封装
struct Light {
void (*turnOn)(Light *self);
void (*turnOff)(Light *self);
void (*bright)(Light *self, const int brightness);
void (*show)(Light *self);
void (*setName)(Light *self, const char *name);
char name[64];
int brightness;
};
static void turnOn(Light *self) {
self->brightness = 100;
}
static void turnOff(Light *self) {
self->brightness = 0;
}
static void bright(Light *self, const int brightness) {
self->brightness = 0;
self->brightness = brightness;
}
static void setName(Light *self, const char *name) {
memset(self->name, 0, sizeof(self->name));
strcpy(self->name, name);
}
static void show(Light *self) {
printf("brightness of %s :> %d\n", self->name, self->brightness);
}
int main() {
Light led1;
led1.turnOn = turnOn;
led1.turnOff = turnOff;
led1.bright = bright;
led1.setName = setName;
led1.show = show;
Light led2;
led2.turnOn = turnOn;
led2.turnOff = turnOff;
led2.bright = bright;
led2.setName = setName;
led2.show = show;
led1.setName(&led1, "led1");
led1.turnOn(&led1);
led1.show(&led1);
led1.bright(&led1, 60);
led1.show(&led1);
led2.setName(&led2, "led2");
led2.turnOn(&led2);
led2.show(&led2);
led2.turnOff(&led2);
led2.show(&led2);
brightness of led1 :> 100
brightness of led1 :> 60
brightness of led2 :> 100
brightness of led2 :> 0
}
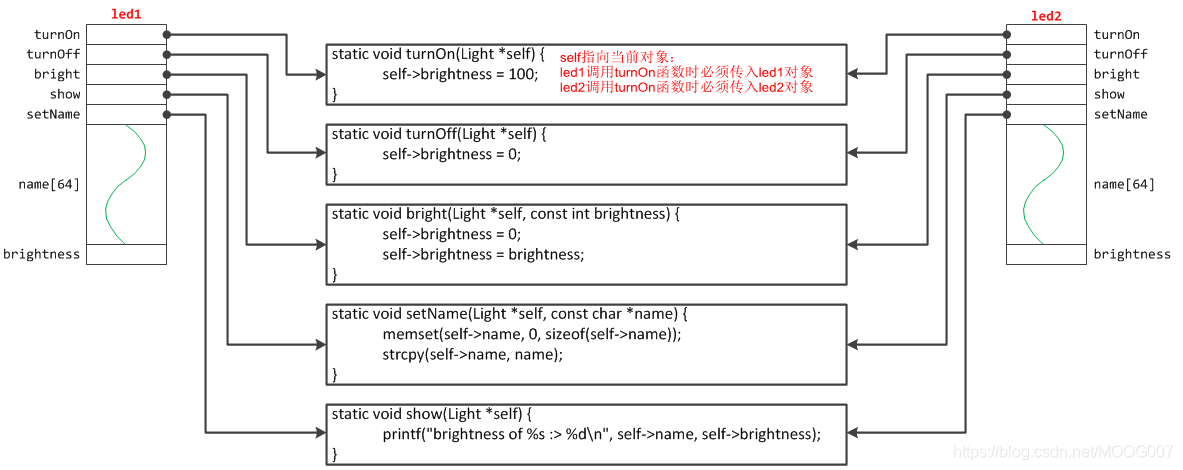
继承
- 从已有的数据类型来创建新的数据类型
- 现代编程语言中的继承的本质是扩展(
extend
):即复制继承
(1)子类继承了父类所有的成员变量(实例成员变量)
(2)子类继承了父类成员方法的方法调用权
- 继承本质:
加机制
:子类对象内部组合了一个父类子对象 - 继承目的:代码复用
- 在C语言中使用继承很简单:将父类的对象作为子类的第一个成员对象即可
#include <stdio.h>
#include <stdlib.h>
struct Shape {
void (*setColor)(Shape *self, const int color);
int (*getColor)(Shape *self);
void (*draw)(Shape *self);
int color;
};
struct Circle {
Shape super;
void (*setDimensions)(Circle *self, double x, double y, double r);
void (*draw)(Circle *self);
double x, y;
double r;
};
static void setColorShape(Shape *self, const int color) {
self->color = color;
}
static int getColorShape(Shape *self) {
return self->color;
}
static void drawShape(Shape *self) {
printf("我是抽象的形状: 不知道怎么绘制哦\n");
}
static void setDimensionsCircle(Circle *self, double x, double y, double r) {
self->x = x;
self->y = y;
self->r = r;
}
static void drawCircle(Circle *self) {
printf("绘制圆:圆心坐标(%g, %g), 圆半径 = %g\n", self->x, self->y, self->r);
}
int main() {
Circle c;
c.super.setColor = setColorShape;
c.super.getColor = getColorShape;
c.super.draw = drawShape;
c.setDimensions = setDimensionsCircle;
c.draw = drawCircle;
c.setDimensions(&c, 10, 20, 10);
c.super.draw((Shape*)&c);
c.draw(&c);
system("pause");
return 0;
}
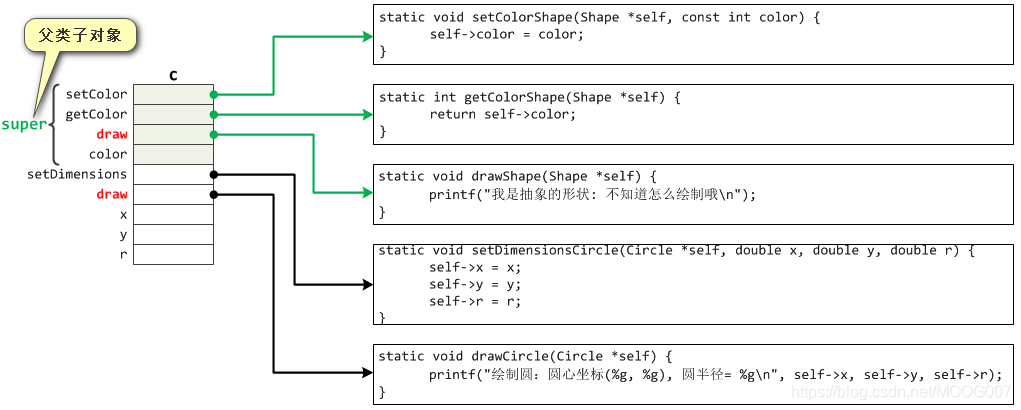
多态
#include <stdio.h>
#include <stdlib.h>
struct Shape {
void (*setColor)(Shape *self, const int color);
int (*getColor)(Shape *self);
void (*draw)(void *self);
int color;
};
struct Circle {
Shape super;
void (*setDimensions)(Circle *self, double x, double y, double r);
void (*draw)(void *self);
double x, y;
double r;
};
struct Rectangle {
Shape super;
void (*setDimensions)(Rectangle *self, int left, int top, int right, int bottom);
void (*draw)(void *self);
int left;
int top;
int right;
int bottom;
};
static void setColorShape(Shape *self, const int color) {
self->color = color;
}
static int getColorShape(Shape *self) {
return self->color;
}
static void drawShape(Shape *self) {
printf("我是抽象的形状: 不知道怎么绘制哦\n");
}
static void setDimensionsCircle(Circle *self, double x, double y, double r) {
self->x = x;
self->y = y;
self->r = r;
}
static void drawCircle(void *self) {
Circle *pCircle = (Circle *)self;
printf("绘制圆:圆心坐标(%g, %g), 圆半径 = %g\n", pCircle->x, pCircle->y, pCircle->r);
}
static void setDimensionsRectangle(Rectangle *self, int left, int top, int right, int bottom) {
self->top = top;
self->left = left;
self->right = right;
self->bottom = bottom;
}
static void drawRectangle(void *self) {
Rectangle *pRect = (Rectangle *)self;
printf("绘制矩形:左上角(%d, %d), 右下角(%d, %d)\n", pRect->left, pRect->top, pRect->right, pRect->bottom);
}
void draw(Shape *pShape) {
pShape->draw(pShape);
}
int main() {
Shape *pShape = 0;
Circle c;
c.super.setColor = setColorShape;
c.super.getColor = getColorShape;
c.super.draw = drawCircle;
c.setDimensions = setDimensionsCircle;
c.draw = drawCircle;
c.setDimensions(&c, 10, 20, 10);
draw((Shape *)&c);
Rectangle rect;
rect.super.setColor = setColorShape;
rect.super.getColor = getColorShape;
rect.super.draw = drawRectangle;
rect.setDimensions = setDimensionsRectangle;
rect.draw = drawRectangle;
rect.setDimensions(&rect, 10, 10, 150, 200);
draw((Shape *)&rect);
system("pause");
return 0;
}
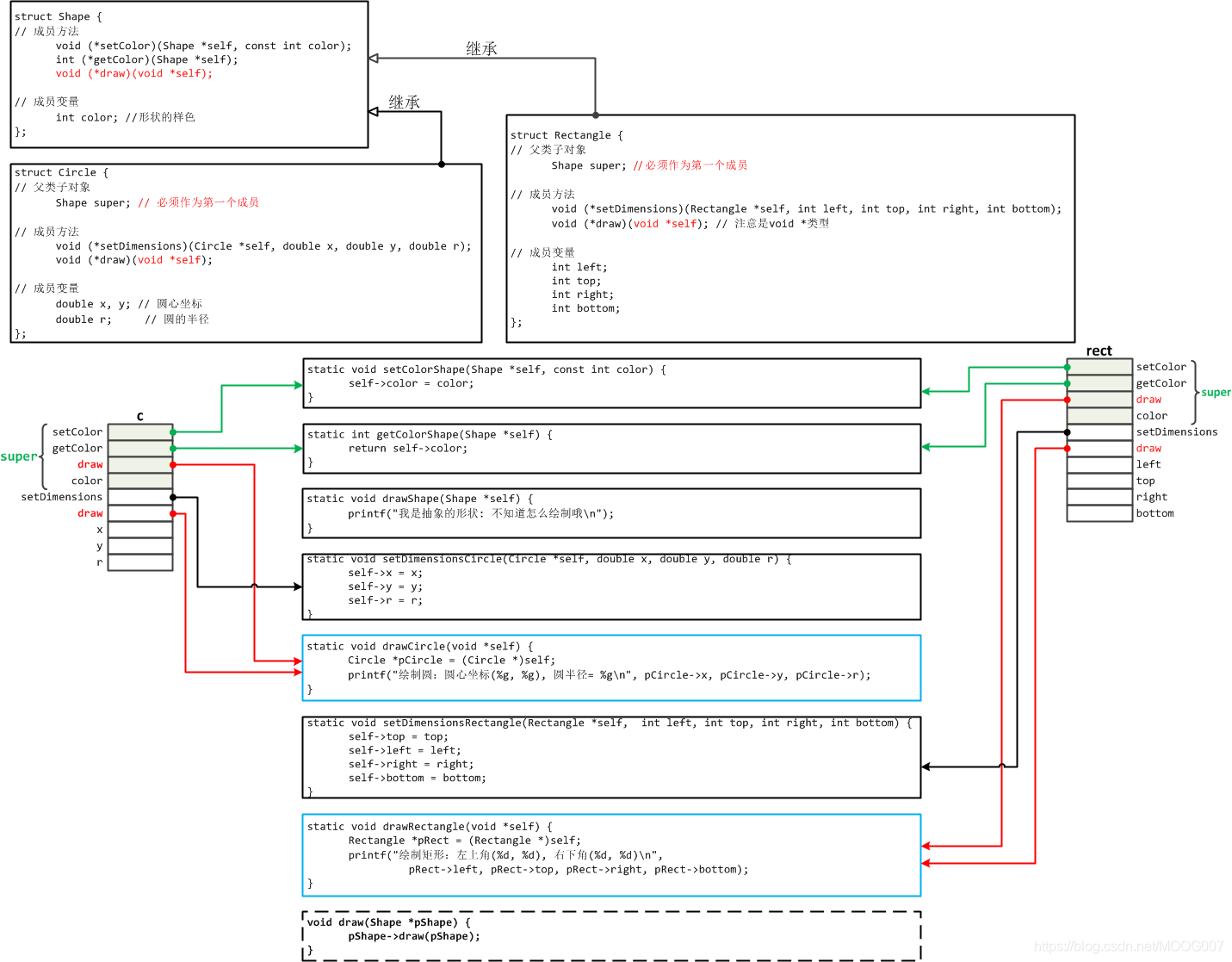