【id:129】【30分】A. DS堆栈--逆序输出(STL栈使用)
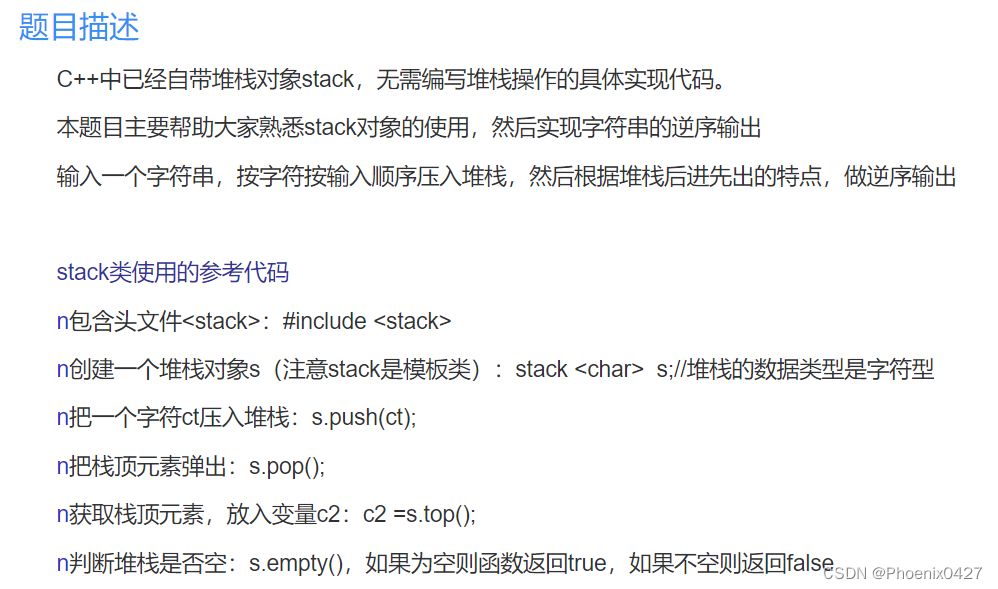
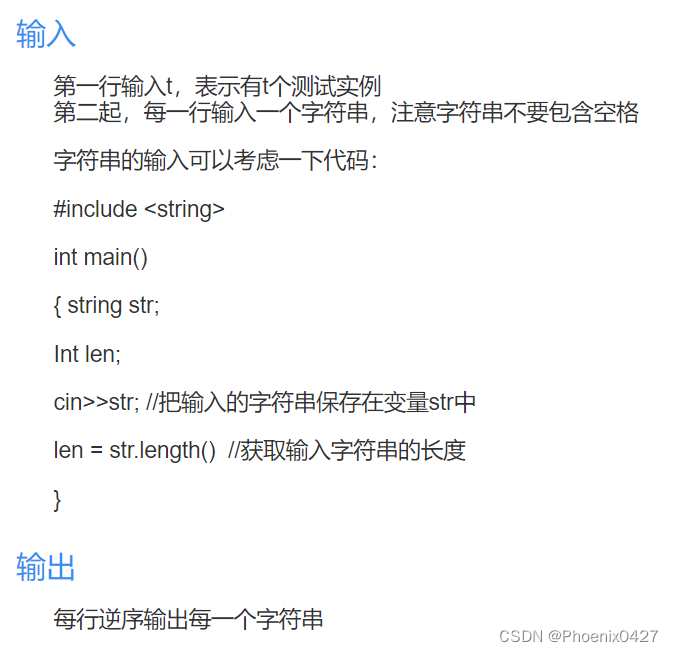
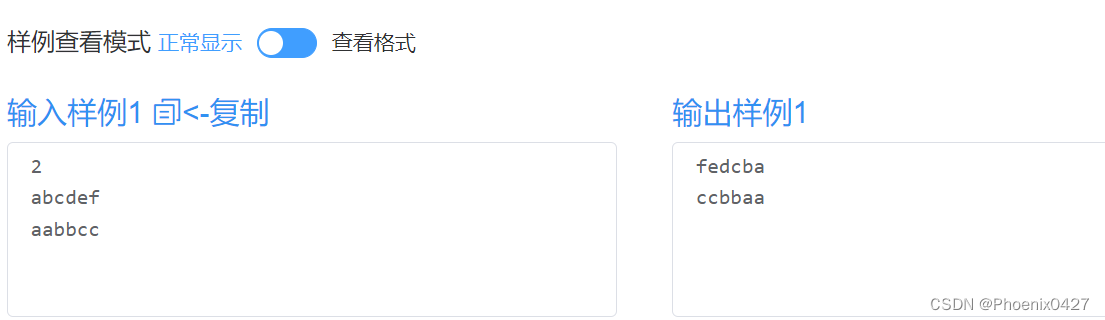
#include <stack>
#include <iostream>
#include <string>
using namespace std;
int main()
{
stack<char> mystack;
int t;
cin >> t;
char ct;
string Str;
for (int i = 0; i < t; i++)
{
cin >> Str;
for (int j = 0; j < Str.length();j++)
{
mystack.push(Str[j]);
}
while (!mystack.empty())
{
ct = mystack.top();
mystack.pop();
cout << ct;
}
cout << endl;
}
return 0;
}
【id:127】【30分】B. DS堆栈--行编辑
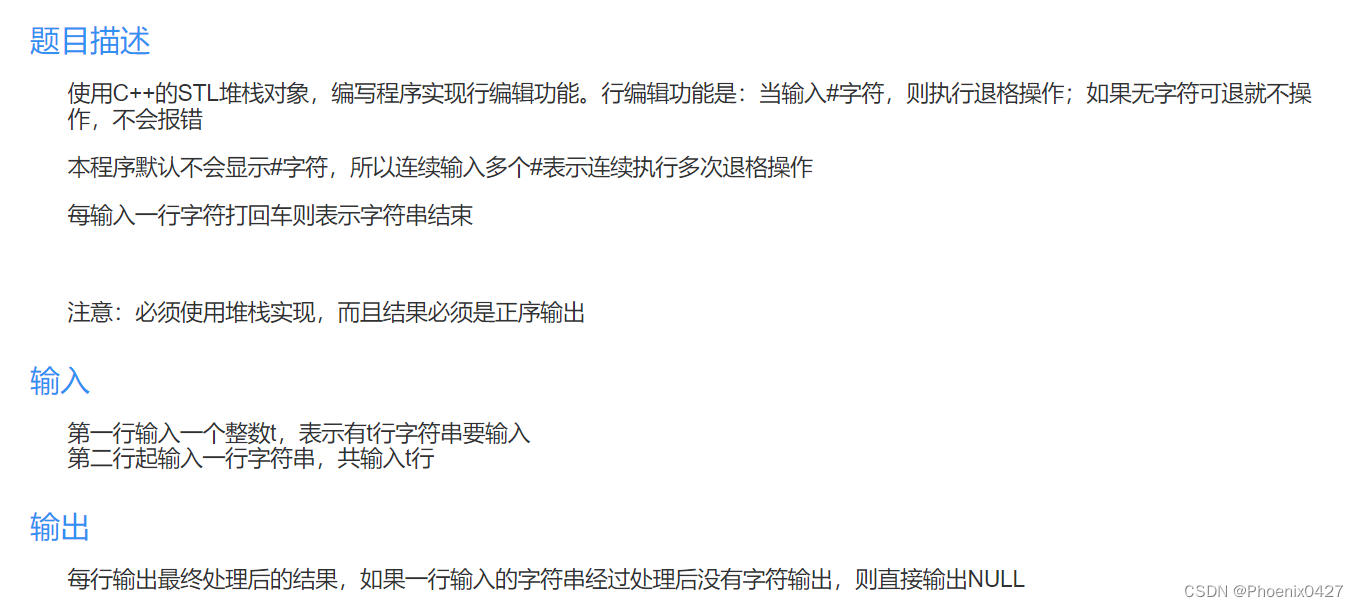
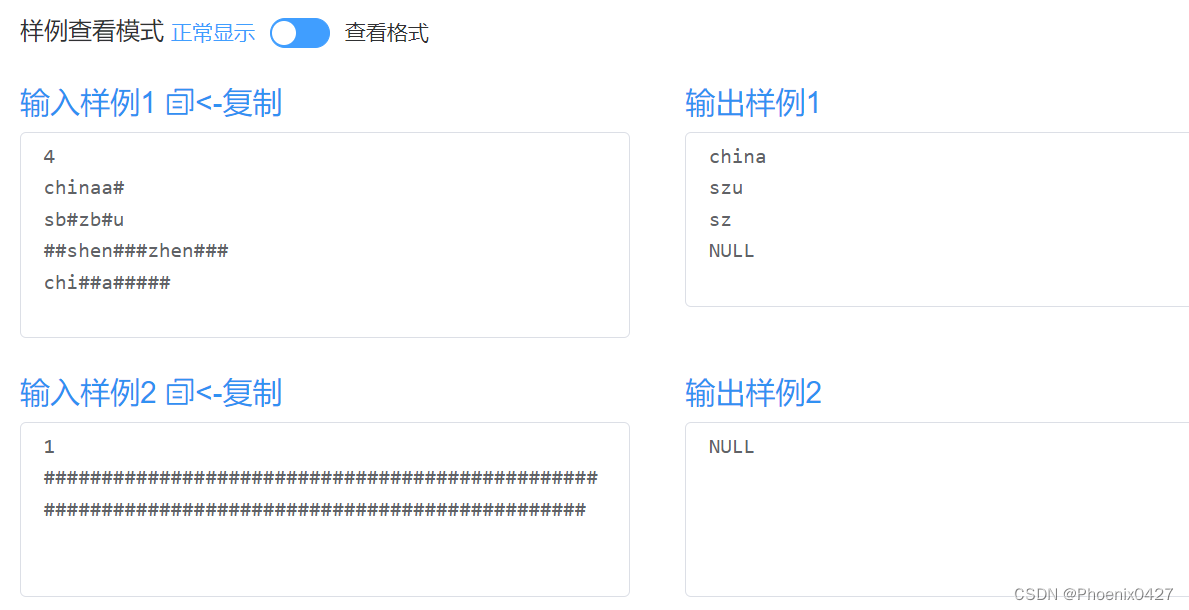
#include <stack>
#include <iostream>
using namespace std;
int main()
{
int t;
cin >> t;
while (t--)
{
stack<char> mystack;
string Str;
cin >> Str;
for (int i = 0; i < Str.length();i++)
{
if (Str[i] == '#' && !mystack.empty())
{
mystack.pop();
}
else if (Str[i] != '#')
{
mystack.push(Str[i]);
}
}
if (mystack.empty())
{
cout << "NULL";
}
else
{
stack<char> str1;
while (!mystack.empty())
{
str1.push(mystack.top());
mystack.pop();
}
while (!str1.empty())
{
cout << str1.top();
str1.pop();
}
}
cout << endl;
}
return 0;
}
【id:126】【15分】C. DS堆栈--括号匹配
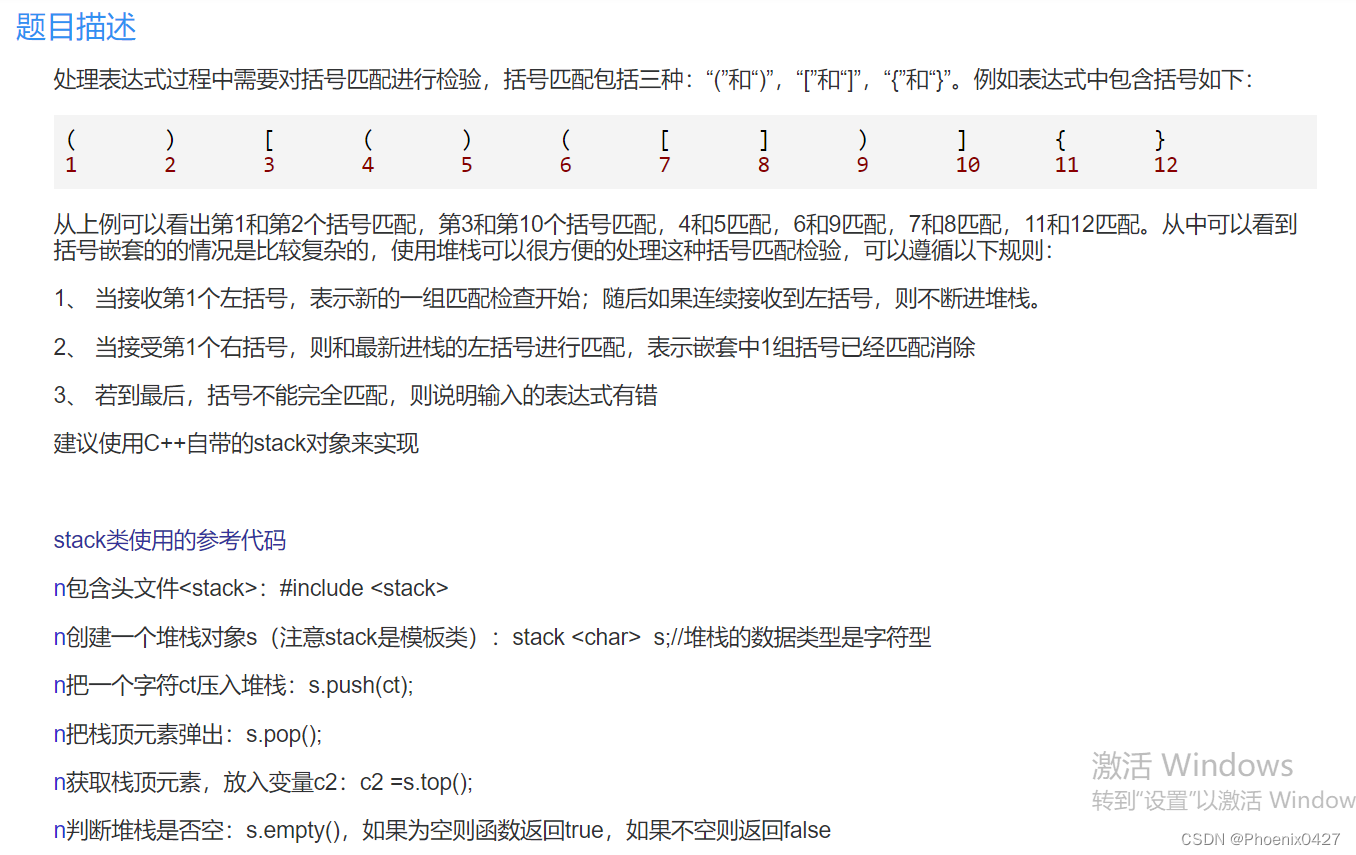
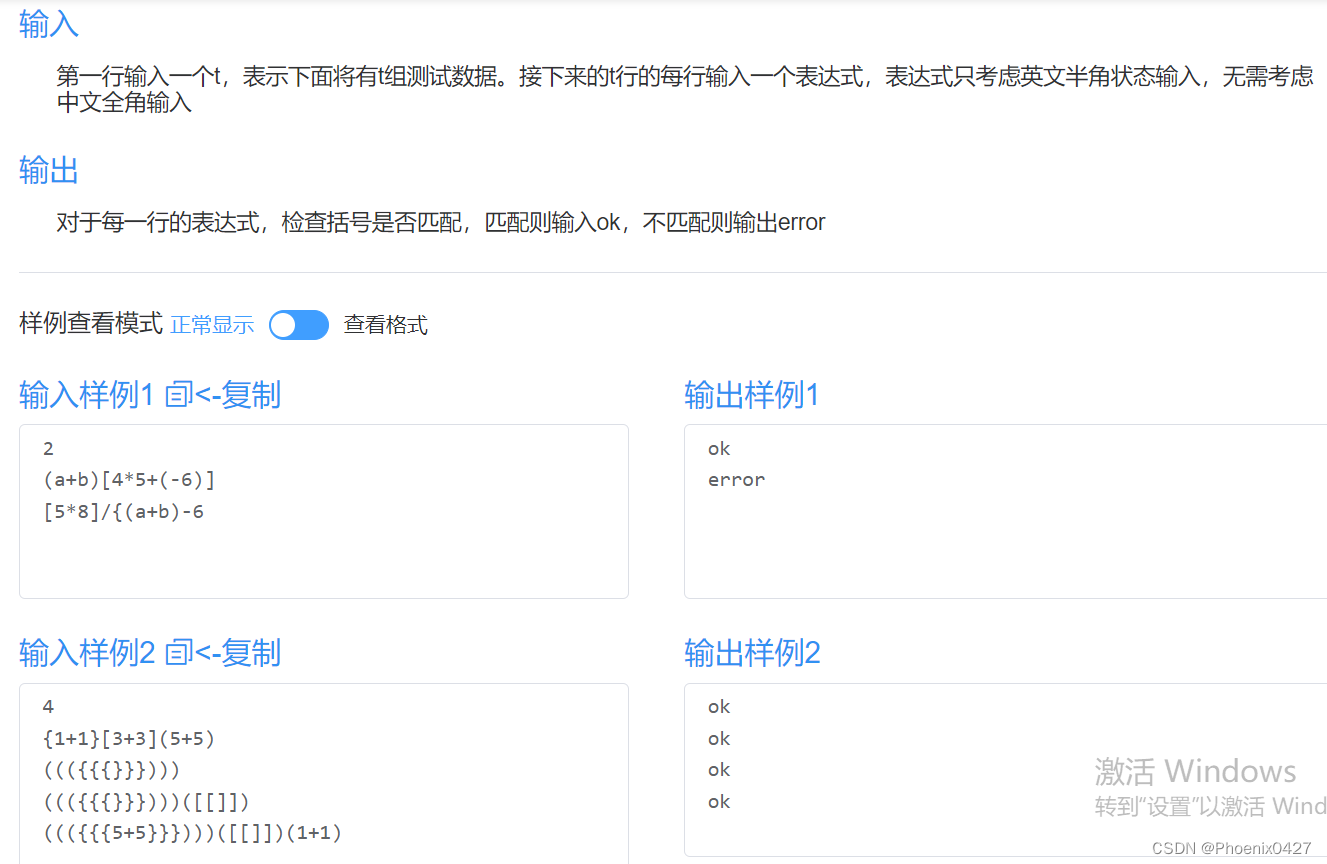
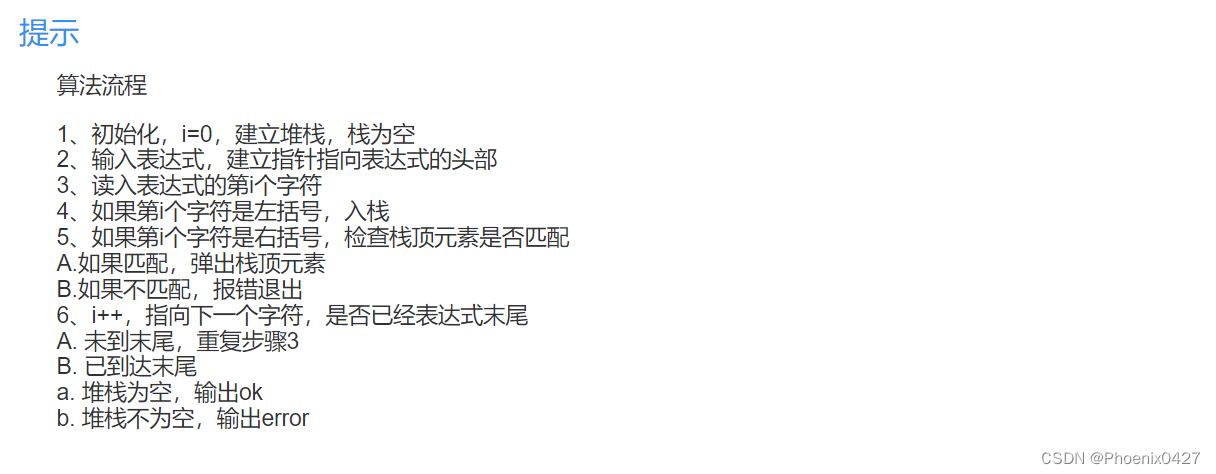
#include<iostream>
#include<string>
#include<stack>
using namespace std;
int main()
{
int t;
cin >> t;
string Str;
while (t--)
{
stack<char>mystack;
stack<char>sta;
cin >> Str;
int i = 0;
int flag = 1, Flag = 1;
for (int j = 0; j < Str.length(); j++)
{
if (Str[j] == '(' || Str[j] == '{' || Str[j] == '[' ||
Str[j] == ']' || Str[j] == '}' || Str[j] == ')')
{
Flag = 0;
break;
}
}
while (i < Str.length())
{
if ((Str[i] == '(') || (Str[i] == '[') || (Str[i] == '{'))
{
mystack.push(Str[i]);
}
if (Str[i] == ']')
{
if (!mystack.empty())
{
char c1 = mystack.top();
if (c1 == '[')
{
flag = 1;
mystack.pop();
}
else
{
flag = 0;
break;
}
}
else
{
flag = 0;
break;
}
}
if (Str[i] == '}')
{
if (!mystack.empty())
{
char c2 = mystack.top();
if (c2 == '{')
{
flag = 1;
mystack.pop();
}
else
{
flag = 0;
break;
}
}
else
{
flag = 0;
break;
}
}
if (Str[i] == ')')
{
if (!mystack.empty())
{
char c3 = mystack.top();
if (c3 == '(')
{
flag = 1;
mystack.pop();
}
else
{
flag = 0;
break;
}
}
else
{
flag = 0;
break;
}
}
i++;
}
if (!mystack.empty() || flag == 0)
{
cout << "error" << endl;
}
else if (mystack.empty() || Flag == 0)
{
cout << "ok" << endl;
}
}
return 0;
}
【id:128】【15分】D. DS堆栈--迷宫求解
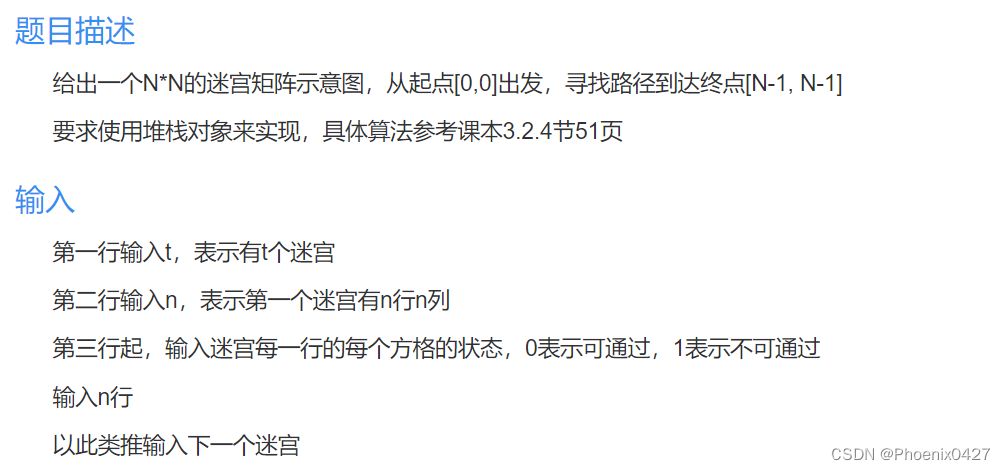
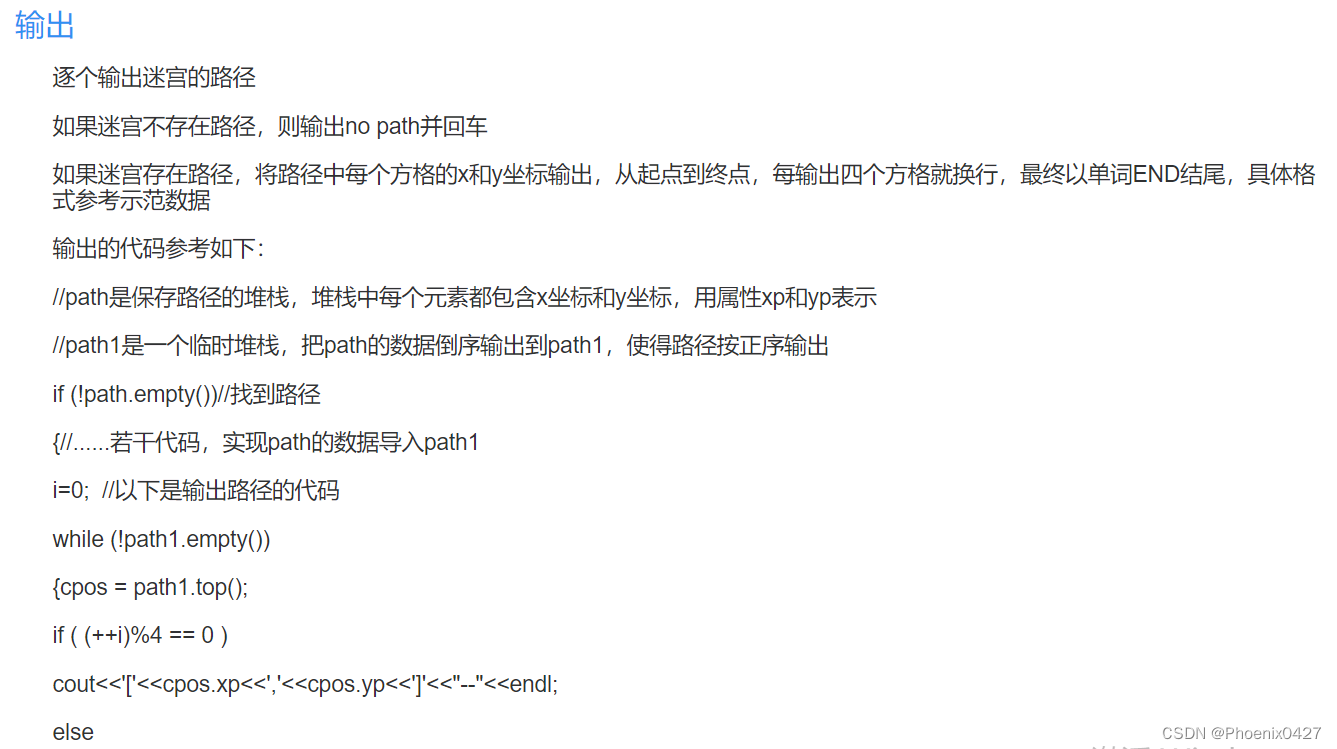
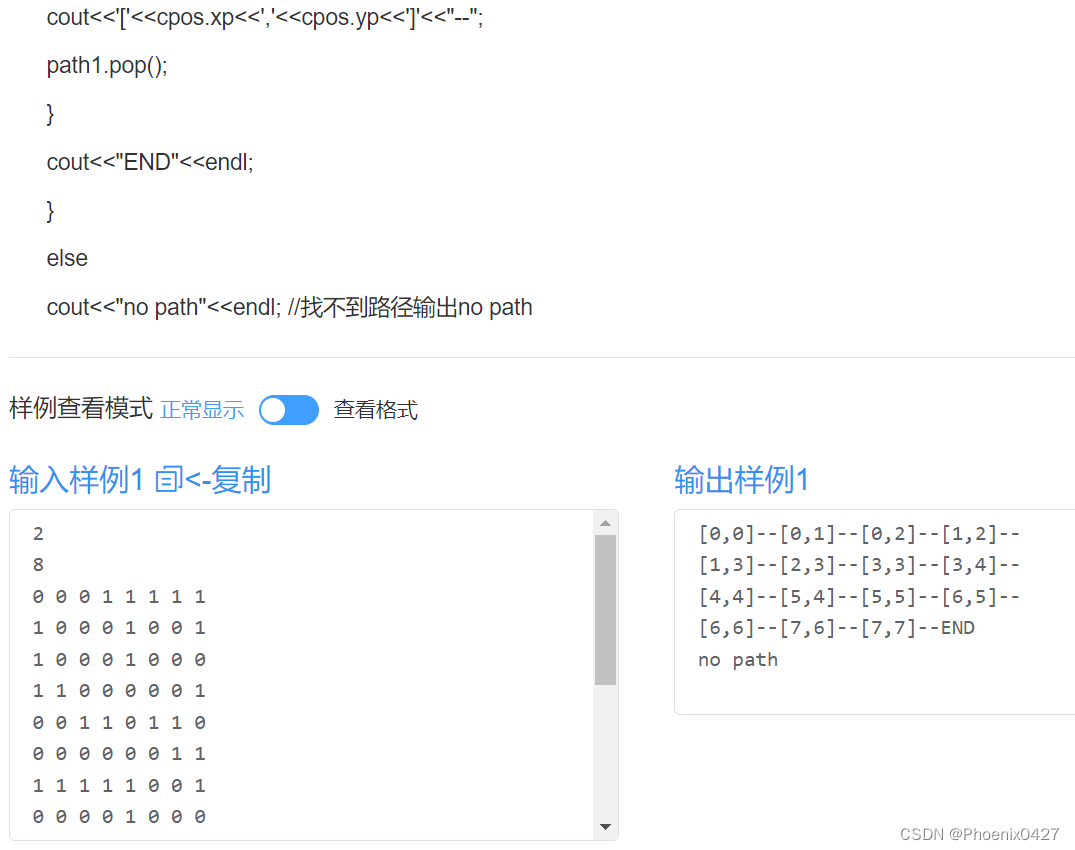
#include <iostream>
#include <stack>
#include <string>
using namespace std;
struct zuobiao
{
int x, y;
};
int main() {
int t, n;
cin >> t;
while (t--)
{
stack<zuobiao> path;
cin >> n;
int** migong = new int* [n];
for (int i = 0; i < n; i++)
{
migong[i] = new int[n];
}
for (int i = 0; i < n; i++)
{
for (int j = 0; j < n; j++)
{
cin >> migong[i][j];
}
}
path.push({ 0,0 });
migong[0][0] = 1;
int i = 0, j = 0;
while (1)
{
if (j + 1 < n && migong[i][j + 1] == 0)
{ //向右走
migong[i][j + 1] = 1;
path.push({ i,++j });
}
else if (i + 1 < n && migong[i + 1][j] == 0)
{ //向下走
migong[i + 1][j] = 1;
path.push({ ++i,j });
}
else if (j - 1 >= 0 && migong[i][j - 1] == 0)
{ //向左走
migong[i][j - 1] = 1;
path.push({ i,--j });
}
else if (i - 1 >= 0 && migong[i - 1][j] == 0)
{ //向上走
migong[i - 1][j] = 1;
path.push({ --i,j });
}
else
{
path.pop();
if (!path.empty())
{ //回到原点时,path为空,防止越界
i = path.top().x;
j = path.top().y;
}
}
if (path.empty() || (i == n - 1 && j == n - 1))
{ //判断是否到达终点或返回起点
break;
}
}
if (path.empty())
{
cout << "no path" << endl;
}
else
{
stack<zuobiao> path1;
while (!path.empty())
{
path1.push(path.top());
path.pop();
}
i = 0;
while (!path1.empty())
{
if ((++i) % 4 == 0) //换行
{
cout << '[' << path1.top().x << ',' << path1.top().y << ']' << "--" << endl;
}
else
{
cout << '[' << path1.top().x << ',' << path1.top().y << ']' << "--";
}
path1.pop();
}
cout << "END" << endl;
}
for (int i = 0; i < n; i++)
delete[]migong[i];
delete[]migong;
}
}
【id:125】【10分】E. DS堆栈--表达式计算
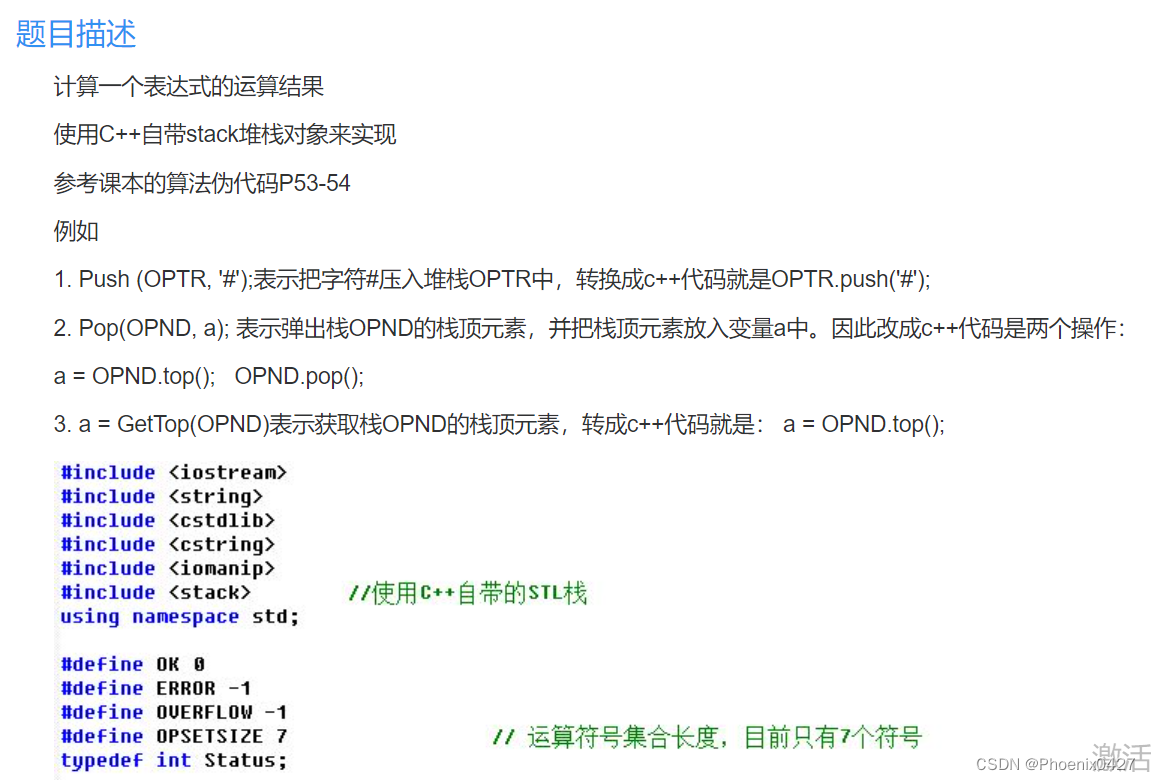
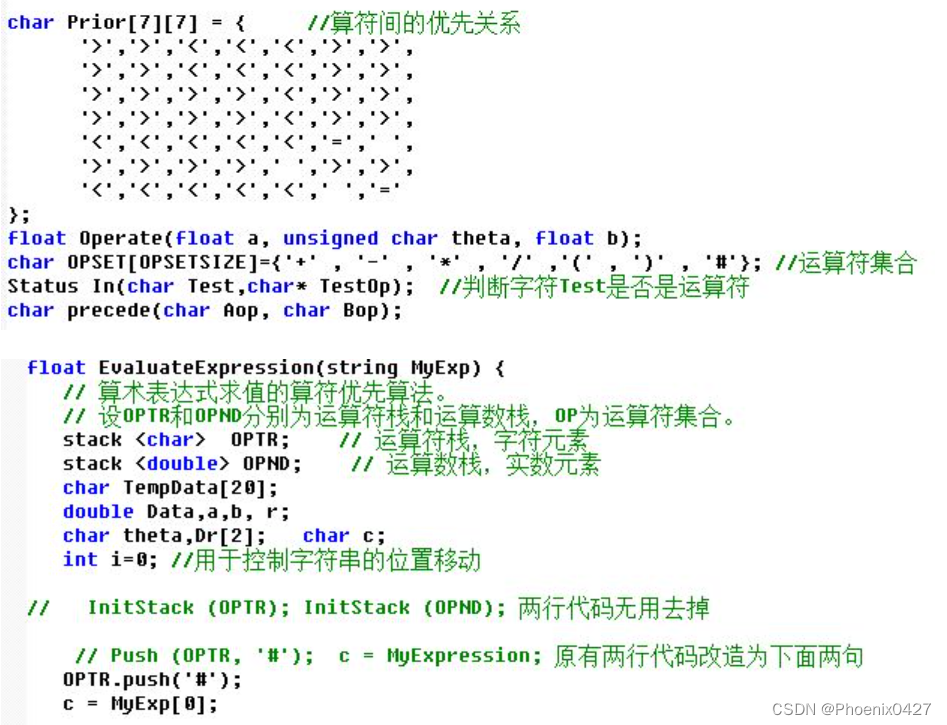
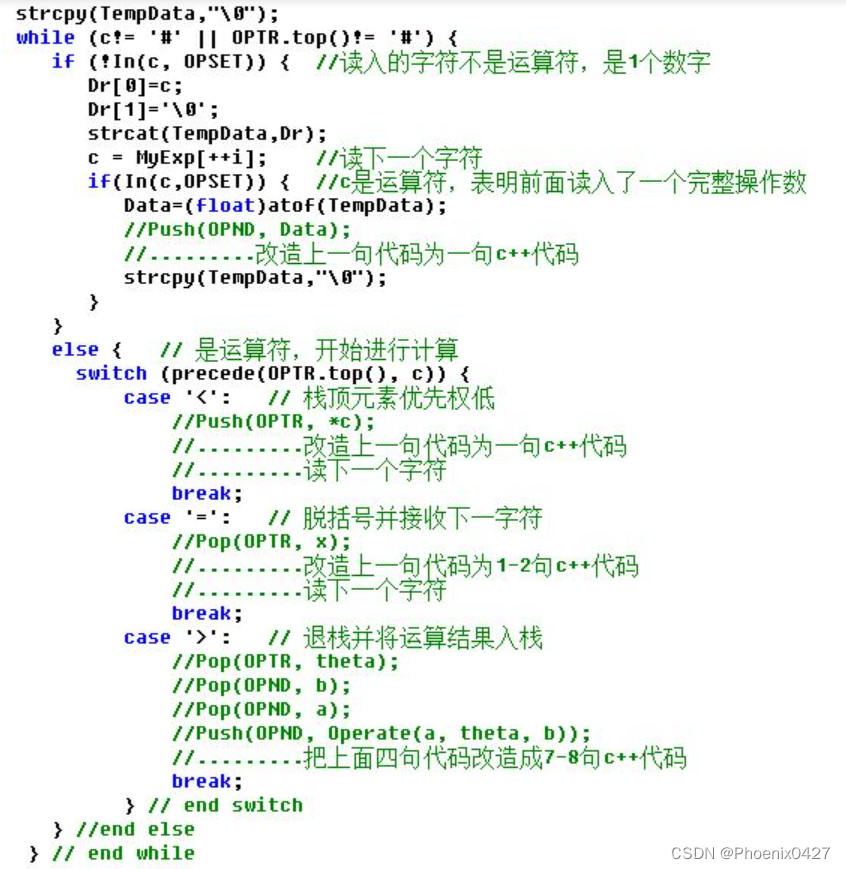
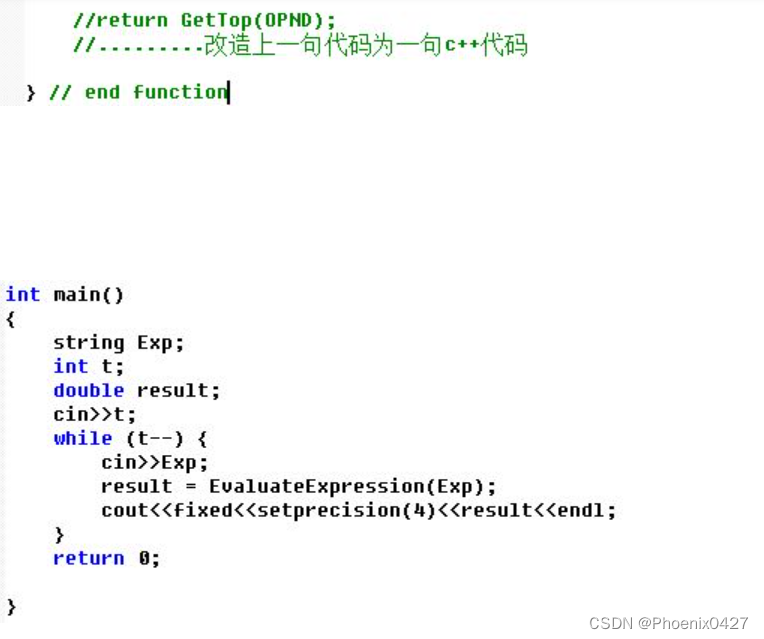
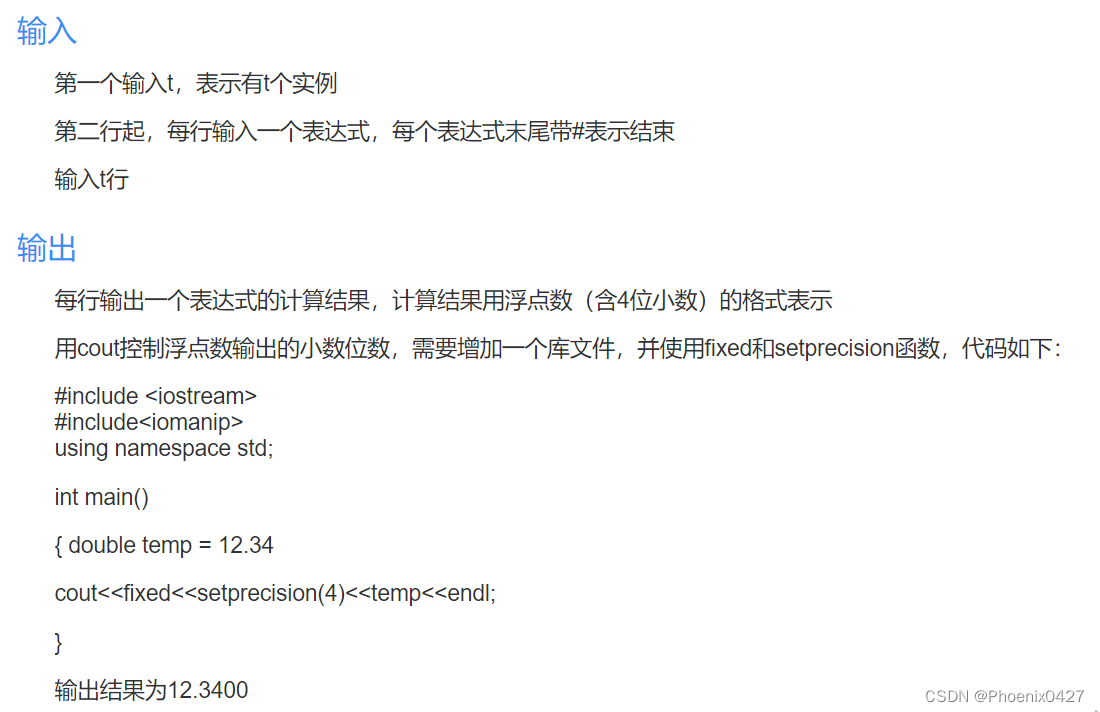
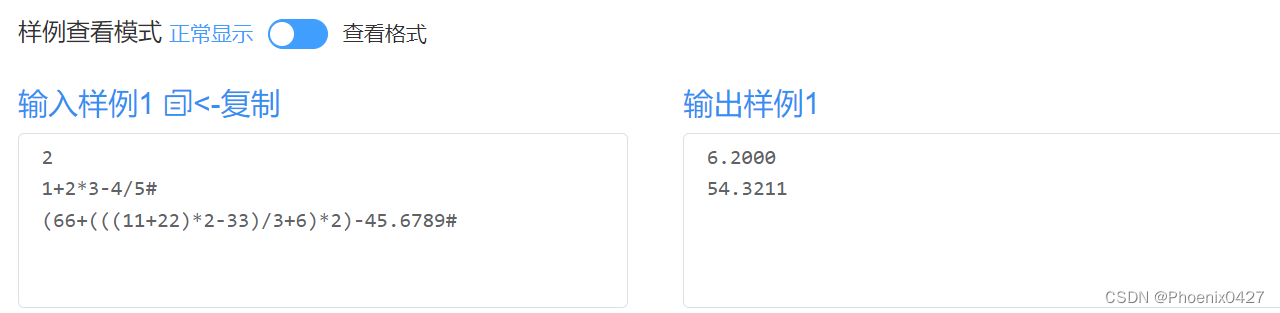
#include <iostream>
#include <iomanip>
#include <stack>
#include <cstring>
using namespace std;
char prior[7][7] = { '>','>','<','<','<','>','>','>','>','<','<','<','>','>','>','>','>','>','<','>','>','>','>','>','>','<','>','>','<','<','<','<','<','=',' ','>','>','>','>',' ','>','>','<','<','<','<','<',' ','=' };
float Operate(float a, unsigned char theta, float b)
{
if ('*' == theta)
return a * b;
else if ('/' == theta)
return a / b;
else if ('+' == theta)
return a + b;
else if ('-' == theta)
return a - b;
else
return -1;
}
char set[7] = { '+','-','*','/','(',')','#' };
int inside(char c, char* p)
{
int i;
for (i = 0; i < 7; i++)
{
if (c == p[i])
return 1;
}
return 0;
}
char priority(char A, char B)
{
int i, j;
for (i = 0; i < 7; i++)
{
if (set[i] == A)
{
for (j = 0; j < 7; j++)
{
if (set[j] == B)
return prior[i][j];
}
}
}
return -1;
}
float EE(string MyExp)
{
stack<char> OPTR;
stack<double> OPND;
char temp[20];
double data, a, b;
char theta, arr[2];
char c;
int i = 0;
OPTR.push('#');
c = MyExp[0];
strcpy(temp, "\0");
while (c != '#' || OPTR.top() != '#')
{
if (!inside(c, set))
{
arr[0] = c;
arr[1] = '\0';
strcat(temp, arr);
c = MyExp[++i];
if (inside(c, set))
{
data = (float)atof(temp);
OPND.push(data);
strcpy(temp, "\0");
}
}
else
{
switch (priority(OPTR.top(), c))
{
case '<':
OPTR.push(c);
c = MyExp[++i];
break;
case '=':
OPTR.pop();
c = MyExp[++i];
break;
case '>':
theta = OPTR.top();
OPTR.pop();
b = OPND.top();
OPND.pop();
a = OPND.top();
OPND.pop();
OPND.push(Operate(a, theta, b));
break;
}
}
}
return OPND.top();
}
int main()
{
string Exp;
int t;
double result;
cin >> t;
while (t--)
{
cin >> Exp;
result = EE(Exp);
cout << fixed << setprecision(4) << result << endl;
}
return 0;
}