- modern machines provide special
atomic
hardware instructions.
Atomic mean non-interruptable (i.e., the instruction executes as one unit)
- a global variable lock is initialized to 0.
- the only P
i
that can enter CS is the one which finds lock=0
- this Pi excludes all other Pj by setting lock to 1.
Advantages
• Applicable to any number of processes on either a single processor or multiple processors sharing main memory
• Simple and easy to verify
• It can be used to support multiple critical sections; each critical section can be defined by its own variable
Disadvantages
Busy-waiting
is employed, thus while a process is waiting for access to a critical section it continues to consume processor time
Starvation
is possible when a process leaves a critical section, and more than one process is waiting
Deadlock
is possible if a low priority process has the critical region and a higher priority process needs, the higher priority process will
obtain the processor to wait for the critical region
Operating Systems and Programming Language Solutions
Special variables: mutex, semaphore (互斥量、信号量)
Mutex Lock / Mutual exclusion
• The simplest tool
• Mutex is a software tool
•
Mutex allow multiple process / thread to access a single resource but not
simultaneously.
To enforce mutex
at the kernel level
and prevent the corruption of shared data structures -
disable interrupts
for the smallest number of
instructions is the best way.
To enforce mutex
in the software areas
– use the
busy-wait
mechanism
-
busy-wait mechanism
or
busy-looping
or
spinning
is a technique in which a process/thread repeatedly checks to see if a lock is available.
Using mutexes is to
acquire
a lock prior to entering a criticalsection, and to
release
it when exiting
Mutex object is locked or unlocked by the process requesting or releasing the resource
This type of mutex lock is called a
spinlock
because the process “spins” while waiting for the lock to become available.
Semaphore
• proposed by Dijkstra in 1965
• a technique to manage concurrent processes by using a simple integer value
• an
integer
variable which is
non-negative
and
shared between threads
• This variable is used to solve the critical section problem
and
to
achieve
process
synchronization
in
the
multiprocessing environment.
•
accessed
only through two standard atomic operations: wait()
and
signal()
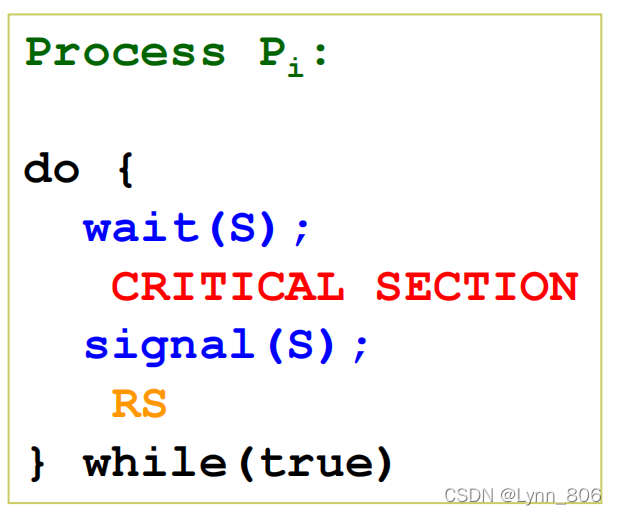
• For n
processes
• Initialize semaphore S
to
1
• Then only one process is allowed
into CS (
mutual exclusion
)
• To allow
k
processes into CS at a
time, simply initialize mutex to
k
two main types of semaphores:
• counting semaphore - allow an arbitrary resource count. Its value can range over an unrestricted domain. It is used to control access to a resource that has multiple instances.
• binary semaphore - also known as mutex lock. It can have only 2 values: 0 and 1(initialized to 1). It is used to implement the solution of critical section problem with multiple processes.
▪
Operating systems often distinguish between counting and binary semaphores.
Counting Semaphores
The semaphore S is initialized to the number of available resources.
Each
process that wishes to use a resource performs a wait() opera
tion on the semaphore (thereby
decrementing the number
of available resources
).
When a
process releases a resource, it performs a signal() op
eration (
incrementing the number of available resources
).
When the count for the semaphore goes to 0, all resources are being used.
After that, processes that wish to use a resource will
block until the count becomes greater than 0.
Binary Semaphores
Implement mutual exclusion, hence it is often called Mutex.
value is restricted to 0 and 1
The
wait()
operation only works when the semaphore is
1
and
the
signal()
operation succeeds when semaphore is
0
.
It is sometimes easier to implement binary semaphoresthan counting semaphores.
Same issues of semaphore
•
Starvation
and
Deadlock
are situations that occur when the processes that require a resource are delayed for a long
time.
Deadlock
is a condition where no process proceeds for execution, and each waits for resources that have been
acquired by the other processes.
in
Starvation
, process with high priorities continuously uses the resources preventing low priority process to acquire the
resources.
Classical Problems of Synchronization(5.7)
The Bounded-Buffer / Producer-Consumer Problem
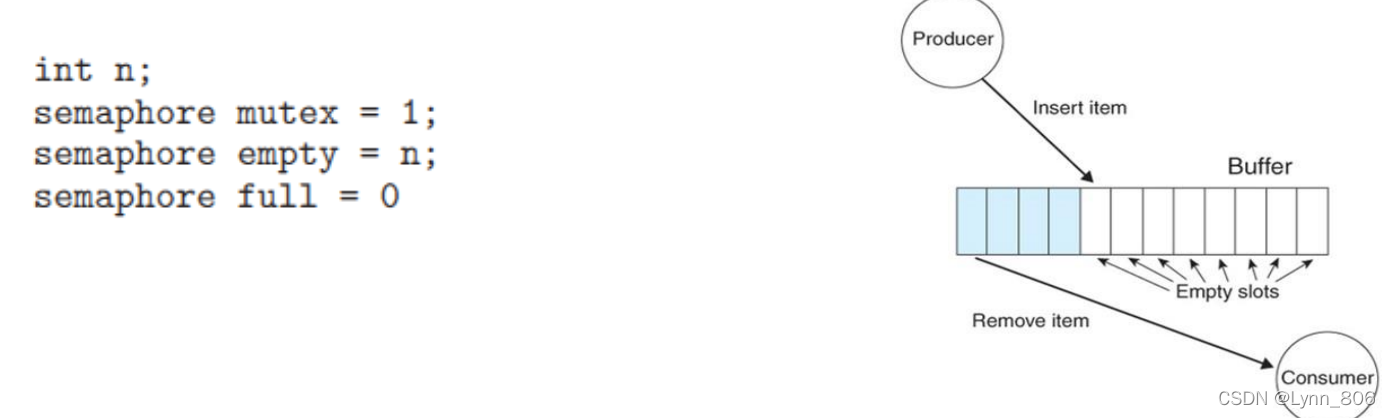
The mutex binary semaphore provides mutual exclusion for accesses to the buffer pool and is initialized to the value 1.
The
empty
and
full
semaphores count the number of empty and full buffers.
The Readers–Writers Problem
A
data set
is shared among a number of concurrent processes.
•
Only one single writer can access the shared data at the same time
, any other writers or readers must be blocked.
•
Allow multiple readers to read at the same time
, any writers must be blocked.
Solution
:
Acquiring a reader–writer lock requires specifying the mode of the lock: either
read
or
write
access.
The Dining-Philosophers Problem
How to allocate several resources among several processes.
Several solutions are possible:
• Allow only 4 philosophers to be hungry at a time.
• Allow pickup only if both chopsticks are available. ( Done in critical section )
• Odd # philosopher always picks up left chopstick 1
st
,
Even # philosopher always picks up right chopstick 1
st
.