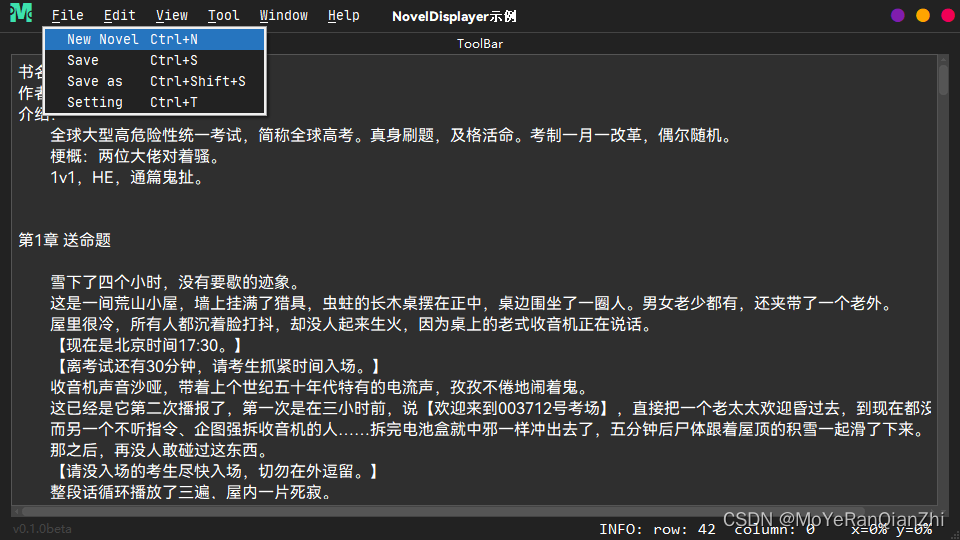
目录
前言
在今天的数字时代,爬虫已经成为了信息搜集和整理的不可或缺的工具。在不断发展的科技生态中,越来越多的语言和技术被开发出来,满足了程序员对爬虫功能的需求。
本文将介绍一系列简易小说爬虫显示器的不同语言实现示例,从而为程序员提供多样的选择和参考。在这里,你不仅能够学到各种语言的代码实现方式,还可以了解它们在实现爬虫功能方面的优缺点。
一起来探索吧!
不同语言的小说爬虫实现
Python+tkinter
代码:
import tkinter as tk
import requests
def show_novel():
# 从网络上获取随机小说
response = requests.get("http://example.com/random-novel")
novel = response.text
# 显示小说内容
text.delete('1.0', tk.END)
text.insert('1.0', novel)
root = tk.Tk()
root.title("随机小说")
text = tk.Text(root, wrap=tk.WORD)
text.pack(fill=tk.BOTH, expand=True)
button = tk.Button(root, text="显示随机小说", command=show_novel)
button.pack()
root.mainloop()
这个程序使用
tkinter
创建了一个 GUI 窗口,其中包含一个文本框和一个按钮。当用户点击按钮时,会从网络上获取随机小说,并将小说内容显示在文本框中。
from tkinter import *
import requests
def show_novel():
# 从网络上获取随机小说
response = requests.get("http://example.com/random-novel")
novel = response.text
# 显示小说内容
text.delete('1.0', END)
text.insert('1.0', novel)
root = Tk()
root.title("随机小说")
text = Text(root, wrap=WORD)
text.pack(fill=BOTH, expand=True)
button = Button(root, text="显示随机小说", command=show_novel)
button.pack()
root.mainloop()
使用
from tkinter import *
可以简化代码,但它也可能带来一些问题,因为它将所有tkinter
中的内容都导入到当前命名空间中。如果你的代码中有与tkinter
中的变量、函数或类同名的内容,那么它们可能会被覆盖,导致代码错误。
运行步骤
安装 Python:首先需要安装 Python 编程语言,tkinter 是 Python 的内置 GUI 库,可以在 Python 安装时选择安装,或者使用命令行工具安装,requests是非常常用的库,可以使用pip安装。
pip install requests
创建程序文件:使用文本编辑器创建一个 Python 源代码文件,例如使用 .py 作为文件后缀;
运行程序:在命令行或者 IDE 中运行程序。
Python+WxPython
代码:
import wx
import requests
class NovelFrame(wx.Frame):
def __init__(self, parent, title):
wx.Frame.__init__(self, parent, title=title, size=(500, 300))
# 从网络上获取随机小说
response = requests.get("http://example.com/random-novel")
self.novel = response.text
self.panel = wx.Panel(self)
self.text = wx.TextCtrl(self.panel, style=wx.TE_MULTILINE|wx.TE_READONLY)
self.text.SetValue(self.novel)
self.text.SetSize(self.text.GetBestSize())
self.button = wx.Button(self.panel, label="显示随机小说")
self.Bind(wx.EVT_BUTTON, self.OnButtonClick, self.button)
sizer = wx.BoxSizer(wx.VERTICAL)
sizer.Add(self.text, proportion=1, flag=wx.EXPAND)
sizer.Add(self.button, flag=wx.ALIGN_CENTER)
self.panel.SetSizer(sizer)
self.Show()
def OnButtonClick(self, event):
# 从网络上获取随机小说
response = requests.get("http://example.com/random-novel")
self.novel = response.text
# 显示小说内容
self.text.SetValue(self.novel)
app = wx.App()
frame = NovelFrame(None, "随机小说")
app.MainLoop()
这段代码创建了一个名为
NovelFrame
的窗口,在该窗口中显示从网络上获取的随机小说。当点击窗口中的按钮时,会重新从网络上获取小说并显示。
运行步骤
- 安装wxPython:可以通过 pip 命令进行安装,命令如下:
pip install wxPython
- 创建程序:使用任意编辑器打开一个新的文件,并创建一个Python文件
- 运行程序:在命令行中,进入该文件的目录,然后键入以下命令:
python novel.py
Python+PyQt5
代码:
import sys
import requests
from PyQt5.QtWidgets import QApplication, QMainWindow, QTextEdit, QPushButton, QVBoxLayout, QWidget
class NovelWindow(QMainWindow):
def __init__(self):
super().__init__()
self.setWindowTitle("随机小说")
self.setGeometry(100, 100, 500, 300)
# 从网络上获取随机小说
response = requests.get("http://example.com/random-novel")
self.novel = response.text
# 创建文本框
self.text = QTextEdit()
self.text.setReadOnly(True)
self.text.setPlainText(self.novel)
# 创建按钮
self.button = QPushButton("显示随机小说")
self.button.clicked.connect(self.show_novel)
# 创建垂直布局
layout = QVBoxLayout()
layout.addWidget(self.text)
layout.addWidget(self.button)
# 创建中央部件
central_widget = QWidget()
central_widget.setLayout(layout)
self.setCentralWidget(central_widget)
def show_novel(self):
# 从网络上获取随机小说
response = requests.get("http://example.com/random-novel")
self.novel = response.text
# 显示小说内容
self.text.setPlainText(self.novel)
if __name__ == '__main__':
app = QApplication(sys.argv)
window = NovelWindow()
window.show()
sys.exit(app.exec_())
运行步骤
除了需要pyqt5库,其余和上述两个代码完全相同
pip install pyqt5
Java
代码:
import java.awt.BorderLayout;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.net.MalformedURLException;
import java.net.URL;
import java.net.URLConnection;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JScrollPane;
import javax.swing.JTextArea;
public class RandomNovel {
private JFrame frame;
private JTextArea textArea;
private JButton button;
public RandomNovel() {
frame = new JFrame("随机小说");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setSize(500, 300);
frame.setLocationRelativeTo(null);
textArea = new JTextArea();
textArea.setEditable(false);
JScrollPane scrollPane = new JScrollPane(textArea);
button = new JButton("显示随机小说");
button.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
String novel = getRandomNovel();
textArea.setText(novel);
}
});
frame.add(scrollPane, BorderLayout.CENTER);
frame.add(button, BorderLayout.SOUTH);
frame.setVisible(true);
}
private String getRandomNovel() {
StringBuilder novel = new StringBuilder();
try {
URL url = new URL("http://example.com/random-novel");
URLConnection connection = url.openConnection();
BufferedReader reader = new BufferedReader(new InputStreamReader(connection.getInputStream()));
String line;
while ((line = reader.readLine()) != null) {
novel.append(line).append("\n");
}
reader.close();
} catch (MalformedURLException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
return novel.toString();
}
public static void main(String[] args) {
new RandomNovel();
}
}
这个程序创建了一个用户界面,在该界面中显示从网络上获取的随机小说。当点击界面中的按钮时,会重新从网络上爬取小说
运行步骤
保存代码为一个 Java 文件,例如
NovelDisplay.java
。安装 Java 开发工具包 (JDK)。如果你还没有安装 JDK,请在这里下载:Java Downloads | Oracle
编译代码。在命令行中运行以下命令:
javac NovelDisplay.java
运行代码。在命令行中运行以下命令:
java NovelDisplay
这将启动一个图形界面,显示网络上随机的一本小说。
C
代码:
#include <stdio.h>
#include <stdlib.h>
#include <curl/curl.h>
struct string {
char *ptr;
size_t len;
};
void init_string(struct string *s) {
s->len = 0;
s->ptr = malloc(s->len+1);
if (s->ptr == NULL) {
fprintf(stderr, "malloc() failed\n");
exit(EXIT_FAILURE);
}
s->ptr[0] = '\0';
}
size_t writefunc(void *ptr, size_t size, size_t nmemb, struct string *s)
{
size_t new_len = s->len + size*nmemb;
s->ptr = realloc(s->ptr, new_len+1);
if (s->ptr == NULL) {
fprintf(stderr, "realloc() failed\n");
exit(EXIT_FAILURE);
}
memcpy(s->ptr+s->len, ptr, size*nmemb);
s->ptr[new_len] = '\0';
s->len = new_len;
return size*nmemb;
}
int main(void)
{
CURL *curl;
CURLcode res;
struct string s;
init_string(&s);
curl = curl_easy_init();
if(curl) {
curl_easy_setopt(curl, CURLOPT_URL, "http://example.com/random-novel");
curl_easy_setopt(curl, CURLOPT_WRITEFUNCTION, writefunc);
curl_easy_setopt(curl, CURLOPT_WRITEDATA, &s);
res = curl_easy_perform(curl);
if(res != CURLE_OK)
fprintf(stderr, "curl_easy_perform() failed: %s\n",
curl_easy_strerror(res));
curl_easy_cleanup(curl);
}
printf("%s\n", s.ptr);
free(s.ptr);
return 0;
}
这个代码使用了 cURL 库,从网络上获取随机小说,并在控制台中打印输出。
运行步骤
保存代码为一个 C 文件,例如
novel.c
。安装 cURL 库。如果你正在使用 Linux 或 macOS,请在终端中运行以下命令:
sudo apt-get install libcurl4-openssl-dev
如果你正在使用 Windows,请在这里下载安装包:https://curl.haxx.se/windows/
编译代码。在终端中运行以下命令:
gcc -o novel novel.c -lcurl
运行代码。在终端中运行以下命令:
./novel
这将在控制台中显示网络上随机的一本小说。
C++
代码:
#include <iostream>
#include <string>
#include <vector>
#include <fstream>
#include <ctime>
#include <cstdlib>
#include <windows.h>
using namespace std;
int main()
{
vector<string> novels; // 用来存储小说的名称
string line;
// 从文件中读取小说的名称
ifstream myfile("novels.txt");
if (myfile.is_open())
{
while (getline(myfile, line))
{
novels.push_back(line);
}
myfile.close();
}
else cout << "Unable to open file";
int novelCount = novels.size();
// 随机选择一本小说
srand(time(NULL));
int randomIndex = rand() % novelCount;
string selectedNovel = novels[randomIndex];
// 打开选中的小说并显示其内容
ifstream selectedNovelFile(selectedNovel);
if (selectedNovelFile.is_open())
{
cout << "Displaying the content of novel: " << selectedNovel << endl;
while (getline(selectedNovelFile, line))
{
cout << line << endl;
Sleep(100);
}
selectedNovelFile.close();
}
else cout << "Unable to open file";
return 0;
}
请注意,这段代码需要文件 novels.txt,该文件包含了要从中随机选择一本小说的所有小说的名称,每行一本。
运行步骤
下面是在 Windows 环境下编译和运行 C++ 代码的步骤:
安装 Visual Studio:如果尚未安装 Visual Studio,请从 Microsoft 的网站上下载并安装它。
创建新项目:打开 Visual Studio,选择“文件”>“新建”>“项目”,然后选择“Win32 Console Application”。输入项目名称,例如“NovelDisplay”,并选择项目的存储位置。
编写代码:在新项目的“源文件”文件夹中打开主 CPP 文件,并将上面的代码复制粘贴到该文件中。
创建 novels.txt:在项目文件夹中创建一个名为 novels.txt 的文本文件,并输入小说的名称,每行一本。
编译代码:选择“解决方案”视图,右键单击项目并选择“生成”。
运行代码:右键单击项目并选择“运行”,即可在控制台中看到随机选择的小说的内容。
请注意,上述步骤仅针对 Windows 环境,如果在其他操作系统上,编译和运行步骤可能有所不同。
C#
代码:
using System;
using System.Net;
using System.Windows.Forms;
namespace RandomNovel
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private void Form1_Load(object sender, EventArgs e)
{
WebClient client = new WebClient();
string novel = client.DownloadString("http://www.randomnovel.com/");
textBox1.Text = novel;
}
}
}
运行步骤
在运行此代码之前,您需要安装Visual Studio,并创建一个Windows Form项目。
- 打开Visual Studio并创建一个Windows Forms项目。
- 将代码粘贴到Form1.cs文件中。
- 在Form1设计视图中添加一个TextBox控件。
- 选择项目解决方案,并点击“运行”按钮,即可看到随机显示的小说内容。
Lua
代码:
-- 打开文件并读入小说列表
local file = io.open("novels.txt", "r")
local novels = {}
for line in file:lines() do
table.insert(novels, line)
end
file:close()
-- 随机选择一本小说
math.randomseed(os.time())
local index = math.random(#novels)
print("随机选择的小说是:" .. novels[index])
运行步骤
首先,你需要安装 Lua 解释器。你可以在以下网站下载安装包:Lua: download
然后,你可以按照以下步骤运行代码:
创建名为 novels.txt 的文件,并在其中列出您想要显示的小说的名称。
在你喜欢的文本编辑器中创建名为 script.lua 的文件,并粘贴以上代码。
使用以下命令在命令行中打开脚本:
lua script.lua
- 如果一切顺利,你将在命令行中看到随机选择的小说的名称。
- 注意:在某些操作系统中,您可能需要在命令前添加“./”,以告诉系统从当前目录中运行脚本。
Go
Go 是一种开源编程语言,被设计用于构建快速,高效且可靠的软件。Go 语言结合了 C 语言的高效编码能力和 Python 语言的易学性,是一种非常适合快速开发的语言。
代码:
package main
import (
"fmt"
"math/rand"
"time"
)
func main() {
// 小说列表
novels := []string{"《追风筝的人》", "《百年孤独》", "《海边的卡夫卡》", "《骆驼祥子》"}
// 设置随机种子
rand.Seed(time.Now().UnixNano())
// 随机选择一本小说
randomNovel := novels[rand.Intn(len(novels))]
// 显示随机选择的小说
fmt.Println("随机选择的小说是:", randomNovel)
}
运行步骤
你可以在以下网站下载 Go 语言的开发工具包:
并可以在命令行中运行代码:
go run script.go
有关 Go 语言的更多信息,请参阅 Go 语言官方文档:
Kotlin
代码:
显示部分:
import javafx.application.Application
import javafx.scene.Scene
import javafx.scene.control.TextArea
import javafx.stage.Stage
class NovelDisplay : Application() {
override fun start(primaryStage: Stage?) {
primaryStage?.apply {
title = "随机小说"
scene = Scene(TextArea("这里显示随机小说的内容"), 640.0, 480.0)
show()
}
}
}
fun main() {
Application.launch(NovelDisplay::class.java)
}
爬虫部分:
import java.io.BufferedReader
import java.io.InputStreamReader
import java.net.URL
fun main(args: Array<String>) {
val url = URL("https://www.gutenberg.org/files/1342/1342-0.txt")
val connection = url.openConnection()
val reader = BufferedReader(InputStreamReader(connection.getInputStream()))
var line = reader.readLine()
while (line != null) {
println(line)
line = reader.readLine()
}
}
在Kotlin中,我们使用
java.net.URL
类和java.io.BufferedReader
类来读取网络上的数据。我们将URL对象作为参数传递给openConnection
方法,以获取一个连接对象。然后,我们使用BufferedReader
从连接对象中读取数据,并在控制台中打印出读取到的每一行。需要注意的是,由于该程序爬取了网络上的数据,请务必遵守爬虫的道德规范,不要爬取那些不应该被爬取的数据。
运行步骤
要运行该代码,你需要先编译它,然后再运行生成的程序。如果你使用的是IntelliJ IDEA,请参考如下步骤:
- 打开IntelliJ IDEA,并新建一个Kotlin项目。
- 将上述代码复制到主函数所在的文件中。
- 点击菜单栏的"Build",然后选择"Build Project"。
- 点击菜单栏的"Run",然后选择"Run"。
这样你就可以看到一个显示随机小说的窗口了。
JavaScript+HTML
代码:
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>网络小说展示</title>
</head>
<body>
<div id="novel-content"></div>
<script>
const displayNovel = async () => {
const response = await fetch("https://www.example.com/api/get_random_novel");
const novel = await response.json();
document.querySelector("#novel-content").innerHTML = novel.content;
};
displayNovel();
</script>
</body>
</html>
这个代码使用了 JavaScript 中的
fetch
API 来请求随机一本小说的内容,并使用innerHTML
属性将小说的内容插入到页面的#novel-content
元素中。
运行步骤
在任意浏览器上都可以运行
Python Flask+HTML
代码:
Python文件Novel.py
from flask import Flask, render_template
import requests
from bs4 import BeautifulSoup
app = Flask(__name__)
@app.route('/')
def index():
# 爬取网页内容
page = requests.get("https://www.gutenberg.org/browse/scores/top")
soup = BeautifulSoup(page.content, 'html.parser')
books = soup.find_all("li")
random_book = books[random.randint(0, len(books) - 1)].find("a")
book_title = random_book.get_text()
book_link = random_book["href"]
# 传递参数到 HTML 模板中渲染
return render_template("index.html", book_title=book_title, book_link=book_link)
if __name__ == '__main__':
app.run()
HTML 模板文件 index.html
<html>
<head>
<title>随机小说</title>
</head>
<body>
<h1>随机小说</h1>
<p>标题:{{ book_title }}</p>
<p>链接:<a href="{{ book_link }}">{{ book_link }}</a></p>
</body>
</html>
该代码使用 Flask 框架作为服务器,通过请求网页获取随机小说页面数据,然后使用 BeautifulSoup 解析网页内容,最后通过 Flask 渲染 HTML 模板并返回给用户。
运行步骤
- 在终端中运行上述代码,例如:
python app.py
。- 打开浏览器,访问
http://127.0.0.1:5000/
,即可查看随机小说的标题和链接。
Visual Basic
代码:
Imports System.Net
Imports System.Text
Module Module1
Sub Main()
Dim url As String = "http://www.example.com/novel.html"
Dim wc As New WebClient()
Dim data As Byte() = wc.DownloadData(url)
Dim html As String = Encoding.UTF8.GetString(data)
Console.WriteLine(html)
Console.ReadLine()
End Sub
End Module
该代码使用VB的WinHTTP组件来爬取网页内容
运行步骤
安装 Visual Basic 环境:如果你尚未安装 Visual Basic 环境,请下载并安装 Microsoft Visual Studio。
创建新项目:在 Visual Studio 中,打开新项目并选择“Windows Forms Application”模板。
导入必要的库:您需要导入 System.Net 库,以便使用 HttpWebRequest 和 HttpWebResponse 类。
编写代码:使用 HttpWebRequest 和 HttpWebResponse 类,创建一个函数,该函数可以向网络发送请求,以获取随机小说的 HTML 代码。
解析 HTML:使用正则表达式或其他方法,从 HTML 代码中提取小说的文本。
显示小说:在 Windows 窗体中,创建一个文本框,将小说的文本显示在文本框中。
运行项目:在 Visual Studio 中,编译并运行您的项目。你应该能够看到随机显示的小说文本。
请注意,这只是一种可能的实现方式,你可以根据需要修改和扩展代码。
PHP
代码:
<?php
// 创建一个cURL资源
$ch = curl_init();
// 设置URL和相应的选项
curl_setopt($ch, CURLOPT_URL, "http://www.example.com/");
curl_setopt($ch, CURLOPT_RETURNTRANSFER, 1);
// 抓取URL并返回结果
$output = curl_exec($ch);
// 关闭cURL资源,并且释放系统资源
curl_close($ch);
// 输出结果
echo $output;
?>
上面的代码使用cURL库通过HTTP协议从指定的URL抓取内容,并在浏览器中输出结果。
为了运行这段代码,你需要在服务器上安装cURL扩展,并在服务器上运行该代码。
运行步骤
- 安装一个Web服务器,比如Apache或Nginx。
- 安装PHP解释器。
- 创建一个PHP文件,写入你的代码。
- 在浏览器中打开该PHP文件,看看效果。
注意:运行步骤可能因系统环境而异。
R
代码:
library(rvest)
library(httr)
url <- "https://example.com/book"
webpage <- read_html(url)
book_content <- html_text(html_node(webpage, "p"))
print(book_content)
在这里,你可以替换example.com/book为您希望爬取的网页的URL。 html_node函数用于提取网页中的HTML节点,并使用html_text函数将其转换为文本。你可以将爬取的内容保存到book_content变量中,并使用print函数将其打印到控制台。
运行步骤
安装R环境:你可以从CRAN(Comprehensive R Archive Network)网站下载并安装R软件。
创建一个新的R文件:您可以在R环境中创建一个新的文件,并将你的代码粘贴到该文件中。R文件通常以".R"为扩展名。
运行代码:你可以选择在R环境的控制台中直接运行代码,也可以选择在R环境的图形用户界面中执行代码。你也可以在R命令行接口中运行代码。
观察输出:你的R程序的输出将在控制台中显示。
你可以根据你的需求进一步调整R程序,并继续进行测试和调试。
本文所示的代码仅作为示例,不可直接运行,皆需要一定修改。读者如有任何疑问,请自行Google。作者不对任何不当使用代码所造成的后果承担任何责任。