def main(self):
self.__app_load_data()
return self.__add_child_widgets()
# private part
# - app load data / add child widgets -
def __app_load_data(self):
all_account_data_list = self.__get_current_accounts_data_list(accounts_data_jsonfile=self.accounts_data_jsonfile)
self.all_account_data_model_list = []
[self.all_account_data_model_list.append(
TQZAccountModel(
account_id=account_data[TQZAccountKeyType.ACCOUNT_ID_KEY.value],
balance=account_data[TQZAccountKeyType.BALANCE_KEY.value],
risk_percent=account_data[TQZAccountKeyType.RISK_PERCENT_KEY.value],
yesterday_accounts_data=TQZJsonOperator.tqz_load_jsonfile(jsonfile=self.__yestertay_accounts_data_jsonfile)
)
) for account_data in all_account_data_list]
self.now_time_second = None
self.current_dropDown_select = TQZDropdownSelected.BALANCE.value
def __add_child_widgets(self):
self.layout_width = '60%'
self.window = gui.VBox(width='100%') # full window
# dropDown:排序选择
self.drop_down = self.__get_dropDown()
# time_label控件: 当前时间
self.time_label = self.__get_time_label()
# table控件: 账户对应的持仓数据
self.table = self.__get_table_list()
return self.__window_add_subviews(
self.drop_down,
self.time_label,
self.table,
window=self.window
)
# -- dropDown widget part --
def __get_dropDown(self):
drop_down = gui.DropDown.new_from_list(
(TQZDropdownSelected.BALANCE.value, TQZDropdownSelected.RISKPERCENT.value, TQZDropdownSelected.PROFIT_AND_LOSS.value),
width=self.layout_width
)
drop_down.onchange.do(self.__drop_down_change)
drop_down.select_by_value(TQZDropdownSelected.BALANCE.value)
return drop_down
def __drop_down_change(self, widget, selected_item):
self.current_dropDown_select = selected_item
self.__web_refresh()
# -- time label widget part --
def __get_time_label(self):
label_test = '更新时间: ' + self.__time_now()
return gui.Label(label_test, width=self.layout_width, height='20%') # 控件: label
@staticmethod
def __time_now():
return time.strftime("%Y/%m/%d %H:%M:%S", time.localtime())
# -- table widget part --
def __get_table_list(self):
content_data = self.__load_table_data()
return gui.Table.new_from_list(
content=content_data,
width=self.layout_width,
fill_title=True
) # fill_title: True代表第一行是蓝色, False代表表格内容全部同色
def __load_table_data(self):
title_cell = (
TQZMonitorWebDataType.INVESTOR.value,
TQZMonitorWebDataType.ACCOUNT_ID.value,
TQZMonitorWebDataType.BALANCE.value,
TQZMonitorWebDataType.USED_DEPOSIT.value,
TQZMonitorWebDataType.PROFIT_AND_LOSS_TODAY.value,
TQZMonitorWebDataType.RISK_PERCENT.value
)
table_data = [title_cell]
accounts_data_list = self.__get_current_accounts_data_list(accounts_data_jsonfile=self.accounts_data_jsonfile)
account_models = TQZAccountModel.list_to_models(
account_data_list=accounts_data_list,
yesterday_accounts_data=TQZJsonOperator.tqz_load_jsonfile(jsonfile=self.__yestertay_accounts_data_jsonfile)
)
if self.current_dropDown_select == TQZDropdownSelected.BALANCE.value:
account_models = sorted(account_models, key=lambda account_model: account_model.balance, reverse=True)
elif self.current_dropDown_select == TQZDropdownSelected.RISKPERCENT.value:
account_models = sorted(account_models, key=lambda account_model: float(account_model.risk_percent), reverse=True)
elif self.current_dropDown_select == TQZDropdownSelected.PROFIT_AND_LOSS.value:
account_models = sorted(account_models, key=lambda account_model: float(account_model.profit_and_loss), reverse=True)
total_balance = 0
total_available = 0
total_used_deposit = 0
total_profit_and_loss = 0
for account in account_models:
empty_cell = [
str(account.account_name),
str(account.account_id),
str(account.balance),
str(account.used_deposit),
str(account.profit_and_loss),
str(account.risk_percent) + "%",
]
table_data.append(tuple(empty_cell))
total_balance += account.balance
total_available += account.available
total_used_deposit += account.used_deposit
total_profit_and_loss += account.profit_and_loss
total_risk_percent_string = "0%"
if total_balance is not 0:
total_risk_percent_string = str(round(((total_balance - total_available) * 100) / total_balance, 2)) + "%"
table_data.append(("------", "------", "------", "------", "------", "------"))
end_cell = (
"总计: ",
" ",
str(round(total_balance, 2)),
str(round(total_used_deposit, 2)),
str(round(total_profit_and_loss, 2)),
total_risk_percent_string
)
table_data.append(end_cell)
return table_data
# -- web refresh part --
@staticmethod
def __is_refresh_time(now_time, interval_time):
"""
Judge current time need callback or not
"""
if now_time % interval_time is 0:
should_refresh = True
else:
should_refresh = False
return should_refresh
def __web_refresh(self):
self.window.remove_child(self.table)
self.window.remove_child(self.time_label)
self.time_label = self.__get_time_label()
self.table = gui.Table.new_from_list(
content=self.__load_table_data(),
width=self.layout_width,
fill_title=True
)
self.__window_add_subviews(
self.time_label,
self.table,
window=self.window
)
@staticmethod
def __window_add_subviews(*subviews, window):
[window.append(subview) for subview in subviews]
return window
def __get_current_accounts_data_list(self, accounts_data_jsonfile):
all_account_data_dictionary = TQZJsonOperator.tqz_load_jsonfile(jsonfile=accounts_data_jsonfile)
if all_account_data_dictionary is None:
all_account_data_dictionary = self.__pre_accounts_data_list
else:
self.__pre_accounts_data_list = all_account_data_dictionary
account_data_list = []
[account_data_list.append(account_data) for account_data in all_account_data_dictionary.values()]
return account_data_list
if __name__ == '__main__':
Server(
gui_class=TQZMonitorAccountsWeb,
update_interval=1,
port=8878,
start_browser=True
) # 参数 update_interval: 程序每1s调用一次 idel() 函数;
量化交易之vnpy篇 - 监控模块 - UI部分
最新推荐文章于 2022-10-29 22:15:36 发布
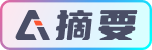