1. 注意点 合计价格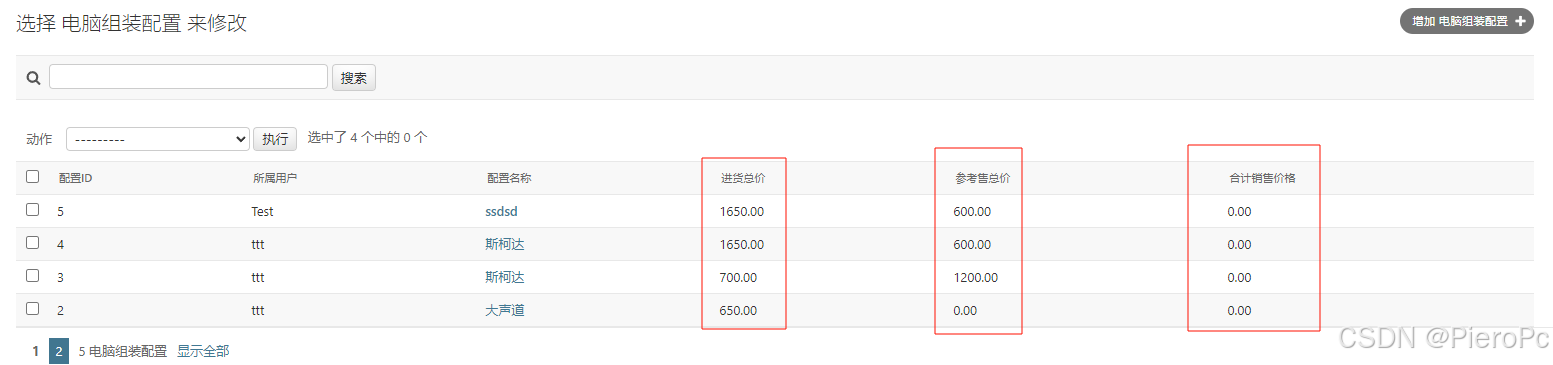
@admin.register(ComputerConfiguration)
class ComputerConfigurationAdmin(admin.ModelAdmin):
inlines = [ConfigurationComponentInline]
list_display = ('config_id', 'user_id', 'config_name', 'total_price', 'total_jh_price', 'total_selling_price')
list_display_links = ('config_name',)
def total_price(self, obj):
total = 0
config_components = obj.configurationcomponent_set.all()
for config_component in config_components:
component = config_component.component_id
quantity = config_component.quantity
total += component.price * quantity
return total
total_price.short_description = '进货总价'
def total_jh_price(self, obj):
total = 0
config_components = obj.configurationcomponent_set.all()
for config_component in config_components:
component = config_component.component_id
quantity = config_component.quantity
total += component.sell_price * quantity
return total
total_jh_price.short_description = '参考售总价'
def total_selling_price(self, obj):
components = ConfigurationComponent.objects.filter(config_id=obj.config_id)
total = sum([component.unit_price * component.quantity for component in components])
return total
total_selling_price.short_description = '合计销售价格'
search_fields = ('config_name',)
list_per_page = 4
2. 注意点 内联Inlime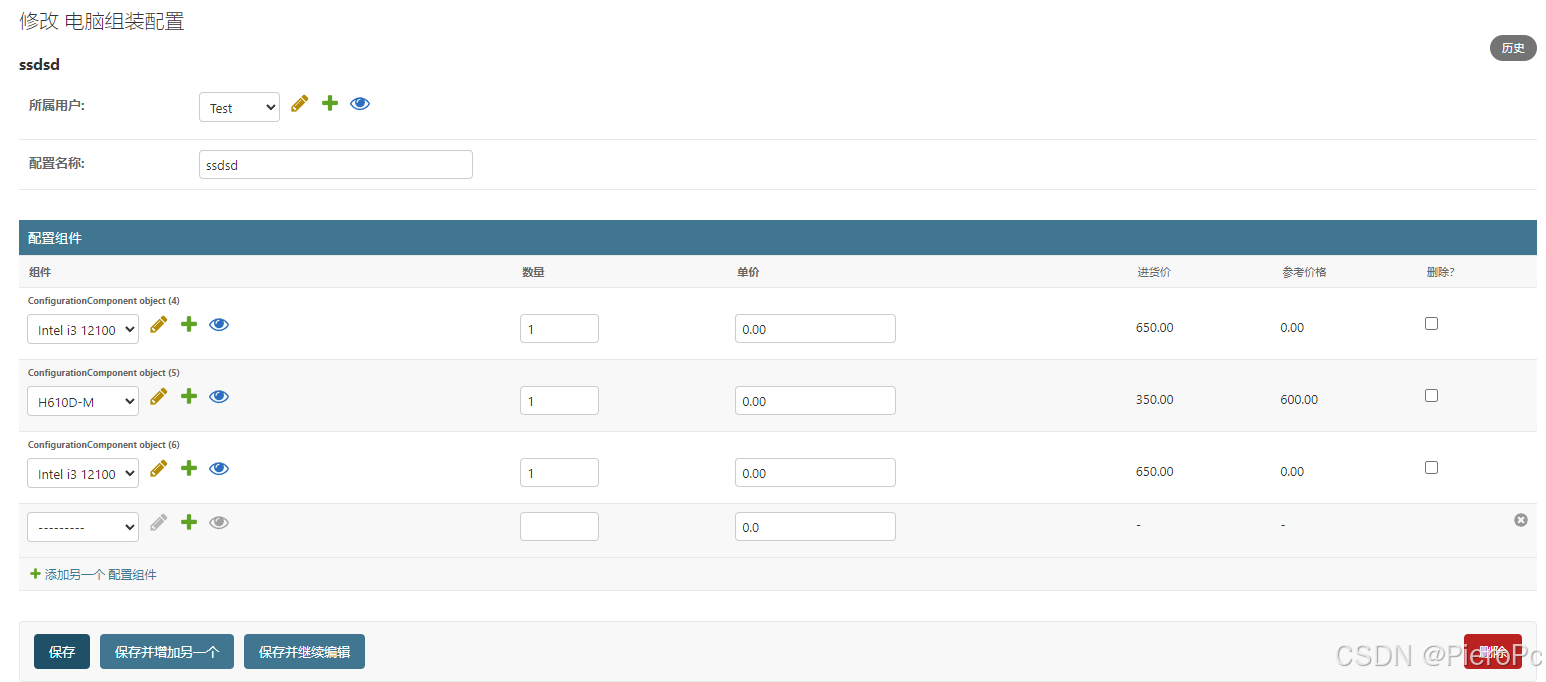
class ConfigurationComponentInline(admin.TabularInline):
model = ConfigurationComponent
extra = 1
# fields = ( 'quantity')
fields = ('component_id', 'quantity','unit_price' ,'show_component_price', 'show_sell_price')
readonly_fields = ('show_component_price', 'show_sell_price')
def show_component_price(self, obj):
component = obj.component_id
return component.price
show_component_price.short_description = '进货价'
def show_sell_price(self, obj):
component = obj.component_id
return component.sell_price
show_sell_price.short_description = '参考价格'
Models.py
from django.db import models
class ComponentCategory(models.Model):
"""
组件分类模型类,用于存储电脑组件的分类信息。
例如:CPU、主板等分类。
"""
category_id = models.AutoField(primary_key=True, verbose_name='分类ID')
category_name = models.CharField(max_length=50, verbose_name='组件分类名称')
category_description = models.TextField(verbose_name='分类描述', blank=True, null=True)
def __str__(self):
return self.category_name
class Meta:
verbose_name = '组件分类'
verbose_name_plural = '组件分类'
class Component(models.Model):
"""
组件模型类,用于存储具体的电脑组件信息。
例如:Intel Core i9 - 13900K等组件及其相关信息。
"""
component_id = models.AutoField(primary_key=True, verbose_name='组件ID')
category_id = models.ForeignKey(ComponentCategory, on_delete=models.CASCADE, verbose_name='所属分类')
component_name = models.CharField(max_length=100, verbose_name='组件名称')
price = models.DecimalField(max_digits=10, decimal_places=2, verbose_name='价格')
sell_price = models.DecimalField(max_digits=10, decimal_places=2, verbose_name='参考价格', default=0.00)
description = models.TextField(verbose_name='描述')
def __str__(self):
return self.component_name
class Meta:
verbose_name = '组件'
verbose_name_plural = '组件'
class User(models.Model):
"""
用户模型类,用于存储用户相关信息。
如用户名、密码和邮箱等信息。
"""
user_id = models.AutoField(primary_key=True, verbose_name='用户ID')
username = models.CharField(max_length=50, verbose_name='用户名')
password = models.CharField(max_length=255, verbose_name='密码')
email = models.CharField(max_length=100, verbose_name='邮箱')
def __str__(self):
return self.username
class Meta:
verbose_name = '用户'
verbose_name_plural = '用户'
class ComputerConfiguration(models.Model):
"""
电脑组装配置模型类,用于存储用户创建的电脑组装配置信息。
每个配置属于一个用户并且有一个配置名称。
"""
config_id = models.AutoField(primary_key=True, verbose_name='配置ID')
user_id = models.ForeignKey(User, on_delete=models.CASCADE, verbose_name='所属用户')
config_name = models.CharField(max_length=100, verbose_name='配置名称')
def __str__(self):
return self.config_name
class Meta:
verbose_name = '电脑组装配置'
verbose_name_plural = '电脑组装配置'
class ConfigurationComponent(models.Model):
"""
配置组件关联模型类,用于存储电脑组装配置与组件之间的关联关系。
包括组件的数量等信息。
"""
config_component_id = models.AutoField(primary_key=True, verbose_name='配置组件ID')
config_id = models.ForeignKey(ComputerConfiguration, on_delete=models.CASCADE, verbose_name='所属配置')
component_id = models.ForeignKey(Component, on_delete=models.CASCADE, verbose_name='组件')
quantity = models.IntegerField(verbose_name='数量')
unit_price = models.DecimalField(max_digits=10, decimal_places=2, verbose_name='单价', default=0.00)
class Meta:
verbose_name = '配置组件'
verbose_name_plural = '配置组件'
admin.py
from django.contrib import admin
from.models import ComponentCategory, Component, User, ComputerConfiguration, ConfigurationComponent
class ConfigurationComponentInline(admin.TabularInline):
model = ConfigurationComponent
extra = 1
# fields = ( 'quantity')
fields = ('component_id', 'quantity','unit_price' ,'show_component_price', 'show_sell_price')
readonly_fields = ('show_component_price', 'show_sell_price')
def show_component_price(self, obj):
component = obj.component_id
return component.price
show_component_price.short_description = '进货价'
def show_sell_price(self, obj):
component = obj.component_id
return component.sell_price
show_sell_price.short_description = '参考价格'
@admin.register(ComputerConfiguration)
class ComputerConfigurationAdmin(admin.ModelAdmin):
inlines = [ConfigurationComponentInline]
list_display = ('config_id', 'user_id', 'config_name', 'total_price', 'total_jh_price', 'total_selling_price')
list_display_links = ('config_name',)
def total_price(self, obj):
total = 0
config_components = obj.configurationcomponent_set.all()
for config_component in config_components:
component = config_component.component_id
quantity = config_component.quantity
total += component.price * quantity
return total
total_price.short_description = '进货总价'
def total_jh_price(self, obj):
total = 0
config_components = obj.configurationcomponent_set.all()
for config_component in config_components:
component = config_component.component_id
quantity = config_component.quantity
total += component.sell_price * quantity
return total
total_jh_price.short_description = '参考售总价'
def total_selling_price(self, obj):
components = ConfigurationComponent.objects.filter(config_id=obj.config_id)
total = sum([component.unit_price * component.quantity for component in components])
return total
total_selling_price.short_description = '合计销售价格'
search_fields = ('config_name',)
list_per_page = 4
@admin.register(ComponentCategory)
class ComponentCategoryAdmin(admin.ModelAdmin):
list_display = ('category_id', 'category_name', 'category_description')
list_display_links = ('category_name',)
@admin.register(Component)
class ComponentAdmin(admin.ModelAdmin):
list_display = ('component_id', 'category_id', 'component_name', 'price', 'description')
list_display_links = ('component_name',)
@admin.register(User)
class UserAdmin(admin.ModelAdmin):
list_display = ('user_id', 'username', 'password', 'email')
list_display_links = ('username',)