【id:81】【40分】A. DS单链表--存储结构与操作
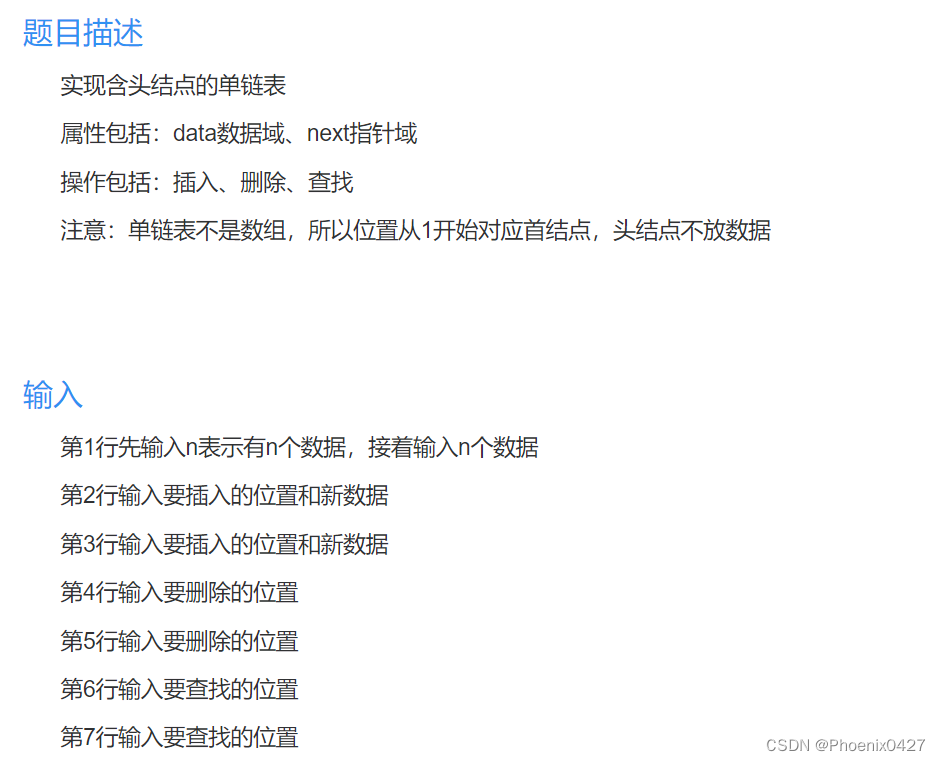
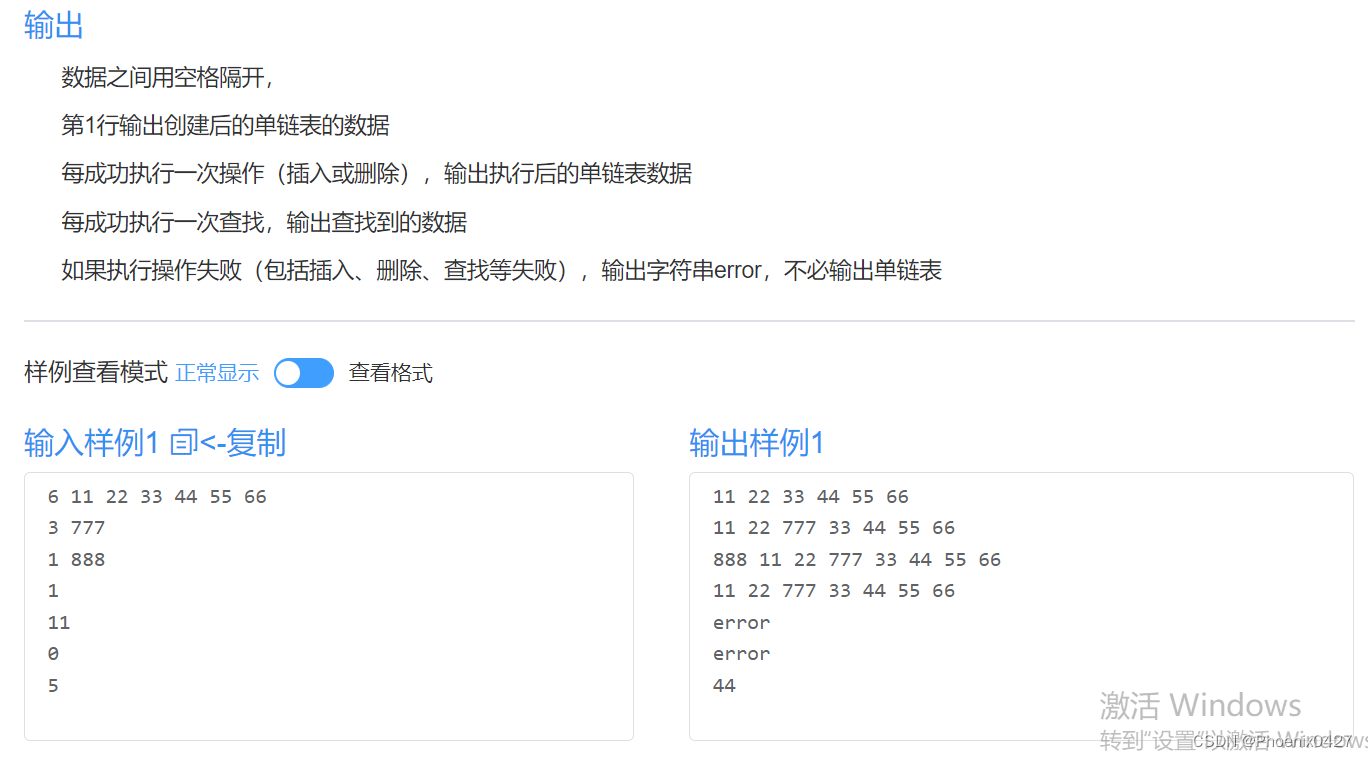
#include<iostream>
#include<stdlib.h>
using namespace std;
class Node
{
public:
int data;
Node* next;
Node()
{
next = NULL;
data = 0;
}
};
class linklist
{
public:
Node* head;
int length;
linklist();
~linklist();
Node* CreateNode(int n);
Node* index(int i);
int getitem(int i);
int listinsert(int i, int e);
int listdelete(int i);
void display();
};
linklist::linklist()
{
head = new Node();
length = 0;
}
linklist::~linklist()
{
Node* p, * q;
p = head;
while (p != NULL)
{
q = p;
p = p->next;
delete q;
}
length = 0;
head = NULL;
}
int linklist::getitem(int i)
{
if (index(i))
return index(i)->data;
else return -1;
}
Node* linklist::index(int i)
{
int j;
Node* p;
p = head->next;
j = 1;
while (p != NULL && j < i)
{
p = p->next;
j++;
}
if (j != i)
return NULL;
else
return p;
}
Node* linklist::CreateNode(int n)
{
Node* tail = head, * s;
int i;
for (i = 0; i < n; i++)
{
s = new Node;
cin >> s->data;
tail->next = s;
tail = s;
}
s = NULL;
delete s;
length = length + n;
return head;
}
void linklist::display()
{
Node* p;
p = head->next;
while (p)
{
cout << p->data << ' ';
p = p->next;
}
cout << endl;
}
int linklist::listdelete(int i)
{
Node* p = head, * q;
int j=0;
while (p->next && j < i - 1)
{
p = p->next;
j++;
}
if (!p->next || j > i - 1)
return -1;
q = p->next;
p->next = q->next;
free(q);
return 0;
}
int linklist::listinsert(int i, int item)
{
Node* p = head, * s;
int j = 0;
while (p && j < i - 1)
{
p = p->next;
j++;
}
if (!p || j > i - 1)
return -1;
s = new Node;
s->data = item;
s->next = p->next;
p->next = s;
return 0;
}
int main()
{
int n, i, num;
cin >> n;
linklist p;
Node* head;
head = p.CreateNode(n);
p.display();
cin >> i >> num;
if (p.listinsert(i, num) == 0)
p.display();
else
cout << "error" << endl;
cin >> i >> num;
if (p.listinsert(i, num) == 0)
p.display();
else
cout << "error" << endl;
cin >> i;
if (p.listdelete(i) == 0)
p.display();
else
cout << "error" << endl;
cin >> i;
if (p.listdelete(i) == 0)
p.display();
else
cout << "error" << endl;
cin >> i;
if (p.getitem(i) != -1)
cout << p.getitem(i);
else
cout << "error" << endl;
cin >> i;
if (p.getitem(i) != -1)
cout << p.getitem(i);
else
cout << "error" << endl;
}
【id:80】【20分】B. DS单链表--结点交换
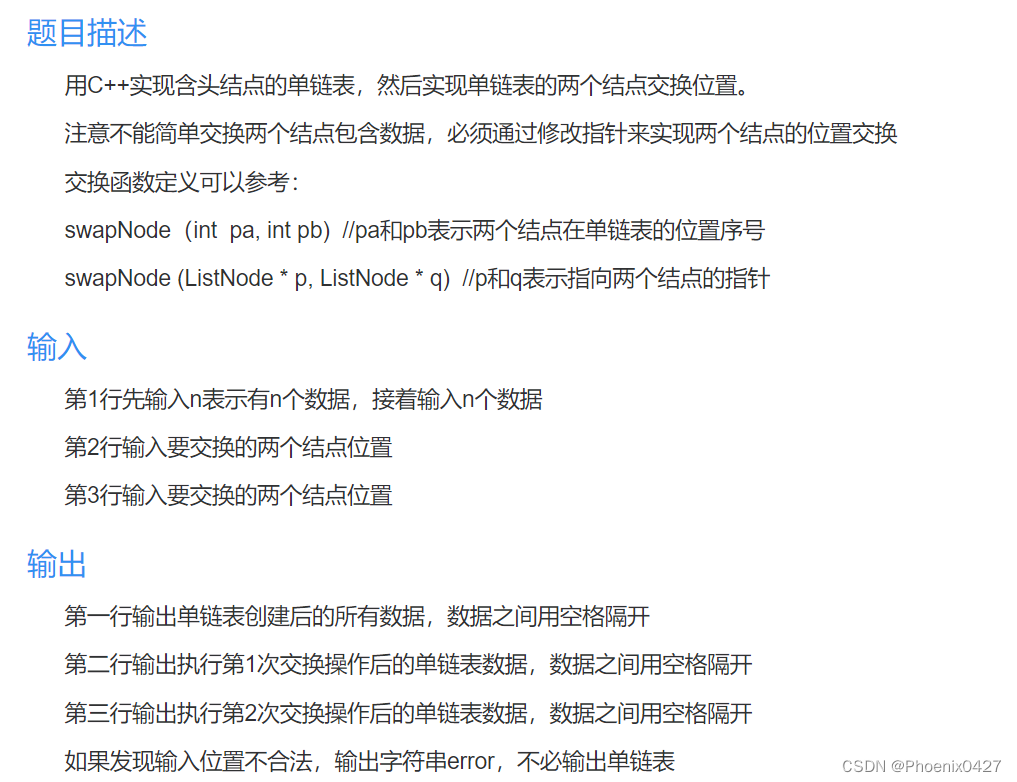
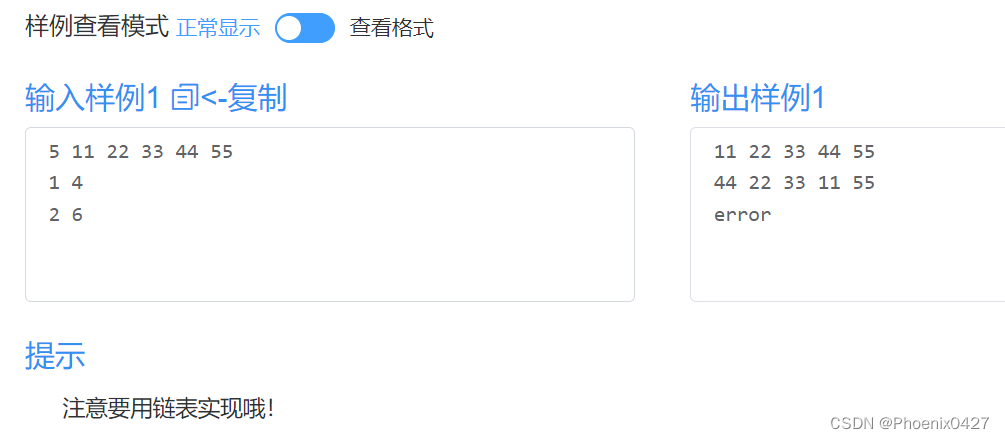
#include <iostream>
#include <stdlib.h>
using namespace std;
class Node
{
public:
int data;
Node* next;
Node()
{
next = NULL;
data = 0;
}
};
class linklist
{
public:
Node* head;
int length;
Node* listindex(int i);
linklist();
~linklist();
Node* CreateNode(int n);
int swap(int pa, int pb);
void display();
};
linklist::linklist()
{
head = new Node();
length = 0;
}
int linklist::swap(int pa, int pb)
{
if ((pa < 1 || pa > length) || (pb < 1 || pb > length))
return -1;
Node* p, * q;
p = new Node;
p = listindex(pa - 1);
q = listindex(pb - 1);
Node* t = p->next;
p->next = q->next;
q->next = t;
Node* tem = p->next->next;
p->next->next = q->next->next;
q->next->next = tem;
return 0;
}
linklist::~linklist()
{
Node* p, * q;
p = head;
while (p != NULL)
{
q = p;
p = p->next;
delete q;
}
length = 0;
head = NULL;
}
Node* linklist::listindex(int i)
{
int j=0;
Node* p=head;
while (p != NULL && j < i)
{
p = p->next;
j++;
}
return p;
}
Node* linklist::CreateNode(int n)
{
Node* tail = head, * s;
int i;
for (i = 0; i < n; i++)
{
s = new Node;
cin >> s->data;
tail->next = s;
tail = s;
}
s = NULL;
delete s;
length += n;
return head;
}
void linklist::display()
{
Node* p;
p = head->next;
while (p)
{
cout << p->data << ' ';
p = p->next;
}
cout << endl;
}
int main()
{
int n;
cin >> n;
linklist p;
Node* head;
head = p.CreateNode(n);
p.display();
int n1, n2;
cin >> n1 >> n2;
if (p.swap(n1, n2) == 0)
p.display();
else cout << "error" << endl;
cin >> n1 >> n2;
if (p.swap(n1, n2) == 0)
p.display();
else cout << "error" << endl;
}
【id:79】【20分】C. DS单链表--合并
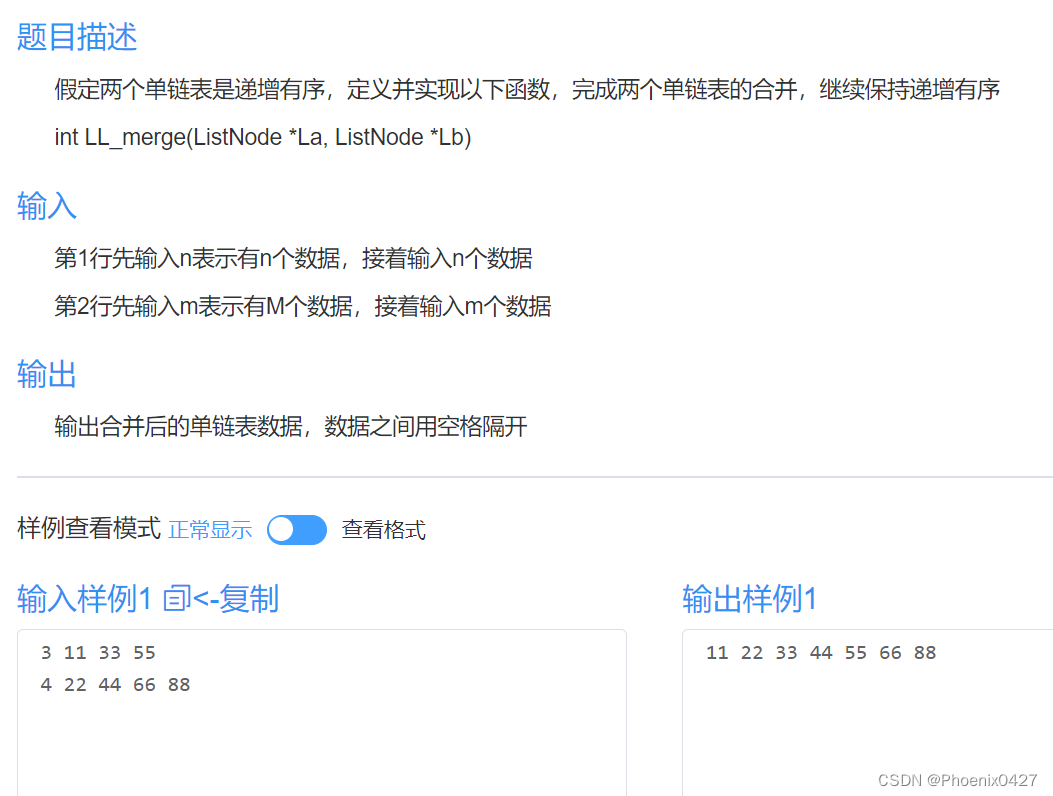
#include <iostream>
#include <stdlib.h>
using namespace std;
class Node
{
public:
int data;
Node* next;
Node()
{
next = NULL;
data = 0;
}
};
class linklist
{
public:
Node* head;
int length;
linklist();
Node* CreateNode(int n);
int listmerge(Node* La, Node* Lb);
void display();
};
linklist::linklist()
{
head = new Node();
length = 0;
}
Node* linklist::CreateNode(int n)
{
Node* tail = head, * s;
int i;
for (i = 0; i < n; i++)
{
s = new Node;
cin >> s->data;
tail->next = s;
tail = s;
}
s = NULL;
delete s;
length += n;
return head;
}
int linklist::listmerge(Node* La, Node* Lb)
{
Node* pa, * pb, * p;
pa = new Node;
pb = new Node;
p = new Node;
pa = La->next;
pb = Lb->next;
p = head;
if (!pa || !pb)
return 0;
while (pa && pb)
{
if (pa->data <= pb->data)
{
p->next = pa;
p = pa;
pa = pa->next;
}
else
{
p->next = pb;
p = pb;
pb = pb->next;
}
}
p->next = pa ? pa : pb;
return 1;
}
void linklist::display()
{
Node* p;
p = head->next;
while (p)
{
cout << p->data << ' ';
p = p->next;
}
cout << endl;
}
int main()
{
int n;
linklist p, q, r;
Node* La, * Lb;
La = new Node;
Lb = new Node;
cin >> n;
La = p.CreateNode(n);
cin >> n;
Lb = q.CreateNode(n);
if (r.listmerge(La, Lb))
r.display();
}
【id:73】【10分】D. DS循环链表—约瑟夫环(Ver. I - A)
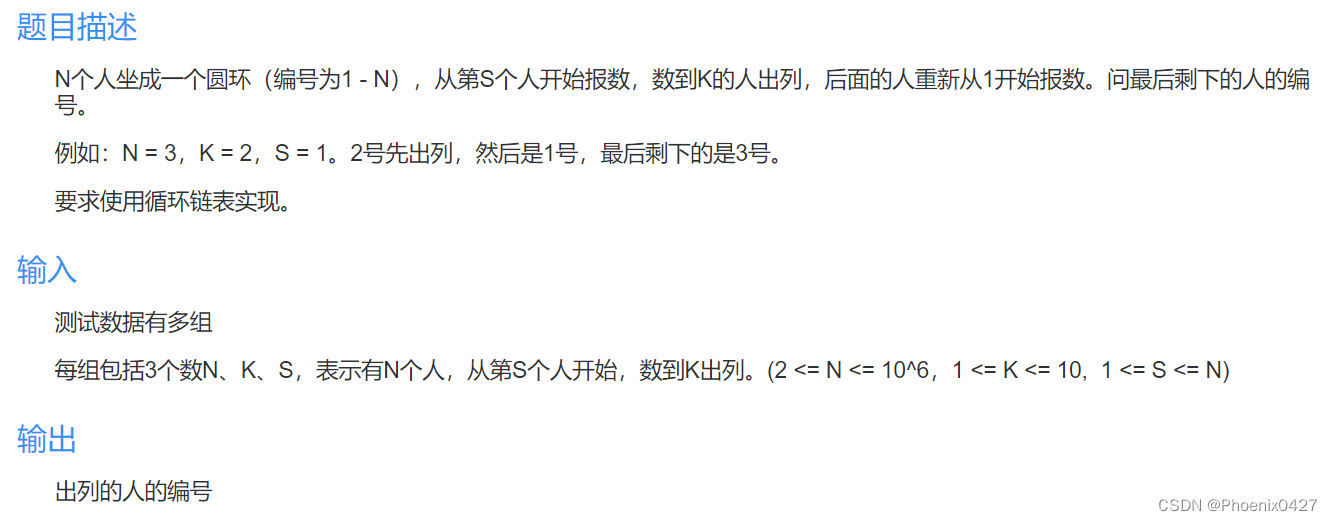
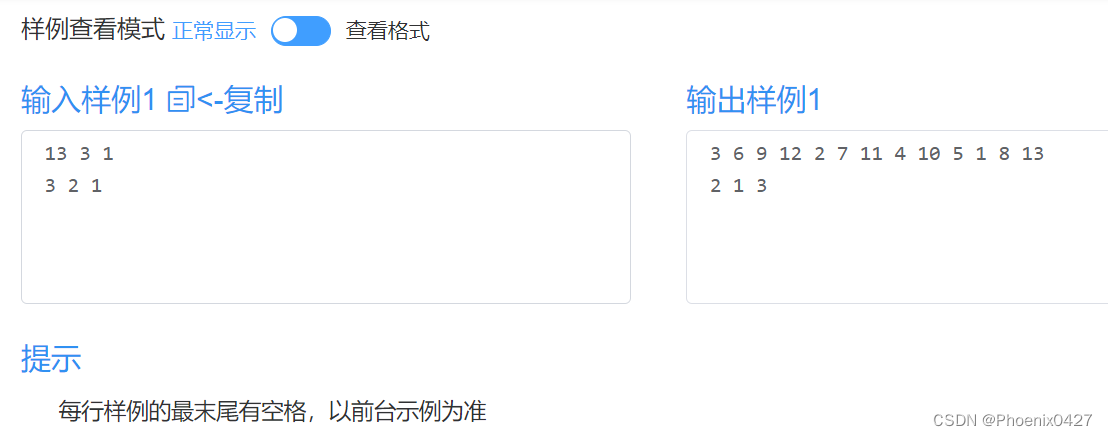
#include<iostream>
#include<stdio.h>
using namespace std;
typedef struct Node
{
int data;
struct Node* next;
}Node;
void CreateNode(Node* H, int& n)
{
H->next = NULL;
struct Node* p = H, * s;
int e = 0, i;
for (i = 0; i < n; i++)
{
++e;
s = new Node; s->next = NULL; s->data = e;
p->next = s;
p = s;
}
p->next = H->next;
}
void find(Node* Jose, int n, int k, int s)
{
int i;
struct Node* p = Jose;
struct Node* q = NULL;
while (p->data != s)
p = p->next;
while (p->next != p)
{
for (i = 0; i < k - 1; i++)
{
q = p;
p = p->next;
}
cout << p->data << " ";
q->next = p->next;
p = q->next;
}
cout << p->data << " ";
cout << endl;
}
int main()
{
int k, n, s, i;
while (scanf("%d%d%d", &n, &k, &s) != EOF)
{
Node* L = new Node;
CreateNode(L, n);
find(L, n, k, s);
delete L;
}
return 0;
}
【id:78】【5分】E. DS线性表—多项式相加
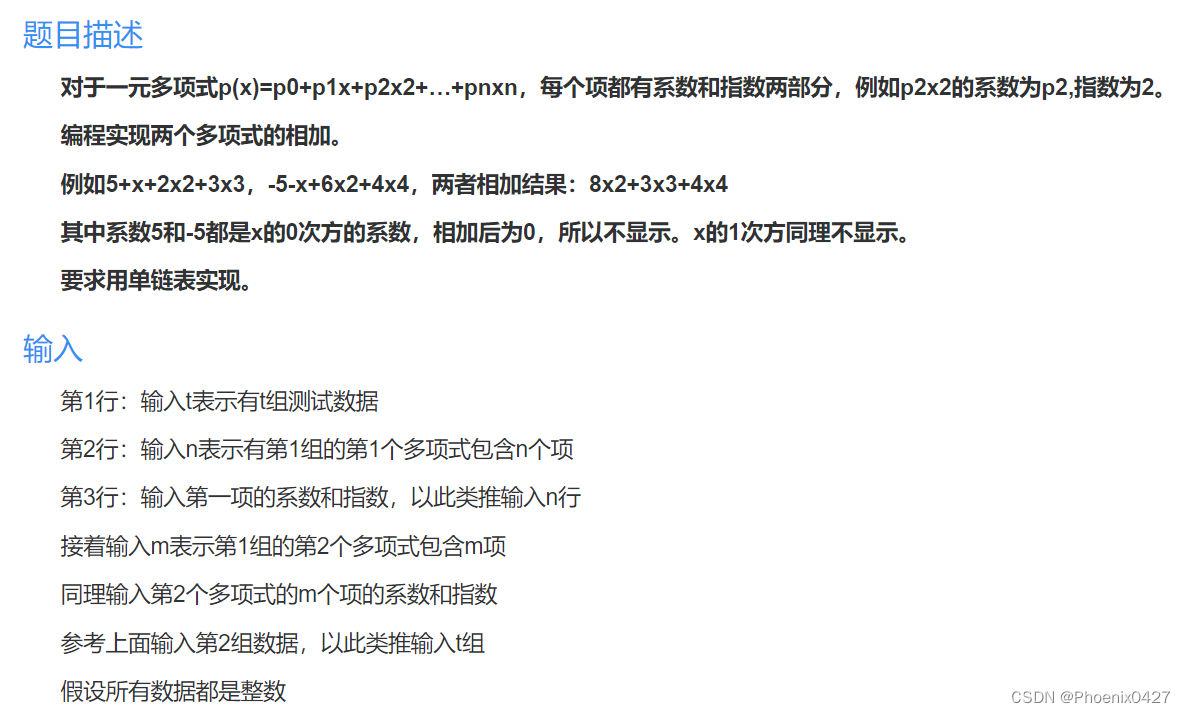
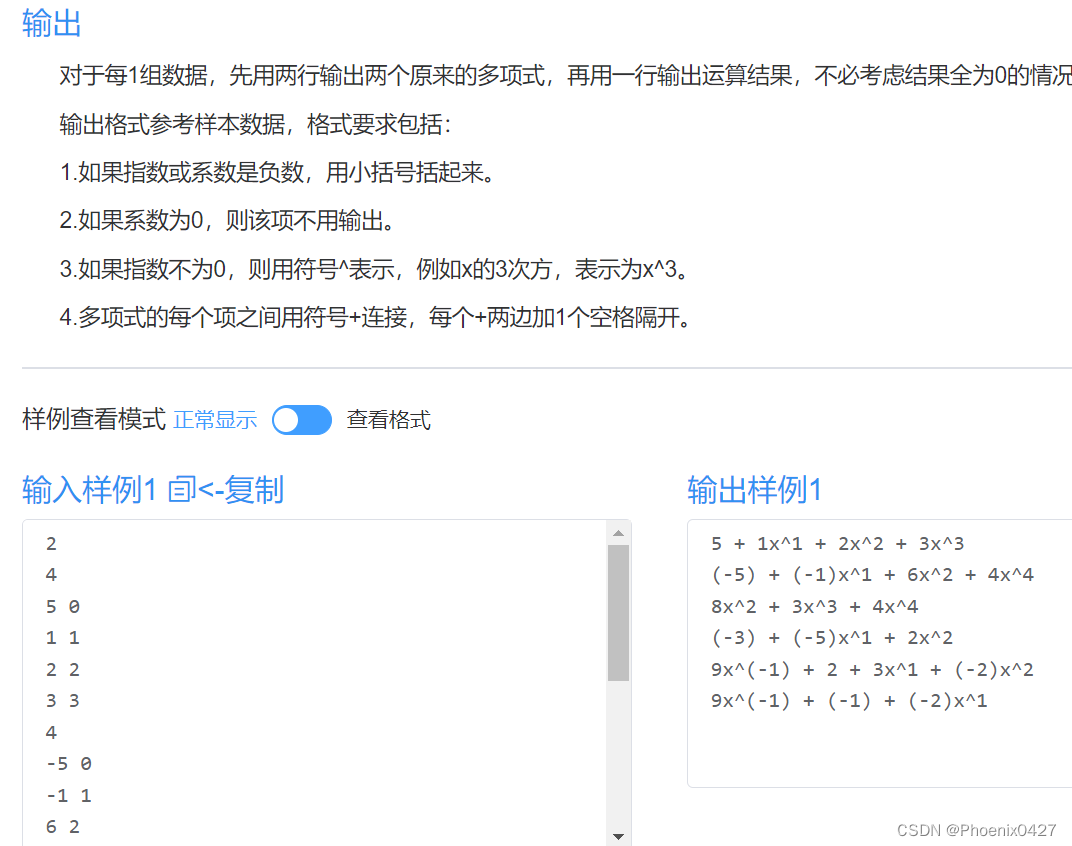
#include <iostream>
#include <stdlib.h>
using namespace std;
int poly1(int a)
{
if (a < 0)
{
cout << '(' << a << ')';
return 1;
}
else if (a > 0)
{
cout << a;
return 1;
}
else
return 0;
}
int poly2(int a)
{
if (a < 0)
{
cout << "x^(" << a << ')';
return 1;
}
else if (a > 0)
{
cout << "x^" << a;
return 1;
}
else
return 0;
}
class Node
{
public:
int xishu;
int zhishu;
Node *next;
Node()
{
next = NULL;
xishu = 0;
zhishu = 0;
}
};
class linklist
{
public:
Node *head;
int length;
void *AddPoly(Node *La, Node *Lb);
linklist();
Node *CreateNode(int n);
void display();
};
linklist::linklist()
{
head = new Node();
length = 0;
}
void *linklist::AddPoly(Node *La, Node *Lb)
{
Node *pa, *pb, *p;
p = head=La;
pa = La->next;
pb = Lb->next;
while (pa != NULL && pb != NULL)
{
if (pa->zhishu < pb->zhishu)
{
p->next = pa;
p = pa;
pa = pa->next;
}
else if (pa->zhishu > pb->zhishu)
{
p->next = pb;
p = pb;
pb = pb->next;
}
else
{
int x = pa->xishu + pb->xishu;
if (x == 0)
{
Node *temp1, *temp2;
temp1 = pa;
pa = pa->next;
free(temp1);
temp2 = pb;
pb = pb->next;
free(temp2);
}
else
{
Node *temp;
p->next = pa;
pa->xishu = x;
p = pa;
pa = pa->next;
temp = pb;
pb = pb->next;
free(temp);
}
}
}
if (pa == NULL)
p->next = pb;
else
p->next = pa;
return p;
}
void linklist::display()
{
Node *p;
p = head->next;
int i, count = 0, j;
while (p)
{
if (p->xishu != 0)
count++;
p = p->next;
}
p = head->next;
for (i = 1, j = 1; i <= count; i++,j++)
{
if (poly1(p->xishu))
{
poly2(p->zhishu);
if (j != count)
{
cout << " + ";
}
}
p = p->next;
}
cout << endl;
}
Node *linklist::CreateNode(int n)
{
Node *tail = head, *s;
int i;
for (i = 0; i < n; i++)
{
s = new Node;
cin >> s->xishu >> s->zhishu;
tail->next = s;
tail = s;
}
s = NULL;
delete s;
length = length + n;
return head;
}
int main()
{
int t, n;
cin >> t;
while (t--)
{
cin >> n;
int i;
linklist p, q, r;
Node *La, *Lb;
La = p.CreateNode(n);
p.display();
cin >> n;
Lb = q.CreateNode(n);
q.display();
r.AddPoly(La, Lb);
r.display();
}
}
【id:77】【5分】F. DS链表—学生宿舍管理(双向列表容器List)
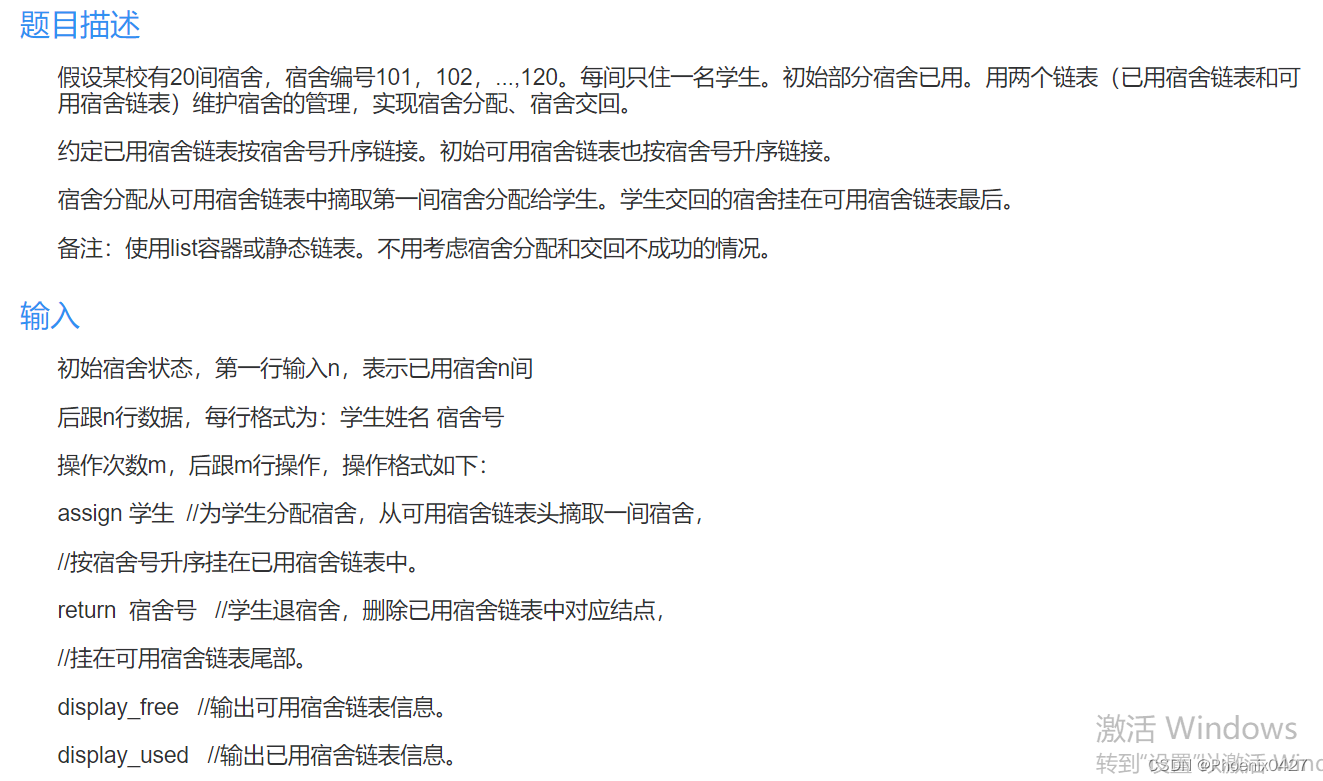
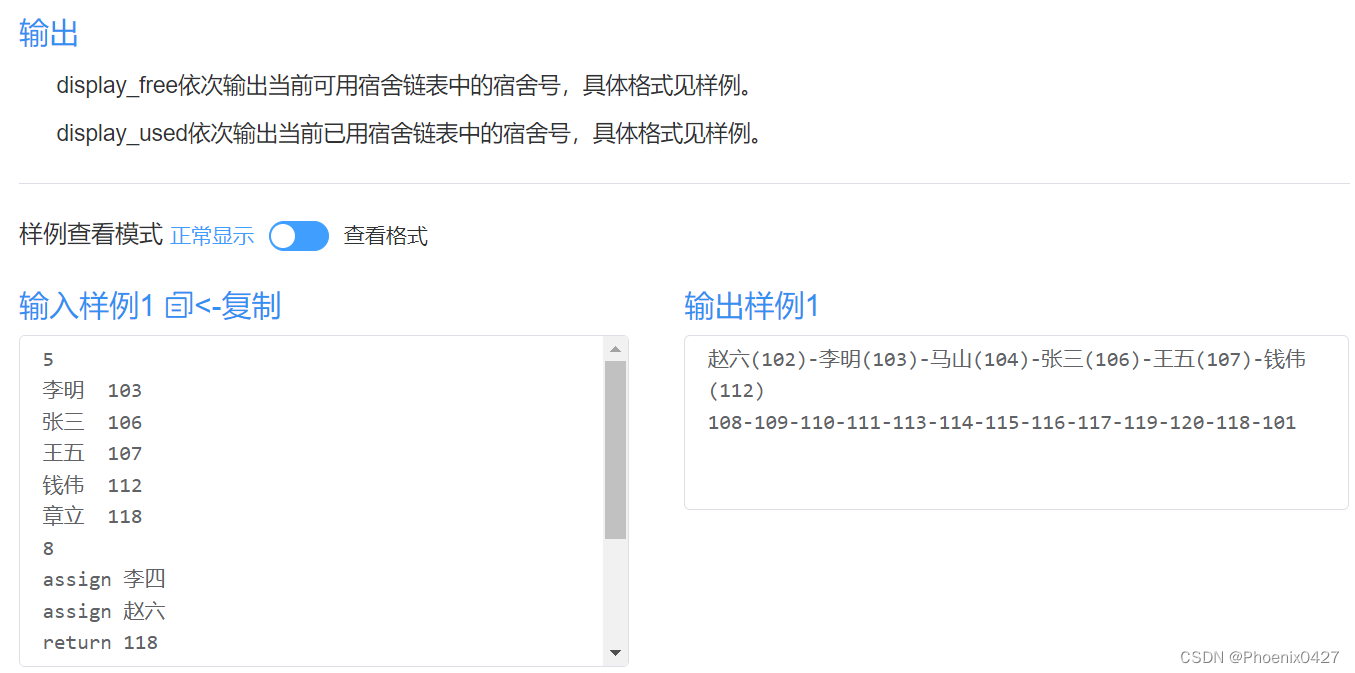
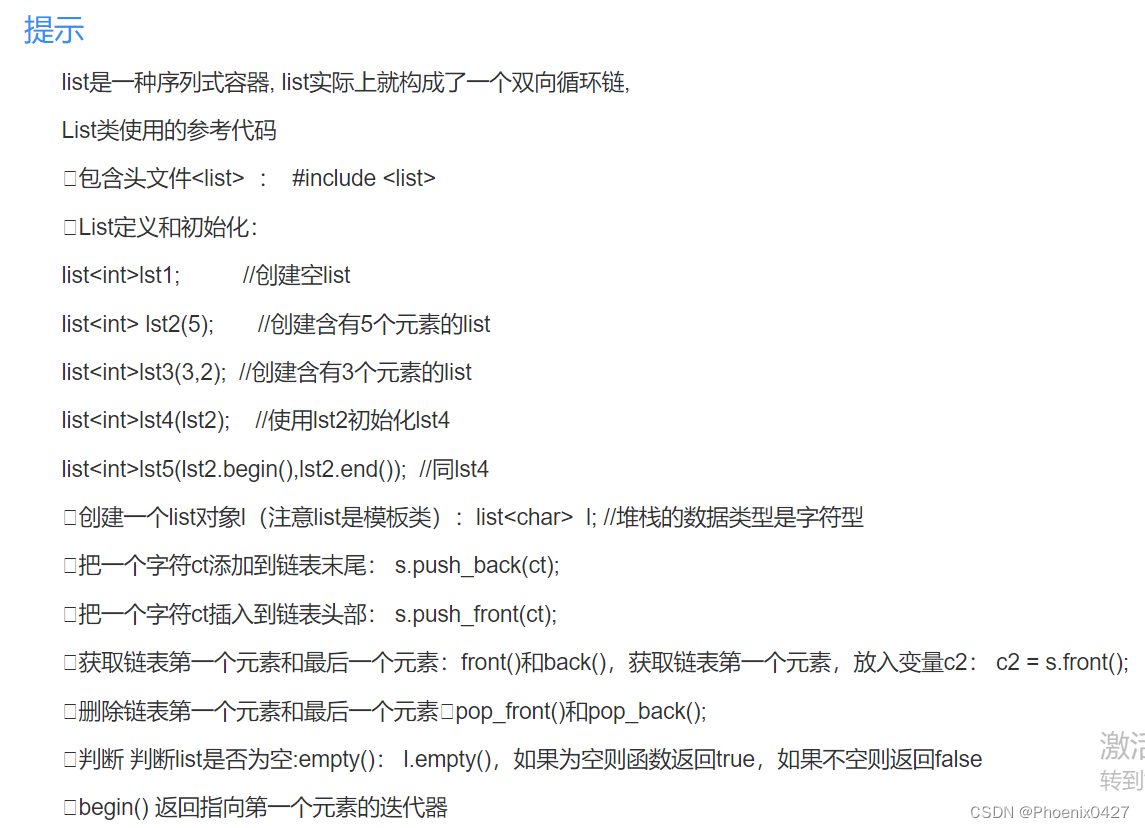
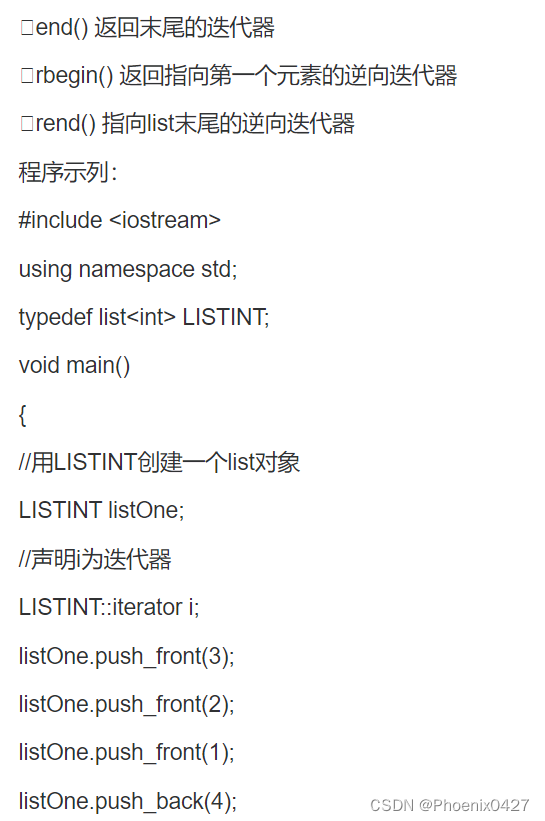
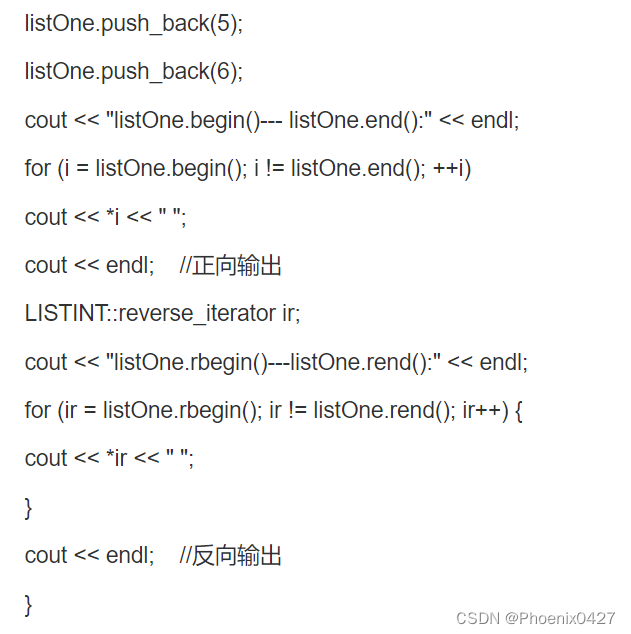
#include <iostream>
#include <list>
using namespace std;
struct Room
{
string name;
int stu_num;
};
bool operator< (const Room& r1, const Room& r2)
{
if (r1.stu_num < r2.stu_num)
{
return 1;
}
return 0;
}
typedef list<Room> listint;
int main()
{
listint used_room;
listint empty_room;
int t;
cin >> t;
int room[100], no_room[20] = { 0 };
for (int i = 0; i < t; ++i)
{
string name;
int stu_num;
cin >> name >> stu_num;
room[i] = stu_num;
used_room.push_back({ name,stu_num });
}
for (int i = 101; i <= 120; ++i)
{
int flag = 0;
for (int j = 0; j < t; ++j)
{
if (room[j] == i)
flag = 1;
}
if (flag == 0)
empty_room.push_back({ "",i });
}
int n;
cin >> n;
while (n--)
{
string control;
cin >> control;
if (control == "assign")
{
string name;
cin >> name;
int roomnum = empty_room.front().stu_num;
empty_room.pop_front();
used_room.push_front({ name,roomnum });
}
else if (control == "return")
{
int num; cin >> num;
for (auto it = used_room.begin(); it != used_room.end(); it++)
{
if (it->stu_num == num)
{
used_room.erase(it);
break;
}
}
empty_room.push_back({ "",num });
}
else if (control == "display_used")
{
listint::iterator it;
used_room.sort();
for (it = used_room.begin(); it != used_room.end(); it++)
{
if (it != used_room.begin())cout << "-";
cout << it->name << "(" << it->stu_num << ")";
}
cout << endl;
}
else if (control == "display_free")
{
listint::iterator it;
for (it = empty_room.begin(); it != empty_room.end(); it++)
{
if (it != empty_room.begin())cout << "-";
cout << it->stu_num;
}
cout << endl;
}
}
}