一、平台驱动的注册函数
int platform_add_devices(struct platform_device **devs, int num)
{
int i, ret = 0;
for (i = 0; i < num; i++) {
ret = platform_device_register(devs[i]);
if (ret) {
while (
platform_device_unregister(devs[i]);
break;
}
}
return ret;
}
int platform_device_register(struct platform_device *pdev)
{
device_initialize(&pdev->dev);
return platform_device_add(pdev);
}
void device_initialize(struct device *dev)
{
dev->kobj.kset = devices_kset;
kobject_init(&dev->kobj, &device_ktype);
INIT_LIST_HEAD(&dev->dma_pools);
mutex_init(&dev->mutex);
lockdep_set_novalidate_class(&dev->mutex);
spin_lock_init(&dev->devres_lock);
INIT_LIST_HEAD(&dev->devres_head);
device_pm_init(dev);
set_dev_node(dev, -1);
}
int platform_device_add(struct platform_device *pdev)
{
int i, ret = 0;
if (!pdev)
return -EINVAL;
if (!pdev->dev.parent)
pdev->dev.parent = &platform_bus;
pdev->dev.bus = &platform_bus_type;
if (pdev->id != -1)
dev_set_name(&pdev->dev, "%s.%d", pdev->name, pdev->id);
else
dev_set_name(&pdev->dev, "%s", pdev->name);
for (i = 0; i < pdev->num_resources; i++) {
struct resource *p, *r = &pdev->resource[i];
if (r->name == NULL)
r->name = dev_name(&pdev->dev);
p = r->parent;
if (!p) {
if (resource_type(r) == IORESOURCE_MEM)
p = &iomem_resource;
else if (resource_type(r) == IORESOURCE_IO)
p = &ioport_resource;
}
if (p && insert_resource(p, r)) {
printk(KERN_ERR
"%s: failed to claim resource %d\n",
dev_name(&pdev->dev), i);
ret = -EBUSY;
goto failed;
}
e_add(&pdev->dev);
if (ret == 0)
return ret;
failed:
while (
struct resource *r = &pdev->resource[i];
unsigned long type = resource_type(r);
if (type == IORESOURCE_MEM || type == IORESOURCE_IO)
release_resource(r);
}
return ret;
}
二、平台驱动要注册的设备(硬件资源)
static struct platform_device *smdk4x12_devices[] __initdata = {
#ifdef CONFIG_ANDROID_PMEM
&pmem_device,
&pmem_gpu1_device,
#endif
&exynos4_device_pd[PD_MFC],
&exynos4_device_pd[PD_G3D],
&exynos4_device_pd[PD_LCD0],
&exynos4_device_pd[PD_CAM],
&exynos4_device_pd[PD_TV],
&exynos4_device_pd[PD_GPS],
&exynos4_device_pd[PD_GPS_ALIVE],
#ifdef CONFIG_VIDEO_EXYNOS_FIMC_IS
&exynos4_device_pd[PD_ISP],
#endif
#ifdef CONFIG_FB_MIPI_DSIM
&s5p_device_mipi_dsim,
#endif
#ifdef CONFIG_FB_S3C
&s5p_device_fimd0,
#if defined(CONFIG_LCD_AMS369FG06) || defined(CONFIG_LCD_LMS501KF03)
&s3c_device_spi_gpio,
#elif defined(CONFIG_LCD_WA101S)
&smdk4x12_lcd_wa101s,
#elif defined(CONFIG_LCD_LTE480WV)
&smdk4x12_lcd_lte480wv,
#elif defined(CONFIG_LCD_MIPI_S6E63M0)
&smdk4x12_mipi_lcd,
#endif
#endif
#ifdef CONFIG_FB_S5P
&s3c_device_fb,
#ifdef CONFIG_FB_S5P_LMS501KF03
&s3c_device_spi_gpio,
#endif
#endif
&s3c_device_wdt,
&s3c_device_rtc,
&s3c_device_i2c0_gpio,
&s3c_device_i2c1,
&s3c_device_i2c3,
&s3c_device_i2c4,
&s3c_device_i2c5,
#ifndef CONFIG_CAN_MCP251X
&s3c_device_i2c6,
#endif
&s3c_device_i2c7,
#if !defined(CONFIG_REGULATOR_MAX8997)
&s5p_device_pmic,
#endif
&tc4_regulator_consumer,
#ifdef CONFIG_USB_EHCI_S5P
&s5p_device_ehci,
#endif
#ifdef CONFIG_USB_OHCI_S5P
&s5p_device_ohci,
#endif
#ifdef CONFIG_USB_GADGET
&s3c_device_usbgadget,
#endif
#ifdef CONFIG_USB_ANDROID_RNDIS
&s3c_device_rndis,
#endif
#ifdef CONFIG_USB_ANDROID
&s3c_device_android_usb,
&s3c_device_usb_mass_storage,
#endif
&s3c_wlan_ar6000_pm_device,
&bt_sysfs,
#ifdef CONFIG_S5P_DEV_MSHC
&s3c_device_mshci,
#endif
#ifdef CONFIG_S3C_DEV_HSMMC
#endif
#ifdef CONFIG_S3C_DEV_HSMMC1
#endif
#ifdef CONFIG_S3C_DEV_HSMMC2
&s3c_device_hsmmc2,
#endif
#ifdef CONFIG_S3C_DEV_HSMMC3
&s3c_device_hsmmc3,
#endif
#ifdef CONFIG_EXYNOS4_DEV_DWMCI
&exynos_device_dwmci,
#endif
#ifdef CONFIG_SND_SAMSUNG_AC97
&exynos_device_ac97,
#endif
#ifdef CONFIG_SND_SAMSUNG_I2S
&exynos_device_i2s0,
#endif
#ifdef CONFIG_SND_SAMSUNG_PCM
&exynos_device_pcm0,
#endif
#ifdef CONFIG_SND_SAMSUNG_SPDIF
&exynos_device_spdif,
#endif
#if defined(CONFIG_SND_SAMSUNG_RP) || defined(CONFIG_SND_SAMSUNG_ALP)
&exynos_device_srp,
#endif
#ifdef CONFIG_VIDEO_EXYNOS_FIMC_IS
&exynos4_device_fimc_is,
#endif
#ifdef CONFIG_VIDEO_TVOUT
&s5p_device_tvout,
&s5p_device_cec,
&s5p_device_hpd,
#endif
#ifdef CONFIG_VIDEO_EXYNOS_TV
&s5p_device_i2c_hdmiphy,
&s5p_device_hdmi,
&s5p_device_sdo,
&s5p_device_mixer,
&s5p_device_cec,
#endif
#if defined(CONFIG_VIDEO_FIMC)
&s3c_device_fimc0,
&s3c_device_fimc1,
&s3c_device_fimc2,
&s3c_device_fimc3,
#elif defined(CONFIG_VIDEO_SAMSUNG_S5P_FIMC)
&s5p_device_fimc0,
&s5p_device_fimc1,
&s5p_device_fimc2,
&s5p_device_fimc3,
#endif
#if defined(CONFIG_VIDEO_FIMC_MIPI)
&s3c_device_csis0,
&s3c_device_csis1,
#elif defined(CONFIG_VIDEO_S5P_MIPI_CSIS)
&s5p_device_mipi_csis0,
&s5p_device_mipi_csis1,
#endif
#ifdef CONFIG_VIDEO_S5P_MIPI_CSIS
&mipi_csi_fixed_voltage,
#endif
#if defined(CONFIG_VIDEO_MFC5X) || defined(CONFIG_VIDEO_SAMSUNG_S5P_MFC)
&s5p_device_mfc,
#endif
#ifdef CONFIG_S5P_SYSTEM_MMU
#ifdef CONFIG_TC4_GB
&s5p_device_sysmmu,
#else
&SYSMMU_PLATDEV(g2d_acp),
&SYSMMU_PLATDEV(fimc0),
&SYSMMU_PLATDEV(fimc1),
&SYSMMU_PLATDEV(fimc2),
&SYSMMU_PLATDEV(fimc3),
&SYSMMU_PLATDEV(jpeg),
&SYSMMU_PLATDEV(mfc_l),
&SYSMMU_PLATDEV(mfc_r),
&SYSMMU_PLATDEV(tv),
#ifdef CONFIG_VIDEO_EXYNOS_FIMC_IS
&SYSMMU_PLATDEV(is_isp),
&SYSMMU_PLATDEV(is_drc),
&SYSMMU_PLATDEV(is_fd),
&SYSMMU_PLATDEV(is_cpu),
#endif
#endif //CONIG_TC4_GB
#endif /* CONFIG_S5P_SYSTEM_MMU */
#ifdef CONFIG_ION_EXYNOS
&exynos_device_ion,
#endif
#ifdef CONFIG_VIDEO_EXYNOS_FIMC_LITE
&exynos_device_flite0,
&exynos_device_flite1,
#endif
#ifdef CONFIG_VIDEO_FIMG2D
&s5p_device_fimg2d,
#endif
#ifdef CONFIG_EXYNOS_MEDIA_DEVICE
&exynos_device_md0,
#endif
#if defined(CONFIG_VIDEO_JPEG_V2X) || defined(CONFIG_VIDEO_JPEG)
&s5p_device_jpeg,
#endif
&samsung_asoc_dma,
&samsung_asoc_idma,
#ifdef CONFIG_BATTERY_SAMSUNG
&samsung_device_battery,
#endif
&samsung_device_keypad,
#ifdef CONFIG_WAKEUP_ASSIST
&wakeup_assist_device,
#endif
#ifdef CONFIG_EXYNOS_C2C
&exynos_device_c2c,
#endif
#ifdef CONFIG_KEYBOARD_GPIO
&gpio_button_device,
#endif
#ifdef CONFIG_S3C64XX_DEV_SPI
#if 0 //remove by cym 20130529
&exynos_device_spi0,
#ifndef CONFIG_FB_S5P_LMS501KF03
&exynos_device_spi1,
#endif
#endif
&exynos_device_spi2,
#endif
#ifdef CONFIG_EXYNOS_SETUP_THERMAL
&exynos_device_tmu,
#endif
#ifdef CONFIG_S5P_DEV_ACE
&s5p_device_ace,
#endif
&exynos4_busfreq,
#ifdef CONFIG_SWITCH_GPIO
&headset_switch_device,
#endif
&s3c_device_gps,
#ifdef CONFIG_MAX485_CTL
&s3c_device_max485_ctl ,
#endif
#ifdef CONFIG_LEDS_CTL
&s3c_device_leds_ctl,
#endif
#ifdef CONFIG_BUZZER_CTL
&s3c_device_buzzer_ctl,
#endif
#ifdef CONFIG_ADC_CTL
&s3c_device_adc_ctl,
#endif
#ifdef CONFIG_SMM6260_MODEM
&smm6260_modem,
#endif
#ifdef CONFIG_VIBRATOR
&s5p_vib_dev,
#endif
};
三、平台驱动的源码位置
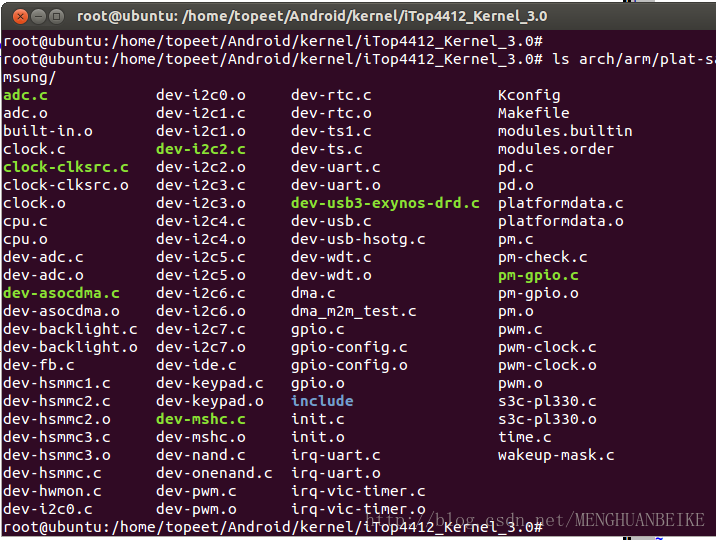