class user3 : Form
{
public user3()
{
InitializeComponent();
Table();
}
public void Table()
{
dataGridView1.Rows.Clear();
Dao dao = new Dao();
string sql = $"select [no],[bid],[datetime] from t_lend where [uid]='{Data.UID}'";
IDataReader dc = dao.read(sql);
while (dc.Read())
{
dataGridView1.Rows.Add(dc[0].ToString(), dc[1].ToString(), dc[2].ToString());
}
dc.Close();
dao.Daoclose();
}
private void user3_Load(object sender, EventArgs e)
{
}
private void 借出图书_Click(object sender, EventArgs e)
{
string no= dataGridView1.SelectedRows[0].Cells[0].Value.ToString();
string id= dataGridView1.SelectedRows[0].Cells[1].Value.ToString();
string sql = $"delete from t_lend where[no] = {no}; update t_book set number = number + 1 where id = '{id}'";
Dao dao = new Dao();
if (dao.Execute(sql) > 1)
{
MessageBox.Show("归还成功");
Table();
}
}
}
}
5、总结
写这篇文章是为了记录一下我自己入门C#的第一个入门项目,从开始的一点不懂,做完之后能懂得一些简简单单的语句布局控件之类的,前前后后经历了差不多一周的时间,光是弄SQL Server就弄了两三天的时间,当然是断断续续的两三天时间,并不是从早到晚的,在自己本来的电脑上一直都连接不上数据库,一直提示什么鬼的添加了C++程序,删了好多和C++有关的程序和软件就是装不上,找了很久都不知道什么鬼原因,后来猜测可能是不兼容Win11吧(因为我几个同学的就是W10的系统,都装上了),就把另外一台学校的电脑格式化成了W10系统就可以连接上了。
需要源码或者需要解答的可以留言哈。
6、感谢栏
感谢B站的UP主:面朝星海我心澎湃
UID124790846
我就是从他的视频开始入门的,讲的非常的好,很详细,特别的适合小白和想入门C#的小伙伴学习。
代码连接如下:
链接:https://pan.baidu.com/s/1jo4By8jx-zY7ySsbInqnTw?pwd=1mko
提取码:1mko
后续我会更新一个书店售卖系统,大家可以支持支持哈哈。