setAccessible(true) 关闭安全访问检测
import java.lang.reflect.InvocationTargetException;
import java.lang.reflect.Method;
public class TestRuntime {
public static void main(String[] args) throws ClassNotFoundException, NoSuchMethodException, IllegalAccessException, InvocationTargetException {
test01();
test02();
test03();
}
public static void test01(){
Player player = new Player();
long startTime = System.currentTimeMillis();
for (int i = 0; i < 2000000000; i++) {
player.getUsername();
}
long endTime = System.currentTimeMillis();
System.out.println("直接调用20亿次方法用时: " + (endTime - startTime) + "ms");
}
public static void test02() throws ClassNotFoundException, NoSuchMethodException, InvocationTargetException, IllegalAccessException {
Player player = new Player();
Class aclass = Class.forName("cn.alan.reflection.part_06.Player");
Method method = aclass.getMethod("getUsername");
long startTime = System.currentTimeMillis();
for (int i = 0; i < 2000000000; i++) {
method.invoke(player);
}
long endTime = System.currentTimeMillis();
System.out.println("反射调用20亿次方法用时: " + (endTime - startTime) + "ms");
}
public static void test03() throws ClassNotFoundException, NoSuchMethodException, InvocationTargetException, IllegalAccessException {
Player player = new Player();
Class aclass = Class.forName("cn.alan.reflection.part_06.Player");
Method method = aclass.getMethod("getUsername");
method.setAccessible(true);
long startTime = System.currentTimeMillis();
for (int i = 0; i < 2000000000; i++) {
method.invoke(player);
}
long endTime = System.currentTimeMillis();
System.out.println("反射调用20亿次方法(但是关闭安全访问检测)用时: " + (endTime - startTime) + "ms");
}
}
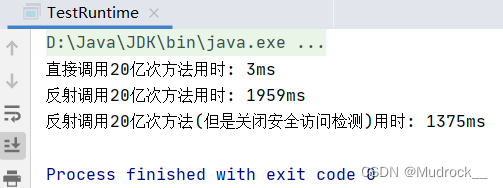